Why Your Solutions Lack Proper Structure and Organization: A Comprehensive Guide
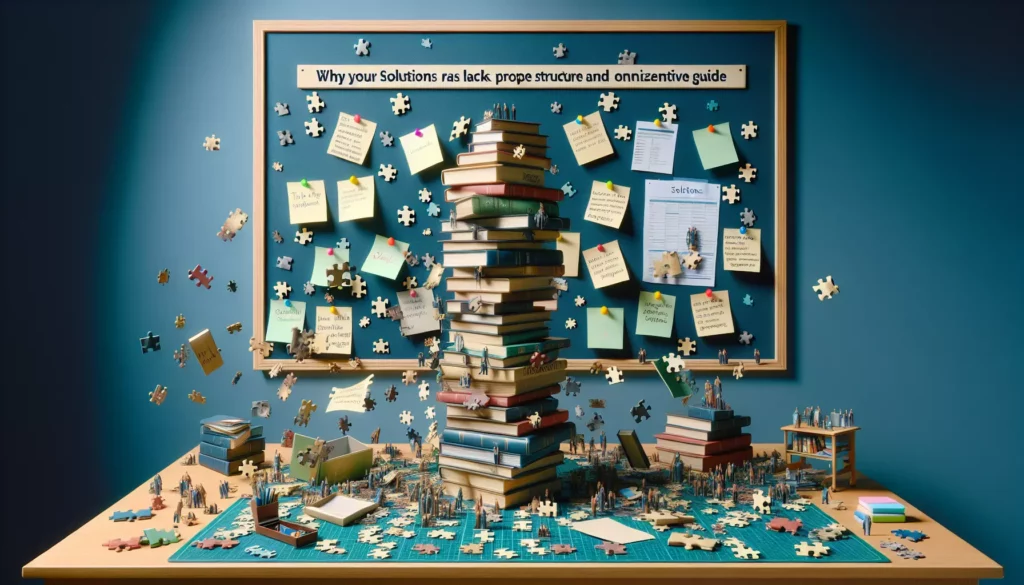
In the world of programming and algorithmic problem-solving, the difference between a working solution and an excellent solution often comes down to structure and organization. Many programmers, especially those new to the field, focus solely on making their code work without considering how well it’s structured or organized. This approach might solve the immediate problem but creates challenges in the long run.
At AlgoCademy, we’ve observed thousands of coding solutions from beginners to advanced programmers preparing for technical interviews at top tech companies. A common pattern emerges: even technically correct solutions frequently lack proper structure and organization, making them difficult to understand, maintain, and optimize.
This comprehensive guide explores why your solutions might lack proper structure and organization, the consequences of this approach, and practical strategies to transform your coding style into one that will impress both automated grading systems and human interviewers alike.
Table of Contents
- Understanding the Importance of Structure and Organization
- Common Structural and Organizational Issues in Code
- The Consequences of Poorly Structured Solutions
- Key Principles for Well-Structured Code
- Practical Techniques for Improving Code Structure
- Language-Specific Organization Patterns
- Structure and Organization in Technical Interviews
- Refactoring: Transforming Disorganized Code
- Tools and Resources for Better Code Structure
- Conclusion: The Path to Well-Structured Solutions
Understanding the Importance of Structure and Organization
Code is read far more often than it is written. This fundamental principle of software development highlights why structure and organization matter so much. When you write code, you’re not just communicating with the computer; you’re communicating with other developers (including your future self) who will need to understand, maintain, and possibly extend your work.
Well-structured code provides several critical benefits:
- Readability: Others can quickly understand what your code does and how it works
- Maintainability: Changes and updates can be made safely and efficiently
- Debuggability: Problems can be isolated and fixed more easily
- Scalability: The codebase can grow without becoming unmanageable
- Reusability: Components can be extracted and reused in other contexts
In an interview context, well-structured code demonstrates that you’re not just a programmer who can make things work but a software engineer who creates sustainable solutions. This distinction can be the difference between receiving an offer and being rejected.
Common Structural and Organizational Issues in Code
Before we can improve code structure, we need to identify common problems. Here are the issues we frequently see in solutions submitted to AlgoCademy:
1. Monolithic Functions
One of the most common issues is writing everything as a single, massive function. These functions often stretch for dozens or even hundreds of lines, handling multiple responsibilities within a single block of code.
Example of a monolithic function for finding prime numbers:
function findPrimes(max) {
const result = [];
for (let num = 2; num <= max; num++) {
let isPrime = true;
// Check if num is divisible by any number less than it
for (let i = 2; i < num; i++) {
if (num % i === 0) {
isPrime = false;
break;
}
}
// If prime, add to results and print
if (isPrime) {
result.push(num);
console.log(`Found prime: ${num}`);
}
}
// Calculate some statistics
let sum = 0;
let average = 0;
for (let i = 0; i < result.length; i++) {
sum += result[i];
}
average = sum / result.length;
console.log(`Found ${result.length} primes with average value ${average}`);
return result;
}
2. Inconsistent Naming Conventions
Variable and function names that don't follow a consistent pattern make code harder to follow. We often see a mix of camelCase, snake_case, and descriptive and non-descriptive names within the same solution.
3. Poor Indentation and Formatting
Code that isn't properly indented or formatted becomes difficult to parse visually. Nested control structures without proper indentation are particularly problematic.
4. Lack of Modularity
Solutions that don't break problems into smaller, manageable components tend to be harder to understand and maintain. This often manifests as duplicate code or missed opportunities for helper functions.
5. Insufficient Comments and Documentation
While excessive commenting can be a problem, the more common issue is a complete lack of comments explaining the "why" behind complex or non-obvious code sections.
6. Mixing Levels of Abstraction
Jumping between high-level operations and low-level implementation details within the same function creates cognitive load for readers.
7. Unclear Data Flow
Solutions where it's difficult to track how data moves through the program, often due to excessive use of global variables or complex parameter passing.
The Consequences of Poorly Structured Solutions
Submitting poorly structured solutions has consequences beyond just aesthetics:
In Learning Environments
- Limited progress in developing professional coding skills
- Formation of bad habits that are difficult to break later
- Difficulty in receiving helpful feedback from mentors
- Challenges when trying to revisit and understand your own solutions
In Technical Interviews
- Negative impression on interviewers, even if your solution is functionally correct
- Communication barriers when explaining your approach
- Difficulties when asked to modify or extend your solution
- Missed opportunities to demonstrate software engineering principles
In Professional Settings
- Increased time for code reviews and onboarding new team members
- Higher risk of introducing bugs during maintenance
- Technical debt that compounds over time
- Limitations on code reuse and scalability
Key Principles for Well-Structured Code
To improve the structure and organization of your solutions, focus on these fundamental principles:
1. Single Responsibility Principle
Each function or class should have one reason to change. This means focusing on a single task or responsibility rather than trying to do everything at once.
Instead of a monolithic function that finds primes, prints them, and calculates statistics, break it into separate functions:
function isPrime(num) {
if (num < 2) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
}
return true;
}
function findPrimes(max) {
const primes = [];
for (let num = 2; num <= max; num++) {
if (isPrime(num)) {
primes.push(num);
}
}
return primes;
}
function calculatePrimeStats(primes) {
const sum = primes.reduce((acc, curr) => acc + curr, 0);
const average = primes.length ? sum / primes.length : 0;
return { count: primes.length, sum, average };
}
// Usage
const primes = findPrimes(100);
const stats = calculatePrimeStats(primes);
console.log(`Found ${stats.count} primes with average value ${stats.average}`);
2. DRY (Don't Repeat Yourself)
Avoid duplicating code by extracting common patterns into reusable functions or classes. Repetition is a sign that abstraction is needed.
3. Consistent Naming Conventions
Choose a naming convention and stick with it throughout your solution. Names should be descriptive and reveal intent:
- Functions should typically be verbs or verb phrases:
calculateTotal()
,findLargestElement()
- Variables should be nouns or adjectives:
userCount
,isValid
- Boolean variables should suggest true/false questions:
isComplete
,hasPermission
4. Appropriate Abstraction Levels
Functions should operate at a consistent level of abstraction. High-level functions should call other functions rather than mixing high-level operations with low-level details.
5. Clear Control Flow
The path of execution through your code should be easy to follow. Avoid deeply nested conditionals and complex boolean logic when possible.
6. Meaningful Comments
Comments should explain why, not what. The code itself should be clear enough to understand what it's doing, while comments explain the reasoning behind non-obvious decisions.
Practical Techniques for Improving Code Structure
Let's explore specific techniques you can apply to improve the structure and organization of your solutions:
1. Function Decomposition
Break large functions into smaller, focused ones. A good rule of thumb is that functions should fit on a single screen (20-30 lines maximum) and do one thing well.
Consider this example of a binary search tree insertion:
// Before: Monolithic approach
function insertIntoTree(root, value) {
if (!root) {
return { value, left: null, right: null };
}
let current = root;
let parent = null;
let direction = null;
// Find where to insert
while (current) {
parent = current;
if (value < current.value) {
current = current.left;
direction = 'left';
} else {
current = current.right;
direction = 'right';
}
}
// Create new node
const newNode = { value, left: null, right: null };
// Insert the node
parent[direction] = newNode;
return root;
}
// After: Decomposed approach
function createNode(value) {
return { value, left: null, right: null };
}
function findInsertionPoint(root, value) {
let current = root;
let parent = null;
let direction = null;
while (current) {
parent = current;
if (value < current.value) {
current = current.left;
direction = 'left';
} else {
current = current.right;
direction = 'right';
}
}
return { parent, direction };
}
function insertIntoTree(root, value) {
if (!root) {
return createNode(value);
}
const { parent, direction } = findInsertionPoint(root, value);
parent[direction] = createNode(value);
return root;
}
2. Extract Helper Functions
Identify segments of code that perform a distinct operation and extract them into helper functions, even if they're only used once. This improves readability and makes the main algorithm clearer.
3. Use Early Returns
Handle edge cases and special conditions at the beginning of functions to reduce nesting and improve readability:
// Before: Nested conditionals
function processUser(user) {
if (user) {
if (user.isActive) {
if (user.hasPermission) {
// Actual processing logic
return doSomethingWithUser(user);
} else {
return "No permission";
}
} else {
return "User not active";
}
} else {
return "No user provided";
}
}
// After: Early returns
function processUser(user) {
if (!user) return "No user provided";
if (!user.isActive) return "User not active";
if (!user.hasPermission) return "No permission";
// Actual processing logic
return doSomethingWithUser(user);
}
4. Consistent Formatting
Use a consistent style for indentation, spacing, and brace placement. Many languages have established style guides, such as PEP 8 for Python or the Google Style Guide for various languages.
5. Meaningful Variable Names
Replace generic variable names with descriptive ones that indicate purpose:
// Before: Generic variable names
function f(a, n) {
let r = 1;
for(let i = 0; i < n; i++) {
r *= a;
}
return r;
}
// After: Descriptive names
function calculatePower(base, exponent) {
let result = 1;
for(let i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
6. Use Appropriate Data Structures
Select data structures that match the problem's requirements. Using the right data structure often simplifies the algorithm and improves readability.
Language-Specific Organization Patterns
Different programming languages have different conventions and idioms for organizing code. Here are some language-specific recommendations:
Python
- Follow PEP 8 style guidelines
- Use docstrings for function and class documentation
- Leverage list comprehensions and generator expressions for concise, readable code
- Organize related functions into modules and packages
def is_prime(num):
"""
Check if a number is prime.
Args:
num: The number to check
Returns:
bool: True if the number is prime, False otherwise
"""
if num < 2:
return False
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
def find_primes(max_value):
"""Generate a list of prime numbers up to max_value."""
return [num for num in range(2, max_value + 1) if is_prime(num)]
JavaScript
- Use camelCase for variables and functions, PascalCase for classes
- Leverage ES6+ features like arrow functions, destructuring, and spread syntax
- Consider functional programming patterns for data transformations
- Use JSDoc comments for documentation
/**
* Checks if a number is prime
* @param {number} num - The number to check
* @returns {boolean} True if prime, false otherwise
*/
const isPrime = (num) => {
if (num < 2) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
}
return true;
};
/**
* Finds all prime numbers up to a maximum value
* @param {number} maxValue - The upper limit
* @returns {number[]} Array of prime numbers
*/
const findPrimes = (maxValue) => {
return Array.from({ length: maxValue - 1 }, (_, i) => i + 2)
.filter(isPrime);
};
Java
- Follow Java naming conventions: camelCase for methods and variables, PascalCase for classes
- Organize code into packages based on functionality
- Use interfaces to define contracts
- Leverage Java's object-oriented features appropriately
public class PrimeCalculator {
/**
* Checks if a number is prime
* @param num The number to check
* @return true if the number is prime, false otherwise
*/
public static boolean isPrime(int num) {
if (num < 2) return false;
for (int i = 2; i <= Math.sqrt(num); i++) {
if (num % i == 0) return false;
}
return true;
}
/**
* Finds all prime numbers up to a maximum value
* @param maxValue The upper limit
* @return List of prime numbers
*/
public static List<Integer> findPrimes(int maxValue) {
List<Integer> primes = new ArrayList<>();
for (int i = 2; i <= maxValue; i++) {
if (isPrime(i)) {
primes.add(i);
}
}
return primes;
}
}
Structure and Organization in Technical Interviews
In technical interviews, especially at top tech companies, your code's structure and organization can significantly impact the interviewer's perception of your abilities. Here's how to approach this in an interview setting:
Before You Start Coding
- Plan your approach: Spend time outlining the key components and data flow of your solution
- Communicate your structure: Tell the interviewer how you plan to organize your solution
- Identify helper functions: Mention which pieces of functionality you'll extract into separate functions
While Coding
- Write function signatures first: Define the interfaces before implementing details
- Use meaningful names: Even under time pressure, choose descriptive variable and function names
- Maintain consistent indentation: Keep your code visually organized
- Add brief comments: Use comments to explain your reasoning for non-obvious decisions
After Implementing
- Refactor if time permits: Look for opportunities to improve structure
- Discuss trade-offs: Explain any structural decisions and their implications
- Suggest improvements: If you're aware of structural issues but didn't have time to fix them, mention them
Remember, interviewers at companies like Google, Amazon, and Facebook are evaluating not just whether you can solve the problem but how you would approach writing production-quality code as a team member.
Interview Example: Two Sum Problem
Here's how you might approach the classic "Two Sum" problem with good structure and organization:
/**
* Problem: Given an array of integers and a target sum, return the
* indices of two numbers that add up to the target.
*/
function twoSum(nums, target) {
// Handle edge cases
if (!nums || nums.length < 2) {
return null;
}
// Create a map to store values we've seen and their indices
const numToIndex = new Map();
// Iterate through the array once
for (let i = 0; i < nums.length; i++) {
const currentNum = nums[i];
const complement = target - currentNum;
// Check if we've seen the complement before
if (numToIndex.has(complement)) {
return [numToIndex.get(complement), i];
}
// Store the current number and its index
numToIndex.set(currentNum, i);
}
// No solution found
return null;
}
// Example usage
const indices = twoSum([2, 7, 11, 15], 9);
console.log(indices); // [0, 1]
Notice the clear structure, descriptive variable names, comments explaining the approach, and handling of edge cases. These elements make the solution more professional and demonstrate your engineering mindset.
Refactoring: Transforming Disorganized Code
Refactoring is the process of restructuring existing code without changing its external behavior. It's a valuable skill for improving poorly structured code. Here's a systematic approach to refactoring:
1. Identify Code Smells
"Code smells" are indicators of potential problems in code structure:
- Long methods or functions
- Duplicate code
- Large classes
- Long parameter lists
- Feature envy (a method that seems more interested in another class than its own)
- Shotgun surgery (a change that requires many small changes throughout the codebase)
2. Apply Refactoring Techniques
Common refactoring techniques include:
- Extract Method: Move a code fragment into a separate method
- Rename Method/Variable: Change names to better reflect purpose
- Introduce Parameter Object: Replace a long parameter list with an object
- Replace Conditional with Polymorphism: Use object-oriented patterns instead of complex conditionals
- Decompose Conditional: Break complex conditional logic into separate methods
3. Refactoring Example
Let's refactor a poorly structured function that calculates statistics for student grades:
// Before refactoring
function processGrades(students) {
let sum = 0;
let highest = 0;
let lowest = 100;
let failCount = 0;
for (let i = 0; i < students.length; i++) {
sum += students[i].grade;
if (students[i].grade > highest) {
highest = students[i].grade;
}
if (students[i].grade < lowest) {
lowest = students[i].grade;
}
if (students[i].grade < 60) {
failCount++;
console.log(`${students[i].name} failed with grade ${students[i].grade}`);
}
}
const average = sum / students.length;
console.log(`Class average: ${average}`);
console.log(`Highest grade: ${highest}`);
console.log(`Lowest grade: ${lowest}`);
console.log(`Number of failing students: ${failCount}`);
return {
average: average,
highest: highest,
lowest: lowest,
failCount: failCount
};
}
After refactoring:
// After refactoring
// Calculate the average grade
function calculateAverage(grades) {
const sum = grades.reduce((total, grade) => total + grade, 0);
return sum / grades.length;
}
// Find the highest grade
function findHighestGrade(grades) {
return Math.max(...grades);
}
// Find the lowest grade
function findLowestGrade(grades) {
return Math.min(...grades);
}
// Count failing grades
function countFailingGrades(students) {
return students.filter(student => student.grade < 60).length;
}
// Identify failing students
function identifyFailingStudents(students) {
return students.filter(student => student.grade < 60);
}
// Log statistics
function logGradeStatistics(stats) {
console.log(`Class average: ${stats.average}`);
console.log(`Highest grade: ${stats.highest}`);
console.log(`Lowest grade: ${stats.lowest}`);
console.log(`Number of failing students: ${stats.failCount}`);
}
// Main function to process grades
function processGrades(students) {
// Extract grades for easier calculation
const grades = students.map(student => student.grade);
// Calculate statistics
const average = calculateAverage(grades);
const highest = findHighestGrade(grades);
const lowest = findLowestGrade(grades);
const failCount = countFailingGrades(students);
// Log failing students
const failingStudents = identifyFailingStudents(students);
failingStudents.forEach(student => {
console.log(`${student.name} failed with grade ${student.grade}`);
});
// Log overall statistics
const stats = { average, highest, lowest, failCount };
logGradeStatistics(stats);
return stats;
}
The refactored version is more modular, with each function having a single responsibility. It's easier to understand, test, and maintain.
Tools and Resources for Better Code Structure
Several tools and resources can help you improve the structure and organization of your code:
Code Linters and Formatters
- ESLint/TSLint: For JavaScript/TypeScript
- Pylint/Flake8: For Python
- RuboCop: For Ruby
- Checkstyle: For Java
- Prettier: Code formatter for JavaScript, TypeScript, and more
- Black: Code formatter for Python
IDE Features
- Code refactoring tools: Most modern IDEs include tools for extracting methods, renaming variables, etc.
- Code structure visualization: Tools that show the structure of your code visually
- Code analysis: Features that highlight potential issues or improvements
Books on Code Structure
- "Clean Code" by Robert C. Martin: A classic guide to writing readable, maintainable code
- "Refactoring" by Martin Fowler: The definitive guide to improving code structure
- "Code Complete" by Steve McConnell: Comprehensive guide to software construction
- "The Pragmatic Programmer" by Andrew Hunt and David Thomas: Practical advice for software craftsmanship
Online Resources
- Language-specific style guides: Google Style Guides, PEP 8, etc.
- Code review platforms: GitHub, GitLab, Bitbucket for feedback on structure
- AlgoCademy's structured approach tutorials: Learn how to solve problems with proper structure
Conclusion: The Path to Well-Structured Solutions
Improving the structure and organization of your code is a journey, not a destination. It requires continuous learning, practice, and refinement. The benefits, however, are substantial: more readable code, fewer bugs, easier maintenance, and a stronger professional reputation.
As you prepare for technical interviews or work on coding projects, remember that a technically correct solution is just the starting point. A well-structured solution demonstrates not just your ability to solve problems but your capacity to create sustainable, professional-quality code.
At AlgoCademy, we emphasize not just getting to the right answer but getting there in the right way. We encourage you to:
- Practice deliberately: When solving problems, focus not just on correctness but on structure
- Seek feedback: Have others review your code for structural improvements
- Study examples: Analyze well-structured code to understand its organization
- Refactor regularly: Return to your solutions and improve their structure
- Apply principles gradually: Incorporate one new structural principle at a time
By investing in the structure and organization of your code, you're not just preparing for technical interviews; you're developing skills that will serve you throughout your programming career. Remember, in the world of software development, how you solve a problem is often as important as solving it at all.
Start today by revisiting a recent solution and asking: "How could I make this more structured and organized?" The journey to better code begins with that simple question.