Why Your Solutions Are Complicated When They Should Be Simple
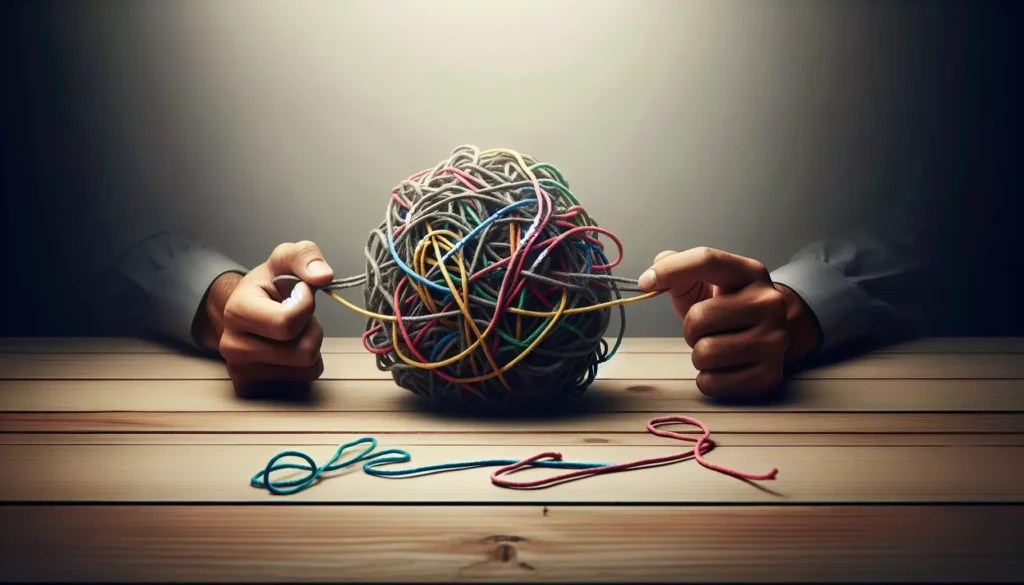
In the world of programming and algorithmic problem-solving, there’s a common phenomenon that plagues both beginners and experienced developers alike: overcomplicated solutions. You might find yourself crafting an intricate, multi-layered approach to a problem that could have been solved with a few simple lines of code. This tendency to overcomplicate is not just frustrating—it can hinder your growth as a programmer and impact your performance in technical interviews, especially for coveted positions at major tech companies.
At AlgoCademy, we’ve observed this pattern repeatedly among our students. Let’s explore why this happens, the consequences of overcomplication, and most importantly, how to train yourself to find elegant, simple solutions that will impress interviewers and make your code more maintainable.
The Overcomplicated Solution Syndrome
Have you ever spent hours building a complex solution to a coding problem, only to discover later that there was a much simpler approach? You’re not alone. This phenomenon, which we might call “Overcomplicated Solution Syndrome,” affects programmers at all skill levels.
Consider this classic example: You’re asked to find if a string is a palindrome (reads the same forward and backward). A beginner might write:
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let reversed = '';
for (let i = cleanStr.length - 1; i >= 0; i--) {
reversed += cleanStr[i];
}
return cleanStr === reversed;
}
While this works, a more experienced developer would recognize a simpler solution:
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
return cleanStr === cleanStr.split('').reverse().join('');
}
And an even more elegant approach might be:
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = cleanStr.length - 1;
while (left < right) {
if (cleanStr[left] !== cleanStr[right]) return false;
left++;
right--;
}
return true;
}
This last solution is not only more efficient (O(n) time with O(1) extra space beyond the cleaned string), but it’s also clearer in expressing the actual logic of palindrome checking.
Why We Overcomplicate Solutions
Understanding the root causes of overcomplication can help us combat this tendency. Here are some key reasons we often make solutions more complex than necessary:
1. Insufficient Problem Analysis
When we rush into coding without fully understanding the problem, we often build solutions that address more than what’s required or miss the core of the challenge entirely. This happens frequently in interview settings where nervousness leads to hasty implementations.
For example, if asked to find the sum of all even numbers in an array, you might write a complex filtering mechanism when a simple modulo check in a loop would suffice.
2. Showing Off Technical Knowledge
Especially in interview situations, there’s a temptation to showcase your entire programming repertoire. This might lead you to implement advanced data structures or algorithms when simpler approaches would be more appropriate.
Remember: impressive code isn’t necessarily complex code. Often, what impresses interviewers most is your ability to identify the simplest, most efficient solution to a problem.
3. Lack of Experience with Design Patterns
Without exposure to common design patterns and algorithmic approaches, developers often reinvent the wheel with unnecessarily complex implementations. This is why studying established patterns is crucial for developing efficient problem-solving skills.
4. The Curse of Knowledge
Sometimes, we overcomplicate solutions because we’re bringing too much background knowledge to a problem. We might be thinking about edge cases or optimizations that aren’t relevant to the current context.
5. Premature Optimization
As Donald Knuth famously said, “Premature optimization is the root of all evil.” Trying to optimize code before you’ve even implemented a working solution often leads to unnecessary complexity.
The Cost of Complexity
Overcomplicating solutions isn’t just an aesthetic issue; it carries real costs:
1. Reduced Maintainability
Complex code is harder to understand, debug, and modify. This becomes particularly problematic in collaborative environments where others need to work with your code.
2. Increased Bug Potential
More moving parts mean more opportunities for things to go wrong. Simple solutions tend to have fewer bugs and are easier to test thoroughly.
3. Poor Performance in Interviews
Technical interviews at major tech companies often evaluate not just whether you can solve a problem, but how elegantly and efficiently you can solve it. Overengineered solutions can signal poor judgment to interviewers.
4. Slower Development
Building and debugging complex solutions takes more time, reducing your productivity and potentially delaying project timelines.
Real-World Examples of Overcomplication
Let’s look at some common scenarios where developers tend to overcomplicate solutions:
Example 1: Finding the Maximum Value in an Array
Overcomplicated approach:
function findMax(arr) {
if (arr.length === 0) return null;
let result = arr[0];
const stack = [...arr];
while (stack.length > 0) {
const current = stack.pop();
if (current > result) {
result = current;
}
}
return result;
}
Simple approach:
function findMax(arr) {
if (arr.length === 0) return null;
return Math.max(...arr);
// Or the manual approach:
// let max = arr[0];
// for (let i = 1; i < arr.length; i++) {
// if (arr[i] > max) max = arr[i];
// }
// return max;
}
Example 2: Checking if an Array Contains Duplicates
Overcomplicated approach:
function containsDuplicates(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = 0; j < arr.length; j++) {
if (i !== j && arr[i] === arr[j]) {
return true;
}
}
}
return false;
}
Simple approach:
function containsDuplicates(arr) {
return new Set(arr).size !== arr.length;
// Or using a hash map for O(n) time complexity:
// const seen = {};
// for (const num of arr) {
// if (seen[num]) return true;
// seen[num] = true;
// }
// return false;
}
Example 3: Reversing a String
Overcomplicated approach:
function reverseString(str) {
const charArray = [];
for (let i = 0; i < str.length; i++) {
charArray.push(str[i]);
}
let left = 0;
let right = charArray.length - 1;
while (left < right) {
const temp = charArray[left];
charArray[left] = charArray[right];
charArray[right] = temp;
left++;
right--;
}
let result = '';
for (let i = 0; i < charArray.length; i++) {
result += charArray[i];
}
return result;
}
Simple approach:
function reverseString(str) {
return str.split('').reverse().join('');
// Or if you want to do it manually:
// let reversed = '';
// for (let i = str.length - 1; i >= 0; i--) {
// reversed += str[i];
// }
// return reversed;
}
The Path to Simplicity: Strategies for Finding Elegant Solutions
Now that we understand why we overcomplicate and the cost of doing so, let’s explore strategies to develop simpler, more elegant solutions:
1. Understand the Problem Deeply Before Coding
Take time to fully comprehend what the problem is asking before writing a single line of code. This might include:
- Restating the problem in your own words
- Identifying the inputs and expected outputs
- Working through examples manually
- Considering edge cases
At AlgoCademy, we encourage students to spend at least 25% of their problem-solving time on understanding and planning before implementation.
2. Start with a Brute Force Solution
Begin with the simplest approach that correctly solves the problem, even if it’s not the most efficient. This gives you a working solution and a baseline to improve upon.
For example, if asked to find pairs in an array that sum to a target value, start with the O(n²) nested loop approach before considering more optimized solutions using hash maps.
3. Refactor Incrementally
Once you have a working solution, look for opportunities to simplify and optimize. Ask yourself:
- Can I eliminate any unnecessary steps?
- Are there built-in functions or data structures that could simplify my code?
- Am I repeating any calculations that could be stored instead?
4. Learn to Recognize Common Patterns
Many programming problems follow established patterns. Familiarizing yourself with these patterns can help you identify simpler solutions more quickly. Some common patterns include:
- Two-pointer technique
- Sliding window
- Binary search
- Breadth-first and depth-first search
- Dynamic programming
5. Practice Explaining Your Solutions
Articulating your approach out loud can help identify unnecessary complexity. If you struggle to explain your solution concisely, it might be more complex than necessary.
6. Study Elegant Solutions
Regularly review well-crafted solutions to common problems. Platforms like AlgoCademy provide access to expert solutions that demonstrate elegant approaches to algorithmic challenges.
7. Embrace the KISS Principle
“Keep It Simple, Stupid” is a design principle that values simplicity over complexity. When evaluating different approaches, lean toward the simpler solution unless there’s a compelling reason not to.
Case Study: Simplifying a Technical Interview Problem
Let’s walk through a common interview problem and see how we might evolve from a complicated solution to a simpler one.
Problem: Given an array of integers, find the longest continuous increasing subsequence.
Initial Complicated Approach:
function findLongestIncreasingSubsequence(nums) {
if (nums.length === 0) return 0;
// Initialize dynamic programming array
const dp = new Array(nums.length).fill(1);
// Track all increasing subsequences
const sequences = {};
for (let i = 0; i < nums.length; i++) {
sequences[i] = [nums[i]];
}
// Build all possible subsequences
for (let i = 1; i < nums.length; i++) {
for (let j = 0; j < i; j++) {
if (nums[i] > nums[j] && nums[i] === nums[j] + 1) {
if (dp[j] + 1 > dp[i]) {
dp[i] = dp[j] + 1;
sequences[i] = [...sequences[j], nums[i]];
}
}
}
}
// Find the maximum length
let maxLength = 0;
let maxIndex = 0;
for (let i = 0; i < dp.length; i++) {
if (dp[i] > maxLength) {
maxLength = dp[i];
maxIndex = i;
}
}
return sequences[maxIndex].length;
}
This solution is unnecessarily complex. It’s using a dynamic programming approach more suitable for the “longest increasing subsequence” problem, not the “longest continuous increasing subsequence” problem. It’s also tracking the actual subsequences, which isn’t required by the problem statement.
Simplified Approach:
function findLongestIncreasingSubsequence(nums) {
if (nums.length === 0) return 0;
let maxLength = 1;
let currentLength = 1;
for (let i = 1; i < nums.length; i++) {
if (nums[i] > nums[i-1]) {
currentLength++;
maxLength = Math.max(maxLength, currentLength);
} else {
currentLength = 1;
}
}
return maxLength;
}
This solution is much simpler and more efficient. It recognizes that we only need to track the length of the current increasing subsequence and the maximum length found so far. By comparing each element with its predecessor, we can easily determine if the current subsequence continues or if we need to start a new one.
The Psychological Barriers to Simplicity
Sometimes, the barriers to finding simple solutions are more psychological than technical:
1. Impostor Syndrome
Many developers feel they need to demonstrate complex solutions to prove their worth, especially in interview situations. This can lead to deliberately overengineered code.
2. The “Not Invented Here” Syndrome
There’s a tendency to distrust or avoid simple, standard solutions in favor of custom-built approaches. This often stems from a desire to demonstrate creativity or a misplaced sense that standard solutions are somehow inadequate.
3. Perfectionism
The pursuit of the “perfect” solution can prevent you from recognizing when a simple solution is actually optimal. Remember that in most real-world scenarios, clarity and maintainability are more important than theoretical perfection.
4. Fear of Missing Edge Cases
Concern about edge cases can lead to overly defensive coding with numerous checks and branches. While edge cases are important, they should be handled in a clean, organized way that doesn’t obscure the main logic.
How Technical Interviews at Top Companies Evaluate Simplicity
At major tech companies like Google, Amazon, Facebook, Apple, and Netflix (often collectively referred to as FAANG), interviewers are specifically trained to evaluate the simplicity and elegance of candidates’ solutions. Here’s what they’re looking for:
1. Problem-Solving Process
Interviewers watch how you approach the problem. Do you dive straight into coding a complex solution, or do you take time to understand the problem and consider different approaches?
2. Code Clarity
Clear, readable code is highly valued. Variable names should be descriptive, and the overall structure should be easy to follow.
3. Efficiency
While brute force solutions are acceptable as starting points, interviewers expect you to recognize opportunities for optimization without unnecessary complexity.
4. Ability to Iterate
The willingness to start with a simple solution and improve it incrementally demonstrates good engineering judgment.
5. Communication
Can you explain your solution clearly? This is often as important as the solution itself.
Tools and Practices for Developing Simplicity
Here are some practical tools and practices to help cultivate the skill of finding simple solutions:
1. Code Review
Regularly having your code reviewed by peers can help identify unnecessary complexity. Questions like “Could this be simpler?” should be standard in code reviews.
2. Refactoring Practice
Dedicate time specifically to refactoring existing code to make it simpler without changing its functionality.
3. The “Explain It to a Five-Year-Old” Test
If you can’t explain your solution in simple terms, it might be more complex than necessary.
4. Time-Boxed Solutions
Practice solving problems with strict time limits to force yourself to focus on straightforward approaches rather than perfect but complex solutions.
5. Study Library Code
Examine the source code of well-regarded libraries and frameworks. They often demonstrate elegant solutions to complex problems.
The Role of Practice in Finding Simple Solutions
Like any skill, finding elegant, simple solutions improves with practice. At AlgoCademy, we’ve observed that students who regularly practice problem-solving develop an intuition for simplicity that serves them well in technical interviews and real-world programming.
Here’s how to practice effectively:
1. Solve Problems Regularly
Aim to solve at least one algorithmic problem daily. Platforms like AlgoCademy provide a structured approach to building this habit.
2. Review Multiple Solutions
After solving a problem, study alternative solutions. What makes some approaches simpler or more elegant than others?
3. Implement the Same Solution Multiple Ways
Challenge yourself to solve the same problem using different approaches, then compare them for simplicity and efficiency.
4. Practice with Time Constraints
Technical interviews typically have time limits. Practice solving problems within similar timeframes to develop the skill of finding simple solutions quickly.
Conclusion: The Art of Simple Solutions
Finding simple, elegant solutions to complex problems is both an art and a science. It requires a deep understanding of fundamentals, a willingness to start with straightforward approaches, and the discipline to refactor toward simplicity.
Remember that in both technical interviews and real-world programming, simple solutions are almost always preferred. They’re easier to understand, maintain, and debug. They demonstrate not just technical skill but also good engineering judgment.
At AlgoCademy, we believe that the ability to find simple solutions to complex problems is what separates good programmers from great ones. By understanding why we tend to overcomplicate, recognizing the value of simplicity, and practicing regularly with a focus on elegant solutions, you can develop this crucial skill and excel in technical interviews at even the most competitive companies.
The next time you find yourself crafting a complex solution, pause and ask: “Could this be simpler?” The answer might just be the key to your next breakthrough.
Key Takeaways
- Understand the problem fully before jumping into code
- Start with a working solution, then simplify
- Learn to recognize common algorithmic patterns
- Practice explaining your solutions to others
- Study well-crafted, elegant solutions
- Remember that simplicity is valued in technical interviews
- Practice regularly to develop an intuition for simplicity
By embracing these principles, you’ll not only perform better in technical interviews but also become a more effective programmer capable of creating maintainable, efficient code that stands the test of time.