Why Your Solution Works But Isn’t How Programmers Think
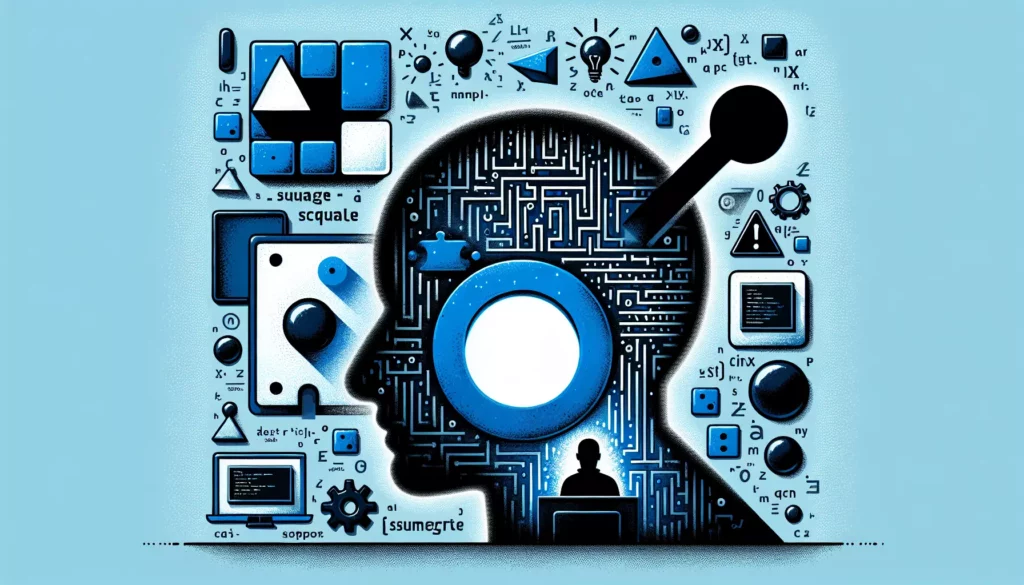
Have you ever submitted a solution to a coding problem that technically worked but received feedback that it wasn’t quite right? Or perhaps you’ve had an instructor look at your perfectly functional code and say, “Well, that’s not how a programmer would approach this.” This disconnect can be frustrating and confusing, especially when you’re just starting your coding journey.
The truth is, there’s often a significant gap between code that simply works and code that reflects how experienced programmers think. At AlgoCademy, we’ve observed this pattern repeatedly among learners preparing for technical interviews at major tech companies.
In this comprehensive guide, we’ll explore this gap, understand why it exists, and learn how to bridge it to not just solve problems, but solve them like a professional programmer.
The Gap Between Working Code and Professional Code
Let’s start with a simple truth: computers don’t care how elegantly your code is written. They’ll execute anything that follows the syntax rules of the programming language. However, professional programmers care deeply about how code is structured, not just whether it produces the correct output.
Consider this simple problem: Write a function that returns the sum of all numbers from 1 to n.
A beginner might write something like:
function sumNumbers(n) {
let sum = 0;
for (let i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
This solution works perfectly fine. It’s correct, it’s reasonably efficient with O(n) time complexity, and it’s easy to understand. But an experienced programmer might immediately recognize that there’s a mathematical formula for this: the sum of numbers from 1 to n is n * (n + 1) / 2.
So they might write:
function sumNumbers(n) {
return n * (n + 1) / 2;
}
Both solutions work correctly, but the second one is O(1) time complexity and demonstrates a deeper understanding of the problem. This is a simple example of the gap we’re discussing.
Understanding How Programmers Think
Professional programmers approach problems with several mindsets that beginners often haven’t developed yet:
1. Pattern Recognition
Experienced programmers have seen hundreds or thousands of problems and solutions. They recognize patterns in problems and can quickly identify which approaches are likely to work best.
For example, when they see a problem involving searching through sorted data, they immediately think “binary search” rather than a linear scan. When they encounter a problem requiring tracking of frequencies, they reach for a hash map without hesitation.
2. Algorithmic Thinking
Professional programmers don’t just write code; they design algorithms. They think about:
- Time complexity: How will performance scale with input size?
- Space complexity: How much memory will this solution require?
- Edge cases: What happens with empty inputs, very large inputs, or unusual inputs?
- Tradeoffs: Is it worth sacrificing readability for performance in this case?
3. Code as Communication
Experienced programmers know that code is read far more often than it’s written. They write code primarily for other humans (including their future selves), not for computers.
This means they focus on:
- Clear variable and function names
- Consistent formatting and style
- Appropriate comments and documentation
- Logical organization of code
4. Systems Thinking
Professional programmers consider how their code fits into the larger system or codebase. They think about:
- Modularity: How can this code be reused?
- Interfaces: How will other parts of the system interact with this code?
- Maintainability: How easy will it be to change this code in the future?
- Testing: How can we verify this code works correctly?
Common Gaps in Beginner Solutions
Now that we understand how professionals think, let’s examine some common ways beginner solutions differ from professional ones:
Brute Force vs. Optimized Approaches
Beginners often implement the first solution that comes to mind, which is typically a brute force approach. Consider finding the maximum subarray sum in an array of integers.
A brute force solution might look like:
function maxSubarraySum(arr) {
let maxSum = Number.MIN_SAFE_INTEGER;
for (let i = 0; i < arr.length; i++) {
for (let j = i; j < arr.length; j++) {
let currentSum = 0;
for (let k = i; k <= j; k++) {
currentSum += arr[k];
}
maxSum = Math.max(maxSum, currentSum);
}
}
return maxSum;
}
This works but has O(n³) time complexity. An experienced programmer would recognize this as a classic problem solvable with Kadane’s algorithm:
function maxSubarraySum(arr) {
let maxSoFar = arr[0];
let maxEndingHere = arr[0];
for (let i = 1; i < arr.length; i++) {
maxEndingHere = Math.max(arr[i], maxEndingHere + arr[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
}
return maxSoFar;
}
This solution is O(n) time complexity and demonstrates knowledge of a well-known algorithm.
Reinventing the Wheel
Beginners often write custom code for problems that have standard library solutions. For example, sorting an array manually when the language provides a sort function, or implementing string manipulation functions that already exist.
Professional programmers know the standard libraries of their languages well and leverage them appropriately. They follow the principle: “Don’t write what you can import.”
Ignoring Edge Cases
Beginners often write code that works for the “happy path” but fails on edge cases:
function divide(a, b) {
return a / b; // What if b is 0?
}
function getFirstElement(arr) {
return arr[0]; // What if arr is empty?
}
Professional programmers anticipate and handle these cases:
function divide(a, b) {
if (b === 0) {
throw new Error("Division by zero");
}
return a / b;
}
function getFirstElement(arr) {
if (!arr || arr.length === 0) {
return null; // or throw an error, depending on requirements
}
return arr[0];
}
Lack of Data Structure Knowledge
Beginners often default to arrays for all data storage, even when other structures would be more appropriate. Consider checking if a collection contains a specific element:
function containsElement(arr, element) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === element) {
return true;
}
}
return false;
}
This works but has O(n) time complexity. A professional might use a Set for O(1) lookups:
function containsElement(collection, element) {
// If collection is already a Set, use it directly
const set = collection instanceof Set ? collection : new Set(collection);
return set.has(element);
}
Unclear Intent
Beginner code often doesn’t clearly communicate its purpose:
function process(arr) {
let result = [];
for (let i = 0; i < arr.length; i++) {
if (arr[i] % 2 === 0) {
result.push(arr[i]);
}
}
return result;
}
What does this function do? It filters even numbers, but the name doesn’t indicate that. A professional would write:
function filterEvenNumbers(numbers) {
return numbers.filter(num => num % 2 === 0);
}
This version is not only clearer but also uses appropriate built-in functions.
Real-World Example: The Two Sum Problem
Let’s look at a popular interview question: Given an array of integers and a target sum, return the indices of two numbers that add up to the target.
A beginner might write:
function twoSum(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return null; // No solution found
}
This brute force approach works with O(n²) time complexity. An experienced programmer would recognize that we can do better with a hash map:
function twoSum(nums, target) {
const numToIndex = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (numToIndex.has(complement)) {
return [numToIndex.get(complement), i];
}
numToIndex.set(nums[i], i);
}
return null; // No solution found
}
This solution has O(n) time complexity and demonstrates:
- Knowledge of appropriate data structures (using a Map for O(1) lookups)
- Understanding of algorithmic efficiency (reducing from O(n²) to O(n))
- Clear variable names that communicate intent (complement, numToIndex)
How to Bridge the Gap
If you recognize that your code works but doesn’t reflect professional thinking, here are strategies to bridge that gap:
1. Study Data Structures and Algorithms
Understanding fundamental data structures (arrays, linked lists, stacks, queues, trees, graphs, hash tables) and algorithms (sorting, searching, dynamic programming, graph algorithms) is crucial. Resources like:
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell
- Courses: AlgoCademy’s curriculum, which builds this knowledge systematically
- Websites: LeetCode, HackerRank, and other coding practice platforms
2. Read Professional Code
Reading high-quality code is one of the best ways to learn how professionals think:
- Explore open-source projects on GitHub
- Study standard libraries in your programming language
- Review code written by experienced colleagues
When reading code, ask yourself:
- Why did they structure it this way?
- What patterns are they using?
- How are they handling edge cases?
- What naming conventions make the code clear?
3. Practice Refactoring
Take working code (your own or others’) and practice improving it without changing its functionality:
- Improve variable and function names
- Break down complex functions into smaller ones
- Replace manual implementations with library functions
- Optimize for time and space complexity
4. Seek Code Reviews
Having experienced programmers review your code is invaluable:
- Ask colleagues or mentors to review your code
- Participate in pair programming sessions
- Join coding communities where you can share and discuss code
- Submit pull requests to open-source projects
5. Solve Problems Multiple Ways
When you solve a problem, challenge yourself to find alternative solutions:
- Can you solve it with a different data structure?
- Is there a more efficient algorithm?
- Can you make it more readable?
- How would you solve it if memory was very limited?
Case Study: Fibonacci Sequence
Let’s see how different approaches to the same problem reflect different levels of professional thinking. The task: Calculate the nth Fibonacci number.
Approach 1: Recursive (Beginner)
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
This solution is elegant and matches the mathematical definition, but it has exponential time complexity O(2ⁿ) due to redundant calculations.
Approach 2: Iterative (Intermediate)
function fibonacci(n) {
if (n <= 1) return n;
let a = 0, b = 1;
for (let i = 2; i <= n; i++) {
const temp = a + b;
a = b;
b = temp;
}
return b;
}
This solution has O(n) time complexity and O(1) space complexity, showing an understanding of algorithm efficiency.
Approach 3: Memoization (Advanced)
function fibonacci(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
This solution combines the elegance of recursion with the efficiency of caching results, demonstrating knowledge of dynamic programming techniques.
Approach 4: Matrix Exponentiation (Expert)
function fibonacci(n) {
if (n <= 1) return n;
let matrix = [[1, 1], [1, 0]];
let result = matrixPower(matrix, n - 1);
return result[0][0];
}
function matrixMultiply(a, b) {
return [
[a[0][0] * b[0][0] + a[0][1] * b[1][0], a[0][0] * b[0][1] + a[0][1] * b[1][1]],
[a[1][0] * b[0][0] + a[1][1] * b[1][0], a[1][0] * b[0][1] + a[1][1] * b[1][1]]
];
}
function matrixPower(matrix, n) {
if (n === 1) return matrix;
if (n % 2 === 0) {
const half = matrixPower(matrix, n / 2);
return matrixMultiply(half, half);
} else {
return matrixMultiply(matrix, matrixPower(matrix, n - 1));
}
}
This solution achieves O(log n) time complexity by using matrix exponentiation and the divide-and-conquer approach, demonstrating deep mathematical understanding and advanced algorithmic techniques.
Each approach works correctly, but they reflect very different levels of algorithmic thinking and optimization knowledge.
The Impact on Technical Interviews
Understanding how programmers think is particularly important for technical interviews at companies like Google, Amazon, Facebook, Apple, and Netflix (often referred to as FAANG).
In these interviews, merely producing working code is insufficient. Interviewers evaluate:
- Your problem-solving process, not just the final solution
- How you analyze time and space complexity
- Your ability to optimize initial solutions
- How you communicate your thinking
- Whether you consider edge cases without prompting
A common interview pattern is:
- You solve the problem with a brute force approach
- The interviewer asks, “Can we do better?”
- You’re expected to recognize optimization opportunities
If you can only implement the brute force solution, you’re unlikely to pass, even if that solution technically works.
Beyond Algorithms: Professional Thinking in Software Engineering
While we’ve focused on algorithmic problem-solving, professional thinking extends to all aspects of software engineering:
Code Organization and Architecture
Professionals think about how to structure code for maintainability:
- Using design patterns appropriately
- Separating concerns (e.g., MVC pattern)
- Creating clear abstractions and interfaces
- Following SOLID principles
Testing
Professionals write code with testing in mind:
- Writing unit tests for critical functionality
- Designing code to be testable (e.g., dependency injection)
- Considering test coverage and edge cases
Performance and Scalability
Professionals consider how code will behave at scale:
- Database query optimization
- Caching strategies
- Asynchronous processing
- Load balancing and distributed systems
Security
Professionals think about potential security vulnerabilities:
- Input validation and sanitization
- Protection against common attacks (XSS, CSRF, SQL injection)
- Secure authentication and authorization
- Data encryption and protection
Conclusion: The Journey to Professional Thinking
Bridging the gap between code that works and code that reflects professional thinking is a journey that takes time and deliberate practice. It’s not just about learning syntax or memorizing algorithms; it’s about developing a mindset that approaches problems systematically, considers tradeoffs thoughtfully, and communicates solutions clearly.
At AlgoCademy, we’ve designed our curriculum to not just teach you how to write working code, but to help you think like a professional programmer. Our interactive tutorials guide you through the process of optimizing solutions, our AI-powered assistance helps identify improvements in your code, and our step-by-step guidance builds the pattern recognition that experienced programmers rely on.
Remember that every professional programmer started as a beginner. The difference is that they continuously pushed themselves beyond solutions that merely work to solutions that demonstrate deeper understanding. With deliberate practice and the right guidance, you can develop this professional thinking too.
So the next time your solution works but receives feedback that “it’s not how a programmer would approach this,” don’t be discouraged. See it as an opportunity to bridge the gap and level up your programming skills. The journey from working code to professional code is what transforms coding from a skill into a craft.
Common Questions About Professional Programming Thinking
Is it always better to optimize for performance?
No. Professional programmers follow the principle: “Make it work, make it right, make it fast” in that order. Premature optimization can lead to complex, hard-to-maintain code. Professionals optimize when there’s a demonstrated need, not by default.
How important are variable names really?
Extremely important. Clear, descriptive variable names make code self-documenting and reduce the cognitive load for anyone reading the code (including your future self). The time saved in typing short variable names is lost many times over in the time spent understanding what they represent.
Should I always use the most advanced algorithm?
Not necessarily. Professional programmers choose algorithms based on the specific requirements, including:
- Expected input size and characteristics
- Performance requirements
- Readability and maintainability needs
- Development time constraints
Sometimes a simple algorithm is preferable if it’s more readable and the performance difference isn’t significant for your use case.
How do I know which data structure to use?
This comes with experience, but generally:
- Arrays/Lists: When you need ordered data with fast iteration
- Hash Maps/Dictionaries: When you need fast lookups by key
- Sets: When you need to track unique values
- Stacks: When you need LIFO (Last In, First Out) behavior
- Queues: When you need FIFO (First In, First Out) behavior
- Trees: When you need hierarchical data or fast search/insert/delete
- Graphs: When you need to represent connections between items
Understanding the time complexity of operations on each structure helps make informed choices.
By continuing to learn and practice, you’ll develop the intuition that helps professional programmers choose the right approach for each problem they encounter.