Why Your Solution Approach Isn’t What Interviewers Want to See
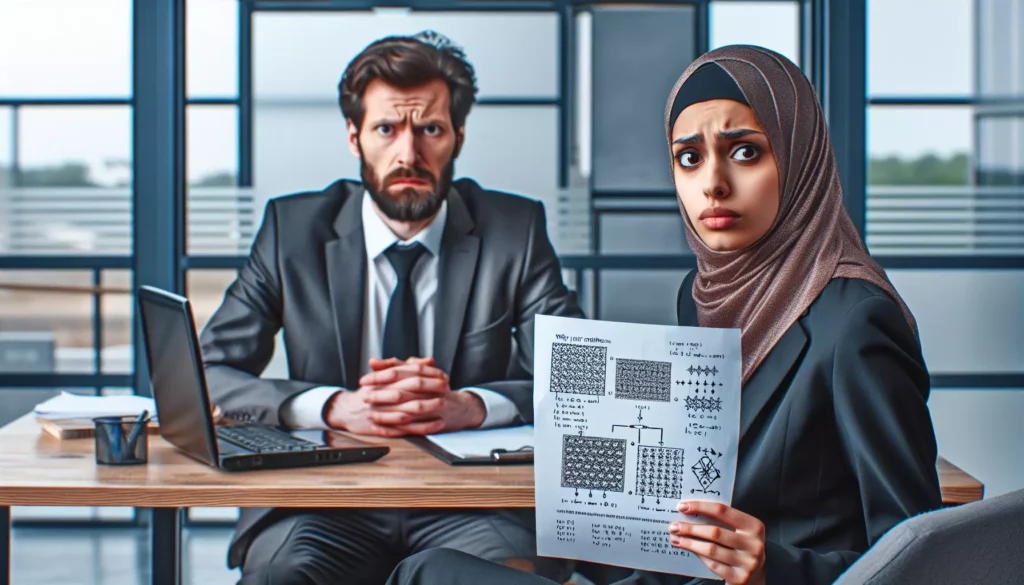
If you’ve been preparing for technical interviews at major tech companies like Google, Amazon, or Meta (formerly Facebook), you’ve likely spent countless hours solving coding problems on platforms like LeetCode, HackerRank, or AlgoExpert. Despite your diligent preparation, you might still find yourself struggling during actual interviews. The reason? Your solution approach might not be what interviewers are looking for.
In this comprehensive guide, we’ll explore why many candidates fail to impress interviewers despite having solid coding skills, and what you can do to align your approach with interviewer expectations.
Table of Contents
- Common Mistakes in Technical Interviews
- What Interviewers Actually Want to See
- The Ideal Solution Process
- The Art of Technical Communication
- Effective Problem Analysis
- Code Quality and Clean Coding Practices
- Testing and Edge Cases
- Optimization and Trade offs
- Real Examples: Before and After
- Practice Strategies That Work
- Conclusion
Common Mistakes in Technical Interviews
Let’s start by identifying the most common pitfalls candidates encounter during technical interviews.
Rushing to Code
One of the most prevalent mistakes is jumping straight into coding without adequate problem analysis. When an interviewer presents a problem, many candidates feel an immediate pressure to start writing code. This rush often leads to incomplete understanding of the problem, overlooked edge cases, and inefficient solutions.
Consider this scenario: An interviewer asks you to implement a function to find the kth largest element in an array. If you immediately start coding a sorting algorithm, you’ve missed an opportunity to discuss potential approaches and their trade offs.
Neglecting Communication
Technical interviews aren’t just about solving problems; they’re about demonstrating your thought process. Many candidates work silently, leaving the interviewer in the dark about their reasoning. This silence can be interpreted as inability to articulate technical concepts or, worse, lack of a structured approach to problem solving.
Implementing the First Solution That Comes to Mind
Often, the first solution that comes to mind isn’t the most efficient one. Candidates frequently implement the first approach they think of without considering alternatives. This demonstrates a lack of critical thinking and problem solving flexibility.
Ignoring Edge Cases
Robust solutions handle all possible inputs, including edge cases. Candidates often focus on the happy path and neglect scenarios like empty arrays, null inputs, or boundary conditions. This oversight suggests a lack of thoroughness in your approach.
Writing Messy, Unreadable Code
Even if your solution is algorithmically correct, messy code with poor variable naming, inconsistent formatting, or lack of structure can significantly detract from your performance. Interviewers value readability and maintainability as much as correctness.
What Interviewers Actually Want to See
Understanding what interviewers are truly evaluating can help you align your approach with their expectations.
Problem Solving Process
Contrary to popular belief, getting the right answer isn’t the primary goal of a technical interview. Interviewers are more interested in your problem solving process. They want to see how you:
- Break down complex problems into manageable components
- Analyze requirements and constraints
- Consider multiple approaches before choosing one
- Adapt when your initial approach doesn’t work
A candidate who articulates a clear, structured approach but doesn’t complete the solution might be preferred over someone who silently produces a working solution without explaining their thought process.
Communication Skills
Software development is a collaborative endeavor. Your ability to communicate technical concepts clearly is crucial for effective teamwork. Interviewers assess whether you can:
- Explain your understanding of the problem
- Articulate your solution approach
- Discuss trade offs between different solutions
- Respond constructively to feedback or hints
Technical Competence
While process and communication are vital, technical competence remains a fundamental requirement. Interviewers evaluate your:
- Understanding of data structures and algorithms
- Familiarity with programming language features
- Ability to translate your approach into working code
- Knowledge of time and space complexity analysis
Attention to Detail
Production code must be robust. Interviewers look for candidates who demonstrate:
- Consideration of edge cases and error handling
- Testing strategies for validating solutions
- Clean, readable code with meaningful variable names
- Consistent formatting and adherence to conventions
The Ideal Solution Process
Now that we understand what interviewers are looking for, let’s outline an ideal approach to technical interview problems.
1. Clarify the Problem
Begin by ensuring you fully understand the problem. Ask questions to clarify requirements, constraints, and expected behavior.
Example questions:
- “Can I assume the input array is always non empty?”
- “Should the function handle invalid inputs like null values?”
- “What should happen if k is larger than the array size?”
- “Are there any constraints on memory usage or execution time?”
2. Work Through Examples
Before coding, work through examples to verify your understanding. Start with simple cases, then consider edge cases.
For the kth largest element problem:
- Simple case: [3, 1, 5, 2], k=2 → Output: 3
- Edge case: [7], k=1 → Output: 7
- Edge case: [5, 5, 5], k=2 → Output: 5 (clarify if duplicates count as separate elements)
3. Outline Multiple Approaches
Before committing to an implementation, briefly outline 2-3 possible approaches and discuss their trade offs.
For finding the kth largest element:
- Approach 1: Sort the array and return the kth element from the end. Time complexity: O(n log n)
- Approach 2: Use a min heap of size k. Time complexity: O(n log k)
- Approach 3: Use quickselect algorithm. Average time complexity: O(n)
4. Select and Explain Your Approach
Choose the most appropriate approach based on the problem constraints and explain your reasoning.
“I’ll implement the min heap approach because it offers a good balance between code simplicity and performance. While quickselect has better average case performance, the heap approach has more predictable performance and is easier to implement correctly in an interview setting.”
5. Plan Your Implementation
Before writing code, outline your implementation plan in pseudocode or high level steps.
1. Create a min heap
2. Iterate through the array:
a. If heap size < k, add element to heap
b. If current element > heap root, remove root and add current element
3. The root of the heap will be the kth largest element
6. Implement the Solution
Now write clean, readable code based on your plan. Narrate your implementation to keep the interviewer engaged.
public int findKthLargest(int[] nums, int k) {
// Create a min heap
PriorityQueue<Integer> minHeap = new PriorityQueue<>();
// Process each element
for (int num : nums) {
// If heap size is less than k, simply add the element
if (minHeap.size() < k) {
minHeap.add(num);
}
// If current element is larger than the smallest in heap,
// remove smallest and add current element
else if (num > minHeap.peek()) {
minHeap.poll();
minHeap.add(num);
}
}
// The root of the heap is the kth largest element
return minHeap.peek();
}
7. Test Your Solution
Walk through your code with test cases, including edge cases.
Example trace for [3, 1, 5, 2], k=2:
- Initialize empty min heap
- Process 3: Add to heap → [3]
- Process 1: Add to heap → [1, 3]
- Process 5: Heap size = k, 5 > 1, remove 1 and add 5 → [3, 5]
- Process 2: Heap size = k, 2 < 3, do nothing → [3, 5]
- Return heap root: 3
8. Analyze Complexity
Discuss the time and space complexity of your solution.
“The time complexity is O(n log k) where n is the array size and k is the parameter. We perform at most n heap operations, each taking O(log k) time. The space complexity is O(k) for storing the heap.”
9. Discuss Optimizations
If time permits, discuss potential optimizations or alternative approaches.
“If we need to optimize further, we could implement the quickselect algorithm which has O(n) average time complexity, though its worst case is O(n²).”
The Art of Technical Communication
Effective communication during a technical interview can significantly enhance your performance. Here are key strategies to improve your communication:
Think Aloud
Narrate your thought process continuously. This gives the interviewer insight into your reasoning and allows them to provide hints if you’re heading in the wrong direction.
Instead of saying “I’ll use a min heap for this,” elaborate: “I’m considering using a min heap structure because it will allow us to efficiently track the k largest elements we’ve seen so far. Each time we encounter a new element, we can compare it with the smallest element in our heap in constant time.”
Use Visual Aids
When explaining complex algorithms or data structures, use diagrams or visual representations. This can make your explanation clearer and demonstrates your ability to communicate technical concepts visually.
For example, when explaining a tree traversal algorithm, sketch the tree and trace the traversal path, indicating the state of your data structures at each step.
Structure Your Communication
Organize your communication logically. Start with a high level overview, then dive into details. When explaining your approach, clearly separate the what (your solution) from the why (your reasoning).
“I’m going to solve this using dynamic programming. Here’s why: The problem exhibits optimal substructure because the solution to the larger problem can be constructed from solutions to its subproblems. Additionally, there are overlapping subproblems that we’ll need to solve repeatedly, making memoization beneficial.”
Seek Validation
Periodically check in with the interviewer to ensure you’re on the right track. This demonstrates collaborative skills and prevents you from spending too much time on an approach the interviewer doesn’t want to see.
“I’m thinking of approaching this using a breadth first search. Does that sound reasonable before I proceed with the implementation?”
Respond Constructively to Feedback
When an interviewer provides feedback or hints, acknowledge them and incorporate them into your approach. This shows adaptability and receptiveness to guidance.
“That’s a great point about the potential for stack overflow with a recursive approach. Let me revise my solution to use an iterative approach instead.”
Effective Problem Analysis
Thorough problem analysis is the foundation of a successful technical interview. Here’s how to analyze problems effectively:
Identify the Problem Type
Recognizing the category of problem can help you apply appropriate patterns and algorithms.
Common problem types include:
- Array manipulation
- String processing
- Tree or graph traversal
- Dynamic programming
- Searching and sorting
- Two pointer or sliding window
- Backtracking
Analyze Constraints
Understanding the constraints can guide you toward the optimal solution approach.
Key constraints to consider:
- Input size limits (affects time complexity requirements)
- Memory restrictions (affects space complexity requirements)
- Range of input values (affects choice of data types and edge cases)
- Special input characteristics (sorted, contains duplicates, etc.)
Decompose the Problem
Break down complex problems into smaller, more manageable subproblems.
For example, if asked to implement a file system, you might decompose it into:
- Directory structure representation
- File/directory creation and deletion
- Path resolution and navigation
- Permission management
Consider Brute Force First
Start with a brute force approach, even if it’s inefficient. This establishes a baseline solution that you can optimize later and demonstrates that you can at least solve the problem.
“A brute force approach would be to check all possible subarrays, which would be O(n³). Let me walk through this approach first, then we can discuss optimizations.”
Look for Patterns and Insights
Analyze example inputs and outputs to identify patterns that might lead to more efficient algorithms.
“I notice that whenever we encounter a closing parenthesis, we need to match it with the most recent opening parenthesis, which suggests a stack might be useful for this problem.”
Code Quality and Clean Coding Practices
Writing clean, maintainable code is a crucial skill that interviewers evaluate. Here are practices to improve your code quality during interviews:
Meaningful Variable and Function Names
Use descriptive names that convey the purpose of variables and functions. This makes your code self documenting and easier to follow.
Instead of:
int a = arr.length;
for (int i = 0; i < a; i++) {
// ...
}
Write:
int arrayLength = array.length;
for (int index = 0; index < arrayLength; index++) {
// ...
}
Consistent Formatting
Maintain consistent indentation, spacing, and brace placement. This improves readability and shows attention to detail.
Modular Design
Break down complex functions into smaller, focused helper functions. This improves readability and demonstrates your ability to design modular code.
public List<List<Integer>> permute(int[] nums) {
List<List<Integer>> result = new ArrayList<>();
generatePermutations(nums, new boolean[nums.length], new ArrayList<>(), result);
return result;
}
private void generatePermutations(int[] nums, boolean[] used,
List<Integer> current,
List<List<Integer>> result) {
// Implementation details
}
Add Comments Judiciously
Use comments to explain complex logic or non obvious decisions, but don’t over comment. Well written code should be largely self explanatory.
// Use a min heap to efficiently track k largest elements
PriorityQueue<Integer> minHeap = new PriorityQueue<>();
Handle Edge Cases Gracefully
Include input validation and handle edge cases explicitly. This demonstrates thoroughness and attention to robustness.
public int findKthLargest(int[] nums, int k) {
// Validate inputs
if (nums == null || nums.length == 0) {
throw new IllegalArgumentException("Input array cannot be null or empty");
}
if (k <= 0 || k > nums.length) {
throw new IllegalArgumentException("k must be between 1 and array length");
}
// Implementation continues...
}
Use Appropriate Data Structures
Choose the most appropriate data structures for your task. This demonstrates your understanding of their strengths and weaknesses.
// Use HashMap for O(1) lookups when we need to check if an element exists
Map<Integer, Integer> frequencyMap = new HashMap<>();
// Use TreeMap when we need keys to be sorted
Map<Integer, Integer> sortedMap = new TreeMap<>();
Testing and Edge Cases
Thorough testing distinguishes exceptional candidates from average ones. Here’s how to approach testing in a technical interview:
Identify Edge Cases
Systematically consider boundary conditions and special inputs.
Common edge cases include:
- Empty collections (empty arrays, strings, etc.)
- Collections with a single element
- Null inputs
- Minimum and maximum possible values
- Duplicate values
- Sorted or reverse sorted inputs
Test Driven Development
Consider outlining test cases before implementing your solution. This ensures your implementation addresses all requirements.
// Test cases for findKthLargest:
// 1. Normal case: [3,2,1,5,6,4], k=2 → Expected: 5
// 2. Single element: [1], k=1 → Expected: 1
// 3. Duplicates: [3,2,3,1,2,4,5,5], k=4 → Expected: 3
// 4. All identical: [1,1,1], k=2 → Expected: 1
Trace Through Code
Manually trace through your code with test cases, simulating execution step by step. This helps catch logical errors before running the code.
“Let me trace through with the example [3,2,1], k=2:”
- Initialize minHeap = []
- Process 3: Add to heap → [3]
- Process 2: Add to heap → [2,3] (min heap property: smallest at root)
- Process 1: 1 < 2, so don't add → [2,3]
- Return heap root: 2
Test After Implementation
After implementing your solution, run through test cases again to verify correctness.
Regression Testing
If you modify your solution, retest previously passing cases to ensure you haven’t introduced regressions.
Optimization and Trade offs
Discussing optimizations and trade offs demonstrates depth of understanding and critical thinking.
Time and Space Complexity Analysis
Analyze the time and space complexity of your solution using Big O notation. Be precise about the factors that influence complexity.
“The time complexity is O(n log k) because we perform n heap operations, each taking O(log k) time. The space complexity is O(k) for the heap storage.”
Identify Bottlenecks
Pinpoint the inefficient parts of your algorithm and suggest targeted optimizations.
“The bottleneck in this solution is the repeated linear search to find the minimum element. We could optimize this by using a heap data structure to find the minimum in O(log n) time instead of O(n).”
Discuss Trade offs
Explicitly discuss the trade offs between different approaches.
“We could use quickselect for O(n) average time complexity, but it has O(n²) worst case and is more complex to implement. The heap approach offers O(n log k) time complexity with simpler implementation and more predictable performance.”
Consider Practical Factors
Discuss practical considerations beyond theoretical complexity.
“While a hash table gives us O(1) lookups theoretically, the high load factor might lead to collisions and degrade performance. Additionally, the memory overhead might be significant for small inputs.”
Optimize for the Common Case
Prioritize optimizations that benefit the most common usage patterns.
“Since read operations will be much more frequent than writes in this scenario, I’m optimizing the data structure for fast reads even if it makes writes slightly more expensive.”
Real Examples: Before and After
Let’s examine before and after examples of interview responses to see how applying these principles can transform your performance.
Example 1: Two Sum Problem
Problem: Given an array of integers and a target sum, return indices of two numbers that add up to the target.
Before: Suboptimal Approach
// Immediately starts coding
public int[] twoSum(int[] nums, int target) {
for (int i = 0; i < nums.length; i++) {
for (int j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] == target) {
return new int[] {i, j};
}
}
}
return null;
}
After: Improved Approach
“Let me make sure I understand the problem: Given an array of integers and a target sum, I need to find the indices of two elements that sum to the target. Let’s work through an example: if the array is [2, 7, 11, 15] and the target is 9, the output should be [0, 1] because 2 + 7 = 9.”
“I can think of two approaches:
- Brute force: Check all pairs of elements with nested loops. This would be O(n²) time and O(1) space.
- Hash map approach: Use a hash map to store elements we’ve seen and their indices. This would be O(n) time and O(n) space.
Let’s go with the hash map approach since it’s more efficient in terms of time complexity.”
public int[] twoSum(int[] nums, int target) {
// Edge case check
if (nums == null || nums.length < 2) {
return new int[0]; // or throw exception
}
// Map to store values we've seen and their indices
Map<Integer, Integer> numToIndex = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
// If we've seen the complement, we found our pair
if (numToIndex.containsKey(complement)) {
return new int[] {numToIndex.get(complement), i};
}
// Otherwise, store current number and index
numToIndex.put(nums[i], i);
}
// No solution found
return new int[0]; // or throw exception
}
“Let me trace through this with the example [2, 7, 11, 15], target = 9:
- Initialize empty map
- i=0: complement = 9-2 = 7, not in map, add {2:0} to map
- i=1: complement = 9-7 = 2, found in map with index 0, return [0,1]
The time complexity is O(n) as we traverse the array once with O(1) hash map operations. The space complexity is O(n) for storing elements in the hash map.”
Example 2: Validate Binary Search Tree
Problem: Determine if a binary tree is a valid binary search tree (BST).
Before: Suboptimal Approach
public boolean isValidBST(TreeNode root) {
if (root == null) return true;
if (root.left != null && root.left.val >= root.val) return false;
if (root.right != null && root.right.val <= root.val) return false;
return isValidBST(root.left) && isValidBST(root.right);
}
After: Improved Approach
“A valid BST requires that all nodes in the left subtree have values less than the node’s value, and all nodes in the right subtree have values greater than the node’s value. This applies recursively to all subtrees.”
“The solution I initially considered just checks if each node’s direct children satisfy the BST property, but this is incorrect. For example, a grandchild on the right might be smaller than the grandparent, violating the BST property.”
“Instead, we need to track valid ranges for each node. As we traverse down the left subtree, the upper bound becomes the parent’s value. As we traverse down the right subtree, the lower bound becomes the parent’s value.”
public boolean isValidBST(TreeNode root) {
// Call helper function with initial bounds
return isValidBSTHelper(root, null, null);
}
private boolean isValidBSTHelper(TreeNode node, Integer lowerBound, Integer upperBound) {
// Empty trees are valid BSTs
if (node == null) {
return true;
}
// Check if current node's value is within bounds
if ((lowerBound != null && node.val <= lowerBound) ||
(upperBound != null && node.val >= upperBound)) {
return false;
}
// Recursively check left and right subtrees with updated bounds
return isValidBSTHelper(node.left, lowerBound, node.val) &&
isValidBSTHelper(node.right, node.val, upperBound);
}
“Let me trace through a simple example tree:
5 / \ 3 7 / \ 1 6
- Root 5: bounds = (null, null) → valid
- Left child 3: bounds = (null, 5) → valid
- Right child 7: bounds = (5, null) → valid
- Left grandchild 1: bounds = (null, 3) → valid
- Right grandchild 6: bounds = (3, 5) → invalid because 6 > 5
This tree is not a valid BST because 6 violates the upper bound inherited from the grandparent.”
“The time complexity is O(n) where n is the number of nodes, as we visit each node exactly once. The space complexity is O(h) where h is the height of the tree, due to the recursion stack.”
Practice Strategies That Work
Effective practice is key to mastering technical interviews. Here are strategies that yield the best results:
Mock Interviews
Practice with a real person who can provide feedback on your communication and approach. Use platforms like Pramp, interviewing.io, or practice with peers.
Verbalize Your Thought Process
Even when practicing alone, speak your thoughts aloud. This builds the habit of clear communication and helps identify gaps in your reasoning.
Time Your Practice Sessions
Set a timer for 45 minutes when practicing problems to simulate real interview conditions and build time management skills.
Record and Review
Record your mock interviews and review them to identify areas for improvement in your communication and problem solving approach.
Implement the Full Process
Practice the entire interview process for each problem: clarification, examples, multiple approaches, implementation, testing, and optimization.
Focus on Weak Areas
Identify patterns in problems you struggle with and focus your practice on those areas. Use spaced repetition to reinforce learning.
Review Good Solutions
After solving a problem, study well written solutions to learn different approaches and coding styles.
Diversify Problem Types
Practice a wide range of problem types to build versatility. Don’t just focus on the problems you find enjoyable.
Conclusion
Technical interviews at top tech companies evaluate much more than your ability to produce working code. They assess your problem solving process, communication skills, attention to detail, and technical competence holistically.
By adopting a structured approach that includes thorough problem analysis, clear communication, clean code implementation, comprehensive testing, and thoughtful optimization discussions, you can significantly improve your interview performance.
Remember that the journey to becoming an excellent technical interviewer is iterative. Each practice session and interview, whether successful or not, provides valuable feedback to refine your approach.
The next time you prepare for a technical interview, focus not just on getting the right answer, but on demonstrating the thought process and communication skills that interviewers are truly looking for. This holistic approach will set you apart from candidates who focus solely on solving problems without showcasing their broader software engineering capabilities