Why Your Software Architecture Isn’t Handling Scale: A Comprehensive Guide
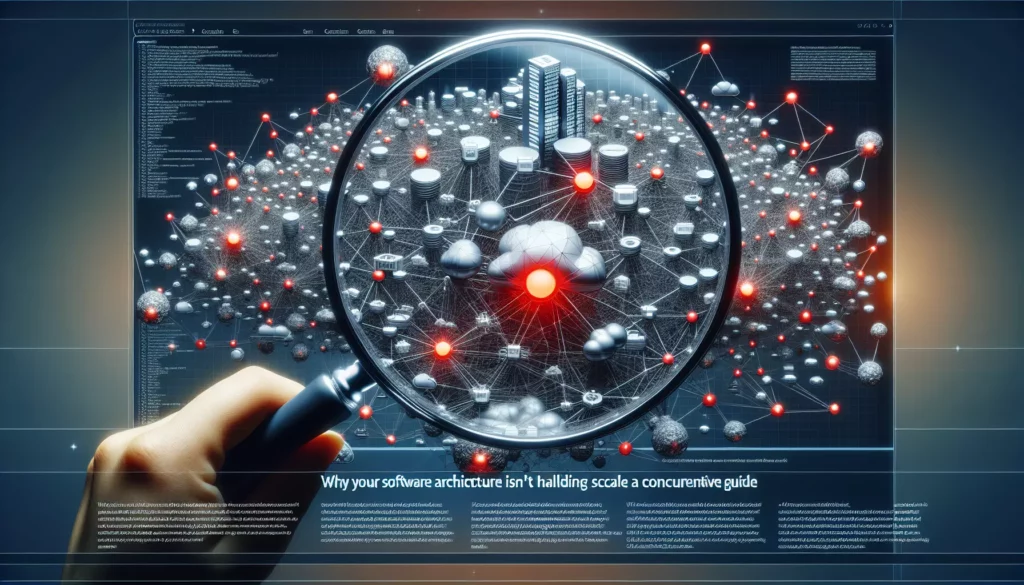
In the fast-paced world of software development, building applications that can handle growth is essential. Yet, many organizations find their systems buckling under increased load, experiencing slowdowns, outages, or complete failures as user numbers climb. This isn’t just frustrating—it can be catastrophic for business.
At AlgoCademy, we’ve helped countless developers transition from writing code that works to designing systems that scale. In this comprehensive guide, we’ll explore why your current architecture might be struggling with scale and provide actionable strategies to transform your systems into robust, scalable solutions capable of handling growth.
Table of Contents
- Understanding Scale: What It Really Means
- Common Architectural Pitfalls That Limit Scalability
- The Monolithic Trap: When Your Architecture Becomes Too Rigid
- Database Bottlenecks: Why Your Data Layer Is Holding You Back
- The Stateful Services Problem: Hidden Scalability Killers
- Tight Coupling: The Enemy of Flexible Scaling
- Missing Architectural Patterns for Scalability
- Monitoring and Observability Gaps
- Solutions: Transforming Your Architecture for Scale
- Real-World Case Studies: Before and After
- Implementation Path: How to Evolve Without Disruption
- Conclusion: Building for the Future
Understanding Scale: What It Really Means
Before diving into what’s wrong with your architecture, let’s clarify what we mean by “scale.” Scale isn’t just about handling more users—it encompasses three distinct dimensions:
Load Scale
This refers to the ability to handle increasing volumes of requests, users, or transactions. When most people talk about scaling, they’re referring to load scale. A system with good load scalability maintains consistent performance as demand increases.
Data Scale
As your application grows, so does your data. Data scale refers to how well your system handles increasing volumes of data without degradation in performance. Many architectures that handle load scale well fall apart when databases grow beyond certain thresholds.
Geographic Scale
Modern applications often need to serve users across different regions and countries. Geographic scale involves delivering consistent performance to users regardless of their location relative to your infrastructure.
A truly scalable architecture addresses all three dimensions. Many systems that claim to be “scalable” only consider one aspect, creating hidden limitations that emerge at the worst possible times.
Common Architectural Pitfalls That Limit Scalability
Let’s examine the most common architectural issues that prevent systems from scaling effectively:
1. Designing for Current Needs Only
One of the most pervasive issues is building systems that perfectly fit current requirements but leave no room for growth. This “just enough” approach leads to architectures that work flawlessly until they suddenly don’t.
Consider a startup that builds a simple monolithic application handling 100 transactions per minute. The system works perfectly until they get featured in the media, and suddenly they’re facing 1,000 transactions per minute. Without architectural considerations for scaling, the system crashes precisely when the business opportunity is greatest.
2. Ignoring Infrastructure Limitations
Many developers build applications assuming infinite resources or ideal conditions. They overlook constraints like:
- Network bandwidth limitations
- Connection limits
- I/O bottlenecks
- Memory constraints
- CPU scheduling challenges
These limitations become critical at scale. For example, a system might work perfectly with 100 concurrent connections but fail completely when attempting to handle 10,000 due to operating system connection limits that weren’t considered during design.
3. Overlooking Asynchronous Processing
Synchronous processing creates a domino effect where slowdowns in one component cascade throughout the system. Many architectures fail to implement asynchronous processing patterns, creating systems that cannot efficiently utilize resources under load.
Consider a user registration flow that synchronously:
- Creates a user record
- Sends a verification email
- Generates analytics events
- Updates related systems
If any single operation slows down, the entire registration process suffers, creating a poor user experience and wasting server resources that could be handling other requests.
The Monolithic Trap: When Your Architecture Becomes Too Rigid
Many systems start as monoliths—single, unified codebases handling all functionality. While monoliths offer simplicity in development and deployment, they create significant scaling challenges:
The Scaling All-or-Nothing Problem
With a monolithic architecture, you must scale the entire application even when only one component needs additional resources. This leads to inefficient resource utilization and unnecessary costs.
For example, if your authentication service experiences high load while the rest of your application has minimal traffic, you still need to scale the entire monolith, wasting resources on components that don’t need them.
Deployment Risk Increases with Size
As monoliths grow, deployments become increasingly risky. A small change to one feature requires deploying the entire application, increasing the chance of unexpected side effects and making continuous deployment difficult.
This deployment friction leads to less frequent, larger deployments, which paradoxically increases risk further and makes it harder to respond quickly to performance issues or scaling needs.
Technology Stack Limitations
Monoliths typically commit to a single technology stack. As your application grows, you may find that certain components would benefit from different technologies, but the monolithic architecture makes this difficult or impossible.
For instance, a CPU-intensive image processing component might benefit from a language like Rust or C++, while your web API might be more maintainable in Node.js or Python. A monolith forces you to choose one approach for all functionality.
Code Complexity Growth
As monoliths grow, the codebase becomes increasingly complex. This complexity makes it harder for developers to understand the entire system, leading to unintended consequences when making changes. These “unknown unknowns” often manifest as scaling problems that are difficult to diagnose and fix.
// A simplified example of a monolithic application structure
class MonolithicApplication {
authenticationService = new AuthenticationService();
userService = new UserService();
billingService = new BillingService();
notificationService = new NotificationService();
analyticsService = new AnalyticsService();
handleUserRegistration(userData) {
// All these operations happen in sequence, in the same process
const user = this.authenticationService.createUser(userData);
this.userService.setupUserProfile(user);
this.billingService.createCustomer(user);
this.notificationService.sendWelcomeEmail(user);
this.analyticsService.trackSignup(user);
return user;
}
}
In the example above, a slowdown in any single service affects the entire registration process, and scaling requires scaling everything together.
Database Bottlenecks: Why Your Data Layer Is Holding You Back
Database issues are among the most common scalability bottlenecks. Here’s why your data layer might be preventing your application from scaling:
The Single Database Instance Problem
Many architectures rely on a single database instance to handle all data operations. While this simplifies development, it creates an inevitable bottleneck as scale increases.
A single database instance has finite resources—CPU, memory, disk I/O, and connection capacity. Once any of these resources reaches capacity, performance degrades rapidly for all operations, even if the rest of your architecture could handle more load.
Connection Pool Exhaustion
Each application server typically maintains a pool of database connections. As you add more application servers to handle increased load, you can quickly exceed the maximum connections your database can handle.
// Common connection pool configuration that becomes problematic at scale
const pool = new DatabasePool({
host: 'primary-database',
maxConnections: 100,
minConnections: 10,
idleTimeoutMillis: 30000
});
If you have 10 application servers each with 100 max connections, you’re potentially asking your database to handle 1,000 connections—far beyond what many database systems can efficiently manage.
Lack of Data Partitioning
As data volumes grow, queries become slower and indexes less effective. Without a strategy for partitioning data, operations that were once fast can become prohibitively expensive.
Consider a system that stores all user activity in a single table. With 1,000 users, queries perform well. With 1,000,000 users, the same queries might take seconds or minutes to complete, bringing your entire system to a crawl.
Read/Write Separation Missing
Most applications have asymmetric read/write patterns, typically with reads outnumbering writes by a significant margin. Without separating these concerns, read operations can suffer due to write contention and vice versa.
Architectures that don’t implement read replicas or other read/write separation patterns force all operations through the same path, creating unnecessary contention and limiting scale.
Inefficient Query Patterns
Even well-designed databases can be brought to their knees by inefficient queries. Common issues include:
- N+1 query problems (executing N additional queries to fetch related data)
- Missing indexes for common query patterns
- Retrieving more data than needed
- Improper use of transactions
// Example of the N+1 query problem
// First query fetches all posts
const posts = await db.query("SELECT * FROM posts LIMIT 100");
// Then for EACH post, we query for the author (100 separate queries!)
for (const post of posts) {
const author = await db.query("SELECT * FROM users WHERE id = ?", [post.author_id]);
post.author = author;
}
As your user base grows, these inefficiencies compound, turning minor performance issues into major bottlenecks.
The Stateful Services Problem: Hidden Scalability Killers
Stateful services store session data, user context, or processing state within the application instance itself. While this approach simplifies development, it creates significant scaling limitations:
Session Affinity Requirements
When state is stored in memory on a specific server, users must be routed back to the same server for subsequent requests (session affinity or “sticky sessions”). This creates several problems:
- Load balancing becomes less effective, as traffic can’t be evenly distributed
- Server failures affect specific users more severely
- Scaling becomes more complex and less efficient
// Problematic in-memory session storage
const sessions = {};
app.post('/login', (req, res) => {
const user = authenticateUser(req.body);
const sessionId = generateSessionId();
// Store session in memory on this specific server
sessions[sessionId] = { user, loginTime: Date.now() };
res.cookie('sessionId', sessionId);
res.redirect('/dashboard');
});
If this server goes down or if the user is routed to a different server, their session data is lost, forcing them to log in again.
Horizontal Scaling Limitations
The true power of cloud computing comes from the ability to horizontally scale—adding more instances of your application to handle increased load. Stateful services make this difficult because each new instance needs to somehow acquire the state from other instances or start with no state at all.
Deployment Complications
Stateful services complicate deployments. You can’t simply replace a running instance with a new version without considering how to transfer or preserve the state, leading to more complex deployment processes and potential downtime.
The Shared-Nothing Alternative
Scalable architectures often adopt a “shared-nothing” approach where application instances are stateless and rely on external systems (like Redis, Memcached, or distributed databases) to store state. This allows any instance to handle any request, making horizontal scaling straightforward.
// More scalable external session storage
const redisClient = createRedisClient();
app.post('/login', async (req, res) => {
const user = authenticateUser(req.body);
const sessionId = generateSessionId();
// Store session in Redis where any server can access it
await redisClient.set(`session:${sessionId}`, JSON.stringify({
user,
loginTime: Date.now()
}), 'EX', 3600); // Expire after 1 hour
res.cookie('sessionId', sessionId);
res.redirect('/dashboard');
});
With this approach, users can be routed to any server, and the system remains resilient to individual server failures.
Tight Coupling: The Enemy of Flexible Scaling
Tightly coupled systems create dependencies between components that should be independent. This coupling manifests in several ways that inhibit scaling:
Direct Service-to-Service Communication
When services directly call each other’s APIs, you create a web of dependencies that makes it difficult to scale individual components. If Service A calls Service B directly, and Service B experiences high load, Service A will also suffer.
// Tightly coupled direct service call
class OrderService {
async createOrder(orderData) {
const order = await this.saveOrder(orderData);
// Direct synchronous call to another service
const paymentResult = await paymentService.processPayment(order.id, orderData.paymentDetails);
if (paymentResult.success) {
await this.updateOrderStatus(order.id, 'PAID');
// Direct call to yet another service
await inventoryService.reserveItems(order.items);
await notificationService.sendOrderConfirmation(order);
}
return order;
}
}
In this example, if the payment service or inventory service slows down, the entire order creation process is affected.
Shared Databases
When multiple services share the same database, they become coupled through the data layer. Changes to database schema for one service can break other services, and database performance issues affect all services equally.
This pattern often emerges as organizations attempt to break monoliths into services without properly separating their data concerns.
Synchronous Processing Chains
Long chains of synchronous operations create systems where the overall performance is determined by the slowest component. This “convoy effect” means that scaling is limited by the least scalable part of your system.
The Decoupling Solution
Scalable architectures use patterns that decouple components:
- Event-driven architecture: Services communicate through events rather than direct calls
- Message queues: Asynchronous communication that buffers between services
- API gateways: Centralized entry points that route requests and handle service discovery
- Database per service: Each service owns its data, eliminating shared database coupling
// Decoupled event-driven approach
class OrderService {
async createOrder(orderData) {
const order = await this.saveOrder(orderData);
// Publish an event for other services to consume
await eventBus.publish('order.created', {
orderId: order.id,
items: order.items,
paymentDetails: orderData.paymentDetails
});
return order;
}
}
// PaymentService subscribes to events
eventBus.subscribe('order.created', async (event) => {
const paymentResult = await processPayment(event.orderId, event.paymentDetails);
if (paymentResult.success) {
await eventBus.publish('payment.succeeded', {
orderId: event.orderId
});
}
});
// OrderService reacts to payment events
eventBus.subscribe('payment.succeeded', async (event) => {
await updateOrderStatus(event.orderId, 'PAID');
});
This decoupled approach allows each service to scale independently and remain resilient to issues in other services.
Missing Architectural Patterns for Scalability
Many architectures struggle with scale because they don’t incorporate patterns specifically designed to address scaling challenges. Here are key patterns that might be missing from your architecture:
Caching Strategies
Effective caching reduces load on your backend systems and improves response times. Many architectures implement basic caching but miss opportunities for more sophisticated approaches:
- Multi-level caching: Implementing caches at different layers (client, CDN, API gateway, service, database)
- Cache warming: Proactively populating caches with frequently accessed data
- Cache invalidation strategies: Ensuring caches stay current without unnecessary refreshes
// Basic caching (often insufficient)
async function getUserProfile(userId) {
const cacheKey = `user:${userId}`;
const cachedData = await cache.get(cacheKey);
if (cachedData) {
return JSON.parse(cachedData);
}
const userData = await database.getUserById(userId);
await cache.set(cacheKey, JSON.stringify(userData), 'EX', 3600);
return userData;
}
// More sophisticated caching with write-through and TTL variation
async function getUserProfile(userId) {
const cacheKey = `user:${userId}`;
const cachedData = await cache.get(cacheKey);
if (cachedData) {
// Track cache hit for analytics
metrics.increment('cache.hit', { entity: 'user_profile' });
return JSON.parse(cachedData);
}
metrics.increment('cache.miss', { entity: 'user_profile' });
const userData = await database.getUserById(userId);
// Cache with TTL based on user activity level
const ttl = userData.activityLevel === 'high' ? 300 : 3600;
await cache.set(cacheKey, JSON.stringify(userData), 'EX', ttl);
return userData;
}
Circuit Breaker Pattern
Circuit breakers prevent cascading failures by detecting when a service is failing and stopping attempts to call it until it recovers. This pattern is essential for building resilient, scalable systems, yet many architectures omit it.
// Example of a circuit breaker implementation
class CircuitBreaker {
constructor(service, options) {
this.service = service;
this.failureThreshold = options.failureThreshold || 5;
this.resetTimeout = options.resetTimeout || 30000;
this.failureCount = 0;
this.status = 'CLOSED';
this.lastFailureTime = null;
}
async call(method, ...args) {
if (this.status === 'OPEN') {
// Check if circuit should be half-open
if (Date.now() - this.lastFailureTime > this.resetTimeout) {
this.status = 'HALF-OPEN';
} else {
throw new Error('Circuit is OPEN');
}
}
try {
const result = await this.service[method](...args);
if (this.status === 'HALF-OPEN') {
this.status = 'CLOSED';
this.failureCount = 0;
}
return result;
} catch (error) {
this.failureCount++;
this.lastFailureTime = Date.now();
if (this.failureCount >= this.failureThreshold ||
this.status === 'HALF-OPEN') {
this.status = 'OPEN';
}
throw error;
}
}
}
Bulkhead Pattern
The bulkhead pattern isolates elements of an application into pools so that if one fails, the others continue to function. This is similar to how ships are divided into compartments to prevent a single breach from sinking the entire vessel.
For example, you might separate your API handling critical user transactions from the API handling analytics or reporting, ensuring that high load on reporting doesn’t affect core business functions.
Throttling and Rate Limiting
Systems without proper throttling and rate limiting are vulnerable to traffic spikes, whether from legitimate users or malicious attacks. Implementing these controls at various levels protects your system from being overwhelmed.
// Basic rate limiting middleware
function rateLimiter(requestsPerMinute) {
const clients = {};
return (req, res, next) => {
const clientId = req.ip;
const now = Date.now();
// Initialize or clean up old requests
clients[clientId] = clients[clientId] || [];
clients[clientId] = clients[clientId].filter(time => now - time < 60000);
// Check if client has exceeded limit
if (clients[clientId].length >= requestsPerMinute) {
return res.status(429).send('Too Many Requests');
}
// Record this request
clients[clientId].push(now);
next();
};
}
// Apply to routes
app.use('/api', rateLimiter(100)); // 100 requests per minute per IP
CQRS (Command Query Responsibility Segregation)
CQRS separates read and write operations, allowing each to be optimized independently. This pattern is particularly valuable for systems with asymmetric read/write loads, which includes most web applications.
By implementing CQRS, you can scale your read and write paths differently, optimize each for its specific patterns, and even use different data stores for reads vs. writes.
Monitoring and Observability Gaps
You can’t fix what you can’t see. Many architectures struggle with scale because they lack adequate monitoring and observability. Here’s what might be missing:
Incomplete Metrics Collection
Basic monitoring often tracks only high-level metrics like CPU and memory usage. While these are important, they don’t provide the full picture. Missing metrics often include:
- Request latency percentiles (not just averages)
- Queue depths and processing times
- Cache hit/miss ratios
- Database query performance
- Third-party API response times
- Custom business metrics relevant to your domain
Lack of Distributed Tracing
In a distributed system, a single user request might touch dozens of services. Without distributed tracing, it’s nearly impossible to identify which component is causing performance issues.
// Adding distributed tracing to a service
app.get('/api/products/:id', async (req, res) => {
// Create a span for this operation
const span = tracer.startSpan('get_product');
span.setTag('product.id', req.params.id);
try {
// Create child span for database operation
const dbSpan = tracer.startSpan('database_query', { childOf: span });
const product = await database.getProductById(req.params.id);
dbSpan.finish();
if (!product) {
span.setTag('error', true);
span.log({ event: 'product_not_found' });
span.finish();
return res.status(404).send('Product not found');
}
// Create child span for inventory check
const inventorySpan = tracer.startSpan('inventory_check', { childOf: span });
const inventory = await inventoryService.checkStock(product.sku);
inventorySpan.finish();
product.inStock = inventory.available > 0;
span.finish();
res.json(product);
} catch (error) {
span.setTag('error', true);
span.log({ event: 'error', message: error.message });
span.finish();
res.status(500).send('Internal Server Error');
}
});
Insufficient Logging
Many systems implement basic logging but miss crucial information that would help diagnose scaling issues:
- Contextual information like user IDs, request IDs, or session IDs
- Performance metrics within log entries
- Resource utilization information
- Correlation IDs to track requests across services
Missing Real User Monitoring (RUM)
Server-side metrics tell only half the story. Without client-side monitoring, you miss critical information about the actual user experience, including:
- Page load times as experienced by users
- Frontend JavaScript execution time
- Network latency from different geographic regions
- Client-side errors
Alert Fatigue and Poor Alerting Strategy
Many systems generate too many alerts (leading to alert fatigue) or alert on symptoms rather than causes. An effective alerting strategy:
- Focuses on user-impacting issues
- Includes context to help diagnose the problem
- Avoids duplicate alerts for the same root cause
- Provides actionable information
Solutions: Transforming Your Architecture for Scale
Now that we’ve identified common problems, let’s explore solutions to transform your architecture for better scalability:
Decompose Monoliths Strategically
Rather than attempting a complete rewrite, identify components within your monolith that would benefit most from extraction:
- High-load components: Services that experience disproportionate traffic or resource usage
- Independently deployable features: Functionality that changes frequently and would benefit from separate deployment cycles
- Different scaling requirements: Components with unique resource needs (CPU-intensive vs. memory-intensive)
Use the strangler pattern to gradually migrate functionality without a risky “big bang” approach:
// Example of the strangler pattern implementation
class OrderRouter {
shouldUseNewService(orderId) {
// Gradually increase traffic to new service
const percentage = configService.getPercentageForNewOrderService();
return (parseInt(orderId, 16) % 100) < percentage;
}
async processOrder(orderId, orderData) {
if (this.shouldUseNewService(orderId)) {
try {
// Route to new microservice
return await newOrderService.process(orderId, orderData);
} catch (error) {
// Fall back to legacy service if new service fails
logger.error('New order service failed, falling back', { orderId, error });
return await legacyOrderService.process(orderId, orderData);
}
} else {
// Use legacy monolith for most traffic
return await legacyOrderService.process(orderId, orderData);
}
}
}
Implement Database Scaling Strategies
Address database bottlenecks with these approaches:
Read Replicas
Direct read traffic to replicas while reserving the primary database for writes. This can be implemented at the application level or through database proxies like ProxySQL or PgBouncer.
Sharding
Divide your data across multiple database instances based on a partition key (like customer ID, geographic region, or tenant ID). This distributes both the data and the query load.
// Example of a simple sharding router
class UserDatabaseRouter {
constructor(shardCount) {
this.shardCount = shardCount;
this.shardConnections = new Array(shardCount).fill(0).map((_, i) =>
createDatabaseConnection(`user-db-shard-${i}`));
}
getUserShard(userId) {
// Determine shard based on userId
const shardId = this.getShardId(userId);
return this.shardConnections[shardId];
}
getShardId(userId) {
// Simple hash function to determine shard
const hash = userId.split('').reduce((acc, char) =>
acc + char.charCodeAt(0), 0);
return hash % this.shardCount;
}
async getUser(userId) {
const shard = this.getUserShard(userId);
return await shard.query('SELECT * FROM users WHERE id = ?', [userId]);
}
async createUser(userData) {
const userId = generateUserId();
const shard = this.getUserShard(userId);
await shard.query('INSERT INTO users (id, name, email) VALUES (?, ?, ?)',
[userId, userData.name, userData.email]);
return userId;
}
}
Command Query Responsibility Segregation (CQRS)
Separate your read and write models, potentially using different database technologies for each. For example, use a relational database for transactional writes and a document database or search engine for reads.
Adopt Event-Driven Architecture
Event-driven architecture decouples components and enables asynchronous processing:
- Identify synchronous processes that could be made asynchronous
- Implement a reliable message broker (like Kafka, RabbitMQ, or cloud-native solutions)
- Design events to carry sufficient information for processing
- Implement idempotent event handlers to ensure reliability
// Producer service
async function completeOrder(orderId) {
const order = await orderRepository.markAsComplete(orderId);
// Publish event instead of