Why Your Side Projects Never Make It Past the “Hello World” Stage
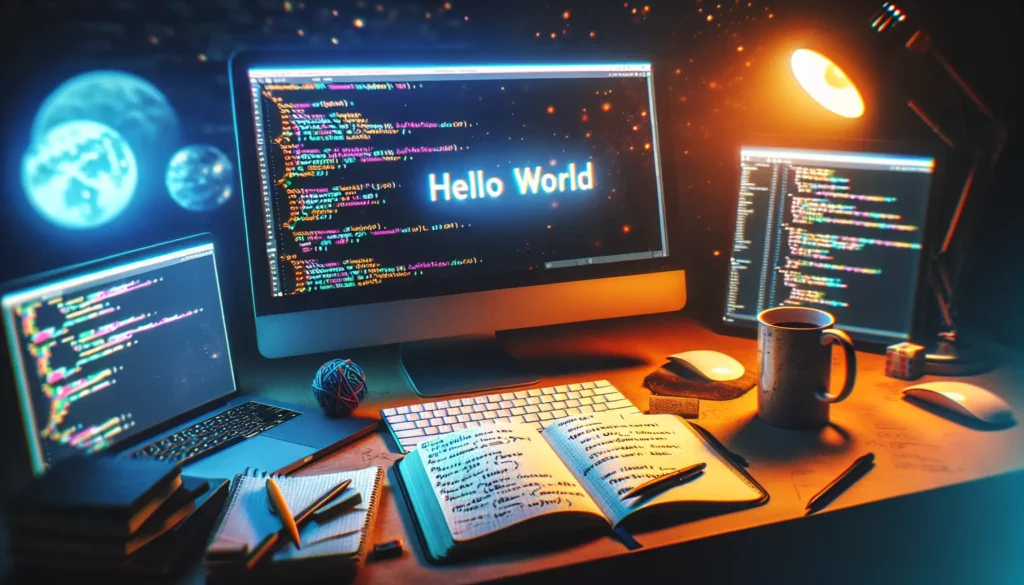
We’ve all been there. A flash of inspiration strikes, and suddenly you’re opening a new code editor, creating a fresh GitHub repository, and typing those familiar words: console.log("Hello, World!")
. The possibilities seem endless, the motivation is high, and this time—yes, this time—your side project will definitely make it to completion.
Fast forward two weeks, and that repository sits untouched, joining the graveyard of other half-started ideas that never made it past the initial commit.
If this sounds painfully familiar, you’re not alone. According to various studies, the vast majority of side projects never reach completion. But why does this happen, especially when we start with such enthusiasm? And more importantly, how can we break this cycle?
In this comprehensive guide, we’ll explore the psychology behind abandoned side projects, identify the common pitfalls that trap even experienced developers, and provide actionable strategies to help you finally push past the “Hello World” stage.
The Psychology Behind Abandoned Side Projects
The Dopamine Rush of Starting Something New
There’s a certain thrill that comes with starting a new project. Your brain releases dopamine—the “feel good” neurotransmitter—when you envision the finished product and take those first steps. This initial rush feels amazing, but it’s also fleeting.
Neuroscience research shows that our brains are wired to seek novelty. The planning phase activates our brain’s reward system, giving us that motivational high. However, once we move into the implementation phase—where the real work happens—that dopamine level drops significantly.
This explains why starting new projects feels so good, while continuing existing ones often feels like a drag. Your brain is literally less excited about continuing than it is about starting fresh.
The Perfectionism Trap
Many developers fall into the perfectionism trap. You envision a flawless application with cutting-edge features, beautiful design, and perfect code. But when reality hits and you realize the gap between your vision and your current capabilities, motivation plummets.
This perfectionism often manifests as:
- Endless research before writing a single line of code
- Constantly rewriting the same functionality to “make it better”
- Paralysis when faced with difficult implementation decisions
- Comparing your beginning to someone else’s middle or end
Remember that even the most impressive projects started with simple, imperfect code that was iteratively improved over time.
The Myth of Motivation
Another psychological factor is the over-reliance on motivation as the primary driver of progress. We tend to wait for inspiration to strike before working on our projects. But motivation is fickle and unreliable—it comes and goes based on numerous factors, many outside our control.
Successful developers understand that consistent progress happens through discipline and habit, not through sporadic bursts of motivation. The most productive programmers often admit they don’t always “feel like coding” but have systems in place to ensure they make progress anyway.
Common Pitfalls That Kill Side Projects
Scope Creep: The Silent Project Killer
Perhaps the most common reason side projects fail is unrealistic scope. What starts as “I’ll build a simple todo app” quickly morphs into “I’ll build a todo app with AI-powered task prioritization, integration with 15 different calendar systems, and a machine learning algorithm that predicts when I’ll actually complete each task.”
Scope creep is particularly dangerous for side projects because, unlike professional projects, there’s no product manager or client to rein in your ambitions. Your imagination is the only limit—and that’s precisely the problem.
Consider this example of how scope creep might evolve:
// Initial idea: Simple todo app
const initialScope = {
features: [
"Add tasks",
"Mark tasks complete",
"Delete tasks"
],
estimatedTimeToComplete: "2 weeks"
};
// After scope creep
const actualScope = {
features: [
"Add tasks",
"Mark tasks complete",
"Delete tasks",
"Categories and tags",
"Priority levels",
"Due dates with notifications",
"Recurring tasks",
"User authentication",
"Cloud sync",
"Mobile app",
"Browser extension",
"API for third-party integration",
"Analytics dashboard",
// ...20 more features
],
estimatedTimeToComplete: "∞"
};
The expanded scope might seem more exciting, but it’s also virtually impossible to complete as a side project.
Technology Overload: Too Many New Tools
Another common pitfall is trying to learn too many new technologies at once. You decide to build a web app, but you also want to learn that hot new JavaScript framework, try out a NoSQL database for the first time, implement GraphQL instead of REST, containerize everything with Docker, and deploy on Kubernetes—all while learning TypeScript.
While learning new technologies is a valid goal for side projects, trying to learn everything at once creates a steep learning curve that can quickly become overwhelming. Each new technology adds complexity and potential points of failure.
The cognitive load becomes too much, and soon you find yourself stuck in tutorial hell rather than making progress on your actual project.
Lack of Clear Goals and Milestones
Many side projects begin with a vague idea rather than a clear vision of what “done” looks like. Without defined milestones and a clear endpoint, it’s easy to lose direction and motivation.
Side projects without clear goals tend to wander aimlessly until they’re eventually abandoned. There’s no sense of progress because there’s no defined destination.
The Isolation Factor
Unlike work projects, side projects often lack social accountability. There’s no team counting on you, no stakeholders waiting for updates, and no deadlines to meet. This isolation removes the external motivation that helps push through the difficult middle stages of a project.
Working in isolation also means you miss out on the collective problem-solving, encouragement, and diverse perspectives that teams provide. When you hit a roadblock alone, it’s easier to give up than to push through.
Strategic Approaches to Finally Finish Your Side Projects
The MVP Mindset: Define Your Minimum Viable Product
One of the most effective strategies for completing side projects is adopting the Minimum Viable Product (MVP) approach. The key is identifying the absolute core functionality that makes your project useful—even if it’s not yet impressive.
Here’s how to apply the MVP mindset:
- Identify the core problem your project solves
- List all possible features, then aggressively cut the list to only what’s essential
- Define “version 1.0” as something you can complete within 2-4 weeks
- Create a separate “future features” list for everything else
For example, if you’re building a recipe app, your MVP might include:
const recipeAppMVP = {
mustHaveFeatures: [
"Add basic recipes (title, ingredients, steps)",
"View a recipe",
"List all recipes"
],
futureFeatures: [
"Search functionality",
"Categories/tags",
"User accounts",
"Sharing capabilities",
"Meal planning",
"Nutrition information",
// and so on
]
};
By defining a limited but functional version 1.0, you create an achievable goal and the satisfaction of completing something useful—which provides motivation to continue to version 1.1 and beyond.
The “One New Thing” Rule
To avoid technology overload, implement the “One New Thing” rule: each project should include a maximum of one new technology or concept you’re trying to learn. Everything else should use tools and languages you’re already comfortable with.
For example:
- If you want to learn React, use a familiar backend technology and database
- If you want to explore MongoDB, pair it with frontend and backend technologies you already know well
- If you’re learning a new programming language, implement a concept or project type you’ve built before
This approach creates a manageable learning curve while still providing the satisfaction of expanding your skills.
Time Boxing and Micro-Commitments
Rather than relying on motivation, create a system of regular, small time commitments to your project. The key is consistency, not duration.
Consider implementing:
- The 20-minute rule: Commit to working on your project for just 20 minutes per day. It’s short enough that you can’t make the “I don’t have time” excuse, but long enough to make incremental progress.
- The “two-day rule”: Never go more than two days without working on your project, even if it’s just for a few minutes.
- Calendar blocking: Schedule specific times for your side project work and treat them with the same respect as your other appointments.
These micro-commitments leverage the psychological principle that starting is often the hardest part. Once you start your 20-minute session, you’ll frequently find yourself continuing beyond the allotted time because you’ve overcome the initial resistance.
Creating Public Accountability
Accountability significantly increases your chances of following through. Consider these approaches:
- Public commitment: Announce your project on social media or your personal blog
- Regular updates: Commit to posting weekly progress, even if it’s small
- Find an accountability partner: Connect with another developer working on their own side project for mutual support
- Join a community: Participate in forums or communities like #100DaysOfCode where progress sharing is encouraged
The social pressure created by public accountability can provide the extra motivation needed to push through challenging phases of your project.
Building in Public
“Building in public” takes the accountability concept further by sharing your journey, including the struggles and failures, not just the successes. This approach has several benefits:
- Creates stronger accountability through regular updates
- Provides opportunities for feedback and suggestions
- Builds an audience interested in your project
- Connects you with potential collaborators
- Documents your learning process for future reference
Platforms like Twitter, Dev.to, or a personal blog are ideal for building in public. The key is consistency and honesty—share both your progress and your challenges.
Practical Implementation: Breaking Down the Process
Week 1: Project Definition and Planning
Start with a clear definition of your project:
- Problem statement: What specific problem does your project solve?
- User stories: Write 3-5 simple user stories that describe the core functionality
- Technology selection: Choose your tech stack, remembering the “One New Thing” rule
- MVP definition: Define exactly what features constitute your version 1.0
- Project structure: Create your repository with a clear README that outlines your goals
Here’s a template for your project README:
# Project Name
## Problem Statement
A clear, concise description of the problem this project solves.
## MVP Features (v1.0)
- Feature 1: Brief description
- Feature 2: Brief description
- Feature 3: Brief description
## Future Features
- Feature A: For later consideration
- Feature B: For later consideration
## Technology Stack
- Frontend: [Your choice]
- Backend: [Your choice]
- Database: [Your choice]
- Deployment: [Your choice]
## Timeline
- Week 1: [Specific goals]
- Week 2: [Specific goals]
- Week 3: [Specific goals]
- Week 4: [Specific goals]
Week 2-3: Implementation with Daily Small Wins
Break your implementation into small, achievable tasks:
- Create a detailed task list where each item can be completed in 1-2 hours maximum
- Prioritize tasks based on dependencies and core functionality
- Set daily goals that are specific and achievable
- Celebrate small wins to maintain momentum
- Document your progress daily, even if just in a personal log
A sample task breakdown might look like:
// Task breakdown for recipe app MVP
const tasks = [
// Setup (Day 1-2)
"Initialize project repository",
"Set up basic project structure",
"Configure development environment",
// Core Functionality (Day 3-7)
"Create data model for recipes",
"Implement 'add recipe' functionality",
"Build recipe display component",
"Create recipe list view",
// Polish (Day 8-10)
"Add basic styling",
"Implement data persistence",
"Add form validation",
// Finalization (Day 11-14)
"Test all functionality",
"Fix bugs",
"Document usage",
"Deploy MVP version"
];
Week 4: Polish, Launch, and Reflect
The final phase focuses on completing your MVP and preparing for what comes next:
- Quality assurance: Test your application thoroughly
- Documentation: Ensure your code is well-documented and includes usage instructions
- Deployment: Get your project online and accessible
- Launch announcement: Share your completed project with your network
- Reflection: Document what you learned, what went well, and what you’d do differently
- Next steps: Plan the next iteration if you’re continuing the project
The reflection step is crucial for improving your process for future projects. Consider questions like:
- What was the most challenging aspect of this project?
- What unexpected obstacles did I encounter?
- Which parts of my process worked well?
- What would I change for my next project?
- Did I achieve my learning goals?
Common Obstacles and How to Overcome Them
The Technical Roadblock
Every project eventually hits technical challenges that seem insurmountable. When this happens:
- Time-box your struggle: Give yourself 60-90 minutes to solve the problem independently
- If still stuck, seek help: Post on Stack Overflow, Discord communities, or Reddit
- Consider simplifying: Is there a less complex approach that would still work?
- Create a temporary workaround: Implement a simpler solution now and revisit the ideal solution later
Remember that professional developers face technical challenges daily—the difference is they have strategies for working through them rather than giving up.
The Motivation Dip
It’s normal for motivation to fluctuate throughout a project. When you hit the inevitable motivation dip:
- Return to your “why”: Remind yourself why you started this project
- Review your progress: Look at how far you’ve come already
- Break down your next steps into even smaller tasks
- Change your environment: Work from a coffee shop, library, or different room
- Connect with community: Share your struggles with other developers
Sometimes, the best approach is to acknowledge the dip but continue with your minimum daily commitment anyway. Motivation often returns once you start making progress again.
The Comparison Trap
In the age of social media, it’s easy to fall into the comparison trap—seeing polished projects from other developers and feeling inadequate. To combat this:
- Remember that you’re seeing their highlight reel, not their struggles and failed attempts
- Focus on your personal growth compared to where you started
- Use others’ work as inspiration, not as a benchmark for your beginner project
- Follow developers who share their process, not just their outcomes
Every developer you admire started with basic projects and gradually improved. Your journey is no different.
The “New Shiny Idea” Syndrome
Sometimes the biggest threat to your current project is your next idea. When a new, exciting project concept emerges:
- Create an “ideas” document where you capture the new concept without abandoning your current project
- Promise yourself you’ll explore it after completing your current MVP
- Evaluate if elements of the new idea could be incorporated into your current project
- Remember that finishing projects is a skill that transfers to all future work
The ability to complete projects is ultimately more valuable than having many started-but-abandoned projects, no matter how innovative the concepts might be.
Case Studies: From “Hello World” to Completion
Case Study 1: The Weekend Project That Grew
Alex, a frontend developer, wanted to build a simple weather app to practice React. Instead of diving straight into code, he:
- Defined a clear MVP: show current weather and 5-day forecast for a searched location
- Set a strict timeline: 2 weekends to complete the MVP
- Used familiar tools (React) and just one new API (OpenWeatherMap)
- Committed to 45 minutes every weekday evening and 3 hours each weekend day
- Shared daily updates on Twitter with the #100DaysOfCode hashtag
By the end of the second weekend, Alex had a functional weather app deployed on Netlify. The public accountability and clear scope were key to his success. Over the following months, he added features incrementally while maintaining the core functionality.
Case Study 2: The Collaborative Approach
Maria struggled with completing solo projects until she tried a collaborative approach:
- She found a coding partner through a local meetup group
- They agreed to build a shared project: a recipe-sharing platform
- They set weekly video calls to review progress and plan next steps
- They used GitHub issues to track tasks and GitHub Projects for project management
- They celebrated milestones together, which reinforced their commitment
The social accountability and shared problem-solving helped both developers push through challenges they might have abandoned if working alone. The project reached completion in 8 weeks and became a portfolio piece for both developers.
Case Study 3: The Incremental Builder
Carlos adopted an incremental approach to build a personal finance tracker:
- He defined four distinct versions, each with increasing functionality
- Version 1.0: Manual expense tracking with basic categories (2 weeks)
- Version 1.1: Data visualization with charts (2 weeks)
- Version 1.2: Budget setting and comparison (2 weeks)
- Version 1.3: Recurring transactions and reminders (2 weeks)
By celebrating the completion of each version and using each as a foundation for the next, Carlos maintained momentum throughout the 8-week project. The key was that each version was fully functional and useful, even if limited compared to the final vision.
Tools and Resources to Keep You on Track
Project Management Tools
Even for personal projects, a project management tool can provide structure and visibility:
- Trello: Simple kanban boards for visualizing workflow
- GitHub Projects: Integrated with your code repository
- Notion: Flexible workspace with databases, kanban, and documentation
- Todoist: Task management with recurring tasks and priorities
Choose a tool that feels lightweight enough that you’ll actually use it, but structured enough to keep you organized.
Time Management Techniques
Effective time management can help maintain consistent progress:
- Pomodoro Technique: 25 minutes of focused work followed by a 5-minute break
- Time blocking: Scheduling specific time slots for project work
- The 2-minute rule: If a task takes less than 2 minutes, do it immediately
- Timeboxing: Allocating a fixed time period to a planned activity
Experiment with different techniques to find what works best for your working style.
Community Resources
Connecting with communities can provide support, accountability, and solutions:
- Dev.to: Developer community with supportive feedback
- #100DaysOfCode: Twitter community for daily coding updates
- Build in Public: Twitter hashtag for sharing ongoing work
- CodeNewbie: Supportive community for those learning to code
- Programming-specific Discord servers: Real-time support and accountability
Active participation in these communities can provide the external motivation that solo projects often lack.
Conclusion: From “Hello World” to “Project Complete”
The journey from “Hello World” to a completed project isn’t just about coding skills—it’s about project management, psychology, and creating systems that support consistent progress. By understanding the common pitfalls and implementing strategies to overcome them, you can break the cycle of abandoned projects.
Remember these key principles:
- Start small: Define a realistic MVP that you can complete in 2-4 weeks
- Create accountability: Whether through public sharing or an accountability partner
- Manage scope rigorously: Be ruthless about what makes it into your first version
- Build consistent habits: Small, daily progress beats sporadic marathon sessions
- Celebrate milestones: Acknowledge and reward your progress along the way
The ability to complete projects is a skill that improves with practice. Each project you finish builds your confidence and capacity for tackling larger challenges. And perhaps most importantly, completing projects transforms you from someone who “is learning to code” to someone who “builds software”—a subtle but crucial distinction in how you view yourself and how others perceive your abilities.
So the next time you type “Hello World,” let it be the beginning of a journey that ends with a completed project you’re proud to share—because the world needs your ideas, not just your intentions.
What side project will you finally complete?