Why Your Service Boundaries Are Causing Data Inconsistencies
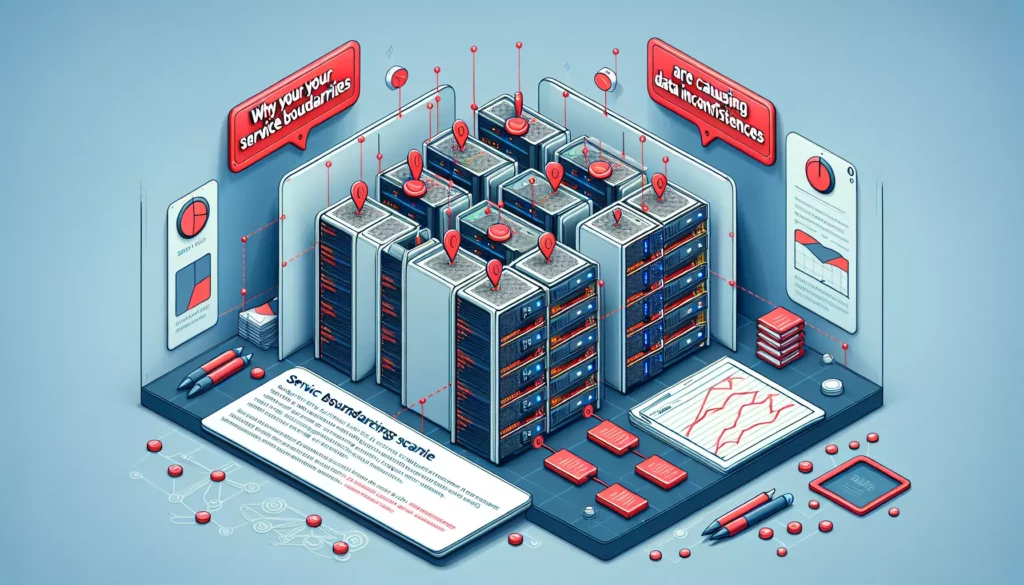
In the world of modern software architecture, microservices have become the go-to approach for building scalable, maintainable systems. Organizations break down monolithic applications into smaller, independent services to improve development velocity and system resilience. However, this architectural shift introduces a critical challenge that many teams underestimate: maintaining data consistency across service boundaries.
At AlgoCademy, we’ve seen firsthand how improper service boundaries can lead to data inconsistencies that impact user experience and system reliability. In this comprehensive guide, we’ll explore why service boundaries often cause data consistency issues and provide practical strategies to address them.
Table of Contents
- Understanding Service Boundaries
- Common Data Consistency Issues
- Why Inconsistencies Occur Across Service Boundaries
- Strategies for Maintaining Data Consistency
- Implementing Eventual Consistency
- Event-Driven Architecture
- The Saga Pattern
- Command Query Responsibility Segregation (CQRS)
- Creating Robust Data Contracts
- Monitoring and Detection
- Conclusion
Understanding Service Boundaries
Service boundaries define where one service ends and another begins. They encapsulate functionality and data, allowing teams to work independently. When designed properly, service boundaries align with business domains and capabilities, creating what Eric Evans calls “bounded contexts” in Domain-Driven Design.
However, determining these boundaries is more art than science. Common approaches include:
- Business capability boundaries: Organizing services around business capabilities (e.g., user management, content delivery, payment processing)
- Data ownership boundaries: Defining services based on the data they own and manage
- Team boundaries: Aligning service boundaries with team structures (Conway’s Law)
When service boundaries are drawn incorrectly, they create artificial seams in data that naturally belongs together. This leads to complex data synchronization requirements and eventual inconsistencies.
Common Data Consistency Issues
Before diving into why these issues occur, let’s examine some common data consistency problems that emerge in microservice architectures:
1. Duplicate Data
Different services often need access to the same data. For example, both a User Service and a Course Progress Service might need user profile information. This leads to data duplication across service boundaries, creating multiple sources of truth.
2. Stale Data
When data is duplicated, it must be synchronized. Any delay in this synchronization results in stale data. For instance, if a user updates their email address in the User Service, the Course Progress Service might continue using the old address until synchronization occurs.
3. Conflicting Updates
When multiple services can modify the same logical data, conflicts arise. If both the User Service and the Notification Service can update a user’s communication preferences, they might make conflicting changes.
4. Failed Distributed Transactions
Operations that span multiple services (like enrolling in a course, which affects both the User Service and the Course Service) can fail partially. If the User Service records enrollment but the Course Service fails to allocate a seat, the system enters an inconsistent state.
5. Referential Integrity Issues
Traditional database constraints don’t work across service boundaries. A Course Service might reference a user ID that no longer exists in the User Service if deletion events aren’t properly propagated.
Why Inconsistencies Occur Across Service Boundaries
Now that we understand the types of inconsistencies, let’s examine why they occur:
1. Distributed Data Ownership
In a microservice architecture, data ownership is distributed. Each service is responsible for its own data store, which creates natural boundaries for data consistency. Within a single service, traditional ACID transactions maintain consistency. However, these guarantees break down when operations span multiple services.
Consider a scenario at AlgoCademy where enrolling a student in a course requires updates to both the User Service (to record enrollment) and the Course Service (to allocate a seat). Without a distributed transaction coordinator, ensuring these updates succeed or fail together becomes challenging.
2. Network Unreliability
Microservices communicate over networks, which are inherently unreliable. Messages can be lost, delayed, or delivered out of order. This network unreliability introduces timing issues that affect data consistency.
For example, when a user completes a coding challenge, the Challenge Service might notify both the Progress Service and the Achievement Service. If the notification to the Achievement Service is delayed, the user might temporarily see inconsistent information about their achievements.
3. Independent Deployment and Versioning
One of the benefits of microservices is independent deployment. However, this means different services might run different versions of code, with different data schemas and business logic.
If the User Service deploys a change to how user names are formatted, but dependent services aren’t updated simultaneously, data inconsistencies will appear until all services are aligned.
4. Improper Domain Boundaries
Perhaps the most fundamental cause of data inconsistencies is improper service boundary definition. When services are designed without considering data cohesion, they artificially split naturally cohesive data.
For instance, if user profile data and learning progress are tightly coupled in the business domain but split between services, maintaining consistency becomes unnecessarily complex.
5. Lack of a Shared Understanding
Different teams developing different services might have varying interpretations of the same business concepts. Without a shared understanding (often formalized as a “ubiquitous language” in Domain-Driven Design), subtle inconsistencies emerge in how data is interpreted and processed.
Strategies for Maintaining Data Consistency
Now that we understand the problem, let’s explore strategies to address data consistency issues across service boundaries:
1. Rethink Your Service Boundaries
The most effective way to reduce data consistency issues is to design proper service boundaries from the start. This means grouping together data that needs to be consistently updated.
Consider the following principles:
- High cohesion: Data that changes together should stay together
- Minimize overlap: Reduce the amount of shared data between services
- Align with business domains: Use Domain-Driven Design to identify natural boundaries
At AlgoCademy, we initially separated our course content and user progress services, only to discover they required frequent synchronized updates. We later redesigned our boundaries to keep this tightly coupled data together, significantly reducing consistency issues.
2. Accept Eventual Consistency
In distributed systems, we often need to accept that consistency will be eventual rather than immediate. This paradigm shift requires both technical adjustments and user experience considerations.
For example, when a user completes a coding challenge, we immediately show their completion in the UI, even though the achievement system might take a few seconds to register the accomplishment. We design the user experience to accommodate this delay gracefully.
3. Use Event-Driven Architecture
Event-driven architecture is a powerful pattern for maintaining data consistency across services. When a service makes a significant state change, it publishes an event that other services can consume to update their local data.
This approach decouples services while providing a mechanism for data synchronization.
4. Implement the Saga Pattern
For operations that span multiple services, the Saga pattern provides a way to maintain consistency without distributed transactions. A saga breaks down a distributed transaction into a sequence of local transactions, each with compensating actions that can be executed if a step fails.
5. Consider CQRS
Command Query Responsibility Segregation (CQRS) separates write operations from read operations. This pattern can help manage consistency by allowing specialized read models that combine data from multiple services.
6. Establish Clear Data Ownership
For each piece of data, establish a single service as the authoritative source of truth. Other services may have copies, but they should be treated as caches that can be refreshed from the authoritative source.
Implementing Eventual Consistency
Eventual consistency acknowledges that data will be inconsistent for short periods but will converge on a consistent state. Here’s how to implement it effectively:
1. Design for Inconsistency
Anticipate that data will sometimes be inconsistent and design your system to handle it gracefully:
- Use version numbers or timestamps to detect and resolve conflicts
- Implement retry mechanisms for failed operations
- Design UIs that can handle and communicate temporary inconsistencies
For example, when a user updates their profile picture, we might show the new picture immediately in the current session, even though other parts of the application might still show the old picture until they refresh their data.
2. Asynchronous Data Synchronization
Implement background processes that periodically reconcile data across services:
// Pseudocode for a data reconciliation job
function reconcileUserData() {
const users = fetchAllUsersFromAuthoritativeSource();
for (const user of users) {
const localUser = fetchUserFromLocalStore(user.id);
if (!localUser || localUser.version < user.version) {
updateLocalUserData(user);
}
}
}
3. Conflict Resolution Strategies
Define clear strategies for resolving conflicts when they occur:
- Last-writer-wins: The most recent update takes precedence
- Merge-based: Combine non-conflicting changes from multiple updates
- Business rule-based: Apply domain-specific rules to resolve conflicts
At AlgoCademy, when conflicting updates to a user’s learning path occur, we apply business rules that prioritize user-initiated changes over automated recommendations.
Event-Driven Architecture
Event-driven architecture is particularly effective for maintaining data consistency across service boundaries. Here’s how to implement it:
1. Identify Key Events
Start by identifying the key events that signal important state changes in your system:
- UserRegistered
- UserProfileUpdated
- CourseCompleted
- ChallengeSubmitted
2. Design Event Schemas
Define clear schemas for your events to ensure they contain all necessary information:
{
"eventType": "UserProfileUpdated",
"version": "1.0",
"timestamp": "2023-10-15T14:30:00Z",
"userId": "12345",
"data": {
"name": "John Doe",
"email": "john.doe@example.com",
"profilePictureUrl": "https://example.com/profiles/12345.jpg",
"updatedFields": ["name", "profilePictureUrl"]
}
}
3. Implement Event Publishing
When a service makes a significant state change, it should publish an event to a message broker like Kafka, RabbitMQ, or AWS SNS:
// Pseudocode for publishing an event when a user profile is updated
function updateUserProfile(userId, profileData) {
// Update the user profile in the database
const result = database.updateUser(userId, profileData);
// Publish an event to notify other services
const event = {
eventType: "UserProfileUpdated",
version: "1.0",
timestamp: new Date().toISOString(),
userId: userId,
data: {
...profileData,
updatedFields: Object.keys(profileData)
}
};
messageQueue.publish("user-events", event);
return result;
}
4. Implement Event Consumers
Services that need to maintain copies of data should subscribe to relevant events and update their local data accordingly:
// Pseudocode for consuming user profile update events
function consumeUserEvents() {
messageQueue.subscribe("user-events", (event) => {
if (event.eventType === "UserProfileUpdated") {
// Update the local copy of user data
const localUser = database.findUser(event.userId);
if (!localUser || new Date(localUser.lastUpdated) < new Date(event.timestamp)) {
database.updateUser(event.userId, event.data);
}
}
});
}
5. Handle Event Delivery Guarantees
Different message brokers provide different delivery guarantees. Consider:
- At-least-once delivery: Events might be delivered multiple times, so consumers should be idempotent
- Exactly-once delivery: More complex but eliminates duplicate processing
- Ordering guarantees: Some systems ensure events for a given entity are processed in order
The Saga Pattern
The Saga pattern helps maintain consistency for operations that span multiple services:
1. Break Down Distributed Transactions
Identify the local transactions that make up a distributed operation. For example, enrolling in a course might involve:
- Verifying user eligibility (User Service)
- Processing payment (Payment Service)
- Allocating a course seat (Course Service)
- Creating enrollment record (Enrollment Service)
2. Define Compensating Actions
For each step, define a compensating action that can undo its effects:
// Saga steps for course enrollment
const enrollmentSaga = {
steps: [
{
action: verifyUserEligibility,
compensation: null // Verification is read-only
},
{
action: processPayment,
compensation: refundPayment
},
{
action: allocateCourseSeat,
compensation: releaseCourseSeat
},
{
action: createEnrollmentRecord,
compensation: deleteEnrollmentRecord
}
]
};
3. Implement Saga Coordination
You can coordinate sagas using either:
- Choreography: Services publish events that trigger the next step or compensation
- Orchestration: A central coordinator manages the execution of saga steps
Here’s a simplified orchestrator implementation:
// Pseudocode for a saga orchestrator
async function executeSaga(saga, context) {
const executedSteps = [];
try {
for (const step of saga.steps) {
const result = await step.action(context);
executedSteps.push(step);
context = { ...context, ...result };
}
return { success: true, context };
} catch (error) {
// Execute compensation actions in reverse order
for (const step of executedSteps.reverse()) {
if (step.compensation) {
await step.compensation(context);
}
}
return { success: false, error };
}
}
Command Query Responsibility Segregation (CQRS)
CQRS separates read and write operations, which can help manage data consistency:
1. Separate Command and Query Models
Define separate models for write operations (commands) and read operations (queries):
- Command models: Optimized for data integrity and business rules
- Query models: Optimized for specific read patterns and can combine data from multiple services
2. Implement Specialized Read Models
Create read models that combine data from multiple services to provide a consistent view for specific use cases:
// Pseudocode for a read model that combines user and course data
class UserDashboardReadModel {
constructor(userRepository, courseRepository, progressRepository) {
this.userRepository = userRepository;
this.courseRepository = courseRepository;
this.progressRepository = progressRepository;
// Subscribe to events to update the read model
eventBus.subscribe("UserProfileUpdated", this.handleUserUpdated.bind(this));
eventBus.subscribe("CourseProgressUpdated", this.handleProgressUpdated.bind(this));
}
async getUserDashboard(userId) {
const user = await this.userRepository.findById(userId);
const enrollments = await this.progressRepository.findEnrollmentsByUserId(userId);
const coursesWithProgress = await Promise.all(
enrollments.map(async (enrollment) => {
const course = await this.courseRepository.findById(enrollment.courseId);
return {
...course,
progress: enrollment.progress,
lastAccessed: enrollment.lastAccessed
};
})
);
return {
user,
courses: coursesWithProgress
};
}
async handleUserUpdated(event) {
// Update the user data in the read model
}
async handleProgressUpdated(event) {
// Update the progress data in the read model
}
}
3. Update Read Models Asynchronously
Read models can be updated asynchronously in response to events, embracing eventual consistency while providing a coherent view of the data.
Creating Robust Data Contracts
Clear data contracts between services help prevent inconsistencies:
1. Define Service Interfaces
Document the APIs that services expose, including:
- Endpoint URLs and methods
- Request and response formats
- Error codes and handling
- Versioning strategy
2. Use Schema Definition Languages
Tools like OpenAPI, Protocol Buffers, or JSON Schema help formalize data contracts:
// Example OpenAPI specification for a user profile endpoint
{
"openapi": "3.0.0",
"info": {
"title": "User Service API",
"version": "1.0.0"
},
"paths": {
"/users/{userId}": {
"get": {
"summary": "Get user profile",
"parameters": [
{
"name": "userId",
"in": "path",
"required": true,
"schema": {
"type": "string"
}
}
],
"responses": {
"200": {
"description": "User profile",
"content": {
"application/json": {
"schema": {
"$ref": "#/components/schemas/UserProfile"
}
}
}
}
}
}
}
},
"components": {
"schemas": {
"UserProfile": {
"type": "object",
"required": ["id", "email", "name"],
"properties": {
"id": {
"type": "string"
},
"email": {
"type": "string",
"format": "email"
},
"name": {
"type": "string"
},
"profilePictureUrl": {
"type": "string",
"format": "uri"
}
}
}
}
}
}
3. Implement Contract Testing
Use contract testing to ensure services adhere to their contracts:
// Example contract test using Pact.js
describe("User Service API Contract", () => {
const provider = new Pact({
consumer: "Dashboard Service",
provider: "User Service"
});
before(() => provider.setup());
after(() => provider.finalize());
describe("GET /users/:userId", () => {
it("returns a user profile when the user exists", async () => {
await provider.addInteraction({
state: "a user with ID 123 exists",
uponReceiving: "a request for user 123",
withRequest: {
method: "GET",
path: "/users/123"
},
willRespondWith: {
status: 200,
headers: { "Content-Type": "application/json" },
body: {
id: "123",
email: Matchers.email,
name: Matchers.string,
profilePictureUrl: Matchers.url
}
}
});
const client = new UserServiceClient(provider.mockService.baseUrl);
const user = await client.getUser("123");
expect(user).to.have.property("id", "123");
expect(user).to.have.property("email");
expect(user).to.have.property("name");
});
});
});
Monitoring and Detection
Even with the best prevention strategies, data inconsistencies will occur. Implement systems to detect and address them:
1. Implement Data Consistency Checks
Run periodic jobs to verify data consistency across services:
// Pseudocode for a consistency check job
async function checkUserEnrollmentConsistency() {
const users = await userService.getAllUsers();
for (const user of users) {
const userEnrollments = await enrollmentService.getEnrollmentsByUserId(user.id);
const userCoursesFromProgress = await progressService.getCoursesByUserId(user.id);
// Check if enrollment records match progress records
const enrollmentCourseIds = new Set(userEnrollments.map(e => e.courseId));
const progressCourseIds = new Set(userCoursesFromProgress.map(p => p.courseId));
const missingInEnrollment = [...progressCourseIds].filter(id => !enrollmentCourseIds.has(id));
const missingInProgress = [...enrollmentCourseIds].filter(id => !progressCourseIds.has(id));
if (missingInEnrollment.length > 0 || missingInProgress.length > 0) {
logInconsistency({
userId: user.id,
missingInEnrollment,
missingInProgress
});
}
}
}
2. Implement Observability
Use observability tools to track events and detect anomalies:
- Distributed tracing to follow requests across services
- Event logging for all data changes
- Metrics to track synchronization delays
3. Automated Reconciliation
When inconsistencies are detected, implement automated processes to reconcile them:
// Pseudocode for data reconciliation
async function reconcileUserEnrollment(userId, courseId) {
const enrollment = await enrollmentService.getEnrollment(userId, courseId);
const progress = await progressService.getProgress(userId, courseId);
if (enrollment && !progress) {
// Create missing progress record
await progressService.initializeProgress(userId, courseId);
logReconciliation("Created missing progress record", { userId, courseId });
} else if (!enrollment && progress) {
// Create missing enrollment record
await enrollmentService.createEnrollment(userId, courseId);
logReconciliation("Created missing enrollment record", { userId, courseId });
}
}
Conclusion
Data consistency across service boundaries is one of the most challenging aspects of microservice architectures. While perfect consistency is often impractical in distributed systems, we can employ various strategies to minimize inconsistencies and their impact:
- Design service boundaries carefully, keeping naturally cohesive data together
- Embrace eventual consistency where appropriate
- Use event-driven architecture to propagate changes
- Implement patterns like Saga and CQRS for complex operations
- Establish clear data contracts between services
- Monitor for inconsistencies and implement automated reconciliation
At AlgoCademy, we’ve learned that data consistency is not just a technical challenge but also a design and organizational one. By aligning our service boundaries with business domains and establishing clear ownership of data, we’ve significantly reduced consistency issues while maintaining the benefits of a microservice architecture.
Remember that perfect consistency, high availability, and partition tolerance cannot all be achieved simultaneously (the CAP theorem). In most microservice architectures, we choose availability and partition tolerance, accepting eventual consistency as a necessary trade-off. With the right patterns and practices, however, we can make this trade-off work effectively for our users and our business.
By addressing service boundary issues thoughtfully, you can build distributed systems that are both flexible and reliable, providing a consistent experience for your users despite the underlying complexity.