Why Your REST API Isn’t Actually RESTful
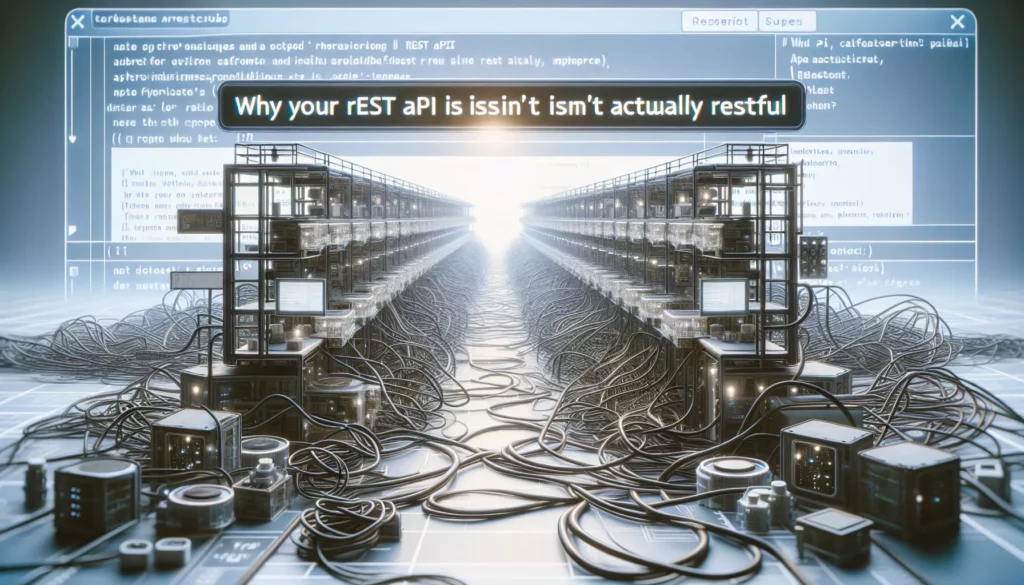
In the world of web development, we often hear terms like “REST API” thrown around casually. Many developers claim to build RESTful services, but upon closer inspection, most of these APIs fall short of truly following REST principles. This disconnect between what we call REST and what REST actually is creates confusion and missed opportunities for building truly scalable and flexible web services.
In this comprehensive guide, we’ll explore what makes a true RESTful API, why most implementations miss the mark, and how you can improve your API design to better align with REST principles.
Table of Contents
- What is REST, Really?
- Common Misconceptions About REST
- The Six Constraints of REST
- The Richardson Maturity Model
- HATEOAS: The Missing Piece
- Common Problems with “REST” APIs
- Building a Better API
- When NOT to Use REST
- Conclusion
What is REST, Really?
REST (Representational State Transfer) is an architectural style for distributed hypermedia systems, introduced by Roy Fielding in his 2000 doctoral dissertation. Fielding, one of the principal authors of the HTTP specification, developed REST as a set of constraints designed to create efficient, reliable, and scalable systems.
The key insight behind REST is that it’s not a protocol, a standard, or a specific technology. Instead, it’s an architectural style defined by a set of constraints that, when followed, create a specific type of application with particular characteristics.
Unfortunately, many developers have reduced REST to simply “an API that uses HTTP methods and returns JSON.” This oversimplification misses the core principles that make REST powerful.
Common Misconceptions About REST
Before diving deeper into what REST is, let’s clear up some common misconceptions:
Misconception 1: REST = HTTP
While REST commonly uses HTTP as its application protocol, REST is not tied to HTTP. REST is an architectural style that can theoretically be implemented over any application protocol that supports its constraints.
Misconception 2: REST = JSON
REST doesn’t specify any particular format for data exchange. While JSON is popular for REST APIs today, REST works with any data format (XML, HTML, plain text, etc.) as long as the media type is properly specified.
Misconception 3: REST = CRUD Operations Over HTTP
Many developers think that mapping HTTP methods (GET, POST, PUT, DELETE) to CRUD operations (Create, Read, Update, Delete) makes an API RESTful. While this is a good practice, it’s only a small part of REST architecture.
Misconception 4: REST APIs Need Versioning in the URL
Proper REST APIs should be evolvable without versioning. Through proper use of media types, hypermedia controls, and other techniques, a true REST API can evolve without breaking existing clients.
The Six Constraints of REST
According to Fielding, a truly RESTful system must satisfy six architectural constraints:
1. Client-Server Architecture
The client and server should be separate from each other and allowed to evolve independently. The server stores and manages the resources while the client requests and displays those resources.
This separation of concerns improves portability across multiple platforms and improves scalability by simplifying server components.
2. Statelessness
Each request from a client to the server must contain all the information needed to understand and process the request. The server cannot use any stored context from previous requests.
This means session state is kept entirely on the client. This constraint improves visibility, reliability, and scalability.
Example of a stateful (non-RESTful) interaction:
// First request
POST /login HTTP/1.1
{
"username": "user123",
"password": "pass456"
}
// Server sets session cookie
// Second request
GET /api/user/profile HTTP/1.1
Cookie: sessionId=abc123
// Server uses session state to determine user
Example of a stateless (RESTful) interaction:
// Each request is self-contained
GET /api/user/profile HTTP/1.1
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
3. Cacheability
Responses must define themselves as cacheable or non-cacheable to prevent clients from reusing stale or inappropriate data in response to further requests.
Well-managed caching partially or completely eliminates some client-server interactions, improving scalability and performance.
Example of proper cache headers:
HTTP/1.1 200 OK
Cache-Control: max-age=3600
ETag: "33a64df551425fcc55e4d42a148795d9f25f89d4"
Content-Type: application/json
{
"data": "This response can be cached for 1 hour"
}
4. Uniform Interface
The uniform interface constraint is fundamental to the design of any RESTful system. It simplifies and decouples the architecture, enabling each part to evolve independently. The uniform interface includes four sub-constraints:
- Resource identification in requests: Individual resources are identified in requests, for example using URIs in web-based REST systems.
- Resource manipulation through representations: When a client holds a representation of a resource, it has enough information to modify or delete the resource.
- Self-descriptive messages: Each message includes enough information to describe how to process it.
- Hypermedia as the engine of application state (HATEOAS): Clients make state transitions only through actions that are dynamically identified within hypermedia by the server.
This constraint is the most often violated in so-called “REST” APIs.
5. Layered System
A client cannot ordinarily tell whether it is connected directly to the end server or to an intermediary along the way. Intermediary servers may improve system scalability by enabling load balancing and providing shared caches.
They may also enforce security policies.
6. Code on Demand (Optional)
Servers can temporarily extend or customize client functionality by transferring executable code. Examples include JavaScript, Java applets, and Flash.
This is the only optional constraint of REST architecture.
The Richardson Maturity Model
Leonard Richardson proposed a model to help categorize the “RESTfulness” of a web service, breaking it down into levels:
Level 0: The Swamp of POX (Plain Old XML)
At this level, you’re essentially using HTTP as a transport protocol for remote interactions, but not taking advantage of any web mechanisms. You typically have a single URL and use POST for all operations.
Example:
POST /api HTTP/1.1
Content-Type: application/json
{
"method": "getUserProfile",
"userId": 123
}
Level 1: Resources
At this level, you’re using multiple URIs to identify different resources, but still using a single HTTP method (typically POST) for all operations.
Example:
POST /api/users/123 HTTP/1.1
Content-Type: application/json
{
"action": "getProfile"
}
Level 2: HTTP Verbs
At this level, you’re using HTTP methods as they were intended. GET for retrieving, POST for creating, PUT for updating, DELETE for removing resources. Most APIs that claim to be RESTful are at this level.
Example:
GET /api/users/123 HTTP/1.1
Accept: application/json
Level 3: Hypermedia Controls (HATEOAS)
At this level, the API returns not just data but also links to related resources and actions that can be performed. This is the level that qualifies as truly RESTful according to Fielding’s definition.
Example:
GET /api/users/123 HTTP/1.1
Accept: application/json
HTTP/1.1 200 OK
Content-Type: application/json
{
"id": 123,
"name": "John Doe",
"email": "john@example.com",
"_links": {
"self": { "href": "/api/users/123" },
"profile": { "href": "/api/users/123/profile" },
"orders": { "href": "/api/users/123/orders" },
"update": {
"href": "/api/users/123",
"method": "PUT"
},
"delete": {
"href": "/api/users/123",
"method": "DELETE"
}
}
}
Most APIs claiming to be RESTful stop at Level 2, missing the crucial hypermedia aspect that makes an API truly RESTful.
HATEOAS: The Missing Piece
HATEOAS (Hypermedia as the Engine of Application State) is perhaps the most distinctive and most frequently overlooked constraint of REST. It’s what separates a truly RESTful API from just an HTTP API.
With HATEOAS, a client interacts with a network application entirely through hypermedia provided dynamically by application servers. The client needs no prior knowledge about how to interact with the application beyond a generic understanding of hypermedia.
Why HATEOAS Matters
HATEOAS provides several significant benefits:
- API Evolution: You can change your API without breaking clients
- Self-discovery: Clients can discover capabilities of your API without external documentation
- Reduced coupling: Clients only need to understand the initial entry point and the hypermedia formats
HATEOAS Example
Let’s look at how HATEOAS might work in practice:
// Initial request to API entry point
GET /api HTTP/1.1
Accept: application/json
HTTP/1.1 200 OK
Content-Type: application/json
{
"version": "1.0",
"_links": {
"users": { "href": "/api/users" },
"products": { "href": "/api/products" },
"orders": { "href": "/api/orders" }
}
}
// Client follows the 'users' link
GET /api/users HTTP/1.1
Accept: application/json
HTTP/1.1 200 OK
Content-Type: application/json
{
"count": 2,
"users": [
{
"id": 123,
"name": "John Doe",
"_links": {
"self": { "href": "/api/users/123" },
"orders": { "href": "/api/users/123/orders" }
}
},
{
"id": 456,
"name": "Jane Smith",
"_links": {
"self": { "href": "/api/users/456" },
"orders": { "href": "/api/users/456/orders" }
}
}
],
"_links": {
"self": { "href": "/api/users" },
"create": {
"href": "/api/users",
"method": "POST",
"encoding": "application/json",
"schema": { "href": "/api/schemas/user-create" }
}
}
}
In this example, the client doesn’t need to know the URL structure in advance. It discovers what it can do through the links provided in each response.
Media Types for Hypermedia
Several standardized formats exist for representing hypermedia controls:
- HAL (Hypertext Application Language): A simple format that gives a consistent and easy way to hyperlink between resources in your API
- JSON-LD: A method of encoding linked data using JSON
- Collection+JSON: A JSON-based read/write hypermedia-type designed to support management and querying of resource collections
- Siren: A hypermedia specification for representing entities
Common Problems with “REST” APIs
Now that we understand what makes an API truly RESTful, let’s examine common problems with APIs that claim to be RESTful but aren’t:
1. Ignoring HATEOAS
As discussed, most APIs stop at Richardson Maturity Level 2, implementing resources and HTTP verbs but not hypermedia controls. This creates rigid APIs that can’t evolve without breaking clients.
2. Using URLs as Command Invocations
Non-RESTful design often uses URLs as command invocations rather than resource identifiers:
// Non-RESTful command-based URL
GET /api/sendEmail?to=user@example.com&subject=Hello
// RESTful resource-oriented approach
POST /api/emails HTTP/1.1
Content-Type: application/json
{
"to": "user@example.com",
"subject": "Hello",
"body": "Hello, world!"
}
3. Ignoring HTTP Status Codes
Many APIs return 200 OK for everything and put error information in the response body, ignoring the rich set of HTTP status codes designed to convey this information.
// Non-RESTful approach
HTTP/1.1 200 OK
Content-Type: application/json
{
"success": false,
"error": "Resource not found",
"error_code": 404
}
// RESTful approach
HTTP/1.1 404 Not Found
Content-Type: application/json
{
"message": "The requested user with ID 123 was not found"
}
4. Ignoring HTTP Methods
Using only GET and POST methods for all operations is a common anti-pattern:
// Non-RESTful approach
POST /api/users/123/delete
// RESTful approach
DELETE /api/users/123
5. Session-based Authentication
Using cookies and sessions for authentication violates the statelessness constraint. Each request should contain all authentication information needed:
// Non-RESTful session-based authentication
POST /api/login HTTP/1.1
Content-Type: application/json
{
"username": "user",
"password": "pass"
}
// Server sets session cookie
HTTP/1.1 200 OK
Set-Cookie: sessionId=abc123; Path=/; HttpOnly
// RESTful token-based authentication
POST /api/tokens HTTP/1.1
Content-Type: application/json
{
"username": "user",
"password": "pass"
}
HTTP/1.1 201 Created
Content-Type: application/json
{
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...",
"expires_at": "2023-12-31T23:59:59Z"
}
6. Misusing HTTP Methods
Using POST for everything or mismatching methods with their intended use:
// Incorrect: Using GET for an operation that changes state
GET /api/users/123/deactivate
// Correct: Using POST or PUT for state changes
POST /api/users/123/actions/deactivate
// Or better:
PUT /api/users/123 HTTP/1.1
Content-Type: application/json
{
"status": "inactive"
}
7. Hard-coding URLs in Clients
When clients hard-code URLs, it creates tight coupling between client and server, making evolution difficult:
// Hard-coded client (problematic)
function getUserOrders(userId) {
return fetch(`https://api.example.com/v1/users/${userId}/orders`);
}
// Hypermedia-aware client (flexible)
async function getUserOrders(userUrl) {
// First, get the user resource
const userResponse = await fetch(userUrl);
const userData = await userResponse.json();
// Find the orders link in the response
const ordersUrl = userData._links.orders.href;
// Follow the link to get orders
return fetch(ordersUrl);
}
Building a Better API
Now that we understand the common issues, let’s look at how to build a more truly RESTful API:
1. Start with a Good Resource Model
Carefully model your domain as resources. Resources should be nouns, not verbs:
- Good:
/users
,/orders
,/products
- Bad:
/getUsers
,/createOrder
,/deleteProduct
2. Use HTTP Methods Properly
Match HTTP methods to their intended semantics:
- GET: Retrieve a resource (safe, idempotent)
- POST: Create a new resource or submit data for processing
- PUT: Update a resource by replacing it entirely (idempotent)
- PATCH: Update a resource partially (not necessarily idempotent)
- DELETE: Remove a resource (idempotent)
- HEAD: Like GET but returns only headers (safe, idempotent)
- OPTIONS: Get information about available communication options (safe)
3. Use Appropriate Status Codes
Use HTTP status codes to communicate outcomes:
- 2xx: Success (200 OK, 201 Created, 204 No Content)
- 3xx: Redirection (301 Moved Permanently, 304 Not Modified)
- 4xx: Client errors (400 Bad Request, 401 Unauthorized, 404 Not Found)
- 5xx: Server errors (500 Internal Server Error, 503 Service Unavailable)
4. Implement HATEOAS
Include hypermedia controls in your responses. You can start with a simple approach:
HTTP/1.1 200 OK
Content-Type: application/json
{
"id": 123,
"name": "John Doe",
"email": "john@example.com",
"_links": {
"self": { "href": "/api/users/123" },
"edit": { "href": "/api/users/123", "method": "PUT" },
"friends": { "href": "/api/users/123/friends" },
"profile-image": { "href": "/api/users/123/profile-image" }
}
}
5. Use Content Negotiation
Support different representations of your resources based on the client’s needs:
// Client requests JSON
GET /api/users/123 HTTP/1.1
Accept: application/json
// Client requests XML
GET /api/users/123 HTTP/1.1
Accept: application/xml
6. Implement Stateless Authentication
Use token-based authentication like JWT (JSON Web Tokens) instead of sessions:
GET /api/users/123 HTTP/1.1
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
7. Enable Caching
Use HTTP caching mechanisms to improve performance:
HTTP/1.1 200 OK
Cache-Control: max-age=3600
ETag: "33a64df551425fcc55e4d42a148795d9f25f89d4"
Last-Modified: Wed, 21 Oct 2023 07:28:00 GMT
Content-Type: application/json
{
"id": 123,
"name": "John Doe"
}
8. Document Your Media Types
If you’re using custom media types, document their structure and semantics:
GET /api/users/123 HTTP/1.1
Accept: application/vnd.example.user+json
HTTP/1.1 200 OK
Content-Type: application/vnd.example.user+json
...
9. Provide an API Entry Point
Give clients a single entry point to discover your API:
GET /api HTTP/1.1
Accept: application/json
HTTP/1.1 200 OK
Content-Type: application/json
{
"version": "1.0",
"_links": {
"users": { "href": "/api/users" },
"products": { "href": "/api/products" },
"documentation": { "href": "/api/docs" }
}
}
When NOT to Use REST
While REST is powerful, it’s not the best solution for every API. Consider alternatives when:
1. You Need Real-time Communication
For real-time applications, consider WebSockets or Server-Sent Events:
// WebSocket example
const socket = new WebSocket('wss://api.example.com/ws');
socket.onmessage = (event) => {
const data = JSON.parse(event.data);
console.log('Received:', data);
};
2. You Need Complex Queries
For complex data requirements, consider GraphQL:
// GraphQL query example
const query = `
query {
user(id: "123") {
name
email
friends {
name
email
}
orders(status: "COMPLETED") {
id
total
items {
product {
name
price
}
quantity
}
}
}
}
`;
3. You Need Remote Procedure Calls
For RPC-style interactions, consider gRPC or JSON-RPC:
// JSON-RPC example
{
"jsonrpc": "2.0",
"method": "calculateTax",
"params": {
"amount": 100,
"category": "food",
"location": "CA"
},
"id": 1
}
4. You Have Limited Resources
For constrained environments (IoT, microcontrollers), consider lightweight protocols like MQTT or CoAP.
Conclusion
While many APIs claim to be RESTful, most only implement a subset of REST principles, missing key constraints like hypermedia and proper resource modeling. True REST goes beyond HTTP methods and JSON responses to create self-describing, evolvable APIs that can change without breaking clients.
Creating a truly RESTful API requires more upfront investment but pays dividends in flexibility, discoverability, and maintainability. That said, REST isn’t always the right choice. Consider your specific requirements and constraints before choosing an architectural style.
Whether you decide to go fully RESTful or opt for a pragmatic approach that borrows some REST principles, understanding the true definition of REST will help you make more informed API design decisions.
Remember, the goal isn’t to achieve perfect REST compliance for its own sake, but to create APIs that serve your users’ needs while remaining flexible, scalable, and maintainable over time.
When designing your next API, consider how many of the REST constraints you’re actually implementing, and whether a different architectural style might better serve your specific use case.