Why Your Refactoring Efforts Aren’t Improving Maintainability
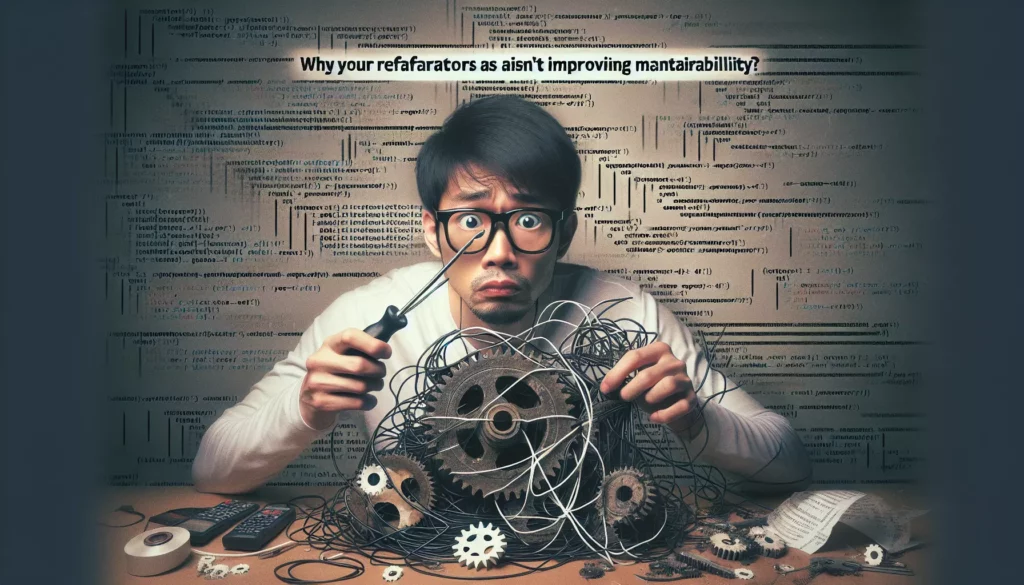
You’ve spent weeks refactoring that legacy codebase. You’ve renamed variables, extracted methods, and even implemented some design patterns. Yet somehow, when the next feature request comes in, it’s still just as painful to implement. What went wrong?
Many developers experience this frustration. They invest significant time in refactoring, only to find that maintainability hasn’t improved as much as expected. In this article, we’ll explore why refactoring efforts sometimes fall short and what you can do to ensure your refactoring truly enhances code maintainability.
Understanding True Maintainability
Before diving into why refactoring efforts might fail, let’s clarify what we mean by maintainability. Code maintainability goes beyond just clean syntax or following best practices. It encompasses:
- Readability: How easily can developers understand the code?
- Modifiability: How easily can the code be changed without introducing bugs?
- Testability: How easily can the code be tested?
- Scalability: How well does the code accommodate growth?
- Documentation: How well is the code’s purpose and function documented?
Many refactoring efforts focus solely on readability or modifiability while neglecting other aspects. This limited focus can explain why your refactoring might not yield the expected benefits.
Common Refactoring Pitfalls
1. Surface-Level Refactoring
One of the most common mistakes is focusing on superficial changes rather than addressing underlying structural issues.
What It Looks Like:
- Renaming variables without reconsidering their purpose
- Formatting code without addressing logical complexity
- Breaking down long methods into smaller ones without reconsidering responsibilities
Consider this example of surface-level refactoring:
// Before refactoring
function calcTot(a, b, c, d) {
return a + b + c + d;
}
// After "refactoring"
function calculateTotal(price, tax, shipping, discount) {
return price + tax + shipping + discount;
}
While the refactored version has better variable names, it hasn’t addressed any structural issues. The function still does the same thing in the same way.
Better Approach:
// Meaningful refactoring
class OrderCalculator {
constructor(order) {
this.order = order;
}
calculateSubtotal() {
return this.order.items.reduce((sum, item) => sum + item.price * item.quantity, 0);
}
calculateTax() {
return this.calculateSubtotal() * this.order.taxRate;
}
calculateShipping() {
// Complex shipping logic here
return this.order.shippingMethod.calculateCost(this.order);
}
calculateDiscount() {
return this.order.promoCode ? this.order.promoCode.apply(this.calculateSubtotal()) : 0;
}
calculateTotal() {
return this.calculateSubtotal() + this.calculateTax() + this.calculateShipping() - this.calculateDiscount();
}
}
This refactoring goes beyond renaming. It introduces a proper object-oriented structure, separates concerns, and makes the code more extensible.
2. Ignoring Business Logic Complexity
Sometimes, developers focus on technical aspects of refactoring while ignoring the complexity of the business logic itself.
What It Looks Like:
- Extracting methods without simplifying convoluted business rules
- Applying design patterns that don’t match the business domain
- Creating abstractions that make business logic harder to trace
For example:
// Before refactoring
function processOrder(order) {
if (order.customer.type === 'premium' && order.total > 1000) {
applyDiscount(order, 0.15);
} else if (order.customer.type === 'premium' || order.total > 1000) {
applyDiscount(order, 0.1);
} else if (order.items.length > 10) {
applyDiscount(order, 0.05);
}
// More complex business rules...
}
// After technical refactoring but still complex business logic
function processOrder(order) {
applyDiscountRules(order);
// Other processing...
}
function applyDiscountRules(order) {
if (isPremiumWithLargeOrder(order)) {
applyDiscount(order, 0.15);
} else if (isPremiumOrLargeOrder(order)) {
applyDiscount(order, 0.1);
} else if (hasManyItems(order)) {
applyDiscount(order, 0.05);
}
}
function isPremiumWithLargeOrder(order) {
return order.customer.type === 'premium' && order.total > 1000;
}
function isPremiumOrLargeOrder(order) {
return order.customer.type === 'premium' || order.total > 1000;
}
function hasManyItems(order) {
return order.items.length > 10;
}
While this refactoring improves readability, it doesn’t address the fundamental complexity of the business rules.
Better Approach:
// Business logic refactoring with strategy pattern
class DiscountStrategy {
apply(order) {
throw new Error("Subclasses must implement apply method");
}
}
class PremiumLargeOrderDiscount extends DiscountStrategy {
apply(order) {
if (order.customer.type === 'premium' && order.total > 1000) {
return 0.15;
}
return 0;
}
}
class PremiumOrLargeOrderDiscount extends DiscountStrategy {
apply(order) {
if (order.customer.type === 'premium' || order.total > 1000) {
return 0.1;
}
return 0;
}
}
class BulkItemDiscount extends DiscountStrategy {
apply(order) {
if (order.items.length > 10) {
return 0.05;
}
return 0;
}
}
class DiscountCalculator {
constructor() {
this.strategies = [
new PremiumLargeOrderDiscount(),
new PremiumOrLargeOrderDiscount(),
new BulkItemDiscount()
];
}
calculateBestDiscount(order) {
return Math.max(...this.strategies.map(strategy => strategy.apply(order)));
}
}
function processOrder(order) {
const discountCalculator = new DiscountCalculator();
const discountRate = discountCalculator.calculateBestDiscount(order);
applyDiscount(order, discountRate);
// Other processing...
}
This approach makes the business rules explicit, testable, and extensible. New discount types can be added without modifying existing code.
3. Refactoring Without Tests
Refactoring without adequate test coverage is like performing surgery without monitoring the patient’s vital signs. You won’t know if you’ve broken something until it’s too late.
What It Looks Like:
- Making significant structural changes without automated tests
- Testing only the happy path after refactoring
- Assuming that if the code compiles, it works correctly
Better Approach:
- Write tests before refactoring to capture current behavior
- Refactor incrementally, running tests after each change
- Add more tests for edge cases and newly discovered behavior
// Example of test-driven refactoring
// 1. First, write tests for existing behavior
test('applies 15% discount for premium customers with orders over $1000', () => {
const order = createOrderWithDetails('premium', 1500, 5);
const originalTotal = order.total;
processOrder(order);
expect(order.total).toEqual(originalTotal * 0.85);
});
// More tests for other scenarios...
// 2. Then refactor with confidence
4. Over-Engineering
Sometimes, developers get carried away with applying design patterns and creating abstractions, resulting in code that’s more complex than necessary.
What It Looks Like:
- Implementing complex design patterns for simple problems
- Creating deep inheritance hierarchies
- Adding layers of abstraction that don’t provide clear benefits
Consider this example of over-engineering:
// Simple original code
function sendEmail(user, subject, body) {
// Code to send email
}
// Over-engineered refactoring
class EmailServiceLocator {
static getEmailService() {
return EmailServiceFactory.createEmailService();
}
}
class EmailServiceFactory {
static createEmailService() {
return new DefaultEmailService();
}
}
class EmailServiceProvider {
provideEmailService() {
return new DefaultEmailService();
}
}
class EmailService {
send(emailRequest) {
throw new Error("Abstract method");
}
}
class DefaultEmailService extends EmailService {
constructor() {
super();
this.emailSender = new EmailSender();
}
send(emailRequest) {
this.emailSender.sendEmail(emailRequest);
}
}
class EmailSender {
sendEmail(emailRequest) {
// Actual code to send email
}
}
class EmailRequest {
constructor(recipient, subject, body) {
this.recipient = recipient;
this.subject = subject;
this.body = body;
}
}
// Usage
function sendEmail(user, subject, body) {
const emailService = EmailServiceLocator.getEmailService();
const emailRequest = new EmailRequest(user, subject, body);
emailService.send(emailRequest);
}
This refactoring introduced numerous classes and indirection without adding much value.
Better Approach:
// Balanced refactoring
class EmailService {
constructor(config = {}) {
this.config = {
defaultSender: 'noreply@example.com',
maxRetries: 3,
...config
};
}
sendEmail(recipient, subject, body, options = {}) {
const emailOptions = {
sender: options.sender || this.config.defaultSender,
retries: options.retries || this.config.maxRetries,
...options
};
// Code to send email with proper error handling
// and retry logic
}
}
// Usage
const emailService = new EmailService();
function sendUserEmail(user, subject, body, options = {}) {
return emailService.sendEmail(user.email, subject, body, options);
}
This version provides flexibility and proper structure without unnecessary complexity.
5. Neglecting Domain Knowledge
Refactoring without understanding the domain can lead to technically clean but functionally inappropriate code.
What It Looks Like:
- Merging concepts that should remain separate in the domain
- Splitting concepts that naturally belong together
- Using technical names instead of domain terminology
For example:
// Before refactoring
function processLoan(customer, amount, duration, interestRate) {
// Logic specific to loans
}
function processDeposit(customer, amount, term, interestRate) {
// Logic specific to deposits
}
// After technically-focused refactoring
function processFinancialProduct(customer, amount, timeframe, interestRate, type) {
if (type === 'loan') {
// Logic specific to loans
} else if (type === 'deposit') {
// Logic specific to deposits
}
}
While this might seem cleaner from a code duplication perspective, it combines two distinct domain concepts (loans and deposits) that have different business rules and lifecycles.
Better Approach:
// Domain-driven refactoring
class FinancialProduct {
constructor(customer, amount, interestRate) {
this.customer = customer;
this.amount = amount;
this.interestRate = interestRate;
}
calculateInterest() {
throw new Error("Subclasses must implement calculateInterest");
}
}
class Loan extends FinancialProduct {
constructor(customer, amount, duration, interestRate) {
super(customer, amount, interestRate);
this.duration = duration;
}
calculateInterest() {
// Loan-specific interest calculation
}
processApproval() {
// Loan approval logic
}
}
class Deposit extends FinancialProduct {
constructor(customer, amount, term, interestRate) {
super(customer, amount, interestRate);
this.term = term;
}
calculateInterest() {
// Deposit-specific interest calculation
}
processMatureAction() {
// Deposit maturity logic
}
}
This refactoring respects the domain boundaries while still leveraging inheritance for shared concepts.
Signs Your Refactoring Isn’t Improving Maintainability
How can you tell if your refactoring efforts are missing the mark? Look for these warning signs:
1. Increasing Complexity Metrics
If complexity metrics like cyclomatic complexity, cognitive complexity, or maintainability index are getting worse, your refactoring might be adding more problems than it’s solving.
Tools like SonarQube, ESLint, or language-specific analyzers can help measure these metrics before and after refactoring.
2. Difficulty Implementing New Features
One of the primary goals of refactoring is to make the code more adaptable to change. If implementing new features still requires changes across multiple files or components, your refactoring hasn’t properly separated concerns.
3. Growing Test Complexity
If your tests become more complex after refactoring, it might indicate that your code’s interfaces or behaviors have become more convoluted.
4. Increased Onboarding Time
If new team members take longer to understand the codebase after refactoring, you may have created abstractions that make the code flow less intuitive or added unnecessary indirection.
5. More Bugs After Refactoring
An increase in bugs following refactoring suggests that either the refactoring broke existing functionality or made the code more prone to errors.
Strategies for Effective Refactoring
Now that we’ve explored common pitfalls, let’s look at strategies that lead to genuinely improved maintainability.
1. Start with a Clear Goal
Before refactoring, identify specific maintainability issues you want to address:
- Are you trying to reduce complexity in a specific module?
- Do you need to improve testability?
- Are you preparing the code for an upcoming feature?
Having a clear goal helps you focus your efforts and measure success.
2. Measure Before and After
Use objective metrics to evaluate the impact of your refactoring:
- Code complexity metrics
- Test coverage
- Build time
- Number of dependencies
- Time to implement standard changes
// Example of using metrics in JavaScript
const complexity = require('complexity-report');
// Before refactoring
const beforeReport = complexity.run('src/before-refactoring.js');
console.log('Before cyclomatic complexity:', beforeReport.aggregate.cyclomatic);
console.log('Before maintainability:', beforeReport.maintainability);
// After refactoring
const afterReport = complexity.run('src/after-refactoring.js');
console.log('After cyclomatic complexity:', afterReport.aggregate.cyclomatic);
console.log('After maintainability:', afterReport.maintainability);
3. Apply Domain-Driven Design Principles
Domain-Driven Design (DDD) provides excellent guidelines for structuring code that aligns with business needs:
- Use ubiquitous language (terminology shared by developers and domain experts)
- Define bounded contexts to separate different parts of the system
- Identify aggregates and entities that represent core domain concepts
4. Follow SOLID Principles
The SOLID principles provide a strong foundation for maintainable code:
- Single Responsibility Principle: A class should have only one reason to change
- Open/Closed Principle: Classes should be open for extension but closed for modification
- Liskov Substitution Principle: Subtypes must be substitutable for their base types
- Interface Segregation Principle: Many specific interfaces are better than one general interface
- Dependency Inversion Principle: Depend on abstractions, not concretions
5. Refactor Incrementally
Instead of massive rewrites, make small, focused changes:
- Identify a specific issue
- Write tests to capture current behavior
- Make the smallest change that addresses the issue
- Run tests to ensure nothing broke
- Commit the change
- Repeat
6. Focus on Reducing Cognitive Load
The primary goal of refactoring should be to make the code easier to understand:
- Use meaningful names that reveal intent
- Keep functions and methods short and focused
- Minimize state and side effects
- Use comments to explain “why” not “what”
7. Consider the Full System Context
Refactoring one component can impact the entire system:
- Consider how changes affect interfaces with other components
- Evaluate impact on performance and resource usage
- Check for compatibility with existing integration points
Real-World Refactoring Success Stories
Case Study 1: Simplifying a Complex API Client
A team was struggling with a complex API client that had grown unwieldy over time. The client had multiple responsibilities: constructing requests, handling authentication, parsing responses, and managing errors.
Before Refactoring:
class ApiClient {
constructor(baseUrl, apiKey) {
this.baseUrl = baseUrl;
this.apiKey = apiKey;
this.timeout = 30000;
// Many more configuration options...
}
async get(endpoint, params) {
// Authentication logic
// Request construction
// Error handling
// Response parsing
// Retry logic
// Logging
// All mixed together in a 200-line method
}
async post(endpoint, data) {
// Similar massive method with duplicate code
}
// More HTTP methods...
// Domain-specific methods
async getUser(userId) {
return this.get(`/users/${userId}`);
}
async updateUser(userId, userData) {
return this.post(`/users/${userId}`, userData);
}
// Dozens more domain-specific methods...
}
Refactoring Approach:
The team applied the Single Responsibility Principle to separate concerns:
// Core HTTP client
class HttpClient {
constructor(config) {
this.config = {
baseUrl: '',
timeout: 30000,
retries: 3,
...config
};
}
async request(method, url, options = {}) {
const requestConfig = this.buildRequestConfig(method, url, options);
return this.executeRequest(requestConfig);
}
buildRequestConfig(method, url, options) {
// Build request configuration
}
async executeRequest(config) {
// Execute request with retry logic
}
get(url, options) {
return this.request('GET', url, options);
}
post(url, data, options) {
return this.request('POST', url, { ...options, data });
}
// Other HTTP methods...
}
// Authentication handler
class AuthHandler {
constructor(credentials) {
this.credentials = credentials;
}
applyAuth(requestConfig) {
// Add authentication to request
}
refreshAuth() {
// Refresh authentication if needed
}
}
// Response processor
class ResponseProcessor {
process(response) {
// Process and validate response
}
handleError(error) {
// Standardize error format
}
}
// Domain-specific API client
class UserApiClient {
constructor(httpClient) {
this.httpClient = httpClient;
this.basePath = '/users';
}
async getUser(userId) {
return this.httpClient.get(`${this.basePath}/${userId}`);
}
async updateUser(userId, userData) {
return this.httpClient.post(`${this.basePath}/${userId}`, userData);
}
// Other user-related methods...
}
// Usage
const httpClient = new HttpClient({
baseUrl: 'https://api.example.com',
// Other config...
});
const authHandler = new AuthHandler({
apiKey: 'your-api-key'
});
httpClient.use(request => authHandler.applyAuth(request));
const responseProcessor = new ResponseProcessor();
httpClient.use(response => responseProcessor.process(response));
const userClient = new UserApiClient(httpClient);
Results:
- Each class had a single responsibility, making it easier to understand and modify
- Testing became simpler as each component could be tested in isolation
- New API resources could be added without modifying existing code
- Error handling became more consistent
- The team could reuse the HTTP client for other APIs
Case Study 2: Untangling Business Logic from UI
A web application had business logic deeply embedded in UI components, making it difficult to maintain and test.
Before Refactoring:
class OrderForm extends Component {
constructor(props) {
super(props);
this.state = {
customer: null,
items: [],
subtotal: 0,
tax: 0,
shipping: 0,
discount: 0,
total: 0,
// More state...
};
}
componentDidMount() {
// Fetch data from API
}
addItem(item) {
const items = [...this.state.items, item];
const subtotal = this.calculateSubtotal(items);
const tax = this.calculateTax(subtotal);
const shipping = this.calculateShipping(items);
const discount = this.calculateDiscount(subtotal, items);
const total = subtotal + tax + shipping - discount;
this.setState({
items,
subtotal,
tax,
shipping,
discount,
total
});
}
calculateSubtotal(items) {
// Complex calculation logic
}
calculateTax(subtotal) {
// Tax calculation with different rates based on location
}
calculateShipping(items) {
// Complex shipping rules
}
calculateDiscount(subtotal, items) {
// Discount logic with multiple rules
}
submitOrder() {
// Validate order
// Submit to API
// Handle response
// Update UI
}
render() {
// Complex UI rendering with conditional logic
}
}
Refactoring Approach:
The team applied the Model-View-ViewModel pattern to separate business logic from UI:
// Domain models
class OrderItem {
constructor(product, quantity) {
this.product = product;
this.quantity = quantity;
}
get price() {
return this.product.price * this.quantity;
}
}
class Order {
constructor(customer, items = []) {
this.customer = customer;
this.items = items;
}
addItem(item) {
this.items.push(item);
}
get subtotal() {
return this.items.reduce((sum, item) => sum + item.price, 0);
}
}
// Business logic services
class TaxCalculator {
calculateTax(order) {
// Tax calculation logic
}
}
class ShippingCalculator {
calculateShipping(order) {
// Shipping calculation logic
}
}
class DiscountCalculator {
calculateDiscount(order) {
// Discount calculation logic
}
}
class OrderService {
constructor() {
this.taxCalculator = new TaxCalculator();
this.shippingCalculator = new ShippingCalculator();
this.discountCalculator = new DiscountCalculator();
}
calculateOrderTotals(order) {
const subtotal = order.subtotal;
const tax = this.taxCalculator.calculateTax(order);
const shipping = this.shippingCalculator.calculateShipping(order);
const discount = this.discountCalculator.calculateDiscount(order);
const total = subtotal + tax + shipping - discount;
return {
subtotal,
tax,
shipping,
discount,
total
};
}
async submitOrder(order) {
// Validation and submission logic
}
}
// View model
class OrderViewModel {
constructor(orderService) {
this.orderService = orderService;
this.order = new Order();
this.totals = {
subtotal: 0,
tax: 0,
shipping: 0,
discount: 0,
total: 0
};
this.loading = false;
this.error = null;
}
addItem(product, quantity) {
const item = new OrderItem(product, quantity);
this.order.addItem(item);
this.updateTotals();
}
updateTotals() {
this.totals = this.orderService.calculateOrderTotals(this.order);
}
async submitOrder() {
this.loading = true;
this.error = null;
try {
await this.orderService.submitOrder(this.order);
this.loading = false;
return true;
} catch (error) {
this.loading = false;
this.error = error;
return false;
}
}
}
// UI Component
class OrderForm extends Component {
constructor(props) {
super(props);
this.orderService = new OrderService();
this.viewModel = new OrderViewModel(this.orderService);
this.state = {
viewModel: this.viewModel,
// UI-specific state
};
}
handleAddItem(product, quantity) {
this.viewModel.addItem(product, quantity);
this.forceUpdate();
}
async handleSubmit() {
const success = await this.viewModel.submitOrder();
if (success) {
// Navigate to confirmation page
}
}
render() {
const { viewModel } = this.state;
// Render UI based on view model state
}
}
Results:
- Business logic became testable independently of the UI
- UI components became simpler and focused on presentation
- Business rules could be modified without touching UI code
- New discount or tax rules could be added without affecting other parts of the system
- The same business logic could be reused in different UI contexts (web, mobile, etc.)
Conclusion
Refactoring that truly improves maintainability goes beyond superficial changes. It requires a deep understanding of the domain, clear goals, proper testing, and adherence to solid design principles.
The next time you embark on a refactoring effort, ask yourself:
- What specific maintainability issues am I trying to solve?
- How will I measure the success of this refactoring?
- Am I addressing the underlying complexity or just moving it around?
- Does this refactoring respect the domain concepts?
- Will this make future changes easier or harder?
By focusing on these questions and avoiding the common pitfalls we’ve discussed, you can ensure that your refactoring efforts genuinely improve maintainability and make your codebase a pleasure to work with.
Remember, the goal of refactoring isn’t just to make code look cleaner—it’s to make the software more adaptable to change, easier to understand, and less prone to bugs. When done right, refactoring is an investment that pays dividends with every new feature or bug fix.