Why Your Programming Solutions Are Harder Than They Need to Be
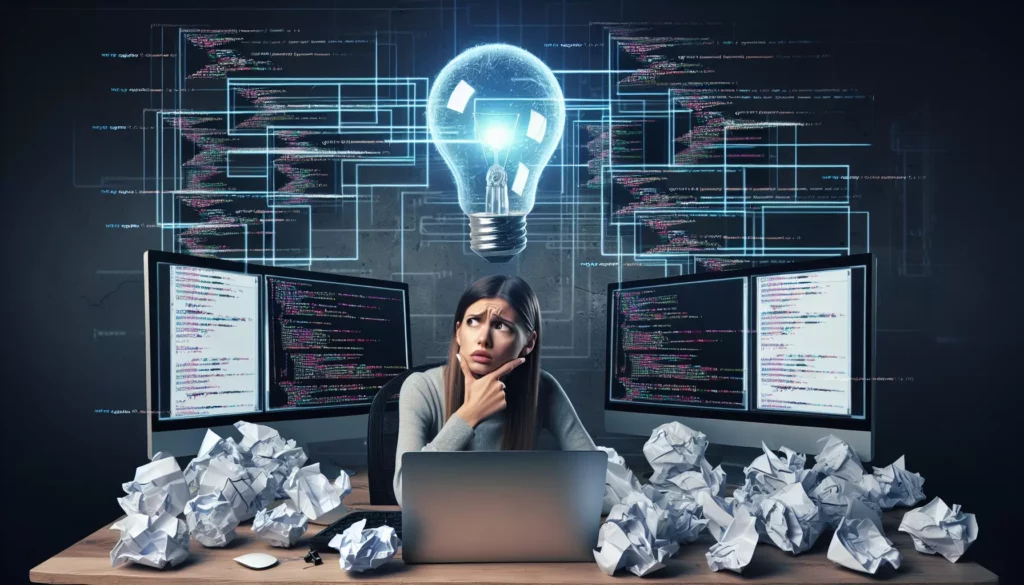
Have you ever spent hours struggling with a coding problem, only to discover there was a much simpler solution? If you’re nodding in agreement, you’re not alone. Many programmers, from beginners to seasoned professionals, tend to overcomplicate their solutions. This phenomenon is so common that it has become a rite of passage in the programming world.
In this article, we’ll explore why we often make programming harder than necessary, identify the common pitfalls that lead to complexity, and provide practical strategies to simplify your approach to problem-solving in code.
The Complexity Trap: Why We Overcomplicate Our Code
Before we dive into solutions, let’s understand why we tend to create overly complex code in the first place.
The Impostor Syndrome Effect
Many programmers suffer from impostor syndrome, a psychological pattern where individuals doubt their accomplishments and have a persistent fear of being exposed as a “fraud.” This mindset can lead developers to believe that a “real programmer” would create something more sophisticated.
Consider this simple task: finding the maximum value in an array. A developer with impostor syndrome might implement a complex sorting algorithm with custom comparators when a simple loop would suffice:
// Overcomplicated approach
function findMax(arr) {
return arr.sort((a, b) => {
// Complex custom comparator
if (typeof a !== typeof b) {
return typeof a === 'number' ? -1 : 1;
}
return b - a;
})[0];
}
// Simple approach
function findMax(arr) {
return Math.max(...arr);
}
The “I Know This Advanced Technique” Syndrome
When we learn a new programming pattern, algorithm, or data structure, we’re eager to apply it, even when it’s not the best tool for the job. This is like using a sledgehammer to hang a picture frame—impressive, but excessive.
For instance, after learning about recursion, you might be tempted to use it for problems that have simpler iterative solutions:
// Recursive approach to sum an array
function sumArray(arr) {
if (arr.length === 0) return 0;
return arr[0] + sumArray(arr.slice(1));
}
// Simpler approach
function sumArray(arr) {
return arr.reduce((sum, num) => sum + num, 0);
}
Premature Optimization
Donald Knuth famously said, “Premature optimization is the root of all evil.” Yet, many programmers spend considerable time optimizing code before they’ve even determined if performance is an issue. This often leads to complex, hard-to-maintain solutions that offer marginal or no real-world benefits.
For example, creating a complex caching mechanism for a function that’s called infrequently with different parameters:
// Overcomplicated caching mechanism
const memoize = (fn) => {
const cache = {};
return (...args) => {
const key = JSON.stringify(args);
if (key in cache) {
return cache[key];
}
const result = fn(...args);
cache[key] = result;
return result;
};
};
const expensiveFunction = memoize((x, y) => {
// A function that's rarely called with the same parameters
return x * y;
});
Overthinking the Future
Anticipating future requirements that may never materialize often leads to overly flexible, complex code. This is sometimes called “architecture astronauting”—building elaborate systems for problems that don’t exist yet.
For instance, creating a plugin system for a simple utility:
// Overcomplicated plugin architecture
class Calculator {
constructor() {
this.plugins = {};
}
registerPlugin(name, implementation) {
this.plugins[name] = implementation;
}
executeOperation(pluginName, ...args) {
if (this.plugins[pluginName]) {
return this.plugins[pluginName](...args);
}
throw new Error(`Plugin ${pluginName} not found`);
}
}
// What you actually needed
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
Common Signs Your Solution Is Too Complex
How do you know if your solution is more complex than it needs to be? Here are some warning signs:
You Can’t Explain It Simply
Albert Einstein supposedly said, “If you can’t explain it simply, you don’t understand it well enough.” If you struggle to explain your code to a colleague in a few sentences, it might be too complex.
Your Solution Is Much Longer Than Others’
After solving a problem, if you compare your solution to others and find yours is significantly longer, it might be a sign that you’ve taken a more complicated approach.
You’re Using Advanced Concepts for Basic Problems
If you’re implementing design patterns, complex data structures, or algorithmic techniques for problems that don’t require them, you might be overengineering.
You Keep Adding Edge Cases
When you find yourself continually adding more conditions and edge cases to your code, it could indicate that your fundamental approach is flawed.
Your Code Has Low Cohesion or High Coupling
If your solution involves many components that are either weakly related (low cohesion) or too interdependent (high coupling), it’s likely more complex than necessary.
Real-World Examples of Overcomplicated Solutions
Let’s look at some common programming problems where developers often create unnecessarily complex solutions:
Example 1: Finding Palindromes
A palindrome is a word, phrase, or sequence that reads the same backward as forward.
// Overcomplicated approach
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let charArray = [];
for (let i = 0; i < str.length; i++) {
charArray.push(str[i]);
}
let reversedArray = [];
for (let i = charArray.length - 1; i >= 0; i--) {
reversedArray.push(charArray[i]);
}
let reversedStr = reversedArray.join('');
return str === reversedStr;
}
// Simpler approach
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
return str === str.split('').reverse().join('');
}
Example 2: Calculating Fibonacci Numbers
The Fibonacci sequence is a series where each number is the sum of the two preceding ones.
// Overcomplicated approach (with unnecessary memoization for small inputs)
function fibonacci(n) {
const memo = {};
function fib(num) {
if (num in memo) return memo[num];
if (num <= 1) return num;
memo[num] = fib(num - 1) + fib(num - 2);
return memo[num];
}
return fib(n);
}
// Simpler approach for small inputs
function fibonacci(n) {
if (n <= 1) return n;
let a = 0, b = 1;
for (let i = 2; i <= n; i++) {
[a, b] = [b, a + b];
}
return b;
}
Example 3: Checking for Prime Numbers
A prime number is a natural number greater than 1 that is not a product of two smaller natural numbers.
// Overcomplicated approach using the Sieve of Eratosthenes for a single number
function isPrime(num) {
if (num <= 1) return false;
if (num <= 3) return true;
const sieve = new Array(num + 1).fill(true);
sieve[0] = sieve[1] = false;
for (let i = 2; i * i <= num; i++) {
if (sieve[i]) {
for (let j = i * i; j <= num; j += i) {
sieve[j] = false;
}
}
}
return sieve[num];
}
// Simpler approach
function isPrime(num) {
if (num <= 1) return false;
if (num <= 3) return true;
if (num % 2 === 0 || num % 3 === 0) return false;
for (let i = 5; i * i <= num; i += 6) {
if (num % i === 0 || num % (i + 2) === 0) return false;
}
return true;
}
Strategies for Simplifying Your Solutions
Now that we understand why we overcomplicate things and can recognize when we’re doing it, let’s explore strategies to simplify our approach to coding problems.
1. Start With the Brute Force Approach
Before optimizing, implement the most straightforward solution that comes to mind. This gives you a working solution and a baseline for comparison. Often, this “naive” approach is sufficient for the problem at hand.
// Start with brute force
function findDuplicates(arr) {
const duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// Then optimize if necessary
function findDuplicates(arr) {
const seen = new Set();
const duplicates = new Set();
for (const item of arr) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return [...duplicates];
}
2. Use Built-in Functions and Libraries
Most programming languages and frameworks provide built-in functions and libraries that are well-tested and optimized. Use them instead of reinventing the wheel.
// Don't reinvent sorting
function sortNumbers(arr) {
// Don't implement bubble sort, quick sort, etc. yourself
return arr.sort((a, b) => a - b);
}
// Don't reinvent date manipulation
function isWeekend(date) {
// Use a library like date-fns instead of complex calculations
const day = date.getDay();
return day === 0 || day === 6;
}
3. Solve the Actual Problem, Not the Imagined One
Focus on the current requirements rather than hypothetical future needs. You can always refactor later if those needs materialize.
// Solving an imagined problem
function calculateTax(amount, rate, country, region, productType, customerType) {
// Complex logic handling international tax rules
// that your application doesn't actually need yet
}
// Solving the actual problem
function calculateTax(amount, rate) {
return amount * rate;
}
4. Write Code for Humans, Not Computers
Prioritize readability and maintainability over clever optimizations. The computer will execute your code either way, but humans need to understand it.
// Clever but hard to read
function isEven(n) {
return !(n & 1);
}
// Clear and readable
function isEven(n) {
return n % 2 === 0;
}
5. Apply the YAGNI Principle
YAGNI stands for “You Aren’t Gonna Need It.” It suggests that you shouldn’t add functionality until it’s necessary. This helps prevent overengineering.
// Violating YAGNI
class UserService {
constructor(database, cache, logger, metrics, notificationSystem) {
this.database = database;
this.cache = cache;
this.logger = logger;
this.metrics = metrics;
this.notificationSystem = notificationSystem;
}
// Only using database in current implementation
getUser(id) {
return this.database.findUserById(id);
}
}
// Following YAGNI
class UserService {
constructor(database) {
this.database = database;
}
getUser(id) {
return this.database.findUserById(id);
}
}
6. Break Down Complex Problems
When faced with a complex problem, break it down into smaller, more manageable parts. Solve each part separately, then combine the solutions.
// Complex problem: Find the median of two sorted arrays
function findMedianSortedArrays(nums1, nums2) {
// Step 1: Merge the arrays
const merged = mergeArrays(nums1, nums2);
// Step 2: Find the median
return findMedian(merged);
}
function mergeArrays(arr1, arr2) {
const result = [];
let i = 0, j = 0;
while (i < arr1.length && j < arr2.length) {
if (arr1[i] < arr2[j]) {
result.push(arr1[i++]);
} else {
result.push(arr2[j++]);
}
}
return result.concat(arr1.slice(i)).concat(arr2.slice(j));
}
function findMedian(arr) {
const mid = Math.floor(arr.length / 2);
return arr.length % 2 === 0
? (arr[mid - 1] + arr[mid]) / 2
: arr[mid];
}
7. Learn From Others’ Solutions
After solving a problem, look at how others approached it. This can help you identify simpler techniques and expand your problem-solving toolkit.
8. Practice Refactoring
Regularly revisit your code and look for opportunities to simplify it. This helps develop your ability to recognize complexity and find more elegant solutions.
// Before refactoring
function getDaysBetweenDates(startDate, endDate) {
const start = new Date(startDate);
const end = new Date(endDate);
const diffTime = Math.abs(end - start);
const diffDays = Math.ceil(diffTime / (1000 * 60 * 60 * 24));
return diffDays;
}
// After refactoring
function getDaysBetweenDates(startDate, endDate) {
const msPerDay = 1000 * 60 * 60 * 24;
return Math.ceil(Math.abs(new Date(endDate) - new Date(startDate)) / msPerDay);
}
Case Study: Technical Interview Problems
Technical interviews are notorious for causing candidates to overcomplicate their solutions due to stress and the desire to impress. Let’s examine a few common interview problems and see how we can simplify our approach.
Case Study 1: Two Sum Problem
Problem: Given an array of integers and a target sum, return the indices of two numbers that add up to the target.
// Overcomplicated approach
function twoSum(nums, target) {
// Sort the array first (unnecessary)
const sorted = [...nums].sort((a, b) => a - b);
// Use binary search (overcomplicated for this problem)
for (let i = 0; i < sorted.length; i++) {
const complement = target - sorted[i];
let left = i + 1;
let right = sorted.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (sorted[mid] === complement) {
// Find original indices (extra work due to sorting)
const index1 = nums.indexOf(sorted[i]);
let index2 = nums.indexOf(sorted[mid]);
if (index1 === index2) {
index2 = nums.indexOf(sorted[mid], index1 + 1);
}
return [index1, index2];
} else if (sorted[mid] < complement) {
left = mid + 1;
} else {
right = mid - 1;
}
}
}
return null;
}
// Simpler approach
function twoSum(nums, target) {
const numMap = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (numMap.has(complement)) {
return [numMap.get(complement), i];
}
numMap.set(nums[i], i);
}
return null;
}
Case Study 2: Valid Parentheses
Problem: Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid (i.e., open brackets must be closed by the same type of brackets and in the correct order).
// Overcomplicated approach
function isValid(s) {
// Create a complex parsing state machine
let state = 'initial';
const states = {
'initial': { '(': 'openParen', '{': 'openBrace', '[': 'openBracket' },
'openParen': { '(': 'openParenParen', '{': 'openParenBrace', '[': 'openParenBracket', ')': 'initial' },
'openBrace': { '(': 'openBraceParen', '{': 'openBraceBrace', '[': 'openBraceBracket', '}': 'initial' },
'openBracket': { '(': 'openBracketParen', '{': 'openBracketBrace', '[': 'openBracketBracket', ']': 'initial' },
// ... many more states
};
for (const char of s) {
if (!states[state] || !states[state][char]) {
return false;
}
state = states[state][char];
}
return state === 'initial';
}
// Simpler approach
function isValid(s) {
const stack = [];
const pairs = {
'(': ')',
'{': '}',
'[': ']'
};
for (const char of s) {
if (pairs[char]) {
// It's an opening bracket
stack.push(char);
} else {
// It's a closing bracket
const last = stack.pop();
if (pairs[last] !== char) {
return false;
}
}
}
return stack.length === 0;
}
Case Study 3: Reverse a Linked List
Problem: Reverse a singly linked list.
// Overcomplicated approach
function reverseList(head) {
// Convert to array, reverse, then back to linked list
if (!head) return null;
// Step 1: Convert to array
const nodes = [];
let current = head;
while (current) {
nodes.push(current);
current = current.next;
}
// Step 2: Reverse the array
nodes.reverse();
// Step 3: Reconnect the nodes
for (let i = 0; i < nodes.length; i++) {
nodes[i].next = i < nodes.length - 1 ? nodes[i + 1] : null;
}
return nodes[0];
}
// Simpler approach
function reverseList(head) {
let prev = null;
let current = head;
while (current) {
const next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev;
}
The Zen of Programming: Embracing Simplicity
In the world of programming, simplicity is not just an aesthetic preference—it's a practical necessity. Simple code is easier to understand, maintain, debug, and extend. It's also less prone to bugs and often performs better than overly complex alternatives.
The journey toward simplicity in programming is ongoing. Even experienced developers regularly catch themselves overcomplicating solutions. The key is to develop an awareness of this tendency and cultivate practices that counteract it.
The Value of Simple Code
Simple code offers numerous benefits:
- Readability: Simple code is easier for others (and your future self) to read and understand.
- Maintainability: When bugs occur or features need to be added, simple code is easier to modify.
- Testability: Simple functions with clear inputs and outputs are easier to test thoroughly.
- Reliability: With fewer moving parts, there are fewer opportunities for things to go wrong.
- Performance: Simple code often performs better because it has less overhead.
The Art of Knowing What to Include
Antoine de Saint-Exupéry once said, "Perfection is achieved, not when there is nothing more to add, but when there is nothing left to take away." This principle applies perfectly to programming. The most elegant solutions are often those that accomplish the task with the minimum necessary code.
Building Simplicity Muscle
Like any skill, writing simple code takes practice. Here are some exercises to help develop this ability:
- Code Golf: Try to solve problems with as few characters as possible (but don't use this approach in production code).
- Refactoring Challenges: Take existing complex code and try to simplify it while maintaining functionality.
- Peer Review: Have colleagues review your code specifically for unnecessary complexity.
- Explain Your Code: Practice explaining your code to someone else. If it's hard to explain, it might be too complex.
Conclusion: The Path to Elegant Solutions
Programming is as much an art as it is a science. While there's no single "right way" to solve a problem, there are approaches that are more elegant, maintainable, and effective than others. By recognizing our tendency to overcomplicate solutions and actively working to simplify our code, we can become more effective programmers and create better software.
Remember, the goal isn't to write the cleverest code—it's to solve problems effectively. Sometimes the most impressive solution is the one that makes others say, "That's it? I thought it would be more complicated."
So the next time you're tackling a programming problem, challenge yourself to find the simplest solution that works. Your future self (and your colleagues) will thank you for it.
Key Takeaways
- Start with the simplest solution that works, then optimize only if necessary.
- Use built-in functions and libraries instead of reinventing the wheel.
- Focus on solving the actual problem at hand, not hypothetical future problems.
- Write code for humans to read, not just for computers to execute.
- Break complex problems into smaller, more manageable parts.
- Learn from others' solutions to expand your problem-solving toolkit.
- Regularly refactor your code to identify and eliminate unnecessary complexity.
- Remember that simplicity leads to code that is more readable, maintainable, and reliable.
By embracing these principles, you'll find that your solutions become not just simpler, but more elegant and effective. And isn't that what great programming is all about?