Why Your Programming Projects Look Nothing Like the Tutorials
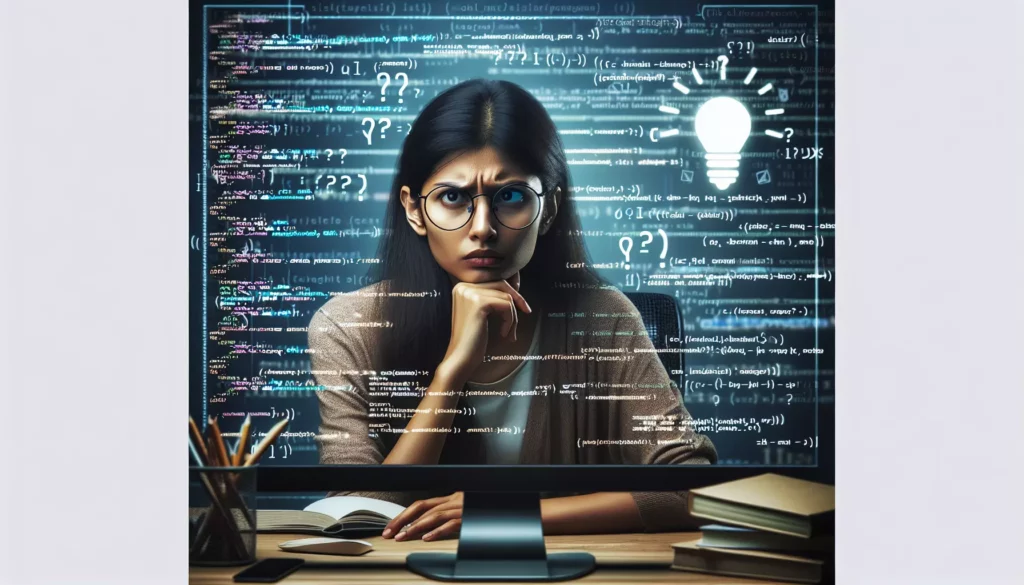
Have you ever followed a coding tutorial, feeling confident and excited about your new skills, only to face a blank screen when starting your own project? The disconnect between tutorials and real-world programming can be jarring. One minute you’re following clear instructions and writing code that works perfectly; the next, you’re staring at your editor wondering where to even begin.
This experience is incredibly common. In fact, it’s so prevalent that it has a name: the “tutorial hell” phenomenon. This article will explore why this happens and provide concrete strategies to bridge the gap between tutorials and personal projects.
The Reality Gap: Tutorial World vs. Project World
Tutorials provide a controlled environment where everything works as expected. They’re designed to teach specific concepts in isolation, with clear starting points and endpoints. Real projects, however, are messy, complex, and full of unexpected challenges.
Why Tutorials Feel Easier Than Personal Projects
- Predefined Path: Tutorials walk you through a predetermined sequence of steps with known outcomes.
- Simplified Scenarios: Complex edge cases and error handling are often minimized or eliminated entirely.
- Perfect Environment: Setup issues, compatibility problems, and configuration hurdles are typically resolved in advance.
- Scope Limitation: Tutorials focus on specific techniques rather than integrating multiple systems and technologies.
- Immediate Feedback: You know immediately if you’re on the right track.
When you start your own project, these scaffolds disappear. Suddenly, you need to make countless decisions about architecture, design patterns, libraries, and implementation details. There’s no script to follow, and the blank screen can be intimidating.
The Missing Elements in Most Tutorials
Most programming tutorials focus on teaching syntax and basic functionality. While these elements are important, they represent only a fraction of what real-world programming involves.
What Tutorials Often Skip
1. Project Planning and Architecture
Tutorials rarely start with the planning phase. You don’t see the instructor spending hours thinking about:
- How to structure the application
- Which design patterns to apply
- How different components will interact
- What the data models should look like
Yet these decisions form the foundation of any successful project. Without proper planning, even simple applications can quickly become unmanageable.
2. Requirement Gathering and Analysis
In tutorials, requirements are clearly stated from the beginning. In real projects, you often need to:
- Identify user needs
- Translate vague ideas into concrete features
- Prioritize functionality
- Define acceptance criteria
This process can be challenging and requires skills beyond coding.
3. Debugging and Troubleshooting
When following a tutorial, errors are either anticipated or edited out. In your own projects, you’ll spend significant time:
- Identifying the source of bugs
- Reading documentation and error messages
- Searching for solutions online
- Testing different approaches
Debugging is often where the real learning happens, but tutorials rarely show this process in detail.
4. Refactoring and Code Maintenance
Tutorials typically present the final, polished version of the code. They don’t show:
- The messy first drafts
- The iterative process of improving code
- How to recognize and fix code smells
- Techniques for maintaining code quality over time
In real projects, you’ll frequently revisit and refine your code as requirements evolve and your understanding deepens.
5. Integration Challenges
Most tutorials focus on building isolated components or features. They rarely address:
- Integrating with external APIs and services
- Managing dependencies between different parts of your application
- Handling version conflicts and compatibility issues
- Working with legacy code or systems
These integration points often present the most significant challenges in real-world development.
The Psychological Aspects of the Tutorial-Project Gap
Beyond the technical differences, there are psychological factors that contribute to the disconnect between tutorials and personal projects.
The Illusion of Understanding
Following along with a tutorial can create a false sense of mastery. You recognize the code, understand each step as it’s explained, and successfully complete the exercise. This leads to what psychologists call the “illusion of understanding” or “knowledge illusion.”
When you follow a tutorial, you’re engaging with the material primarily through recognition rather than recall. Recognition is much easier than recall, which is what you need when starting from scratch.
Decision Fatigue and Analysis Paralysis
In tutorials, most decisions are made for you. When building your own project, you face countless choices:
- Which framework or library should I use?
- How should I structure my database?
- What naming conventions should I follow?
- How should I handle errors?
This abundance of choices can lead to decision fatigue and analysis paralysis, where you spend more time researching options than actually building.
The Perfectionism Trap
Tutorials present polished, working code. This can create unrealistic expectations for your own projects. Many beginners get stuck trying to write perfect code from the start, rather than embracing an iterative approach where imperfect code is gradually improved.
Bridging the Gap: Strategies for Moving Beyond Tutorials
Now that we understand the differences between tutorials and real projects, let’s explore strategies to help you make the transition.
1. Modify Tutorials Before Creating From Scratch
Instead of jumping directly from following tutorials to building your own projects, create an intermediate step:
- Follow the tutorial exactly as presented
- Modify the completed tutorial by adding new features or changing functionality
- Rebuild the same project from memory without following the steps
- Create a similar but different project using the same concepts
This graduated approach helps you develop independence while still having a reference point.
2. Start With Small, Well-Defined Projects
Begin with projects that are small enough to complete but complex enough to be interesting. Clear requirements help overcome the analysis paralysis that comes with open-ended projects.
Some examples of well-scoped beginner projects:
- A to-do list application with basic CRUD operations
- A weather app that uses a public API
- A simple calculator with a clean UI
- A personal portfolio website
As you gain confidence, gradually increase the complexity of your projects.
3. Embrace Planning and Documentation
Before writing any code, spend time planning your project:
- Write user stories or requirements
- Sketch the user interface
- Create diagrams of data models and application flow
- List the technologies and libraries you’ll use
This planning phase forces you to think through the project holistically and creates a roadmap to follow when you start coding.
4. Build in Public and Seek Feedback
Working on projects in isolation can reinforce bad habits and limit your growth. Instead:
- Share your code on GitHub
- Participate in code reviews
- Join programming communities and forums
- Document your learning journey on social media or a blog
External feedback provides valuable insights and keeps you accountable.
5. Embrace Incremental Development
Rather than trying to build the entire project at once, focus on implementing one feature at a time:
- Create a minimal viable product (MVP) with core functionality
- Test thoroughly to ensure it works as expected
- Add additional features incrementally
- Refactor and improve code quality along the way
This approach is more manageable and reflects how real software development works.
6. Study Open Source Projects
Tutorials show you isolated examples, but open source projects demonstrate how professionals structure complete applications:
- Examine how files and directories are organized
- Study how different components interact
- Review coding conventions and patterns
- Observe how tests are implemented
Start with smaller, well-documented projects in technologies you’re familiar with.
7. Learn to Read and Understand Documentation
Tutorials often shield you from the need to read documentation. Developing this skill is crucial for independent development:
- Practice navigating official documentation for languages and frameworks
- Learn to identify the most relevant sections for your needs
- Use documentation as your first resource when stuck, before searching for tutorials
Strong documentation skills make you more self-sufficient and adaptable.
The Role of Debugging in Learning
One of the most significant differences between tutorials and real projects is the amount of time spent debugging. Embrace this as a learning opportunity rather than viewing it as a distraction.
Developing Systematic Debugging Skills
Effective debugging is a skill that can be developed with practice:
- Reproduce the issue consistently before attempting to fix it
- Isolate the problem by testing components individually
- Use debugging tools like browser developer tools, logging, and debugger statements
- Read error messages carefully rather than immediately searching for solutions
- Make one change at a time and test after each change
These systematic approaches will serve you better than the trial-and-error methods many beginners resort to.
Learning From Errors
Each error you encounter and resolve teaches you something valuable about how your code works. Consider keeping an “error journal” where you document:
- The error message and context
- What caused the error
- How you resolved it
- What you learned from the experience
Over time, this creates a personalized reference that speeds up your problem-solving process.
Tutorial-Driven Development vs. Problem-Driven Development
There’s a fundamental mindset shift that needs to happen when moving from tutorials to projects.
Tutorial-Driven Development
When following tutorials, your approach is typically:
- Follow instructions step by step
- Implement solutions as presented
- Focus on completing the tutorial
This is a passive learning process where someone else has done the problem-solving for you.
Problem-Driven Development
When working on your own projects, you need to adopt a different approach:
- Identify a specific problem or feature to implement
- Break it down into smaller, manageable tasks
- Research potential solutions for each task
- Implement, test, and refine your solution
This active learning process develops your problem-solving abilities and deepens your understanding.
Making the Transition
To shift from tutorial-driven to problem-driven development:
- Start with clearly defined problems rather than open-ended projects
- Practice breaking down problems before looking for solutions
- Use tutorials as references for specific techniques, not as comprehensive guides
- Combine ideas from multiple sources rather than following a single tutorial
This approach builds the mental muscles needed for independent development.
Code Examples: From Tutorial to Project
Let’s examine how code might evolve from a tutorial example to a real project implementation.
Tutorial Example: A Simple To-Do List
A typical tutorial might present a to-do list with basic functionality:
// HTML
<input id="taskInput" type="text" placeholder="Add a new task">
<button id="addButton">Add</button>
<ul id="taskList"></ul>
// JavaScript
document.getElementById('addButton').addEventListener('click', function() {
const taskInput = document.getElementById('taskInput');
const taskText = taskInput.value.trim();
if (taskText !== '') {
const taskList = document.getElementById('taskList');
const newTask = document.createElement('li');
newTask.textContent = taskText;
taskList.appendChild(newTask);
taskInput.value = '';
}
});
This code works but is limited in functionality and doesn’t address many real-world concerns.
Real Project Implementation
In a real project, you might need to consider:
- Persisting tasks across page reloads
- Validating input
- Handling task completion and deletion
- Organizing code for maintainability
- Adding error handling
Here’s how the same functionality might be implemented in a more robust way:
// HTML structure with more semantic elements and accessibility features
<form id="taskForm" aria-label="Add a new task">
<label for="taskInput">Task description:</label>
<input id="taskInput" type="text" placeholder="What needs to be done?" required>
<button type="submit">Add Task</button>
</form>
<div id="errorContainer" role="alert" class="hidden"></div>
<section>
<h2>Tasks</h2>
<ul id="taskList" class="task-list"></ul>
</section>
// JavaScript with more structure and features
class TodoApp {
constructor() {
this.tasks = JSON.parse(localStorage.getItem('tasks')) || [];
this.taskForm = document.getElementById('taskForm');
this.taskInput = document.getElementById('taskInput');
this.taskList = document.getElementById('taskList');
this.errorContainer = document.getElementById('errorContainer');
this.bindEvents();
this.renderTasks();
}
bindEvents() {
this.taskForm.addEventListener('submit', this.addTask.bind(this));
this.taskList.addEventListener('click', this.handleTaskAction.bind(this));
}
addTask(event) {
event.preventDefault();
const taskText = this.taskInput.value.trim();
try {
if (!taskText) {
throw new Error('Task cannot be empty');
}
const newTask = {
id: Date.now(),
text: taskText,
completed: false,
createdAt: new Date()
};
this.tasks.push(newTask);
this.saveTasks();
this.renderTasks();
this.taskInput.value = '';
this.hideError();
} catch (error) {
this.showError(error.message);
}
}
handleTaskAction(event) {
const taskItem = event.target.closest('li');
if (!taskItem) return;
const taskId = Number(taskItem.dataset.id);
if (event.target.classList.contains('delete-button')) {
this.deleteTask(taskId);
} else if (event.target.classList.contains('complete-button')) {
this.toggleTaskCompletion(taskId);
}
}
deleteTask(taskId) {
this.tasks = this.tasks.filter(task => task.id !== taskId);
this.saveTasks();
this.renderTasks();
}
toggleTaskCompletion(taskId) {
this.tasks = this.tasks.map(task => {
if (task.id === taskId) {
return { ...task, completed: !task.completed };
}
return task;
});
this.saveTasks();
this.renderTasks();
}
renderTasks() {
this.taskList.innerHTML = '';
if (this.tasks.length === 0) {
const emptyMessage = document.createElement('li');
emptyMessage.textContent = 'No tasks yet. Add one above!';
emptyMessage.classList.add('empty-message');
this.taskList.appendChild(emptyMessage);
return;
}
this.tasks.forEach(task => {
const taskItem = document.createElement('li');
taskItem.dataset.id = task.id;
taskItem.classList.add('task-item');
if (task.completed) {
taskItem.classList.add('completed');
}
const taskText = document.createElement('span');
taskText.textContent = task.text;
taskText.classList.add('task-text');
const completeButton = document.createElement('button');
completeButton.textContent = task.completed ? 'Undo' : 'Complete';
completeButton.classList.add('complete-button');
const deleteButton = document.createElement('button');
deleteButton.textContent = 'Delete';
deleteButton.classList.add('delete-button');
taskItem.appendChild(taskText);
taskItem.appendChild(completeButton);
taskItem.appendChild(deleteButton);
this.taskList.appendChild(taskItem);
});
}
saveTasks() {
localStorage.setItem('tasks', JSON.stringify(this.tasks));
}
showError(message) {
this.errorContainer.textContent = message;
this.errorContainer.classList.remove('hidden');
}
hideError() {
this.errorContainer.textContent = '';
this.errorContainer.classList.add('hidden');
}
}
// Initialize the app when the DOM is fully loaded
document.addEventListener('DOMContentLoaded', () => {
new TodoApp();
});
This implementation demonstrates several important differences from the tutorial version:
- Architecture: Uses a class-based structure to organize functionality
- Persistence: Stores tasks in localStorage to persist across page reloads
- Error Handling: Includes validation and error display
- Event Delegation: Uses a single event listener for all task actions
- State Management: Maintains application state and synchronizes with the UI
- Accessibility: Includes ARIA attributes and semantic HTML
These considerations reflect the complexity of real-world applications compared to tutorial examples.
Learning to Think Like a Developer
The transition from tutorials to projects isn’t just about technical skills; it’s about developing a different way of thinking.
Embracing Uncertainty
Professional developers don’t know all the answers in advance. They develop comfort with uncertainty and confidence in their ability to find solutions. This mindset shift involves:
- Accepting that not knowing is normal and expected
- Breaking problems into smaller, more manageable pieces
- Knowing how to find information efficiently
- Testing hypotheses systematically
Developing Technical Intuition
As you gain experience, you’ll develop intuition about:
- Which approaches are likely to work for different problems
- Where bugs might be hiding
- How to structure code for maintainability
- When to use different design patterns and techniques
This intuition comes from experience, particularly from making and fixing mistakes.
Learning to Research Effectively
Effective research is a core developer skill that tutorials rarely teach:
- Formulating precise search queries
- Evaluating the relevance and reliability of sources
- Adapting solutions to your specific context
- Synthesizing information from multiple sources
Practice these skills deliberately as you work on projects.
The Role of Project-Based Learning in Skill Development
While tutorials have their place in the learning journey, project-based learning offers unique benefits that are essential for becoming a proficient developer.
Benefits of Project-Based Learning
- Contextual Understanding: You learn how concepts fit together in a complete application.
- Retention: Knowledge gained through active problem-solving is better retained.
- Motivation: Building something meaningful provides intrinsic motivation.
- Portfolio Development: Projects demonstrate your capabilities to potential employers.
- Confidence Building: Successfully completing projects builds self-efficacy.
Finding the Right Balance
The most effective learning approach combines tutorials and projects:
- Use tutorials to learn new concepts and techniques
- Apply what you’ve learned in small projects
- Return to tutorials when you encounter specific challenges
- Gradually tackle more complex projects as your skills grow
This cyclical approach leverages the strengths of both learning methods.
Conclusion: Embracing the Journey from Tutorial to Project
The gap between tutorials and personal projects is real, but it’s also an essential part of the learning process. Every developer goes through this transition, and the challenges you face are not a sign of failure but a normal part of growth.
Remember that:
- The struggle is where the deepest learning happens
- Building projects is a skill that improves with practice
- Each project teaches you something valuable, even if it doesn’t turn out as expected
- The ability to create without a tutorial is what distinguishes developers from tutorial-followers
By understanding the differences between tutorials and projects, developing systematic approaches to planning and problem-solving, and embracing the challenges as learning opportunities, you can successfully bridge the gap and become a confident, independent developer.
Start small, be patient with yourself, and celebrate each step forward. The journey from tutorial to project isn’t always easy, but it’s where true growth as a developer happens.