Why Your Programming Knowledge Isn’t Turning Into Programming Intuition
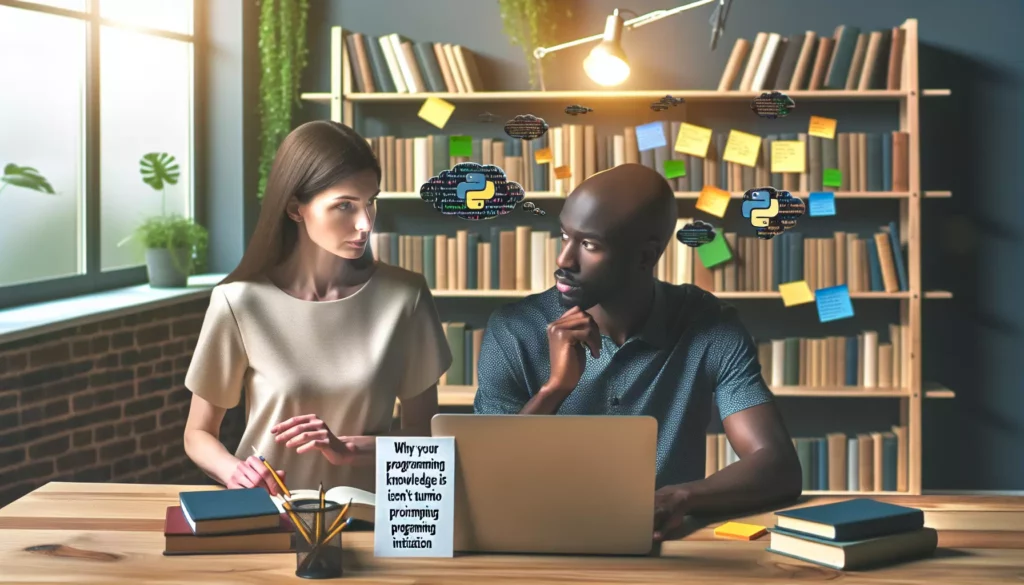
Have you ever found yourself staring at a blank code editor, knowing all the syntax and concepts but struggling to piece together a solution? You’re not alone. Many programmers, from beginners to those with years of experience, often face a puzzling disconnect between what they know about programming and their ability to apply it intuitively.
This gap between programming knowledge and programming intuition is more common than you might think. In this article, we’ll explore why this happens and, more importantly, how to bridge that gap effectively.
The Knowledge-Intuition Gap in Programming
Programming knowledge is relatively straightforward to acquire. You can learn syntax, memorize algorithms, and understand programming concepts through books, courses, and tutorials. However, programming intuition—the ability to instinctively know how to approach problems and write efficient code—is much harder to develop.
This intuition is what separates those who can recite programming concepts from those who can build elegant solutions under pressure. It’s the difference between knowing what a binary search is and immediately recognizing when and how to implement it in a novel situation.
But why does this gap exist in the first place?
Why Knowledge Doesn’t Automatically Become Intuition
1. Passive vs. Active Learning
One of the main reasons programming knowledge doesn’t translate to intuition is the way many people learn to code. Watching video tutorials or reading documentation is passive learning. While you might understand the material, passive learning rarely builds the neural pathways necessary for intuitive problem-solving.
Consider this scenario: You’ve watched hours of tutorials on recursion and understand the concept perfectly. But when faced with a problem that requires recursive thinking, you freeze up. This is because understanding a concept in theory is vastly different from applying it in practice.
2. Isolated Learning vs. Contextual Application
Many programming courses teach concepts in isolation. You learn variables, then loops, then functions, and so on. But real-world programming involves using these concepts together in complex ways.
For example, you might learn about arrays and sorting algorithms separately, but combining them to solve a specific problem requires a different kind of understanding altogether.
3. The Missing Experience of Debugging and Problem-Solving
Programming intuition is built through the experience of encountering and solving problems. Every time you debug a piece of code or refactor a solution to make it more efficient, you’re building intuition.
If your learning journey has been smooth sailing without much troubleshooting, you’re missing out on crucial experience that builds intuition.
4. The Curse of Knowledge
Sometimes, having too much theoretical knowledge can actually hinder intuition. This phenomenon, known as “the curse of knowledge,” happens when you know so many approaches and concepts that you overthink problems instead of seeing the straightforward solutions.
This is particularly common among those who have studied computer science academically but haven’t built many practical projects.
Signs Your Programming Knowledge Isn’t Becoming Intuition
How do you know if you’re experiencing this knowledge-intuition gap? Here are some telltale signs:
You Struggle to Start Projects from Scratch
If you find yourself comfortable modifying existing code but freeze when facing a blank file, you’re likely relying on knowledge rather than intuition. Intuitive programmers can envision the structure of a solution before writing a single line of code.
You Need to Look Up Basic Syntax Frequently
While it’s normal to reference documentation for complex or rarely-used features, constantly needing to check basic syntax suggests that the knowledge hasn’t been internalized into intuition.
You Can Follow Tutorials But Can’t Create Similar Projects Independently
This is extremely common. You follow a tutorial to build a to-do app, understanding every step. But when you try to build a similar app on your own, you struggle to replicate the process.
You Excel at Multiple Choice Questions But Struggle with Open-Ended Problems
If you can recognize the correct approach when presented with options but can’t generate solutions on your own, your knowledge hasn’t transformed into intuition.
You Can Explain Concepts But Struggle to Implement Them
Being able to explain how a binary tree works but struggling to implement one from scratch indicates a gap between theoretical knowledge and practical intuition.
Building Programming Intuition: The Path Forward
Now that we understand the problem, let’s explore how to transform programming knowledge into intuition effectively.
1. Embrace Active Learning Through Project-Based Approaches
The most effective way to build intuition is through active learning, particularly by working on projects. Instead of passively consuming tutorials, challenge yourself to build something with minimal guidance.
Start with small projects that slightly exceed your current comfort level. As you struggle through challenges and solve problems, you’ll build the neural pathways necessary for intuition.
2. Deliberate Practice: Focus on What’s Difficult
Not all practice is created equal. Deliberate practice involves focusing specifically on the areas where you struggle the most. If you find recursion challenging, don’t avoid it—tackle it head-on with increasingly complex recursive problems.
Research in skill acquisition shows that working at the edge of your capabilities—where tasks are challenging but not impossible—accelerates the development of intuition.
3. Code Review and Refactoring
One of the best ways to develop intuition is to review and refactor code—both your own and others’. When you analyze code critically, asking questions like “Why was it written this way?” and “How could this be improved?”, you’re building the mental models that support intuition.
Consider this example of refactoring a simple function:
// Original function
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price * items[i].quantity;
}
return total;
}
// Refactored function using reduce
function calculateTotal(items) {
return items.reduce((total, item) => total + (item.price * item.quantity), 0);
}
By thinking through such refactorings, you develop an intuitive sense of code elegance and efficiency.
4. Learn by Teaching and Explaining
The “Feynman Technique,” named after physicist Richard Feynman, suggests that explaining concepts in simple terms is one of the best ways to truly understand them. When you can explain a programming concept to someone else—especially a non-programmer—you’re forced to break it down to its essence, which strengthens your intuitive grasp.
Consider starting a blog, contributing to forums, or mentoring a junior developer to practice explaining concepts.
5. Study Patterns, Not Just Solutions
Instead of memorizing specific solutions to problems, focus on recognizing patterns. Programming intuition is largely about pattern recognition—seeing similarities between the current problem and problems you’ve solved before.
For example, many problems can be solved using common patterns like:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Dynamic programming
When you understand these patterns deeply, you’ll intuitively recognize when to apply them.
6. Embrace Debugging as a Learning Opportunity
Many programmers see debugging as a necessary evil, but it’s actually one of the most powerful tools for building intuition. Every bug you solve teaches you something about how the language or system works.
Instead of getting frustrated when your code doesn’t work, get curious. Ask “Why is this happening?” and work through the problem methodically. This process builds a deep, intuitive understanding of how code behaves.
7. Pair Programming and Collaborative Coding
Working with other programmers exposes you to different approaches and thought processes. When you see how someone else tackles a problem, it can challenge your assumptions and expand your intuitive repertoire.
If you don’t have a coding partner, consider joining open-source projects or coding communities where you can collaborate with others.
The Role of Spaced Repetition and Interleaved Practice
Cognitive science has revealed two powerful learning techniques that can accelerate the development of programming intuition: spaced repetition and interleaved practice.
Spaced Repetition: Timing Matters
Spaced repetition involves reviewing material at increasing intervals over time. Instead of cramming all your learning into one session, space it out. This approach has been proven to strengthen long-term memory and build intuitive understanding.
For programming, this might mean:
- Learning a new concept today
- Practicing it again tomorrow
- Then three days later
- Then a week later
- And so on
Tools like Anki can help implement spaced repetition for programming concepts, but the most effective approach is to apply the concepts in different projects over time.
Interleaved Practice: Mix It Up
Interleaved practice involves mixing different types of problems rather than focusing on one type at a time. This approach forces your brain to actively select the appropriate strategy for each problem, building more robust neural connections.
For example, instead of practicing 20 sorting problems in a row, mix sorting problems with tree traversal, string manipulation, and dynamic programming problems. This variety forces your brain to actively recall and apply different concepts, strengthening your intuitive grasp of when to use each approach.
The Four Stages of Programming Intuition Development
Building programming intuition doesn’t happen overnight. It typically progresses through four distinct stages:
Stage 1: Conscious Incompetence
At this stage, you know what you don’t know. You understand programming concepts but are acutely aware of your inability to apply them fluently. This can be frustrating but is actually a positive sign—recognizing the gap is the first step to bridging it.
Stage 2: Conscious Competence
With practice, you reach a stage where you can solve programming problems, but it requires conscious effort and deliberate thinking. You might need to talk yourself through the steps or refer to notes, but you can get to a solution.
Most programmers get stuck at this stage because it’s functional—you can complete tasks, even if it takes longer than it should.
Stage 3: Unconscious Competence
This is where true intuition begins. You can solve common programming problems without consciously thinking through each step. Solutions come to you naturally, and you can focus your conscious attention on the novel aspects of the problem rather than the basics.
Stage 4: Reflective Competence
The highest level of intuition is reflective competence, where you not only solve problems intuitively but can also articulate your intuitive process to others. You understand why your intuition works the way it does and can deliberately improve it.
This level of intuition is what makes someone an exceptional mentor and technical leader.
Common Obstacles to Developing Programming Intuition
Even with the right approach, several obstacles can hinder the development of programming intuition:
Tutorial Hell
One of the most common traps is getting stuck in “tutorial hell”—continuously consuming tutorials without building projects independently. This creates an illusion of learning without developing true intuition.
The solution? Limit tutorials to 20% of your learning time, with the remaining 80% spent on projects and practice.
Perfectionism
Waiting until you “know enough” to start building is a perfectionist trap. Intuition develops through the messy process of building, making mistakes, and refactoring—not from perfect knowledge.
Remember: The best programmers aren’t those who never make mistakes; they’re those who make mistakes quickly, learn from them, and iterate.
Isolation
Programming in isolation limits your exposure to different approaches and feedback. Without seeing how others solve problems or receiving feedback on your code, your intuition develops in a vacuum.
Join coding communities, participate in code reviews, and pair program when possible to counter this obstacle.
Skipping Fundamentals
In the rush to build impressive projects, many programmers skip over fundamentals like data structures, algorithms, and system design. While you can write functional code without these, deep intuition requires a solid foundation.
Take the time to understand how things work under the hood, even if it seems unnecessary for your immediate goals.
Practical Exercises to Develop Programming Intuition
Let’s explore some specific exercises designed to bridge the gap between knowledge and intuition:
Exercise 1: Implement the Same Feature in Multiple Ways
Choose a simple feature, like a user authentication system, and implement it using different approaches:
- First, with plain functions
- Then using object-oriented programming
- Then with a functional programming approach
- Finally, using a framework or library
By solving the same problem in different ways, you develop a deeper intuition for the strengths and weaknesses of each approach.
Exercise 2: The Code Prediction Game
Before running code, predict what it will do—including any errors or edge cases. This forces you to mentally execute the code, building your intuitive understanding of how the language works.
For example, look at this JavaScript code and predict the output:
let x = 5;
setTimeout(() => console.log(x), 0);
x = 10;
console.log(x);
If you predicted it would output “10” followed by “10,” your intuition about JavaScript’s event loop is developing well!
Exercise 3: Code Golf
Take working code and challenge yourself to rewrite it in fewer lines without losing readability or efficiency. This exercise forces you to deeply understand language features and programming patterns.
For example, converting this:
function getEvenNumbers(array) {
const result = [];
for (let i = 0; i < array.length; i++) {
if (array[i] % 2 === 0) {
result.push(array[i]);
}
}
return result;
}
To this:
function getEvenNumbers(array) {
return array.filter(num => num % 2 === 0);
}
Exercise 4: The Whiteboard Challenge
While whiteboard coding interviews are controversial, practicing coding without an IDE builds intuition. Try solving problems on paper or a whiteboard, without syntax highlighting, autocomplete, or the ability to run the code.
This forces you to truly understand language syntax and logic rather than relying on tools.
Exercise 5: Reverse Engineering
Take a piece of code you didn’t write and try to understand what it does without running it. Then modify it to add a new feature or fix a bug. This builds your ability to read code intuitively, a crucial skill for professional developers.
The Role of Mental Models in Programming Intuition
At its core, programming intuition is built on mental models—simplified representations of how programming concepts work. The stronger and more accurate your mental models, the better your intuition.
Developing Mental Models for Programming
To build effective mental models:
Visualize Code Execution
Train yourself to mentally trace through code, visualizing variables changing and functions being called. Tools like Python Tutor can help by showing code execution step-by-step, but the goal is to develop this ability mentally.
Use Analogies and Metaphors
Connect programming concepts to real-world analogies. For example:
- Variables are like labeled boxes that hold values
- Recursion is like nested Russian dolls
- Object-oriented programming is like creating blueprints (classes) for manufacturing objects
These analogies provide mental hooks that make abstract concepts more intuitive.
Draw Diagrams
Visual representations of code can strengthen your mental models. Practice drawing memory diagrams, call stacks, or data structures on paper as you work through problems.
Connect New Concepts to Existing Knowledge
When learning something new, explicitly connect it to concepts you already understand. This creates a web of knowledge rather than isolated facts, supporting intuitive problem-solving.
From Intermediate to Advanced: Developing Deep Programming Intuition
For those who have already developed basic programming intuition, the journey continues. Here’s how to develop deeper, more sophisticated intuition:
Study System Design and Architecture
Advanced programming intuition includes knowing how to structure entire systems, not just individual functions or classes. Study system design patterns, architectural approaches, and trade-offs between different designs.
Read High-Quality Code
Regularly reading well-written code from open-source projects or books like “Beautiful Code” exposes you to elegant solutions and approaches you might not discover on your own.
Develop Cross-Language Intuition
Learning multiple programming languages deepens your intuition by helping you distinguish between language-specific features and universal programming concepts. This builds a more abstract, powerful form of intuition that transcends specific languages.
Practice Performance Optimization
Take working code and optimize it for performance, memory usage, or readability. This develops intuition for the hidden costs and benefits of different approaches.
Build Tools for Programmers
Building developer tools forces you to think deeply about how programming works and what makes for a good developer experience. This meta-level thinking builds sophisticated intuition.
The Role of Failure in Building Programming Intuition
Perhaps counterintuitively, failure is one of the most powerful builders of programming intuition. Each time your code doesn’t work as expected, you have an opportunity to update your mental models and strengthen your intuition.
Embracing Productive Failure
Not all failure is equally valuable for learning. To make failure productive:
Analyze Root Causes
When your code fails, don’t just fix the immediate issue. Dig deeper to understand the root cause. Ask “Why did I make this mistake?” and “What misconception led me here?”
Maintain an Error Journal
Keep a log of bugs you encounter, how you fixed them, and what you learned. Over time, patterns will emerge that strengthen your intuition about common pitfalls.
Share Your Failures
Discussing your mistakes with other programmers normalizes the learning process and often reveals insights you might have missed on your own.
The Continuous Journey of Intuition Building
Developing programming intuition isn’t a destination but a continuous journey. Even the most experienced programmers are constantly refining their intuition as languages evolve, new paradigms emerge, and they encounter novel problems.
The key is to maintain a growth mindset—viewing challenges not as evidence of your limitations but as opportunities to expand your intuitive capabilities.
Measuring Progress in Intuition Development
Unlike knowledge, which can be tested directly, intuition is harder to measure. Here are some signs your programming intuition is growing:
- You can identify potential bugs before running your code
- You find yourself writing fewer comments because your code is naturally readable
- You can estimate the performance characteristics of code at a glance
- You spend less time Googling basic syntax and more time researching higher-level concepts
- You can quickly evaluate trade-offs between different approaches
- Your first attempts at solving problems are increasingly likely to work
Conclusion: Bridging the Gap Between Knowledge and Intuition
The gap between programming knowledge and programming intuition is real, but it’s not insurmountable. By understanding why this gap exists and deliberately practicing in ways that build intuition rather than just knowledge, you can transform your programming capabilities.
Remember that developing intuition is not about memorizing more facts or learning more languages—it’s about deepening your understanding through deliberate practice, embracing challenges, and building robust mental models.
The journey from knowledge to intuition may be challenging, but it’s also incredibly rewarding. As your intuition develops, you’ll find programming becomes less about remembering syntax and more about creative problem-solving—which is where the true joy of programming lies.
So the next time you find yourself staring at that blank editor, remember: building intuition takes time, but with the right approach, your fingers will eventually know what to type before your conscious mind has fully formulated the thought.
Your programming knowledge is the foundation. Your programming intuition is what you build on top of it. And like any worthwhile structure, building it properly takes time, effort, and a thoughtful approach.