Why Your Problem Solving Stops at the First Solution (And How to Fix It)
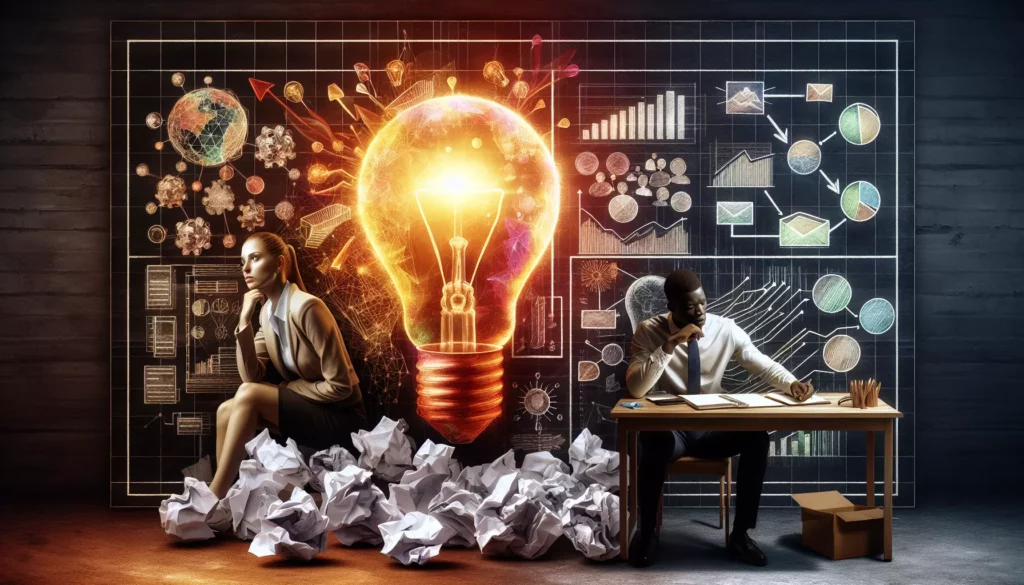
Have you ever felt the rush of satisfaction when you finally crack a coding problem? That moment when everything clicks and your solution works is undeniably rewarding. But what if I told you that stopping at your first working solution might be holding you back from becoming a truly exceptional programmer?
In the world of software development and algorithmic thinking, finding a solution is just the beginning. The best engineers know that the journey from a working solution to an optimal one is where real growth happens.
The First Solution Trap
When tackling coding problems, there’s a common pattern many developers fall into:
- Understand the problem
- Devise a solution
- Implement it
- Test it
- If it works: celebrate and move on
This approach seems logical. After all, in real-world scenarios, we’re often under time constraints. But this habit can severely limit your growth as a programmer, especially when preparing for technical interviews or building systems that need to scale.
Why We Stop at the First Solution
Several psychological factors contribute to our tendency to stop at the first working solution:
1. The Relief of Resolution
The moment we solve a problem, our brain releases dopamine, creating a sense of accomplishment. This chemical reward makes us eager to move on to the next challenge rather than refining our current solution.
2. Cognitive Fatigue
Problem solving is mentally taxing. After investing significant cognitive resources to find a solution, we naturally want to conserve energy rather than expend more on optimization.
3. The “Good Enough” Fallacy
We often rationalize that if a solution works, it’s “good enough.” This mindset overlooks the fact that in professional settings, code quality, efficiency, and maintainability are just as important as functionality.
4. Confirmation Bias
Once we have a working solution, we tend to focus on evidence that confirms our approach is optimal, rather than critically examining its weaknesses.
The Cost of Settling
Stopping at your first solution carries several hidden costs:
Technical Debt
Unoptimized solutions often create technical debt. What works for a small dataset might crash when scaled up. What seems clean now might become a maintenance nightmare later.
Missed Learning Opportunities
Each problem contains multiple lessons. By settling for your first solution, you miss the deeper insights that come from exploring alternative approaches.
Stunted Growth
Professional growth in programming comes from pushing beyond your comfort zone. Settling creates a plateau in your development.
Interview Underperformance
Technical interviews at top companies like Google, Amazon, and Facebook (Meta) explicitly evaluate your ability to optimize solutions. Stopping at your first answer can be the difference between success and failure.
A Real-World Example: The Two Sum Problem
Let’s illustrate this concept with the classic “Two Sum” problem:
Given an array of integers and a target sum, return the indices of the two numbers that add up to the target.
First Solution: Brute Force
Most programmers immediately think of a nested loop approach:
function twoSum(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return null;
}
This works! But it has O(n²) time complexity. If you stop here, you’re missing out.
Optimized Solution: Hash Map
With further reflection, you might realize you can use a hash map to achieve O(n) time complexity:
function twoSum(nums, target) {
const numMap = {};
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (numMap[complement] !== undefined) {
return [numMap[complement], i];
}
numMap[nums[i]] = i;
}
return null;
}
This solution is dramatically more efficient for large inputs. By trading space complexity for time complexity, we’ve created a solution that scales much better.
The Difference in Practice
For an array of 10,000 elements:
- Brute force: Up to 50,000,000 operations
- Hash map: Only 10,000 operations
That’s a 5,000x improvement in performance! This is the kind of optimization that separates junior developers from senior ones.
Breaking the First Solution Habit
Now that we understand the problem, let’s explore strategies to overcome this limiting habit:
1. Adopt a Multi-Solution Mindset
Before coding, train yourself to brainstorm multiple approaches. Ask questions like:
- What’s the simplest way to solve this?
- What’s the most efficient way?
- Is there a way to trade space for time (or vice versa)?
- Can I use a different data structure to optimize this?
By considering multiple approaches before implementation, you’ll develop a more flexible problem-solving toolkit.
2. Analyze Time and Space Complexity
For every solution you develop, make it a habit to analyze both time and space complexity using Big O notation. This practice helps you objectively compare different approaches and understand their scalability limitations.
3. Implement the “Rule of Three”
Challenge yourself to come up with at least three different solutions to each significant problem you encounter. They might vary in:
- Algorithm choice
- Data structures used
- Recursive vs. iterative approaches
- Time vs. space trade-offs
This exercise expands your problem-solving repertoire and prevents premature satisfaction with suboptimal solutions.
4. Practice Deliberate Refactoring
Even after you’ve found a working solution, set aside time specifically for refactoring. Ask yourself:
- Can I make this code more readable?
- Are there edge cases I haven’t considered?
- Could I reduce the algorithmic complexity?
- Is this solution maintainable in the long term?
Treating refactoring as a distinct phase of development helps ensure it doesn’t get skipped.
The Advanced Problem-Solving Framework
To move beyond the first solution trap, consider adopting this comprehensive problem-solving framework:
1. Understand
- Clarify the problem statement
- Identify constraints and edge cases
- Work through examples manually
2. Plan
- Brainstorm multiple approaches
- Analyze complexity for each approach
- Select an initial implementation strategy
3. Implement
- Write clean, well-structured code
- Add comments for clarity
- Test with examples
4. Optimize
- Identify bottlenecks
- Consider alternative algorithms or data structures
- Implement and test improvements
5. Reflect
- Compare different solutions
- Document lessons learned
- Consider how the approach might apply to other problems
Case Study: Fibonacci Sequence
Let’s see how this framework applies to calculating the nth Fibonacci number:
Approach 1: Recursive
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n-1) + fibonacci(n-2);
}
This solution is elegant and mirrors the mathematical definition, but it has exponential O(2^n) time complexity due to redundant calculations.
Approach 2: Dynamic Programming (Memoization)
function fibonacci(n, memo = {}) {
if (n <= 1) return n;
if (memo[n]) return memo[n];
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo);
return memo[n];
}
By storing previously calculated values, we reduce time complexity to O(n) while maintaining the recursive structure.
Approach 3: Iterative
function fibonacci(n) {
if (n <= 1) return n;
let a = 0, b = 1;
for (let i = 2; i <= n; i++) {
let temp = a + b;
a = b;
b = temp;
}
return b;
}
This solution achieves O(n) time complexity with O(1) space complexity, making it more efficient than the memoized version for large inputs.
Approach 4: Matrix Exponentiation
function fibonacci(n) {
if (n <= 1) return n;
let F = [[1, 1], [1, 0]];
power(F, n-1);
return F[0][0];
}
function power(F, n) {
if (n <= 1) return;
let M = [[1, 1], [1, 0]];
power(F, Math.floor(n/2));
multiply(F, F);
if (n % 2 !== 0) {
multiply(F, M);
}
}
function multiply(F, M) {
let a = F[0][0] * M[0][0] + F[0][1] * M[1][0];
let b = F[0][0] * M[0][1] + F[0][1] * M[1][1];
let c = F[1][0] * M[0][0] + F[1][1] * M[1][0];
let d = F[1][0] * M[0][1] + F[1][1] * M[1][1];
F[0][0] = a;
F[0][1] = b;
F[1][0] = c;
F[1][1] = d;
}
This advanced approach achieves O(log n) time complexity using matrix exponentiation, making it significantly faster for very large inputs.
By exploring all four approaches, we gain a much deeper understanding of both the Fibonacci sequence and various algorithmic techniques. Stopping at the first recursive solution would have missed these valuable insights.
Beyond Algorithms: System Design Considerations
The “first solution trap” extends beyond algorithms to system design and architecture. Consider these examples:
Database Schema Design
A first-draft schema might work for initial requirements but fail to accommodate future growth. By exploring multiple schema designs before implementation, you can create more flexible, scalable systems.
API Design
Your first API design might focus solely on current functionality. A more thoughtful approach considers:
- Backward compatibility
- Versioning strategy
- Error handling patterns
- Authentication and authorization
- Rate limiting and quotas
Each of these considerations improves the robustness of your API beyond the first working solution.
Frontend Architecture
A quick implementation might tightly couple components, making future changes difficult. Taking time to consider component boundaries, state management, and rendering optimization leads to more maintainable frontends.
How Top Companies Evaluate Problem Solving
Understanding how companies like Google, Amazon, Facebook (Meta), Apple, and Netflix evaluate problem-solving can provide additional motivation to move beyond your first solution.
Google’s interview process explicitly evaluates candidates on their ability to:
- Develop multiple approaches to a problem
- Analyze trade-offs between different solutions
- Optimize for both correctness and efficiency
Stopping at your first solution would be a red flag in a Google interview.
Amazon
Amazon’s leadership principles include “Dive Deep” and “Invent and Simplify.” These principles reflect an expectation that engineers will thoroughly explore problem spaces rather than implementing the first solution that comes to mind.
Facebook/Meta
Meta’s interviews often include explicit optimization rounds where candidates are expected to improve upon their initial solutions. The ability to iterate and refine is considered a core engineering skill.
Practical Exercises to Develop Multi-Solution Thinking
Here are some exercises you can practice to break the habit of stopping at your first solution:
1. The Three-Solution Challenge
Pick a coding problem from platforms like LeetCode, HackerRank, or AlgoCademy. Force yourself to implement three different solutions with different approaches or data structures. Compare their time and space complexity.
2. Optimization Sprints
Set a timer for 25 minutes to find your first working solution to a problem. Then set another 25-minute timer specifically for optimizing that solution. This dedicated optimization time prevents the tendency to move on after the first solution.
3. Reverse Engineering
After solving a problem, look at other developers’ solutions. Analyze approaches that differ from yours and understand their trade-offs. This exposes you to alternative thinking patterns.
4. Constraint Practice
After solving a problem, add artificial constraints and solve it again:
- Solve it without using extra space
- Solve it in a single pass through the data
- Solve it without a particular data structure you used previously
These constraints force you to find alternative approaches.
Learning from Masters: Case Studies in Optimization
Some of the most impressive examples of moving beyond first solutions come from real-world optimizations by tech giants:
Google’s MapReduce
The naive approach to large-scale data processing would be to use bigger, more powerful servers. Instead, Google developed MapReduce, a paradigm for processing vast amounts of data across distributed clusters of computers.
This wasn’t their first solution—it was the result of deep thinking about the limitations of traditional approaches and a willingness to redesign from first principles.
Facebook’s React
The conventional approach to UI updates was direct DOM manipulation. React introduced a virtual DOM and a reconciliation algorithm that dramatically improved performance for complex UIs.
This innovation came from questioning the fundamental assumptions of web development rather than incrementally improving existing patterns.
Amazon’s DynamoDB
Traditional relational databases were the first solution for most data storage needs. Amazon’s development of DynamoDB represented a fundamental rethinking of database design for scale, creating a NoSQL solution optimized for high availability and throughput.
The Psychological Benefits of Multi-Solution Thinking
Beyond technical advantages, developing the habit of exploring multiple solutions offers psychological benefits:
Increased Confidence
Knowing you can approach problems from multiple angles builds confidence in your problem-solving abilities.
Reduced Anxiety
When your identity isn’t tied to your first solution, you become more comfortable with critique and iteration.
Greater Creativity
Regular practice in finding alternative solutions enhances your creative thinking in all aspects of work.
Improved Collaboration
Being open to multiple approaches makes you a better team member, able to appreciate and build upon others’ ideas.
Building a Personal Improvement System
To systematically improve your ability to move beyond first solutions, consider implementing these practices:
1. Keep a Problem-Solving Journal
For each significant problem you solve, document:
- Your initial solution and its complexity
- Alternative approaches you considered
- Optimizations you implemented
- Lessons learned in the process
This reflection reinforces the multi-solution mindset and creates a valuable reference for future problems.
2. Form a Code Review Group
Regular code reviews with peers can provide valuable feedback on your solutions and expose you to different approaches. Even explaining your solution to others often reveals optimization opportunities you hadn’t considered.
3. Set Explicit Optimization Goals
For important projects, set specific goals like:
- Reduce time complexity by one order of magnitude
- Cut memory usage in half
- Improve readability by reducing function size
These concrete targets prevent complacency with first solutions.
4. Study Algorithm Design Patterns
Familiarize yourself with common design patterns like:
- Sliding window
- Two pointers
- Binary search variations
- Dynamic programming approaches
- Graph traversal techniques
Having this toolkit allows you to quickly recognize when a problem might benefit from a particular approach.
Conclusion: The Journey Beyond the First Solution
Moving beyond your first solution isn’t just about writing better code—it’s about developing a mindset of continuous improvement that distinguishes exceptional engineers from average ones.
The ability to critically evaluate your own work, consider alternatives, and refine your approach is valuable not just in coding interviews but throughout your career. It leads to more scalable systems, more maintainable code, and ultimately, greater impact.
Remember that every problem contains multiple lessons. By exploring different solutions, you extract maximum learning from each challenge and build a diverse toolkit of problem-solving strategies.
The next time you solve a problem and feel that rush of accomplishment, take a moment to ask: “Is there a better way?” That simple question is the first step on the journey from a good programmer to a great one.
Your first solution is just the beginning. The real growth happens when you keep going.