Why Your Problem-Solving Process Falls Apart in Interviews
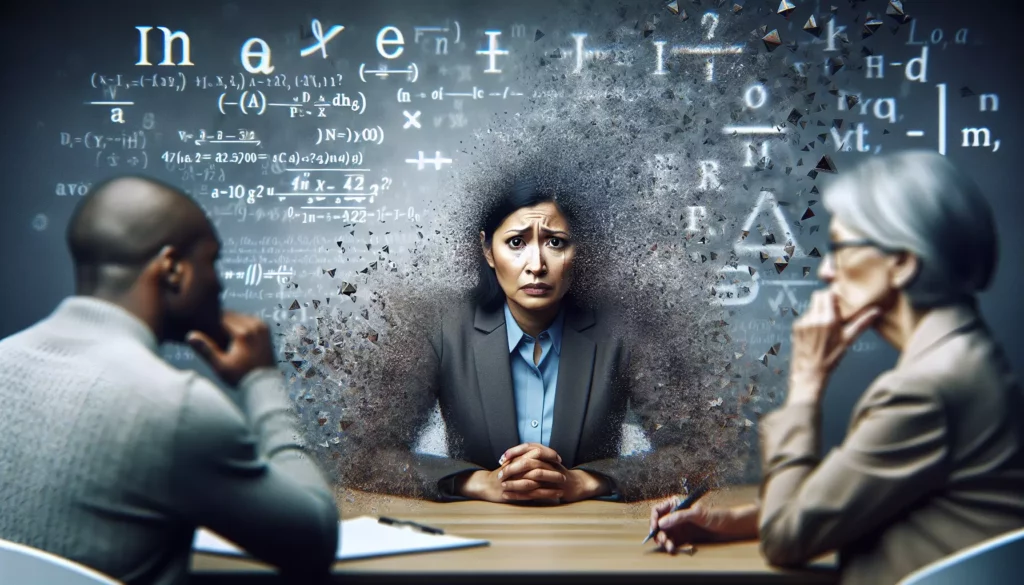
You’ve spent countless hours mastering data structures, memorizing algorithms, and solving hundreds of LeetCode problems. Yet, when you sit across from an interviewer at your dream tech company, something unexpected happens: your carefully honed problem-solving process crumbles under pressure.
This phenomenon is frustratingly common. Even experienced developers who can breeze through complex problems in their daily work find themselves stumbling during technical interviews. Why does this happen, and more importantly, how can you prevent it?
In this comprehensive guide, we’ll explore the psychology behind interview performance, identify the common pitfalls that derail candidates, and provide actionable strategies to strengthen your problem-solving process under pressure.
The Anatomy of a Technical Interview Meltdown
Technical interviews at top tech companies like Google, Amazon, Meta, and other FAANG/MAANG organizations are designed to test not just your coding ability, but your problem-solving approach. Let’s examine what typically happens when a candidate’s process breaks down:
The Initial Freeze
You’re presented with a problem that seems vaguely familiar. Instead of methodically working through it, you experience a moment of panic. Your mind races to recall a similar problem you’ve seen before, but the details are fuzzy. The interviewer is waiting, and the silence grows uncomfortable.
The Rushed Solution
To break the silence, you start coding immediately without fully understanding the problem. You skip crucial steps like clarifying requirements or exploring different approaches. The interviewer notices your hasty approach but lets you continue.
The Spiral of Errors
Midway through implementation, you realize your approach has a fundamental flaw. You try to patch it with increasingly complex fixes, making your code messy and error-prone. Each new bug compounds your anxiety, making it harder to think clearly.
The Defeated Conclusion
By the end of the interview, your solution is incomplete or incorrect. The interviewer asks you about edge cases you didn’t consider or efficiency improvements you could have made. You leave feeling that you’ve failed to demonstrate your true abilities.
Sound familiar? If so, you’re not alone. This pattern affects even highly skilled developers. The good news is that with the right preparation, you can overcome these challenges.
Why Your Brain Betrays You: The Psychology of Interview Performance
To improve your interview performance, it’s essential to understand the psychological factors at play:
Cognitive Load and Working Memory
During an interview, your working memory is taxed by multiple demands: understanding the problem, recalling relevant algorithms, planning your approach, writing syntactically correct code, and maintaining awareness of the interviewer’s reactions. This cognitive load can overwhelm your brain’s processing capacity.
Research in cognitive psychology shows that under stress, working memory capacity decreases significantly. This explains why you might struggle with problems during interviews that you could easily solve in a relaxed environment.
Performance Anxiety and the Limbic Response
Technical interviews trigger what psychologists call “evaluative stress” — the anxiety that comes from knowing your performance is being judged. This activates your limbic system (particularly the amygdala), which can initiate a fight-or-flight response.
When this happens, blood flow actually decreases to your prefrontal cortex — the part of your brain responsible for logical reasoning and problem-solving. Instead, resources are diverted to systems that would help you escape physical danger. This biological response is extremely unhelpful when you’re trying to solve a complex algorithmic problem!
Impostor Syndrome and Self-Fulfilling Prophecies
Many candidates enter interviews with a lingering fear that they’re not qualified or that they’ll be “found out.” This impostor syndrome creates a dangerous feedback loop: anxiety leads to poor performance, which confirms your fears, increasing anxiety further.
Additionally, stereotype threat can affect performance for individuals from underrepresented groups in tech. The mere awareness of negative stereotypes about one’s group can create additional cognitive burden and harm performance.
The 7 Most Common Problem-Solving Failures in Technical Interviews
Now that we understand the psychological underpinnings, let’s examine the specific ways your problem-solving process typically breaks down during interviews:
1. Skipping Problem Clarification
In their rush to start coding, many candidates fail to fully understand the problem. They don’t ask clarifying questions about input constraints, expected output formats, or edge cases. This often leads to solving the wrong problem entirely.
For example, a candidate might immediately start coding a solution for finding duplicates in an array without clarifying whether the array is sorted, if memory constraints exist, or what should happen with multiple duplicates.
2. Neglecting to Verbalize Your Thought Process
Interviewers value your thought process as much as your final solution. When candidates go silent or jump straight to coding, interviewers can’t evaluate how they approach problems.
Remember: in a technical interview, correct code that appears without explanation might lead interviewers to wonder if you’ve simply memorized the solution rather than understood the underlying concepts.
3. Failing to Consider Multiple Approaches
Strong problem-solvers explore multiple potential solutions before committing to one. Under interview pressure, many candidates grasp the first approach that comes to mind, even if it’s suboptimal.
Consider a string manipulation problem: a brute force solution might be immediately obvious, but taking time to consider whether a hash map, two-pointer technique, or dynamic programming approach would be more efficient can demonstrate sophisticated thinking.
4. Ignoring Time and Space Complexity
In real-world development, code that works is often enough. In technical interviews, however, efficiency matters tremendously. Many candidates implement solutions without analyzing or discussing the time and space complexity until prompted by the interviewer.
This is particularly problematic because optimizing an already-implemented solution often requires substantial rewriting, which is difficult to do under time pressure.
5. Writing Code Without a Clear Plan
Diving into implementation without a high-level plan leads to disorganized code that’s difficult to debug. This “code first, think later” approach rarely works well in interviews.
For instance, when solving a graph problem, starting to code without first deciding on the representation (adjacency list or matrix) and traversal method (BFS or DFS) can lead to a tangled implementation.
6. Overlooking Test Cases and Edge Conditions
Many candidates finish coding and declare “done” without testing their solution against various inputs, including edge cases. This gives the impression that you don’t prioritize correctness or thoroughness.
Common edge cases that get overlooked include empty inputs, single-element inputs, maximum/minimum values, and inputs that trigger boundary conditions in your algorithm.
7. Giving Up Too Easily on Bugs
When faced with an error or unexpected output, some candidates quickly become demoralized and lose confidence. Instead of systematically debugging their code, they might make random changes or suggest starting over entirely.
This reaction signals to interviewers that you might struggle when facing inevitable challenges in real-world development scenarios.
Building a Bulletproof Problem-Solving Framework
Now that we’ve identified the common pitfalls, let’s develop a structured approach that can withstand interview pressure:
The UMPIRE Framework: A Step-by-Step Process
One effective framework that addresses the common failures is the UMPIRE method, which stands for:
- Understand the problem
- Match the problem to potential approaches
- Plan your solution
- Implement the code
- Review your solution
- Evaluate alternative approaches
Let’s break down each step:
1. Understand the Problem (2-3 minutes)
Start by restating the problem in your own words to confirm your understanding. Ask clarifying questions about:
- Input constraints (size, type, range of values)
- Expected output format
- Edge cases and how they should be handled
- Performance requirements
Example dialogue:
“So if I understand correctly, I need to find the kth largest element in an unsorted array. A few questions: Can the array contain duplicates? Should I consider the kth largest distinct element or just the kth largest including duplicates? Are there any constraints on memory usage? What’s the expected behavior if k is larger than the array size?”
2. Match the Problem to Potential Approaches (2-3 minutes)
Consider which data structures and algorithms might be applicable. Verbalize multiple approaches, even if they’re not all optimal:
“For this problem, I see a few potential approaches:
1. We could sort the array and return the (length-k)th element. This would be O(n log n) time.
2. We could use a min-heap of size k, which would give us O(n log k) time.
3. We could use the QuickSelect algorithm for an average case O(n) solution.
Let me think about the trade-offs…”
3. Plan Your Solution (3-4 minutes)
Before writing any code, outline your approach in plain English or pseudocode. Discuss:
- The high-level steps of your algorithm
- Any helper functions you’ll need
- The time and space complexity analysis
“I’ll go with the min-heap approach. Here’s my plan:
1. Create a min-heap and insert the first k elements from the array.
2. For the remaining elements, if an element is larger than the smallest element in the heap, remove the smallest and add this new element.
3. After processing all elements, the smallest element in the heap is our kth largest element.
This gives us O(n log k) time complexity and O(k) space complexity.”
4. Implement the Code (10-15 minutes)
Now that you have a clear plan, translate it into code. As you write, continue to verbalize your thought process. If you’re unsure about syntax, mention it rather than going silent:
def findKthLargest(nums, k):
# Create a min heap
import heapq
heap = nums[:k]
heapq.heapify(heap) # O(k) operation
# Process remaining elements
for num in nums[k:]:
if num > heap[0]:
heapq.heappop(heap)
heapq.heappush(heap, num)
# The root of the heap is the kth largest element
return heap[0]
5. Review Your Solution (3-5 minutes)
After implementation, trace through your code with a simple example to verify correctness. Check for:
- Off-by-one errors
- Edge cases (empty arrays, k = 1, k = array length)
- Logical errors in your implementation
“Let me trace through this with a simple example. If nums = [3,2,1,5,6,4] and k = 2, we want the 2nd largest element, which is 5.
First, we create a min-heap with [3,2,1], so the heap is [1,2,3].
Then we process 5: Since 5 > 1, we remove 1 and add 5, so the heap becomes [2,3,5].
Next, we process 6: Since 6 > 2, we remove 2 and add 6, so the heap becomes [3,5,6].
Finally, we process 4: Since 4 > 3, we remove 3 and add 4, so the heap becomes [4,5,6].
The smallest element in the heap is 4, but that’s not correct — the 2nd largest should be 5.
Wait, I see the issue. We need to maintain a min-heap of size k, not 3. Let me fix that…”
This self-correction demonstrates your debugging skills and attention to detail.
6. Evaluate Alternative Approaches (2-3 minutes)
If time permits, discuss other approaches you considered and why your chosen solution was appropriate:
“While the QuickSelect algorithm could give us O(n) average time complexity, it has a worst-case O(n²). The heap solution guarantees O(n log k), which is very efficient when k is small compared to n. If memory was extremely constrained, we could consider in-place QuickSelect as an alternative.”
Practical Strategies to Maintain Your Process Under Pressure
Having a framework is essential, but executing it under pressure requires additional strategies:
Simulate Interview Conditions During Practice
The most effective preparation mimics actual interview conditions:
- Time yourself: Use a visible timer during practice sessions to get comfortable with time constraints.
- Practice with a live person: Mock interviews with peers or mentors provide realistic feedback and help you get comfortable explaining your thought process.
- Use a whiteboard or shared document: Practice in the medium you’ll use during actual interviews, whether that’s writing code on a whiteboard or typing in a shared document.
- Record yourself: Review recordings of your practice sessions to identify patterns in your communication and problem-solving approach.
Develop Stress Management Techniques
Controlling your physiological response to stress can significantly improve performance:
- Controlled breathing: Practice 4-7-8 breathing (inhale for 4 seconds, hold for 7, exhale for 8) before and during interviews to regulate your nervous system.
- Positive visualization: Mentally rehearse successful interview scenarios, including how you’ll handle challenging moments.
- Perspective shifting: Reframe the interview as an opportunity to solve interesting problems rather than a judgment of your worth.
- Physical preparation: Ensure you’re well-rested, hydrated, and comfortable before interviews to minimize additional stressors.
Develop a “Reset” Protocol for When You Get Stuck
Even with thorough preparation, you may encounter moments where your mind goes blank. Having a predefined recovery strategy can prevent a temporary setback from derailing your entire interview:
- Acknowledge the block: It’s okay to say, “I’m feeling a bit stuck. Let me take a step back and reconsider the problem.”
- Return to concrete examples: Working through specific examples can often reignite your problem-solving process.
- Simplify the problem: Try solving a simpler version of the problem first, then build up to the full solution.
- Ask targeted questions: Rather than asking for the solution, request a hint about a specific aspect you’re struggling with.
Common Problem Types and Their Structured Approaches
Different problem categories often require specific approaches. Familiarizing yourself with these patterns can help you quickly identify appropriate strategies:
Array and String Problems
For array and string manipulation problems, consider these common techniques:
- Two-pointer technique: Useful for problems involving searching, comparing, or manipulating elements from opposite ends or at different rates.
- Sliding window: Effective for finding subarrays or substrings that meet certain criteria.
- Prefix sums: Helpful for quickly calculating sums of subarrays.
Example problem approach for a sliding window problem:
def max_sum_subarray(nums, k):
# Initialize variables
window_sum = sum(nums[:k])
max_sum = window_sum
# Slide the window
for i in range(k, len(nums)):
# Add the incoming element and remove the outgoing element
window_sum = window_sum + nums[i] - nums[i - k]
max_sum = max(max_sum, window_sum)
return max_sum
Tree and Graph Problems
For tree and graph problems, structured traversal is key:
- BFS (Breadth-First Search): Ideal for finding shortest paths or level-order traversals.
- DFS (Depth-First Search): Well-suited for exploring all possibilities or paths.
- Recursion: Often the most elegant approach for tree problems.
Example approach for a tree problem:
def max_depth(root):
if not root:
return 0
# Recursive approach: the max depth is 1 (current level)
# plus the max depth of either subtree
left_depth = max_depth(root.left)
right_depth = max_depth(root.right)
return 1 + max(left_depth, right_depth)
Dynamic Programming Problems
Dynamic programming problems require a methodical approach:
- Identify the subproblems and define state variables
- Formulate the recurrence relation
- Determine the base cases
- Decide between bottom-up (tabulation) or top-down (memoization) implementation
Example approach for a DP problem:
def coin_change(coins, amount):
# Initialize dp array with amount+1 (which is greater than any possible answer)
dp = [amount + 1] * (amount + 1)
dp[0] = 0 # Base case: 0 coins needed to make amount 0
# Build up the dp array
for coin in coins:
for x in range(coin, amount + 1):
dp[x] = min(dp[x], dp[x - coin] + 1)
return dp[amount] if dp[amount] != amount + 1 else -1
Learning from Interview Failures
Even with the best preparation, you may not succeed in every interview. How you respond to these setbacks can determine your long-term success:
Conducting a Proper Post-Interview Analysis
After each interview, regardless of outcome, perform a thorough review:
- Document the problems asked and your solutions while they’re fresh in your mind
- Identify breakdown points in your problem-solving process
- Research optimal solutions to problems you struggled with
- Note any patterns in your performance across multiple interviews
Turning Rejection into Growth
Reframe interview rejections as valuable learning opportunities:
- Request feedback when possible, though not all companies provide detailed feedback
- Identify specific skills to improve based on your performance
- Set concrete goals for addressing weaknesses before your next interview
- Maintain perspective – even top engineers face rejection in the highly competitive tech hiring landscape
The Role of Mock Interviews in Strengthening Your Process
Mock interviews are perhaps the most effective way to reinforce your problem-solving process:
Finding Quality Mock Interview Partners
The right practice partners can significantly accelerate your improvement:
- Peer programming groups at your current workplace or school
- Online communities dedicated to interview preparation
- Professional mock interview services that connect you with experienced interviewers
- Friends or colleagues who work at companies you’re targeting
Structuring Effective Mock Interview Sessions
To maximize the value of mock interviews:
- Set clear goals for each session (e.g., focusing on verbalization, time management, or specific problem types)
- Simulate realistic conditions, including time constraints and environment
- Alternate roles as interviewer and candidate to develop perspective
- Provide and request specific feedback on both technical solutions and communication
Advanced Techniques for Seasoned Programmers
If you’re already comfortable with basic problem-solving but want to elevate your performance further:
Developing Intuition for Algorithmic Complexity
Beyond memorizing Big O notation, develop an intuitive understanding of efficiency:
- Visualize algorithm execution on different input sizes
- Benchmark common operations to develop a feel for their relative costs
- Practice quickly identifying bottlenecks in solutions
Mastering the Art of Problem Simplification
Complex problems become manageable when broken down effectively:
- Identify the core challenge within a complex problem
- Solve a simplified version first, then add complexity
- Look for opportunities to reuse solutions to known problems
Developing Your Own Problem-Solving Heuristics
Over time, develop personal rules of thumb that guide your approach:
- Create a mental checklist of techniques to consider for different problem types
- Develop pattern recognition for problem categories
- Build a repertoire of elegant solutions that can be adapted to new problems
Conclusion: Building Confidence Through Process
The difference between succeeding and failing in technical interviews often comes down not to knowledge, but to process. By developing and practicing a structured problem-solving framework, you can maintain clear thinking even under pressure.
Remember that interviewers are evaluating not just your technical skills, but your approach to solving problems you haven’t seen before. They want to hire colleagues who can break down complex challenges, communicate their thought process, and systematically work toward solutions.
The strategies outlined in this article will help you:
- Understand why your problem-solving process typically breaks down in interviews
- Implement a structured framework that withstands pressure
- Practice effectively to build confidence and consistency
- Recover quickly when you encounter difficulties
With deliberate practice and the right mindset, you can transform your interview performance from a source of anxiety to a demonstration of your true capabilities as a problem solver and software engineer.
Your next technical interview is an opportunity to showcase not just what you know, but how you think. By focusing on your process rather than just the outcome, you’ll not only perform better in interviews but also develop skills that will serve you throughout your career as a software engineer.