Why Your Problem-Solving Process Breaks Down Under Pressure (And How to Fix It)
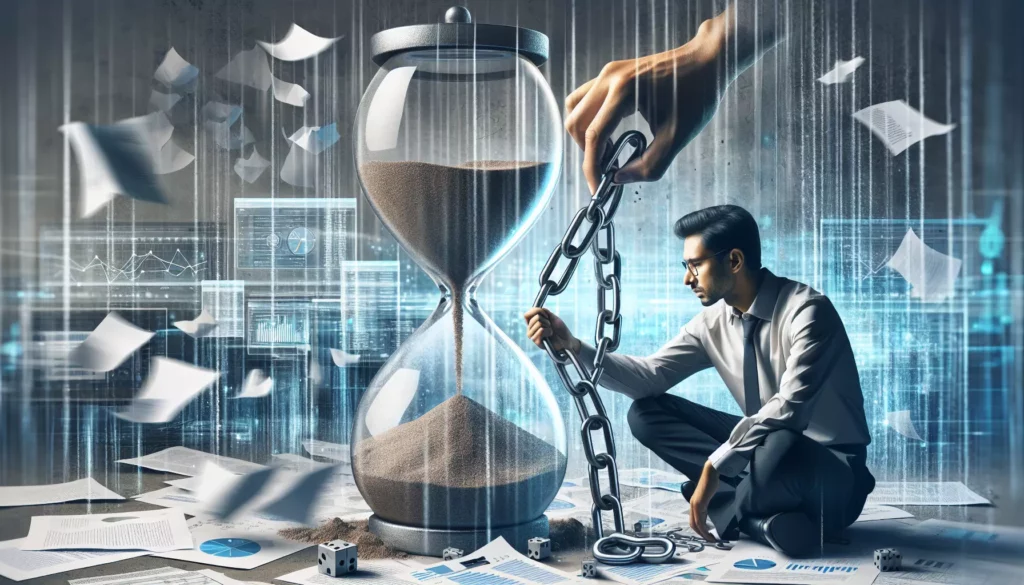
Have you ever blanked during a coding interview? Or found yourself staring at a problem during a timed assessment, your mind suddenly empty of all the algorithms and data structures you’ve spent months studying?
If so, you’re not alone. Even the most technically proficient programmers can crumble under pressure, watching helplessly as their carefully honed problem-solving process falls apart.
In this comprehensive guide, we’ll explore why your brain betrays you when the stakes are high, and more importantly, how you can build mental resilience to maintain your problem-solving abilities even when the pressure mounts.
The Science Behind Pressure-Induced Performance Decline
Before we dive into solutions, it’s important to understand what’s happening in your brain when you experience pressure during coding challenges or technical interviews.
The Working Memory Bottleneck
Working memory is your brain’s temporary workspace—the mental scratch pad where you manipulate information while solving problems. When solving a coding problem, your working memory juggles multiple pieces of information:
- The problem requirements
- Potential algorithms to apply
- Data structures you might use
- Edge cases to consider
- Code you’ve written so far
- Variables and their values
Research in cognitive psychology shows that working memory has limited capacity—most people can only hold about 4-7 chunks of information at once. Under pressure, this capacity shrinks even further.
The Anxiety-Performance Connection
When you feel pressured, your body activates its stress response. Your brain releases cortisol and adrenaline, preparing you for “fight or flight.” While this response was evolutionarily advantageous for physical threats, it’s counterproductive for cognitive tasks like coding.
Anxiety-related thoughts consume precious working memory resources:
“What if I can’t solve this?”
“They’re going to think I’m not qualified.”
“Everyone else would find this easy.”
“I’m running out of time!”
These intrusive thoughts compete with problem-solving processes for your limited mental resources, creating what psychologists call “cognitive interference.”
The Paradox of Expertise Under Pressure
Interestingly, research has found that experts can be particularly vulnerable to choking under pressure. When you become highly skilled at something, many of your processes become automated and unconscious. Under pressure, you might revert to consciously controlling these automated processes—a phenomenon called “explicit monitoring” or “paralysis by analysis.”
For a programmer, this might mean overthinking basic syntax or second-guessing your approach to a familiar algorithm pattern.
Common Ways Your Problem-Solving Process Breaks Down
Let’s examine the specific ways pressure can disrupt your coding process during technical interviews or assessments:
1. Rushing to Code Without a Plan
When the clock is ticking, there’s a natural tendency to jump straight into coding. This premature action often leads to:
- Implementing the first solution that comes to mind, even if it’s suboptimal
- Getting stuck in implementation details before understanding the problem
- Writing code that needs significant refactoring later
A candidate who rushes might start coding a brute force solution to a graph problem that clearly requires a more efficient approach like breadth-first search.
2. Analysis Paralysis
The opposite problem occurs when pressure causes you to overthink. You might:
- Get stuck evaluating too many possible approaches
- Obsess over finding the perfect solution instead of a working one
- Spend too much time on abstract planning without making concrete progress
For example, you might waste precious minutes debating between three different data structures, when any of them would work adequately for the problem at hand.
3. Tunnel Vision
Pressure can cause cognitive narrowing, where you fixate on one approach and fail to consider alternatives:
- Getting stuck on a particular algorithm even when it’s not yielding results
- Missing obvious simplifications or optimizations
- Failing to step back and reassess when progress stalls
A programmer experiencing tunnel vision might stubbornly try to make a dynamic programming approach work for a problem that has a simpler greedy solution.
4. Memory Retrieval Failures
Under pressure, accessing your knowledge becomes harder:
- Forgetting algorithms or data structures you’ve studied extensively
- Blanking on syntax for operations you use regularly
- Having difficulty recalling similar problems you’ve solved before
You might suddenly forget how to implement a binary search algorithm despite having coded it dozens of times in practice.
5. Communication Breakdown
Technical interviews assess not just your coding ability but also how you communicate your thinking:
- Struggling to articulate your approach clearly
- Forgetting to think aloud as you solve the problem
- Missing opportunities to demonstrate your reasoning process
Even if you’re making progress on the problem, failing to communicate effectively can significantly impact your interview performance.
Building a Pressure-Resistant Problem-Solving Framework
Now that we understand the problem, let’s develop a structured approach to maintain your problem-solving abilities under pressure.
The UPSET Method: A Framework for High-Pressure Problem Solving
I’ve developed a framework called UPSET that’s specifically designed to help programmers maintain their problem-solving capabilities under pressure:
- Understand the problem thoroughly
- Plan before coding
- Start with a simple solution
- Evaluate and optimize
- Test thoroughly
Let’s break down each step:
Understand: Pressure-Proof Your Problem Analysis
The foundation of effective problem-solving is a thorough understanding of the problem itself. Under pressure, it’s tempting to skim the problem statement and jump to conclusions. Resist this urge.
Practical techniques:
- Restate the problem in your own words, either verbally to your interviewer or in comments in your code
- Identify inputs and outputs explicitly, including their types, ranges, and constraints
- Work through examples methodically, including the provided test cases
- Create additional examples, especially edge cases (empty inputs, single elements, maximum values)
- Ask clarifying questions about any ambiguities in the problem statement
For example, if given a string manipulation problem:
// Problem: Find the longest palindromic substring
// Input: String s (1 <= s.length <= 1000)
// Output: Longest palindromic substring in s
// Example 1: Input: "babad" → Output: "bab" or "aba" (both valid)
// Example 2: Input: "cbbd" → Output: "bb"
// Edge cases to consider:
// - Single character: "a" → "a"
// - All same characters: "aaaaa" → "aaaaa"
// - No palindromes longer than 1: "abc" → "a" or "b" or "c"
Taking the time to understand the problem thoroughly creates a solid foundation for your solution and reduces anxiety by giving you clear parameters to work within.
Plan: Create a Pressure-Resistant Roadmap
Planning becomes even more critical under pressure. A good plan acts as an external memory aid, reducing the cognitive load on your working memory.
Practical techniques:
- Break down the problem into smaller subproblems
- Sketch your approach using pseudocode or flowcharts
- Consider multiple algorithms and their tradeoffs
- Estimate time and space complexity before implementing
- Verbalize your plan to your interviewer to get early feedback
For our palindromic substring example:
// Approach 1: Brute Force
// - Check all possible substrings
// - Time: O(n³), Space: O(1)
// Too slow for constraints (s.length up to 1000)
// Approach 2: Dynamic Programming
// - Create a table to store if substring[i..j] is palindrome
// - Time: O(n²), Space: O(n²)
// Better approach
// Approach 3: Expand Around Center
// - For each position, expand outward checking for palindromes
// - Time: O(n²), Space: O(1)
// Best approach for this problem
Having a clear plan reduces anxiety because you’re not making decisions on the fly. You can focus on implementing one step at a time rather than holding the entire solution in your head.
Start Simple: Combat Perfectionism Under Pressure
When under pressure, perfectionism can be your worst enemy. Starting with a simple, working solution gives you a foundation to build upon.
Practical techniques:
- Implement a brute force solution first if you can’t immediately see the optimal approach
- Use clear, readable code over clever, compact code
- Focus on correctness before efficiency
- Use helper functions to manage complexity
- Comment your code as you write it
For our palindrome example, we might start with the “expand around center” approach:
function longestPalindrome(s) {
if (!s || s.length < 1) return "";
let start = 0, maxLength = 1;
// Helper function to expand around center
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
// Found longer palindrome
if (right - left + 1 > maxLength) {
maxLength = right - left + 1;
start = left;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
// Expand for odd-length palindromes
expandAroundCenter(i, i);
// Expand for even-length palindromes
expandAroundCenter(i, i + 1);
}
return s.substring(start, start + maxLength);
}
Starting with a simple, working solution gives you a safety net. If time runs out, you have something functional to show. If time permits, you can optimize further.
Evaluate and Optimize: Systematic Improvement Under Pressure
Once you have a working solution, you can evaluate and optimize it. This step-by-step approach prevents the panic that can come from trying to find the perfect solution immediately.
Practical techniques:
- Analyze the current time and space complexity
- Identify bottlenecks in your algorithm
- Consider standard optimization techniques (caching, precomputation, more efficient data structures)
- Refactor incrementally, testing after each change
- Communicate tradeoffs to your interviewer
For our palindrome example, we might note that our solution is already optimal in terms of time complexity (O(n²)), but we could improve readability:
// Refactoring for clarity
function longestPalindrome(s) {
if (!s || s.length < 1) return "";
let result = "";
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
left--;
right++;
}
// Return the palindrome without the characters that broke the while loop
return s.substring(left + 1, right);
}
for (let i = 0; i < s.length; i++) {
// Check odd-length palindromes
const odd = expandAroundCenter(i, i);
// Check even-length palindromes
const even = expandAroundCenter(i, i + 1);
// Update result if we found a longer palindrome
if (odd.length > result.length) result = odd;
if (even.length > result.length) result = even;
}
return result;
}
The systematic approach to evaluation and optimization keeps you focused on concrete improvements rather than getting overwhelmed by the pressure to find the perfect solution immediately.
Test Thoroughly: Validate Under Pressure
Testing is often rushed or skipped entirely under pressure, leading to easily preventable errors. A systematic testing approach catches bugs and demonstrates thoroughness to interviewers.
Practical techniques:
- Test with the provided examples first
- Add edge cases (empty inputs, single elements, etc.)
- Trace through your code with a small example
- Use print statements or a debugger if available
- Check for off-by-one errors and boundary conditions
For our palindrome solution:
// Test cases
console.log(longestPalindrome("babad")); // Should output "bab" or "aba"
console.log(longestPalindrome("cbbd")); // Should output "bb"
// Edge cases
console.log(longestPalindrome("a")); // Should output "a"
console.log(longestPalindrome("aa")); // Should output "aa"
console.log(longestPalindrome("aaa")); // Should output "aaa"
console.log(longestPalindrome("")); // Should output ""
Thorough testing not only catches bugs but also demonstrates your commitment to quality code, even under pressure.
Mental Strategies for Maintaining Clarity Under Pressure
Beyond the UPSET framework, specific mental strategies can help you maintain clarity and focus during high-pressure coding situations.
Preparation Strategies
What you do before facing a high-pressure situation significantly impacts your performance during it.
1. Simulate Pressure in Practice
The most effective way to improve performance under pressure is to practice under similar conditions:
- Use timed practice sessions for coding problems
- Record yourself explaining solutions to simulate the interview experience
- Participate in competitive programming contests like LeetCode competitions or Codeforces rounds
- Conduct mock interviews with peers or using platforms that offer them
Research on “stress inoculation” shows that controlled exposure to stressors builds resilience when facing similar stressors later.
2. Develop Strong Fundamentals
Pressure exposes gaps in your knowledge. Build rock-solid fundamentals that you can rely on even when anxious:
- Master core data structures (arrays, linked lists, trees, graphs, hash tables)
- Understand common algorithms and their implementations (sorting, searching, graph traversal)
- Practice implementing these from scratch until they become second nature
- Create cheat sheets of common patterns and review them regularly
When fundamental knowledge is deeply ingrained, it remains accessible even when working memory is compromised by pressure.
3. Create Pre-Performance Routines
Athletes and performers use routines to center themselves before high-pressure situations:
- Develop a consistent pre-interview routine (e.g., reviewing key algorithms, doing a few easy problems, brief meditation)
- Practice deep breathing exercises to manage physiological arousal
- Use positive self-talk and affirmations to counter negative thoughts
- Visualize successfully working through difficult problems
These routines create a sense of familiarity and control, reducing the perceived threat of the high-pressure situation.
In-the-Moment Strategies
Even with thorough preparation, you’ll need strategies to manage pressure as it arises.
1. Recognize and Reframe Anxiety
Research shows that how you interpret physiological arousal affects performance:
- Acknowledge anxiety as a normal response (“I notice I’m feeling nervous”)
- Reframe anxiety as excitement (“My body is getting energized to tackle this challenge”)
- View stress responses as performance-enhancing rather than debilitating
- Remember past successes under similar conditions
This cognitive reappraisal can transform anxiety from a hindrance into a resource.
2. Use Strategic Time-Outs
When feeling overwhelmed, brief mental breaks can restore cognitive capacity:
- Take a deep breath and reset your focus
- Use the “micro-pause” technique: close your eyes for 5 seconds and clear your mind
- Communicate with your interviewer: “I’d like to take a moment to gather my thoughts”
- Use physical cues like stretching or adjusting your posture to break tension
These brief pauses prevent the spiral of anxiety and help maintain working memory capacity.
3. Externalize Your Thinking
Reduce the burden on working memory by using external aids:
- Write out your thoughts on paper or a whiteboard
- Draw diagrams to visualize the problem
- Use comments in your code to track your reasoning
- Verbalize your thinking process to the interviewer
Externalizing your thinking not only reduces cognitive load but also demonstrates your problem-solving approach to interviewers.
4. Employ the “What Would I Tell a Friend?” Technique
When stuck or panicking, ask yourself: “What advice would I give to a friend in this situation?”
- Creates psychological distance from the problem
- Activates your knowledge without the interference of anxiety
- Shifts perspective from threat to challenge
- Often reveals solutions that anxiety was blocking
This simple perspective shift can unlock your problem-solving abilities when they seem inaccessible under pressure.
Recovery Strategies: When Your Process Breaks Down Anyway
Even with the best preparation and frameworks, you may still experience moments where your problem-solving process falters. Having recovery strategies is crucial.
1. The Reset Protocol
When you find yourself stuck or spiraling, use this structured reset approach:
- Acknowledge the breakdown (“I notice I’m getting stuck here”)
- Pause and take three deep breaths
- Return to the problem statement and re-read it carefully
- Revisit your examples and test cases
- Consider a completely different approach
This protocol interrupts the anxiety cycle and gives you a fresh starting point.
2. The Simplification Strategy
When overwhelmed by a complex problem:
- Solve a simpler version of the problem first
- Remove constraints temporarily
- Focus on a subset of the functionality
- Build up incrementally from the simplified version
For example, if asked to find all permutations with certain constraints, first solve for generating all permutations, then add the constraints.
3. The Transparency Approach
Sometimes, the best recovery is honest communication:
- Admit when you’re stuck: “I’m finding myself going in circles here”
- Share your thought process: “Here’s where I’m getting confused…”
- Ask for a hint if appropriate: “Could you point me in the right direction?”
- Demonstrate resilience: “Let me try a different approach”
Interviewers often value transparency and resilience over perfect performance.
Building Long-Term Pressure Resilience
Beyond immediate strategies, developing pressure resilience is a long-term investment in your programming career.
1. Deliberate Practice with Feedback
The most effective way to build resilience is through structured practice:
- Regularly solve problems under time constraints
- Analyze your performance after each practice session
- Identify patterns in how you respond to pressure
- Seek feedback from peers or mentors
Track your progress over time, noting improvements in your ability to maintain clarity under pressure.
2. Develop a Growth Mindset About Pressure
Your beliefs about pressure significantly impact your response to it:
- View pressure situations as opportunities for growth
- Embrace challenges rather than avoiding them
- Learn from setbacks rather than being defined by them
- Focus on improvement rather than proving your worth
Research by Carol Dweck shows that a growth mindset leads to greater resilience and better performance under pressure.
3. Build Your Technical Foundation
The stronger your technical foundation, the more resilient you’ll be under pressure:
- Master fundamental data structures and algorithms
- Practice implementing common algorithms from scratch
- Develop pattern recognition for different problem types
- Build a repository of solved problems you can reference
When knowledge is deeply ingrained, it remains accessible even when working memory is compromised by pressure.
Conclusion: From Pressure Points to Performance
Problem-solving under pressure isn’t just about technical knowledge—it’s about maintaining your cognitive processes when stakes are high. The strategies outlined in this guide can help you transform pressure from a performance killer to a performance enhancer.
Remember that becoming pressure-resistant is a journey, not a destination. Each high-pressure situation is an opportunity to apply these strategies, learn from the experience, and improve for next time.
By understanding the cognitive mechanisms behind pressure-induced performance decline, implementing the UPSET framework, applying mental strategies for clarity, having recovery protocols ready, and building long-term resilience, you’ll be well-equipped to maintain your problem-solving abilities even in the most challenging situations.
The next time you face a technical interview, timed assessment, or high-stakes coding challenge, you won’t just survive the pressure—you’ll thrive under it.
Key Takeaways
- Pressure disrupts problem-solving by consuming working memory resources with anxiety-related thoughts
- The UPSET framework provides a structured approach to maintain problem-solving abilities under pressure
- Mental strategies like reframing anxiety, taking strategic time-outs, and externalizing thinking help maintain clarity
- Recovery protocols give you a path forward when your process breaks down
- Long-term resilience comes from deliberate practice, a growth mindset, and strong technical foundations
What pressure situations have you faced in your programming journey? What strategies have worked for you? Share your experiences and continue building your pressure resilience with each new challenge.