Why Your Practice Problems Aren’t Challenging Enough
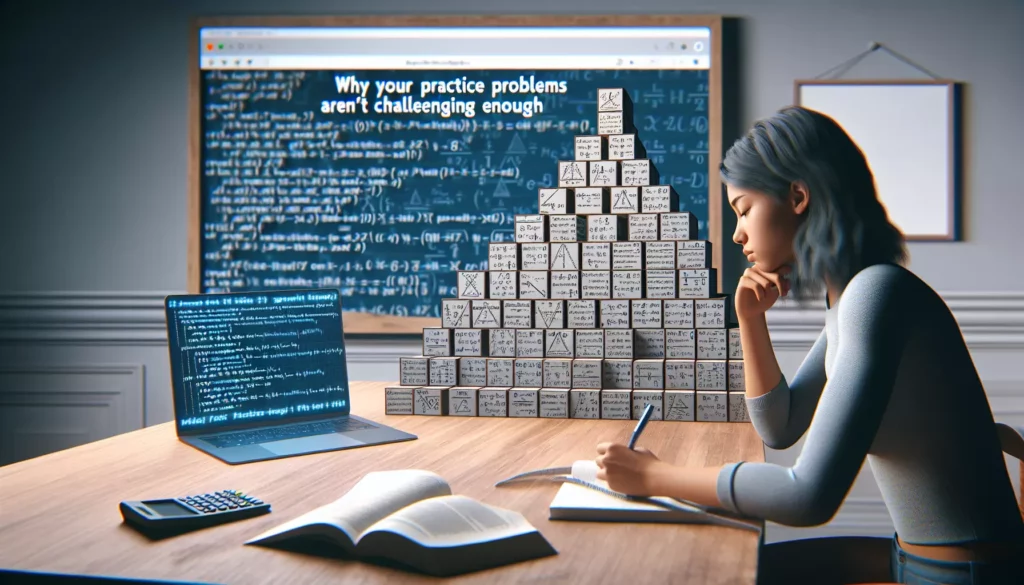
In the journey of becoming a proficient programmer, practice problems serve as the stepping stones that bridge the gap between theoretical knowledge and practical application. However, many aspiring developers find themselves in a peculiar predicament: despite solving numerous practice problems, they still struggle when faced with real coding challenges in technical interviews or on the job.
This disconnect often stems from a fundamental issue: the practice problems you’re working on simply aren’t challenging enough. They fail to push your boundaries, expand your thinking, or prepare you for the complex, multifaceted problems you’ll encounter in professional settings.
In this comprehensive guide, we’ll explore why your current practice regimen might be falling short, how to identify problems that truly challenge you, and strategies to level up your problem solving skills to meet the demands of top tech companies like Google, Amazon, and Meta.
The Comfort Zone Trap
When learning to code, there’s a natural tendency to gravitate toward problems that feel comfortable and familiar. You stick to what you know, solving variations of the same problem types using techniques you’ve already mastered. While this approach might boost your confidence, it creates a false sense of progress.
Think about it this way: if you can solve a problem within minutes without much deliberation or struggle, you’re likely not learning anything new. You’re simply reinforcing existing neural pathways rather than forging new ones.
Consider these signs that you might be trapped in your comfort zone:
- You solve most problems using the same handful of techniques
- You rarely need to look up new concepts or algorithms
- You finish problems quickly without much mental strain
- You haven’t felt genuinely stuck or confused in weeks
- Your solutions look remarkably similar across different problems
If these signs resonate with you, it’s time to acknowledge that your practice routine needs an overhaul. Growth happens at the edge of discomfort, not within the safety of what you already know.
The Gap Between Practice and Reality
Many coding platforms and educational resources offer problems that are artificially simplified. They’re designed to teach specific concepts in isolation, stripped of the messiness and complexity that characterize real world programming challenges.
While these simplified problems serve a purpose in early learning stages, they create a significant gap between practice and reality. Here’s how typical practice problems differ from what you’ll encounter in professional settings:
Problem Statement Clarity
Practice Problems: Clear, concise instructions with explicit input/output formats and constraints.
Reality: Ambiguous requirements that require clarification, hidden edge cases, and often no clear indication of what constitutes a “correct” solution.
Problem Scope
Practice Problems: Focus on a single algorithm or data structure.
Reality: Require integration of multiple concepts, algorithms, and data structures, often spanning different domains of computer science.
Evaluation Criteria
Practice Problems: Judged primarily on correctness, with time and space complexity as secondary considerations.
Reality: Evaluated on correctness, efficiency, code quality, maintainability, scalability, and sometimes even deployment considerations.
Data Scale
Practice Problems: Small, manageable input sizes that can be reasoned about easily.
Reality: Potentially massive datasets that require careful consideration of performance characteristics and resource constraints.
This gap explains why many developers who excel at coding challenges still struggle in technical interviews or when tasked with building real systems. They’ve been practicing a fundamentally different skill set than what’s actually required.
The Problem with Problem Difficulty Classification
Many coding platforms classify problems as “Easy,” “Medium,” or “Hard,” creating the illusion that working through these categories sequentially will prepare you for any coding challenge. However, this classification system has several inherent flaws:
Subjective Classification
What’s “Hard” for one person might be “Medium” or even “Easy” for another, depending on their background, experience, and familiarity with specific concepts. These classifications are inherently subjective and don’t account for individual learning paths.
One Dimensional Difficulty
Problems can be difficult in various ways: they might require complex algorithmic insights, careful implementation details, or clever mathematical observations. A single difficulty label fails to capture these nuances.
Artificial Progression
The progression from “Easy” to “Hard” problems often doesn’t mirror the progression of skills needed in professional development. Some “Easy” problems might involve concepts that are rarely used in practice, while some “Medium” problems might be more representative of everyday coding challenges.
Focus on Solutions, Not Process
These classifications emphasize finding the correct answer rather than developing the problem solving process that leads to that answer. In professional settings, the journey is often more important than the destination.
Instead of blindly following these classifications, it’s more effective to curate a set of problems that specifically target your weak areas and push the boundaries of your current capabilities.
Signs Your Practice Problems Aren’t Challenging Enough
How can you tell if your current practice regimen is too easy? Look for these warning signs:
Minimal Learning from Solutions
After solving a problem, you check the solution and find it’s nearly identical to yours. You rarely encounter new approaches or techniques in the solution sections.
Quick Solve Times
You consistently solve problems in minutes rather than hours. While efficiency is important, consistently quick solve times often indicate that the problems aren’t pushing your boundaries.
Limited Need for Research
You rarely need to look up new algorithms, data structures, or techniques to solve problems. Everything you need is already in your mental toolkit.
Predictable Problem Patterns
You can quickly categorize most problems into familiar patterns and apply template solutions with minor modifications.
Lack of Debugging Challenges
Your solutions work on the first or second try. You rarely encounter subtle bugs or edge cases that require careful debugging and analysis.
No Implementation Hurdles
Once you conceptualize a solution, implementing it is straightforward. You don’t struggle with translating your high level approach into working code.
If several of these signs apply to your practice routine, it’s a clear indication that you need to seek out more challenging problems that will actually advance your skills.
What Makes a Problem Truly Challenging?
To elevate your practice, it’s important to understand what constitutes a genuinely challenging problem. Here are the key characteristics:
Multiple Viable Approaches
Challenging problems often have several valid solutions, each with different tradeoffs in terms of time complexity, space complexity, and implementation difficulty. They force you to evaluate multiple approaches and make informed decisions.
Non Obvious Insights
The path to an efficient solution requires insights that aren’t immediately apparent. These insights might involve mathematical properties, clever algorithmic techniques, or creative problem reframing.
Integration of Multiple Concepts
Rather than focusing on a single data structure or algorithm, challenging problems require you to combine multiple concepts in novel ways. For example, a problem might involve both dynamic programming and binary search.
Subtle Edge Cases
The problem contains edge cases that aren’t explicitly mentioned but can cause solutions to fail if not carefully handled. Identifying and addressing these edge cases requires thorough analysis and testing.
Implementation Complexity
Even after understanding the high level approach, implementing the solution remains challenging due to intricate details, complex state management, or tricky algorithmic steps.
Optimization Requirements
The naive solution is obvious but far too inefficient. The problem requires significant optimization to meet time and space constraints, pushing you to find more sophisticated approaches.
By seeking out problems with these characteristics, you’ll ensure that your practice sessions contribute meaningfully to your growth as a developer.
Beyond Algorithmic Challenges: System Design and Real World Problems
While algorithmic problems form the foundation of coding interviews, they represent only one dimension of the challenges you’ll face as a professional developer. To truly prepare for success in the tech industry, you need to expand your practice to include:
System Design Problems
System design questions assess your ability to architect scalable, reliable, and maintainable systems. These problems are inherently open ended and require you to make tradeoffs between competing concerns like performance, cost, reliability, and development time.
Unlike algorithmic problems with clear “correct” answers, system design problems have multiple valid solutions depending on the specific requirements and constraints. They test your ability to:
- Break down complex systems into manageable components
- Choose appropriate technologies and architectural patterns
- Design for scalability, reliability, and performance
- Consider operational aspects like monitoring, deployment, and maintenance
- Communicate technical decisions effectively
Open Ended Implementation Tasks
Real world programming often involves implementing features or systems with incomplete or evolving requirements. Practicing open ended tasks helps develop the flexibility and judgment needed in professional settings:
- Build a simplified version of a familiar application (e.g., a basic Twitter clone)
- Implement a library or framework component from scratch
- Create a tool to solve a personal pain point
- Contribute to open source projects
These projects force you to make design decisions, manage complexity, and deal with the messy realities of software development that are often abstracted away in algorithmic problems.
Debugging and Maintenance Challenges
A significant portion of professional development involves understanding, debugging, and improving existing code rather than writing new code from scratch. To practice these skills:
- Debug intentionally broken code samples
- Optimize inefficient implementations
- Refactor poorly structured code
- Add features to existing codebases
These exercises develop the code comprehension and modification skills that are essential in professional environments but rarely tested in standard coding challenges.
Strategies for Finding Appropriately Challenging Problems
Now that we understand what makes a problem truly challenging, let’s explore strategies for finding practice material that will push your boundaries and accelerate your growth:
Strategic Platform Usage
Most coding platforms like LeetCode, HackerRank, and CodeForces offer filtering options beyond simple difficulty ratings. Use these filters to target specific areas of weakness:
- Filter by topics you’re less familiar with
- Look for problems with low acceptance rates
- Seek out problems tagged as interview questions from companies you’re interested in
- Focus on problems with high ratings from other users
Competitive Programming
Participating in coding competitions provides exposure to problems designed to challenge even experienced programmers. Platforms like CodeForces, AtCoder, and TopCoder host regular contests with problems of varying difficulty:
- Start with Division 2 or Educational Rounds if you’re new to competitive programming
- Review editorial solutions after contests to learn new techniques
- Participate regularly to expose yourself to diverse problem types
Interview Question Collections
Several resources compile actual interview questions from top tech companies:
- Books like “Cracking the Coding Interview” and “Elements of Programming Interviews”
- GitHub repositories of interview experiences
- Platforms like AlgoCademy that focus specifically on interview preparation
These questions tend to be more representative of the challenges you’ll face in actual interviews and often come with detailed explanations and approaches.
Open Source Contributions
Contributing to open source projects exposes you to real world codebases and challenges:
- Look for “good first issue” tags in repositories that interest you
- Start with documentation or testing contributions to familiarize yourself with the codebase
- Gradually tackle more complex features or bug fixes
Personal Project Challenges
Set ambitious goals for personal projects that push you to learn new technologies and techniques:
- Implement a complex algorithm or data structure from scratch
- Build a system with specific performance requirements
- Recreate a feature from a product you admire
Peer Programming and Code Reviews
Engaging with other developers can introduce you to challenges you wouldn’t encounter on your own:
- Participate in pair programming sessions
- Join coding communities that offer code reviews
- Explain your solutions to others to identify gaps in your understanding
How to Approach Truly Challenging Problems
Finding challenging problems is only half the battle. You also need an effective approach for tackling them without becoming discouraged. Here’s a structured methodology:
Set Realistic Time Expectations
Challenging problems take time. Allocate at least 1 2 hours per problem, and don’t be discouraged if you can’t solve it immediately. Professional developers often spend days or weeks on complex problems.
Follow a Systematic Process
- Understand the problem thoroughly: Restate it in your own words, identify inputs and outputs, clarify constraints, and generate example cases including edge cases.
- Explore brute force solutions: Even if inefficient, a brute force approach helps understand the problem space and may reveal insights for optimization.
- Look for patterns and simplifications: Can you solve a simpler version of the problem first? Are there patterns in the examples that suggest an approach?
- Consider standard techniques: Methodically evaluate whether common algorithms and data structures (sorting, binary search, dynamic programming, etc.) might apply.
- Implement carefully: Write clean, modular code even for complex solutions. This reduces bugs and makes debugging easier.
- Test rigorously: Verify your solution with multiple test cases, including edge cases and large inputs if possible.
Embrace Productive Struggle
The feeling of being stuck is uncomfortable but essential for growth. Instead of immediately looking up solutions:
- Take short breaks to reset your thinking
- Explain the problem out loud (rubber duck debugging)
- Draw diagrams or work through examples manually
- Break the problem into smaller subproblems
Use Hints Strategically
If you remain stuck after genuine effort:
- Look for progressive hints rather than complete solutions
- Try to proceed with minimal information
- Return to independent problem solving as soon as possible
Learn from Solutions
After solving a problem (or attempting it for a reasonable time):
- Study multiple solution approaches, not just the most efficient one
- Analyze the tradeoffs between different solutions
- Implement alternative approaches yourself rather than just reading them
- Take notes on new techniques or insights for future reference
Revisit Challenging Problems
True mastery requires reinforcement:
- Schedule revisits to problems that challenged you
- Try to solve them from scratch without referring to previous solutions
- Look for opportunities to further optimize or improve your approach
Creating a Progressive Challenge Ladder
Rather than randomly selecting difficult problems, create a structured progression that systematically increases challenge across multiple dimensions:
Concept Progression
Start with fundamental algorithms and data structures, then move to more advanced topics:
- Basic arrays, strings, and hash tables
- Searching and sorting algorithms
- Tree and graph traversal
- Dynamic programming fundamentals
- Advanced dynamic programming patterns
- Graph algorithms (shortest path, minimum spanning tree, etc.)
- Advanced data structures (segment trees, Fenwick trees, etc.)
- Specialized algorithms (computational geometry, string matching, etc.)
Implementation Complexity Progression
Gradually increase the implementation difficulty of problems:
- Problems with straightforward implementations
- Problems requiring careful handling of edge cases
- Problems with complex state management
- Problems requiring custom data structures
- Problems with intricate algorithmic implementations
Problem Scope Progression
Move from focused problems to those requiring integration of multiple concepts:
- Single algorithm/data structure problems
- Problems combining 2 3 standard techniques
- Multi step problems requiring different algorithms for each step
- Open ended problems with multiple valid approaches
- System design and architecture problems
Constraint Progression
Gradually tighten performance requirements:
- Problems where brute force solutions are acceptable
- Problems requiring O(n log n) or O(n) solutions
- Problems requiring sublinear time complexity
- Problems with strict space constraints
- Problems requiring optimization of constant factors
By advancing along these dimensions simultaneously, you create a comprehensive skill development path that prepares you for the full spectrum of programming challenges.
The Role of Deliberate Practice
Simply solving challenging problems isn’t enough for optimal growth. You need to engage in deliberate practice, a focused approach to improvement that involves:
Specific Skill Targeting
Identify particular skills or concepts you want to improve and select problems specifically designed to develop those areas. For example, if you struggle with dynamic programming, focus on problems that require increasingly complex DP approaches.
Immediate Feedback
Seek ways to get rapid feedback on your solutions, whether through automated tests, peer reviews, or comparison with reference implementations. This feedback helps identify misconceptions and inefficiencies in your approach.
Reflection and Analysis
After solving each problem, take time to reflect on your process:
- What was difficult about this problem?
- Which insights were key to the solution?
- How could I have reached the solution more efficiently?
- What new techniques or patterns did I learn?
- How might this approach apply to other problems?
Document these reflections in a learning journal to reinforce insights and create a personalized reference for future problem solving.
Consistency and Volume
Skill development requires consistent practice over time. Establish a regular schedule for problem solving, even if it’s just 1 2 problems per day. The cumulative effect of consistent practice far outweighs sporadic intensive sessions.
Balancing Challenge and Success
While this article emphasizes the need for more challenging problems, it’s important to strike a balance. If every problem you attempt leaves you completely stuck and demoralized, your learning efficiency will suffer.
The concept of “desirable difficulty” in learning science suggests that optimal learning occurs when tasks are challenging enough to require effort and create some struggle, but not so difficult that they lead to frustration and abandonment.
A good rule of thumb is the 70/30 approach:
- 70% of problems should stretch your abilities but be solvable with sustained effort
- 30% of problems should be at or slightly below your current level to build confidence and reinforce fundamentals
This balance keeps you in the “learning zone” where growth occurs most rapidly, while providing enough success experiences to maintain motivation.
Beyond Individual Problems: Building a Comprehensive Skill Set
While challenging individual problems are important, developing as a programmer requires a more holistic approach that includes:
Project Based Learning
Complement problem solving with project work that requires you to build complete systems. Projects develop skills that isolated problems can’t, such as:
- System architecture and component design
- Managing technical debt and code evolution
- Testing strategies for complex systems
- Deployment and operational considerations
Computer Science Fundamentals
Strengthen your theoretical foundation through structured study of:
- Algorithm analysis and complexity theory
- Formal data structure properties and guarantees
- Operating systems concepts
- Database theory and design
- Computer architecture and systems programming
This theoretical knowledge provides the context that makes problem solving more intuitive and solutions more robust.
Software Engineering Practices
Develop professional engineering habits that translate to workplace success:
- Writing clean, maintainable code
- Creating comprehensive test suites
- Documenting code and design decisions
- Using version control effectively
- Conducting code reviews
Communication and Collaboration
Technical skills alone aren’t sufficient in professional environments. Practice:
- Explaining technical concepts clearly
- Documenting your problem solving process
- Giving and receiving constructive feedback
- Working effectively in team programming contexts
Conclusion: Embracing the Challenge
If you’ve been diligently solving coding problems but still feel underprepared for technical interviews or real world development, the issue likely isn’t your dedication or intelligence—it’s the level of challenge in your practice regimen.
By deliberately seeking out problems that push your boundaries, integrating multiple concepts, require non obvious insights, and present implementation challenges, you create the conditions for genuine growth. Combined with a systematic approach to problem solving and deliberate practice techniques, this higher level of challenge will transform your capabilities as a programmer.
Remember that feeling challenged isn’t a sign of inadequacy; it’s a prerequisite for improvement. The most valuable learning happens when you’re working at the edge of your capabilities, making mistakes, struggling with new concepts, and ultimately expanding your problem solving toolkit.
So embrace the challenge. Seek out problems that make you think deeply, force you to learn new techniques, and occasionally leave you temporarily stuck. These are the problems that will prepare you for success in technical interviews and beyond.
Your future self—confidently solving complex problems that once seemed impossible—will thank you for it.