Why Your Portfolio Should Include One Challenging Project
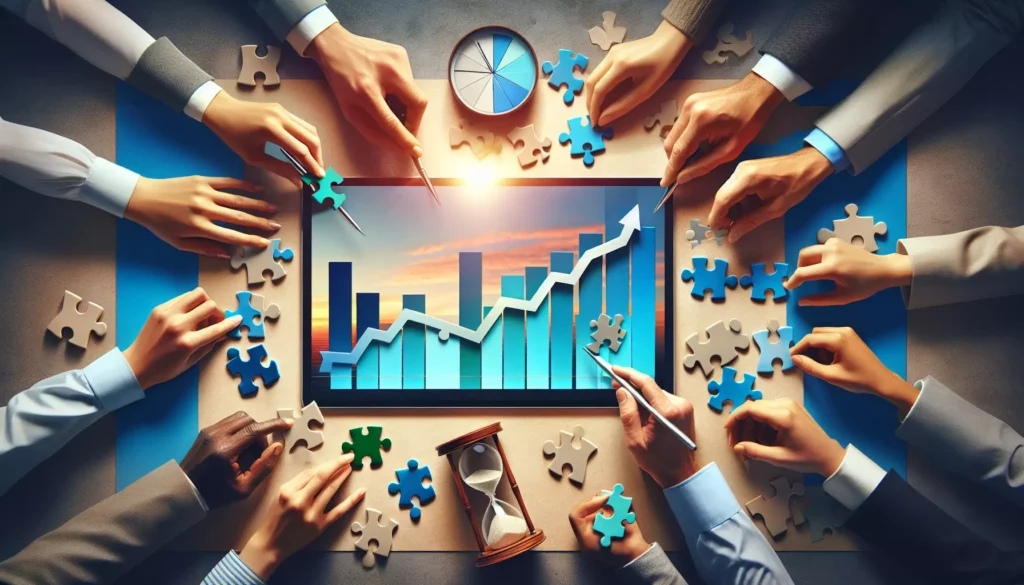
In the competitive world of software development and tech careers, having a strong portfolio is crucial. It’s your chance to showcase your skills, creativity, and problem-solving abilities to potential employers or clients. While it’s important to have a diverse range of projects in your portfolio, there’s a compelling argument for including at least one particularly challenging project. This article will explore why tackling a difficult project can significantly boost your portfolio and career prospects.
The Power of Challenge in Skill Development
Before we dive into the specifics of why you should include a challenging project in your portfolio, let’s consider the broader context of skill development in programming. Learning to code is a journey that requires continuous growth and adaptation. As you progress from a beginner to an intermediate and eventually an advanced programmer, the challenges you face become increasingly complex.
At AlgoCademy, we understand this progression intimately. Our platform is designed to guide learners through this journey, providing resources and tools that evolve with your skills. From basic coding tutorials to advanced algorithmic problem-solving, we emphasize the importance of tackling increasingly difficult challenges.
The Comfort Zone Trap
It’s human nature to gravitate towards tasks and projects that feel comfortable and familiar. In programming, this might mean sticking to languages or frameworks you know well or working on projects that don’t push your boundaries. While there’s value in reinforcing your existing skills, true growth often happens when you step outside your comfort zone.
Including a challenging project in your portfolio is a deliberate step out of this comfort zone. It’s a statement to yourself and others that you’re willing to take on difficult tasks and learn new things in the process.
Why Include a Challenging Project?
Now, let’s explore the specific reasons why your portfolio should feature at least one project that really stretched your abilities:
1. Demonstrates Problem-Solving Skills
Complex projects often involve intricate problems that require creative solutions. By showcasing a challenging project, you’re providing concrete evidence of your problem-solving capabilities. This is particularly important for roles at major tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), where problem-solving skills are highly valued.
For example, if your challenging project involved implementing a complex algorithm or optimizing a system for scalability, it shows that you can think critically and find solutions to non-trivial problems.
2. Shows Willingness to Learn
Difficult projects often require learning new technologies, frameworks, or concepts. By including such a project in your portfolio, you’re demonstrating your ability and willingness to acquire new skills. This is a trait that’s highly valued in the fast-paced tech industry, where technologies are constantly evolving.
For instance, if your challenging project involved learning a new programming language or working with a cutting-edge technology, it shows that you’re adaptable and eager to expand your skill set.
3. Highlights Perseverance
Challenging projects are often accompanied by setbacks and obstacles. By completing such a project, you’re showing that you have the perseverance to see things through, even when the going gets tough. This resilience is a valuable trait in any professional setting, but particularly in software development where complex problems are the norm.
4. Stands Out from the Crowd
In a sea of portfolios featuring similar projects, a uniquely challenging project can help you stand out. It gives you a talking point in interviews and can pique the interest of potential employers or clients.
5. Provides Depth to Your Portfolio
While it’s good to have a breadth of projects showing different skills, having at least one project with significant depth can be very impactful. It allows you to dive deep into the details of your problem-solving process, architecture decisions, and the complexities you navigated.
What Constitutes a Challenging Project?
The definition of a “challenging” project can vary depending on your skill level and experience. However, here are some characteristics that often define challenging projects:
- Requires learning new technologies or concepts
- Involves complex algorithms or data structures
- Addresses scalability or performance optimization
- Solves a real-world problem in a novel way
- Integrates multiple technologies or systems
- Pushes the boundaries of what you thought you could accomplish
At AlgoCademy, we encourage learners to take on projects that align with these characteristics. Our AI-powered assistance and step-by-step guidance can help you navigate the complexities of such projects, providing support when you need it most.
Examples of Challenging Projects
To give you a better idea of what a challenging project might look like, here are a few examples:
1. Building a Distributed System
Creating a distributed system, such as a simple distributed database or a distributed file system, can be an excellent challenging project. This type of project involves tackling complex issues like concurrency, fault tolerance, and network communication.
Here’s a simple example of how you might start implementing a basic distributed key-value store in Python:
import socket
import threading
import json
class DistributedKVStore:
def __init__(self, host, port):
self.host = host
self.port = port
self.data = {}
self.lock = threading.Lock()
def start(self):
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.bind((self.host, self.port))
s.listen()
while True:
conn, addr = s.accept()
threading.Thread(target=self.handle_client, args=(conn,)).start()
def handle_client(self, conn):
with conn:
while True:
data = conn.recv(1024)
if not data:
break
request = json.loads(data.decode())
if request["type"] == "GET":
response = self.get(request["key"])
elif request["type"] == "SET":
response = self.set(request["key"], request["value"])
conn.sendall(json.dumps(response).encode())
def get(self, key):
with self.lock:
return {"status": "success", "value": self.data.get(key)}
def set(self, key, value):
with self.lock:
self.data[key] = value
return {"status": "success"}
if __name__ == "__main__":
store = DistributedKVStore("localhost", 8000)
store.start()
This is just a starting point. A full implementation would need to handle things like data replication, consistency, and failure recovery.
2. Implementing a Machine Learning Pipeline
Creating an end-to-end machine learning pipeline can be a challenging and rewarding project. This might involve data collection, preprocessing, model training, evaluation, and deployment.
Here’s a simple example of a machine learning pipeline using scikit-learn:
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.ensemble import RandomForestClassifier
from sklearn.pipeline import Pipeline
from sklearn.metrics import accuracy_score
import numpy as np
# Assume X and y are your features and target variables
X = np.random.rand(100, 5)
y = np.random.randint(2, size=100)
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a pipeline
pipeline = Pipeline([
("scaler", StandardScaler()),
("rf_classifier", RandomForestClassifier())
])
# Fit the pipeline
pipeline.fit(X_train, y_train)
# Make predictions
y_pred = pipeline.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
A more complex version might include feature engineering, hyperparameter tuning, and model interpretability components.
3. Building a Compiler or Interpreter
Creating a compiler or interpreter for a simple programming language can be an extremely challenging but rewarding project. It involves understanding language design, parsing, and code generation.
Here’s a very basic example of how you might start building a simple interpreter in Python:
class Interpreter:
def __init__(self):
self.variables = {}
def parse(self, code):
return code.split()
def evaluate(self, parsed_code):
if parsed_code[0] == "PRINT":
return self.variables.get(parsed_code[1], "Undefined variable")
elif parsed_code[0] == "SET":
self.variables[parsed_code[1]] = parsed_code[2]
return f"Set {parsed_code[1]} to {parsed_code[2]}"
else:
return "Unknown command"
def run(self, code):
parsed = self.parse(code)
return self.evaluate(parsed)
interpreter = Interpreter()
print(interpreter.run("SET x 5"))
print(interpreter.run("PRINT x"))
print(interpreter.run("PRINT y"))
This is a very simplistic interpreter. A more complex version would include proper tokenization, parsing (perhaps using a context-free grammar), and more sophisticated execution logic.
How to Approach a Challenging Project
Taking on a challenging project can be daunting, but with the right approach, it can be a rewarding experience. Here are some tips to help you tackle your next challenging project:
1. Start with Research
Before diving into the code, take time to research the problem domain thoroughly. Understand the concepts, existing solutions, and potential pitfalls. This research phase will give you a solid foundation to build upon.
2. Break It Down
Complex projects can be overwhelming when viewed as a whole. Break the project down into smaller, manageable tasks. This makes the project less daunting and allows you to make steady progress.
3. Plan Your Architecture
Before writing any code, plan out your project’s architecture. Consider how different components will interact, what design patterns might be useful, and how you’ll structure your code for maintainability and scalability.
4. Start Small and Iterate
Begin with a minimal viable product (MVP) that implements the core functionality. Once you have this working, you can iteratively add features and refine your implementation.
5. Learn as You Go
Don’t be afraid to learn new things as you work on your project. Whether it’s a new language feature, a design pattern, or a entire new technology, embrace the learning opportunity.
6. Seek Help When Needed
When you encounter obstacles, don’t hesitate to seek help. This could be through online forums, documentation, or platforms like AlgoCademy that offer guidance and support.
7. Document Your Process
As you work on your project, document your thought process, the challenges you faced, and how you overcame them. This documentation will be valuable when you present your project in your portfolio or discuss it in interviews.
Showcasing Your Challenging Project
Once you’ve completed your challenging project, it’s important to showcase it effectively in your portfolio. Here are some tips:
1. Provide Context
Explain why you chose this particular project and what made it challenging. This gives viewers insight into your motivations and the project’s significance.
2. Highlight Key Features
Clearly outline the main features or functionalities of your project. Use screenshots, diagrams, or even short video demos if appropriate.
3. Discuss Technical Challenges
Don’t shy away from discussing the technical challenges you faced. Explain how you approached these challenges and what you learned in the process.
4. Share Your Code
If possible, provide a link to the project’s source code (e.g., on GitHub). This allows interested parties to dive deeper into your implementation.
5. Reflect on Learnings
Include a section where you reflect on what you learned from the project. This demonstrates your ability to grow and learn from challenges.
6. Discuss Potential Improvements
If there are aspects of the project you’d like to improve or expand upon, mention these. It shows that you’re thoughtful about your work and always looking to improve.
Conclusion
Including a challenging project in your portfolio is more than just a way to showcase your technical skills. It’s a testament to your problem-solving abilities, your willingness to learn, and your perseverance in the face of obstacles. These are qualities that are highly valued in the tech industry, particularly by major companies like those in the FAANG group.
At AlgoCademy, we encourage all our learners to push their boundaries and take on projects that challenge them. Our platform provides the resources, guidance, and support you need to tackle complex problems and develop the skills that will set you apart in your tech career.
Remember, the journey of a programmer is one of continuous learning and growth. By including a challenging project in your portfolio, you’re not just showcasing what you’ve already accomplished – you’re demonstrating your potential for future growth and your readiness to take on whatever challenges come your way in your programming career.
So, as you continue to build your portfolio, consider what challenging project you might take on next. It could be the key that unlocks new opportunities in your programming journey.