Why Your Perfect Code Fails in Production Environments
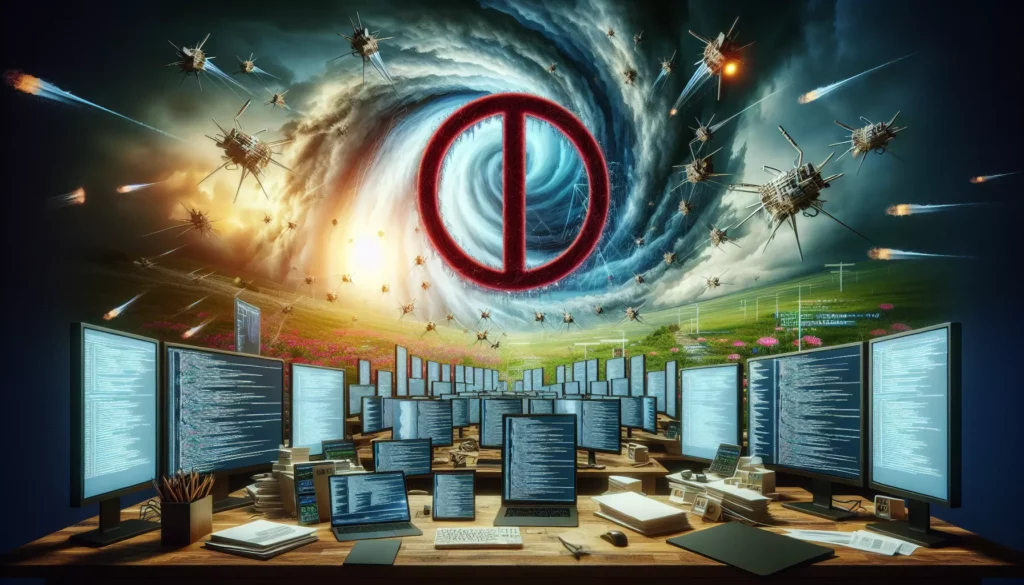
You’ve spent hours crafting what you believe is flawless code. It runs perfectly in your development environment, passes all unit tests, and even your colleagues have given it a thumbs up during code review. Yet, somehow, when deployed to production, it mysteriously fails. This scenario is all too familiar for developers at every experience level, from beginners to seasoned professionals.
In this comprehensive guide, we’ll explore the common reasons why code that works flawlessly in development environments can fail spectacularly when deployed to production. We’ll also provide practical strategies to prevent these issues and ensure your code performs reliably regardless of where it runs.
Table of Contents
- Understanding Environment Differences
- Common Causes of Production Failures
- Data Related Issues
- Performance and Scalability Problems
- Configuration and Dependency Management
- Security Considerations
- Tools and Techniques for Prevention
- Real World Case Studies
- Best Practices for Deployment
- Conclusion
Understanding Environment Differences
The fundamental issue behind many production failures is the difference between environments. Your development setup is likely significantly different from your production environment in numerous ways.
Local vs. Production: Key Differences
- Operating Systems: Developing on Windows but deploying to Linux? Differences in file path conventions, line endings, and case sensitivity can cause unexpected behaviors.
- Hardware Resources: Your development machine might have 32GB of RAM and 8 cores, while your production environment might be more constrained or distributed differently.
- Network Configurations: Local development often has minimal latency and perfect reliability, unlike real world networks.
- Database Instances: Production databases contain real, often messy data and experience actual load patterns.
- External Services: In development, you might mock external APIs, but in production, these services have rate limits, downtime, and other real world constraints.
Consider this common scenario: A developer creates a feature that works perfectly on their local machine but fails in production because they unconsciously relied on files being stored in a specific location that doesn’t exist in the production environment.
// Works in development because the path exists locally
const configPath = 'C:/Users/Developer/project/config.json';
const config = require(configPath);
// Better approach with relative paths
const configPath = path.join(__dirname, '../config.json');
const config = require(configPath);
Common Causes of Production Failures
Environment Variables and Configuration
One of the most common causes of the “works on my machine” syndrome is improper handling of environment variables and configuration.
In development, you might hardcode values or use default configurations, while production requires specific settings. Failure to properly manage these differences can lead to immediate failures when your code is deployed.
// Problematic approach
const databaseUrl = 'mongodb://localhost:27017/myapp';
// Better approach
const databaseUrl = process.env.DATABASE_URL || 'mongodb://localhost:27017/myapp';
Always use environment variables with sensible defaults for configuration. This allows different settings in different environments without code changes.
Timing and Race Conditions
Race conditions are particularly insidious because they may never appear during development testing but emerge under production load.
Consider this Node.js example where two operations might interfere with each other:
// Potential race condition
let userCount = 0;
app.post('/users', (req, res) => {
userCount++; // This could be problematic with concurrent requests
saveUser(req.body)
.then(() => res.status(201).send({ count: userCount }))
.catch(err => res.status(500).send(err));
});
// Better approach using atomic operations
app.post('/users', (req, res) => {
saveUser(req.body)
.then(() => incrementUserCount())
.then(count => res.status(201).send({ count }))
.catch(err => res.status(500).send(err));
});
Resource Limitations
In development, you rarely push your application to its limits. Production environments, however, reveal resource constraints quickly.
- Memory Leaks: Small memory leaks that go unnoticed in development can crash production servers that run for weeks or months.
- CPU Bound Operations: Computationally expensive operations might seem fast enough on your powerful development machine but cause timeouts in production.
- File Descriptors and Connection Pools: Failing to properly close connections or files can exhaust system resources over time.
// Potential memory leak in Node.js
const cache = {};
function processRequest(data) {
// Cache keeps growing without bounds
cache[data.id] = data;
// Process data...
}
// Better approach with a size-limited cache
const LRU = require('lru-cache');
const cache = new LRU({
max: 500, // Store max 500 items
maxAge: 1000 * 60 * 60 // Items expire after 1 hour
});
function processRequest(data) {
cache.set(data.id, data);
// Process data...
}
Data Related Issues
Database Differences
Database issues are a major source of production failures, especially when development and production databases differ significantly.
Schema Inconsistencies
Production databases often contain legacy data that doesn’t match your current schema expectations. A field that’s always populated in your test data might be null for some production records.
// Problematic approach
function processUser(user) {
return user.email.toLowerCase(); // Will fail if email is null
}
// Better approach
function processUser(user) {
return user.email ? user.email.toLowerCase() : '';
}
Data Volume Differences
Queries that return a few rows in development might return thousands in production, exposing inefficient algorithms or missing indexes.
// May work fine with small data sets but fail with large ones
async function getAllUserComments() {
const users = await db.users.find({});
// For each user, get all their comments - this creates N+1 query problem
for (const user of users) {
user.comments = await db.comments.find({ userId: user.id });
}
return users;
}
// Better approach with proper joins or aggregation
async function getAllUserComments() {
return db.users.aggregate([
{
$lookup: {
from: 'comments',
localField: 'id',
foreignField: 'userId',
as: 'comments'
}
}
]);
}
Edge Cases
Production data often contains edge cases that developers never anticipated:
- Unusually long strings that overflow UI elements or buffers
- Special characters that cause encoding issues
- Values at the extreme ends of allowed ranges
- Legacy data formats from previous versions of your application
Always validate input data and handle edge cases gracefully:
// Vulnerable to edge cases
function displayUsername(user) {
document.getElementById('username').textContent = user.name;
}
// Better approach
function displayUsername(user) {
const name = user.name || 'Anonymous';
const sanitizedName = name.substring(0, 50); // Prevent overly long names
document.getElementById('username').textContent = sanitizedName;
}
Performance and Scalability Problems
Load Testing Inadequacies
Many applications are never properly load tested before deployment. When real users hit your system, patterns emerge that weren’t visible during development:
- Concurrent users causing lock contention
- Spikes in traffic overwhelming resources
- Slow degradation as caches fill up
Implement proper load testing with tools like JMeter, Locust, or k6 to simulate realistic user behavior before deployment.
N+1 Query Problems
This common performance issue occurs when code makes one database query, then makes additional queries for each result from the first query.
// N+1 query problem in Express/Sequelize
app.get('/articles', async (req, res) => {
const articles = await Article.findAll();
// This makes a separate query for each article
for (const article of articles) {
article.author = await User.findByPk(article.authorId);
}
res.json(articles);
});
// Better approach
app.get('/articles', async (req, res) => {
const articles = await Article.findAll({
include: [{
model: User,
as: 'author'
}]
});
res.json(articles);
});
Caching Issues
Caching is a double edged sword. While it can dramatically improve performance, it also introduces complexity and potential for inconsistency.
Common caching issues in production include:
- Cache invalidation failures leading to stale data
- Cache stampedes when many requests hit an empty cache simultaneously
- Memory pressure from overly aggressive caching
// Naive caching approach
const cache = {};
async function getUserById(id) {
if (cache[id]) return cache[id];
const user = await db.users.findOne({ id });
cache[id] = user; // Cache forever - never updates if user changes
return user;
}
// Better approach with TTL and invalidation
const NodeCache = require('node-cache');
const cache = new NodeCache({ stdTTL: 300 }); // 5 minute expiration
async function getUserById(id) {
const cacheKey = `user:${id}`;
const cachedUser = cache.get(cacheKey);
if (cachedUser) return cachedUser;
const user = await db.users.findOne({ id });
cache.set(cacheKey, user);
return user;
}
// Function to invalidate cache when user is updated
function invalidateUserCache(id) {
cache.del(`user:${id}`);
}
Configuration and Dependency Management
Dependency Version Mismatches
One of the most common issues occurs when dependencies in production don’t match what you used during development.
This can happen due to:
- Using
^
or~
in version specifiers, allowing minor updates - Not using lock files (
package-lock.json
,yarn.lock
, etc.) - Different package managers or Node.js versions between environments
Always use lock files and exact versions for critical dependencies:
// package.json with potential version drift
{
"dependencies": {
"express": "^4.17.1", // Could update to any 4.x version
"mongoose": "~5.9.0" // Could update to any 5.9.x version
}
}
// Better approach with exact versions
{
"dependencies": {
"express": "4.17.1",
"mongoose": "5.9.0"
}
}
Missing Dependencies
Sometimes code works locally because you have globally installed packages that aren’t in your project dependencies.
// Using a package that might be installed globally but not listed in dependencies
const moment = require('moment');
// Fix: Add to package.json
// npm install moment --save
Environment Specific Configuration
Different environments often require different configurations. Hardcoded values will cause problems when moving between environments.
// Bad: Hardcoded configuration
const config = {
port: 3000,
database: 'mongodb://localhost:27017/myapp',
apiKey: 'development-key-1234'
};
// Better: Environment-based configuration
const config = {
port: process.env.PORT || 3000,
database: process.env.DATABASE_URL || 'mongodb://localhost:27017/myapp',
apiKey: process.env.API_KEY || 'development-key-1234',
environment: process.env.NODE_ENV || 'development'
};
Security Considerations
Exposed Secrets
Hardcoded credentials or API keys in source code can lead to security breaches when code is deployed.
// Dangerous: Credentials in source code
const dbConnection = mysql.createConnection({
host: 'production-db.example.com',
user: 'admin',
password: 'super-secret-password'
});
// Better: Environment variables
const dbConnection = mysql.createConnection({
host: process.env.DB_HOST,
user: process.env.DB_USER,
password: process.env.DB_PASSWORD
});
CORS and Security Headers
Development environments often have relaxed security settings that become problematic in production.
// Overly permissive CORS in development
app.use(cors({ origin: '*' }));
// Better: Environment-specific CORS
const allowedOrigins = process.env.NODE_ENV === 'production'
? ['https://myapp.com', 'https://admin.myapp.com']
: ['http://localhost:3000'];
app.use(cors({
origin: function(origin, callback) {
if (!origin || allowedOrigins.includes(origin)) {
callback(null, true);
} else {
callback(new Error('Not allowed by CORS'));
}
}
}));
Input Validation
Insufficient input validation is a common source of security vulnerabilities:
// Dangerous: No input validation
app.post('/api/users', (req, res) => {
db.users.create(req.body)
.then(user => res.json(user));
});
// Better: Validate input
const Joi = require('joi');
const userSchema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
email: Joi.string().email().required(),
age: Joi.number().integer().min(18).max(120)
});
app.post('/api/users', (req, res) => {
const { error, value } = userSchema.validate(req.body);
if (error) {
return res.status(400).json({ error: error.details[0].message });
}
db.users.create(value)
.then(user => res.json(user));
});
Tools and Techniques for Prevention
Containerization
Containers like Docker help ensure consistency between environments by packaging your application with its dependencies and configuration.
# Example Dockerfile
FROM node:14-alpine
WORKDIR /app
COPY package*.json ./
RUN npm ci --only=production
COPY . .
ENV NODE_ENV=production
EXPOSE 3000
CMD ["node", "server.js"]
Infrastructure as Code
Tools like Terraform, AWS CloudFormation, or Pulumi allow you to define your infrastructure in code, making it reproducible and consistent.
// Example Terraform configuration for AWS
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "WebServer"
}
user_data = <<-EOF
#!/bin/bash
echo "Hello, World" > index.html
nohup busybox httpd -f -p 8080 &
EOF
}
Feature Flags
Feature flags allow you to gradually roll out features or disable problematic code without redeployment.
// Simple feature flag implementation
const features = {
newLoginSystem: process.env.FEATURE_NEW_LOGIN === 'true',
betaReporting: process.env.FEATURE_BETA_REPORTING === 'true'
};
function authenticateUser(credentials) {
if (features.newLoginSystem) {
return newAuthSystem(credentials);
} else {
return legacyAuthSystem(credentials);
}
}
Comprehensive Testing
Implement a robust testing strategy including:
- Unit Tests: Test individual functions and components
- Integration Tests: Test how components work together
- End-to-End Tests: Test complete user flows
- Load Tests: Test performance under expected and peak loads
- Chaos Tests: Test resilience by deliberately introducing failures
// Example Jest unit test
test('calculateTotal adds items correctly', () => {
const cart = [
{ price: 10, quantity: 2 },
{ price: 15, quantity: 1 }
];
expect(calculateTotal(cart)).toBe(35);
});
// Example integration test with Supertest
const request = require('supertest');
const app = require('../app');
describe('User API', () => {
it('should create a new user', async () => {
const res = await request(app)
.post('/api/users')
.send({
username: 'testuser',
email: 'test@example.com'
});
expect(res.statusCode).toEqual(201);
expect(res.body).toHaveProperty('id');
});
});
Monitoring and Observability
Implement comprehensive monitoring to catch issues before or soon after they impact users:
- Application Performance Monitoring (APM): Tools like New Relic, Datadog, or Elastic APM
- Error Tracking: Sentry, Rollbar, or Bugsnag
- Logging: Centralized logging with ELK stack or similar
- Metrics: Prometheus, Grafana for visualizing system performance
// Example with Winston logger
const winston = require('winston');
const logger = winston.createLogger({
level: process.env.LOG_LEVEL || 'info',
format: winston.format.json(),
defaultMeta: { service: 'user-service' },
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
// In production, also log to console
if (process.env.NODE_ENV === 'production') {
logger.add(new winston.transports.Console({
format: winston.format.simple()
}));
}
// Usage
function processOrder(order) {
logger.info('Processing order', { orderId: order.id });
try {
// Process order...
logger.info('Order processed successfully', { orderId: order.id });
} catch (error) {
logger.error('Order processing failed', {
orderId: order.id,
error: error.message,
stack: error.stack
});
throw error;
}
}
Real World Case Studies
Case Study 1: The Database Connection Pool
A team deployed a Node.js application that worked perfectly in development but started crashing in production after a few hours.
The Issue: The application was creating new database connections for each request without properly closing them or using a connection pool. In development with minimal traffic, this wasn’t noticeable, but in production it quickly exhausted available connections.
The Solution: Implementing a proper connection pool with appropriate sizing:
// Before: Creating new connections for each request
function handleRequest(req, res) {
const db = mysql.createConnection({
host: 'database',
user: 'user',
password: 'password'
});
db.query('SELECT * FROM data', (err, results) => {
res.json(results);
// Connection never properly closed
});
}
// After: Using a connection pool
const pool = mysql.createPool({
host: 'database',
user: 'user',
password: 'password',
connectionLimit: 10
});
function handleRequest(req, res) {
pool.query('SELECT * FROM data', (err, results) => {
res.json(results);
// Connection automatically returned to pool
});
}
Case Study 2: The Timezone Bug
A financial application calculated daily reports correctly in development but produced incorrect results in production.
The Issue: The developer’s machine was set to EST timezone, while the production server used UTC. The code didn’t explicitly handle timezone differences, causing reports to be generated with incorrect date boundaries.
The Solution: Explicitly handling timezones with a library like moment-timezone:
// Before: Implicit timezone dependency
function generateDailyReport(date) {
const startOfDay = new Date(date);
startOfDay.setHours(0, 0, 0, 0);
const endOfDay = new Date(date);
endOfDay.setHours(23, 59, 59, 999);
return getTransactions(startOfDay, endOfDay);
}
// After: Explicit timezone handling
const moment = require('moment-timezone');
function generateDailyReport(date, timezone = 'America/New_York') {
const startOfDay = moment.tz(date, timezone).startOf('day').toDate();
const endOfDay = moment.tz(date, timezone).endOf('day').toDate();
return getTransactions(startOfDay, endOfDay);
}
Case Study 3: The Memory Leak
A Node.js API would run fine for a few days in production before gradually slowing down and eventually crashing with an “out of memory” error.
The Issue: The application was caching results without any eviction strategy, causing memory usage to grow unbounded.
The Solution: Implementing a proper caching strategy with TTL and size limits:
// Before: Unbounded cache
const cache = {};
function fetchUserData(userId) {
if (cache[userId]) {
return Promise.resolve(cache[userId]);
}
return api.getUser(userId)
.then(userData => {
cache[userId] = userData; // Cache grows forever
return userData;
});
}
// After: Bounded LRU cache
const LRU = require('lru-cache');
const userCache = new LRU({
max: 1000, // Store max 1000 users
maxAge: 1000 * 60 * 60 // Cache for 1 hour
});
function fetchUserData(userId) {
if (userCache.has(userId)) {
return Promise.resolve(userCache.get(userId));
}
return api.getUser(userId)
.then(userData => {
userCache.set(userId, userData);
return userData;
});
}
Best Practices for Deployment
Deployment Checklist
Create a deployment checklist to ensure consistency:
- Run comprehensive test suite
- Verify environment variables are properly set
- Check database migrations and schema changes
- Validate third party service credentials
- Ensure monitoring is configured
- Verify backup systems are operational
- Plan rollback strategy in case of issues
Blue Green Deployments
Blue green deployments involve maintaining two identical production environments:
- One environment (blue) is currently live
- Deploy to the other environment (green)
- Test the green environment
- Switch traffic from blue to green
- Keep blue as a fallback in case issues arise
This approach minimizes downtime and provides a quick rollback option.
Canary Releases
With canary releases, you gradually roll out changes to a small subset of users before deploying to everyone:
- Deploy the new version to a small portion of your infrastructure
- Route a small percentage of users to the new version
- Monitor for issues
- Gradually increase traffic to the new version if no issues are found
- Roll back quickly if problems emerge
Automated Deployments
Implement CI/CD (Continuous Integration/Continuous Deployment) pipelines to automate the testing and deployment process:
# Example GitHub Actions workflow
name: Deploy
on:
push:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: '14'
- run: npm ci
- run: npm test
deploy:
needs: test
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Deploy to production
uses: some-deployment-action@v1
with:
api-key: ${{ secrets.DEPLOY_API_KEY }}
Conclusion
The gap between development and production environments is one of the most challenging aspects of software development. By understanding the common pitfalls and implementing robust strategies to address them, you can significantly reduce the likelihood of seeing your “perfect” code fail in production.
Remember these key principles:
- Assume Differences: Always assume your production environment differs from development in ways you haven’t anticipated.
- Test Realistically: Test with production like data volumes, traffic patterns, and constraints.
- Monitor Everything: Implement comprehensive monitoring and alerting to catch issues early.
- Design for Failure: Assume components will fail and design your system to be resilient.
- Automate Deployments: Reduce human error through automation and consistent processes.
By applying these practices, you’ll build more reliable systems that work as expected regardless of the environment they’re running in. The gap between “works on my machine” and “works in production” will narrow, leading to more successful deployments and fewer late night emergency fixes.
Remember that even the most experienced developers encounter production issues. The difference is in how prepared you are to prevent, detect, and resolve them quickly. Building this mindset and these skills is what separates good developers from great ones in real world application development.