Why Your Perfect Architecture Isn’t Practical: The Reality of Software Design
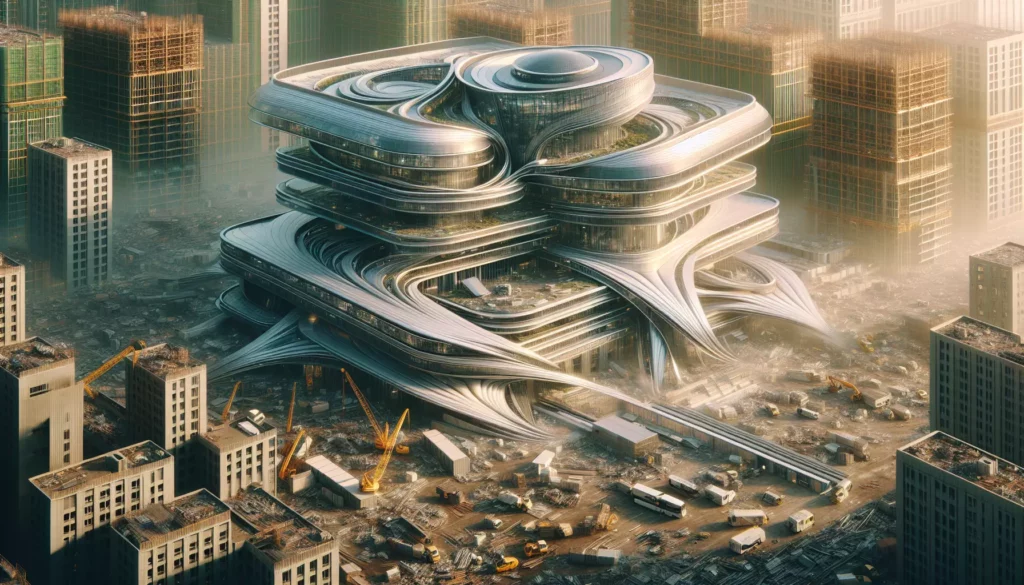
Every software developer has experienced that moment of architectural euphoria. You diagram an elegant system on a whiteboard, with perfectly separated concerns, beautifully abstracted interfaces, and theoretically infinite scalability. It feels like you’ve solved software engineering itself.
Then reality hits.
In this article, we’ll explore why those perfect architectures often fail in practice, what trade-offs actually matter, and how to design systems that balance theoretical purity with practical constraints. This is especially important for those preparing for technical interviews or working at major tech companies, where architectural decisions have real consequences.
The Myth of the Perfect Architecture
Software architecture is often taught as if there were a platonic ideal to strive for. Clean Architecture, Hexagonal Architecture, Microservices, Event-Driven Systems—each presented as a solution to all your problems. In bootcamps and university courses, instructors draw neat diagrams with boxes and arrows, suggesting that if you follow their pattern, your system will be maintainable, scalable, and bug-free.
The reality? Every architecture involves trade-offs.
Consider this example: a junior developer might design a microservices architecture for a simple application because they’ve read about its scalability benefits, only to find themselves drowning in deployment complexity, network latency issues, and distributed debugging nightmares—all for an application that could have been a monolith handling 100 requests per minute.
As the famous quote often attributed to Donald Knuth states: “Premature optimization is the root of all evil.” The same applies to premature architectural complexity.
When Perfect Becomes the Enemy of Practical
Let’s explore several ways that pursuit of the “perfect” architecture can lead us astray:
1. Overengineering for Future Scale
One of the most common mistakes is designing for a scale you don’t need yet. Companies like Google, Amazon, and Facebook have architectures designed to handle billions of users and petabytes of data. But their solutions are responses to specific problems they encountered at scale.
Consider Instagram’s architecture evolution. When they launched in 2010, they were a small Python monolith. They didn’t start with a complex microservice architecture, Kubernetes clusters, and globally distributed databases. They scaled their architecture as they grew.
The practical approach is to design for perhaps 10x your current scale, not 1000x. You can evolve your architecture as you grow, and the problems you anticipate might not be the ones you actually encounter.
2. Excessive Abstraction
Abstraction is a powerful tool in software design, but excessive abstraction leads to what’s often called “abstraction inversion”—where you create so many layers that you end up writing more code to work around your abstractions than you would have without them.
Consider this Java example:
// Excessive abstraction
public interface IDataFetcherFactory {
IDataFetcher createFetcher(IDataSource source);
}
public interface IDataFetcher {
IDataResult fetch(IQuery query);
}
public interface IDataResult {
<T> T getResult();
}
// Versus a more practical approach
public class DataService {
public <T> T fetchData(String query, Class<T> resultType) {
// Implementation here
}
}
The first approach might seem more “architecturally pure,” but it creates cognitive overhead, makes debugging harder, and often results in developers creating workarounds to get things done.
3. Ignoring Team Capabilities
A perfect architecture on paper becomes impractical if your team can’t implement or maintain it. Consider adopting a complex event-sourcing architecture when your team has primarily worked with traditional CRUD applications. The learning curve might be so steep that productivity plummets.
Architecture should match not just technical requirements but also team capabilities and organizational context. Sometimes a “good enough” architecture that your team understands is better than a theoretically superior one that becomes a maintenance nightmare.
4. Forgetting About Time and Budget Constraints
In the real world, projects have deadlines and budgets. A perfect architecture might take months to implement, while a pragmatic approach could deliver value to users in weeks.
This doesn’t mean embracing technical debt indiscriminately, but rather making conscious decisions about where to invest architectural effort and where to accept compromise.
Real-World Architecture Case Studies
Let’s examine some real-world examples where practical compromises led to successful outcomes:
Amazon’s Service-Oriented Architecture
Amazon’s transition to a service-oriented architecture is often cited as a microservices success story. However, their approach was pragmatic, not dogmatic:
- They didn’t rewrite everything at once; they incrementally decomposed their monolith
- They allowed teams to choose their own technologies where appropriate
- They focused on clear service boundaries and interfaces rather than enforcing a particular implementation style
Amazon’s “two-pizza team” philosophy (teams small enough to be fed by two pizzas) drove their architectural decisions more than abstract principles. Their architecture served their organizational needs, not the other way around.
Spotify’s “Accidental” Architecture
Spotify’s famous “Squads and Tribes” model influenced their technical architecture. While often presented as a carefully planned microservices implementation, former Spotify engineers have admitted it was more evolutionary than revolutionary.
Their architecture grew organically to solve specific problems, with squads making local decisions that sometimes led to inconsistencies across the platform. But this pragmatic approach allowed them to move quickly and adapt to changing requirements.
Netflix’s Chaos Engineering
Netflix embraced the reality that perfect reliability is impossible at scale. Rather than designing a theoretically perfect system, they built tools like Chaos Monkey to randomly take down production instances, forcing their architecture to be resilient to failure.
This practical approach acknowledges that failures will happen and designs systems to be resilient rather than perfect. It’s a fundamentally different philosophy than striving for a flawless architecture.
Practical Architecture: A Better Approach
So how should we approach architecture if perfection is unattainable? Here are some principles for practical architecture:
1. Start With Clear Requirements and Constraints
Before designing any architecture, be crystal clear about:
- Functional requirements: What must the system do?
- Non-functional requirements: How well must it do it? (performance, security, scalability)
- Constraints: Budget, timeline, team skills, existing systems
These should drive your architectural decisions, not abstract principles or the latest trends.
2. Embrace Evolutionary Architecture
Rather than trying to design the perfect architecture upfront, embrace an evolutionary approach:
- Start with the simplest architecture that could work
- Build in “architectural fitness functions” to evaluate how well the architecture is meeting requirements
- Refactor and evolve as requirements change and problems emerge
This approach recognizes that requirements will change, and no initial design will be perfect.
3. Focus on Boundaries and Interfaces
The most important architectural decisions are often about boundaries and interfaces between components, not the internal implementation details:
// Define clear interfaces between components
public interface PaymentProcessor {
PaymentResult processPayment(PaymentRequest request);
}
// Different implementations can exist behind this interface
public class StripePaymentProcessor implements PaymentProcessor {
@Override
public PaymentResult processPayment(PaymentRequest request) {
// Stripe-specific implementation
}
}
public class PayPalPaymentProcessor implements PaymentProcessor {
@Override
public PaymentResult processPayment(PaymentRequest request) {
// PayPal-specific implementation
}
}
With clear boundaries, individual components can evolve independently as long as they honor their contracts.
4. Prioritize Developer Experience
A practical architecture considers how developers will work with it day-to-day:
- How easily can new team members understand it?
- How quickly can developers make changes and see results?
- How effectively can they debug issues?
Sometimes a slightly less “pure” architecture that’s more developer-friendly is the better choice. For example, a monolith with well-defined modules might be preferable to microservices for a small team that needs to make frequent, coordinated changes across the codebase.
5. Make Trade-offs Explicit
Every architectural decision involves trade-offs. Make these explicit:
// Architecture Decision Record (ADR) example
# Decision: Use a message queue for order processing
## Context
Our order processing system needs to handle spikes in traffic during sales events.
## Decision
We will use RabbitMQ to queue orders for asynchronous processing.
## Consequences
* (+) Can handle traffic spikes without scaling the entire system
* (+) Order processing can continue if downstream systems are temporarily unavailable
* (-) Introduces additional operational complexity
* (-) Requires handling of message failures and retries
* (-) Increases end-to-end latency for order confirmation
Documenting trade-offs helps future maintainers understand why decisions were made and when they might need to be revisited.
Common Architectural Trade-offs
Let’s examine some common trade-offs in software architecture and how to approach them practically:
Monolith vs. Microservices
The monolith vs. microservices debate often ignores the practical realities:
Monolith | Microservices |
---|---|
Simpler deployment and testing | More complex deployment and testing |
Higher coupling between components | Lower coupling between services |
Easier local development | More complex local development |
Limited technology diversity | Freedom to use different technologies |
Vertical scaling (bigger machines) | Horizontal scaling (more machines) |
The practical approach? Many successful companies start with a modular monolith and extract microservices only when specific benefits (like independent scaling or team autonomy) justify the added complexity.
Synchronous vs. Asynchronous Communication
Another common trade-off is between synchronous (request/response) and asynchronous (event-based) communication:
// Synchronous approach
public Order createOrder(OrderRequest request) {
// Validate order
validateOrder(request);
// Process payment
PaymentResult result = paymentService.processPayment(request.getPaymentDetails());
if (result.isSuccessful()) {
// Create order
Order order = orderRepository.save(new Order(request));
// Update inventory
inventoryService.updateStock(order.getItems());
return order;
} else {
throw new PaymentFailedException(result.getErrorMessage());
}
}
// Asynchronous approach
public OrderCreationResponse createOrder(OrderRequest request) {
// Validate order
validateOrder(request);
// Create pending order
Order pendingOrder = orderRepository.save(new Order(request, OrderStatus.PENDING));
// Publish event for payment processing
eventBus.publish(new OrderCreatedEvent(pendingOrder.getId(), request.getPaymentDetails()));
return new OrderCreationResponse(pendingOrder.getId(), "Order is being processed");
}
The synchronous approach is simpler but can lead to longer response times and tight coupling. The asynchronous approach can improve responsiveness and resilience but introduces complexity in tracking the overall state and handling failures.
The practical choice depends on factors like:
- User expectations for immediate feedback
- System reliability requirements
- The cost of eventual consistency
Data Storage: SQL vs. NoSQL
The SQL vs. NoSQL debate often ignores practical considerations:
- SQL databases excel at complex queries, transactions, and data integrity
- NoSQL databases can offer better scalability, schema flexibility, and specialized data models
A practical approach might use both: SQL for transactional data where consistency is critical, and NoSQL for high-volume data where schema flexibility or specialized query patterns are needed.
Architectural Patterns for Practical Systems
Some architectural patterns are particularly well-suited to practical, evolvable systems:
The Modular Monolith
A modular monolith combines the deployment simplicity of a monolith with the clear boundaries of microservices:
// Project structure for a modular monolith
com.example.app/
├── common/ // Shared utilities and models
├── orders/ // Order management module
│ ├── api/ // Public interfaces
│ ├── internal/ // Implementation details
│ └── OrderModule.java // Module configuration
├── payments/ // Payment processing module
│ ├── api/
│ ├── internal/
│ └── PaymentModule.java
├── inventory/ // Inventory management module
│ ├── api/
│ ├── internal/
│ └── InventoryModule.java
└── Application.java // Main application class
With clear module boundaries and well-defined APIs between modules, a modular monolith can evolve into microservices if and when specific modules need independent scaling or deployment.
CQRS (Command Query Responsibility Segregation)
CQRS separates operations that modify state (commands) from operations that read state (queries), allowing them to be optimized independently:
// Command side (optimized for writes)
public class OrderCommandService {
private final OrderRepository repository;
private final EventPublisher eventPublisher;
public void createOrder(CreateOrderCommand command) {
// Validate command
validateCommand(command);
// Create order
Order order = new Order(command);
repository.save(order);
// Publish event
eventPublisher.publish(new OrderCreatedEvent(order));
}
}
// Query side (optimized for reads)
public class OrderQueryService {
private final OrderReadModel readModel;
public OrderSummary getOrderSummary(String orderId) {
return readModel.getOrderSummary(orderId);
}
public List<OrderSummary> getRecentOrders(String userId) {
return readModel.getRecentOrders(userId);
}
}
This pattern can be implemented within a single application or database, or with separate read and write datastores for maximum scalability. The practical approach is to start simple and add complexity only when needed.
API Gateway Pattern
An API gateway provides a single entry point for client applications, handling cross-cutting concerns like authentication, rate limiting, and request routing:
// Pseudo-code for an API Gateway
app.use(authenticate);
app.use(rateLimit);
// Route requests to appropriate services
app.get('/api/products', async (req, res) => {
const products = await productService.getProducts(req.query);
res.json(products);
});
app.post('/api/orders', async (req, res) => {
try {
const order = await orderService.createOrder(req.body, req.user);
res.status(201).json(order);
} catch (error) {
res.status(400).json({ error: error.message });
}
});
This pattern simplifies client applications and provides a layer where the system can evolve without impacting clients.
Architecture for Different Project Sizes
Practical architecture looks different depending on project size and team structure:
Small Projects (1-5 Developers)
For small projects or startups, practical architecture often means:
- A simple monolith with clean separation of concerns
- A single database (usually relational)
- Minimal infrastructure (perhaps a single cloud provider)
- Focus on developer productivity and fast iteration
The goal is to minimize overhead while maintaining enough structure to keep the codebase manageable.
Medium Projects (5-20 Developers)
As projects grow, practical architecture evolves:
- A modular monolith or a small number of services
- More formalized interfaces between components
- Potentially multiple databases for different data types
- More attention to deployment automation and testing
At this scale, teams start to feel the pain of tight coupling but may not yet need the full complexity of microservices.
Large Projects (20+ Developers)
For large projects, practical architecture addresses team coordination:
- Service boundaries often align with team boundaries
- More investment in platform capabilities and developer tooling
- Standardized communication patterns between services
- Greater emphasis on observability and operational concerns
At this scale, the organizational challenges often outweigh the technical ones, making team autonomy and clear interfaces particularly important.
Implementing Practical Architecture in Your Projects
How can you apply these principles to your own projects? Here’s a practical approach:
1. Start With Core Domains
Identify the core domains of your application—the areas that provide the most business value and differentiation. These deserve the most architectural attention.
For example, in an e-commerce application:
- Core domains: Product catalog, order processing, pricing
- Supporting domains: User management, notifications, analytics
- Generic domains: Authentication, logging, configuration
Invest more architectural effort in core domains, while potentially using off-the-shelf solutions for generic domains.
2. Define Clear Boundaries
Establish clear boundaries between different parts of your system:
// Example of a bounded context in Java
package com.example.orderprocessing;
// This class is part of the order processing context
public class Order {
private OrderId id;
private CustomerId customerId;
private List<OrderItem> items;
private OrderStatus status;
// Methods related to order processing
}
// In a different bounded context (shipping)
package com.example.shipping;
// This is a different concept of "Order" specific to shipping
public class ShippingOrder {
private ShippingOrderId id;
private Address deliveryAddress;
private List<Package> packages;
private ShippingStatus status;
// Methods related to shipping
}
Each bounded context can have its own models, language, and potentially its own persistent storage.
3. Choose Appropriate Communication Patterns
Select communication patterns based on practical needs:
- Synchronous API calls for user-facing operations where immediate feedback is needed
- Message queues for operations that can be processed asynchronously
- Event streams for propagating state changes across the system
Start with the simplest approach that meets your requirements, and evolve as needed.
4. Implement Incrementally
Don’t try to implement your entire architecture at once. Start with a minimal viable architecture and evolve it:
- Begin with the core domain and a simple implementation
- Add supporting capabilities as needed
- Refactor and improve based on real-world usage patterns
- Extract components into services only when there’s a clear benefit
This incremental approach reduces risk and allows you to learn as you go.
5. Monitor and Measure
Implement monitoring and metrics to understand how your architecture is performing in practice:
- Response times and throughput for key operations
- Error rates and types
- Resource utilization (CPU, memory, disk, network)
- Business-level metrics that show system effectiveness
These measurements will guide your architectural evolution more effectively than abstract principles.
When to Embrace Architectural Purity
While this article has emphasized practical compromises, there are times when architectural purity is worth pursuing:
1. When Building Platforms or Frameworks
If you’re building a platform or framework that others will build upon, investing in a clean, consistent architecture is usually worthwhile. The cost of architectural flaws is multiplied across all users of your platform.
2. For Critical System Components
Components with stringent reliability, security, or performance requirements may justify a more rigorous architectural approach. For example, a payment processing system might warrant more architectural purity than a content management system.
3. When Refactoring Problematic Areas
Areas of your codebase that have accumulated significant technical debt might benefit from a more purist approach during refactoring, establishing a cleaner foundation for future development.
Preparing for Technical Interviews
For those preparing for technical interviews at major tech companies, understanding practical architecture is crucial:
System Design Interview Tips
In system design interviews:
- Start by clarifying requirements and constraints
- Discuss trade-offs explicitly, showing you understand there’s no perfect solution
- Scale your design appropriately to the problem (don’t propose Google-scale solutions for simple problems)
- Show evolution paths—how your design could adapt to changing requirements
Interviewers are often more impressed by practical, thoughtful designs than by candidates who immediately jump to complex architectures without justification.
Coding Interview Considerations
Even in coding interviews, architectural thinking matters:
- Structure your code with clear boundaries between components
- Choose abstractions that match the problem’s complexity
- Be prepared to explain the trade-offs in your design
// Simple, clear design for a coding interview
public class RateLimiter {
private final Map<String, Integer> requestCounts = new HashMap<>();
private final Map<String, Long> lastRequestTime = new HashMap<>();
private final int maxRequests;
private final long timeWindowMs;
public RateLimiter(int maxRequests, long timeWindowMs) {
this.maxRequests = maxRequests;
this.timeWindowMs = timeWindowMs;
}
public synchronized boolean allowRequest(String clientId) {
long currentTime = System.currentTimeMillis();
// Reset counter if time window has passed
if (!lastRequestTime.containsKey(clientId) ||
currentTime - lastRequestTime.get(clientId) > timeWindowMs) {
requestCounts.put(clientId, 0);
}
// Update last request time
lastRequestTime.put(clientId, currentTime);
// Check and update count
int currentCount = requestCounts.getOrDefault(clientId, 0);
if (currentCount < maxRequests) {
requestCounts.put(clientId, currentCount + 1);
return true;
} else {
return false;
}
}
}
This example shows a practical design: it solves the problem without unnecessary complexity, uses appropriate data structures, and has clear methods with a single responsibility.
Conclusion: The Art of Practical Architecture
Software architecture is more art than science. The most successful architects are not those who rigidly adhere to theoretical ideals, but those who find the right balance between architectural purity and practical constraints.
Remember these key principles:
- Start with clear requirements and constraints
- Make deliberate trade-offs based on your specific context
- Design for evolution rather than perfection
- Focus on boundaries and interfaces
- Consider the human factors—team skills, organization structure, and developer experience
By embracing a practical approach to architecture, you'll build systems that not only work well today but can adapt to the challenges of tomorrow. And that's more valuable than any theoretically perfect design.
As you continue your coding journey, whether you're learning to code or preparing for technical interviews at major tech companies, remember that the ability to make pragmatic architectural decisions is what separates exceptional software engineers from the merely competent.
Perfect architecture isn't practical—but practical architecture can be perfect for your needs.