Why Your Microservices Communication Is Creating Chaos
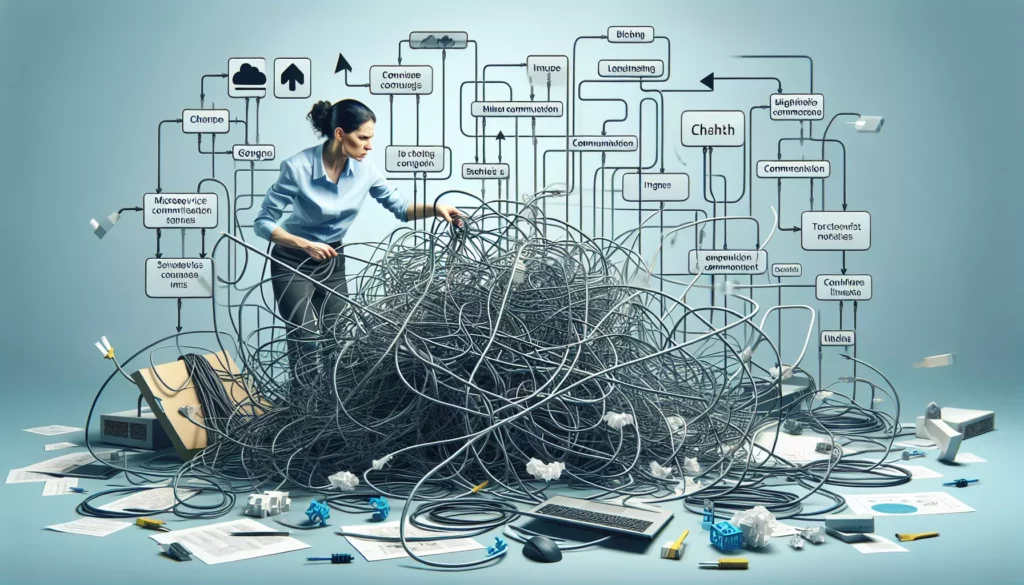
Microservices architecture has become the gold standard for building scalable, resilient applications. But with this architectural shift comes a new set of challenges, particularly in how services communicate with each other. Poor communication patterns between microservices can lead to system instability, performance bottlenecks, and maintenance nightmares.
In this comprehensive guide, we’ll explore why your microservices communication might be causing chaos in your system, and how to implement better patterns to create a more reliable and maintainable architecture.
Table of Contents
- Understanding the Problem: Communication Chaos
- Common Pitfalls in Microservices Communication
- Synchronous vs. Asynchronous Communication
- Effective Communication Patterns
- Service Discovery and Registry
- API Gateways: Friend or Foe?
- Message Brokers and Event Streaming
- Contract Testing for Reliable Interactions
- Monitoring and Observability
- Best Practices for Microservices Communication
- Conclusion
Understanding the Problem: Communication Chaos
The promise of microservices is enticing: independent deployment, technological diversity, and team autonomy. However, what many teams discover is that they’ve traded the complexity of a monolith for the complexity of distributed systems. And at the heart of this complexity is communication.
In a monolithic application, components communicate through in-memory function calls. This is fast, reliable, and straightforward. In a microservices architecture, these function calls are replaced by network calls, which introduce latency, potential failures, and complex coordination challenges.
The chaos often emerges gradually:
- Services become tightly coupled despite being physically separate
- Network latency creates cascading performance issues
- Failed communications lead to inconsistent states
- Versioning and compatibility challenges emerge
- Debugging becomes a complex distributed puzzle
Let’s dive deeper into the specific pitfalls that create this chaos.
Common Pitfalls in Microservices Communication
1. The Distributed Monolith
One of the most common issues is creating what’s essentially a distributed monolith: services that are physically separate but so tightly coupled that they must be deployed together. This negates one of the primary benefits of microservices.
Signs you have a distributed monolith include:
- Changes to one service frequently require changes to others
- Services need to be deployed in a specific order
- Service boundaries are unclear or constantly changing
- Direct database access across service boundaries
2. Synchronous Communication Overuse
Many teams default to RESTful HTTP calls for all service-to-service communication. While this is familiar and straightforward, it creates tight runtime coupling. If Service A needs Service B, C, and D to respond before it can complete a request, you’ve created a fragile chain of dependencies.
Consider this example of a checkout flow with excessive synchronous dependencies:
// Pseudocode for a problematic checkout service
function processCheckout(cart) {
// Synchronous call to inventory service
let inventoryStatus = inventoryService.checkAvailability(cart.items);
if (!inventoryStatus.allAvailable) {
return "Some items are out of stock";
}
// Synchronous call to payment service
let paymentResult = paymentService.processPayment(cart.paymentDetails);
if (!paymentResult.success) {
return "Payment failed: " + paymentResult.reason;
}
// Synchronous call to shipping service
let shippingResult = shippingService.createShipment(cart.items, cart.address);
if (!shippingResult.success) {
// Now we need to reverse the payment!
paymentService.refundPayment(paymentResult.transactionId);
return "Shipping arrangement failed";
}
// Synchronous call to notification service
notificationService.sendConfirmation(cart.customerEmail);
return "Order placed successfully";
}
In this example, if any service is slow or unavailable, the entire checkout process fails. Furthermore, if a later step fails (like shipping), you need complex compensation logic to undo previous steps.
3. Chatty Communication
When services make numerous small calls to each other to accomplish a task, they create “chatty” interfaces. This increases latency and network load, and makes the system more vulnerable to network issues.
For example, a product page that makes separate calls for:
- Basic product information
- Pricing information
- Inventory status
- User-specific discounts
- Related products
- Product reviews
Each call adds latency and increases the chance of failure.
4. Ignoring Partial Failures
In a distributed system, partial failures are inevitable. Services that don’t implement proper error handling, retries, circuit breakers, and fallback mechanisms will create cascading failures throughout the system.
5. Inconsistent Data Models
When services evolve independently, their data models often drift apart. Without careful management, this leads to constant translation and mapping, increasing complexity and the chance of errors.
Synchronous vs. Asynchronous Communication
Understanding the trade-offs between synchronous and asynchronous communication is crucial for designing robust microservices.
Synchronous Communication
Characteristics:
- The caller waits for a response
- Typically implemented via REST, gRPC, or GraphQL
- Simpler to implement and reason about
- Creates temporal coupling
Best used when:
- You need an immediate response
- The operation is critical path and cannot proceed without the response
- Simplicity is more important than resilience
Asynchronous Communication
Characteristics:
- The caller doesn’t wait for a response (fire-and-forget)
- Typically implemented via message queues or event streams
- More complex to implement but more resilient
- Decouples services temporally
Best used when:
- The operation can be processed later
- You need to decouple services for better scalability
- The system needs to be resilient to service outages
- You want to implement event-driven architectures
Let’s rewrite our earlier checkout example using a more resilient, event-driven approach:
// Event-driven checkout process
function initiateCheckout(cart) {
// Generate a unique order ID
let orderId = generateUniqueId();
// Store the order in a "pending" state
orderRepository.save({
id: orderId,
items: cart.items,
customer: cart.customer,
status: "PENDING"
});
// Publish an event to begin processing
eventBus.publish("checkout.initiated", {
orderId: orderId,
cart: cart
});
return {
orderId: orderId,
status: "PENDING",
message: "Your order is being processed"
};
}
// Each service subscribes to relevant events
// Inventory Service
eventBus.subscribe("checkout.initiated", async (event) => {
let result = await checkInventory(event.cart.items);
if (result.success) {
eventBus.publish("inventory.reserved", {
orderId: event.orderId,
items: event.cart.items
});
} else {
eventBus.publish("checkout.failed", {
orderId: event.orderId,
reason: "INVENTORY_UNAVAILABLE",
details: result.unavailableItems
});
}
});
This approach decouples the services, allowing them to operate independently and making the system more resilient to failures.
Effective Communication Patterns
Let’s explore some patterns that can help tame the chaos in microservices communication.
1. Command Query Responsibility Segregation (CQRS)
CQRS separates read and write operations, allowing them to be optimized independently. This can be particularly useful in microservices architectures.
- Commands: Write operations that change state, often handled asynchronously
- Queries: Read operations that return data, optimized for performance
This pattern allows you to have different data models for reading and writing, which can improve performance and scalability.
2. Event Sourcing
Event sourcing stores all changes to application state as a sequence of events. Instead of storing the current state, you store the history of actions that led to that state.
This pattern works well with microservices because:
- It provides a complete audit trail
- It enables replaying events to rebuild state or debug issues
- It facilitates event-driven communication between services
3. Saga Pattern
The Saga pattern helps manage distributed transactions across multiple services. Since traditional ACID transactions don’t work well across service boundaries, sagas break a transaction into a sequence of local transactions, each with compensating actions in case of failure.
There are two main approaches to implementing sagas:
- Choreography: Each service publishes events that trigger the next step in other services
- Orchestration: A central coordinator directs the steps and manages failures
Here’s a simplified example of a choreographed saga for our checkout process:
// Order Service
eventBus.subscribe("checkout.initiated", (event) => {
// Create order
createOrder(event.orderId, event.cart);
eventBus.publish("order.created", { orderId: event.orderId });
});
// Payment Service
eventBus.subscribe("order.created", (event) => {
let result = processPayment(event.orderId);
if (result.success) {
eventBus.publish("payment.completed", { orderId: event.orderId });
} else {
eventBus.publish("payment.failed", {
orderId: event.orderId,
reason: result.reason
});
}
});
// Order Service (handling failure)
eventBus.subscribe("payment.failed", (event) => {
updateOrderStatus(event.orderId, "CANCELLED", event.reason);
});
4. Backend for Frontend (BFF)
The BFF pattern involves creating specialized backend services for specific frontend applications or client types. This reduces the need for multiple API calls from the client and allows for better optimization.
Instead of having your web, mobile, and third-party clients all calling multiple microservices directly, you create dedicated BFFs that:
- Aggregate data from multiple services
- Transform data into the exact format needed by the client
- Handle authentication and authorization
- Implement client-specific logic
5. Bulkhead Pattern
The Bulkhead pattern isolates components of your application into pools so that if one fails, the others continue to function. In the context of microservices communication, this might involve:
- Separate thread pools for different types of operations
- Connection pools per service
- Resource quotas for different clients or operations
This prevents one misbehaving service from consuming all resources and affecting other parts of the system.
Service Discovery and Registry
In a microservices environment, service instances come and go due to scaling, deployments, and failures. Service discovery solves the problem of finding where services are located.
Client-side Discovery
With client-side discovery, the client is responsible for determining the locations of available service instances and load balancing requests across them.
// Example using a client-side discovery library
const serviceRegistry = require('service-registry-client');
async function callUserService(userId) {
// Get all available instances of the user service
const instances = await serviceRegistry.getInstances('user-service');
// Select an instance (simple round-robin)
const instance = instances[requestCounter % instances.length];
// Make the request to the selected instance
return axios.get(`http://${instance.host}:${instance.port}/users/${userId}`);
}
Server-side Discovery
With server-side discovery, clients make requests through a router or load balancer that handles instance selection.
// With server-side discovery, the client simply calls a well-known URL
async function callUserService(userId) {
// The router/load balancer at api-gateway.example.com
// handles finding an appropriate instance
return axios.get(`http://api-gateway.example.com/user-service/users/${userId}`);
}
Service Registry Tools
Several tools can help with service discovery:
- Consul: Service discovery, health checking, and distributed configuration
- Eureka: Service discovery for the AWS cloud environment
- etcd: Distributed key-value store often used for service discovery
- Kubernetes: Built-in service discovery through Services and DNS
API Gateways: Friend or Foe?
API gateways act as the entry point for client requests, routing them to appropriate services. They can be powerful tools but also introduce complexity.
Benefits of API Gateways
- Request routing: Direct requests to the appropriate service
- API composition: Aggregate responses from multiple services
- Protocol translation: Convert between protocols (e.g., HTTP to gRPC)
- Authentication and authorization: Centralized security
- Rate limiting and throttling: Protect services from overload
- Monitoring and analytics: Centralized visibility
Potential Drawbacks
- Single point of failure: If not properly designed for high availability
- Performance bottleneck: Can introduce latency
- Operational complexity: Another component to maintain
- Potential for tight coupling: If not carefully managed
Types of API Gateways
- Single gateway: One gateway for all clients
- Gateway per client: Specialized gateways for web, mobile, etc. (BFF pattern)
- Gateway mesh: Multiple gateways with different responsibilities
Popular API gateway solutions include:
- Kong
- Amazon API Gateway
- Azure API Management
- Spring Cloud Gateway
- Netflix Zuul
Message Brokers and Event Streaming
Message brokers and event streaming platforms are essential for implementing asynchronous communication between microservices.
Message Queues
Message queues implement point-to-point communication, where messages are consumed by a single recipient.
Key characteristics:
- Messages are retained until processed
- Each message is consumed by one consumer
- Good for work distribution and load leveling
Popular message queue technologies include:
- RabbitMQ
- ActiveMQ
- Amazon SQS
- Azure Service Bus
Publish-Subscribe (Pub/Sub)
Pub/Sub systems allow messages to be broadcast to multiple consumers.
Key characteristics:
- Publishers send messages to topics
- Subscribers receive all messages from topics they subscribe to
- Good for event distribution and notifications
Event Streaming Platforms
Event streaming platforms like Apache Kafka and AWS Kinesis provide persistent, ordered logs of events that can be consumed by multiple services.
Key characteristics:
- Events are stored in order
- Events are retained for a configured period
- Multiple consumers can read the same events
- Consumers track their position in the stream
- Support for replaying events
Implementing Event-Driven Communication
Here’s an example of implementing event-driven communication using a message broker:
// Producer service (Node.js with AMQP library)
const amqp = require('amqplib');
async function publishOrderCreatedEvent(order) {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const exchange = 'order_events';
await channel.assertExchange(exchange, 'topic', { durable: true });
const routingKey = 'order.created';
const message = JSON.stringify({
orderId: order.id,
customerId: order.customerId,
items: order.items,
total: order.total,
timestamp: new Date().toISOString()
});
channel.publish(exchange, routingKey, Buffer.from(message));
console.log(`Published order.created event for order ${order.id}`);
setTimeout(() => {
connection.close();
}, 500);
}
// Consumer service (Node.js with AMQP library)
async function subscribeToOrderEvents() {
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
const exchange = 'order_events';
await channel.assertExchange(exchange, 'topic', { durable: true });
const queue = await channel.assertQueue('', { exclusive: true });
await channel.bindQueue(queue.queue, exchange, 'order.#');
console.log(`Waiting for order events...`);
channel.consume(queue.queue, (msg) => {
const content = JSON.parse(msg.content.toString());
const routingKey = msg.fields.routingKey;
console.log(`Received ${routingKey} event:`, content);
if (routingKey === 'order.created') {
processNewOrder(content);
}
}, { noAck: true });
}
Contract Testing for Reliable Interactions
As your microservices ecosystem grows, ensuring that services can communicate correctly becomes increasingly important. Contract testing helps ensure that services adhere to their agreed-upon interfaces.
What is Contract Testing?
Contract testing verifies that the interactions between services meet their contractual agreements. Unlike end-to-end testing, which tests the entire system, contract testing focuses solely on the boundaries between services.
Consumer-Driven Contracts
In consumer-driven contract testing, the consumer of an API defines the expectations (the contract) that it has of the provider. The provider then verifies that it can meet these expectations.
This approach has several advantages:
- Providers only implement what consumers actually need
- Changes that would break consumers can be caught early
- Tests are faster and more focused than end-to-end tests
Tools for Contract Testing
- Pact: A contract testing tool that allows consumer-driven contract testing
- Spring Cloud Contract: For contract testing in Spring applications
- Postman: Can be used for simple contract verification
Example of a Pact Contract Test
// Consumer-side contract test (JavaScript with Pact)
const { Pact } = require('@pact-foundation/pact');
const { UserService } = require('./user-service');
const { expect } = require('chai');
describe('User Service Client', () => {
const provider = new Pact({
consumer: 'OrderService',
provider: 'UserService',
port: 8888
});
before(() => provider.setup());
after(() => provider.finalize());
describe('get user', () => {
before(() => {
return provider.addInteraction({
state: 'a user exists',
uponReceiving: 'a request for a user',
withRequest: {
method: 'GET',
path: '/users/123',
headers: { 'Accept': 'application/json' }
},
willRespondWith: {
status: 200,
headers: { 'Content-Type': 'application/json' },
body: {
id: '123',
name: 'John Doe',
email: 'john@example.com'
}
}
});
});
it('returns the user', async () => {
const userService = new UserService('http://localhost:8888');
const user = await userService.getUser('123');
expect(user).to.deep.equal({
id: '123',
name: 'John Doe',
email: 'john@example.com'
});
});
afterEach(() => provider.verify());
});
});
Monitoring and Observability
In a distributed system, understanding what’s happening is crucial for identifying and resolving issues. This requires robust monitoring and observability.
The Three Pillars of Observability
- Logs: Detailed records of events that happened in the system
- Metrics: Numerical data about system behavior over time
- Traces: Records of requests as they flow through the system
Distributed Tracing
Distributed tracing is particularly important for microservices, as it allows you to follow a request as it travels through multiple services.
Key components of distributed tracing:
- Trace ID: A unique identifier for a request that spans all services
- Span: A unit of work within a trace, typically representing a single service’s handling of the request
- Span Context: Metadata that’s propagated between services to maintain the trace
Implementing Distributed Tracing
// Example using OpenTelemetry in Node.js
const opentelemetry = require('@opentelemetry/api');
const { NodeTracerProvider } = require('@opentelemetry/node');
const { ConsoleSpanExporter, SimpleSpanProcessor } = require('@opentelemetry/tracing');
// Set up the tracer
const provider = new NodeTracerProvider();
const exporter = new ConsoleSpanExporter();
provider.addSpanProcessor(new SimpleSpanProcessor(exporter));
provider.register();
const tracer = opentelemetry.trace.getTracer('order-service');
// Using the tracer in an Express middleware
app.use((req, res, next) => {
const span = tracer.startSpan('process_request');
// Add attributes to the span
span.setAttribute('http.method', req.method);
span.setAttribute('http.url', req.url);
// Store the span in the request context
req.span = span;
// Add a callback to end the span when the response is sent
const originalEnd = res.end;
res.end = function(...args) {
span.setAttribute('http.status_code', res.statusCode);
span.end();
return originalEnd.apply(this, args);
};
next();
});
Monitoring Tools
Several tools can help with monitoring and observability:
- Prometheus: For metrics collection and alerting
- Grafana: For metrics visualization
- Jaeger or Zipkin: For distributed tracing
- ELK Stack: For log aggregation and analysis
- New Relic, Datadog, Dynatrace: Commercial APM solutions
Best Practices for Microservices Communication
Based on our exploration, here are key best practices to improve microservices communication:
1. Design for Failure
- Implement circuit breakers to prevent cascading failures
- Use timeouts to prevent indefinite waiting
- Implement retries with exponential backoff
- Provide fallback mechanisms when services are unavailable
// Example using Hystrix-like circuit breaker in JavaScript
const CircuitBreaker = require('opossum');
const options = {
timeout: 3000, // If function takes longer than 3 seconds, trigger a failure
errorThresholdPercentage: 50, // Open circuit if 50% of requests fail
resetTimeout: 10000 // Try again after 10 seconds
};
const breaker = new CircuitBreaker(callUserService, options);
breaker.fallback(() => {
return { id: 'unknown', name: 'Unknown User', isDefault: true };
});
breaker.on('open', () => console.log('Circuit breaker opened'));
breaker.on('close', () => console.log('Circuit breaker closed'));
breaker.on('halfOpen', () => console.log('Circuit breaker half-open'));
// Use the circuit breaker
async function getUserDetails(userId) {
try {
return await breaker.fire(userId);
} catch (error) {
console.error('Error getting user details:', error);
throw error;
}
}
2. Choose the Right Communication Pattern
- Use synchronous communication only when necessary
- Prefer asynchronous communication for better resilience
- Consider event-driven architectures for complex workflows
- Use the request-response pattern for simple queries
3. Define Clear Contracts
- Use API specifications like OpenAPI or gRPC protobufs
- Implement contract testing
- Consider semantic versioning for APIs
- Plan for backward compatibility
4. Implement Proper Error Handling
- Return meaningful error responses
- Include correlation IDs in error messages
- Log errors with context
- Handle partial failures gracefully
5. Optimize for Performance
- Use connection pooling
- Consider binary protocols for high-performance needs
- Implement caching strategies
- Batch requests when appropriate
6. Implement Comprehensive Monitoring
- Track service health and performance
- Implement distributed tracing
- Monitor communication patterns and identify bottlenecks
- Set up alerts for communication issues
Conclusion
Microservices communication is often the source of chaos in distributed systems, but it doesn’t have to be. By understanding the common pitfalls and implementing appropriate patterns and best practices, you can create a more reliable, maintainable, and scalable architecture.
Remember these key points:
- Choose the right communication style (synchronous vs. asynchronous) for each interaction
- Implement resilience patterns like circuit bre