Why Your Logical Thinking Breaks Down When Coding
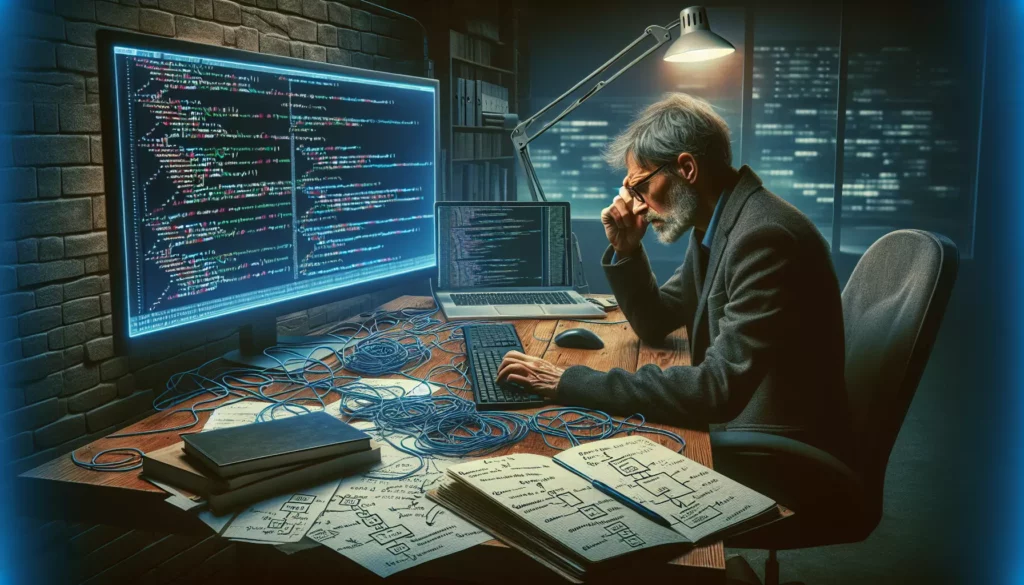
Have you ever sat down to code a seemingly simple program only to find yourself staring at the screen an hour later, completely baffled by why your solution isn’t working? You’re not alone. Even experienced programmers regularly encounter situations where their logical thinking seems to abandon them entirely when faced with coding challenges.
In this comprehensive guide, we’ll explore why our normally reliable logical thinking can fail us when programming, and more importantly, what we can do about it. Whether you’re a beginner or preparing for technical interviews at major tech companies, understanding these cognitive pitfalls can dramatically improve your coding effectiveness.
The Gap Between Human Logic and Computer Logic
One of the fundamental challenges in programming is that humans and computers “think” in fundamentally different ways. This disconnect is often at the root of our logical breakdowns.
The Human Mind: Flexible but Imprecise
Human thinking is:
- Intuitive: We make logical leaps based on incomplete information
- Context-sensitive: We naturally consider the broader situation
- Forgiving: We can understand meaning despite ambiguities or errors
- Pattern-oriented: We excel at recognizing patterns, even partial ones
Computer Logic: Precise but Inflexible
Computer logic is:
- Literal: Executes exactly what you tell it to, not what you meant
- Sequential: Follows instructions step by step
- Binary: Operates on clear true/false distinctions
- Unforgiving: Has zero tolerance for ambiguity or syntax errors
Consider this simple example:
if (userAge > 18) {
console.log("You can vote!");
}
A human might interpret this as “if you’re an adult, you can vote,” but the computer interprets it as “if your age is strictly greater than 18, output this message.” Someone who is exactly 18 wouldn’t see the message, which might not be what the programmer intended.
Common Logical Breakdowns in Programming
Let’s explore some specific ways our logical thinking tends to falter when we code:
1. Assumption Blindness
We make countless unconscious assumptions when thinking through problems. While humans can often work around incorrect assumptions, computers cannot.
Example: Imagine writing a function to calculate the average of an array of numbers:
function calculateAverage(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
This function has a hidden assumption: that the array isn’t empty. If it is, we’ll divide by zero, potentially causing an error or returning NaN. A more robust solution would be:
function calculateAverage(numbers) {
if (numbers.length === 0) return 0; // or throw an error
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
2. Off-by-One Errors
These errors occur when a loop or algorithm iterates one time too many or too few. They’re notoriously common because humans tend to think inclusively about ranges, while programming often requires exclusive thinking.
Example: Consider a function to generate numbers from start to end:
function generateRange(start, end) {
const result = [];
for (let i = start; i <= end; i++) {
result.push(i);
}
return result;
}
If we call generateRange(1, 5)
, we get [1, 2, 3, 4, 5]
, which is correct if we want to include both endpoints. But if we’re generating array indices, we might accidentally go out of bounds because arrays are zero-indexed in many languages.
3. State Blindness
Humans struggle to keep track of multiple changing variables simultaneously. In programming, where state changes constantly, this limitation becomes painfully apparent.
Example: Consider this attempt to swap two variables:
// Incorrect way to swap
let a = 5;
let b = 10;
a = b; // a is now 10
b = a; // b is still 10!
The correct approach requires a temporary variable to hold one value:
let a = 5;
let b = 10;
let temp = a; // Store a's value
a = b; // a becomes 10
b = temp; // b becomes 5
4. Logical Fallacies in Conditionals
Boolean logic in programming can be counterintuitive, especially when dealing with complex conditions involving AND (&&
), OR (||
), and NOT (!
) operators.
Example: Consider a login system that should allow access if a user is either an admin OR has both a valid subscription AND has verified their email:
// What we mean
if (isAdmin || (hasValidSubscription && hasVerifiedEmail)) {
grantAccess();
}
// What we might mistakenly write
if (isAdmin || hasValidSubscription && hasVerifiedEmail) {
grantAccess();
}
Due to operator precedence, these two statements are actually equivalent. But if we meant “either an admin OR has a valid subscription, AND has verified their email,” we would need:
if ((isAdmin || hasValidSubscription) && hasVerifiedEmail) {
grantAccess();
}
5. Recursion Confusion
Recursive thinking requires visualizing a process that calls itself. This creates a mental stack that humans find difficult to track.
Example: A classic recursive function to calculate factorial:
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
}
The logical breakdown often happens when we fail to properly define the base case or when we struggle to trace the execution stack mentally.
Why These Breakdowns Happen: The Cognitive Science
Understanding the cognitive mechanisms behind these logical failures can help us develop strategies to overcome them.
Cognitive Load Theory
Programming places enormous demands on our working memory. According to cognitive load theory, humans can only keep about 4-7 items in working memory simultaneously. Complex code easily exceeds this capacity, leading to errors when we lose track of variables, conditions, or execution flow.
System 1 vs. System 2 Thinking
Psychologist Daniel Kahneman describes two modes of thinking:
- System 1: Fast, intuitive, and automatic
- System 2: Slow, deliberate, and effortful
Programming requires System 2 thinking, but we naturally default to System 1. When we’re tired, rushed, or overconfident, we may rely on intuition rather than methodically working through the logic step by step.
Confirmation Bias
We tend to search for and interpret information in ways that confirm our preexisting beliefs. In programming, this manifests as looking for evidence that our code is correct rather than trying to find flaws in our logic.
Strategies to Strengthen Your Programming Logic
Now that we understand why our logical thinking breaks down, let’s explore practical strategies to strengthen it:
1. Visualize Code Execution
One of the most effective ways to improve your programming logic is to practice visualizing exactly how the computer will execute your code.
Manual Tracing
Get in the habit of “playing computer” by tracing through your code line by line on paper, tracking all variable changes and control flow decisions.
Example: Let’s trace through a bubble sort algorithm:
function bubbleSort(arr) {
let n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
let temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
return arr;
}
For the input [5, 3, 8, 4]
, you would track each comparison and swap:
- Initial:
[5, 3, 8, 4]
- Compare 5 > 3? Yes, swap:
[3, 5, 8, 4]
- Compare 5 > 8? No, continue:
[3, 5, 8, 4]
- Compare 8 > 4? Yes, swap:
[3, 5, 4, 8]
- And so on…
Use Visualization Tools
Tools like Python Tutor (for multiple languages) or JavaScript Visualizer can show you the step-by-step execution of your code, making it easier to identify logical errors.
2. Break Down Complex Problems
Complex problems overwhelm our cognitive capacity. Break them down into smaller, manageable pieces.
Use Pseudocode
Before writing actual code, sketch your solution in pseudocode:
// Problem: Find the longest substring without repeating characters
// Pseudocode:
// 1. Initialize an empty set to track seen characters
// 2. Initialize two pointers (start and end) at the beginning of the string
// 3. Initialize maxLength to 0
// 4. While end pointer is less than string length:
// a. If the current character is not in the set:
// i. Add it to the set
// ii. Move end pointer forward
// iii. Update maxLength if current window is larger
// b. If the current character is in the set:
// i. Remove the character at start pointer from the set
// ii. Move start pointer forward
// 5. Return maxLength
Test Each Component Independently
Write and test each function or component in isolation before integrating them into your complete solution.
3. Develop a Disciplined Debugging Process
When your code doesn’t work as expected, resist the urge to make random changes. Instead:
Form and Test Hypotheses
Approach debugging scientifically:
- Observe the specific behavior or error
- Form a hypothesis about what might be causing it
- Design a test that would confirm or refute your hypothesis
- Execute the test and analyze the results
- Refine your understanding and repeat
Use Strategic Print Statements or Debuggers
Place print statements (or breakpoints) at strategic points in your code to verify your assumptions about variable values and execution flow.
function processData(data) {
console.log("Input data:", data);
let result = data.filter(item => item.value > 10);
console.log("After filtering:", result);
result = result.map(item => item.value * 2);
console.log("After mapping:", result);
return result.reduce((sum, value) => sum + value, 0);
}
4. Practice Explicit Logic
Train yourself to be explicit about your logical steps, avoiding intuitive leaps that computers can’t follow.
Write Self-Documenting Code
Use meaningful variable names and add comments explaining your logic:
// Bad example
function calc(a, b, c) {
return a ? b + c : b - c;
}
// Good example
function calculateTotal(hasDiscount, basePrice, taxAmount) {
// Apply discount by subtracting tax, otherwise add tax
return hasDiscount ? basePrice - taxAmount : basePrice + taxAmount;
}
Avoid Logical Shortcuts
Be explicit about all conditions and edge cases:
// Implicit logic with potential issues
function getElementAtIndex(array, index) {
return array[index]; // What if index is out of bounds?
}
// Explicit logic handling edge cases
function getElementAtIndex(array, index) {
if (!Array.isArray(array)) {
throw new Error("First argument must be an array");
}
if (index < 0 || index >= array.length) {
return null; // Or throw an error, depending on requirements
}
return array[index];
}
5. Leverage Test-Driven Development
Writing tests before code forces you to think through your logic more carefully.
Start with Edge Cases
Test the boundaries of your function’s expected behavior:
// Testing a function that finds the minimum value in an array
function testFindMinimum() {
// Test with normal case
assert(findMinimum([5, 3, 8, 1, 9]) === 1);
// Test with negative numbers
assert(findMinimum([-5, -3, -8, -1, -9]) === -9);
// Test with mixed numbers
assert(findMinimum([-5, 3, 0, -1, 9]) === -5);
// Test with single element
assert(findMinimum([42]) === 42);
// Test with empty array
assert(findMinimum([]) === null); // Or whatever we define for empty input
}
Advanced Logical Challenges in Programming
As you progress in your programming journey, you’ll encounter more sophisticated logical challenges. Let’s examine some of these advanced scenarios:
Concurrency and Asynchronous Logic
Asynchronous code introduces a new dimension of logical complexity because the execution order isn’t strictly sequential.
Example: Consider this JavaScript code:
console.log("Start");
setTimeout(() => {
console.log("Timeout finished");
}, 0);
Promise.resolve().then(() => {
console.log("Promise resolved");
});
console.log("End");
Many programmers would expect this to log “Start”, “Timeout finished”, “Promise resolved”, “End”. However, it actually logs “Start”, “End”, “Promise resolved”, “Timeout finished” due to the event loop’s priority system.
To strengthen your asynchronous logic:
- Study the event loop in JavaScript or equivalent mechanisms in other languages
- Visualize async operations using sequence diagrams
- Use async/await to make asynchronous code more sequential and readable
Algorithmic Thinking
Advanced algorithms require a different kind of logical thinking, often involving mathematical induction, graph theory, or dynamic programming.
Example: Consider the classic dynamic programming problem of calculating Fibonacci numbers:
// Naive recursive approach - exponential time complexity
function fibRecursive(n) {
if (n <= 1) return n;
return fibRecursive(n - 1) + fibRecursive(n - 2);
}
// Dynamic programming approach - linear time complexity
function fibDP(n) {
if (n <= 1) return n;
let a = 0, b = 1;
for (let i = 2; i <= n; i++) {
let temp = a + b;
a = b;
b = temp;
}
return b;
}
To improve your algorithmic thinking:
- Practice recognizing problem patterns (divide and conquer, greedy, dynamic programming)
- Learn to analyze time and space complexity
- Visualize algorithms using diagrams or animation tools
System Design Logic
As you work on larger systems, logical thinking extends beyond individual functions to the architecture level.
To strengthen your system design logic:
- Study design patterns and architectural principles
- Practice breaking down systems into components with clear interfaces
- Consider tradeoffs systematically (performance vs. simplicity, flexibility vs. complexity)
Real-World Examples: When Logic Broke Down
Let's examine some notorious real-world examples where logical thinking failed, resulting in serious bugs:
The Ariane 5 Rocket Explosion
In 1996, the Ariane 5 rocket exploded just 40 seconds after launch due to a software error. The issue? A 64-bit floating-point number was converted to a 16-bit integer, causing an overflow. The logical breakdown: engineers reused code from the Ariane 4 without considering that the Ariane 5 had different flight characteristics that produced larger values.
The Y2K Bug
To save memory, many early programs represented years with just two digits (e.g., "99" for 1999). The logical breakdown: developers didn't anticipate their code would still be running when the year 2000 arrived, causing the potential for "00" to be interpreted as 1900 instead of 2000.
The Mars Climate Orbiter Crash
In 1999, NASA lost the $125 million Mars Climate Orbiter because one team used metric units while another used imperial units. The logical breakdown: assumptions about units weren't explicitly documented or verified between teams.
Building Your Programming Logic Muscles
Like any skill, logical thinking in programming improves with deliberate practice. Here are some effective exercises:
1. Solve Algorithm Challenges
Platforms like LeetCode, HackerRank, and AlgoCademy offer thousands of problems designed to strengthen your logical thinking:
- Start with easier problems to build confidence
- Use a systematic approach rather than jumping straight to coding
- Review multiple solutions after solving a problem to see different logical approaches
2. Implement Data Structures from Scratch
Building fundamental data structures helps solidify your understanding of how they work:
- Implement a linked list with operations like insert, delete, and reverse
- Build a binary search tree with traversal methods
- Create a hash table with collision resolution
3. Code Reviews
Reviewing others' code (and having your code reviewed) is invaluable for improving logical thinking:
- Question assumptions in the code
- Look for edge cases that might not be handled
- Consider alternative approaches and their tradeoffs
4. Refactoring Exercises
Take working but messy code and refactor it to be cleaner and more logical:
- Break down long functions into smaller, focused ones
- Eliminate duplicate logic
- Clarify complex conditional logic
5. Pair Programming
Working with another programmer forces you to articulate your logical thinking:
- Explain your approach before implementing it
- Listen to your partner's logical reasoning
- Collaboratively identify logical flaws
When to Trust Your Intuition vs. When to Be Methodical
Programming requires a balance between intuitive and methodical thinking:
When to Trust Your Intuition
- During the initial design phase when exploring possible approaches
- When you have extensive experience with similar problems
- For high-level architectural decisions where tradeoffs are subjective
When to Be Methodical
- When implementing critical algorithms where correctness is essential
- When debugging complex issues that resist quick fixes
- When working with unfamiliar languages or frameworks
- When handling edge cases that might be rare but important
Conclusion: Embracing the Computer's Way of Thinking
The disconnect between human and computer logic isn't a flaw to overcome but a fundamental difference to understand and work with. The most successful programmers aren't those who never experience logical breakdowns but those who have developed strategies to recognize and address them effectively.
By understanding why our logical thinking breaks down when coding, implementing the strategies we've discussed, and practicing consistently, you can bridge the gap between human intuition and computer precision. This will not only make you a more effective programmer but also prepare you for technical interviews at top tech companies where logical problem-solving is rigorously tested.
Remember that developing strong programming logic is a journey, not a destination. Even the most experienced developers encounter logical challenges regularly. The difference is that they've built a toolkit of strategies to work through these challenges systematically.
So the next time you find yourself staring at a bug that "shouldn't be possible," take a deep breath, step back, and approach the problem with the strategies we've discussed. Your computer isn't being stubborn or illogical; it's simply following your instructions exactly as given. Learning to think like a computer while leveraging your human creativity and problem-solving abilities is the essence of becoming an exceptional programmer.