Why Your Logging Strategy Isn’t Helping With Debugging
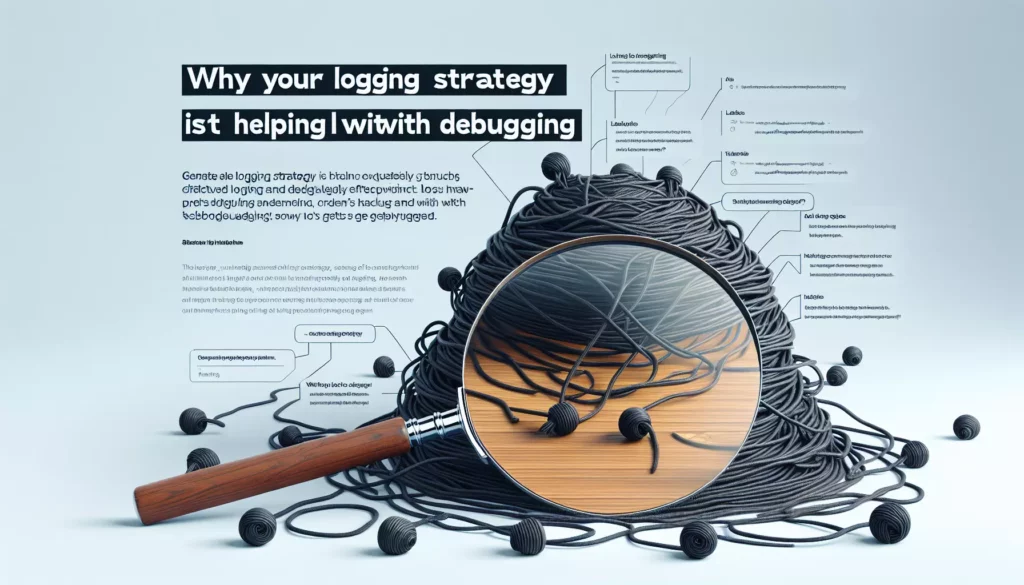
Debugging is often like detective work. You search for clues, follow leads, and try to piece together what’s happening in your code. Logging seems like the perfect tool for this job—it’s like having security cameras installed throughout your application. Yet many developers find themselves drowning in log messages without getting closer to solving their problems.
If you’ve ever stared at endless logs wondering why they’re not helping you track down that elusive bug, this article is for you. We’ll explore common logging pitfalls and how to transform your logging strategy from an overwhelming flood of information into a precise debugging instrument.
The False Promise of “Log Everything”
When faced with a difficult bug, many developers resort to the “log everything” approach. The thinking goes: “If I can see everything that happens, I’ll surely catch the problem.” This leads to code like:
function processOrder(order) {
console.log("Starting to process order");
console.log("Order details:", order);
const validationResult = validateOrder(order);
console.log("Validation result:", validationResult);
if (!validationResult.valid) {
console.log("Order validation failed");
return { success: false, error: validationResult.error };
}
const processedOrder = transformOrder(order);
console.log("Processed order:", processedOrder);
const saveResult = saveOrder(processedOrder);
console.log("Save result:", saveResult);
console.log("Order processing complete");
return { success: true, orderId: saveResult.id };
}
The problem? When your application runs at scale, this approach quickly becomes counterproductive. You end up with gigabytes of logs where finding the relevant information is like finding a needle in a haystack. Even worse, excessive logging can significantly impact performance.
Common Logging Mistakes That Hinder Debugging
1. Inconsistent Log Levels
One of the most common mistakes is using inappropriate log levels or, worse, using only a single level for everything (usually console.log
or its equivalent).
Consider this example:
// Everything is at the same level
logger.info("Application started");
logger.info("Database connection established");
logger.info("Failed to process payment: Invalid card number");
logger.info("User profile updated");
When a critical error occurs, it gets buried among routine informational messages. A better approach uses appropriate log levels:
logger.info("Application started");
logger.debug("Database connection established");
logger.error("Failed to process payment: Invalid card number", { userId: user.id });
logger.info("User profile updated");
Most logging frameworks support at least these standard levels:
- ERROR: Application errors that need immediate attention
- WARN: Potentially harmful situations that might lead to errors
- INFO: Informational messages highlighting application progress
- DEBUG: Detailed information useful for debugging
- TRACE: Very detailed information, typically including function entry/exit
2. Logging Without Context
Another common mistake is logging messages without sufficient context:
logger.error("Database query failed");
This tells you something went wrong, but provides no information to help you diagnose why. A better approach includes relevant context:
logger.error("Database query failed", {
query: sqlQuery,
parameters: queryParams,
errorCode: err.code,
errorMessage: err.message,
userId: currentUser.id
});
Now you have enough information to understand what happened and potentially reproduce the issue.
3. Not Structuring Your Logs
Unstructured logs make automated analysis nearly impossible:
logger.info("User " + username + " logged in at " + new Date().toISOString() + " from IP " + ipAddress);
Instead, use structured logging where each piece of information is a distinct field:
logger.info("User login", {
username: username,
timestamp: new Date().toISOString(),
ipAddress: ipAddress,
action: "LOGIN"
});
Structured logs can be easily parsed, filtered, and analyzed by log management tools.
4. Logging Sensitive Information
Security should never be compromised for debugging convenience:
// DON'T DO THIS
logger.debug("User credentials", { username: user.email, password: password });
Instead, sanitize sensitive data:
logger.debug("Authentication attempt", {
username: user.email,
hasPassword: !!password, // Just log if password was provided
passwordLength: password ? password.length : 0
});
5. Neglecting Log Correlation
In distributed systems, failing to correlate logs across services makes debugging nearly impossible. Without correlation IDs, you can’t trace a request as it moves through your system.
// Service A
logger.info("Processing payment request");
// Service B (called by Service A)
logger.info("Validating payment details");
// Service C (called by Service B)
logger.info("Charging credit card");
A better approach uses a correlation ID that flows through all services:
// Service A
const requestId = generateUniqueId();
logger.info("Processing payment request", { requestId });
// Service B (received requestId from Service A)
logger.info("Validating payment details", { requestId });
// Service C (received requestId from Service B)
logger.info("Charging credit card", { requestId });
Building a Better Logging Strategy
Now that we’ve identified common pitfalls, let’s explore how to build an effective logging strategy that actually helps with debugging.
1. Define Clear Logging Objectives
Before adding a single log statement, ask yourself:
- What information would help diagnose problems in this code?
- What business events should be tracked for operational visibility?
- What metrics are important for monitoring system health?
Having clear objectives prevents logging sprawl and ensures your logs contain meaningful information.
2. Implement a Consistent Logging Framework
Choose a logging framework that supports:
- Multiple log levels
- Structured logging
- Configuration of output formats
- Runtime log level adjustment
For Node.js applications, libraries like Winston, Pino, or Bunyan are excellent choices. For Java, consider SLF4J with Logback or Log4j2. Python developers often use the built-in logging module or more advanced options like structlog.
Here’s an example of configuring Winston in a Node.js application:
const winston = require('winston');
const logger = winston.createLogger({
level: process.env.LOG_LEVEL || 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.json()
),
defaultMeta: { service: 'user-service' },
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
3. Create Logical Log Categories
Organize your logs into categories that make sense for your application domain. This might include:
- System events (startup, shutdown, configuration changes)
- Security events (login attempts, permission changes)
- Business transactions (orders, payments, user registrations)
- Integration points (API calls, database queries)
- Performance metrics (execution times, resource usage)
In many logging frameworks, you can create category-specific loggers:
// Java example with SLF4J
private static final Logger securityLogger = LoggerFactory.getLogger("security");
private static final Logger transactionLogger = LoggerFactory.getLogger("transactions");
private static final Logger integrationLogger = LoggerFactory.getLogger("integration");
4. Log at Boundaries and State Changes
Rather than logging everything, focus on system boundaries and state changes:
- Entry and exit points of key functions
- API request/response details
- Database or external service interactions
- Important state changes in your application
- Exception conditions and error handling
This approach provides visibility without overwhelming volume:
async function processPayment(paymentDetails) {
logger.debug('Payment processing started', { paymentId: paymentDetails.id });
try {
// Validate payment details
const validationResult = await validatePayment(paymentDetails);
if (!validationResult.valid) {
logger.warn('Payment validation failed', {
paymentId: paymentDetails.id,
reason: validationResult.reason
});
return { success: false, reason: validationResult.reason };
}
// Process the payment with payment provider
logger.debug('Sending payment to provider', {
paymentId: paymentDetails.id,
provider: paymentDetails.provider
});
const providerResult = await paymentProvider.processPayment(paymentDetails);
if (providerResult.success) {
logger.info('Payment processed successfully', {
paymentId: paymentDetails.id,
transactionId: providerResult.transactionId
});
return { success: true, transactionId: providerResult.transactionId };
} else {
logger.error('Payment provider rejected payment', {
paymentId: paymentDetails.id,
providerReason: providerResult.reason,
providerCode: providerResult.code
});
return { success: false, reason: providerResult.reason };
}
} catch (error) {
logger.error('Unexpected error processing payment', {
paymentId: paymentDetails.id,
error: error.message,
stack: error.stack
});
return { success: false, reason: 'internal_error' };
}
}
5. Implement a Request Context Pattern
In web applications, maintaining context across the request lifecycle is crucial. Use a request context pattern to ensure all logs for a single request are linked together:
// Express middleware example
app.use((req, res, next) => {
// Generate or extract request ID
const requestId = req.headers['x-request-id'] || uuidv4();
// Create request-scoped logger
req.logger = logger.child({
requestId,
path: req.path,
method: req.method,
ip: req.ip
});
req.logger.info('Request received');
// Track response
const start = Date.now();
res.on('finish', () => {
req.logger.info('Request completed', {
statusCode: res.statusCode,
duration: Date.now() - start
});
});
next();
});
Now in your route handlers, you can use req.logger
which automatically includes the request context in every log message.
6. Use Semantic Logging
Semantic logging means your log messages convey meaning beyond just text. Each log entry should represent a specific event or action in your system:
// Instead of:
logger.info("User updated their profile");
// Use semantic logging:
logger.info("user.profile.updated", {
userId: user.id,
changedFields: ['email', 'displayName'],
timestamp: new Date().toISOString()
});
This approach makes it much easier to search, filter, and analyze logs later. You could easily find all profile updates or specifically email changes.
Advanced Logging Techniques for Better Debugging
Once you’ve established a solid logging foundation, these advanced techniques can further enhance your debugging capabilities:
1. Contextual Exception Logging
Don’t just log exceptions; log the context in which they occurred:
try {
await processUserData(userData);
} catch (error) {
logger.error("Failed to process user data", {
userId: userData.id,
errorType: error.name,
errorMessage: error.message,
stackTrace: error.stack,
// Include relevant application state
currentStep: processingStep,
dataSize: JSON.stringify(userData).length,
// Include system context if relevant
memoryUsage: process.memoryUsage(),
// Include business context
userTier: userData.accountTier,
processingMode: config.processingMode
});
}
This comprehensive approach helps you understand not just what went wrong, but the entire context surrounding the failure.
2. Conditional Debug Logging
For performance-sensitive code, use conditional logging that only activates when needed:
function processLargeDataset(data) {
// Only log if debug is enabled
if (logger.isDebugEnabled()) {
logger.debug("Processing dataset", {
size: data.length,
firstRecordId: data[0]?.id,
lastRecordId: data[data.length-1]?.id
});
}
// Process data without logging overhead when debug is disabled
return transformData(data);
}
This approach lets you keep detailed logging in your code without performance penalties in production.
3. Timed Operations Logging
For performance debugging, log the duration of critical operations:
async function fetchAndProcessData() {
const startTime = Date.now();
logger.debug("Starting data fetch");
try {
const data = await fetchData();
logger.debug("Data fetched", {
duration: Date.now() - startTime,
recordCount: data.length
});
const processStart = Date.now();
const result = processData(data);
logger.debug("Data processing complete", {
fetchDuration: processStart - startTime,
processDuration: Date.now() - processStart,
totalDuration: Date.now() - startTime,
resultSize: result.length
});
return result;
} catch (error) {
logger.error("Data operation failed", {
phase: Date.now() - startTime > processStart ? "processing" : "fetching",
duration: Date.now() - startTime,
error: error.message
});
throw error;
}
}
This pattern helps identify performance bottlenecks and timing-related issues.
4. Progressive Logging Detail
Implement a strategy where logging detail increases as the code progresses through more specific error conditions:
function validateUserInput(input) {
// Basic validation with minimal logging
if (!input) {
logger.warn("Missing user input");
return { valid: false, reason: "missing_input" };
}
// More detailed logging for specific validation failures
if (!input.email) {
logger.warn("User input missing email", { inputFields: Object.keys(input) });
return { valid: false, reason: "missing_email" };
}
// Even more detailed logging for complex validation
if (!isValidEmail(input.email)) {
logger.warn("Invalid email format", {
providedEmail: input.email,
validationRule: EMAIL_REGEX.toString()
});
return { valid: false, reason: "invalid_email_format" };
}
return { valid: true };
}
This approach provides more detail exactly where it’s most useful, without cluttering logs with unnecessary information in the common case.
5. Feature Flag-Based Logging
For troubleshooting specific issues, implement feature flags that can dynamically increase logging detail for specific components or users:
function processUserAction(user, action) {
// Check if enhanced logging is enabled for this user
const enhancedLogging = featureFlags.isEnabled('enhanced-logging', user.id);
if (enhancedLogging) {
logger.debug("Enhanced logging enabled for user", { userId: user.id });
}
try {
// Normal processing code
const result = performAction(action);
if (enhancedLogging) {
logger.debug("Action result details", {
userId: user.id,
action: action.type,
resultDetails: JSON.stringify(result),
processingTime: result.processingTime
});
}
return result;
} catch (error) {
// Always log errors, but with more detail if enhanced logging is on
if (enhancedLogging) {
logger.error("Action processing failed with details", {
userId: user.id,
action: action.type,
actionDetails: JSON.stringify(action),
error: error.message,
stack: error.stack,
state: getCurrentState()
});
} else {
logger.error("Action processing failed", {
userId: user.id,
action: action.type,
error: error.message
});
}
throw error;
}
}
This approach lets you temporarily increase logging detail for specific users or features without changing code or affecting all users.
Leveraging Log Analysis Tools
Even the best logging strategy falls short if you can’t effectively analyze the logs. Modern log management tools can transform debugging from a needle-in-haystack search to a targeted investigation.
Key Features to Look For
- Real-time log aggregation: Collecting logs from all services in one place
- Structured log support: Parsing and indexing log fields for efficient searching
- Full-text search: Finding specific text across all logs
- Field-based filtering: Narrowing results by specific fields like user ID or error type
- Visualization: Graphing log trends and patterns
- Alerting: Notifying you when important log patterns emerge
- Log correlation: Following requests across distributed systems
Popular Log Management Solutions
- ELK Stack (Elasticsearch, Logstash, Kibana): Open-source solution with powerful search capabilities
- Splunk: Enterprise-grade log management with advanced analytics
- Datadog: Cloud monitoring with integrated logging and APM
- Grafana Loki: Horizontally-scalable, multi-tenant log aggregation system
- New Relic: Application performance monitoring with log management
- Sentry: Error tracking with contextual logging
Effective Log Searching Techniques
When debugging with logs, these search patterns often yield results:
- Trace a specific request: Search by request ID or correlation ID to see the complete journey
- Find similar errors: Search for error codes or message patterns
- Time-based investigation: Look at all logs around the time an issue was reported
- User-centric view: Filter logs by user ID to see everything that happened for a specific user
- Component-based filtering: Focus on logs from a specific service or component
- Error frequency analysis: Group by error types to identify the most common issues
Case Study: Transforming a Failing Logging Strategy
Let’s examine a real-world example of how improving a logging strategy dramatically improved debugging capabilities.
The Problem
A fintech company was experiencing intermittent payment processing failures that were difficult to diagnose. Their existing logging looked like this:
// Original code with poor logging
async function processPayment(paymentData) {
console.log("Processing payment", paymentData);
try {
const validated = validatePayment(paymentData);
if (!validated) {
console.log("Payment validation failed");
return false;
}
const paymentResult = await paymentGateway.charge(paymentData);
console.log("Payment result", paymentResult);
if (paymentResult.status === "success") {
await updateOrderStatus(paymentData.orderId, "PAID");
return true;
} else {
console.log("Payment failed");
return false;
}
} catch (err) {
console.error("Error processing payment", err);
return false;
}
}
The issues with this approach:
- No correlation between payment attempts and orders
- Inconsistent log levels
- Lack of structured data
- Missing important context for failures
- No timing information
The Solution
The team implemented a comprehensive logging overhaul:
async function processPayment(paymentData) {
const paymentContext = {
orderId: paymentData.orderId,
paymentId: paymentData.id,
amount: paymentData.amount,
currency: paymentData.currency,
paymentMethod: paymentData.method
};
logger.info("payment.processing.started", paymentContext);
const startTime = Date.now();
try {
// Validation
const validationStart = Date.now();
const validationResult = validatePayment(paymentData);
logger.debug("payment.validation.completed", {
...paymentContext,
duration: Date.now() - validationStart,
valid: validationResult.valid
});
if (!validationResult.valid) {
logger.warn("payment.validation.failed", {
...paymentContext,
reason: validationResult.reason,
failedFields: validationResult.failedFields
});
return { success: false, reason: validationResult.reason };
}
// Payment processing
const gatewayStart = Date.now();
logger.info("payment.gateway.request", {
...paymentContext,
gateway: paymentGateway.name
});
const paymentResult = await paymentGateway.charge(paymentData);
logger.info("payment.gateway.response", {
...paymentContext,
gateway: paymentGateway.name,
gatewayReference: paymentResult.reference,
status: paymentResult.status,
duration: Date.now() - gatewayStart
});
if (paymentResult.status === "success") {
// Order update
const updateStart = Date.now();
await updateOrderStatus(paymentData.orderId, "PAID");
logger.info("payment.completed.success", {
...paymentContext,
totalDuration: Date.now() - startTime,
orderUpdateDuration: Date.now() - updateStart,
gatewayReference: paymentResult.reference
});
return {
success: true,
reference: paymentResult.reference
};
} else {
logger.warn("payment.completed.failed", {
...paymentContext,
totalDuration: Date.now() - startTime,
gatewayReference: paymentResult.reference,
gatewayMessage: paymentResult.message,
gatewayCode: paymentResult.code
});
return {
success: false,
reason: "gateway_declined",
gatewayReason: paymentResult.message
};
}
} catch (error) {
logger.error("payment.error", {
...paymentContext,
errorType: error.name,
errorMessage: error.message,
stack: error.stack,
duration: Date.now() - startTime
});
return {
success: false,
reason: "processing_error"
};
}
}
The Results
After implementing the new logging strategy:
- Average time to diagnose payment issues decreased by 67%
- Customer support could quickly look up payment status by order ID
- The team identified a pattern of gateway timeouts during peak hours
- Performance bottlenecks in the validation process were discovered
- Security team could easily audit payment processing
The key improvements were:
- Consistent context in all log messages
- Semantic log events with clear naming
- Appropriate log levels for different situations
- Detailed error context
- Performance timing for each processing phase
- Structured data that could be easily queried
Conclusion: From Logging to Effective Debugging
Effective debugging isn’t about having more logs—it’s about having the right logs. A strategic approach to logging transforms it from a troubleshooting hindrance to your most powerful debugging ally.
To recap the key principles:
- Be intentional about what you log and why
- Use appropriate log levels consistently
- Provide rich context with structured data
- Focus on boundaries and state changes
- Maintain request context across your system
- Implement correlation IDs for distributed tracing
- Use semantic logging for better searchability
- Leverage advanced techniques like conditional and progressive logging
- Invest in proper log analysis tools
Remember that logging is ultimately about observability—making the internal state of your system visible and understandable. When done right, it transforms debugging from an exercise in frustration to a methodical process of discovery.
By evolving your logging strategy from “log everything” to “log strategically,” you’ll not only solve problems faster but also gain deeper insights into how your systems actually behave in production.
The next time you encounter a difficult bug, you won’t be drowning in logs—you’ll be following a clear trail of breadcrumbs straight to the root cause.