Why Your Local Environment Doesn’t Match Production: Solving the “It Works on My Machine” Syndrome
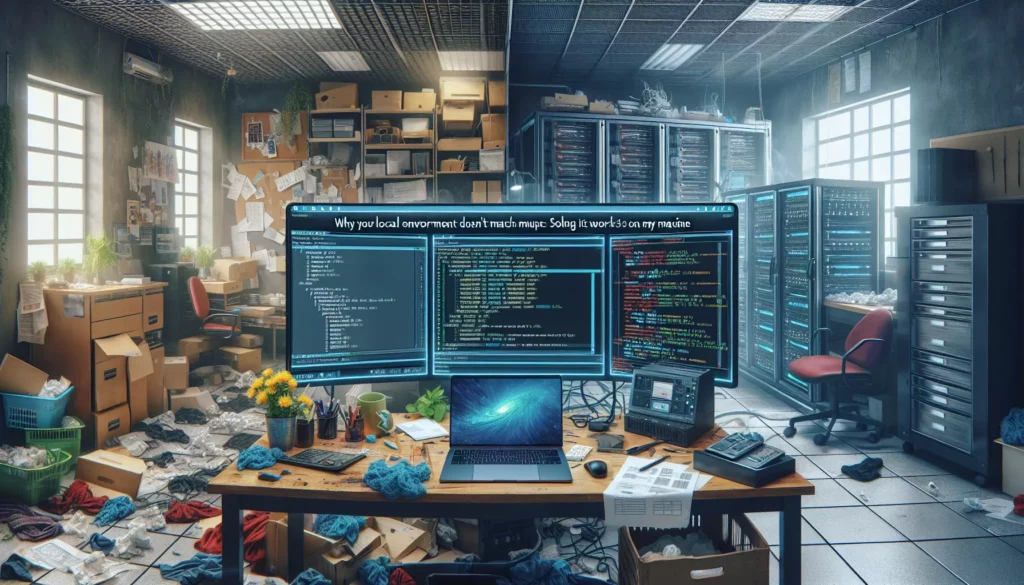
Every developer has experienced the frustration of code working flawlessly in their local environment only to break mysteriously when deployed to production. This phenomenon, often called the “It works on my machine” syndrome, is a persistent challenge in software development that can lead to unexpected bugs, deployment failures, and late nights debugging seemingly inexplicable issues.
In this comprehensive guide, we’ll explore the common causes of environment discrepancies, their impact on development workflows, and practical strategies to minimize these differences. By understanding and addressing these inconsistencies, you can create more reliable applications and spend less time troubleshooting production issues.
The Environment Mismatch Problem
Before diving into solutions, let’s clarify what we mean by environment mismatch and why it’s such a prevalent issue in software development.
What Is Environment Mismatch?
Environment mismatch occurs when code behaves differently across various environments (development, testing, staging, production) due to differences in configuration, dependencies, infrastructure, or other environmental factors. These discrepancies can cause applications to function correctly in one environment but fail in another.
This problem is often summarized by the classic developer excuse: “But it works on my machine!” This statement highlights the core issue: the local development environment differs from the production environment in ways that affect application behavior.
Why Environment Parity Matters
Achieving environment parity—where all environments closely resemble each other—is crucial for several reasons:
- Predictable deployments: When environments match, deployments become more predictable and less risky
- Faster debugging: Issues can be reproduced and fixed more easily when environments are similar
- Reduced time to market: Less time spent on environment-specific bugs means faster feature delivery
- Improved collaboration: Consistent environments make it easier for team members to work together
- Better testing accuracy: Tests yield more reliable results when testing environments mirror production
Common Causes of Environment Discrepancies
Let’s examine the most frequent culprits behind environment mismatches:
1. Operating System Differences
One of the most fundamental sources of discrepancy is the operating system itself. Developers might use Windows, macOS, or various Linux distributions locally, while production environments typically run on Linux servers. These differences can affect:
- File path handling: Windows uses backslashes (\) while Unix-based systems use forward slashes (/)
- Case sensitivity: Linux file systems are typically case-sensitive, while Windows is not
- Line endings: Windows uses CRLF (\r\n) while Unix systems use LF (\n)
- Process handling: Process creation, monitoring, and termination differ across operating systems
- Available system calls: Certain system functions may be available on one OS but not another
For example, consider this seemingly innocent file import in JavaScript:
import config from './Config.json';
On Windows, this works regardless of whether the file is named “Config.json” or “config.json” due to case insensitivity. On a Linux production server, however, if the actual filename is “config.json” (lowercase), this import will fail with a “file not found” error.
2. Dependency Version Mismatches
Dependencies are another major source of environment discrepancies. These issues can manifest in several ways:
- Different versions: Using different versions of libraries or frameworks across environments
- Missing dependencies: Dependencies installed locally but not specified in dependency management files
- Transitive dependency conflicts: Different resolution of dependency trees
- Native dependencies: Libraries that require compilation or have OS-specific binaries
Consider a common Python scenario where a developer might have installed a package globally:
import some_package # Works locally but not in production
If this package isn’t listed in requirements.txt or setup.py, it will be missing in production, causing import errors.
3. Configuration Differences
Configuration differences are subtle but significant sources of environment mismatch:
- Environment variables: Different values or missing variables across environments
- Configuration files: Different settings in config files or using different config files altogether
- Default values: Relying on default values that differ between environments
- Feature flags: Different feature flag settings across environments
A typical example is hardcoded local paths:
const logPath = '/Users/developer/projects/app/logs/'; // Works locally but fails in production
4. Database and Data Store Differences
Data-related discrepancies can be particularly challenging:
- Database versions: Using different database versions across environments
- Database engine differences: Using SQLite locally but PostgreSQL in production
- Initial data: Different seed data or missing data in various environments
- Schema differences: Inconsistent database schemas or missing migrations
- Connection parameters: Different connection pooling, timeout settings, etc.
A common scenario is using SQLite in development but a different database in production:
// Works in SQLite but fails in PostgreSQL
const query = "SELECT strftime('%Y-%m-%d', date_column) as formatted_date FROM table";
This query uses SQLite’s strftime
function, which doesn’t exist in PostgreSQL (which uses to_char
instead).
5. Infrastructure and Network Differences
The underlying infrastructure can vary significantly:
- Hardware resources: Different CPU, memory, or disk specifications
- Network topology: Different network configurations, latencies, or firewall rules
- Service availability: Services accessible locally but not in production (or vice versa)
- Cloud provider specifics: Features specific to AWS, Azure, GCP, etc.
A common example is code that assumes low network latency:
// Works locally but times out in production
const response = await fetch('https://api.example.com/data', { timeout: 500 });
6. Third-party Service Integration
External services introduce their own challenges:
- API versions: Using different API versions across environments
- Mock vs. real services: Using mocks locally but real services in production
- Rate limiting: Different rate limits or throttling behavior
- Authentication: Different authentication mechanisms or credentials
For instance, using a test API key locally that has different permissions than the production key.
7. Time and Locale Differences
Time and locale settings can cause subtle bugs:
- Timezone differences: Different server timezones across environments
- Locale settings: Different language, number formatting, or currency settings
- Date and time handling: Inconsistent date/time formatting or parsing
A classic example is date formatting that works differently across locales:
// May parse differently depending on locale settings
const date = new Date('04/05/2023'); // Is this April 5 or May 4?
Detecting Environment Discrepancies
Before you can fix environment mismatches, you need to identify them. Here are effective techniques for detecting discrepancies:
Logging and Monitoring
Comprehensive logging and monitoring can help identify environment-specific issues:
- Log environment information at startup (OS, versions, configuration)
- Implement detailed error logging with context information
- Use application performance monitoring (APM) tools to track behavior across environments
- Set up alerts for environment-specific anomalies
Example of logging environment details in Node.js:
function logEnvironmentInfo() {
console.log({
nodeVersion: process.version,
platform: process.platform,
architecture: process.arch,
env: process.env.NODE_ENV,
dependencies: process.versions,
// Add other relevant information
});
}
// Call at application startup
logEnvironmentInfo();
Environment Validation
Implement validation checks that run at startup to verify environment correctness:
- Check for required dependencies and their versions
- Validate configuration settings
- Test connections to critical services
- Verify file system permissions and access
Example of a Python environment validation function:
def validate_environment():
"""Validate that the environment is properly configured."""
# Check Python version
import sys
if sys.version_info < (3, 8):
raise RuntimeError("Python 3.8 or higher is required")
# Check critical dependencies
try:
import required_package
if required_package.__version__ < "2.0.0":
raise RuntimeError(f"required_package 2.0.0+ needed, found {required_package.__version__}")
except ImportError:
raise RuntimeError("required_package is missing")
# Check database connection
try:
from app import db
db.engine.connect()
except Exception as e:
raise RuntimeError(f"Database connection failed: {e}")
# Check environment variables
for var in ["API_KEY", "DATABASE_URL", "REDIS_URL"]:
if var not in os.environ:
raise RuntimeError(f"Required environment variable {var} is missing")
print("Environment validation passed")
Integration Tests Across Environments
Design tests specifically to catch environment discrepancies:
- Run the same test suite across all environments
- Include tests for environment-specific features
- Use canary deployments to test in production-like environments
- Implement smoke tests that run after deployment
Strategies to Minimize Environment Differences
Now that we understand the causes and detection methods, let's explore strategies to minimize environment differences:
1. Containerization with Docker
Containers provide a consistent environment by packaging your application with its dependencies:
- Identical runtime: Same container image runs locally and in production
- Dependency isolation: All dependencies are packaged within the container
- OS-level consistency: Same base OS regardless of host system
- Reproducible builds: Containers are built from declarative Dockerfiles
A basic Dockerfile example:
FROM node:16-alpine
WORKDIR /app
COPY package*.json ./
RUN npm ci
COPY . .
ENV NODE_ENV=production
EXPOSE 3000
CMD ["node", "server.js"]
With Docker Compose, you can define your entire application stack:
version: '3'
services:
app:
build: .
ports:
- "3000:3000"
environment:
- DATABASE_URL=postgres://user:password@db:5432/mydb
depends_on:
- db
db:
image: postgres:13
environment:
- POSTGRES_USER=user
- POSTGRES_PASSWORD=password
- POSTGRES_DB=mydb
volumes:
- postgres_data:/var/lib/postgresql/data
volumes:
postgres_data:
2. Infrastructure as Code (IaC)
IaC tools like Terraform, AWS CloudFormation, or Pulumi help define infrastructure in a consistent, reproducible way:
- Environment consistency: Same infrastructure definitions across environments
- Version-controlled infrastructure: Track infrastructure changes like code
- Automated provisioning: Reduce manual configuration errors
- Documentation as code: Infrastructure definition serves as documentation
Example Terraform configuration for consistent infrastructure:
provider "aws" {
region = var.aws_region
}
resource "aws_s3_bucket" "app_data" {
bucket = "${var.environment}-app-data"
acl = "private"
tags = {
Environment = var.environment
Project = "MyApp"
}
}
resource "aws_dynamodb_table" "app_table" {
name = "${var.environment}-app-table"
billing_mode = "PAY_PER_REQUEST"
hash_key = "id"
attribute {
name = "id"
type = "S"
}
tags = {
Environment = var.environment
Project = "MyApp"
}
}
3. Environment Configuration Management
Proper configuration management ensures consistent settings across environments:
- Environment variables: Use environment variables for environment-specific configuration
- Configuration files: Use environment-specific configuration files with a common structure
- Configuration services: Consider centralized configuration management (AWS Parameter Store, HashiCorp Vault, etc.)
- Secrets management: Use dedicated secrets management tools
Example of configuration management in a Node.js application:
// config.js
const environment = process.env.NODE_ENV || 'development';
// Base configuration with defaults
const baseConfig = {
logging: {
level: 'info',
format: 'json',
},
server: {
port: 3000,
timeout: 30000,
}
};
// Environment-specific configurations
const envConfigs = {
development: {
logging: {
level: 'debug',
format: 'pretty',
},
database: {
url: 'postgres://localhost:5432/myapp_dev',
pool: { max: 5 }
}
},
production: {
server: {
port: process.env.PORT || 8080,
},
database: {
url: process.env.DATABASE_URL,
ssl: true,
pool: { max: 20 }
}
}
};
// Merge configurations
const config = {
...baseConfig,
...(envConfigs[environment] || {}),
// Always allow environment variables to override
server: {
...(baseConfig.server || {}),
...((envConfigs[environment] || {}).server || {}),
port: process.env.PORT || (envConfigs[environment] || {}).server?.port || baseConfig.server.port,
}
};
module.exports = config;
4. Dependency Management
Strict dependency management ensures consistent libraries across environments:
- Lock files: Use package lock files (package-lock.json, yarn.lock, Pipfile.lock, etc.)
- Semantic versioning: Specify exact versions or appropriate version ranges
- Dependency scanning: Regularly audit dependencies for issues
- Private package repositories: Consider using private repositories for critical dependencies
Example of proper dependency specification in package.json:
{
"name": "my-app",
"version": "1.0.0",
"dependencies": {
"express": "4.17.1",
"lodash": "4.17.21",
"react": "17.0.2"
},
"devDependencies": {
"jest": "27.0.6",
"eslint": "7.32.0"
},
"engines": {
"node": ">=14.0.0 <17.0.0"
}
}
5. Database Migration and Seeding
Consistent database management helps prevent data-related environment issues:
- Database migrations: Use migration tools to maintain consistent schema across environments
- Data seeding: Provide consistent test data across environments
- Database version control: Track database changes alongside code
- Database abstraction: Use ORMs or query builders that handle database differences
Example of a database migration with Alembic (Python/SQLAlchemy):
"""add_user_table
Revision ID: a1b2c3d4e5f6
Revises:
Create Date: 2023-04-10 14:30:45.123456
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers
revision = 'a1b2c3d4e5f6'
down_revision = None
branch_labels = None
depends_on = None
def upgrade():
op.create_table(
'users',
sa.Column('id', sa.Integer(), nullable=False),
sa.Column('username', sa.String(50), nullable=False),
sa.Column('email', sa.String(100), nullable=False),
sa.Column('created_at', sa.DateTime(), server_default=sa.text('now()'), nullable=False),
sa.PrimaryKeyConstraint('id'),
sa.UniqueConstraint('username'),
sa.UniqueConstraint('email')
)
def downgrade():
op.drop_table('users')
6. Local Development Environments
Tools that create consistent development environments help reduce "works on my machine" issues:
- Development containers: VS Code Dev Containers, GitHub Codespaces
- Virtual machines: Vagrant for consistent VM-based development
- Local Kubernetes: Minikube, k3d, or Docker Desktop Kubernetes
- Environment setup scripts: Automated setup of local development environments
Example of a VS Code development container configuration:
{
"name": "Python Development",
"dockerFile": "Dockerfile",
"extensions": [
"ms-python.python",
"ms-python.vscode-pylance",
"ms-azuretools.vscode-docker"
],
"settings": {
"python.linting.enabled": true,
"python.linting.pylintEnabled": true,
"python.formatting.provider": "black",
"editor.formatOnSave": true
},
"forwardPorts": [5000],
"postCreateCommand": "pip install -r requirements.txt"
}
7. Continuous Integration and Deployment (CI/CD)
A robust CI/CD pipeline helps catch environment issues early:
- Build once, deploy many: Build artifacts once and promote them across environments
- Automated testing: Test in environments that mirror production
- Deployment automation: Reduce manual steps that can introduce inconsistencies
- Infrastructure validation: Verify infrastructure before deploying
Example GitHub Actions workflow for consistent CI/CD:
name: CI/CD Pipeline
on:
push:
branches: [main]
pull_request:
branches: [main]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v1
- name: Build and cache Docker image
uses: docker/build-push-action@v2
with:
context: .
push: false
load: true
tags: myapp:${{ github.sha }}
cache-from: type=gha
cache-to: type=gha,mode=max
- name: Run tests inside container
run: |
docker run --rm myapp:${{ github.sha }} npm test
- name: Login to DockerHub
if: github.event_name != 'pull_request'
uses: docker/login-action@v1
with:
username: ${{ secrets.DOCKERHUB_USERNAME }}
password: ${{ secrets.DOCKERHUB_TOKEN }}
- name: Push image
if: github.event_name != 'pull_request'
uses: docker/build-push-action@v2
with:
context: .
push: true
tags: |
myorg/myapp:latest
myorg/myapp:${{ github.sha }}
deploy-staging:
needs: build
if: github.event_name != 'pull_request'
runs-on: ubuntu-latest
steps:
- name: Deploy to staging
run: |
# Deploy the image to staging environment
echo "Deploying myorg/myapp:${{ github.sha }} to staging"
- name: Run integration tests
run: |
# Run integration tests against staging environment
echo "Running integration tests against staging"
deploy-production:
needs: deploy-staging
if: github.event_name != 'pull_request'
runs-on: ubuntu-latest
steps:
- name: Deploy to production
run: |
# Deploy the image to production environment
echo "Deploying myorg/myapp:${{ github.sha }} to production"
Advanced Strategies for Environment Parity
For teams facing particularly challenging environment discrepancies, consider these advanced strategies:
1. Feature Flags and Toggles
Feature flags allow you to control feature availability across environments:
- Gradually roll out features to production
- Test features in production without exposing them to all users
- Quickly disable problematic features without redeployment
- Support different feature sets across environments
Example implementation using a feature flag service:
// Feature flag check
function isFeatureEnabled(featureName, userId) {
// Check if feature is enabled for this environment and user
return featureFlagService.isEnabled(featureName, {
environment: process.env.NODE_ENV,
userId: userId
});
}
// Usage in code
if (isFeatureEnabled('new-payment-process', user.id)) {
// Use new payment process
return processPaymentV2(payment);
} else {
// Use old payment process
return processPaymentV1(payment);
}
2. Service Virtualization and API Mocking
For third-party service dependencies, consider:
- Mock external APIs in development and testing
- Record and replay actual API responses
- Simulate various API conditions (latency, errors, etc.)
- Use consistent API mocks across environments
Example using a service virtualization tool:
// Configure API mocking
const mockServer = setupMockServer({
baseUrl: 'https://api.payment-provider.com',
mode: process.env.API_MODE || 'replay', // 'replay', 'record', or 'live'
fixtures: './test/fixtures/api-responses',
});
// Add mock responses
mockServer.mock({
path: '/v1/payments',
method: 'POST',
response: {
status: 200,
body: {
id: 'pay_mock123',
status: 'succeeded',
amount: 1000
}
},
// Only use this mock in development and testing
environments: ['development', 'test']
});
3. Production-like Staging Environments
Create staging environments that closely mirror production:
- Use the same infrastructure providers and configurations
- Implement similar scaling and load patterns
- Use anonymized copies of production data
- Apply the same security controls and monitoring
4. Chaos Engineering
Proactively test system resilience to environmental differences:
- Simulate infrastructure failures
- Introduce network latency and partitions
- Test with resource constraints (CPU, memory, disk)
- Randomly terminate services to test recovery
Example using a chaos engineering tool:
// Define a chaos experiment
const experiment = {
name: "database_connection_failure",
hypothesis: "The application remains available when the database connection fails temporarily",
steadyState: {
// Verify the system is healthy before starting
request: {
url: "http://myapp.internal/health",
method: "GET"
},
expect: { statusCode: 200 }
},
method: [
{
// Introduce network latency to database
type: "network",
target: { host: "database.internal", port: 5432 },
action: "delay",
parameters: { latency: 3000, jitter: 500 }
},
{
// Then briefly terminate connections
type: "network",
target: { host: "database.internal", port: 5432 },
action: "disconnect",
parameters: { duration: 15 }
}
],
verification: [
// Verify the application remains responsive
{
request: {
url: "http://myapp.internal/api/status",
method: "GET"
},
expect: { statusCode: 200 }
}
]
};
Handling Unavoidable Environment Differences
Despite your best efforts, some environment differences may be unavoidable. Here's how to handle them gracefully:
1. Environment-aware Code
Design your code to adapt to different environments:
- Use environment detection to adjust behavior
- Implement fallbacks and graceful degradation
- Design for different operational parameters
Example of environment-aware code:
// Determine cache strategy based on environment
function getCacheStrategy() {
switch (process.env.NODE_ENV) {
case 'production':
// In production, use Redis
return new RedisCache({
host: process.env.REDIS_HOST,
port: process.env.REDIS_PORT
});
case 'development':
// In development, use in-memory cache
return new InMemoryCache();
default:
// In testing, use no-op cache
return new NoOpCache();
}
}
2. Graceful Degradation
Design your application to handle missing services or features:
- Implement fallback mechanisms
- Provide meaningful error messages
- Degrade functionality rather than failing completely
Example of graceful degradation:
async function fetchUserRecommendations(userId) {
try {
// Try to get personalized recommendations
return await recommendationService.getPersonalizedRecommendations(userId);
} catch (error) {
// Log the error
logger.error('Failed to fetch personalized recommendations', { userId, error });
try {
// Fall back to popular items
return await recommendationService.getPopularItems();
} catch (fallbackError) {
// Log the fallback error
logger.error('Failed to fetch popular items fallback', { fallbackError });
// Return a hardcoded default list as last resort
return DEFAULT_RECOMMENDATIONS;
}
}
}
3. Comprehensive Logging and Monitoring
When differences can't be eliminated, ensure they're visible:
- Log environment-specific behavior
- Track environment-specific metrics
- Set up alerts for significant deviations
- Collect detailed context for troubleshooting
Real-world Case Studies
Let's examine how real companies have tackled environment discrepancies:
Case Study 1: Netflix and Chaos Engineering
Netflix pioneered chaos engineering with their Chaos Monkey tool, which randomly terminates instances in production to ensure their systems can handle unexpected environment changes. This approach has helped them build resilient systems that work consistently across their global infrastructure.
Case Study 2: Spotify's Deployment Pipeline
Spotify implemented a build-once-deploy-many approach where the same artifact moves through development, testing, and production environments. This ensures that what gets tested is exactly what goes to production, minimizing environment-specific issues.
Case Study 3: Etsy's Feature Flagging
Etsy uses feature flags extensively to control feature rollout across environments. This allows them to test features in production with limited exposure, gradually increasing availability as confidence grows.
Conclusion
The "it works on my machine" problem is a persistent challenge in software development, but with the right strategies, you can minimize environment discrepancies and their impact:
- Containerization provides consistent runtime environments