Why Your Learning Isn’t Sticking Despite Daily Practice
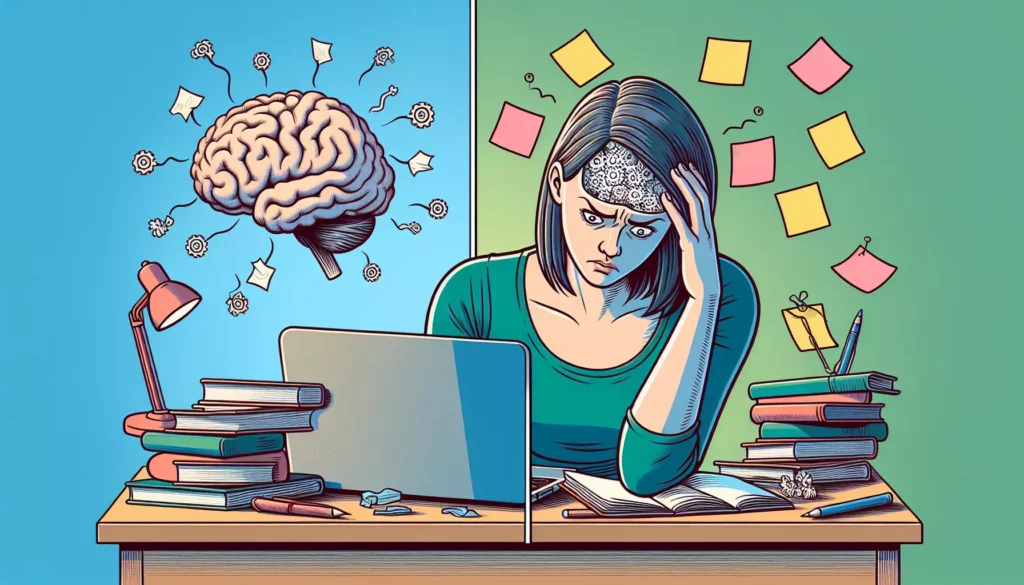
You’ve been diligently practicing coding every day. You’ve watched countless tutorials, solved numerous problems, and even built a few projects. Yet, when you sit down for that technical interview or try to solve a new problem, your mind goes blank. Why isn’t all that learning sticking?
This frustrating phenomenon isn’t unique to you. Many aspiring programmers and even experienced developers face this challenge. In this comprehensive guide, we’ll explore why your learning might not be translating into lasting knowledge and practical skills, despite your consistent practice.
The Illusion of Learning vs. Actual Learning
One of the most common pitfalls in learning to code is mistaking familiarity with mastery. When you read through code examples or watch tutorials, the solutions often seem obvious. This creates what psychologists call the “illusion of learning.”
Research published in the Journal of Educational Psychology suggests that passive learning methods (like watching videos or reading) create a false sense of understanding. You recognize the concepts being taught and might think, “Yes, I know this,” but recognition is not the same as recall or application.
The Recognition vs. Recall Problem
Consider this scenario: You’ve just finished watching a tutorial on implementing a binary search algorithm. The instructor’s explanation makes perfect sense, and you follow along with every step. You feel confident that you understand the concept.
But when you close the tutorial and try to implement the algorithm from scratch, you struggle to remember the key steps or the logic behind them. This gap between recognizing information when it’s presented to you and being able to recall and apply it independently is at the heart of the learning challenge.
Here’s a simple code example that might look familiar when you see it:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
But could you write this function from scratch without looking at a reference? That’s the difference between recognition and recall.
The Problem with Passive Learning Techniques
Many traditional learning resources in programming rely heavily on passive consumption. You watch a video, read documentation, or follow along with tutorials. While these resources provide valuable information, they often fail to engage the deeper cognitive processes necessary for long term retention.
Why Tutorial Hell Is Real
“Tutorial hell” is a term many programmers use to describe the state of endlessly consuming tutorials without being able to build anything independently. It’s a common trap because tutorials provide a structured path and immediate gratification. You feel productive as you follow along, but this approach rarely builds the mental models needed for independent problem solving.
Research in cognitive science shows that learning is most effective when it involves:
- Active engagement with the material
- Retrieval practice (testing yourself)
- Elaboration (explaining concepts in your own words)
- Interleaving (mixing different topics rather than focusing on one)
Most tutorials and passive learning resources don’t naturally incorporate these elements, which is why your daily practice might not be as effective as you hope.
The Forgetting Curve and Spaced Repetition
In the 1880s, Hermann Ebbinghaus discovered what he called the “forgetting curve,” which shows how information is lost over time when there is no attempt to retain it. His research demonstrated that without reinforcement, people forget approximately:
- 50% of new information within an hour
- 70% within 24 hours
- 90% within a week
This explains why concepts you learned last week might seem foreign when you encounter them again today.
How Spaced Repetition Counteracts Forgetting
Spaced repetition is a learning technique that involves reviewing information at increasing intervals. Instead of cramming all at once, you revisit material just as you’re about to forget it, which strengthens the neural pathways associated with that knowledge.
For programmers, this might look like:
- Learning a new concept (e.g., recursion)
- Practicing it immediately
- Reviewing it again the next day
- Then reviewing after 3 days
- Then after a week
- Then after two weeks, and so on
Many programmers practice daily but don’t strategically revisit previously learned concepts, leading to knowledge decay over time.
The Importance of Deliberate Practice
Not all practice is created equal. Psychologist K. Anders Ericsson, known for his research on expertise, distinguished between mere repetition and what he called “deliberate practice.” Deliberate practice is characterized by:
- Focused effort on improving specific aspects of performance
- Working at the edge of your current abilities
- Receiving immediate, informative feedback
- Developing mental models that allow for continued improvement
Simply coding daily without these elements may not lead to significant improvement.
The Zone of Proximal Development
Educational psychologist Lev Vygotsky introduced the concept of the “zone of proximal development” (ZPD), which describes the gap between what a learner can do without help and what they can achieve with guidance. Effective learning happens within this zone, where challenges are neither too easy nor too difficult.
Many programming learners either:
- Practice with problems that are too easy, leading to minimal growth
- Attempt problems far beyond their current abilities, leading to frustration
Finding that sweet spot is crucial for effective learning.
The Missing Link: Mental Models and Conceptual Understanding
One of the biggest reasons learning doesn’t stick is a focus on syntax and procedures rather than building robust mental models of how programming concepts work.
What Are Mental Models?
A mental model is an explanation of how something works. It’s your internal representation of a concept that allows you to reason about it, make predictions, and apply it in new contexts.
For example, many beginners learn array methods as isolated functions to memorize:
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(num => num * 2); // [2, 4, 6, 8, 10]
But developers with strong mental models understand the deeper concepts:
- Arrays as ordered collections with indexed access
- Higher order functions that can transform collections
- The relationship between map, filter, reduce, and other array methods
- The functional programming principles underlying these methods
This deeper understanding allows them to apply these concepts in novel situations, even when the syntax might be different.
Building Better Mental Models
To build stronger mental models:
- Explain concepts in your own words (the Feynman Technique)
- Draw diagrams to visualize relationships between concepts
- Implement concepts from scratch rather than copy pasting
- Compare and contrast related concepts to understand their differences and similarities
For example, instead of just memorizing how to use a hash map, try implementing one from scratch to understand how it works under the hood:
class SimpleHashMap {
constructor(size = 10) {
this.buckets = new Array(size).fill(null).map(() => []);
}
hash(key) {
let hashCode = 0;
for (let i = 0; i < key.length; i++) {
hashCode += key.charCodeAt(i);
}
return hashCode % this.buckets.length;
}
set(key, value) {
const index = this.hash(key);
const bucket = this.buckets[index];
for (let i = 0; i < bucket.length; i++) {
if (bucket[i][0] === key) {
bucket[i][1] = value;
return;
}
}
bucket.push([key, value]);
}
get(key) {
const index = this.hash(key);
const bucket = this.buckets[index];
for (let i = 0; i < bucket.length; i++) {
if (bucket[i][0] === key) {
return bucket[i][1];
}
}
return undefined;
}
}
This exercise builds a much deeper understanding than simply using a built in Map object.
The Role of Context in Learning
Learning isolated facts or techniques without context can lead to what cognitive scientists call “inert knowledge” — information that exists in your mind but isn’t readily accessible when needed.
Contextual Learning vs. Isolated Facts
Consider these two approaches to learning about sorting algorithms:
Approach 1 (Isolated): Memorize the steps of quicksort, bubble sort, and merge sort separately.
Approach 2 (Contextual): Learn about sorting algorithms in the context of:
- When each algorithm performs best or worst
- The trade offs between time complexity and space complexity
- Real world scenarios where each might be preferred
- How the algorithms relate to broader concepts like divide and conquer
The second approach creates a rich network of associations that makes the knowledge more accessible and applicable.
Creating Meaningful Context
To enhance contextual learning:
- Build projects that integrate multiple concepts
- Study real world codebases to see how concepts are applied in practice
- Relate new information to what you already know
- Discuss concepts with peers or mentors to hear different perspectives
For example, instead of just learning React hooks in isolation, build a small application that uses multiple hooks together to solve a real problem.
The Problem of Transfer: Applying Knowledge in New Contexts
One of the most challenging aspects of learning programming is the ability to transfer knowledge from one context to another. This is known as “transfer of learning” in educational psychology.
Near Transfer vs. Far Transfer
Near transfer involves applying knowledge in situations very similar to the original learning context. For example, using a sorting algorithm you learned in JavaScript to implement the same algorithm in Python.
Far transfer involves applying principles in substantially different contexts. For example, using the principles of recursion you learned when solving tree problems to implement a recursive approach for parsing nested JSON.
Studies show that far transfer is much more difficult and requires deeper conceptual understanding.
Improving Transfer Ability
To improve your ability to transfer knowledge:
- Practice with varied examples rather than similar ones
- Abstract principles from specific implementations
- Explicitly look for connections between different domains
- Challenge yourself to apply concepts in new ways
For instance, if you’ve learned about event handling in web development, try to identify similar patterns in mobile app development or even in non programming contexts.
Emotional and Psychological Barriers to Learning
Learning isn’t just a cognitive process; it’s deeply influenced by emotional and psychological factors.
The Impact of Anxiety and Impostor Syndrome
Research shows that anxiety can significantly impair working memory, which is crucial for problem solving. Many programmers experience “blank mind syndrome” during interviews or when facing challenging problems, not because they don’t know the material, but because anxiety is blocking access to that knowledge.
Impostor syndrome, the persistent feeling that you’re not as competent as others perceive you to be, can also undermine learning by:
- Causing you to doubt your abilities even when you’re making progress
- Creating fear of asking questions or seeking help
- Leading to avoidance of challenging problems that would promote growth
Mindset and Learning
Psychologist Carol Dweck’s research on mindset shows that how you view your abilities can significantly impact learning outcomes:
- Fixed mindset: Believing your abilities are static traits (“I’m just not good at algorithms”)
- Growth mindset: Believing your abilities can be developed through effort and practice (“I haven’t mastered algorithms yet”)
Studies show that learners with a growth mindset tend to persist longer when facing challenges and ultimately achieve higher levels of mastery.
Strategies for Emotional Resilience
To address these psychological barriers:
- Normalize struggle as an essential part of learning
- Celebrate small wins to build confidence
- Practice self compassion when facing difficulties
- Build a supportive community of fellow learners
Practical Strategies to Make Learning Stick
Now that we understand the common obstacles to effective learning, let’s explore practical strategies to overcome them.
1. Implement Active Recall
Active recall involves testing yourself on material rather than passively reviewing it. This strengthens memory pathways and identifies knowledge gaps.
Implementation:
- After learning a concept, close all references and try to explain it in your own words
- Implement algorithms from memory, then check your solution
- Create flashcards for key concepts and review them regularly
- Teach concepts to someone else (or even to a rubber duck)
2. Practice Spaced Repetition Systematically
Rather than random practice, implement a systematic approach to spaced repetition.
Implementation:
- Use spaced repetition software like Anki for conceptual knowledge
- Keep a “revision schedule” for algorithms and data structures you’ve learned
- Review older projects periodically and try to enhance them with new knowledge
- Maintain a personal knowledge base that you regularly update and review
3. Focus on Understanding, Not Just Implementation
Prioritize deep understanding over quick solutions.
Implementation:
- When learning a new algorithm, trace through it with different inputs
- Analyze the time and space complexity of your solutions
- Compare multiple approaches to the same problem
- Implement the same solution in different programming languages
For example, when implementing a breadth first search algorithm, don’t just memorize the code pattern, but understand why a queue is used instead of a stack, and how the algorithm traverses the data structure:
function breadthFirstSearch(graph, startNode) {
const visited = new Set();
const queue = [startNode];
visited.add(startNode);
while (queue.length > 0) {
const currentNode = queue.shift();
console.log(currentNode); // Process the node
for (const neighbor of graph[currentNode]) {
if (!visited.has(neighbor)) {
visited.add(neighbor);
queue.push(neighbor);
}
}
}
}
4. Apply Deliberate Practice Principles
Structure your practice sessions for maximum effectiveness.
Implementation:
- Set specific learning goals for each practice session
- Focus on areas where you struggle rather than what you already know
- Seek immediate feedback through testing, code reviews, or mentorship
- Reflect on your practice sessions to identify patterns and areas for improvement
5. Build Projects That Challenge You
Projects provide context and motivation for learning.
Implementation:
- Start with projects slightly beyond your current abilities
- Break large projects into manageable components
- Implement features that require you to learn new concepts
- Refactor your projects as you learn better practices
6. Teach and Explain What You Learn
Teaching is one of the most effective ways to solidify understanding.
Implementation:
- Write blog posts explaining concepts you’re learning
- Create tutorials or code walkthroughs for others
- Participate in pair programming sessions
- Answer questions on platforms like Stack Overflow or Reddit
7. Create Meaningful Connections Between Concepts
Building a network of related concepts enhances recall and application.
Implementation:
- Create mind maps showing relationships between programming concepts
- Compare and contrast similar algorithms or data structures
- Identify patterns across different programming paradigms
- Connect computer science concepts to real world analogies
Customizing Your Learning Approach
There’s no one size fits all approach to learning programming. Different individuals have different learning preferences, strengths, and challenges.
Identifying Your Learning Style
While the concept of fixed “learning styles” (visual, auditory, kinesthetic) has been largely debunked by research, it’s still valuable to reflect on how you learn most effectively:
- Do you understand concepts better through visual representations?
- Do you learn best by discussing concepts with others?
- Do you prefer to dive into code examples first or understand theory first?
- Do you learn better through structured courses or through exploration?
Understanding these preferences can help you select learning resources and strategies that work best for you.
Adapting to Different Types of Programming Knowledge
Different aspects of programming may require different learning approaches:
- Syntax and language features: Benefit from spaced repetition and regular practice
- Algorithms and data structures: Require conceptual understanding and varied problem solving
- System design: Benefits from case studies and building increasingly complex systems
- Domain specific knowledge: Often learned through project work and application
Tailor your learning strategies to the type of knowledge you’re trying to acquire.
The Role of Community and Collaboration
Learning in isolation can significantly limit your growth as a programmer. The programming community offers diverse perspectives, feedback, and support that can enhance your learning.
Benefits of Collaborative Learning
Research in educational psychology shows that collaborative learning can:
- Expose you to different problem solving approaches
- Help identify blind spots in your understanding
- Provide motivation and accountability
- Develop communication skills essential for professional success
Finding and Engaging with Learning Communities
To leverage collaborative learning:
- Participate in coding communities like GitHub, Stack Overflow, or Discord servers
- Join or form study groups with peers at similar learning stages
- Contribute to open source projects to learn from experienced developers
- Attend hackathons or coding meetups to collaborate on projects
- Find a mentor who can guide your learning journey
Measuring Progress Effectively
Without effective ways to measure progress, it’s easy to feel stuck or to miss evidence of your growth.
Metrics Beyond Completion
Many learners track progress by counting completed tutorials or problems, but more meaningful metrics include:
- Problem solving independence: Can you solve problems without looking up solutions?
- Implementation time: Are you solving problems more quickly than before?
- Code quality: Is your code becoming more elegant, efficient, and maintainable?
- Conceptual connections: Can you see relationships between different programming concepts?
- Teaching ability: Can you explain concepts clearly to others?
Creating Learning Feedback Loops
Effective learning requires regular feedback:
- Seek code reviews from more experienced developers
- Compare your solutions to reference implementations
- Use automated testing to validate your code
- Keep a learning journal to track insights and challenges
- Periodically revisit old problems to see how your approach has evolved
Conclusion: Building a Sustainable Learning Practice
The journey to programming mastery is a marathon, not a sprint. Making your learning stick requires not just daily practice, but thoughtful, strategic practice that addresses the cognitive, emotional, and social aspects of learning.
By understanding why traditional approaches often fail and implementing evidence based learning strategies, you can transform your daily practice from a frustrating cycle of forgetting to a sustainable path toward expertise.
Remember that learning is not linear. You’ll experience plateaus, breakthroughs, and occasional setbacks. The key is to maintain curiosity, embrace challenges, and continuously refine your learning approach based on what works best for you.
Most importantly, be patient with yourself. Developing true programming expertise takes time, but with the right approaches, your knowledge will not only stick but will continue to grow and evolve throughout your career.
The next time you find yourself frustrated that your learning isn’t sticking despite consistent practice, revisit these strategies and consider which aspects of your learning approach might need adjustment. Small changes in how you practice can lead to dramatic improvements in how well you retain and apply programming knowledge.