Why Your Learning Approach Needs to Change: A New Paradigm for Coding Education
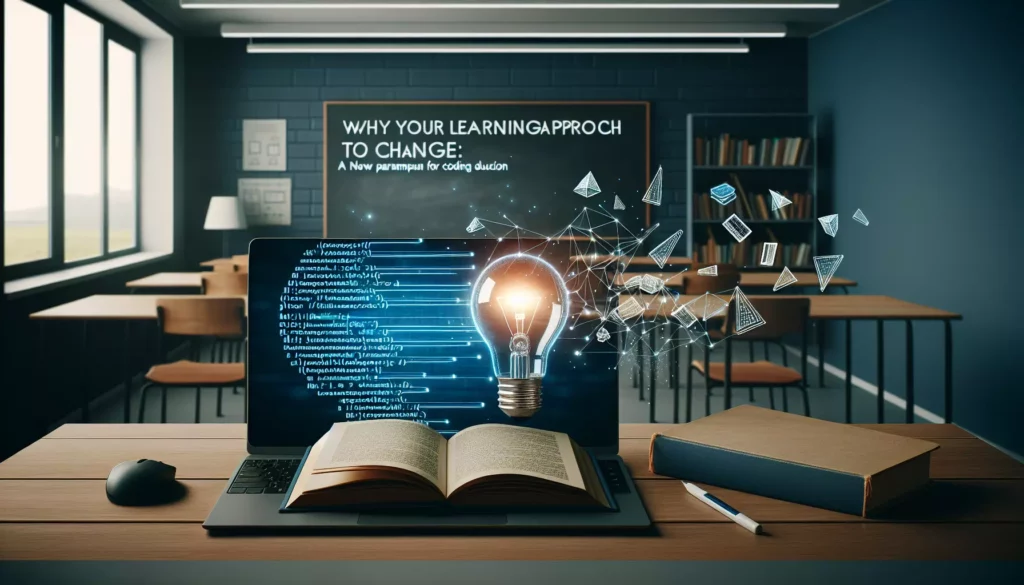
In the rapidly evolving landscape of technology, the way we learn to code has remained surprisingly static. Despite the dramatic shifts in how programming is applied in the real world, many learners still approach coding education with outdated methodologies that fail to prepare them for the challenges of modern software development.
The traditional approach—watching video lectures, reading documentation, and completing isolated exercises—simply isn’t cutting it anymore. It’s time for a fundamental shift in how we approach learning programming.
This article explores why your current learning approach might be holding you back and presents a more effective paradigm for mastering coding skills in today’s competitive tech environment.
The Problem with Traditional Coding Education
Traditional coding education often falls short in several key areas:
Passive Learning Doesn’t Stick
Watching someone else code is seductively comfortable. You follow along, nodding in agreement, convinced you understand the concepts. But this passive consumption creates an illusion of learning without the neural connections that come from actually solving problems yourself.
Studies in cognitive science show that passive learning—reading or watching without active engagement—results in dramatically lower retention rates. According to research on the “forgetting curve,” learners can lose up to 90% of what they’ve learned within a week if they don’t actively apply it.
Disconnected From Real World Applications
Many coding courses teach programming concepts in isolation, divorced from practical applications. You might learn syntax perfectly but struggle to understand how these pieces fit together in actual software development.
This disconnect creates “tutorial hell”—the frustrating cycle where learners can follow guided examples but feel lost when facing a blank editor and a real problem to solve.
One-Size-Fits-All Pacing
Traditional courses move at a predetermined pace that rarely matches an individual’s optimal learning speed. Some concepts might be belabored when you’ve already grasped them, while complex ideas might be rushed through before you’ve had time to internalize them.
This inflexible pacing means most learners are either bored or overwhelmed at any given point, neither of which is conducive to effective learning.
Lack of Immediate, Personalized Feedback
Perhaps most critically, traditional learning approaches often lack the immediate feedback loop essential for rapid improvement. When you submit an assignment in a traditional course, you might wait days for feedback—by which time you’ve lost the context and mental state in which you wrote the code.
The Science of Effective Learning
To understand why we need to change our approach to learning programming, it helps to examine what cognitive science tells us about how humans actually learn complex skills.
Active Recall Trumps Passive Review
Research consistently shows that testing yourself—actively retrieving information from memory—is far more effective than simply reviewing material. This “testing effect” or “retrieval practice” strengthens neural pathways and improves long-term retention.
For programmers, this means that writing code from scratch is significantly more valuable than reading or watching others code. The mental effort required to recall syntax, algorithms, and problem-solving approaches cements these concepts in your memory.
Spaced Repetition Optimizes Memory
Our brains are designed to forget information that doesn’t seem important. Spaced repetition—revisiting material at gradually increasing intervals—signals to your brain that certain information is worth retaining.
This is why revisiting programming concepts just as you’re about to forget them is more effective than cramming or constant review. Modern learning systems can track your performance on different concepts and schedule optimal review times.
Deliberate Practice Drives Mastery
Not all practice is created equal. Deliberate practice—focused, structured practice on specific weaknesses with immediate feedback—is what separates experts from amateurs in any field.
For coding, this means identifying your specific weaknesses (whether it’s understanding recursion, optimizing algorithms, or structuring large codebases) and deliberately practicing those aspects with immediate feedback on your performance.
The Importance of Mental Models
Experts in any domain organize knowledge differently than novices. They develop robust mental models that connect concepts and allow them to reason about novel situations.
Effective learning approaches help you build these mental models by emphasizing the relationships between concepts rather than teaching them in isolation. Understanding how variables, functions, data structures, and algorithms interact within a coherent system is more valuable than memorizing their individual definitions.
The New Paradigm: Interactive, Adaptive Learning
Given what we know about effective learning, a new paradigm for coding education is emerging—one that leverages technology to create more interactive, personalized, and effective learning experiences.
Interactive Learning Environments
Modern coding education platforms provide interactive environments where you can write, test, and debug code in real-time. These environments eliminate the friction of setting up development environments and allow you to focus on learning.
For example, consider this simple interactive exercise for understanding array manipulation:
// Try to implement a function that reverses an array without using the built-in reverse method
function reverseArray(arr) {
// Your code here
}
// Test your solution
console.log(reverseArray([1, 2, 3, 4, 5])); // Should output [5, 4, 3, 2, 1]
With an interactive environment, you can write your solution, run it immediately, and see if it works—creating a tight feedback loop that accelerates learning.
Adaptive Learning Paths
One-size-fits-all courses are being replaced by adaptive learning systems that adjust to your individual needs. These systems track your performance on different concepts and dynamically adjust the difficulty and focus of exercises.
If you’re struggling with recursion but excelling at iteration, an adaptive system will provide more practice with recursive problems at an appropriate difficulty level. This ensures you’re always working in your “zone of proximal development”—challenging enough to promote growth but not so difficult as to cause frustration.
AI-Powered Feedback and Guidance
Perhaps the most significant advancement in coding education is the integration of AI systems that can provide immediate, personalized feedback on your code.
Unlike traditional approaches where feedback might be delayed or generic, AI systems can analyze your code in real-time, identifying not just syntax errors but also logical flaws, performance issues, and stylistic improvements.
For example, if you write a function to find the maximum value in an array using a nested loop with O(n²) complexity, an AI assistant might suggest:
“Your solution works correctly, but it has a time complexity of O(n²) due to the nested loops. Consider using a single loop to track the maximum value seen so far, which would reduce the complexity to O(n).”
This immediate, specific feedback allows you to learn from mistakes and improve your approach on the spot, rather than developing bad habits that need to be unlearned later.
Project-Based Learning
The new paradigm emphasizes learning through building real projects rather than completing isolated exercises. This approach connects theoretical knowledge to practical applications and helps you develop the problem-solving skills needed in real-world development.
Projects also provide context for learning new concepts. Instead of learning about API calls in the abstract, you might build a weather application that requires fetching and displaying data from a weather API. This context makes the learning more meaningful and memorable.
Implementing the New Learning Paradigm
How can you apply these principles to your own coding education journey? Here are practical strategies for implementing this new learning paradigm:
Adopt an Active Learning Mindset
Shift from passive consumption to active engagement:
- For every tutorial you watch or article you read, challenge yourself to implement the concepts without looking at the reference material.
- Explain concepts in your own words, either through writing, teaching others, or simply talking through your understanding out loud.
- Modify example code to see how changes affect the outcome, developing an intuitive understanding of how the code works.
This active approach might feel more difficult initially, but the increased effort leads to stronger neural connections and better long-term retention.
Embrace Productive Struggle
Learning happens at the edge of your comfort zone. When you encounter a challenging problem, resist the urge to immediately look up the solution.
Give yourself time to struggle with the problem, trying different approaches and reasoning through the logic. This struggle is where deep learning occurs, as your brain forms new connections and refines its mental models.
Of course, there’s a balance—if you’re completely stuck after a reasonable effort, seeking help is appropriate. But the key is to exhaust your own problem-solving resources first.
Create a Feedback-Rich Environment
Seek out learning resources and communities that provide rapid feedback on your code:
- Use platforms with integrated testing that immediately validates your solutions.
- Participate in code reviews, either professionally or through online communities.
- Leverage AI coding assistants for immediate feedback on your code quality and approach.
- Find a mentor or peer group to discuss solutions and approaches.
The faster and more specific the feedback, the more quickly you’ll improve.
Build a Strategic Learning Plan
Rather than jumping between unrelated tutorials or courses, develop a structured learning plan that builds knowledge incrementally:
- Identify your specific goals (e.g., web development, data science, mobile apps)
- Map out the core concepts and skills needed for those goals
- Create a progression that builds from fundamentals to advanced topics
- Incorporate regular review of previously learned material
- Include projects that integrate multiple concepts
This structured approach ensures you’re building a comprehensive skill set rather than collecting fragmented knowledge.
Focus on Algorithmic Thinking
Beyond syntax and specific technologies, develop your ability to think algorithmically—breaking down problems into logical steps and translating those steps into code.
Platforms focused on algorithmic challenges provide structured practice in this critical skill. For example, consider this problem:
/*
Problem: Find all pairs of numbers in an array that sum to a target value.
Input: An array of integers and a target sum
Output: All pairs of integers that add up to the target sum
*/
function findPairs(numbers, targetSum) {
// Your solution here
}
This type of problem forces you to think about efficient approaches, data structures, and edge cases—skills that transfer across all programming domains.
Real-World Applications: Beyond the Basics
As you advance in your coding journey, the new learning paradigm becomes even more critical. Here’s how it applies to more advanced aspects of software development:
System Design and Architecture
Understanding how to design larger systems is challenging to learn through passive approaches. Interactive learning environments that let you experiment with different architectural patterns and see their implications are far more effective.
For example, exploring how different database choices affect application performance by actually implementing and testing alternatives provides insights that reading about databases never could.
Performance Optimization
Learning to write efficient code requires immediate feedback on performance characteristics. Modern learning platforms can show you real-time analysis of your code’s time and space complexity, helping you develop an intuition for optimization.
Consider this example showing how different implementations affect performance:
// Implementation 1: Using nested loops (O(n²))
function hasDuplicates1(array) {
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j]) return true;
}
}
return false;
}
// Implementation 2: Using a Set (O(n))
function hasDuplicates2(array) {
const seen = new Set();
for (const item of array) {
if (seen.has(item)) return true;
seen.add(item);
}
return false;
}
// Performance comparison with large input
const largeArray = Array.from({length: 10000}, () => Math.floor(Math.random() * 1000));
Seeing the dramatic performance difference between these implementations creates a deeper understanding than simply being told that Sets provide faster lookups.
Collaborative Development
Real-world development happens in teams, using version control, code reviews, and collaborative tools. Modern learning approaches incorporate these aspects from the beginning:
- Learning Git through interactive visualizations of branches and merges
- Participating in simulated code reviews where you both give and receive feedback
- Working on collaborative projects with other learners
These experiences prepare you for the social and collaborative aspects of professional development that are often neglected in traditional education.
Overcoming Challenges in the New Paradigm
While this new approach to learning programming offers significant benefits, it also comes with challenges:
Dealing with Information Overload
The abundance of learning resources can be overwhelming. To combat this:
- Be selective about your information sources, prioritizing quality over quantity
- Focus on fundamentals before specializing
- Create a learning schedule that limits the new information you consume in a given period
- Use spaced repetition systems to optimize review of previously learned material
Balancing Breadth and Depth
Modern software development requires both broad knowledge across multiple domains and deep expertise in specific areas. Finding this balance is challenging.
A helpful approach is to develop T-shaped knowledge: broad awareness across many areas with deep expertise in a few selected domains. This allows you to contribute meaningfully in your areas of expertise while still collaborating effectively with specialists in other domains.
Maintaining Motivation for the Long Haul
Learning to code is a marathon, not a sprint. To maintain motivation:
- Set both short-term and long-term goals to provide a sense of progress
- Build projects that genuinely interest you
- Celebrate small wins and milestones
- Connect with a community of learners for support and accountability
- Focus on progress rather than perfection
Remember that even experienced developers continually learn and adapt. The journey never truly ends—which is part of what makes software development such an engaging field.
Case Studies: Success with the New Learning Paradigm
To illustrate the effectiveness of this new approach, let’s look at some real-world examples:
From Tutorial Hell to Professional Developer
Alex had spent months jumping between tutorials, feeling like he was learning but unable to build anything independently. Frustrated with his lack of progress, he switched to a project-based approach with integrated feedback systems.
He started with a simple personal website, then added features incrementally—a blog system, user authentication, and finally a comment system. Each feature introduced new challenges that required him to apply concepts in context rather than isolation.
The immediate feedback from automated tests and code quality tools helped him identify and fix problems quickly. Within six months, he had a portfolio of projects that demonstrated his capabilities and secured his first developer role.
Accelerated Learning Through Deliberate Practice
Maria was preparing for technical interviews at major tech companies. Rather than trying to memorize solutions to common problems, she focused on developing her algorithmic thinking through deliberate practice.
She used a platform that analyzed her solutions and identified specific weaknesses—she struggled with dynamic programming and graph algorithms. Instead of continuing with random practice, she concentrated on these weak areas with progressively challenging problems.
The platform’s AI assistant provided immediate feedback on her solutions, suggesting optimizations and alternative approaches. This targeted practice allowed her to improve rapidly, and she successfully passed interviews at several top companies within three months.
Building Mental Models Through Interactive Learning
James was confused by asynchronous JavaScript despite watching numerous tutorials. The concepts made sense in isolation, but he couldn’t apply them effectively in his code.
He switched to an interactive learning environment that visualized the execution of asynchronous code, showing how the event loop, call stack, and callback queue interacted. By experimenting with different scenarios and seeing the results immediately, he developed a robust mental model of how asynchronous JavaScript works.
This understanding transferred to his projects, where he could now confidently implement complex asynchronous operations without getting lost in “callback hell” or misusing promises.
The Future of Coding Education
As we look ahead, several emerging trends are likely to further transform how we learn to code:
AI-Augmented Learning
AI systems are becoming increasingly sophisticated at understanding code and providing personalized guidance. Future learning platforms might include AI mentors that can:
- Analyze your coding patterns and suggest personalized learning paths
- Generate custom practice problems targeting your specific weaknesses
- Provide conversational explanations of complex concepts tailored to your learning style
- Simulate pair programming experiences, helping you reason through problems
These AI systems won’t replace human teachers but will augment them, providing personalized support at scale.
Immersive Learning Environments
Virtual and augmented reality technologies offer new possibilities for visualizing and interacting with code. Imagine:
- Manipulating data structures in 3D space to understand their operations
- Walking through a visual representation of an algorithm’s execution
- Collaborating with remote learners in shared virtual environments
These immersive experiences could make abstract concepts more concrete and accessible, especially for visual and kinesthetic learners.
Community-Driven Learning
The future of coding education will likely be more social and community-oriented, with platforms that facilitate:
- Peer review and feedback on code
- Collaborative problem-solving sessions
- Mentorship matching based on skills and learning goals
- Competitive and cooperative coding challenges
These social aspects not only enhance learning but also develop the collaboration skills essential for professional success.
Conclusion: Embracing the Change
The way we learn to code is undergoing a fundamental transformation—from passive, isolated study to active, interactive, and adaptive learning experiences. This shift isn’t just about using new tools or platforms; it’s about aligning our learning approaches with how our brains actually work.
By embracing active recall, spaced repetition, deliberate practice, and immediate feedback, we can learn more effectively and efficiently. By focusing on building robust mental models and connecting concepts to real-world applications, we can develop the problem-solving skills that define exceptional programmers.
The challenges of modern software development—increasing complexity, rapidly evolving technologies, and growing specialization—demand this new approach to learning. Those who adapt their learning methods will be better equipped to thrive in this dynamic field.
Whether you’re just beginning your coding journey or looking to level up your existing skills, consider how you can incorporate these principles into your learning process. Seek out interactive environments, embrace productive struggle, prioritize projects over tutorials, and build a feedback-rich learning ecosystem.
The future belongs to those who can learn, unlearn, and relearn effectively. By changing your approach to learning now, you’re investing in your ability to adapt and grow throughout your programming career.
Your learning approach doesn’t just determine how quickly you’ll master coding concepts—it shapes the kind of developer you’ll become. Choose wisely.