Why Your ‘Learn Python in 30 Days’ Course Isn’t Making You a Programmer
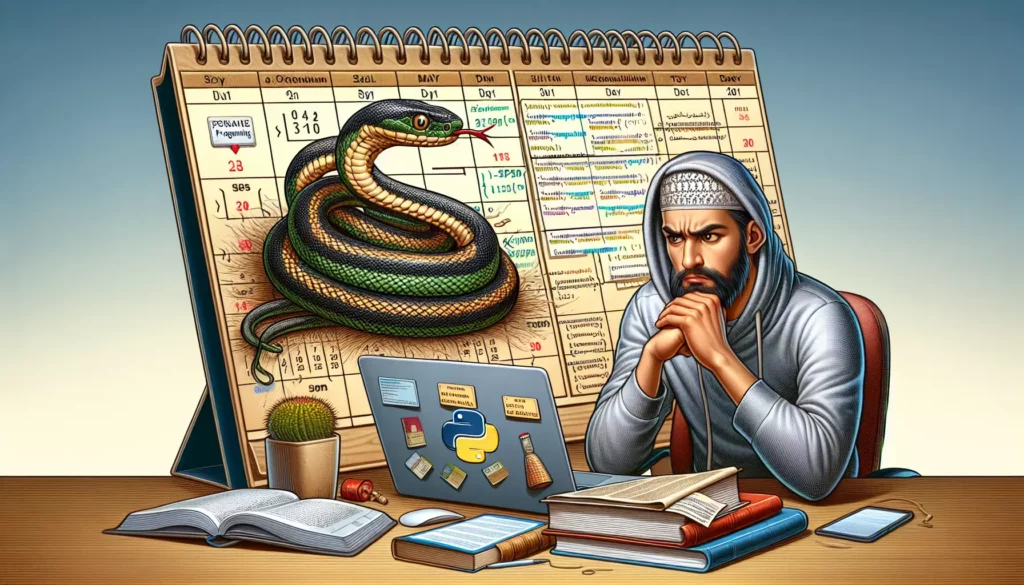
You’ve done it. You’ve completed that “Learn Python in 30 Days” course. You’ve earned all the badges, collected all the certificates, and watched all the videos. But something doesn’t feel right. When you look at job descriptions or try to build your own project, there’s a gap between what you’ve learned and what seems to be expected of a programmer.
If this resonates with you, you’re not alone. Thousands of aspiring developers find themselves in this peculiar limbo: technically educated but practically unprepared.
In this article, we’ll explore why many coding courses fall short of creating job-ready programmers, what’s missing from the typical learning journey, and how to bridge the gap between course completion and professional competence.
The False Promise of Rapid Mastery
The internet is brimming with courses promising to transform you into a programmer in remarkably short timeframes: 30 days, 14 days, even 24 hours. These promises are alluring, especially to those eager to transition into tech careers quickly.
But here’s the uncomfortable truth: becoming a programmer is not about racing through syntax lessons or memorizing language features. It’s a journey of developing a particular mindset, one that allows you to solve complex problems methodically.
The Reality Behind “30 Days to Mastery”
These accelerated courses typically focus on teaching you the syntax and basic functionality of a programming language. You’ll learn how variables work, how to write loops, and maybe even some basic object-oriented programming principles.
But knowing the parts of a language is fundamentally different from knowing how to use those parts to solve real-world problems. Consider this analogy: learning vocabulary and grammar rules doesn’t automatically make you a novelist. Similarly, learning Python syntax doesn’t automatically make you a programmer.
The problem isn’t with the content these courses teach; it’s with what they leave out and the expectations they set.
What Most Courses Miss: The Hidden Curriculum of Programming
Beyond syntax and basic concepts, professional programming requires a constellation of skills that many courses simply don’t address. Let’s explore what’s often missing.
1. Problem-Solving Methodology
At its core, programming is problem-solving. Yet many courses focus on teaching solutions rather than the process of arriving at those solutions. They might show you how to implement a specific algorithm but not how to determine which algorithm is appropriate for a given problem.
Professional programmers don’t just write code; they:
- Break complex problems into manageable parts
- Identify patterns and relationships within those parts
- Systematically explore potential solutions
- Evaluate trade-offs between different approaches
These skills come from practice, mentorship, and exposure to diverse problems, not from following prescribed exercises with predetermined solutions.
2. Reading and Understanding Existing Code
In the real world, programmers spend far more time reading code than writing it. Whether you’re debugging, refactoring, or extending existing functionality, the ability to navigate and comprehend code written by others is essential.
Yet most courses focus exclusively on writing new code from scratch. They rarely teach you how to:
- Navigate large codebases
- Understand coding patterns and conventions
- Trace execution paths through complex systems
- Infer the intent behind code decisions
This gap leaves many new programmers feeling overwhelmed when they encounter their first professional codebase.
3. Debugging and Troubleshooting
In controlled course environments, the exercises are often designed to work if you follow the instructions correctly. But in real development, things rarely go as planned. Code breaks, exceptions occur, and systems behave unexpectedly.
Professional debugging involves:
- Systematic hypothesis testing
- Effective use of debugging tools
- Log analysis and interpretation
- Root cause analysis
- Creating minimal reproducible examples
These skills are developed through experience with real bugs in complex systems, something most courses don’t provide.
4. Software Design and Architecture
Writing code that works is one thing; writing code that’s maintainable, extensible, and robust is another entirely. Professional programming requires understanding how to structure code at both small and large scales.
This includes:
- Applying appropriate design patterns
- Creating clean, modular interfaces
- Managing dependencies effectively
- Ensuring separation of concerns
- Planning for future changes and requirements
Most introductory courses understandably focus on getting beginners to write working code, leaving these architectural considerations for “advanced” topics that many learners never reach.
5. Collaboration and Version Control
Professional programming is rarely a solo endeavor. Modern development teams collaborate using version control systems, code reviews, and shared coding standards.
Essential collaboration skills include:
- Effective use of Git beyond basic commands
- Writing clear commit messages
- Managing and resolving merge conflicts
- Participating constructively in code reviews
- Documenting code for other developers
While some courses touch on Git basics, few provide realistic practice with the collaborative workflows used in professional environments.
The Missing Practice: Why Exercises Don’t Equal Experience
Another limitation of many coding courses is the nature of their practice exercises. These typically fall into two categories:
The “Fill in the Blank” Problem
Many course exercises provide most of the solution and ask you to complete small portions:
# Complete the function to return the sum of two numbers
def add_numbers(a, b):
# Your code here
return ___
While these exercises help reinforce specific concepts, they don’t develop the skill of creating solutions from scratch.
The “Follow Along” Project
Other courses guide you through building a project step-by-step, with the instructor providing the exact code to write at each stage. While these projects can be engaging, they often create an illusion of understanding.
When you follow precise instructions to build something, you’re not practicing the critical skills of:
- Deciding what to build next based on requirements
- Researching solutions to unfamiliar problems
- Making design decisions and evaluating alternatives
- Diagnosing and fixing unexpected issues
These guided projects can leave you with a false sense of readiness, only to find yourself stuck when trying to build something independently.
The Knowledge vs. Skill Gap
At this point, we need to address a fundamental distinction: the difference between knowledge and skill.
Knowledge is information you possess; skill is your ability to apply that information effectively in various contexts. Many coding courses successfully impart knowledge but fail to develop skill.
Consider these parallels:
- Reading about swimming techniques vs. actually swimming
- Studying chess strategies vs. playing competitive matches
- Learning musical theory vs. performing a piece
In each case, the knowledge component is necessary but insufficient. Skill comes from application, practice, and feedback in realistic conditions.
Programming is no different. Understanding how a for-loop works is knowledge; knowing when to use it, how to structure it for readability, and how to debug it when it produces unexpected results is skill.
The Algorithmic Thinking Gap
Perhaps the most significant gap in many coding courses is their failure to develop algorithmic thinking, the foundation of computational problem-solving.
Algorithmic thinking involves:
- Breaking problems down into precise, unambiguous steps
- Identifying patterns and relationships within data
- Understanding time and space complexity
- Recognizing when and how to apply common algorithmic approaches
This type of thinking doesn’t come naturally to most people; it’s developed through practice with diverse problems and feedback on your approach.
Many courses introduce basic algorithms like sorting or searching but don’t provide enough varied practice to develop this thinking style. As a result, when faced with novel problems, many learners find themselves unable to formulate an approach.
The Data Structures Foundation
Closely related to algorithmic thinking is a deep understanding of data structures. Professional programmers know that choosing the right data structure is often the key to efficient solutions.
While most courses cover basic data structures like lists, dictionaries, and perhaps stacks and queues, they rarely provide enough practice in:
- Selecting appropriate data structures for specific problems
- Understanding the performance characteristics of different structures
- Implementing and customizing data structures for particular needs
- Combining multiple data structures to solve complex problems
This knowledge gap becomes apparent when tackling real-world programming challenges that don’t fit neatly into the examples covered in courses.
The Technical Interview Reality Check
For many aspiring programmers, the first stark revelation of these gaps comes during technical interviews. After completing multiple courses, they feel confident in their programming abilities, only to freeze when asked to solve seemingly simple problems on a whiteboard or in a live coding environment.
This disconnect occurs because technical interviews typically assess:
- Your problem-solving process, not just your knowledge of syntax
- Your ability to think algorithmically under pressure
- Your communication of technical concepts and approaches
- Your facility with data structures and algorithm selection
These are precisely the areas that many courses underemphasize, creating a painful reality check for job seekers.
Consider this common interview question:
# Given a string, find the first non-repeating character and return its index.
# If it doesn't exist, return -1.
# Example: s = "leetcode" should return 0
# Example: s = "loveleetcode" should return 2
This problem isn’t particularly complex, but it requires:
- Selecting appropriate data structures to track character occurrences
- Designing an efficient algorithm that doesn’t require multiple full passes through the string
- Handling edge cases correctly
- Analyzing the time and space complexity of your solution
Many course graduates struggle with such questions, not because they don’t know Python syntax, but because they haven’t developed the problem-solving approach that professional programming requires.
Bridging the Gap: From Course Graduate to Programmer
If you’ve recognized these gaps in your own learning journey, don’t despair. Awareness is the first step toward improvement, and there are concrete strategies to bridge the gap between course completion and professional competence.
1. Focus on Problem-Solving, Not Just Solutions
Start approaching programming as a problem-solving discipline:
- Before writing code, thoroughly understand the problem
- Practice breaking problems down on paper or a whiteboard
- Consider multiple approaches before implementing any one solution
- After solving a problem, reflect on your process and consider alternative approaches
Resources like LeetCode, HackerRank, and AlgoCademy provide structured problem-solving practice with increasing levels of difficulty.
2. Build Projects Without Tutorials
The leap from following tutorials to independent development is significant but essential:
- Start with a simple project idea that interests you
- Define clear requirements before writing code
- Research as needed, but avoid copying complete solutions
- Embrace the struggle of figuring things out independently
- When stuck, seek principles and approaches rather than exact code to copy
Your first independent projects will take longer and contain more mistakes than tutorial projects, but they’ll develop the self-sufficiency that professional programming requires.
3. Study Existing Codebases
To develop code-reading skills:
- Explore open-source projects in areas that interest you
- Start by understanding the overall structure and architecture
- Trace the execution flow of specific features
- Try to fix simple bugs or add minor features
- Read documentation and commit histories to understand the reasoning behind decisions
This practice will expose you to professional coding standards and patterns that courses often don’t cover.
4. Embrace Debugging as a Skill
Rather than seeing bugs as obstacles, view them as opportunities to develop crucial skills:
- Use debugging tools like pdb in Python rather than relying solely on print statements
- Practice creating minimal reproducible examples
- Document your debugging process to identify patterns in your approach
- Deliberately introduce bugs in working code and practice finding them
The ability to systematically debug is often what separates professional programmers from hobbyists.
5. Learn Computer Science Fundamentals
While not all programming requires deep CS knowledge, understanding fundamentals will strengthen your problem-solving abilities:
- Study common data structures beyond those covered in basic courses
- Learn algorithm design paradigms like divide-and-conquer, dynamic programming, and greedy algorithms
- Understand complexity analysis (Big O notation)
- Explore basic computer architecture to understand how your code actually runs
Resources like “Grokking Algorithms” by Aditya Bhargava or MIT’s OpenCourseWare can provide accessible introductions to these topics.
6. Practice Collaborative Development
To develop collaboration skills:
- Contribute to open-source projects, starting with documentation or simple bug fixes
- Participate in code reviews, both giving and receiving feedback
- Practice Git workflows that simulate team environments
- Join coding communities or find study partners for collaborative projects
These experiences will prepare you for the team-based nature of professional development.
The Role of Structured Learning in Becoming a Programmer
While this article has highlighted the limitations of many coding courses, the solution isn’t to abandon structured learning entirely. Rather, it’s to approach courses as one component of a broader learning strategy.
Courses as Foundation, Not Destination
Well-designed courses provide valuable foundations:
- They introduce concepts in a logical, progressive order
- They provide immediate feedback on basic exercises
- They offer explanations tailored to beginners
- They create a structured path through fundamental concepts
The key is to view course completion as the beginning of your learning journey, not its culmination.
Complementing Courses with Deliberate Practice
Effective learning combines structured instruction with deliberate practice:
- Use courses to learn concepts and syntax
- Apply those concepts to solve independent problems
- Seek feedback on your solutions from more experienced programmers
- Reflect on your approaches and identify areas for improvement
This combination accelerates the development of both knowledge and skill.
The Mindset Shift: From Language Learner to Problem Solver
Perhaps the most important transition in becoming a programmer is the shift in how you see yourself: from someone learning a programming language to someone solving problems with code.
This mindset shift manifests in several ways:
From “How do I do X in Python?” to “What’s the best way to solve this problem?”
Beginners often frame questions in terms of syntax: “How do I sort a list in Python?” or “How do I read a file in Python?”
As you evolve into a programmer, your questions become more problem-centered: “What’s the most efficient way to find patterns in this data?” or “How should I structure this application to make it maintainable?”
From “I need to learn more” to “I need to build more”
Early in the learning journey, it’s common to feel that you need to complete just one more course or tutorial before you’re ready to build real projects.
Professional programmers recognize that building is learning. They expect to encounter unknowns in every project and view research and problem-solving as part of the development process, not prerequisites to it.
From “I don’t know enough” to “I can figure this out”
Perhaps the most empowering shift is from knowledge-dependency to problem-solving confidence. Professional programmers don’t know everything, but they trust their ability to find solutions through research, experimentation, and systematic thinking.
This confidence comes from experience with the problem-solving process, not from comprehensive knowledge of language features or libraries.
The Continuous Learning Reality of Programming
A final truth that many courses fail to emphasize is that programming is a field of continuous learning. The tools, languages, and best practices evolve constantly, and even experienced programmers regularly encounter unfamiliar problems.
Professional programmers aren’t distinguished by having completed their learning journey, but by having mastered the process of learning itself:
- They know how to read documentation effectively
- They can evaluate new tools and technologies critically
- They recognize patterns across different languages and paradigms
- They build mental models that facilitate learning related concepts
This meta-skill of “learning how to learn” is perhaps the most valuable outcome of the journey from course-taker to programmer.
Conclusion: The Path Forward
If you’ve completed a “Learn Python in 30 Days” course but don’t yet feel like a programmer, take heart. You haven’t failed, and the course hasn’t necessarily failed you; you’re simply experiencing the natural gap between initial learning and professional competence.
The path forward involves:
- Recognizing that programming is primarily problem-solving, not syntax memorization
- Developing algorithmic thinking through diverse practice problems
- Building independent projects that force you to research and make decisions
- Studying existing code to learn professional patterns and practices
- Embracing debugging and troubleshooting as core skills
- Participating in collaborative development to prepare for team environments
This journey takes time and involves periods of frustration and uncertainty. But with deliberate practice and the right mindset, you can bridge the gap between course completion and programming competence.
Remember that every professional programmer has walked this path. The difference between those who succeed and those who don’t isn’t innate talent or the quality of their first courses; it’s their willingness to embrace the challenges of independent problem-solving and persist through the uncomfortable growth phase that follows initial learning.
So if your “30 Days to Python” course hasn’t made you a programmer yet, don’t give up. Instead, reframe your expectations, adjust your learning approach, and continue the journey with a clearer understanding of what lies ahead. The path may be longer than advertised, but the destination is well worth the effort.