Why Your Interview Solutions Lack Proper Structure (And How to Fix It)
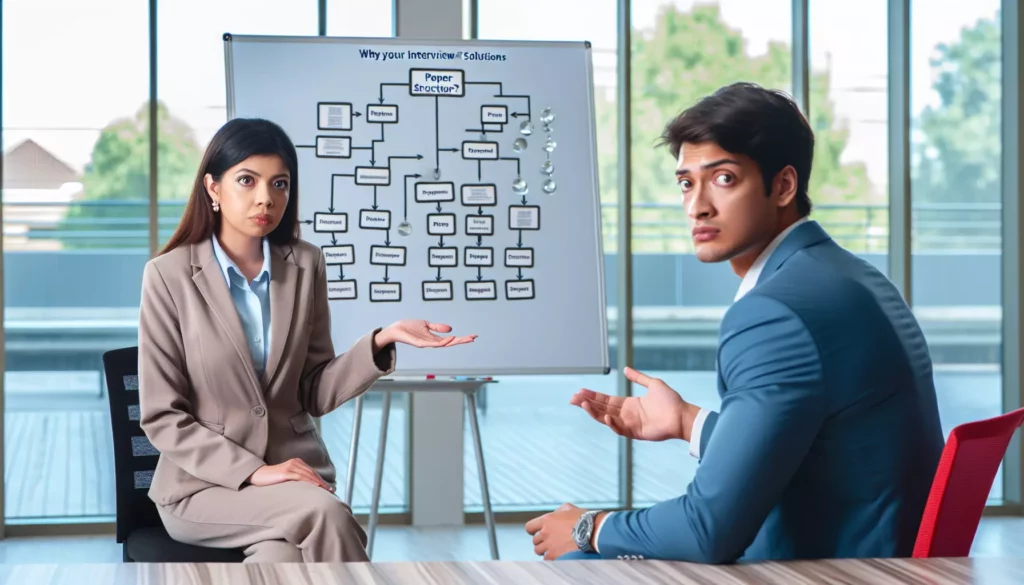
Technical interviews can be intimidating, especially when you’re competing for positions at prestigious tech companies. While many candidates focus on memorizing algorithms and data structures, they often overlook a crucial aspect: the structure of their solutions. A well structured solution not only demonstrates your technical abilities but also showcases your problem solving methodology and communication skills.
In this comprehensive guide, we’ll explore why proper structure matters in technical interviews, identify common structural pitfalls, and provide actionable strategies to transform your coding solutions from chaotic to coherent.
Table of Contents
- Why Structure Matters in Technical Interviews
- Common Structural Pitfalls in Interview Solutions
- The Ideal Structure for Technical Interview Solutions
- Communication Techniques That Enhance Solution Structure
- Language Specific Structuring Best Practices
- Practical Examples: Before and After Restructuring
- Understanding the Interviewer’s Perspective
- Effective Practice Methods to Improve Solution Structure
- Advanced Structuring Techniques for Complex Problems
- Conclusion: Elevating Your Technical Interview Performance
Why Structure Matters in Technical Interviews
When interviewers evaluate candidates, they’re looking beyond whether the solution works. They’re assessing how you think, how you communicate, and how you organize complex ideas. Here’s why structure is a critical component of successful technical interviews:
Demonstrates Clear Thinking
A structured solution reflects structured thinking. When you present a well organized solution, you demonstrate that you can break down complex problems into manageable components, a skill that’s invaluable in real world software development.
Enhances Readability and Maintainability
Code that’s easy to read is easy to maintain. By structuring your solution clearly, you show that you can write code that others can understand and build upon, a crucial skill in collaborative environments.
Facilitates Debugging and Testing
Well structured code makes it easier to identify and fix bugs. It also simplifies the process of writing tests, which is increasingly important in modern software development practices.
Showcases Professional Standards
Professional developers adhere to coding standards and best practices. By presenting a structured solution, you signal that you’re familiar with industry standards and ready to contribute to production code.
Improves Communication with the Interviewer
A clear structure helps the interviewer follow your thought process. This makes it easier for them to provide guidance if you get stuck and to evaluate your approach accurately.
Common Structural Pitfalls in Interview Solutions
Before we discuss how to structure your solutions effectively, let’s identify the common pitfalls that can undermine your performance:
Diving Straight into Coding
Many candidates start coding immediately without planning their approach. This often leads to backtracking, inefficient solutions, and confusion for both the candidate and the interviewer.
For example, consider a problem asking you to find the kth largest element in an array. Diving straight into a sorting solution without considering heap based approaches might lead to a less optimal solution.
Lack of Modularization
Writing monolithic functions that handle multiple responsibilities makes your code difficult to understand and test. This approach also makes it harder to reuse components of your solution.
Consider this example of a poorly modularized solution for validating a binary search tree:
function isValidBST(root) {
// A single function that tries to do everything
if (!root) return true;
// Complex logic all in one place
if ((root.left && root.left.val >= root.val) ||
(root.right && root.right.val <= root.val))
return false;
// Recursive calls without clear separation of concerns
return isValidBST(root.left) && isValidBST(root.right);
}
Inconsistent Naming Conventions
Using unclear or inconsistent variable and function names makes your code harder to follow. This is particularly problematic in interviews where you need to clearly communicate your thought process.
Absence of Comments or Documentation
Without appropriate comments, interviewers may struggle to understand your intentions, especially for complex algorithms or edge cases.
Neglecting Edge Cases
Failing to structure your solution to handle edge cases (empty inputs, boundary conditions, etc.) suggests incomplete thinking and can lead to incorrect solutions.
Disorganized Approach to Problem Solving
Jumping between different approaches or mixing implementation details with high level design creates confusion and indicates a lack of methodical thinking.
The Ideal Structure for Technical Interview Solutions
Now that we understand the importance of structure and common pitfalls, let’s outline an ideal approach to structuring your technical interview solutions:
1. Understand the Problem
Begin by ensuring you fully understand the problem statement. This includes:
- Clarifying the inputs and expected outputs
- Asking questions about constraints and edge cases
- Discussing assumptions with the interviewer
For example, if asked to implement a function to reverse a linked list, you might ask:
- Should I reverse it in place or create a new list?
- What should happen if the list is empty or has only one node?
- Are there any time or space complexity requirements?
2. Plan Your Approach
Before writing any code, outline your solution strategy:
- Discuss multiple approaches if applicable
- Analyze time and space complexity
- Select the most appropriate approach based on the constraints
- Sketch the high level algorithm steps
For instance, when solving a problem like finding all pairs in an array that sum to a target value, you might outline:
- Approach 1: Nested loops (O(n²) time, O(1) space)
- Approach 2: Hash map approach (O(n) time, O(n) space)
- Decision: Use the hash map approach for better time complexity
3. Structure Your Code
Organize your code implementation with these principles:
- Use descriptive variable and function names
- Break down complex logic into helper functions
- Follow consistent indentation and formatting
- Group related functionality together
Here’s an example of a well structured solution for finding the maximum depth of a binary tree:
/**
* Finds the maximum depth of a binary tree
* @param {TreeNode} root - The root node of the binary tree
* @return {number} - The maximum depth
*/
function maxDepth(root) {
// Base case: empty tree has depth 0
if (root === null) return 0;
// Recursive case: compute depth of subtrees
const leftDepth = maxDepth(root.left);
const rightDepth = maxDepth(root.right);
// Return the larger depth plus 1 for the current node
return Math.max(leftDepth, rightDepth) + 1;
}
4. Handle Edge Cases Explicitly
Always include explicit handling of edge cases:
- Empty or null inputs
- Minimum and maximum values
- Single element inputs
- Invalid inputs (if applicable)
5. Test Your Solution
Demonstrate thoroughness by testing your solution:
- Walk through with a simple example
- Test edge cases
- Verify the solution against the expected output
- Discuss potential optimizations
6. Analyze Time and Space Complexity
Conclude by analyzing the efficiency of your solution:
- Provide a clear time complexity analysis
- Explain the space complexity
- Discuss any tradeoffs made
Communication Techniques That Enhance Solution Structure
Effective communication is integral to presenting a well structured solution. Here are techniques to enhance your communication during technical interviews:
Think Aloud Strategically
Sharing your thought process helps interviewers follow your reasoning, but do so strategically:
- Articulate high level approaches before diving into details
- Explain why you’re choosing certain data structures or algorithms
- Verbalize your reasoning when making tradeoffs
For example: “I’m considering using a hash map here because we need O(1) lookups, which will help us achieve a linear time solution overall.”
Use Visual Aids
For complex problems, visual representations can clarify your thinking:
- Draw diagrams to illustrate data structures
- Use tables to track algorithm progress
- Sketch execution flow for recursive solutions
Establish Clear Transitions
Signal when you’re moving between different phases of your solution:
- “Now that I understand the problem, let me outline my approach…”
- “Before implementing, let me analyze the time complexity…”
- “Let me start by handling the edge cases…”
Seek Validation at Key Points
Check in with the interviewer to ensure you’re on the right track:
- “Does this approach make sense so far?”
- “Are there any constraints I should be considering?”
- “Is this the level of detail you’re looking for?”
Acknowledge Limitations
Demonstrate self awareness by recognizing the limitations of your solution:
- “This approach works well for small inputs but might be inefficient for very large datasets.”
- “An alternative approach would be X, which would improve Y at the cost of Z.”
Language Specific Structuring Best Practices
Different programming languages have their own conventions and best practices for code structure. Here are guidelines for some commonly used languages in technical interviews:
Python
Python emphasizes readability and simplicity:
- Use 4 spaces for indentation (not tabs)
- Follow PEP 8 style guidelines
- Use docstrings for function documentation
- Leverage list comprehensions for concise operations
- Use meaningful variable names in snake_case
def two_sum(nums, target):
"""
Find indices of two numbers that add up to target.
Args:
nums: List of integers
target: Integer target sum
Returns:
Tuple of two indices
"""
num_to_index = {} # value -> index mapping
for i, num in enumerate(nums):
complement = target - num
if complement in num_to_index:
return (num_to_index[complement], i)
num_to_index[num] = i
return None # No solution found
Java
Java code typically follows more formal structuring:
- Use camelCase for variables and methods
- Use PascalCase for class names
- Include access modifiers (public, private, etc.)
- Structure code with clear class hierarchies
- Use Javadoc comments for documentation
/**
* Solution for the Two Sum problem.
*/
public class TwoSum {
/**
* Finds indices of two numbers that add up to target.
*
* @param nums Array of integers
* @param target Target sum
* @return Array containing the two indices
*/
public int[] twoSum(int[] nums, int target) {
// Using a map to store value -> index mapping
Map<Integer, Integer> numToIndex = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
// Check if complement exists in map
if (numToIndex.containsKey(complement)) {
return new int[] {numToIndex.get(complement), i};
}
// Store current number and its index
numToIndex.put(nums[i], i);
}
// No solution found
return new int[] {-1, -1};
}
}
JavaScript
JavaScript offers flexibility but benefits from consistent structuring:
- Use camelCase for variables and functions
- Use PascalCase for classes and constructors
- Apply consistent semicolon usage
- Leverage ES6+ features (arrow functions, destructuring)
- Use JSDoc for documentation
/**
* Finds indices of two numbers that add up to target.
* @param {number[]} nums - Array of integers
* @param {number} target - Target sum
* @return {number[]} - Array containing the two indices
*/
function twoSum(nums, target) {
// Using a map to store value -> index mapping
const numToIndex = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
// Check if complement exists in map
if (numToIndex.has(complement)) {
return [numToIndex.get(complement), i];
}
// Store current number and its index
numToIndex.set(nums[i], i);
}
// No solution found
return null;
}
Practical Examples: Before and After Restructuring
Let’s examine some practical examples of poorly structured solutions and how they can be improved:
Example 1: Finding Palindromes
Before (Poorly Structured):
function isPalindrome(s) {
s = s.toLowerCase().replace(/[^a-z0-9]/g, '');
for (let i = 0; i < s.length / 2; i++)
if (s[i] !== s[s.length - 1 - i]) return false;
return true;
}
After (Well Structured):
/**
* Determines if a string is a palindrome, considering only alphanumeric characters
* and ignoring case.
*
* @param {string} s - The input string
* @return {boolean} - True if the string is a palindrome, false otherwise
*/
function isPalindrome(s) {
// Edge case: empty string is considered a palindrome
if (s.length === 0) return true;
// Preprocess: convert to lowercase and remove non-alphanumeric characters
const cleanString = preprocessString(s);
// Check if the string reads the same forwards and backwards
return checkPalindrome(cleanString);
}
/**
* Converts string to lowercase and removes non-alphanumeric characters
*/
function preprocessString(s) {
return s.toLowerCase().replace(/[^a-z0-9]/g, '');
}
/**
* Checks if a clean string is a palindrome using two-pointer technique
*/
function checkPalindrome(s) {
let left = 0;
let right = s.length - 1;
while (left < right) {
if (s[left] !== s[right]) {
return false;
}
left++;
right--;
}
return true;
}
Example 2: Merge Sort Implementation
Before (Poorly Structured):
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid));
const right = mergeSort(arr.slice(mid));
let result = [], i = 0, j = 0;
while (i < left.length && j < right.length) {
if (left[i] < right[j]) result.push(left[i++]);
else result.push(right[j++]);
}
return result.concat(left.slice(i)).concat(right.slice(j));
}
After (Well Structured):
/**
* Sorts an array using the merge sort algorithm.
*
* @param {number[]} arr - The array to sort
* @return {number[]} - The sorted array
* Time Complexity: O(n log n)
* Space Complexity: O(n)
*/
function mergeSort(arr) {
// Base case: arrays of length 0 or 1 are already sorted
if (arr.length <= 1) {
return arr;
}
// Divide the array into two halves
const mid = Math.floor(arr.length / 2);
const leftHalf = arr.slice(0, mid);
const rightHalf = arr.slice(mid);
// Recursively sort both halves
const sortedLeft = mergeSort(leftHalf);
const sortedRight = mergeSort(rightHalf);
// Merge the sorted halves
return merge(sortedLeft, sortedRight);
}
/**
* Merges two sorted arrays into a single sorted array.
*
* @param {number[]} left - First sorted array
* @param {number[]} right - Second sorted array
* @return {number[]} - Merged sorted array
*/
function merge(left, right) {
const result = [];
let leftIndex = 0;
let rightIndex = 0;
// Compare elements from both arrays and add the smaller one to the result
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
// Add any remaining elements
return result
.concat(left.slice(leftIndex))
.concat(right.slice(rightIndex));
}
Understanding the Interviewer’s Perspective
To structure your solutions effectively, it helps to understand what interviewers are looking for:
Problem Solving Process
Interviewers value how you approach problems more than whether you get the perfect answer immediately:
- They want to see systematic thinking
- They evaluate how you break down complex problems
- They assess your ability to consider different approaches
Code Quality Signals
Your code structure sends important signals about your professional capabilities:
- Clean, well organized code suggests attention to detail
- Modular design indicates software engineering maturity
- Consistent naming shows commitment to readability
- Explicit edge case handling demonstrates thoroughness
Collaboration Potential
How you structure and communicate your solution indicates how you might work with others:
- Clear explanations suggest you can communicate technical concepts effectively
- Receptiveness to feedback shows you can work collaboratively
- Structured thinking indicates you can contribute to system design discussions
Effective Practice Methods to Improve Solution Structure
Improving your solution structure requires deliberate practice. Here are effective methods to enhance your structuring skills:
Code Review Practice
Regularly review and refactor your own code:
- Solve a problem, then put it aside for a day
- Return to your solution and evaluate its structure
- Identify areas for improvement and refactor
- Compare your solution with well structured examples
Template Development
Create personal templates for common problem types:
- Develop a standard approach for array problems
- Create templates for tree traversals
- Establish patterns for dynamic programming solutions
For example, a template for binary search might look like:
/**
* Binary search template
*/
function binarySearch(nums, target) {
// Initialize search boundaries
let left = 0;
let right = nums.length - 1;
// Continue searching while boundaries are valid
while (left <= right) {
// Calculate middle index
const mid = Math.floor((left + right) / 2);
// Found target
if (nums[mid] === target) {
return mid;
}
// Adjust boundaries based on comparison
if (nums[mid] < target) {
left = mid + 1; // Target in right half
} else {
right = mid - 1; // Target in left half
}
}
// Target not found
return -1;
}
Mock Interviews with Structure Focus
Practice with peers or online platforms, specifically focusing on structure:
- Ask your mock interviewer to evaluate your solution structure
- Record your mock interviews to review your communication
- Set specific goals for structural improvements in each session
Study Well Structured Code
Analyze high quality code from open source projects or solution repositories:
- Identify structuring patterns used by experienced developers
- Note how complex functionality is broken down
- Observe documentation and commenting practices
Verbalization Practice
Practice explaining your code structure out loud:
- Explain your solution as if teaching someone else
- Practice articulating your structural choices
- Record yourself and identify areas where your explanation lacks clarity
Advanced Structuring Techniques for Complex Problems
For particularly complex interview problems, consider these advanced structuring techniques:
State Management Abstraction
For problems with complex state tracking:
- Encapsulate state variables in classes or objects
- Create methods that update state according to specific rules
- Separate state manipulation from algorithm logic
Example of state management in a solution for the “Game of Life” problem:
class GameOfLife {
constructor(board) {
this.board = board;
this.rows = board.length;
this.cols = board[0].length;
}
/**
* Advances the game by one generation
*/
nextGeneration() {
const nextState = this.computeNextState();
this.updateBoard(nextState);
}
/**
* Computes the next state without modifying the current board
*/
computeNextState() {
const nextState = Array(this.rows).fill()
.map(() => Array(this.cols).fill(0));
for (let r = 0; r < this.rows; r++) {
for (let c = 0; c < this.cols; c++) {
const liveNeighbors = this.countLiveNeighbors(r, c);
nextState[r][c] = this.applyCellRules(this.board[r][c], liveNeighbors);
}
}
return nextState;
}
/**
* Counts live neighbors for a cell
*/
countLiveNeighbors(row, col) {
// Implementation details
}
/**
* Applies game rules to determine next cell state
*/
applyCellRules(currentState, liveNeighbors) {
// Implementation details
}
/**
* Updates the board with the new state
*/
updateBoard(nextState) {
for (let r = 0; r < this.rows; r++) {
for (let c = 0; c < this.cols; c++) {
this.board[r][c] = nextState[r][c];
}
}
}
}
Algorithm Decomposition
For algorithms with multiple phases:
- Divide the algorithm into distinct phases
- Implement each phase as a separate function
- Create clear interfaces between phases
Example of algorithm decomposition for a solution to find all permutations:
/**
* Generates all permutations of a given array
*/
function permute(nums) {
const result = [];
// Phase 1: Initialize the backtracking process
backtrack(nums, [], new Set(), result);
return result;
}
/**
* Phase 2: Backtracking to build permutations
*/
function backtrack(nums, currentPermutation, used, result) {
// Base case: permutation is complete
if (currentPermutation.length === nums.length) {
// Phase 3: Store the result
savePermutation(currentPermutation, result);
return;
}
// Try each number that hasn't been used yet
for (let i = 0; i < nums.length; i++) {
if (used.has(i)) continue;
// Phase 4: Make a choice
used.add(i);
currentPermutation.push(nums[i]);
// Recurse
backtrack(nums, currentPermutation, used, result);
// Phase 5: Undo the choice
currentPermutation.pop();
used.delete(i);
}
}
/**
* Phase 3: Save a completed permutation
*/
function savePermutation(permutation, result) {
// Create a deep copy to avoid reference issues
result.push([...permutation]);
}
Design Pattern Integration
Applying appropriate design patterns can elevate your solution structure:
- Strategy pattern for different algorithm approaches
- Factory pattern for creating different types of objects
- Observer pattern for event based problems
- Iterator pattern for custom traversal logic
While full pattern implementations might be excessive for interviews, showing awareness of these patterns demonstrates advanced software design knowledge.
Conclusion: Elevating Your Technical Interview Performance
Proper solution structure is not merely a cosmetic concern but a fundamental aspect of demonstrating your capabilities as a software engineer. By implementing the strategies outlined in this guide, you can transform your interview solutions from disorganized attempts to professional quality code that showcases your technical abilities and problem solving methodology.
Remember these key takeaways:
- Structure reflects thinking; clear code structure demonstrates clear thinking
- Planning before coding leads to more coherent solutions
- Modularization improves readability and maintainability
- Consistent naming and formatting enhances communication
- Explicit edge case handling shows thoroughness
- Language specific best practices signal professionalism
As you prepare for technical interviews, dedicate time specifically to improving your solution structure. This investment will not only enhance your interview performance but also develop skills that translate directly to real world software development.
The difference between a candidate who merely solves problems and one who presents well structured solutions can be the deciding factor in competitive technical interviews. By mastering proper solution structure, you position yourself as a candidate who not only knows algorithms but also understands how to write professional quality code that others can read, maintain, and build upon.
Start implementing these structuring techniques in your practice today, and watch as your technical interview performance reaches new heights.