Why Your Interview Preparation Isn’t Matching Real Scenarios
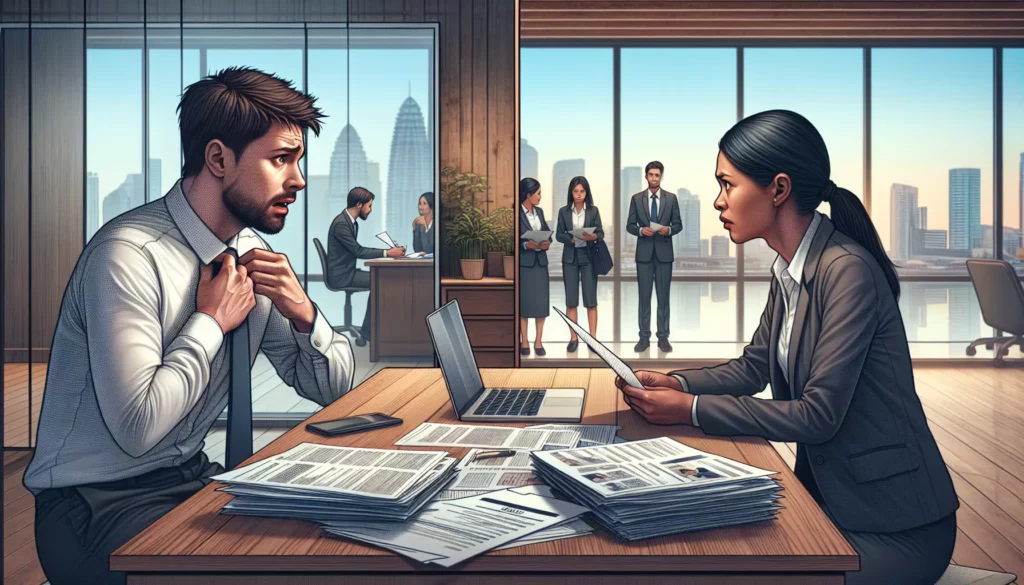
Have you ever walked out of a technical interview feeling blindsided? Despite hours of preparation, the questions you faced seemed completely different from what you studied. This disconnect between interview preparation and the actual interview experience is more common than you might think.
In this comprehensive guide, we’ll explore why traditional interview preparation methods often fall short, what real technical interviews actually look like, and how you can bridge this gap to ace your next coding interview.
The Reality Gap in Technical Interview Preparation
Many aspiring developers find themselves in a frustrating cycle: they spend weeks or months preparing for technical interviews, only to face questions and scenarios that seem to come out of nowhere when they’re in the hot seat.
Let’s examine why this happens and what we can do about it.
Common Preparation Pitfalls
1. Memorizing Solutions Instead of Understanding Concepts
One of the most prevalent mistakes in interview preparation is focusing on memorizing solutions to specific problems instead of understanding the underlying concepts and patterns.
Consider this common scenario: A candidate memorizes the solution to a classic binary search tree problem. During the interview, they’re asked a variation that requires the same underlying knowledge but with a slight twist. Without a deep understanding of the concept, they struggle to adapt their memorized solution.
Real interviews test your ability to think on your feet and apply concepts to new problems, not regurgitate memorized code.
2. Focusing Only on Algorithm Complexity
While understanding time and space complexity is crucial, many candidates obsess over optimizing algorithms to O(n) or O(log n) without considering other important aspects of code quality that interviewers evaluate:
- Readability and maintainability
- Edge case handling
- Testing strategies
- Code organization
In real interviews, writing clean, well-structured code that handles edge cases properly often matters more than shaving off a few milliseconds of theoretical runtime.
3. Studying in Isolation
Many candidates prepare by solving problems alone, without the pressure and interaction that characterizes actual interviews. This fails to prepare them for:
- Explaining their thought process aloud
- Receiving and incorporating feedback in real time
- Managing the social and psychological aspects of interviews
- Handling unexpected questions or hints
Technical interviews are as much about communication as they are about coding.
What Real Technical Interviews Actually Look Like
To prepare effectively, you need to understand what you’re actually preparing for. Let’s examine what technical interviews at top companies truly entail.
The Collaborative Nature of Modern Interviews
Contrary to popular belief, most technical interviews aren’t designed to be adversarial “gotcha” sessions. Instead, they’re increasingly structured as collaborative problem-solving exercises where the interviewer wants to see:
- How you think through problems
- How you respond to hints and guidance
- How you collaborate with others
- How you communicate technical concepts
Mark, a senior engineer at Google, shares: “I’m not looking for candidates who immediately know the perfect solution. I’m looking for candidates who can work with me to develop a solution, who ask clarifying questions, and who can explain their thinking clearly.”
Real World Problems vs. Textbook Algorithms
While many preparation resources focus on classic algorithms and data structures problems, real interviews often include:
System Design Questions
These open-ended questions ask you to design a system (like a URL shortener or a social media feed) and discuss tradeoffs, scalability concerns, and implementation details.
Example: “Design a system that can handle millions of users uploading and sharing photos.”
Behavioral Scenarios with Technical Components
Questions that assess both your technical knowledge and your approach to workplace situations.
Example: “Tell me about a time when you had to optimize underperforming code. What approach did you take and what were the results?”
Practical Coding Tasks
Problems that mirror real work more closely than pure algorithm challenges.
Example: “Write a function to parse this log file format and extract specific metrics.”
The Emphasis on Testing and Edge Cases
In real interviews, candidates are often evaluated more on their thoroughness than their speed:
- Do they consider edge cases?
- Do they test their code?
- Do they validate inputs?
- Do they handle error conditions gracefully?
Sarah, a hiring manager at a fintech company, notes: “I’ve seen brilliant candidates fail because they rushed to implement a complex algorithm but didn’t handle basic edge cases like empty inputs or boundary conditions. In real work, those details matter enormously.”
Bridging the Gap: How to Prepare More Effectively
Now that we understand the disconnect, let’s explore strategies to align your preparation with actual interview experiences.
Focus on Patterns, Not Problems
Instead of trying to memorize solutions to hundreds of individual problems, focus on recognizing and mastering common patterns in algorithm and data structure questions:
- Sliding window techniques
- Two-pointer approaches
- Breadth-first and depth-first search patterns
- Dynamic programming frameworks
- Divide and conquer strategies
By understanding these patterns, you can adapt to variations more easily during interviews.
Consider this example pattern: the “sliding window” technique for array or string problems.
function slidingWindowExample(array, k) {
let windowStart = 0;
let result = 0;
let currentSum = 0;
for (let windowEnd = 0; windowEnd < array.length; windowEnd++) {
// Add the next element to the window
currentSum += array[windowEnd];
// If we've reached the window size k
if (windowEnd >= k - 1) {
// Process the window (in this case, tracking maximum sum)
result = Math.max(result, currentSum);
// Remove the element going out of the window
currentSum -= array[windowStart];
windowStart++;
}
}
return result;
}
Understanding this pattern allows you to solve dozens of related problems by adapting the core approach rather than memorizing individual solutions.
Practice Collaborative Problem Solving
To simulate the interactive nature of real interviews:
1. Find a Practice Partner
Regular mock interviews with a peer or mentor provide invaluable experience in verbalizing your thought process and receiving feedback.
2. Use Mock Interview Platforms
Services like Pramp, interviewing.io, or AlgoCademy’s mock interview features pair you with other candidates or professionals for realistic interview practice.
3. Record Yourself
If you can’t find a partner, record yourself solving problems while speaking aloud. Review the recordings to identify areas where your explanation is unclear or your process could be improved.
Develop a Structured Approach to Problems
Interviewers value a methodical approach to problem-solving. Practice following these steps for every problem:
- Clarify the problem: Ask questions to ensure you understand requirements, constraints, and expected outputs.
- Work through examples: Use test cases to understand the problem better and validate your approach.
- Outline your approach: Before coding, explain your high-level strategy.
- Consider alternatives: Discuss tradeoffs between different approaches.
- Implement carefully: Write clean, well-structured code.
- Test thoroughly: Walk through your code with test cases, including edge cases.
- Analyze complexity: Discuss time and space complexity.
- Consider optimizations: If time permits, discuss how you might improve your solution.
This structured approach demonstrates your problem-solving methodology, which is often more important than getting to the optimal solution immediately.
Prepare for the Full Spectrum of Questions
Expand your preparation beyond standard algorithm challenges:
System Design Practice
For mid to senior-level positions, system design questions are increasingly common. Practice designing scalable systems by:
- Studying existing architectures of popular applications
- Reading engineering blogs from major tech companies
- Working through system design case studies
- Understanding distributed systems concepts
A basic system design discussion might look like this:
// System Design: URL Shortener
// Requirements
- Convert long URLs to short URLs
- Redirect users from short URLs to original long URLs
- High availability and low latency
- Analytics on URL usage
// Component Design
1. API Gateway - handles incoming requests
2. Application servers - process URL creation and redirection
3. Database - stores URL mappings
4. Cache layer - for frequently accessed URLs
5. Analytics service - tracks usage statistics
// URL Generation Approaches
- Hash function approach (MD5/SHA256 + base62 encoding)
- Counter-based approach with base62 encoding
- Random string generation with collision checking
// Database Schema
Table: urls
- id: bigint (primary key)
- short_key: varchar(10) (indexed)
- original_url: text
- created_at: timestamp
- user_id: bigint (optional, if user accounts exist)
- expiry_date: timestamp (optional)
// Scaling Considerations
- Horizontal scaling of application servers
- Database partitioning by short_key
- Read replicas for the database
- CDN integration for global performance
Behavioral Preparation
Prepare stories from your experience that demonstrate:
- Technical problem-solving
- Collaboration and teamwork
- Handling challenging situations
- Learning and growth
Use the STAR method (Situation, Task, Action, Result) to structure your responses.
Language and Framework Specific Questions
If you’re interviewing for a role that requires specific technologies, be prepared to answer detailed questions about those tools:
- JavaScript: closures, promises, event loop
- React: component lifecycle, hooks, state management
- Python: generators, decorators, context managers
- Java: memory management, concurrency, collections framework
Common Real-World Interview Scenarios You Should Prepare For
Let’s examine some interview scenarios that candidates often encounter but don’t adequately prepare for.
The Ambiguous Problem Statement
In real interviews, problem statements are often intentionally ambiguous to see if you ask clarifying questions.
Example: “Design a function to find duplicate files in a file system.”
This seemingly straightforward problem has many ambiguities:
- What constitutes a “duplicate”? Identical content? Name? Size?
- Should the search be recursive through subdirectories?
- How should we handle very large files?
- What about file permissions or inaccessible directories?
Candidates who dive straight into coding without clarifying these points often create solutions that don’t address the actual requirements.
The Iterative Improvement Scenario
Interviewers often want to see how you improve a solution iteratively rather than expecting perfection immediately.
Example: “Write a function to find the kth largest element in an unsorted array.”
A strong candidate might progress through:
- A simple sort-based approach (O(n log n))
- A heap-based solution (O(n log k))
- A quickselect algorithm (average O(n))
Each step demonstrates deeper understanding and consideration of tradeoffs.
// First approach: Sorting (O(n log n))
function findKthLargest(nums, k) {
nums.sort((a, b) => b - a); // Sort in descending order
return nums[k-1];
}
// Second approach: Min Heap (O(n log k))
function findKthLargestWithHeap(nums, k) {
// Using a min heap of size k
const minHeap = [];
for (const num of nums) {
if (minHeap.length < k) {
// If heap not full, add element
minHeap.push(num);
bubbleUp(minHeap, minHeap.length - 1);
} else if (num > minHeap[0]) {
// If current element larger than smallest in heap
minHeap[0] = num;
bubbleDown(minHeap, 0);
}
}
return minHeap[0];
}
// Third approach: QuickSelect (average O(n))
function findKthLargestQuickSelect(nums, k) {
// Implementation of quickselect algorithm
// (Simplified for brevity)
return quickSelect(nums, 0, nums.length - 1, nums.length - k);
}
The “How Would You Test This?” Follow-up
After you’ve implemented a solution, interviewers increasingly ask about testing strategies.
Example: “How would you test your function that validates email addresses?”
Strong responses include:
- Unit test cases for various inputs (valid emails, invalid formats, edge cases)
- Property-based testing approaches
- Integration testing considerations
- Performance testing for large inputs
// Example test cases for email validation
function testEmailValidator() {
const testCases = [
// Valid emails
{ input: "user@example.com", expected: true },
{ input: "user.name+tag@example.co.uk", expected: true },
// Invalid emails
{ input: "user@", expected: false },
{ input: "@example.com", expected: false },
{ input: "user@.com", expected: false },
{ input: "", expected: false },
// Edge cases
{ input: "a@b.c", expected: true }, // Minimal valid email
{ input: "user@127.0.0.1", expected: true }, // IP address domain
{ input: "very.long.email.address.with.many.parts@very.long.domain.name.with.many.subdomains.example", expected: true }
];
for (const testCase of testCases) {
const result = validateEmail(testCase.input);
console.assert(
result === testCase.expected,
`Failed for input: ${testCase.input}, got: ${result}, expected: ${testCase.expected}`
);
}
}
The “Explain Your Code to a Non-Technical Person” Challenge
Technical communication skills are increasingly valued, and some interviewers will ask you to explain your solution in non-technical terms.
This tests your ability to:
- Abstract away unnecessary details
- Use appropriate analogies
- Focus on the problem and solution rather than implementation specifics
Practicing this skill can differentiate you from other technically proficient candidates.
Practical Implementation: A Week-by-Week Preparation Plan
Let’s translate these insights into a concrete preparation plan for the four weeks leading up to your interview.
Week 1: Fundamentals and Assessment
- Day 1-2: Take a practice assessment to identify your strengths and weaknesses
- Day 3-5: Review fundamental data structures (arrays, linked lists, trees, graphs, hash tables)
- Day 6-7: Practice basic algorithm patterns (two pointers, sliding window, DFS/BFS)
Weekend Project: Implement a data structure from scratch that you find challenging (e.g., a balanced binary search tree or a priority queue)
Week 2: Problem-Solving Patterns
- Day 1-2: Dynamic programming problems
- Day 3-4: Graph algorithms and problems
- Day 5: Sorting and searching algorithms
- Day 6-7: Bit manipulation and mathematical problems
Daily Practice: For each problem, practice explaining your approach aloud before coding
Week 3: Mock Interviews and System Design
- Day 1-3: Schedule 3-5 mock interviews with peers or using interview platforms
- Day 4-5: System design practice (if relevant for your level)
- Day 6-7: Review and refine your answers to common behavioral questions
Focus Area: Get comfortable with collaborative problem-solving and thinking aloud
Week 4: Refinement and Company-Specific Preparation
- Day 1-2: Research common question types at your target company
- Day 3-4: Practice language-specific questions and optimization techniques
- Day 5: Final mock interviews focusing on your weak areas
- Day 6-7: Light review, rest, and mental preparation
Key Activity: Practice explaining your past projects in a structured, concise way that highlights your technical contributions
Tools and Resources to Bridge the Preparation Gap
To implement the strategies we’ve discussed, here are some recommended resources:
Interactive Platforms with Realistic Environments
- AlgoCademy: Offers AI-powered guidance and realistic interview simulations
- Pramp: Peer-to-peer mock interviews with collaborative coding environments
- interviewing.io: Practice with professional interviewers from top companies
Books That Focus on Problem-Solving Approaches
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: Covers the interview process and common patterns
- “Elements of Programming Interviews”: Focuses on understanding problems rather than memorizing solutions
- “System Design Interview” by Alex Xu: Excellent for preparing for system design questions
Communities for Feedback and Support
- Tech Interview Handbook GitHub: Open-source interview preparation resources
- Blind: Anonymous community with recent interview experiences
- Reddit’s r/cscareerquestions: Discussion forum for interview experiences and advice
Conclusion: Aligning Your Preparation with Reality
The disconnect between interview preparation and actual interviews stems from focusing too much on solving specific problems and not enough on developing the problem-solving process and communication skills that interviewers actually evaluate.
By shifting your preparation to emphasize:
- Understanding patterns rather than memorizing solutions
- Practicing collaborative problem-solving
- Developing a structured approach to technical challenges
- Preparing for the full spectrum of question types
You’ll bridge the gap between preparation and reality, significantly improving your chances of success in technical interviews.
Remember that interviewers are typically looking for colleagues they can work with, not just programmers who can solve abstract puzzles. By aligning your preparation with this reality, you’ll not only perform better in interviews but also develop skills that will serve you throughout your career.
The most successful candidates approach interviews not as tests to pass but as opportunities to demonstrate how they think, communicate, and collaborate on technical challenges. With the right preparation approach, you can ensure that your interview performance truly reflects your capabilities as a developer.