Why Your Interview Nerves Are Affecting Your Coding (And How To Overcome Them)
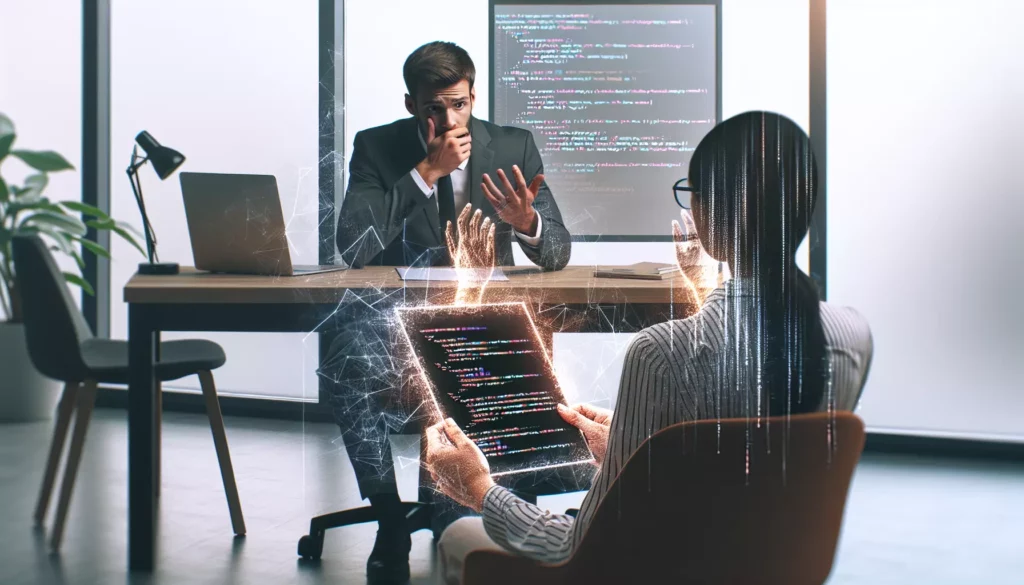
Technical interviews can be intimidating. You’re sitting across from someone who might be your future colleague, trying to solve a complex coding problem while they scrutinize your every keystroke. It’s no wonder that even the most competent programmers can freeze up when the pressure is on.
If you’ve ever blanked on an algorithm you knew perfectly the night before, or found yourself struggling with basic syntax during an interview, you’re not alone. Interview anxiety affects countless developers, from beginners to seasoned professionals.
In this comprehensive guide, we’ll explore how nervousness impacts your coding performance during interviews and provide actionable strategies to overcome these challenges. By understanding the psychological factors at play and implementing targeted techniques, you can showcase your true coding abilities even under pressure.
Understanding Interview Anxiety and Its Impact on Coding Performance
Before we dive into solutions, it’s important to understand what happens in your brain when anxiety takes over during a coding interview.
The Science Behind Interview Stress
When you experience stress, your body activates its “fight or flight” response. Your amygdala (the brain’s alarm system) signals the release of stress hormones like cortisol and adrenaline. These hormones prepare your body to either confront or escape from danger—a response that was useful for our ancestors facing physical threats but is less helpful when you’re trying to implement a binary search algorithm.
This stress response causes several physiological changes:
- Increased heart rate and blood pressure
- Shallow breathing
- Muscle tension
- Digestive disruptions (the infamous “butterflies” in your stomach)
More importantly for coding interviews, stress affects your cognitive functions in several critical ways:
Cognitive Impacts of Interview Anxiety
1. Working Memory Impairment
Working memory is your brain’s temporary workspace where you manipulate information. It’s essential for coding tasks like tracking variables, visualizing data structures, and stepping through algorithms.
Research has shown that stress can significantly reduce working memory capacity. This explains why you might suddenly forget the steps of a sorting algorithm you’ve implemented dozens of times before.
2. Attention Narrowing
Under stress, your attention tends to narrow, making it difficult to consider multiple approaches to a problem. This “tunnel vision” can prevent you from seeing elegant solutions or recognizing patterns that would be obvious in a relaxed state.
3. Executive Function Disruption
Stress impairs executive functions like planning, organization, and flexible thinking. These are precisely the skills you need to break down complex problems, organize your code efficiently, and pivot when your initial approach isn’t working.
4. Self-Monitoring Overload
During interviews, many developers fall into a trap of excessive self-monitoring—constantly evaluating their own performance while trying to solve the problem. This dual cognitive load consumes valuable mental resources that could otherwise be directed toward problem-solving.
Real-World Manifestations in Coding Interviews
These cognitive effects manifest in several common interview scenarios:
- Syntax Errors: Forgetting basic syntax for languages you use daily
- Algorithm Amnesia: Blanking on algorithms and data structures you’ve studied extensively
- Problem-Solving Paralysis: Inability to break down problems into manageable components
- Communication Breakdown: Struggling to articulate your thought process clearly
- Time Management Issues: Either rushing through problems or getting stuck in details
One study from the Journal of Experimental Psychology found that participants under stress took up to 50% longer to solve complex problems and made nearly twice as many errors compared to relaxed participants. For coding interviews where both speed and accuracy matter, this performance gap can be the difference between receiving an offer and being rejected.
Signs Your Nerves Are Affecting Your Coding
Recognizing when anxiety is impacting your coding performance is the first step toward addressing it. Here are some telltale signs:
During Interview Preparation
- Procrastination: Avoiding practice because it triggers anxiety
- Catastrophizing: Mentally rehearsing worst-case scenarios
- Perfectionism: Setting unrealistic standards for your preparation
- Sleep Disruption: Difficulty sleeping due to interview-related worries
During the Interview
- Mind Blanks: Suddenly forgetting familiar concepts
- Physical Symptoms: Shaky hands, dry mouth, racing heart
- Code Paralysis: Staring at the screen, unable to start coding
- Overexplaining or Underexplaining: Either rambling nervously or becoming uncommunicative
- Error Blindness: Missing obvious bugs in your code
- Time Distortion: Feeling like time is passing too quickly
Consider this example of how anxiety might affect a typical coding interview problem:
Problem: Implement a function to find if a binary tree is balanced
How a relaxed developer might approach it:
- Clarify the definition of “balanced” (height difference between subtrees is no more than 1)
- Consider a recursive approach to check heights of left and right subtrees
- Implement a helper function to calculate height
- Write the main function to compare heights and return the result
- Test with examples and edge cases
How an anxious developer might struggle:
- Freeze initially, feeling overwhelmed by the problem
- Jump straight to coding without planning
- Get confused about the tree traversal logic
- Make basic syntax errors while implementing the recursive function
- Forget to handle edge cases like null nodes
- Run out of time before completing a working solution
The difference isn’t in knowledge or ability—it’s in how anxiety interferes with the application of that knowledge under pressure.
Strategies to Overcome Interview Anxiety
Now that we understand how anxiety affects coding performance, let’s explore practical strategies to manage it before and during interviews.
Long-Term Preparation Strategies
1. Systematic Desensitization Through Mock Interviews
One of the most effective ways to reduce interview anxiety is through repeated exposure in simulated conditions. Regular mock interviews help desensitize you to the stress of being evaluated while coding.
Implementation tips:
- Schedule weekly mock interviews with peers, mentors, or using platforms like Pramp or interviewing.io
- Gradually increase the difficulty and formality of these sessions
- Record your sessions to identify patterns in how anxiety affects your performance
- Focus on recreating realistic interview conditions (time constraints, explaining your thought process aloud)
2. Develop a Consistent Problem-Solving Framework
Having a structured approach to problem-solving provides a mental safety net during high-pressure situations. When anxiety strikes, you can fall back on your framework rather than relying on spontaneous thinking.
A robust framework might include:
- Understand: Clarify the problem and requirements
- Plan: Sketch your approach before coding
- Simplify: Start with a basic solution before optimizing
- Implement: Write clean, modular code
- Test: Verify with examples and edge cases
- Optimize: Improve time/space complexity if needed
This framework becomes an autopilot mode you can activate when anxiety threatens to derail your thinking.
3. Overlearning Key Concepts
“Overlearning” refers to practicing beyond the point of initial mastery. When you overlearn something, it becomes resistant to the effects of stress and anxiety.
How to implement overlearning:
- Identify your core weaknesses (e.g., dynamic programming, graph algorithms)
- Solve multiple variations of problems in these areas
- Implement solutions from scratch repeatedly until they become second nature
- Practice explaining your solutions to reinforce understanding
For example, don’t just solve one binary tree problem—solve 20 different variations until the patterns become automatic, even under stress.
4. Cognitive Restructuring
Many developers sabotage their interview performance with negative thought patterns. Cognitive restructuring involves identifying and challenging these thoughts.
Common negative thoughts and their alternatives:
Negative Thought | Restructured Thought |
---|---|
“If I make a mistake, they’ll think I’m incompetent.” | “Everyone makes mistakes. How I handle them shows my problem-solving abilities.” |
“I need to find the optimal solution immediately.” | “It’s better to start with a working solution and optimize incrementally.” |
“The interviewer probably thinks I’m not good enough.” | “The interviewer wants to see my problem-solving process, not just the final solution.” |
“I should know this instantly.” | “Taking time to think demonstrates thoroughness and care.” |
Day-Before Interview Strategies
1. Strategic Review vs. Cramming
The day before your interview is not the time to learn new algorithms or tackle challenging problems. This last-minute cramming can increase anxiety without improving performance.
Instead, focus on:
- Reviewing your personal “greatest hits”—problems you’ve solved well in the past
- Lightly refreshing key concepts with flashcards or quick notes
- Practicing 1-2 medium difficulty problems to maintain momentum
2. Physical Preparation
Your physical state directly impacts your cognitive performance. The day before your interview:
- Sleep: Prioritize getting 7-8 hours of quality sleep
- Exercise: Moderate physical activity can reduce anxiety and improve cognitive function
- Nutrition: Avoid excessive caffeine or alcohol, which can disrupt sleep and increase anxiety
- Prepare your environment: Set up your interview space with all necessary tools and resources
3. Visualization
Athletes use visualization techniques to prepare for competition, and programmers can benefit from the same approach:
- Find a quiet space and close your eyes
- Imagine yourself in the interview, feeling calm and confident
- Visualize successfully working through a challenging problem
- Picture yourself handling difficulties with composure
- Imagine the interview ending successfully
This mental rehearsal helps your brain establish neural pathways for success, making it easier to access those states during the actual interview.
During-Interview Anxiety Management
1. The Two-Minute Reset
If you feel anxiety rising during an interview, this quick reset can help you regain control:
- Recognize: Acknowledge that you’re experiencing anxiety
- Breathe: Take 3-5 deep, diaphragmatic breaths
- Reorient: Return to your problem-solving framework
- Communicate: Share your thought process with the interviewer
For example: “I’m going to take a moment to think about this problem systematically” gives you space to reset while showing the interviewer your methodical approach.
2. Strategic Communication
Many candidates forget that technical interviews are as much about communication as they are about coding. Strategic communication can reduce anxiety by:
- Creating natural pauses for thinking
- Building rapport with the interviewer
- Demonstrating your problem-solving approach even if you’re stuck
Key communication techniques:
- Think aloud: “I’m considering two approaches here: a hash map solution and a two-pointer technique.”
- Ask clarifying questions: “Can I assume the input array is sorted?”
- Signpost your process: “First, I’ll handle the edge cases, then implement the main algorithm.”
- Acknowledge limitations: “I’m not immediately seeing the optimal solution, but let me start with a brute force approach we can refine.”
3. Micro-Recoveries for When You Blank
If you completely blank on an approach or algorithm, try these micro-recovery techniques:
- Return to first principles: Break down the problem into the most basic components
- Try concrete examples: Work through a specific example by hand to spark insights
- Consider related problems: “This reminds me of [similar problem]. Let me see if that approach can be adapted.”
- Start with brute force: Begin with the simplest possible solution, then look for optimization opportunities
Remember that interviewers often value your problem-solving process more than whether you reach the optimal solution immediately.
Specific Coding Techniques for High-Pressure Situations
Beyond general anxiety management, there are specific coding techniques that can help you perform better under pressure.
1. Incremental Coding
Rather than trying to write the entire solution at once, break it down into small, testable increments:
- Start with function signatures and input validation
- Implement core logic one step at a time
- Test each component before moving to the next
This approach reduces cognitive load and provides a sense of progress, which can help manage anxiety.
For example, when implementing a solution to find the longest substring without repeating characters:
// Step 1: Define function and handle edge cases
function lengthOfLongestSubstring(s) {
if (!s || s.length === 0) return 0;
if (s.length === 1) return 1;
// Step 2: Implement sliding window variables
let maxLength = 0;
let start = 0;
const charMap = new Map();
// Step 3: Implement the main algorithm logic
for (let end = 0; end < s.length; end++) {
const currentChar = s[end];
// Step 4: Handle character repetition
if (charMap.has(currentChar) && charMap.get(currentChar) >= start) {
start = charMap.get(currentChar) + 1;
}
charMap.set(currentChar, end);
maxLength = Math.max(maxLength, end - start + 1);
}
// Step 5: Return the result
return maxLength;
}
By breaking down the implementation into steps, you can maintain focus even when anxious.
2. Strategic Commenting
Using comments strategically serves multiple purposes in high-pressure situations:
- Creates a roadmap for your implementation
- Demonstrates your thought process to the interviewer
- Provides mental checkpoints to return to if you get lost
- Gives you a moment to think while typing something productive
For example, when implementing a solution for merging k sorted lists:
function mergeKLists(lists) {
// Edge case: empty input
if (!lists || lists.length === 0) return null;
// Create min heap for merging
// (Will use array + helper functions for interview simplicity)
// Helper: get parent, left child, right child indices
const getParentIndex = (i) => Math.floor((i - 1) / 2);
const getLeftChildIndex = (i) => 2 * i + 1;
const getRightChildIndex = (i) => 2 * i + 2;
// Helper: heapify function to maintain heap property
// ...
// Initialize heap with first node from each list
// ...
// Main merging algorithm
// ...
// Return the merged list
// ...
}
This comment structure allows you to think through the entire solution before diving into implementation details.
3. Test-Driven Approach
Starting with test cases before implementing your solution can reduce anxiety by:
- Ensuring you fully understand the problem requirements
- Providing concrete examples to guide your implementation
- Giving you a clear way to validate your solution
For example, when solving a string manipulation problem:
function isValidParentheses(s) {
// Test cases:
// isValidParentheses("()") => true
// isValidParentheses("()[]{}") => true
// isValidParentheses("(]") => false
// isValidParentheses("([)]") => false
// isValidParentheses("{[]}") => true
// Edge case: isValidParentheses("") => true
// Implementation:
const stack = [];
const mapping = {
')': '(',
'}': '{',
']': '['
};
for (let i = 0; i < s.length; i++) {
const char = s[i];
// If opening bracket, push to stack
if (!mapping[char]) {
stack.push(char);
}
// If closing bracket, check if it matches the last opening bracket
else if (stack.pop() !== mapping[char]) {
return false;
}
}
// If stack is empty, all brackets were properly closed
return stack.length === 0;
}
Starting with test cases helps you catch edge cases early and provides a roadmap for implementation.
4. Time Management Techniques
Poor time management during interviews often stems from anxiety. Try these techniques:
- The 5-minute rule: Spend the first 5 minutes planning before coding
- Timeboxing: “I’ll spend 10 minutes on the initial solution, then 5 minutes optimizing”
- Progress checkpoints: “By the halfway mark, I should have the basic algorithm implemented”
Being explicit about time management shows interviewers your organizational skills while helping you stay on track.
Learning from Interview Anxiety
Interview anxiety, while challenging, can also be a valuable learning opportunity. Here’s how to use your experiences to improve:
Post-Interview Reflection
After each interview, set aside time for structured reflection:
- Document the experience: What questions were asked? How did you respond?
- Identify anxiety triggers: What specific moments caused your anxiety to spike?
- Analyze performance gaps: Where did anxiety most impact your performance?
- Note what worked: Which coping strategies were effective?
This reflection helps transform each interview into a learning experience, regardless of the outcome.
Developing Anxiety Resilience
Over time, you can build resilience to interview anxiety through:
- Exposure therapy: Continually putting yourself in challenging interview situations
- Skills development: Building stronger technical foundations to increase confidence
- Perspective shift: Viewing interviews as opportunities to learn rather than tests to pass
- Growth mindset: Believing your interview abilities can improve with practice
Many successful developers report that while they still experience interview anxiety, they’ve learned to perform effectively despite it.
When to Seek Additional Support
While some level of interview anxiety is normal, persistent, severe anxiety might require additional support:
- Therapy: Cognitive-behavioral therapy (CBT) has proven effective for performance anxiety
- Meditation and mindfulness: Regular practice can reduce general anxiety levels
- Peer support groups: Connecting with others facing similar challenges
- Professional coaching: Working with interview coaches specialized in anxiety management
Remember that seeking help is a sign of strength, not weakness, and many top performers work with coaches or therapists to optimize their performance.
Conclusion: From Anxiety to Advantage
Interview nerves affect nearly every developer at some point in their career. The difference between those who succeed despite anxiety and those who are derailed by it often comes down to preparation, mindset, and the strategic application of anxiety management techniques.
By understanding how anxiety impacts your coding performance and implementing the strategies outlined in this guide, you can transform interview anxiety from a liability into an opportunity to demonstrate your resilience and problem-solving abilities.
Remember that interviewers are evaluating not just your technical skills but your ability to perform under conditions similar to real-world development environments, where pressure and uncertainty are common. Showing that you can think clearly and code effectively despite nervousness may actually strengthen your candidacy.
The next time you feel your heart racing before a coding interview, remember: your anxiety doesn’t define your abilities, and with the right approach, you can showcase your true potential even under pressure.
Keep practicing, keep reflecting, and keep growing—both as a developer and as a confident interview performer.