Why Your Interview Communication Isn’t Showing Your Knowledge
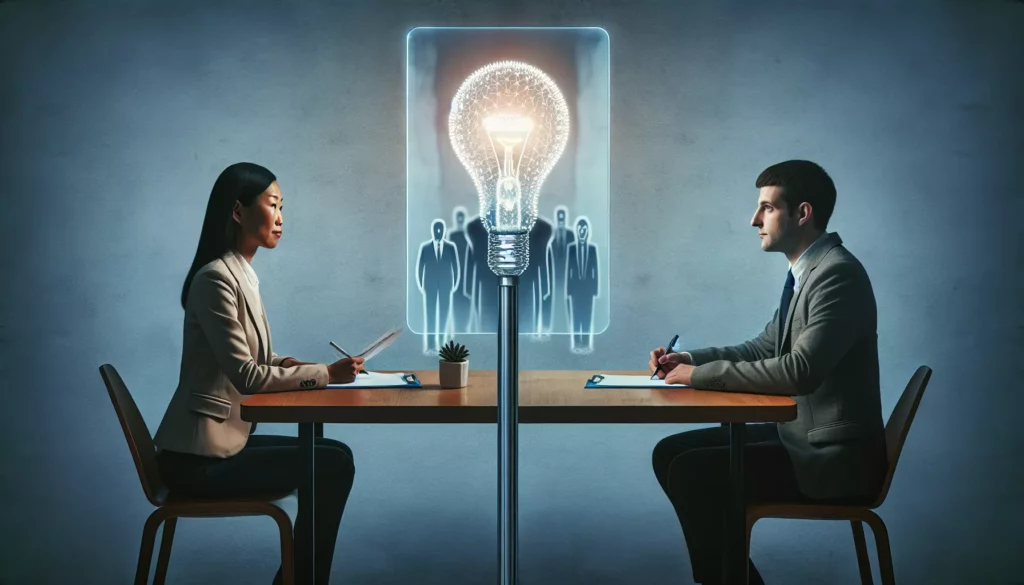
For software engineers and developers, technical interviews can be a frustrating experience. You might have spent years honing your programming skills, mastering complex algorithms, and building impressive projects, only to stumble when trying to communicate your knowledge during an interview. The disconnect between what you know and how you present it can be the difference between landing your dream job and continuing your search.
This phenomenon is particularly common when interviewing at top tech companies like Google, Amazon, Meta, or other major players in the industry. These companies don’t just evaluate your technical abilities; they assess how effectively you can communicate your thought process and collaborate with others.
The Hidden Barrier Between Knowledge and Communication
Most engineers focus intensely on technical preparation. They solve countless LeetCode problems, memorize algorithm complexities, and study system design patterns. While this preparation is necessary, it often neglects a crucial component: the ability to articulate your thinking clearly and effectively during high-pressure situations.
Let’s explore why your interview communication might not be showcasing your true capabilities and how you can bridge this gap.
Common Communication Pitfalls in Technical Interviews
1. Diving Into Code Too Quickly
One of the most common mistakes candidates make is rushing straight into coding without adequately discussing their approach. When an interviewer presents a problem, they’re not just looking for the correct solution; they want insight into your problem-solving methodology.
Consider this scenario: You’re asked to implement a function to find all pairs in an array that sum to a target value. Instead of discussing potential approaches, you immediately start writing:
def findPairs(nums, target):
result = []
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
result.append([nums[i], nums[j]])
return result
While this solution works, you’ve missed an opportunity to demonstrate your analytical thinking by discussing:
- The brute force approach and its time complexity (O(n²))
- More efficient alternatives like using a hash map (O(n))
- Trade-offs between different approaches
- Edge cases and potential optimizations
2. Unclear Explanation of Your Thought Process
Even when candidates do verbalize their thinking, they often do so in a disorganized or incomplete manner. Your internal thought process may be logical, but if you can’t externalize it coherently, the interviewer can’t evaluate it properly.
Unclear explanations often sound like:
“So I think we can use a hash map here… actually, maybe a two-pointer approach… wait, let me think… yeah, I’ll go with a hash map because… actually, let me just start coding and I’ll figure it out.”
This stream-of-consciousness style leaves the interviewer wondering if you actually understand the problem and have a clear solution strategy.
3. Failing to Establish Context and Requirements
Many candidates jump into solving problems without confirming their understanding or clarifying requirements. This can lead to solving the wrong problem or overlooking important constraints.
For example, if asked to implement a function to find the kth largest element in an array, you might need to clarify:
- Whether duplicates should be counted distinctly
- If the array is sorted or unsorted
- Whether the solution needs to be optimized for time or space
- The expected input ranges and potential edge cases
Skipping this clarification phase not only risks misinterpreting the problem but also misses an opportunity to demonstrate thoroughness and attention to detail.
4. Silence During Implementation
Many candidates fall silent when they begin coding, leaving the interviewer in the dark about their reasoning. This creates an awkward atmosphere and prevents the interviewer from providing guidance if you’re heading down the wrong path.
Remember that in most technical interviews, the process matters more than the final solution. An interviewer would rather see an incomplete solution with clear reasoning than a perfect solution with no explanation of how you arrived there.
5. Technical Jargon Without Context
Using technical terminology can demonstrate knowledge, but overusing jargon without explaining concepts can make your communication less effective. Not all interviewers will be experts in every domain, and throwing around terms like “memoization,” “polymorphism,” or “idempotent” without context might create confusion rather than confidence.
The Psychology Behind Interview Communication Failures
Cognitive Load and Performance Anxiety
Technical interviews place enormous cognitive demands on candidates. You’re simultaneously trying to:
- Understand a new problem
- Recall relevant algorithms and data structures
- Develop a solution strategy
- Write correct code
- Explain your thinking clearly
- Manage time constraints
- Handle the pressure of being evaluated
This cognitive load can overwhelm your brain’s processing capacity, leading to communication breakdown. When your working memory is saturated with problem-solving tasks, the quality of your verbal expression often suffers.
Additionally, performance anxiety activates your body’s stress response, redirecting blood flow away from the prefrontal cortex (responsible for clear thinking and communication) toward systems needed for “fight or flight.” This physiological response can literally make it harder to form coherent thoughts and sentences.
The Curse of Knowledge
As an experienced developer, you may fall victim to what psychologists call the “curse of knowledge” — the cognitive bias where individuals who are knowledgeable about a subject have difficulty understanding how it appears to someone with less knowledge.
You might skip explaining certain concepts because they seem obvious to you, not realizing that the interviewer needs to hear your reasoning to evaluate your understanding. What’s intuitive to you after years of programming experience isn’t necessarily evident to someone hearing your thought process for the first time.
How Top Candidates Communicate During Technical Interviews
Let’s examine how successful candidates structure their communication during coding interviews:
1. The STAR Method for Technical Problems
Adapting the STAR method (Situation, Task, Action, Result) to technical problem-solving provides a clear framework for your response:
- Situation: Restate the problem to confirm understanding
- Task: Clarify requirements and constraints
- Action: Explain your approach before coding
- Result: Implement the solution while explaining key decisions
Here’s how this might look in practice for our earlier array sum problem:
Situation: “So I understand we need to find all pairs in this array that sum to the target value.”
Task: “Before I start, I want to clarify a few things. Should I return the indices of these pairs or the actual values? Are there any constraints on the input array, like size or whether it contains duplicates? Can a number be used twice to form a pair?”
Action: “I’m thinking of two possible approaches. The brute force method would be to use nested loops to check every possible pair, which would be O(n²) time complexity. A more efficient approach would use a hash map to store values we’ve seen, allowing us to find complements in O(1) time, giving us an overall O(n) solution. Given the efficiency advantage, I’ll implement the hash map approach.”
Result: “Here’s my implementation…” [proceeds to code while explaining key steps]
2. Think-Aloud Protocol
The think-aloud protocol involves verbalizing your thoughts as they occur. This technique gives interviewers insight into your problem-solving process and demonstrates your ability to communicate technical concepts in real time.
For example, while implementing a solution:
“I’m creating a hash map to store the values I’ve seen so far. For each element in the array, I’ll calculate what value would need to be in the hash map to form a pair summing to our target. If that value exists, I’ve found a valid pair. Now I need to consider edge cases like empty arrays or no valid pairs…”
This running commentary helps interviewers follow your logic and demonstrates your ability to think critically while coding.
3. Structured Problem Decomposition
Top candidates break down complex problems into manageable components before diving into implementation. This structured approach typically follows these steps:
- Problem Understanding: Restate the problem and ask clarifying questions
- Examples: Work through simple examples to understand the expected behavior
- Approach Brainstorming: Discuss multiple potential solutions
- Complexity Analysis: Analyze time and space complexity of each approach
- Approach Selection: Choose an approach and justify your selection
- Implementation Planning: Outline your code structure before writing it
- Implementation: Code the solution while explaining key steps
- Testing: Verify your solution with test cases and edge cases
This methodical communication style demonstrates not just technical knowledge but also a systematic approach to problem-solving.
Practical Strategies to Improve Your Interview Communication
Before the Interview: Preparation Techniques
1. Practice Verbalization Through Mock Interviews
The best way to improve communication during actual interviews is to practice in simulated conditions. Mock interviews force you to articulate your thought process while solving problems under time constraints.
Resources for mock interview practice:
- Peer programming sessions with colleagues
- Mock interview platforms like Pramp or interviewing.io
- Recording yourself solving problems and reviewing your explanations
- Working with a coding interview coach
The key is to receive feedback specifically on your communication, not just your technical solution.
2. Develop a Problem-Solving Template
Create a personal template for approaching interview problems. Having a consistent structure helps reduce cognitive load and ensures you don’t skip important steps in your explanation.
A basic template might include:
- Problem restatement and clarification questions
- Discussion of input/output examples
- Identification of potential approaches
- Analysis of time and space complexity
- Selection of an approach with justification
- High-level algorithm description
- Implementation with ongoing explanation
- Testing and edge case handling
Practicing with this template until it becomes second nature will help you communicate more effectively under pressure.
3. Build a Technical Communication Vocabulary
Develop a repertoire of clear, concise phrases for explaining common programming concepts. Having ready-made explanations reduces the mental effort required to articulate complex ideas during interviews.
For example, instead of stumbling through an explanation of dynamic programming, you might prepare something like:
“Dynamic programming is an optimization technique where we solve complex problems by breaking them down into simpler subproblems. We solve each subproblem only once and store the results to avoid redundant calculations, trading memory for speed.”
Having these explanations prepared makes your communication more fluent and precise.
During the Interview: Active Communication Techniques
1. Implement the 10-Second Rule
Before writing any code, take at least 10 seconds to outline your approach verbally. This brief pause forces you to organize your thoughts and gives the interviewer insight into your reasoning.
For example:
“Before I start coding, let me outline my approach. I’ll use a breadth-first search algorithm to traverse the graph, keeping track of visited nodes to avoid cycles. I’ll need a queue to manage the traversal order and a set to track visited nodes. The time complexity will be O(V+E) where V is the number of vertices and E is the number of edges.”
This brief overview demonstrates your analytical thinking and gives the interviewer a roadmap of your solution.
2. Use Visual Aids
Complex algorithms and data structures are often easier to explain with visual representations. Don’t hesitate to use diagrams or tables to illustrate your thinking.
For example, when explaining a tree traversal algorithm, you might draw the tree structure and trace the traversal path, explaining each step. This visual component can make your explanation more comprehensible and demonstrates your ability to communicate technical concepts in multiple formats.
3. Implement Checkpoint Communication
During implementation, establish regular communication checkpoints to summarize what you’ve done and what you’re about to do next. This technique keeps the interviewer engaged and demonstrates your ability to maintain a high-level perspective while working on details.
For example:
“So far I’ve initialized our data structures and implemented the helper function. Now I’m going to implement the main algorithm, which will iterate through the array and update our result according to the logic we discussed.”
These checkpoints create natural pauses for the interviewer to provide feedback or ask questions, making the interview more interactive.
4. Verbalize Code as You Write It
As you implement your solution, explain each line or block of code as you write it. This running commentary helps the interviewer follow your implementation and demonstrates your ability to think and communicate simultaneously.
For example:
// I'm creating a hash map to store values we've seen so far
const seen = new Map();
// Now I'll iterate through each element in the array
for (let i = 0; i < nums.length; i++) {
// Calculate the complement we need to reach our target sum
const complement = target - nums[i];
// Check if we've seen this complement before
if (seen.has(complement)) {
// If found, we have a valid pair
return [seen.get(complement), i];
}
// Otherwise, add the current number to our map
seen.set(nums[i], i);
}
This narration demonstrates your understanding of each step in your implementation and helps the interviewer follow your logic.
Adapting Your Communication Style to Different Interview Formats
Remote Coding Interviews
Remote interviews present unique communication challenges. Without in-person cues, it's even more important to be explicit in your communication. Consider these adaptations:
- Check for understanding more frequently: "Does that explanation make sense?" or "Would you like me to elaborate on any part of my approach?"
- Use screen annotations: Utilize drawing tools in shared coding environments to highlight important aspects of your solution
- Manage technical issues proactively: "I notice there's a slight delay. I'll pause more frequently to ensure you can follow my explanation."
- Be more explicit about your actions: "I'm now scrolling up to reference the function I defined earlier."
System Design Interviews
System design interviews require a different communication approach than algorithm problems. The focus shifts from code implementation to high-level architecture and design decisions.
Effective communication in system design interviews includes:
- Requirements gathering: "Before diving into the design, let's clarify the requirements. What scale are we designing for? What are the key features needed?"
- Component breakdown: "I'll break this system into key components: the client interface, application servers, data storage layer, and caching mechanism."
- Tradeoff analysis: "We could use SQL or NoSQL for storage. SQL gives us transaction guarantees but might limit our scaling options. NoSQL offers better horizontal scaling but with eventual consistency tradeoffs."
- Iteration based on feedback: "Based on your point about traffic patterns, let's revisit our load balancing strategy."
Behavioral Interviews
Technical communication extends beyond code discussions. Behavioral interviews assess how you communicate about past experiences and soft skills.
When discussing technical projects in behavioral interviews:
- Adjust technical depth based on your audience: A technical interviewer might appreciate implementation details, while a hiring manager might prefer to hear about impact and business outcomes
- Connect technical decisions to business value: "By implementing this caching layer, we reduced database load by 40% and improved response times by 200ms, which directly increased user engagement."
- Highlight collaboration: "When we faced this technical challenge, I collaborated with the infrastructure team to design a solution that addressed both performance and security requirements."
Case Study: Before and After Communication Transformation
Let's examine how improved communication can transform the same candidate's performance on a typical interview problem.
The Problem: Implement a function to find the longest substring without repeating characters.
Before: Poor Communication
Interviewer: "Can you implement a function to find the longest substring without repeating characters?"
Candidate: "Ok, let me think... we need to find the longest substring without repeats. I think I can use a sliding window approach."
[Begins coding immediately without further explanation]
function lengthOfLongestSubstring(s) { let maxLength = 0; let start = 0; const charMap = {}; for (let end = 0; end < s.length; end++) { if (charMap[s[end]] !== undefined && charMap[s[end]] >= start) { start = charMap[s[end]] + 1; } charMap[s[end]] = end; maxLength = Math.max(maxLength, end - start + 1); } return maxLength; }
[Works in silence, occasionally muttering to self]
Interviewer: "Can you walk me through your approach?"
Candidate: "Sure, I'm using a sliding window with a hash map to keep track of characters. When I find a repeat, I move the window."
Interviewer: "What's the time complexity?"
Candidate: "Um, I think it's O(n) because we're just going through the string once."
In this example, the candidate:
- Provided minimal explanation of their approach
- Started coding immediately without discussing alternatives
- Worked silently, making it difficult for the interviewer to follow their thinking
- Gave a brief, incomplete explanation when prompted
- Didn't discuss edge cases or test their solution
After: Effective Communication
Interviewer: "Can you implement a function to find the longest substring without repeating characters?"
Candidate: "I'd be happy to. Let me first make sure I understand the problem correctly. We need to find the length of the longest substring that doesn't have any character appearing more than once, correct?"
Interviewer: "That's right."
Candidate: "Let me think about some examples to clarify. For the string 'abcabcbb', the answer would be 3, corresponding to the substring 'abc'. For 'bbbbb', the answer would be 1, just a single character. And for 'pwwkew', the answer would be 3, which is 'wke'. Is that understanding correct?"
Interviewer: "Yes, that's correct."
Candidate: "Great. Let me consider possible approaches. A brute force solution would be to check every possible substring and verify it has no repeating characters. That would be O(n³) in the worst case – O(n²) to generate all substrings and O(n) to check each for duplicates.
A more efficient approach would use a sliding window technique with a hash map or set to track characters in the current window. This would give us O(n) time complexity since we only need to traverse the string once.
I'll implement the sliding window approach since it's more efficient. The idea is to maintain a window that contains only unique characters. When we encounter a repeated character, we shrink the window from the left until we eliminate the duplicate."
Interviewer: "Sounds good."
Candidate: "I'll use a hash map to store the most recent index of each character. Here's my implementation:"
function lengthOfLongestSubstring(s) { // Edge case: empty string if (s.length === 0) return 0; let maxLength = 0; let start = 0; // Start of current window const charMap = {}; // Maps characters to their most recent index for (let end = 0; end < s.length; end++) { const currentChar = s[end]; // If we've seen this character before and it's within our current window if (charMap[currentChar] !== undefined && charMap[currentChar] >= start) { // Move window start to just after the previous occurrence start = charMap[currentChar] + 1; } // Update character's most recent position charMap[currentChar] = end; // Update max length if current window is larger maxLength = Math.max(maxLength, end - start + 1); } return maxLength; }
"Now let me trace through this with an example. For the string 'abcabcbb':
- We start with an empty window, maxLength = 0, start = 0
- Process 'a': Add to charMap, window = 'a', maxLength = 1
- Process 'b': Add to charMap, window = 'ab', maxLength = 2
- Process 'c': Add to charMap, window = 'abc', maxLength = 3
- Process 'a': Found repeat, move start to index 1, window = 'bca', maxLength = 3
- Continue this process...
The time complexity is O(n) where n is the string length, as we process each character once. The space complexity is O(min(m, n)) where m is the size of the character set, as our hash map will at most contain each unique character in the string."
Interviewer: "What about edge cases?"
Candidate: "I've handled the empty string case explicitly. For strings with a single character, our algorithm correctly returns 1. If the input contains only repeating characters like 'aaaaa', we'll correctly return 1. The algorithm should work for any valid string input, including those with special characters or numbers, as we're just tracking character indices without any character-specific logic."
In this improved example, the candidate:
- Restated the problem and confirmed understanding
- Worked through examples to clarify the expected behavior
- Discussed multiple approaches and their complexities
- Explained their chosen approach before implementing it
- Commented their code and explained each step
- Traced through an example to verify correctness
- Analyzed time and space complexity
- Proactively addressed edge cases
The technical solution is the same in both cases, but the communication quality dramatically affects how the interviewer perceives the candidate's abilities.
The Long-Term Benefits of Strong Technical Communication
Improving your interview communication skills doesn't just help you pass technical interviews; it enhances your effectiveness as a software engineer throughout your career.
Career Advancement Beyond the Interview
Engineers who communicate effectively:
- Receive better performance reviews: Managers value team members who can clearly explain technical concepts and decisions
- Lead more successful projects: Clear communication reduces misunderstandings and improves collaboration
- Mentor junior developers more effectively: Teaching requires translating complex concepts into understandable explanations
- Have more influence on technical decisions: The most persuasive technical arguments are often those most clearly articulated
Building a Growth Mindset Around Communication
Just as you continuously improve your technical skills, approach communication as a skill to be developed over time. Each interview, code review, and technical discussion is an opportunity to refine your ability to articulate complex ideas.
Consider keeping a journal of communication challenges you face and strategies that work well for you. Regularly seek feedback on your communication clarity, not just your technical solutions.
Conclusion: Bridging the Knowledge-Communication Gap
Technical knowledge alone isn't enough to succeed in today's collaborative software development environment. The ability to clearly communicate your thought process, explain technical decisions, and collaborate effectively is equally important—especially during interviews at top tech companies.
By implementing the strategies outlined in this article, you can ensure that your interview performance accurately reflects your technical abilities. Remember that communication is not just about expressing what you know; it's about making your knowledge accessible and valuable to others.
The next time you prepare for a technical interview, dedicate as much time to practicing your communication as you do to solving problems. Record yourself explaining solutions, participate in mock interviews that focus on verbalization, and develop a structured approach to problem-solving that incorporates clear communication at every step.
With practice, the gap between what you know and what you can effectively communicate will narrow, allowing your true technical abilities to shine through in every interview.