Why Your Impressive GitHub Profile Isn’t Translating to Real World Skills
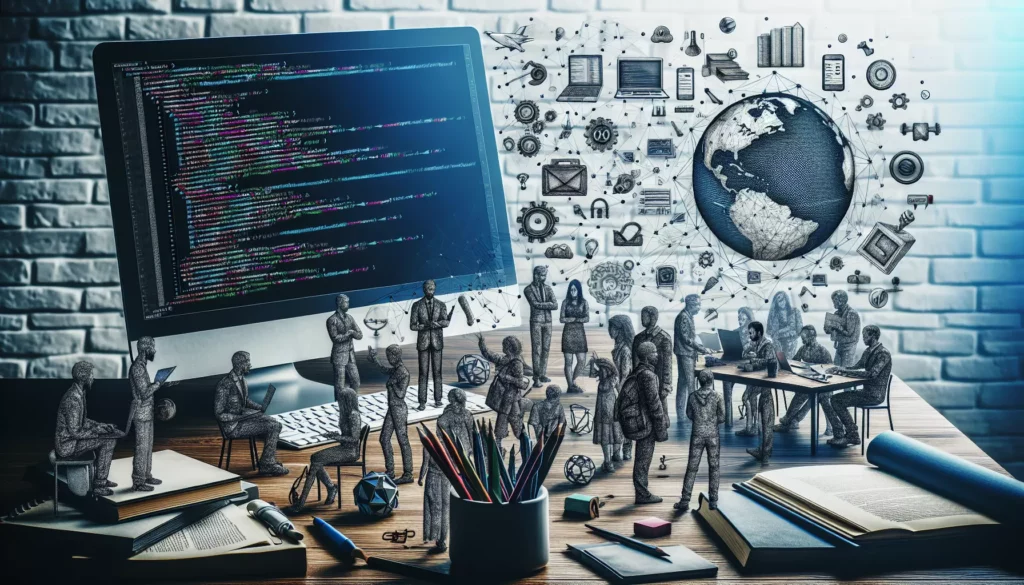
The green squares on your GitHub contribution graph look impressive. Your repositories showcase numerous projects. Your README is polished and professional. Yet, when faced with real coding challenges at work or during interviews, something doesn’t click. This disconnect between GitHub appearances and practical coding abilities is more common than you might think.
In this article, we’ll explore why an impressive GitHub profile doesn’t always translate to real world programming skills, and more importantly, what you can do to bridge that gap.
The GitHub Illusion: When Green Squares Deceive
GitHub has become the de facto portfolio for developers. It’s often the first place potential employers look to assess your coding abilities. However, there’s a growing recognition that GitHub activity can create a misleading impression of a developer’s actual capabilities.
Quantity Over Quality
One of the most visible features of GitHub is the contribution graph—those green squares that show your activity. While consistent contributions can demonstrate dedication, they say little about the quality or complexity of your work.
Consider these scenarios that generate green squares:
- Making minor documentation updates
- Fixing simple typos
- Committing code that doesn’t actually work
- Pushing trivial changes across multiple days instead of meaningful commits
None of these activities necessarily improve your coding skills, yet they all make your GitHub profile look more active.
Repository Quantity vs. Depth of Understanding
Having dozens of repositories might seem impressive, but quantity alone doesn’t equate to skill. Many developers fall into the trap of starting numerous projects without diving deep into any of them. This results in a GitHub profile filled with:
- Abandoned projects that never reached completion
- Tutorial code copied without deep understanding
- Forked repositories never actually modified
- “Hello World” level projects across multiple frameworks
True skill development comes from wrestling with complex problems and seeing projects through to completion, not from starting many shallow projects.
Missing Elements: What GitHub Doesn’t Showcase
GitHub is an excellent platform for showcasing code, but it fails to capture many crucial aspects of real world programming proficiency.
Collaborative Skills
Professional software development is inherently collaborative. GitHub can show pull requests and issues, but it doesn’t fully capture your ability to:
- Communicate technical concepts clearly to team members
- Navigate disagreements about implementation approaches
- Mentor junior developers
- Adapt to established team workflows and coding standards
- Give and receive constructive code reviews
These soft skills are often just as important as technical abilities in professional settings.
Working Within Constraints
Personal projects typically don’t face the same constraints as professional work:
- Strict deadlines
- Legacy code integration requirements
- Scalability concerns for thousands or millions of users
- Security considerations for sensitive data
- Accessibility requirements
- Cross browser compatibility needs
Your GitHub projects might work perfectly in ideal conditions, but professional development rarely offers such luxury.
Problem Solving Under Pressure
GitHub doesn’t show how you perform when:
- A critical production bug needs immediate fixing
- You’re asked to estimate completion time for unfamiliar tasks
- Requirements change midway through implementation
- You need to debug issues without complete information
These scenarios require a combination of technical knowledge, experience, and mental fortitude that isn’t evident from repository listings.
The Tutorial Trap: Following vs. Creating
Many GitHub repositories result from following tutorials rather than independent problem solving. While tutorials are valuable learning tools, they can create a false sense of mastery.
Tutorial Projects vs. Original Work
There’s a significant difference between following step by step instructions and creating solutions from scratch. When following tutorials:
- The problem is pre defined
- The solution approach is predetermined
- Potential obstacles are typically addressed in advance
- Edge cases may be ignored for simplicity
In contrast, real world development requires you to:
- Define the problem clearly before solving it
- Evaluate multiple possible approaches
- Anticipate and handle edge cases
- Debug unexpected issues
The Copy/Paste Syndrome
It’s easy to copy code from Stack Overflow or tutorials without fully understanding how it works. Your GitHub might contain impressive looking code that you couldn’t recreate independently or modify if requirements changed.
This knowledge gap becomes apparent during technical interviews or when faced with similar but not identical problems at work.
The Algorithmic Disconnect
Many GitHub projects focus on creating functional applications without emphasizing algorithmic efficiency or computer science fundamentals.
Application Development vs. Algorithmic Thinking
Building a web application with a modern framework demonstrates different skills than solving algorithmic challenges. Your GitHub might showcase:
- Connecting APIs to display data
- Implementing authentication flows
- Creating responsive layouts
While these are valuable skills, they don’t necessarily develop your ability to:
- Analyze time and space complexity
- Select appropriate data structures
- Optimize algorithms for performance
- Solve complex computational problems
This disconnect becomes apparent during technical interviews that focus on algorithmic problem solving.
Framework Knowledge vs. Language Fundamentals
Many repositories demonstrate framework usage without showcasing deep understanding of the underlying language. You might be proficient with React components or Django models, but struggle with JavaScript closures or Python memory management.
Framework abstractions can hide complexity that becomes important when debugging or optimizing code in professional settings.
// Example: Using React hooks without understanding closures
function Counter() {
const [count, setCount] = useState(0);
// This might seem simple in a small component
// but could lead to bugs in complex applications
// if you don't understand JavaScript's closure mechanism
useEffect(() => {
const interval = setInterval(() => {
setCount(count + 1); // This will not work as expected
}, 1000);
return () => clearInterval(interval);
}, []);
return <div>{count}</div>;
}
The Reality Gap: Academic vs. Professional Coding
The coding style showcased in personal projects often differs significantly from professional requirements.
Code Quality Standards
Personal projects typically don’t adhere to the same standards as professional code. Your GitHub repositories might lack:
- Comprehensive test coverage
- Detailed documentation
- Consistent code style
- Error handling for edge cases
- Performance optimization
- Security considerations
These elements are often non negotiable in professional environments but easily overlooked in personal projects.
Project Scale Differences
Most GitHub projects are relatively small compared to enterprise applications. Skills that become crucial at scale include:
- Designing maintainable architecture
- Managing technical debt
- Implementing effective logging and monitoring
- Creating deployment pipelines
- Optimizing database queries
Without experience on larger codebases, these skills remain underdeveloped regardless of how many small projects you complete.
Bridging the Gap: From GitHub to Real World Competence
If you’ve recognized a disconnect between your GitHub profile and your practical coding abilities, here are strategies to bridge that gap.
Focus on Depth Over Breadth
Rather than creating many shallow projects, invest in deeper learning:
- Select one or two significant projects and develop them thoroughly
- Implement proper testing, documentation, and error handling
- Refactor code to improve its quality and maintainability
- Add advanced features that stretch your abilities
A single well developed project demonstrates more skill than dozens of half finished repositories.
Contribute to Open Source
Contributing to established open source projects offers invaluable experience:
- You’ll work with larger, more complex codebases
- You’ll need to follow established coding standards and processes
- Your code will be reviewed by experienced developers
- You’ll practice collaboration in a real world context
Start with small contributions like documentation improvements or bug fixes, then gradually tackle more complex issues.
Practice Algorithmic Problem Solving
Supplement your project work with dedicated algorithm practice:
- Solve problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Analyze the time and space complexity of your solutions
- Study different approaches to the same problem
- Implement classic algorithms and data structures from scratch
This practice develops the analytical thinking needed for both interviews and efficient code implementation.
Seek Code Reviews
Having your code reviewed is one of the fastest ways to improve:
- Share your projects with more experienced developers
- Participate in coding communities that offer peer review
- Consider pair programming sessions
- Take feedback constructively, even when it’s critical
Code reviews highlight blind spots in your knowledge and practices that self study might miss.
Build Projects That Solve Real Problems
Move beyond tutorial projects by building applications that:
- Solve actual problems you or others face
- Have real users with real feedback
- Require maintenance and updates over time
- Need to scale as usage grows
These projects will naturally encounter the constraints and challenges of professional development.
Developing a Balanced Skill Set
True programming proficiency requires a balance of various skills, many of which aren’t immediately visible on GitHub.
Technical Breadth and Depth
Develop both breadth across technologies and depth in core areas:
- Breadth: Familiarity with different languages, frameworks, and paradigms
- Depth: Mastery of fundamentals like data structures, algorithms, and design patterns
This combination allows you to select appropriate tools for different problems while implementing solutions effectively.
Soft Skills for Developers
Complement your technical abilities with crucial soft skills:
- Clear technical communication
- Collaboration and teamwork
- Time management and estimation
- Receiving and implementing feedback
- Problem solving methodology
These skills often differentiate successful professionals from those who struggle despite technical knowledge.
System Design and Architecture
As you advance, develop your ability to design larger systems:
- Study architecture patterns and their tradeoffs
- Learn about distributed systems concepts
- Practice designing for scalability and reliability
- Consider security implications at the design level
These skills become increasingly important as you work on larger applications or move into senior roles.
Practical Exercises to Build Real World Skills
Here are specific exercises to develop the skills that matter in professional settings:
Code Reading and Comprehension
Professional developers spend more time reading code than writing it:
- Select an unfamiliar open source project and document how it works
- Debug an application without looking at its implementation first
- Implement a new feature in an existing codebase without rewriting it
These exercises build the code comprehension skills essential for team environments.
Refactoring Challenges
Improving existing code is a common professional task:
- Take a working but messy project and refactor it without changing behavior
- Add tests to untested code, then refactor with confidence
- Identify and resolve code smells in your own projects
Refactoring builds your understanding of code quality and maintainability.
// Before refactoring
function getUsers(active) {
let users = [];
for (let i = 0; i < allUsers.length; i++) {
if (active) {
if (allUsers[i].status === 'active') {
users.push(allUsers[i]);
}
} else {
users.push(allUsers[i]);
}
}
return users;
}
// After refactoring
function getUsers(activeOnly = false) {
return allUsers.filter(user => !activeOnly || user.status === 'active');
}
Integration Challenges
Professional development often involves integrating different systems:
- Combine multiple APIs into a cohesive application
- Integrate a third party library into an existing project
- Connect a frontend to a backend you didn’t write
These exercises develop your ability to work with code and systems beyond your control.
Performance Optimization
Optimizing code is a valuable professional skill:
- Profile an application to identify performance bottlenecks
- Optimize database queries in an existing application
- Reduce the bundle size of a web application
- Improve rendering performance in a UI
These exercises develop the analytical skills needed to improve application performance.
Showcasing Real Skills on GitHub
While GitHub has limitations in representing your full abilities, you can use it more effectively to showcase real skills.
Quality Documentation
Well documented repositories signal professional approach:
- Create comprehensive READMEs with setup instructions, screenshots, and usage examples
- Document architectural decisions and their rationales
- Include comments explaining complex code sections
- Maintain change logs for significant updates
Good documentation demonstrates communication skills and consideration for other developers.
Demonstrated Testing Practices
Including tests in your repositories shows professional rigor:
- Implement unit tests for critical functionality
- Add integration tests for component interactions
- Set up continuous integration to run tests automatically
- Track and display test coverage
Testing demonstrates your commitment to code quality and reliability.
Project Evolution Through Commits
Your commit history can showcase your development process:
- Use meaningful commit messages that explain why changes were made
- Structure commits to show logical progression
- Use pull requests to document feature additions or major changes
- Show refactoring separate from feature additions
This approach gives viewers insight into your thought process and work methodology.
Case Studies and Problem Statements
Add context to your projects to demonstrate problem solving:
- Explain the problem each project addresses
- Describe alternative approaches you considered
- Document challenges encountered and how you overcame them
- Include performance or user metrics if available
This context helps others understand the depth of your work beyond just the code.
The Continuous Learning Mindset
Perhaps the most important skill for long term success is adopting a continuous learning mindset.
Embracing Feedback
Treat feedback as a gift rather than criticism:
- Actively seek code reviews from more experienced developers
- Ask specific questions about how to improve
- Implement suggestions and learn from them
- Track your progress over time
This approach accelerates growth and builds resilience.
Learning from Failure
Failed projects and mistakes often teach more than successes:
- Analyze what went wrong in unsuccessful projects
- Document lessons learned from failures
- Apply those lessons to future work
- Share your experiences to help others
This reflection converts setbacks into valuable experience.
Deliberate Practice
Not all coding practice is equally valuable:
- Identify specific skills you need to improve
- Design practice exercises that target those skills
- Seek feedback on your progress
- Gradually increase difficulty as you improve
This focused approach builds skills more efficiently than unfocused coding.
The Reality Check: Assessing Your True Skill Level
Accurately gauging your abilities is essential for improvement. Here are ways to reality check your skills:
Technical Interviews
Even if you’re not job hunting, interview practice provides valuable feedback:
- Participate in mock technical interviews
- Take coding assessments on platforms like HackerRank or CodeSignal
- Analyze where you struggle and target those areas for improvement
Interviews quickly reveal gaps between perceived and actual abilities.
Peer Programming and Code Reviews
Working directly with other developers provides immediate feedback:
- Participate in pair programming sessions
- Join coding communities that offer code reviews
- Contribute to open source and receive maintainer feedback
These interactions highlight both strengths and improvement areas.
Building Without Tutorials
Test your independent abilities:
- Set a goal to build something without following tutorials
- Use documentation rather than step by step guides
- Solve problems by thinking through solutions rather than searching for answers
This approach reveals your true understanding beyond tutorial repetition.
Conclusion: Beyond the Green Squares
An impressive GitHub profile with numerous repositories and frequent contributions can look good at first glance, but real programming proficiency goes much deeper. True skill development requires deliberate practice, comprehensive projects, collaboration experience, and a willingness to tackle challenging problems.
Remember that many crucial development skills aren’t immediately visible on GitHub: problem solving methodology, communication abilities, performance optimization knowledge, and the capacity to work within constraints. These skills must be deliberately cultivated alongside your visible code contributions.
As you continue your programming journey, focus on depth over breadth, seek meaningful feedback, and challenge yourself with projects that stretch your abilities. Contribute to open source, practice algorithmic thinking, and develop the soft skills that complement your technical knowledge.
By taking this comprehensive approach to skill development, you’ll build real world programming abilities that translate to success in professional environments and technical interviews—going far beyond what your GitHub profile alone might suggest.
The path to becoming a truly skilled developer isn’t measured in green squares, but in the depth of understanding, quality of code, and ability to solve complex problems effectively. Focus on these fundamentals, and both your GitHub profile and your practical skills will reflect genuine expertise.