Why Your First Approach Is Wrong: Learning the Value of Iteration in Coding Problems
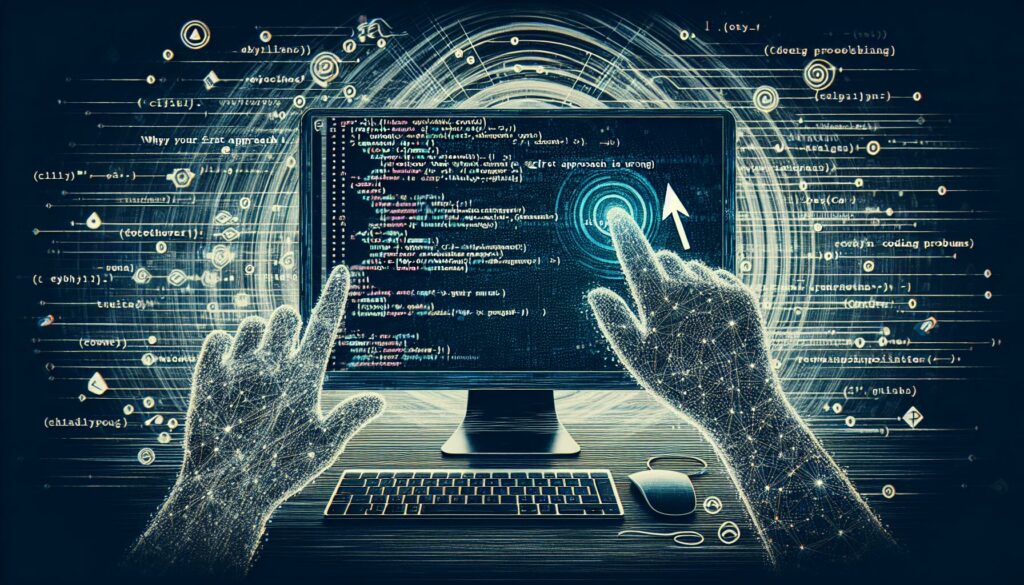
In the world of coding and software development, there’s a common misconception that great programmers come up with perfect solutions on their first try. This couldn’t be further from the truth. In fact, the most successful problem-solvers in the programming world understand and embrace the value of iteration. They know that the path to an optimal solution often begins with a less-than-perfect first attempt. In this article, we’ll explore why your first approach to a coding problem is likely wrong, and why that’s not only okay but an essential part of the learning and problem-solving process.
The Myth of the Perfect First Solution
When faced with a coding problem, especially in high-pressure situations like technical interviews or coding challenges, there’s often an urge to come up with the most efficient, elegant solution right off the bat. This pressure can be paralyzing, leading to analysis paralysis or, worse, giving up before even starting.
However, the reality is that even experienced programmers rarely, if ever, arrive at the optimal solution on their first attempt. The journey from problem to solution is usually an iterative process, involving multiple steps of refinement and optimization.
The Power of the Brute Force Approach
One of the most valuable lessons in problem-solving is understanding the importance of the brute force approach. A brute force solution, while often inefficient, serves several crucial purposes:
- It helps you understand the problem better: By implementing a straightforward solution, you gain insights into the problem’s nuances and edge cases.
- It provides a baseline: A brute force solution gives you a working implementation to compare against as you optimize.
- It builds confidence: Successfully implementing any solution, even if inefficient, boosts your confidence and motivation to improve it.
- It’s a starting point for optimization: Many optimized solutions are born from refining and improving brute force approaches.
The Iterative Process of Problem-Solving
Successful problem-solvers in the coding world follow an iterative process that typically looks something like this:
- Understand the problem thoroughly
- Brainstorm potential approaches
- Implement a brute force solution
- Analyze the brute force solution for inefficiencies
- Brainstorm optimizations
- Implement and test improvements
- Repeat steps 4-6 until satisfied with the solution
This process emphasizes continuous improvement and learning. Each iteration brings you closer to a more efficient and elegant solution.
Case Study: The Two Sum Problem
Let’s illustrate this iterative process with a common coding problem: the Two Sum problem. The problem statement is as follows:
Given an array of integers
nums
and an integertarget
, return indices of the two numbers in the array such that they add up totarget
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Step 1: Brute Force Approach
A typical first approach might look like this:
def two_sum(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
This solution works, but it has a time complexity of O(n^2), which is inefficient for large inputs.
Step 2: Analyze and Optimize
After implementing the brute force solution, we can analyze its inefficiencies. The main issue is that we’re doing repeated work by checking every pair of numbers.
Step 3: Implement an Improved Solution
A more efficient approach uses a hash table:
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
This solution has a time complexity of O(n), a significant improvement over the brute force approach.
The Importance of Time and Space Complexity
As we iterate on our solutions, it’s crucial to consider both time and space complexity. These metrics help us understand how our algorithm’s performance scales with input size.
- Time Complexity: Measures how the running time of an algorithm increases with input size.
- Space Complexity: Measures how much additional memory an algorithm uses as input size grows.
In our Two Sum example, we traded space complexity for time complexity. The brute force approach used O(1) extra space but had O(n^2) time complexity. The optimized solution uses O(n) extra space but achieves O(n) time complexity.
Common Optimization Techniques
As you iterate on your solutions, several common optimization techniques can help improve efficiency:
- Hash Tables: Often used to trade space for time, as in our Two Sum example.
- Two Pointers: Useful for problems involving sorted arrays or linked lists.
- Sliding Window: Efficient for problems involving subarrays or substrings.
- Dynamic Programming: Breaks complex problems into simpler subproblems and stores results for reuse.
- Divide and Conquer: Splits the problem into smaller parts, solves them independently, and combines the results.
The Role of Practice and Experience
Recognizing optimization opportunities and applying appropriate techniques comes with practice and experience. As you solve more problems and iterate on your solutions, you’ll start to recognize patterns and develop intuition for efficient approaches.
Platforms like AlgoCademy provide an excellent environment for this kind of practice. With interactive coding tutorials, AI-powered assistance, and a focus on algorithmic thinking, AlgoCademy helps learners progress from basic coding skills to tackling complex problems efficiently.
Embracing the Iterative Process in Technical Interviews
Understanding and embracing the iterative nature of problem-solving is particularly crucial in technical interviews, especially for major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google).
In these interviews, the process of arriving at a solution is often as important as the solution itself. Interviewers want to see how you think, how you approach problems, and how you improve your solutions. Here’s how to leverage the iterative process in an interview setting:
- Start with a brute force solution: Don’t be afraid to propose a simple, inefficient solution to start. This shows you can quickly come up with a working solution.
- Analyze and communicate: Discuss the time and space complexity of your initial solution. This demonstrates your understanding of algorithmic efficiency.
- Propose improvements: Suggest ways to optimize your solution. Even if you’re not sure how to implement them yet, this shows your problem-solving skills and your drive for efficiency.
- Iterate and implement: Work on implementing your proposed improvements. This showcases your ability to translate ideas into code.
- Continuously communicate: Throughout the process, explain your thought process. This gives the interviewer insight into how you approach problems and how you might work in a team setting.
Learning from Mistakes and Suboptimal Solutions
It’s important to remember that mistakes and suboptimal solutions are not failures – they’re opportunities for learning and improvement. Every time you realize why a solution isn’t optimal, you’re gaining valuable knowledge that will help you in future problem-solving endeavors.
Consider keeping a “mistake journal” where you document the problems you’ve solved, your initial approaches, and how you improved them. This can be an invaluable resource for reinforcing your learning and tracking your progress over time.
The Importance of Code Refactoring
Iteration in coding doesn’t stop at finding a working solution. Code refactoring – the process of restructuring existing code without changing its external behavior – is another crucial aspect of the iterative process. Refactoring helps improve code readability, reduces complexity, and can often lead to performance improvements.
Here are some common refactoring techniques:
- Extracting Methods: Breaking down large methods into smaller, more manageable pieces.
- Renaming Variables: Using clear, descriptive names for variables and functions.
- Eliminating Duplicate Code: Identifying and removing repeated code patterns.
- Simplifying Conditional Expressions: Making complex if-else statements more readable and maintainable.
Regular refactoring not only improves your current code but also enhances your overall coding skills and ability to write clean, efficient code from the start.
Leveraging Tools and Resources
As you embrace the iterative process in your coding journey, don’t hesitate to leverage available tools and resources. These can significantly enhance your learning experience and help you iterate more effectively:
- Version Control Systems: Tools like Git allow you to track changes in your code over time, making it easier to experiment with different approaches and revert if necessary.
- Code Profilers: These tools help identify performance bottlenecks in your code, guiding your optimization efforts.
- Online Coding Platforms: Websites like AlgoCademy, LeetCode, and HackerRank provide a wealth of coding problems and allow you to compare your solutions with others.
- AI-powered Coding Assistants: Tools like GitHub Copilot or AlgoCademy’s AI assistant can provide suggestions and help you explore different approaches to problems.
- Programming Communities: Engaging with communities on platforms like Stack Overflow or Reddit’s programming subreddits can expose you to different perspectives and approaches.
Conclusion: Embracing Iteration as a Path to Mastery
In the world of coding and problem-solving, your first approach is often wrong – and that’s perfectly okay. What matters is your ability to recognize areas for improvement and your willingness to iterate and optimize your solutions.
Remember, even the most complex and efficient algorithms often start as simple, brute-force solutions. The path from a naive approach to an optimized solution is where the real learning happens. It’s in this journey that you develop the critical thinking skills, pattern recognition, and deep understanding that characterize expert programmers.
So the next time you face a coding challenge, don’t be discouraged if your first attempt isn’t perfect. Instead, embrace it as the first step in an iterative process that will ultimately lead you to a better solution and, more importantly, to becoming a better programmer.
Keep practicing, keep iterating, and remember – in coding, as in life, progress is often more important than perfection. Happy coding!