Why Your Feature Flags Are Making Code Maintenance Harder
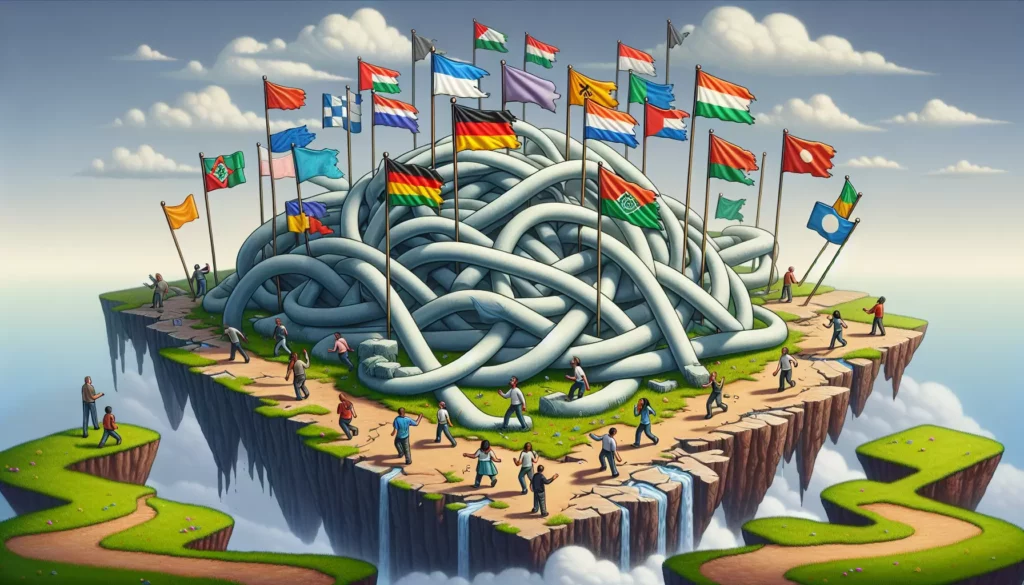
Feature flags have become a staple in modern software development, allowing teams to deploy code to production while controlling its visibility to users. While they offer tremendous benefits for continuous delivery and experimentation, poorly implemented feature flags can silently transform into a maintenance nightmare. This article explores why your feature flags might be making code maintenance harder and provides practical solutions to avoid these pitfalls.
What Are Feature Flags?
Feature flags (also known as feature toggles or feature switches) are a software development technique that allows developers to enable or disable functionality without deploying new code. At its core, a feature flag is a conditional statement that determines whether a particular feature is available to users:
if (featureFlags.isEnabled("new_recommendation_algorithm")) {
// New algorithm code
} else {
// Old algorithm code
}
Feature flags serve multiple purposes in modern development workflows:
- Continuous delivery: Deploy incomplete features behind flags
- A/B testing: Show different variants to different user segments
- Canary releases: Gradually roll out features to increasing percentages of users
- Kill switches: Quickly disable problematic features without a new deployment
- Subscription-based features: Enable functionality based on user plans
When implemented correctly, feature flags empower development teams to move quickly and safely. However, without proper management, they can introduce significant technical debt and make your codebase increasingly difficult to maintain.
The Hidden Costs of Feature Flags
1. Exponential Complexity in Testing
Each feature flag effectively doubles the number of code paths that need testing. With just 10 independent feature flags, you theoretically have 2^10 = 1,024 possible combinations of enabled and disabled features. This combinatorial explosion makes comprehensive testing nearly impossible.
Consider this example where multiple feature flags interact:
if (featureFlags.isEnabled("new_ui")) {
// New UI code
if (featureFlags.isEnabled("advanced_filtering")) {
// Advanced filtering with new UI
} else {
// Basic filtering with new UI
}
} else {
// Old UI code
if (featureFlags.isEnabled("advanced_filtering")) {
// Advanced filtering with old UI
} else {
// Basic filtering with old UI
}
}
Each additional nested flag multiplies the complexity, making it easy for bugs to hide in rarely tested combinations.
2. Stale Flags and Dead Code
Once a feature flag has served its purpose (the feature is fully rolled out or the experiment is complete), it should be removed. However, in fast paced development environments, flag cleanup often falls by the wayside. The result is codebases littered with toggles that are permanently set to one position, creating dead code paths that are never executed but must still be maintained.
For example, consider a feature flag that was used for a one time migration:
if (featureFlags.isEnabled("data_migration_2021")) {
// Code to handle the 2021 data migration
} else {
// Original code path
}
Once the migration is complete, this flag may remain in the “on” position indefinitely, making the “else” branch dead code. Future developers will waste time understanding both branches, even though only one is ever used.
3. Unclear Feature Boundaries
As features evolve, flags can spread throughout the codebase, making it difficult to understand the full scope of what a single flag controls. This diffusion makes removing flags much more challenging, as developers must hunt down all instances before they can safely eliminate the toggle.
Consider a feature flag that started in one component but gradually spread:
// UserProfileComponent.js
if (featureFlags.isEnabled("enhanced_profiles")) {
renderEnhancedProfile();
} else {
renderBasicProfile();
}
// UserSettingsComponent.js
if (featureFlags.isEnabled("enhanced_profiles")) {
showEnhancedSettingsOptions();
}
// NotificationService.js
if (featureFlags.isEnabled("enhanced_profiles")) {
sendEnhancedNotifications();
}
// And so on across multiple files...
The scattered nature of this flag makes it extremely difficult to understand its full impact and even harder to remove cleanly.
4. Increased Cognitive Load
Every feature flag adds conditional logic that developers must parse when reading the code. This additional cognitive load slows down comprehension and makes the codebase harder to navigate, especially for new team members.
Feature flag checks scattered throughout the code create mental context switches as developers must constantly evaluate: “What happens if this flag is on? What happens if it’s off? When would each state occur?”
5. Flag Configuration Drift
As environments multiply (development, testing, staging, production), keeping feature flag configurations consistent becomes challenging. A feature might work perfectly in testing with one flag configuration but fail in production with another.
This problem compounds when flags are managed through different mechanisms in different environments, such as environment variables in development but a database or remote configuration service in production.
Best Practices for Sustainable Feature Flagging
Feature flags don’t have to be a maintenance burden. By adopting these best practices, you can enjoy the benefits of feature flags while minimizing their negative impact on code maintainability.
1. Categorize Your Flags
Not all feature flags are created equal. By categorizing your flags based on their purpose and expected lifespan, you can manage them more effectively:
- Release flags: Short lived toggles used to enable incomplete features in production
- Experiment flags: Medium term toggles for A/B testing
- Operational flags: Long lived toggles that control system behavior (kill switches, performance controls)
- Permission flags: Permanent toggles that enable features based on user permissions or subscription level
This categorization helps set expectations about how long each flag should live and guides cleanup efforts.
2. Implement Flag Expiration Dates
For temporary flags, build in expiration dates or review triggers. This could be as simple as a comment indicating when the flag should be removed:
// TODO: Remove this flag after the Q2 2023 release
if (featureFlags.isEnabled("new_checkout_flow")) {
// New checkout implementation
} else {
// Old checkout implementation
}
Better yet, some feature flag management systems allow you to set actual expiration dates that will trigger notifications when a flag should be reviewed for removal.
3. Centralize Flag Logic
Instead of sprinkling feature flag checks throughout your codebase, centralize the conditional logic in as few places as possible. This approach makes it much easier to understand the scope of each flag and simplifies eventual cleanup.
For example, instead of this:
// Scattered across multiple components
if (featureFlags.isEnabled("new_ui")) {
// Component A with new UI
} else {
// Component A with old UI
}
// In another file
if (featureFlags.isEnabled("new_ui")) {
// Component B with new UI
} else {
// Component B with old UI
}
Consider this approach:
// In a single factory or provider
function getUIComponents() {
if (featureFlags.isEnabled("new_ui")) {
return {
componentA: NewComponentA,
componentB: NewComponentB,
// etc.
};
} else {
return {
componentA: OldComponentA,
componentB: OldComponentB,
// etc.
};
}
}
This centralization creates a clear boundary for the feature flag’s influence and makes it much easier to remove when the time comes.
4. Document Your Flags
Maintain a centralized inventory of all active feature flags, including:
- The flag’s purpose
- Who owns it
- When it was created
- When it should be removed
- Which code areas it affects
This documentation makes feature flags discoverable and helps prevent them from being forgotten.
5. Regularly Audit and Clean Up
Schedule regular “flag cleaning days” where the team reviews all active flags and removes those that are no longer needed. This could be part of your sprint cycle or tied to major releases.
Some teams use metrics to identify flags that should be removed, such as flags that have been 100% on or 100% off for an extended period.
6. Use Feature Flag Management Systems
As your use of feature flags grows, consider investing in a dedicated feature flag management system like LaunchDarkly, Split.io, or Optimizely. These platforms provide:
- Centralized management of flags across environments
- Detailed targeting and gradual rollout capabilities
- Usage metrics to identify stale flags
- Audit logs of flag changes
- APIs and SDKs for consistent implementation
These tools can significantly reduce the maintenance burden of feature flags, especially in larger organizations.
7. Implement Technical Gates
Technical gates can help enforce good feature flag hygiene. For example:
- Require an expiration date for new feature flags
- Set up linting rules to detect certain types of flag anti patterns
- Add warnings for nested feature flag conditions
- Create CI checks that fail if too many flags are active
These gates create friction that encourages teams to think carefully about their feature flag strategy.
Architectural Approaches to Feature Flagging
Beyond individual best practices, certain architectural approaches can make feature flags more maintainable:
1. The Strangler Pattern
When replacing a major component, use the strangler pattern to gradually transition traffic from the old implementation to the new one using a feature flag:
function processOrder(order) {
if (featureFlags.isEnabled("new_order_processing", order.context)) {
return newOrderProcessor.process(order);
} else {
return legacyOrderProcessor.process(order);
}
}
This pattern keeps the feature flag at the entry point to the component, rather than scattered throughout the implementation details. Once the transition is complete, you can remove the conditional and the old implementation in one clean step.
2. The Strategy Pattern
Use the strategy pattern to encapsulate different implementations behind a common interface:
// Factory that provides the appropriate strategy based on feature flags
function getRecommendationStrategy(context) {
if (featureFlags.isEnabled("ml_recommendations", context)) {
return new MachineLearningRecommendationStrategy();
} else {
return new RuleBasedRecommendationStrategy();
}
}
// Consumer code just uses the strategy without knowing about the flag
const recommendationStrategy = getRecommendationStrategy(userContext);
const recommendations = recommendationStrategy.getRecommendationsForUser(userId);
This approach isolates the feature flag logic to a single decision point and keeps the rest of the codebase clean.
3. Dependency Injection
Use dependency injection to provide the appropriate implementation based on feature flags:
class UserService {
constructor(userRepository) {
this.userRepository = userRepository;
}
// Methods that use this.userRepository
}
// At application startup or in a factory
function createUserService(context) {
let userRepository;
if (featureFlags.isEnabled("new_user_storage", context)) {
userRepository = new CloudUserRepository();
} else {
userRepository = new SQLUserRepository();
}
return new UserService(userRepository);
}
This approach keeps feature flag logic out of the core application code, making it easier to maintain and eventually remove.
4. Branch by Abstraction
For larger refactorings, use the branch by abstraction pattern:
- Create an abstraction layer over the component you want to replace
- Ensure all code uses this abstraction
- Create a new implementation of the abstraction
- Use a feature flag to switch between implementations
- Once the new implementation is fully rolled out, remove the old one
- Optionally, remove the abstraction if it’s no longer needed
This approach allows for major changes while maintaining a clean architecture and clear feature boundaries.
Managing Feature Flags in Different Programming Paradigms
Object Oriented Programming
In OOP languages, inheritance and polymorphism can help manage feature flags:
// Base implementation
class PaymentProcessor {
process(payment) {
// Common logic
}
}
// New implementation
class EnhancedPaymentProcessor extends PaymentProcessor {
process(payment) {
// Enhanced logic
}
}
// Factory that uses feature flags
class PaymentProcessorFactory {
static create(context) {
if (featureFlags.isEnabled("enhanced_payments", context)) {
return new EnhancedPaymentProcessor();
} else {
return new PaymentProcessor();
}
}
}
This approach keeps the feature flag logic isolated to the factory class.
Functional Programming
In functional programming, higher order functions can help manage feature flags:
// Define both implementations
const standardSearch = (query) => {
// Standard search implementation
};
const advancedSearch = (query) => {
// Advanced search implementation
};
// Higher order function that selects the implementation
const createSearchFunction = (context) => {
return featureFlags.isEnabled("advanced_search", context)
? advancedSearch
: standardSearch;
};
// Usage
const search = createSearchFunction(userContext);
const results = search(query);
This functional approach keeps the core logic clean while isolating the feature flag decision.
Frontend Applications
In frontend applications, feature flags often control UI components. React’s component composition model works well with feature flags:
// Feature flag wrapper component
function FeatureFlag({ flagName, fallback, children }) {
const enabled = useFeatureFlag(flagName);
return enabled ? children : fallback;
}
// Usage
function UserProfile() {
return (
<div>
<FeatureFlag
flagName="enhanced_profile"
fallback={<BasicProfileView />}
>
<EnhancedProfileView />
</FeatureFlag>
</div>
);
}
This approach makes feature flags explicit in the component hierarchy and centralizes the flag checking logic.
Real World Feature Flag Horror Stories
To illustrate the potential pitfalls of feature flags, here are some anonymized real world horror stories:
The Forgotten Flag
A team implemented a feature flag for a major algorithm change. The flag was set to 50% for an A/B test, and after six months, they determined the new algorithm was better. However, they forgot to remove the flag or set it to 100%. Two years later, they discovered that half their users were still using the old algorithm. By this time, the original developers had left, and nobody fully understood the differences between the implementations.
The Nested Nightmare
A company had implemented feature flags for nearly every feature, resulting in deeply nested conditional logic:
if (featureFlags.isEnabled("new_ui")) {
if (featureFlags.isEnabled("new_checkout")) {
if (featureFlags.isEnabled("payment_options_v2")) {
// Implementation A
} else {
// Implementation B
}
} else {
if (featureFlags.isEnabled("payment_options_v2")) {
// Implementation C
} else {
// Implementation D
}
}
} else {
// Similar nesting for old UI...
}
When a critical bug appeared in production, it took days to reproduce because it only occurred with a specific combination of flag states that was difficult to identify.
The Configuration Drift
A team used feature flags to control access to a new API. In development and staging, the flags were stored in environment variables, but in production, they were stored in a database. During a deployment, the database configuration wasn’t updated, but the code expecting the new API was deployed. The result was a major production outage that took hours to diagnose because the team didn’t immediately recognize it as a feature flag issue.
The Performance Penalty
A company implemented hundreds of feature flags without considering the performance implications. Each page load required multiple network requests to a feature flag service to evaluate all the relevant flags. This added significant latency to the application, especially for users with slower connections. The solution required a major refactoring to batch flag evaluations and implement caching.
Measuring Feature Flag Health
To maintain a healthy feature flag system, consider tracking these metrics:
1. Flag Count
Track the total number of active feature flags. A steadily increasing count may indicate flags aren’t being removed appropriately.
2. Flag Age
Monitor how long each flag has been in place. Temporary flags (release toggles, experiment flags) should have a relatively short lifespan, typically less than 90 days.
3. Toggle Frequency
How often is each flag’s state changing? Flags that haven’t changed state in months may be candidates for removal.
4. Flag Distribution
Categorize your flags by type and track the distribution. If release flags dominate your codebase, you may need to focus on flag cleanup.
5. Flag Complexity
Measure how many code paths are affected by each flag. Flags that touch many parts of the codebase are riskier and harder to maintain.
6. Flag Evaluation Performance
Track the performance impact of your feature flag system, including evaluation time and any network requests required.
When to Avoid Feature Flags
While feature flags are powerful, they’re not always the right solution:
1. For Simple Changes
For small, low risk changes, the overhead of a feature flag may not be justified.
2. For Database Schema Changes
Feature flags work best for code changes, not database schema changes. Schema migrations usually require a different approach.
3. When Clean Removal Is Unlikely
If you know your team has a poor track record of removing temporary flags, consider alternative approaches.
4. For Highly Critical Systems
In systems where reliability is paramount, the additional complexity and potential for errors introduced by feature flags may not be acceptable.
Alternative Approaches
Feature flags aren’t the only way to manage feature rollouts:
1. Blue Green Deployments
Deploy the new version to a separate environment and switch traffic over when ready. This approach keeps the codebase clean but requires more infrastructure.
2. Canary Deployments
Deploy the new version to a small subset of servers and gradually increase the deployment as confidence grows.
3. Separate Services
Instead of using feature flags to toggle between implementations, deploy the new implementation as a separate service and gradually migrate traffic.
4. Branch by Abstraction Without Flags
Use the branch by abstraction pattern but control the implementation choice through configuration rather than runtime feature flags.
Conclusion
Feature flags are a powerful tool in modern software development, enabling continuous delivery, experimentation, and operational safety. However, they come with significant maintenance costs that can accumulate over time if not properly managed.
By following best practices for feature flag implementation, establishing clear processes for flag management, and choosing appropriate architectural patterns, you can minimize the negative impact of feature flags on code maintainability.
Remember that feature flags are a means to an end, not an end in themselves. The goal is to deliver value to users quickly and safely, not to accumulate an ever growing collection of toggles. With disciplined management and regular cleanup, feature flags can be a net positive for both your development process and your codebase.
The next time you reach for a feature flag, take a moment to consider its full lifecycle. How will it be implemented? How will it be tested? And most importantly, how and when will it be removed? By thinking through these questions, you’ll be well on your way to a more maintainable feature flagging strategy.