Why Your Favorite Data Structure is Actually Your Spirit Animal
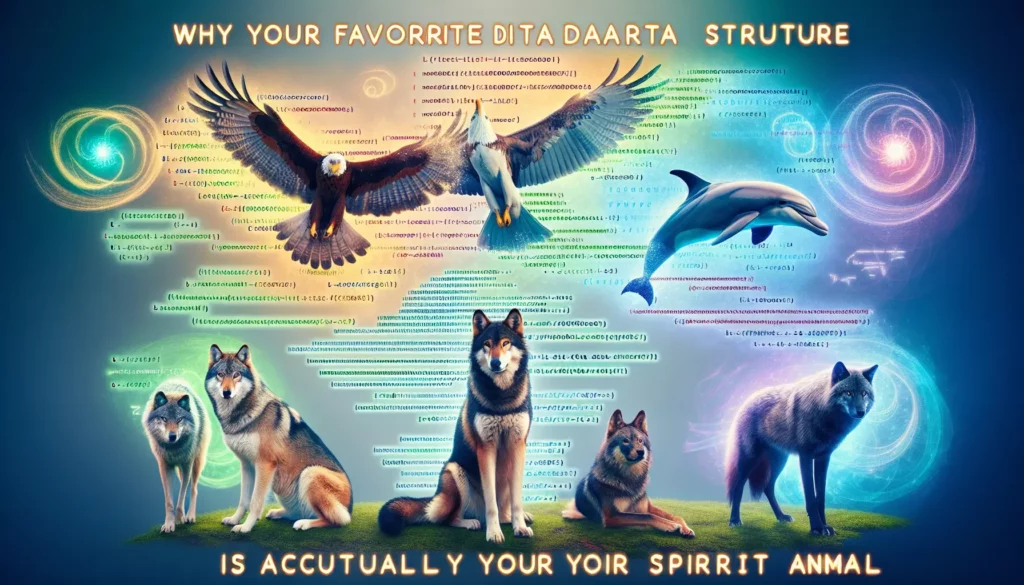
In the vast wilderness of computer science, where algorithms roam free and code flows like rivers, there’s a peculiar phenomenon that every programmer experiences but rarely acknowledges: the deep, spiritual connection between coders and their favorite data structures. Yes, you read that right. Your go-to data structure isn’t just a tool in your coding toolkit; it’s your digital spirit animal, guiding you through the treacherous terrain of software development.
Welcome to AlgoCademy’s whimsical journey through the forest of data structures, where we’ll explore how these fundamental components of programming mirror our personalities, problem-solving approaches, and even our quirks. Whether you’re a seasoned developer or just starting your coding adventure, prepare to see your beloved data structures in a whole new light.
The Array: The Steadfast Buffalo
Let’s start with the array, the sturdy buffalo of data structures. Like these majestic beasts of the plains, arrays are robust, reliable, and have been around since the dawn of programming time. If the array is your spirit animal, you’re likely someone who appreciates order, efficiency, and direct access to your resources.
Arrays are simple yet powerful, much like the buffalo that can weather harsh conditions and still thrive. As an “Array Whisperer,” you probably enjoy:
- Quick, constant-time access to elements
- The satisfaction of neatly organized data
- The versatility to handle various programming challenges
However, like the buffalo, arrays can be a bit inflexible when it comes to changing their size. If you find yourself constantly declaring new arrays or copying data to larger arrays, it might be time to explore more dynamic pastures.
Code Example: The Array in Action
Here’s a simple demonstration of our sturdy array in Python:
# Creating and using an array in Python
buffalo_herd = ["Bison", "American Buffalo", "Plains Bison", "Wood Bison"]
# Accessing elements
print(f"The leader of the herd is: {buffalo_herd[0]}")
# Adding a new buffalo (requires creating a new array)
buffalo_herd = buffalo_herd + ["European Bison"]
print(f"The herd now consists of: {', '.join(buffalo_herd)}")
The Linked List: The Curious Squirrel
If arrays are buffalos, then linked lists are surely the squirrels of the data structure world. Agile, adaptable, and always ready to leap to the next node, linked lists embody the spirit of exploration and flexibility. Those who resonate with linked lists often have a knack for connecting ideas and navigating complex relationships.
As a “Linked List Lover,” you likely appreciate:
- The ease of inserting and deleting elements
- The dynamic nature of memory allocation
- The challenge of traversing through data, one hop at a time
However, like a squirrel searching for a specific acorn, you might find yourself frustrated by the lack of random access. If you’re constantly iterating through your entire list to find a single element, it might be time to consider a more direct approach.
Code Example: The Linked List in Action
Let’s implement a simple linked list in Python to showcase its squirrel-like agility:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def display(self):
elements = []
current = self.head
while current:
elements.append(current.data)
current = current.next
return ' -> '.join(map(str, elements))
# Creating our squirrel-like linked list
acorn_stash = LinkedList()
acorn_stash.append("Oak Acorn")
acorn_stash.append("Beech Nut")
acorn_stash.append("Hazelnut")
print(f"Squirrel's stash: {acorn_stash.display()}")
The Stack: The Industrious Woodpecker
Moving on to the stack, we find ourselves face to face with the woodpecker of data structures. Like these diligent birds that methodically peck away at trees, stacks operate on a Last-In-First-Out (LIFO) principle, efficiently managing data in a very specific order.
If the stack is your spirit animal, you’re likely someone who:
- Enjoys solving problems in a structured, step-by-step manner
- Appreciates the elegance of recursive solutions
- Has a knack for backtracking and undo operations
However, like a woodpecker that can only access the outermost layer of a tree, stacks have limited access to their elements. If you find yourself constantly popping and pushing just to get to a specific piece of data, you might need to reconsider your approach.
Code Example: The Stack in Action
Let’s implement a simple stack to demonstrate its woodpecker-like precision:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Creating our woodpecker-like stack
tree_layers = Stack()
tree_layers.push("Bark")
tree_layers.push("Cambium")
tree_layers.push("Sapwood")
tree_layers.push("Heartwood")
print(f"The woodpecker is currently pecking at: {tree_layers.peek()}")
print(f"Layers pecked: {tree_layers.size()}")
while not tree_layers.is_empty():
print(f"Pecked through: {tree_layers.pop()}")
The Queue: The Patient Sloth
In the world of data structures, the queue embodies the spirit of the sloth. Slow and steady, operating on a First-In-First-Out (FIFO) principle, queues are the epitome of patience and orderly processing. If you find yourself drawn to queues, you’re likely someone who values fairness and methodical progression.
As a “Queue Enthusiast,” you probably appreciate:
- The inherent fairness of processing data in the order it arrives
- The ability to manage tasks or data in a predictable sequence
- The simplicity of enqueue and dequeue operations
However, like a sloth that can’t quickly jump to a different branch, queues don’t offer random access to their elements. If you’re frequently searching for specific items in your queue, you might need to consider a more flexible structure.
Code Example: The Queue in Action
Let’s implement a simple queue to showcase its sloth-like patience:
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
def front(self):
if not self.is_empty():
return self.items[0]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Creating our sloth-like queue
leaf_buffet = Queue()
leaf_buffet.enqueue("Cecropia")
leaf_buffet.enqueue("Guarumo")
leaf_buffet.enqueue("Ficus")
leaf_buffet.enqueue("Cacao")
print(f"The sloth will eat first: {leaf_buffet.front()}")
print(f"Leaves in the queue: {leaf_buffet.size()}")
while not leaf_buffet.is_empty():
print(f"Nom nom, eating: {leaf_buffet.dequeue()}")
The Hash Table: The Clever Octopus
Now, let’s dive into the depths of the hash table, the octopus of data structures. With its ability to quickly retrieve information using keys, the hash table mirrors the octopus’s remarkable intelligence and adaptability. If hash tables resonate with you, you’re likely someone who values efficiency and quick problem-solving.
As a “Hash Table Aficionado,” you probably enjoy:
- The near-constant time complexity for insertions and lookups
- The flexibility to store and retrieve data using meaningful keys
- The challenge of designing good hash functions
However, like an octopus that might occasionally get its tentacles tangled, hash tables can suffer from collisions. If you find yourself constantly dealing with collision resolution, it might be time to reassess your hashing strategy or consider alternative structures for your specific use case.
Code Example: The Hash Table in Action
Let’s implement a simple hash table to demonstrate its octopus-like cleverness:
class HashTable:
def __init__(self, size=10):
self.size = size
self.table = [[] for _ in range(self.size)]
def _hash(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
item[1] = value
return
self.table[index].append([key, value])
def get(self, key):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
return item[1]
raise KeyError(key)
def remove(self, key):
index = self._hash(key)
for i, item in enumerate(self.table[index]):
if item[0] == key:
del self.table[index][i]
return
raise KeyError(key)
# Creating our octopus-like hash table
octopus_facts = HashTable()
octopus_facts.insert("arms", 8)
octopus_facts.insert("hearts", 3)
octopus_facts.insert("blue_blood", True)
octopus_facts.insert("intelligence", "High")
print(f"Number of octopus arms: {octopus_facts.get('arms')}")
print(f"Octopus intelligence level: {octopus_facts.get('intelligence')}")
octopus_facts.remove("blue_blood")
try:
print(octopus_facts.get("blue_blood"))
except KeyError:
print("Fact about blue blood has been removed!")
The Tree: The Wise Owl
Perched atop the hierarchy of data structures, we find the tree, the wise owl of our digital forest. With its branching structure and ability to represent hierarchical relationships, the tree embodies the owl’s wisdom and far-reaching vision. If you’re drawn to trees, you likely have a knack for seeing the big picture and understanding complex relationships.
As a “Tree Hugger” (in the coding sense), you probably appreciate:
- The ability to represent hierarchical data naturally
- The efficiency of operations like searching and insertion in balanced trees
- The elegance of recursive algorithms for traversal and manipulation
However, like an owl that might struggle in confined spaces, trees can become unwieldy if not properly balanced. If you find yourself constantly rebalancing your tree or dealing with skewed structures, it might be time to explore self-balancing tree variants or alternative structures.
Code Example: The Tree in Action
Let’s implement a simple binary search tree to showcase its owl-like wisdom:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = TreeNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
def inorder_traversal(self):
result = []
self._inorder_recursive(self.root, result)
return result
def _inorder_recursive(self, node, result):
if node:
self._inorder_recursive(node.left, result)
result.append(node.value)
self._inorder_recursive(node.right, result)
# Creating our owl-like binary search tree
wisdom_tree = BinarySearchTree()
wisdom_tree.insert(5) # Root
wisdom_tree.insert(3) # Left subtree
wisdom_tree.insert(7) # Right subtree
wisdom_tree.insert(2)
wisdom_tree.insert(4)
wisdom_tree.insert(6)
wisdom_tree.insert(8)
print(f"Tree in order: {wisdom_tree.inorder_traversal()}")
print(f"Is 6 in the tree? {'Yes' if wisdom_tree.search(6) else 'No'}")
print(f"Is 9 in the tree? {'Yes' if wisdom_tree.search(9) else 'No'}")
The Graph: The Social Butterfly
Last but certainly not least, we have the graph, the social butterfly of data structures. With its ability to represent complex relationships and connections, the graph mirrors the intricate social networks of butterflies. If graphs are your go-to structure, you’re likely someone who excels at seeing connections and solving network-related problems.
As a “Graph Guru,” you probably enjoy:
- The flexibility to model a wide variety of real-world scenarios
- The challenge of implementing and optimizing graph algorithms
- The satisfaction of solving problems like shortest path and connectivity
However, like a butterfly that might get disoriented in a vast garden, graphs can become computationally expensive as they grow larger and more complex. If you find yourself struggling with performance issues in large graphs, it might be time to explore more specialized graph algorithms or consider alternative representations for your specific use case.
Code Example: The Graph in Action
Let’s implement a simple undirected graph to demonstrate its butterfly-like social nature:
class Graph:
def __init__(self):
self.graph = {}
def add_vertex(self, vertex):
if vertex not in self.graph:
self.graph[vertex] = []
def add_edge(self, vertex1, vertex2):
if vertex1 not in self.graph:
self.add_vertex(vertex1)
if vertex2 not in self.graph:
self.add_vertex(vertex2)
self.graph[vertex1].append(vertex2)
self.graph[vertex2].append(vertex1) # For undirected graph
def remove_edge(self, vertex1, vertex2):
if vertex1 in self.graph and vertex2 in self.graph:
self.graph[vertex1] = [v for v in self.graph[vertex1] if v != vertex2]
self.graph[vertex2] = [v for v in self.graph[vertex2] if v != vertex1]
def get_vertices(self):
return list(self.graph.keys())
def get_edges(self):
edges = []
for vertex in self.graph:
for neighbor in self.graph[vertex]:
if {vertex, neighbor} not in edges:
edges.append({vertex, neighbor})
return edges
def bfs(self, start_vertex):
visited = set()
queue = [start_vertex]
visited.add(start_vertex)
while queue:
vertex = queue.pop(0)
print(vertex, end=" ")
for neighbor in self.graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Creating our butterfly-like social graph
butterfly_network = Graph()
butterfly_network.add_edge("Monarch", "Swallowtail")
butterfly_network.add_edge("Monarch", "Morpho")
butterfly_network.add_edge("Swallowtail", "Painted Lady")
butterfly_network.add_edge("Morpho", "Blue Morpho")
butterfly_network.add_edge("Painted Lady", "Blue Morpho")
print("Butterfly Social Network:")
print(f"Vertices: {butterfly_network.get_vertices()}")
print(f"Edges: {butterfly_network.get_edges()}")
print("\nBFS traversal starting from 'Monarch':")
butterfly_network.bfs("Monarch")
Conclusion: Embracing Your Data Structure Spirit Animal
As we conclude our whimsical journey through the forest of data structures, it’s important to remember that just as no single animal can thrive in every environment, no single data structure is perfect for every situation. The key to becoming a master programmer lies in understanding the strengths and weaknesses of each structure and knowing when to apply them.
Your favorite data structure might indeed be your spirit animal in the coding world, guiding your problem-solving approach and reflecting your programming style. But true mastery comes from being able to adapt and use the right tool for the job, even if it means stepping out of your comfort zone.
At AlgoCademy, we encourage you to explore all these data structures and more. Dive deep into their implementations, experiment with different use cases, and challenge yourself to see problems from multiple perspectives. Remember, the most elegant solutions often come from understanding the nuances of various data structures and algorithms.
So, whether you’re a steadfast buffalo array, a curious squirrel linked list, an industrious woodpecker stack, a patient sloth queue, a clever octopus hash table, a wise owl tree, or a social butterfly graph, embrace your data structure spirit animal. Let it inspire you to write better code, solve complex problems, and continue growing as a programmer.
Happy coding, and may your chosen data structure guide you through the wilderness of software development!