Why Your Error Messages Aren’t Helping Users (And How to Fix Them)
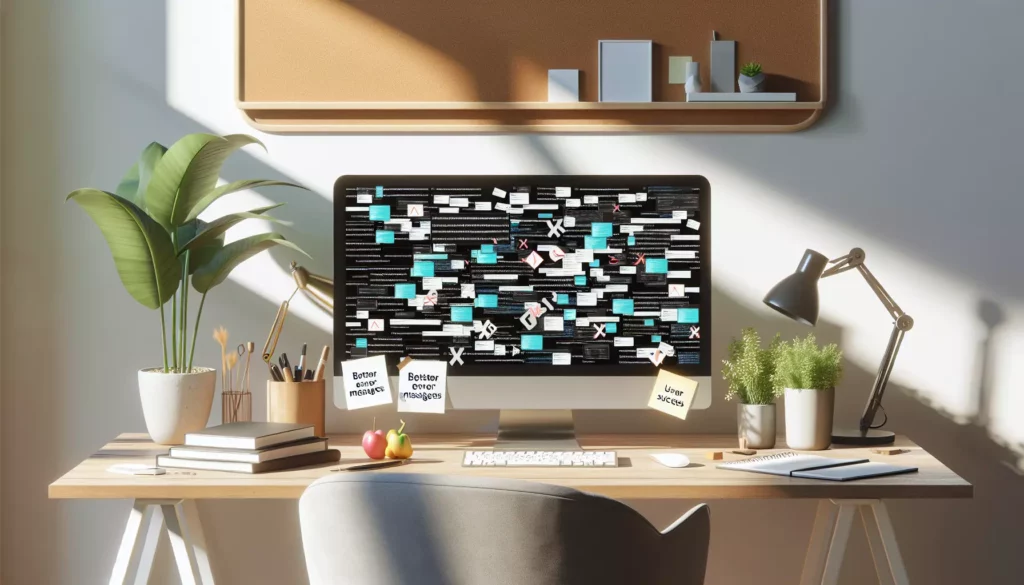
Error messages are a fundamental part of any software application. They serve as critical communication points between your code and your users when something goes wrong. Yet, despite their importance, error messages are often an afterthought in development, leading to confusion, frustration, and ultimately, a poor user experience.
For those learning to code or developing applications, understanding how to craft effective error messages isn’t just about politeness—it’s about creating software that people can actually use. In this comprehensive guide, we’ll explore why many error messages fail to serve their purpose and provide practical strategies to transform them into helpful communication tools.
Table of Contents
- Why Error Messages Matter
- Common Problems with Error Messages
- The Psychology Behind Effective Error Messages
- Core Principles for Writing Helpful Error Messages
- Before and After: Error Message Transformations
- Special Considerations for Technical Users
- Implementation Strategies for Developers
- Testing Your Error Messages
- Conclusion
Why Error Messages Matter
Error messages serve multiple critical functions in applications:
- Problem identification: They alert users that something has gone wrong
- Guidance: They help users understand what happened and why
- Resolution: They provide a path forward to fix the issue
- Education: They teach users about system constraints and expectations
When done right, error messages can transform a potentially frustrating experience into an opportunity for users to learn and succeed. When done poorly, they become barriers that drive users away from your application.
Consider this: According to a study by the Nielsen Norman Group, users who encounter unclear error messages are 79% less likely to retry a task. That’s a significant impact on user retention and satisfaction.
Common Problems with Error Messages
Before we can fix our error messages, we need to understand where they typically go wrong. Here are the most common issues:
1. Technical Jargon Overload
One of the most pervasive problems is error messages filled with technical terminology that makes sense to developers but is meaningless to average users.
Consider this example:
ERROR: Uncaught TypeError: Cannot read property 'value' of undefined
While this makes perfect sense to a JavaScript developer, it’s completely unhelpful to most users who just want to complete their task.
2. Vague and Uninformative Messages
Generic error messages that provide no specific information about what went wrong or how to fix it leave users guessing.
Examples include:
- “An error occurred.”
- “Something went wrong.”
- “Invalid input.”
These messages acknowledge a problem exists but offer no path to resolution.
3. Blaming the User
Error messages that place blame on users create negative experiences and feelings of incompetence.
For example:
- “You entered an invalid email.”
- “You must complete all required fields.”
- “Your password is wrong.”
This accusatory language makes users feel reprimanded rather than helped.
4. Cryptic Error Codes
Numerical error codes without explanation force users to search for meaning elsewhere.
For instance:
Error 0x800704CF
This tells the user absolutely nothing about what happened or how to proceed.
5. Missing Recovery Options
Error messages that identify a problem but don’t suggest any solution leave users stranded.
Consider:
Login failed. Please try again.
This offers no guidance on why the login failed or what to adjust on the next attempt.
6. Inconsistent Tone and Style
Error messages that don’t match the overall tone of your application create a disjointed experience.
For example, a playful application that suddenly produces stern, formal error messages creates cognitive dissonance for users.
The Psychology Behind Effective Error Messages
Understanding the psychological impact of error messages helps us craft more effective communication. When users encounter errors, they typically experience:
Frustration and Confusion
Users often feel blocked from achieving their goals when they encounter errors. This frustration can quickly turn into abandonment if they don’t see a clear path forward.
Attribution and Blame
Users tend to blame themselves when they encounter errors, especially if the message implies user fault. This negative self-attribution can diminish confidence and satisfaction.
Cognitive Load
Deciphering complex error messages adds unnecessary mental burden. When users are already trying to complete a task, adding the job of “error detective” overwhelms them.
The Importance of Control
Providing users with clear next steps gives them a sense of control over the situation. This transforms the error experience from a roadblock into a detour with clear signage.
Core Principles for Writing Helpful Error Messages
Based on user psychology and experience design best practices, here are the fundamental principles for creating effective error messages:
1. Be Clear and Specific
Explain exactly what went wrong in plain language. Avoid vague statements that leave users guessing.
Poor: “An error occurred during form submission.”
Better: “Your form couldn’t be submitted because the email address is missing.”
2. Use Positive, Non-Blaming Language
Frame messages to focus on the situation rather than accusing the user.
Poor: “You entered an invalid password.”
Better: “The password doesn’t match our records.”
3. Provide Actionable Solutions
Always include guidance on how to resolve the issue.
Poor: “Payment failed.”
Better: “The payment couldn’t be processed. Please check your card details or try a different payment method.”
4. Use Plain, Human Language
Write as if you’re explaining the problem to someone in conversation.
Poor: “HTTP 404: Resource not found”
Better: “We couldn’t find the page you’re looking for. It might have been moved or deleted.”
5. Maintain Consistency with Your Brand
Error messages should match your overall tone and voice.
If your application has a friendly, conversational tone, your error messages should too. If your brand is more formal and professional, maintain that consistency.
6. Provide Context
Help users understand where the error occurred and why it matters.
Poor: “Invalid input.”
Better: “The date you entered (02/30/2023) isn’t valid because February doesn’t have 30 days.”
7. Use Appropriate Visual Cues
Visual indicators help users quickly identify and understand errors.
- Use consistent colors (typically red for errors)
- Add icons that intuitively signal an error
- Provide visual emphasis for the specific field or area with the issue
8. Consider Severity Levels
Not all errors are created equal. Adjust your messaging based on the seriousness of the error:
- Critical errors (data loss, security issues): Be clear about potential consequences and provide immediate actions
- Functional errors (incomplete fields, validation issues): Focus on guiding users to correct the problem
- Minor issues (non-optimal choices): Consider using warnings instead of errors
Before and After: Error Message Transformations
Let’s examine some common error scenarios and how to transform ineffective messages into helpful communication.
Example 1: Form Validation
Before:
Invalid input. Please correct the errors and try again.
After:
We need a few corrections before submitting:
- Phone number should be 10 digits with no spaces or dashes
- Password must be at least 8 characters long
- Please accept the terms of service
The transformed message specifically identifies what needs correction and provides guidance on the expected format.
Example 2: Login Failure
Before:
Login failed. Invalid credentials.
After:
We couldn't log you in with those details. Either your email or password doesn't match our records.
Need help? Try:
- Checking for typos
- Resetting your password
- Using the "Log in with Google" option
The improved message explains the issue and offers multiple paths to resolution.
Example 3: Technical Error
Before:
Error: Exception of type 'System.Data.SqlClient.SqlException' was thrown.
After:
We're having trouble connecting to our database right now.
Your information hasn't been lost, but we couldn't save your recent changes. Please try again in a few minutes or contact support if the problem continues.
Reference: ERR-DB-2301 (Our team can use this code to investigate the issue)
The revised message translates the technical error into understandable terms, provides reassurance about data, offers clear next steps, and still includes a reference code for support purposes.
Example 4: File Upload Error
Before:
Error: File exceeds maximum size limit.
After:
This file (resume.pdf - 15MB) is too large to upload.
Files must be under 5MB. You can:
- Compress the PDF to reduce its size
- Split large documents into multiple smaller files
- Use our document optimizer tool
The improved message specifies the file causing the problem, explains the limit, and provides multiple options to resolve the issue.
Special Considerations for Technical Users
For applications aimed at developers or technical users, error messages require a different approach. These users often need more detailed technical information to troubleshoot issues effectively.
Balancing Technicality with Usability
Even technical users benefit from clear, well-structured error messages. Consider a layered approach:
- Primary message: Clear, concise explanation of what went wrong
- Technical details: Expandable section with stack traces, error codes, and technical specifics
- Next steps: Suggested troubleshooting actions or documentation links
Example: API Error Response
For RESTful APIs or developer tools, structure error responses to be both human-readable and machine-parseable:
{
"error": {
"status": 400,
"message": "Invalid request parameters",
"details": "The 'user_id' field must be a valid integer",
"code": "INVALID_PARAMETER",
"documentation_url": "https://api.example.com/docs/errors#INVALID_PARAMETER",
"request_id": "req_7PtGLouduLWDvH"
}
}
This provides enough information for programmatic handling while remaining understandable to developers debugging the issue.
Compiler and Coding Errors
For programming languages and development environments, error messages should:
- Precisely identify the location of the error (file, line number, column)
- Explain what’s wrong in programming terms
- Suggest potential fixes or direct to relevant documentation
- Provide context about the expected syntax or behavior
Consider this improved compiler error message:
Error in src/components/UserProfile.js (line 42, column 3):
Cannot destructure property 'name' of 'user' as it is undefined.
This likely means the 'user' object is not available when this component renders.
Consider adding a conditional check:
if (!user) return <Loading /> or null
Learn more: https://docs.example.com/common-errors#undefined-destructuring
Implementation Strategies for Developers
Implementing effective error handling requires both technical and communication considerations. Here are practical strategies for developers:
Centralized Error Handling
Create a centralized error handling system that translates technical errors into user-friendly messages. This approach allows for:
- Consistent messaging across your application
- Easier maintenance and updates to error content
- Separation of technical error details from user-facing messages
A basic implementation might look like:
// Error message mapping
const errorMessages = {
'auth/wrong-password': {
message: 'The password you entered doesn't match our records.',
action: 'Double-check your password or use the "Forgot Password" link below.'
},
'auth/user-not-found': {
message: 'We couldn't find an account with that email address.',
action: 'Check for typos or create a new account.'
},
// Additional error mappings...
};
// Error handler function
function handleError(errorCode, fallbackMessage = 'Something went wrong') {
const errorInfo = errorMessages[errorCode] || {
message: fallbackMessage,
action: 'Please try again or contact support if the problem persists.'
};
return {
message: errorInfo.message,
action: errorInfo.action,
errorCode: errorCode // For logging/debugging
};
}
Contextual Error Messages
Different parts of your application may need different error handling approaches. Consider implementing context-specific error handlers for:
- Form validation errors
- Authentication issues
- Network and API errors
- Resource availability problems
Logging and Monitoring
While keeping user-facing messages clear and non-technical, maintain detailed error logs for troubleshooting:
- Log technical details of errors for developer analysis
- Include unique error IDs that connect user experiences to backend logs
- Monitor frequency and patterns of errors to identify systemic issues
Internationalization Considerations
For applications serving multiple languages:
- Store error messages in translation files rather than hardcoding them
- Consider cultural differences in how errors are perceived and communicated
- Ensure error messages maintain their clarity and helpfulness across all supported languages
Progressive Enhancement for Error Handling
Implement layers of error handling to gracefully degrade if certain parts fail:
try {
// Attempt the operation
saveUserData(userData);
} catch (error) {
// First try to use our mapped error messages
try {
const friendlyError = errorMapper.getFriendlyMessage(error.code);
displayErrorToUser(friendlyError);
} catch (mappingError) {
// If that fails, fall back to a generic but still helpful message
displayErrorToUser({
title: 'Unable to save your information',
message: 'We encountered a problem while saving your data.',
action: 'Please try again or contact our support team for help.'
});
}
// Always log the original error for debugging
logger.error('Data save error', { originalError: error });
}
Testing Your Error Messages
Even well-crafted error messages need validation with real users. Here’s how to ensure your error messages actually help:
Usability Testing
Conduct structured usability tests focused on error scenarios:
- Create tasks that are likely to trigger errors
- Observe how users react to and interpret error messages
- Note whether users can successfully recover from errors
- Gather feedback on clarity and helpfulness
A/B Testing
For high-impact error scenarios (like checkout failures or registration issues), consider A/B testing different error message approaches to see which leads to better outcomes.
Error Message Inventory and Audit
Periodically review all error messages in your application:
- Create an inventory of all possible error states
- Evaluate each message against the principles outlined in this guide
- Prioritize improvements based on frequency and impact
Accessibility Considerations
Ensure error messages are accessible to all users:
- Use proper ARIA attributes to announce errors to screen readers
- Maintain sufficient color contrast for error text and indicators
- Don’t rely solely on color to indicate errors
- Ensure error messages are keyboard navigable
Example of accessible error implementation:
<div class="form-field">
<label for="email">Email Address</label>
<input
type="email"
id="email"
aria-describedby="email-error"
aria-invalid="true"
>
<div
id="email-error"
class="error-message"
role="alert"
>
Please enter a valid email address (e.g., name@example.com)
</div>
</div>
Conclusion: From Frustration to Facilitation
Error messages represent critical moments in the user experience—points where users are most vulnerable to frustration and abandonment. By transforming these messages from technical notifications into helpful guidance, you can turn potential points of failure into opportunities for user education and support.
Remember these key takeaways:
- Be human: Write error messages in clear, non-technical language that focuses on solutions, not problems
- Be specific: Clearly identify what went wrong and how to fix it
- Be supportive: Use a tone that helps users feel supported rather than blamed
- Be actionable: Always provide clear next steps or recovery options
Implementing these principles requires a shift in how we think about errors—from necessary technical notifications to essential elements of user communication. By investing time in crafting helpful error messages, you’re not just reducing user frustration; you’re building trust in your application and brand.
For developers and designers, this means collaborating to create error handling systems that balance technical accuracy with human empathy. For organizations, it means recognizing that error messages are not an afterthought but a fundamental component of the user experience worthy of attention and resources.
When done right, your error messages won’t just help users—they’ll demonstrate your commitment to creating software that truly serves people, even when things go wrong.