Why Your Error Handling Strategy Is Missing Edge Cases
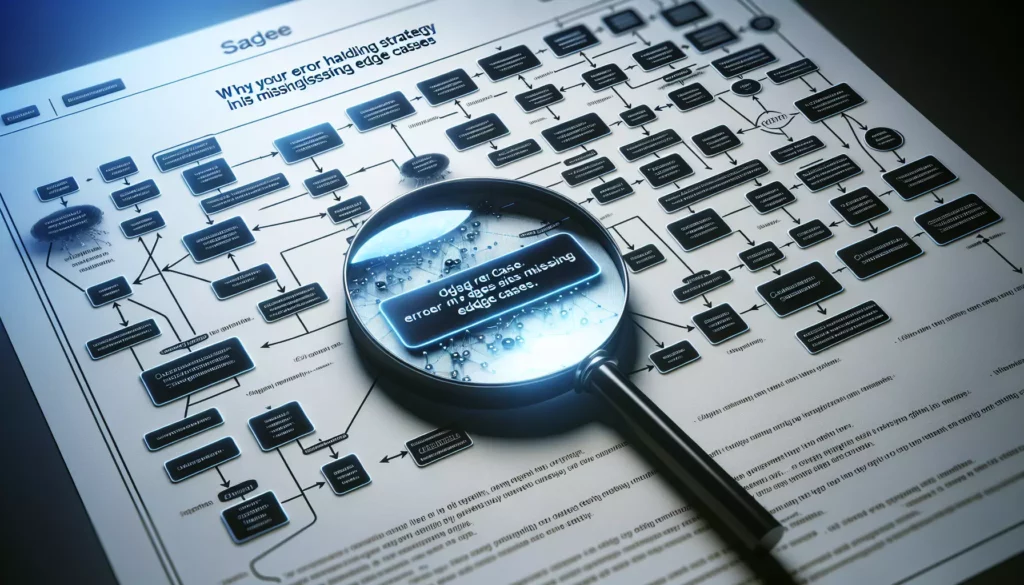
Error handling is often treated as an afterthought in programming. Many developers focus on the happy path—the expected flow of execution when everything works perfectly. But in the real world, things go wrong. APIs fail, networks drop, users input unexpected data, and systems run out of resources. A robust error handling strategy is not just about catching exceptions; it’s about anticipating and gracefully managing the unexpected.
In this comprehensive guide, we’ll explore why most error handling strategies fall short when it comes to edge cases, and how you can build more resilient applications by addressing these blind spots.
Table of Contents
- Understanding Edge Cases in Error Handling
- Common Mistakes in Error Handling Strategies
- A Comprehensive Approach to Error Handling
- Language-Specific Error Handling Techniques
- Testing for Edge Cases
- Monitoring and Handling Errors in Production
- Case Studies: When Error Handling Goes Wrong
- Best Practices for Robust Error Handling
- Conclusion
Understanding Edge Cases in Error Handling
Edge cases are situations that occur at the extremes of operating parameters. In the context of error handling, these are the rare, unexpected scenarios that your application might encounter. While they may be infrequent, failing to handle them properly can lead to catastrophic failures, data corruption, or security vulnerabilities.
Types of Edge Cases Often Missed
Resource Exhaustion: Applications can run out of memory, disk space, file handles, or other resources. Many error handling strategies fail to account for these scenarios.
Cascading Failures: When one component fails, it can trigger failures in dependent components. A robust error handling strategy should prevent these cascading effects.
Timing and Race Conditions: Concurrent operations can lead to unexpected states and errors that are difficult to reproduce and debug.
Partial Failures: Sometimes operations fail after partially completing, leaving the system in an inconsistent state.
Silent Failures: Some errors occur without raising exceptions or returning error codes, making them particularly insidious.
External System Failures: Dependencies on third-party services or APIs introduce additional failure modes that are often overlooked.
The Cost of Ignoring Edge Cases
Failing to handle edge cases properly can result in:
- Unplanned downtime and service outages
- Data loss or corruption
- Security vulnerabilities and breaches
- Poor user experience and customer dissatisfaction
- Increased maintenance costs and technical debt
- Reputation damage and loss of trust
A study by Gartner found that the average cost of IT downtime is $5,600 per minute, which translates to over $300,000 per hour. Many of these incidents could have been prevented with more thorough error handling.
Common Mistakes in Error Handling Strategies
Even when developers attempt to implement error handling, they often make critical mistakes that leave their applications vulnerable. Let’s examine some of the most common pitfalls.
Catching All Exceptions
One of the most prevalent mistakes is using overly broad exception handlers:
try {
// Code that might throw multiple types of exceptions
} catch (Exception e) {
// Generic handling for all exceptions
log.error("An error occurred", e);
}
This approach fails to distinguish between different types of errors, each of which might require specific handling. It also masks bugs that should cause the application to fail fast and visibly.
Swallowing Exceptions
Even worse than catching all exceptions is catching them and doing nothing:
try {
riskyOperation();
} catch (Exception e) {
// Empty catch block - exception is swallowed
}
This pattern hides errors, making debugging nearly impossible and potentially leading to silent failures that corrupt data or create security vulnerabilities.
Inadequate Logging
Logging errors without sufficient context limits your ability to diagnose and fix issues:
try {
processUserData(userData);
} catch (Exception e) {
log.error("Error processing user data"); // No exception details or user context
}
Effective error logs should include the exception stack trace, relevant context data, and a clear description of what the code was trying to do when the error occurred.
Ignoring Resource Cleanup
Failing to properly release resources in error scenarios can lead to resource leaks:
FileOutputStream fos = null;
try {
fos = new FileOutputStream("file.txt");
// Write to file
} catch (IOException e) {
log.error("Failed to write to file", e);
}
// Missing finally block to close fos
Modern languages provide better constructs for resource management (like Java’s try-with-resources or Python’s context managers), but they’re often underutilized.
Returning Null Instead of Throwing Exceptions
Some developers avoid exceptions by returning null or special values to indicate errors:
public User findUserById(String id) {
if (id == null) {
return null; // Returning null instead of throwing IllegalArgumentException
}
// Normal processing
}
This approach pushes error handling responsibility to the caller, who might not check for null returns, leading to NullPointerExceptions further down the call stack.
Inconsistent Error Handling Across the Codebase
When different parts of the application handle errors differently, it becomes difficult to reason about error flows and ensure proper recovery:
// Module A
try {
// Operation
} catch (Exception e) {
throw new ServiceException("Operation failed", e);
}
// Module B
try {
// Similar operation
} catch (Exception e) {
return ErrorResult.of(e.getMessage());
}
This inconsistency makes the codebase harder to maintain and can lead to unexpected behavior when modules interact.
A Comprehensive Approach to Error Handling
A robust error handling strategy requires a systematic approach that considers all potential failure modes. Here’s a framework for developing such a strategy:
Categorize Errors
Not all errors are created equal. Categorizing errors helps determine the appropriate response:
- Recoverable vs. Non-recoverable: Can the application continue after this error, or should it terminate?
- Expected vs. Unexpected: Is this an anticipated failure mode that should be handled specifically?
- Internal vs. External: Did the error originate within your code or in a dependency?
- Transient vs. Persistent: Is the error likely to resolve if the operation is retried?
Define Error Handling Policies
For each category of error, define clear policies:
- Retry Policy: Which errors should trigger retries? How many retries? What backoff strategy?
- Fallback Policy: When should alternative paths be taken? What are the fallback options?
- Notification Policy: Which errors require immediate attention? Who should be notified?
- Logging Policy: What information should be logged for each type of error?
- User Communication Policy: How should errors be communicated to users?
Implement Circuit Breakers
Circuit breakers prevent cascading failures by automatically detecting when a dependency is failing and stopping requests to it:
CircuitBreaker circuitBreaker = CircuitBreakerFactory.create(
"api-service",
3, // Failure threshold
1000, // Reset timeout in milliseconds
TimeUnit.MILLISECONDS
);
public Response callExternalService() {
return circuitBreaker.execute(() -> {
// Call to external service
return apiClient.makeRequest();
}, (e) -> {
// Fallback when circuit is open or call fails
return Response.fallback();
});
}
This pattern is especially valuable for microservices architectures where dependencies on external systems are common.
Use Timeouts
Every external call should have a timeout to prevent hanging operations:
CompletableFuture<Result> future = CompletableFuture.supplyAsync(() -> {
return slowOperation();
});
try {
Result result = future.get(5, TimeUnit.SECONDS);
// Process result
} catch (TimeoutException e) {
// Handle timeout
log.warn("Operation timed out after 5 seconds");
future.cancel(true); // Attempt to cancel the operation
return fallbackResult();
}
Implement Graceful Degradation
Design your application to function at reduced capacity when components fail:
public SearchResults search(String query) {
SearchResults results = new SearchResults();
// Try to get results from primary search engine
try {
results.addAll(primarySearch.search(query));
} catch (SearchException e) {
log.warn("Primary search failed, falling back to backup", e);
// Fall back to backup search engine
try {
results.addAll(backupSearch.search(query));
} catch (SearchException e2) {
log.error("Backup search also failed", e2);
// Return empty results rather than failing completely
}
}
// Try to add recommendations if available
try {
results.setRecommendations(recommendationService.getRecommendations(query));
} catch (Exception e) {
// Non-critical feature can fail without affecting core functionality
log.info("Recommendations unavailable", e);
}
return results;
}
Use Bulkheads
Bulkheads isolate components to prevent failures in one area from affecting others:
// Define separate thread pools for different components
ExecutorService ordersPool = Executors.newFixedThreadPool(10);
ExecutorService inventoryPool = Executors.newFixedThreadPool(5);
ExecutorService notificationsPool = Executors.newFixedThreadPool(3);
// Use the appropriate pool for each type of operation
public void processOrder(Order order) {
CompletableFuture.supplyAsync(() -> {
return orderService.process(order);
}, ordersPool).thenAcceptAsync(result -> {
inventoryService.update(result);
}, inventoryPool).thenAcceptAsync(result -> {
notificationService.notify(result);
}, notificationsPool);
}
This approach ensures that, for example, a flood of notifications won’t prevent order processing from continuing.
Language-Specific Error Handling Techniques
Different programming languages provide different mechanisms for error handling. Understanding these language-specific features is crucial for implementing effective error handling.
Java
Java uses a combination of checked and unchecked exceptions:
// Using try-with-resources for automatic resource cleanup
try (Connection conn = dataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement("SELECT * FROM users WHERE id = ?")) {
stmt.setString(1, userId);
try (ResultSet rs = stmt.executeQuery()) {
if (rs.next()) {
return mapToUser(rs);
} else {
throw new UserNotFoundException("User not found with ID: " + userId);
}
}
} catch (SQLException e) {
throw new DatabaseException("Database error while fetching user", e);
} catch (UserNotFoundException e) {
// Rethrow application-specific exceptions
throw e;
} catch (Exception e) {
// Unexpected exceptions
throw new ServiceException("Unexpected error fetching user", e);
}
Python
Python uses a try/except/finally mechanism and context managers:
def get_user(user_id):
try:
with db.session() as session:
user = session.query(User).filter(User.id == user_id).first()
if not user:
raise UserNotFoundError(f"User not found with ID: {user_id}")
return user
except SQLAlchemyError as e:
logger.error(f"Database error: {str(e)}")
raise DatabaseError("Database error while fetching user") from e
except UserNotFoundError:
# Log and rethrow
logger.info(f"User not found: {user_id}")
raise
except Exception as e:
logger.exception("Unexpected error fetching user")
raise ServiceError("Unexpected error fetching user") from e
JavaScript/TypeScript
JavaScript traditionally uses try/catch blocks but has evolved to include Promises and async/await:
async function getUser(userId) {
try {
const response = await fetch(`/api/users/${userId}`);
if (!response.ok) {
if (response.status === 404) {
throw new UserNotFoundError(`User not found with ID: ${userId}`);
}
throw new ApiError(`API error: ${response.status}`);
}
const userData = await response.json();
return new User(userData);
} catch (error) {
if (error instanceof UserNotFoundError) {
// Handle specific error
console.log(error.message);
throw error;
} else if (error instanceof ApiError) {
// Handle API errors
console.error('API Error:', error);
throw new ServiceError('Service temporarily unavailable');
} else if (error instanceof TypeError) {
// Network errors often manifest as TypeErrors
console.error('Network Error:', error);
throw new ConnectionError('Unable to connect to the server');
} else {
// Unexpected errors
console.error('Unexpected Error:', error);
throw new Error('An unexpected error occurred');
}
}
}
Go
Go uses a different approach, returning errors as values rather than throwing exceptions:
func GetUser(id string) (*User, error) {
if id == "" {
return nil, errors.New("user ID cannot be empty")
}
db, err := sql.Open("postgres", connectionString)
if err != nil {
return nil, fmt.Errorf("failed to connect to database: %w", err)
}
defer db.Close()
var user User
err = db.QueryRow("SELECT id, name, email FROM users WHERE id = $1", id).Scan(&user.ID, &user.Name, &user.Email)
if err != nil {
if err == sql.ErrNoRows {
return nil, &UserNotFoundError{ID: id}
}
return nil, fmt.Errorf("database error: %w", err)
}
return &user, nil
}
Testing for Edge Cases
Identifying and testing edge cases is essential for robust error handling. Here are techniques to ensure your error handling strategy is comprehensive:
Chaos Engineering
Chaos engineering involves deliberately introducing failures to test system resilience:
@Test
public void testDatabaseFailure() {
// Simulate database connection failure
when(dataSource.getConnection()).thenThrow(new SQLException("Connection refused"));
// Verify the service handles the failure gracefully
assertThatThrownBy(() -> userService.getUser("123"))
.isInstanceOf(ServiceUnavailableException.class)
.hasMessageContaining("Database unavailable");
// Verify proper logging
verify(logger).error(contains("Database connection failed"), any(SQLException.class));
}
Fault Injection
Systematically inject faults at various points in your application:
public class FaultInjectingHttpClient implements HttpClient {
private final HttpClient delegate;
private final double failureRate;
private final Random random = new Random();
@Override
public HttpResponse send(HttpRequest request) throws IOException {
if (random.nextDouble() < failureRate) {
throw new IOException("Simulated network failure");
}
return delegate.send(request);
}
}
Property-Based Testing
Generate a wide range of inputs to discover edge cases:
@Property
void handlesAllInputTypes(
@ForAll @AlphaChars String alphabeticInput,
@ForAll @NumericChars String numericInput,
@ForAll @StringLength(min = 0, max = 1000) String varyingLengthInput,
@ForAll @Chars(from = 0, to = 127) String asciiInput
) {
// Test that the function doesn't throw unexpected exceptions
assertDoesNotThrow(() -> processor.process(alphabeticInput));
assertDoesNotThrow(() -> processor.process(numericInput));
assertDoesNotThrow(() -> processor.process(varyingLengthInput));
assertDoesNotThrow(() -> processor.process(asciiInput));
}
Load and Stress Testing
Test how your error handling performs under high load:
@Test
public void testConcurrentRequests() throws InterruptedException {
int numThreads = 100;
CountDownLatch latch = new CountDownLatch(numThreads);
AtomicInteger successCount = new AtomicInteger(0);
AtomicInteger errorCount = new AtomicInteger(0);
for (int i = 0; i < numThreads; i++) {
new Thread(() -> {
try {
service.processRequest();
successCount.incrementAndGet();
} catch (Exception e) {
errorCount.incrementAndGet();
} finally {
latch.countDown();
}
}).start();
}
latch.await(30, TimeUnit.SECONDS);
System.out.println("Successful requests: " + successCount.get());
System.out.println("Failed requests: " + errorCount.get());
// Even under load, we should have a reasonable success rate
assertThat(successCount.get()).isGreaterThan(numThreads * 0.8);
}
Boundary Testing
Test at the boundaries of valid inputs and resource limits:
@Test
public void testMaximumInputSize() {
String largeInput = "A".repeat(MAX_INPUT_SIZE);
String tooLargeInput = "A".repeat(MAX_INPUT_SIZE + 1);
// Should handle maximum valid size
assertDoesNotThrow(() -> validator.validate(largeInput));
// Should reject input that's too large
assertThatThrownBy(() -> validator.validate(tooLargeInput))
.isInstanceOf(InvalidInputException.class)
.hasMessageContaining("exceeds maximum size");
}
Monitoring and Handling Errors in Production
Even with the best testing, errors will occur in production. A comprehensive error handling strategy includes monitoring and responding to these errors.
Implementing Proper Logging
Structured logging provides context for debugging:
try {
processPayment(order);
} catch (PaymentException e) {
log.error("Payment processing failed", Map.of(
"orderId", order.getId(),
"amount", order.getAmount(),
"customerId", order.getCustomerId(),
"paymentMethod", order.getPaymentMethod(),
"errorCode", e.getErrorCode()
), e);
notifyPaymentTeam(e, order);
return PaymentResult.failure(e.getErrorCode());
}
Real-time Monitoring and Alerting
Set up monitoring systems to detect error patterns:
// Define an alert rule in Prometheus
alert: HighErrorRate
expr: sum(rate(http_requests_total{status=~"5.."}[5m])) / sum(rate(http_requests_total[5m])) > 0.05
for: 1m
labels:
severity: critical
annotations:
summary: High HTTP error rate
description: More than 5% of requests are failing with 5xx errors for the past minute.
Implementing Health Checks
Health checks help detect and isolate failing components:
@GetMapping("/health")
public ResponseEntity<HealthStatus> healthCheck() {
HealthStatus status = new HealthStatus();
// Check database connectivity
try {
boolean dbHealthy = databaseService.ping();
status.addComponent("database", dbHealthy ? "UP" : "DOWN");
} catch (Exception e) {
status.addComponent("database", "DOWN");
status.addError("database", e.getMessage());
}
// Check cache connectivity
try {
boolean cacheHealthy = cacheService.ping();
status.addComponent("cache", cacheHealthy ? "UP" : "DOWN");
} catch (Exception e) {
status.addComponent("cache", "DOWN");
status.addError("cache", e.getMessage());
}
// Overall status is UP only if all critical components are UP
boolean isHealthy = status.isCriticalComponentsHealthy();
return ResponseEntity
.status(isHealthy ? HttpStatus.OK : HttpStatus.SERVICE_UNAVAILABLE)
.body(status);
}
Implementing Feature Flags
Feature flags allow quick disabling of problematic features:
public SearchResult search(String query) {
SearchResult result = new SearchResult();
// Add basic search results
result.addItems(basicSearch(query));
// Only include advanced features if enabled
if (featureFlags.isEnabled("advanced-search")) {
try {
result.addItems(advancedSearch(query));
} catch (Exception e) {
log.error("Advanced search failed", e);
// Disable the feature if it fails repeatedly
if (errorTracker.shouldDisableFeature("advanced-search", e)) {
featureFlags.disable("advanced-search");
log.warn("Advanced search feature automatically disabled due to errors");
}
}
}
return result;
}
Case Studies: When Error Handling Goes Wrong
Learning from real-world failures can help improve your error handling strategy. Here are some notable examples:
Amazon S3 Outage (2017)
In February 2017, a typo in a command during routine server maintenance took down a significant portion of Amazon S3 for over four hours. The system didn't have adequate safeguards against removing too many servers at once, and the restart process was slower than expected due to the system's scale.
Lessons Learned:
- Implement safeguards against destructive operations
- Test recovery procedures at scale
- Design systems to gracefully handle partial failures
Knight Capital Group (2012)
Knight Capital lost $440 million in 45 minutes due to a software error. They deployed new code to only some of their servers, creating inconsistent behavior. When an error occurred, the system continued to execute erroneous trades rather than shutting down.
Lessons Learned:
- Implement circuit breakers for critical operations
- Ensure consistent deployment across all servers
- Have automated safeguards against unusual patterns
Cloudflare Memory Leak (2017)
A buffer overflow in Cloudflare's edge servers caused sensitive data to leak into cached web pages. The error occurred in an HTML parser designed to modify web pages for optimization.
Lessons Learned:
- Use memory-safe languages or tools for critical components
- Implement bounds checking and other safety measures
- Have a robust incident response plan for security issues
Best Practices for Robust Error Handling
Based on everything we've covered, here are the key best practices for a comprehensive error handling strategy:
Design for Failure
- Assume every operation can fail and plan accordingly
- Design systems to be resilient to partial failures
- Use defensive programming techniques
Be Specific About Exceptions
// Bad
try {
processData(input);
} catch (Exception e) {
log.error("Error", e);
}
// Good
try {
processData(input);
} catch (InvalidInputException e) {
log.warn("Invalid input: {}", e.getMessage());
return Result.error("Invalid input format");
} catch (DatabaseException e) {
log.error("Database error while processing data", e);
return Result.error("Service temporarily unavailable");
} catch (Exception e) {
log.error("Unexpected error processing data", e);
return Result.error("An unexpected error occurred");
}
Use a Consistent Error Model
Define a consistent approach to error handling across your codebase:
public class Result<T> {
private final boolean success;
private final T data;
private final ErrorInfo error;
private Result(boolean success, T data, ErrorInfo error) {
this.success = success;
this.data = data;
this.error = error;
}
public static <T> Result<T> success(T data) {
return new Result<>(true, data, null);
}
public static <T> Result<T> error(String message) {
return new Result<>(false, null, new ErrorInfo(message));
}
public static <T> Result<T> error(String message, String code) {
return new Result<>(false, null, new ErrorInfo(message, code));
}
// Additional methods...
}
Fail Fast
Detect and report errors as early as possible:
public void processOrder(Order order) {
// Validate inputs immediately
if (order == null) {
throw new IllegalArgumentException("Order cannot be null");
}
if (order.getItems() == null || order.getItems().isEmpty()) {
throw new InvalidOrderException("Order must contain at least one item");
}
if (order.getCustomerId() == null) {
throw new InvalidOrderException("Order must have a customer ID");
}
// Proceed with processing
// ...
}
Provide Meaningful Error Messages
Error messages should be actionable and informative:
// Bad
throw new Exception("Error");
// Good
throw new ConfigurationException(
"Database connection failed: Unable to connect to MySQL server at db.example.com:3306. " +
"Please check that the database server is running and network connectivity is available. " +
"Error details: Connection refused (Connection refused)"
);
Implement Proper Resource Management
Always clean up resources, even when errors occur:
// Java example with try-with-resources
try (
Connection conn = dataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement(SQL_QUERY);
ResultSet rs = stmt.executeQuery()
) {
// Process results
} catch (SQLException e) {
// Handle exception
}
Log Errors with Context
Include relevant context in error logs:
try {
processOrder(order);
} catch (Exception e) {
log.error("Failed to process order: {}, customer: {}, items: {}",
order.getId(),
order.getCustomerId(),
order.getItems().size(),
e);
}
Use Retry with Backoff for Transient Failures
Implement exponential backoff for retrying operations:
public <T> T executeWithRetry(Supplier<T> operation) {
int maxRetries = 3;
int retryCount = 0;
int waitTimeMs = 1000; // Start with 1 second
while (true) {
try {
return operation.get();
} catch (Exception e) {
retryCount++;
if (isTransientException(e) && retryCount <= maxRetries) {
log.warn("Operation failed with transient error, retrying ({}/{}): {}",
retryCount, maxRetries, e.getMessage());
try {
Thread.sleep(waitTimeMs);
// Exponential backoff
waitTimeMs *= 2;
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
throw new RuntimeException("Retry interrupted", ie);
}
} else {
log.error("Operation failed permanently after {} tries", retryCount, e);
throw e;
}
}
}
}
Conclusion
Error handling is not just about catching