Why Your Engineering Processes Are Slowing Down Development
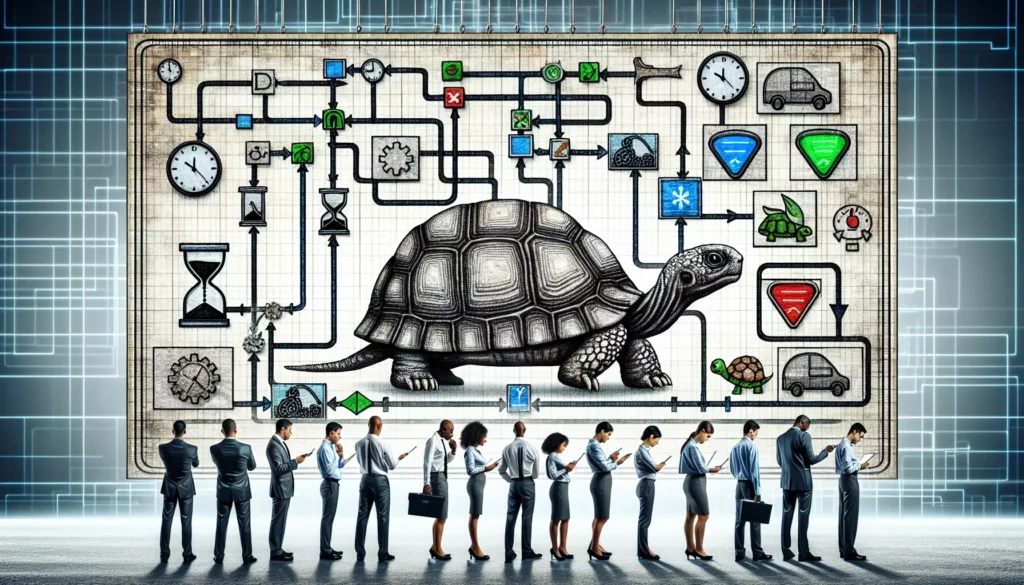
In the fast-paced world of software development, speed and efficiency are critical to staying competitive. Yet many engineering teams find themselves struggling with slow development cycles despite having talented engineers and modern tools. The culprit often lies not in the technical skills of your team but in the engineering processes that guide their work.
This comprehensive guide examines how common engineering processes can inadvertently create bottlenecks and how to optimize them for faster, more efficient development without sacrificing quality.
Table of Contents
- Understanding Development Slowdowns
- When Agile Becomes Fragile
- Code Review Bottlenecks
- Testing Overhead and Automation Gaps
- Deployment Challenges
- Communication Overload
- Technical Debt Management
- Documentation Issues
- Measuring Process Improvement
- Real-World Examples of Process Optimization
- Conclusion
Understanding Development Slowdowns
Before diving into specific processes, it’s important to understand what a development slowdown actually looks like. Common symptoms include:
- Increasing time between feature ideation and deployment
- Growing backlog of bugs and technical debt
- Missed deadlines and extended sprint commitments
- Developer frustration and reduced morale
- Frequent context switching
- Increased time spent in meetings versus coding
While it’s tempting to blame external factors like scope creep or insufficient resources, the truth is that internal processes often contribute significantly to these issues. Let’s examine the most common process-related bottlenecks and how to address them.
When Agile Becomes Fragile
Agile methodologies were designed to increase development speed and adaptability, but poorly implemented Agile processes can have the opposite effect.
The Problem: Agile Theater
Many organizations practice what experts call “Agile Theater” – going through the motions of Agile ceremonies without embracing the underlying philosophy. This manifests as:
- Excessively long daily standups that become status reports rather than coordination meetings
- Sprint planning that packs too much work into fixed timeframes
- Retrospectives that identify issues but don’t lead to meaningful changes
- Story points becoming targets rather than estimates
- Product backlogs that grow endlessly without proper refinement
The Solution: Authentic Agile
To make Agile work as intended:
- Time-box ceremonies strictly: Limit standups to 15 minutes, with detailed discussions taken offline.
- Focus on outcomes, not outputs: Measure value delivered, not just story points completed.
- Implement retrospective action items: Create specific, assigned tasks from retrospectives and track their completion.
- Maintain a healthy backlog: Regularly prune and prioritize the backlog, removing outdated items.
- Respect the sustainable pace principle: Avoid the temptation to consistently overcommit.
Consider this example of a refined sprint planning process:
// Example: Improved Sprint Planning Process
1. Pre-planning: Product owner ensures stories are properly refined
- All stories have clear acceptance criteria
- Dependencies are identified
- Technical requirements are understood
2. Capacity planning:
- Team calculates actual capacity (considering PTO, meetings, etc.)
- Apply a 20% buffer for unexpected work
3. Commitment:
- Team pulls in work based on priority until capacity is reached
- No pressure to increase velocity artificially
4. Mid-sprint checkpoint:
- Brief meeting at sprint midpoint to assess progress
- Early identification of blockers
- Adjust sprint scope if necessary
By embracing the true spirit of Agile – adaptability, continuous improvement, and sustainable development – teams can eliminate many process-related delays.
Code Review Bottlenecks
Code reviews are essential for maintaining code quality, but they frequently become major bottlenecks in the development process.
The Problem: Review Purgatory
Pull requests often get stuck in what developers call “review purgatory” due to:
- Overly large pull requests that are difficult to review
- Unclear expectations about review turnaround time
- Reviewers being overcommitted and unable to prioritize reviews
- Nitpicking on stylistic issues rather than focusing on functionality
- Lack of context for reviewers
- Sequential rather than parallel reviews
The Solution: Streamlined Review Processes
To make code reviews an accelerator rather than a bottleneck:
- Keep pull requests small: Aim for PRs that can be reviewed in under 30 minutes.
- Set clear SLAs: Establish expectations that reviews should be completed within a specific timeframe (e.g., 24 hours).
- Automate style checks: Use linters and formatters to handle style issues automatically.
- Provide context: Include clear descriptions, links to requirements, and even screenshots or videos for UI changes.
- Implement pair programming for complex features to reduce review time later.
- Rotate reviewers to distribute the review workload and share knowledge.
Here’s an example of a more effective pull request template:
## Description
[Brief description of the changes]
## Type of Change
- [ ] Bug fix
- [ ] New feature
- [ ] Breaking change
- [ ] Performance improvement
## How to Test
1. [Step-by-step testing instructions]
2. [Expected results]
## Screenshots/Videos
[If applicable]
## Related Issues
[Link to related tickets/issues]
## Checklist
- [ ] Tests added/updated
- [ ] Documentation updated
- [ ] Performance impact considered
By optimizing the review process, teams can maintain quality while significantly reducing the time code spends waiting for approval.
Testing Overhead and Automation Gaps
Testing is crucial for software quality, but inefficient testing processes can significantly slow down development.
The Problem: Testing Bottlenecks
Common testing-related slowdowns include:
- Manual testing processes that could be automated
- Slow, flaky, or unreliable test suites
- Excessive test coverage in low-risk areas
- Inadequate test environments
- Testing as a separate phase rather than an integrated part of development
- Lack of testing strategy aligned with business risk
The Solution: Strategic Test Automation
To optimize testing without compromising quality:
- Adopt test pyramids: Focus on fast, reliable unit tests with fewer integration and UI tests.
- Implement continuous testing: Run tests automatically with each commit.
- Focus on test quality over quantity: Prioritize tests that cover critical paths and high-risk areas.
- Address flaky tests immediately: Unreliable tests erode confidence and waste time.
- Implement parallel test execution to reduce overall test runtime.
- Use production-like test environments to catch environment-specific issues early.
Consider this approach to test optimization:
// Test Strategy Prioritization Framework
1. Critical Path Tests (highest priority)
- Core user journeys
- Revenue-generating functionality
- Data integrity operations
2. Regression Protection Tests
- Areas with history of bugs
- Complex business logic
- Integration points
3. Edge Case Tests
- Boundary conditions
- Error handling
- Rare but important scenarios
4. Performance-Critical Tests
- Operations with strict performance requirements
- High-volume transactions
5. Nice-to-Have Tests (lowest priority)
- Minor UI variations
- Rarely used features
- Already covered by higher-level tests
By taking a strategic approach to testing, teams can maintain or even improve quality while reducing the testing burden on the development process.
Deployment Challenges
The final step of getting code to production is often fraught with delays and complications that can significantly slow the development cycle.
The Problem: Deployment Friction
Common deployment-related issues include:
- Manual, error-prone deployment processes
- Infrequent deployments leading to large, risky changes
- Complex approval processes with multiple gatekeepers
- Deployment windows limited to specific times
- Lack of rollback mechanisms that create fear of deployment
- Environment inconsistencies causing unexpected issues in production
The Solution: Continuous Deployment and Infrastructure as Code
To streamline the path to production:
- Implement CI/CD pipelines: Automate building, testing, and deployment processes.
- Adopt feature flags: Decouple deployment from release to enable safe, frequent deployments.
- Use infrastructure as code: Ensure environment consistency and enable easy replication.
- Implement canary deployments: Gradually roll out changes to reduce risk.
- Create self-service deployment capabilities for developers with appropriate guardrails.
- Establish fast, reliable rollback mechanisms to increase confidence in deployments.
A modern deployment pipeline might look like this:
// Modern Deployment Pipeline
1. Code Commit
↓
2. Automated Build & Unit Tests
↓
3. Static Code Analysis
↓
4. Integration Tests
↓
5. Performance Tests
↓
6. Security Scans
↓
7. Automated Deployment to Staging
↓
8. Smoke Tests in Staging
↓
9. Automated Deployment to Production (behind feature flag)
↓
10. Gradual Rollout (canary/percentage-based)
↓
11. Automated Rollback if Monitoring Detects Issues
↓
12. Post-Deployment Verification
By removing manual steps and building safety mechanisms into the deployment process, teams can deploy more frequently with less risk and overhead.
Communication Overload
While communication is essential for team coordination, excessive or inefficient communication processes can significantly impact development velocity.
The Problem: Meeting Madness and Notification Noise
Communication overload manifests as:
- Calendar filled with back-to-back meetings
- Constant interruptions from chat tools and notifications
- Requirement details scattered across multiple communication channels
- Excessive synchronous communication for asynchronous issues
- Duplicate status reporting to different stakeholders
- Unclear decision-making processes requiring multiple discussions
The Solution: Structured Communication and Focus Time
To optimize communication without losing alignment:
- Implement no-meeting days: Designate specific days or time blocks for focused development work.
- Adopt asynchronous communication by default, using tools like documentation and recorded videos.
- Consolidate status reporting into a single, accessible format that all stakeholders can reference.
- Create a decision log to document decisions and their rationale, reducing the need to revisit discussions.
- Set expectations for notification response times, allowing developers to batch-process communications.
- Use the right medium for the message: documentation for complex information, chat for quick questions, meetings for discussions requiring real-time interaction.
Here’s an example of a structured communication framework:
// Communication Framework
1. Urgent/Blocking Issues
- Channel: Direct message or call
- Expected response: ASAP
- Examples: Production outage, blocked deployment
2. Important but Not Urgent
- Channel: Team chat with @mention
- Expected response: Same day
- Examples: Code review requests, clarification needed
3. FYI/Non-urgent
- Channel: Team chat without @mention or email
- Expected response: Within 24-48 hours
- Examples: Status updates, non-critical information
4. Knowledge Sharing
- Channel: Documentation, wiki
- Expected response: N/A
- Examples: Architecture decisions, onboarding info
5. Team Coordination
- Channel: Scheduled meetings with agenda
- Frequency: Only as needed with clear outcomes
- Examples: Sprint planning, architecture discussions
By creating clear communication protocols and protecting focused development time, teams can reduce the overhead of excessive communication while maintaining alignment.
Technical Debt Management
Unmanaged technical debt accumulates over time and can significantly slow down development as teams struggle with legacy code and architectural constraints.
The Problem: The Technical Debt Spiral
Technical debt creates a vicious cycle:
- Time pressure leads to shortcuts and quick fixes
- These shortcuts create technical debt
- Technical debt makes future development slower
- Slower development creates more time pressure
- The cycle repeats, with velocity continuously decreasing
The Solution: Deliberate Technical Debt Management
To break the technical debt spiral:
- Make technical debt visible: Track it in your backlog or dedicated tools.
- Allocate dedicated time for debt reduction in each sprint (e.g., 20% of capacity).
- Apply the “boy scout rule”: Leave code better than you found it with each change.
- Prioritize debt based on impact: Focus on areas causing the most friction.
- Refactor strategically, especially before adding new features to problematic areas.
- Measure the cost of technical debt in terms of development slowdown and bugs.
A technical debt prioritization framework might look like this:
// Technical Debt Prioritization Matrix
HIGH IMPACT, LOW EFFORT (Do First)
- Flaky tests slowing CI pipeline
- Missing documentation for core APIs
- Inconsistent error handling
HIGH IMPACT, HIGH EFFORT (Plan & Schedule)
- Monolith breaking into microservices
- Database schema refactoring
- Major framework upgrades
LOW IMPACT, LOW EFFORT (Quick Wins)
- Code style inconsistencies
- Unused code removal
- Minor dependency updates
LOW IMPACT, HIGH EFFORT (Consider Alternatives)
- Complete rewrite of rarely used features
- Cosmetic refactoring
- Nice-to-have architecture improvements
By making technical debt management a continuous, deliberate process rather than an afterthought, teams can prevent the accumulation of debt that slows down development.
Documentation Issues
Poor or outdated documentation forces developers to spend excessive time figuring out how systems work, significantly slowing down development, especially for new team members.
The Problem: Documentation Debt
Documentation-related issues include:
- Missing or outdated documentation for critical systems
- Tribal knowledge accessible only to long-tenured team members
- Scattered documentation across multiple tools and repositories
- Lack of context in documentation (why decisions were made)
- No clear ownership for maintaining documentation
- Excessive documentation that’s difficult to navigate
The Solution: Documentation as a First-Class Citizen
To improve documentation without creating excessive overhead:
- Treat documentation as a product requirement, not an optional extra.
- Keep documentation close to code using tools like README files and code comments.
- Focus on the “why” not just the “what” in documentation.
- Create living documentation that updates automatically when possible.
- Implement a documentation review process alongside code reviews.
- Use a documentation hierarchy with different levels of detail for different audiences.
A practical documentation strategy might include:
// Documentation Hierarchy
1. High-Level Architecture (for everyone)
- System overview diagrams
- Component relationships
- Key design decisions and rationale
2. Service/Module Documentation (for developers)
- Purpose and responsibilities
- API contracts
- Dependencies and integration points
- Configuration options
3. Implementation Details (for maintainers)
- Algorithm explanations
- Class/function documentation
- Performance considerations
- Known limitations
4. Operational Documentation (for SRE/DevOps)
- Deployment procedures
- Monitoring points
- Common failure modes
- Troubleshooting guides
By elevating documentation to a first-class concern, teams can reduce the time spent rediscovering how systems work and enable faster onboarding and development.
Measuring Process Improvement
To effectively optimize engineering processes, teams need to measure the impact of their changes. Without data, it’s difficult to know if process improvements are actually working.
Key Metrics to Track
Consider measuring the following metrics to gauge process effectiveness:
- Lead Time: Time from idea to production
- Cycle Time: Time from code commit to production deployment
- Deployment Frequency: How often code is deployed to production
- Change Failure Rate: Percentage of deployments causing incidents
- Mean Time to Recovery: How quickly incidents are resolved
- Code Review Turnaround Time: How long PRs wait for review
- Build/Test Duration: Time spent in CI/CD pipelines
- Developer Satisfaction: Survey-based metric on process satisfaction
Implementing Process Improvements
When making process changes:
- Start small: Implement changes incrementally to isolate their impact.
- Establish baselines: Measure current performance before making changes.
- Set clear goals: Define what success looks like for each process improvement.
- Gather feedback: Regularly check with the team about how process changes are working.
- Be willing to revert: If a process change isn’t working, don’t be afraid to roll it back.
A structured approach to process improvement might look like this:
// Process Improvement Cycle
1. Measure current performance (baseline)
- Collect quantitative metrics
- Gather qualitative feedback
2. Identify bottlenecks
- Which process has the biggest impact on velocity?
- What causes the most developer frustration?
3. Design targeted improvements
- Small, focused changes
- Clear success criteria
4. Implement changes
- Communicate clearly to the team
- Provide necessary training/support
5. Measure impact
- Compare to baseline
- Collect feedback
6. Adjust or expand
- Refine the process based on results
- Roll out to more teams if successful
By taking a data-driven approach to process improvement, teams can ensure they’re making changes that actually increase development velocity rather than adding new forms of overhead.
Real-World Examples of Process Optimization
Let’s look at how some organizations have successfully optimized their engineering processes to increase development velocity.
Example 1: Reducing Build Times at Spotify
Spotify faced challenges with long build times that were slowing down their development cycle. They addressed this by:
- Creating a dedicated “Build Time Task Force”
- Implementing incremental builds
- Moving to a microservices architecture that allowed smaller, faster builds
- Investing in better CI infrastructure
The result was a reduction in build times from over 20 minutes to under 5 minutes, significantly improving developer productivity.
Example 2: GitHub’s Code Review Transformation
GitHub noticed that code reviews were becoming a major bottleneck. They improved the process by:
- Setting clear expectations for PR size (under 250 lines when possible)
- Implementing automated code analysis to catch issues before human review
- Creating a “review swarm” culture where team members prioritize reviews
- Using metrics to identify and address review bottlenecks
These changes reduced their average review time from 2 days to less than 4 hours.
Example 3: Continuous Deployment at Amazon
Amazon transformed their deployment process to enable thousands of deployments per day by:
- Decentralizing deployment authority to development teams
- Building sophisticated monitoring and rollback capabilities
- Implementing feature flags for safer deployments
- Creating self-service deployment infrastructure
This approach allowed them to move from infrequent, high-risk deployments to continuous, low-risk updates.
Example 4: Google’s Documentation Strategy
Google addressed documentation challenges by:
- Creating a culture where documentation is valued as highly as code
- Implementing a “readability” process where code isn’t accepted unless it’s well-documented
- Using automated tools to detect when documentation and code drift apart
- Including documentation quality in performance reviews
This approach has helped Google maintain high-quality documentation despite rapid growth and change.
Conclusion
Engineering processes are essential for coordinating work and maintaining quality, but they can easily become bottlenecks that slow down development. By critically examining your processes and optimizing them with a focus on developer experience and efficiency, you can significantly increase your team’s velocity without sacrificing quality.
Key takeaways include:
- Regularly audit your processes to identify bottlenecks
- Measure the impact of process changes to ensure they’re actually helping
- Focus on eliminating wait states where work sits idle
- Automate repetitive tasks to free up developer time
- Optimize for developer experience and flow
- Balance process discipline with flexibility
- Continuously refine processes based on team feedback and changing needs
Remember that the goal of engineering processes is to enable teams to deliver value quickly and sustainably. When processes begin to hinder rather than help, it’s time to step back and reconsider your approach.
By applying the principles and practices outlined in this guide, you can transform your engineering processes from impediments to accelerators, enabling your team to deliver better software faster.
What process bottlenecks is your team facing, and which of these strategies might help address them? The journey to optimized engineering processes starts with identifying your biggest constraints and taking concrete steps to address them.