Why Your Development Workflow Isn’t Efficient Enough (And How to Fix It)
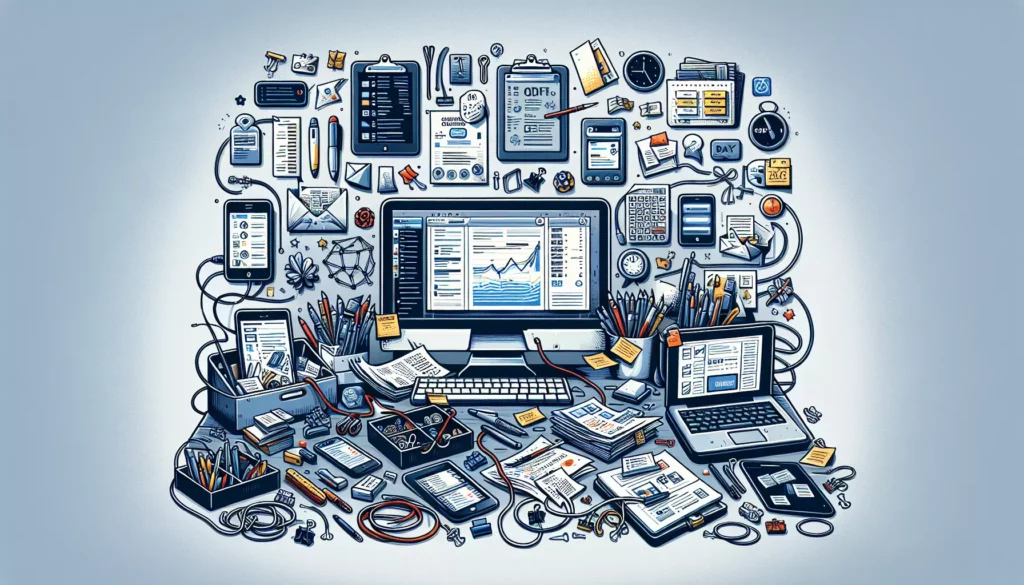
In the fast-paced world of software development, your workflow efficiency can make or break your success. Whether you’re a coding beginner or an experienced programmer preparing for technical interviews at top tech companies, optimizing your development workflow is crucial for maximizing productivity and code quality.
Many developers struggle with inefficient workflows without even realizing it. They accept the status quo of context switching, manual processes, and disorganized approaches as “just part of coding.” But the truth is, these inefficiencies accumulate over time, leading to missed deadlines, buggy code, and unnecessary stress.
In this comprehensive guide, we’ll explore the common pitfalls that hamper development workflows and provide actionable strategies to transform your coding process into a streamlined, efficient system that will help you become a more effective programmer.
Table of Contents
- Signs Your Development Workflow Needs Improvement
- Optimizing Your Development Environment
- Embracing Automation
- Mastering Version Control
- Implementing Effective Testing Strategies
- Enhancing Team Collaboration
- Continuous Learning and Improvement
- Essential Tools for Modern Development Workflows
- Measuring and Tracking Workflow Improvements
- Conclusion: Building Your Optimal Workflow
Signs Your Development Workflow Needs Improvement
Before diving into solutions, it’s important to recognize the symptoms of an inefficient workflow. Here are telltale signs that your development process could use an overhaul:
Excessive Context Switching
If you find yourself constantly jumping between different tasks, tools, and mental frameworks, you’re experiencing context switching. Research shows that it can take up to 23 minutes to fully regain focus after an interruption. When developers switch contexts frequently, they lose valuable coding momentum.
For example, if you’re coding an algorithm solution, then stopping to check email, then returning to your code only to switch to a meeting, your brain never gets to operate at peak efficiency on any single task.
Repetitive Manual Tasks
Are you manually performing the same actions over and over? Whether it’s setting up development environments, running test suites, or deploying code, repetitive manual tasks are a red flag. Not only do they waste time, but they’re also prone to human error.
Inconsistent Code Quality
If your codebase quality varies wildly depending on who wrote it or when it was written, your workflow lacks standardization. This inconsistency makes maintenance more difficult and introduces potential bugs.
Frequent Blockers and Bottlenecks
Do you often find yourself waiting for others or for processes to complete before you can continue your work? These bottlenecks indicate workflow issues that need addressing.
Difficulty Onboarding New Team Members
When new developers struggle to get up to speed with your project, it often points to workflow documentation problems or overly complex processes.
Merge Conflicts and Integration Issues
Frequent code conflicts when merging branches suggest that your team’s collaboration workflow needs refinement.
Optimizing Your Development Environment
Your development environment is your digital workshop. Just as a carpenter needs a well-organized workspace with quality tools, you need a thoughtfully configured development environment.
IDE Optimization
Your Integrated Development Environment (IDE) should be customized to your specific needs:
- Keyboard shortcuts: Learn and customize shortcuts for common actions. The time saved adds up significantly.
- Extensions and plugins: Install only what you need. Too many extensions can slow down your IDE.
- Code snippets: Create templates for code patterns you use frequently.
- Theme and font: Choose options that reduce eye strain during long coding sessions.
For example, if you’re using VS Code for algorithmic problem solving, you might set up snippets for common patterns like:
// Binary search template
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
Terminal and Shell Configuration
A well-configured terminal can significantly speed up your workflow:
- Use a modern shell like Zsh with frameworks such as Oh My Zsh
- Configure aliases for common commands
- Implement auto-completion for faster navigation
- Use a multiplexer like tmux to manage multiple terminal sessions
Consider this example of useful aliases:
# Git aliases
alias gs='git status'
alias gc='git commit'
alias gp='git push'
alias gl='git pull'
# Project navigation
alias proj='cd ~/projects'
alias algo='cd ~/projects/algorithms'
Consistent Development Environments
Environment inconsistencies between team members or between development and production can cause the dreaded “it works on my machine” problem. Solutions include:
- Containerization: Using Docker to ensure consistent environments
- Virtual development environments: Tools like Vagrant or cloud development environments
- Configuration as code: Storing environment configurations in version control
Workspace Organization
An organized workspace helps maintain focus:
- Use multiple desktops/workspaces to separate different contexts
- Implement a consistent project structure across all your repositories
- Keep documentation close to the code it describes
- Consider the physical aspects of your workspace (monitor setup, desk organization, etc.)
Embracing Automation
Automation is perhaps the single most powerful way to improve workflow efficiency. Any task that’s performed more than once is a candidate for automation.
Build and Deployment Automation
Continuous Integration/Continuous Deployment (CI/CD) pipelines automate the build, test, and deployment processes:
- Automatically run tests when code is pushed
- Build artifacts and deploy to staging environments
- Perform code quality checks
Tools like GitHub Actions, Jenkins, CircleCI, or GitLab CI can handle these workflows. Here’s a simple example of a GitHub Actions workflow:
name: Node.js CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Use Node.js
uses: actions/setup-node@v3
with:
node-version: '16.x'
- run: npm ci
- run: npm test
- run: npm run build
Task Runners and Build Tools
Task runners automate repetitive development tasks:
- Compiling code
- Minifying assets
- Running tests
- Linting code
Popular tools include npm scripts, Gulp, Webpack, and Gradle. Here’s a simple npm scripts example:
{
"scripts": {
"start": "node server.js",
"dev": "nodemon server.js",
"test": "jest",
"lint": "eslint .",
"build": "webpack --mode production"
}
}
Code Generation and Scaffolding
Don’t write boilerplate code by hand. Use code generators and scaffolding tools to create initial project structures, components, or files:
- Create React App for React projects
- Angular CLI for Angular projects
- Spring Initializr for Spring applications
- Custom templates for repetitive code patterns
Scripting Routine Tasks
Create scripts for tasks you perform regularly. For example, a script to set up a new algorithm practice environment might:
- Create a new directory with the problem name
- Initialize necessary files (solution file, test file)
- Open the files in your editor
#!/bin/bash
# Script to create a new algorithm practice environment
if [ "$#" -ne 1 ]; then
echo "Usage: $0 problem_name"
exit 1
fi
mkdir -p "$1"
cd "$1"
# Create solution file
cat > solution.js << EOL
/**
* Problem: $1
*/
function solution() {
// Your code here
}
module.exports = solution;
EOL
# Create test file
cat > solution.test.js << EOL
const solution = require('./solution');
describe('$1', () => {
test('example case', () => {
expect(solution()).toBe();
});
});
EOL
# Open in VS Code
code .
echo "Created practice environment for $1"
Mastering Version Control
Version control is fundamental to modern development workflows. Git, the most widely used version control system, offers powerful capabilities that many developers underutilize.
Effective Branching Strategies
A clear branching strategy helps teams collaborate efficiently:
- Git Flow: A strict branching model with dedicated branches for features, releases, and hotfixes
- GitHub Flow: A simpler approach with feature branches merged directly to main after review
- Trunk-Based Development: Focuses on keeping the main branch always releasable
Choose a strategy that matches your team’s size and release cadence.
Meaningful Commits and Messages
Commit messages are documentation. They should clearly explain what changed and why:
- Use a consistent format (e.g., conventional commits)
- Write in the imperative (“Add feature” not “Added feature”)
- Reference issue or ticket numbers
- Separate subject from body with a blank line
Example of a good commit message:
feat(auth): implement JWT-based authentication
- Add JWT token generation on login
- Implement middleware for token verification
- Update user routes to use authentication
Resolves #123
Pull Request Workflows
Pull requests (PRs) facilitate code review and discussion:
- Keep PRs focused on a single concern
- Include clear descriptions of changes
- Add screenshots or videos for UI changes
- Use PR templates to ensure consistency
Git Hooks
Git hooks automate actions at specific points in the Git workflow:
- pre-commit: Run linters, formatters, or tests before commits
- pre-push: Run more comprehensive tests before pushing
- post-merge: Update dependencies after pulling changes
Tools like Husky make Git hooks easier to manage:
{
"husky": {
"hooks": {
"pre-commit": "lint-staged",
"pre-push": "npm test"
}
},
"lint-staged": {
"*.js": ["eslint --fix", "prettier --write"]
}
}
Implementing Effective Testing Strategies
Testing is not just about finding bugs; it’s about enabling confident, rapid development. A solid testing strategy is essential for maintaining workflow efficiency.
Test-Driven Development
Test-Driven Development (TDD) follows a simple cycle:
- Write a failing test for the functionality you want to implement
- Write the minimum code needed to make the test pass
- Refactor the code while ensuring tests still pass
TDD helps maintain focus and produces naturally testable code. For algorithm practice, writing tests first helps clarify the problem requirements:
// Testing a function that finds the two numbers in an array that sum to a target
test('twoSum should find indices of two numbers that add up to target', () => {
expect(twoSum([2, 7, 11, 15], 9)).toEqual([0, 1]);
expect(twoSum([3, 2, 4], 6)).toEqual([1, 2]);
expect(twoSum([3, 3], 6)).toEqual([0, 1]);
});
Balanced Testing Pyramid
A balanced testing approach includes:
- Unit tests: Testing individual functions or components in isolation
- Integration tests: Testing how components work together
- End-to-end tests: Testing complete user flows
The testing pyramid suggests having many unit tests, fewer integration tests, and even fewer end-to-end tests for optimal efficiency.
Automated Testing
Tests should run automatically:
- During development (via watch mode)
- Before commits (via pre-commit hooks)
- Before merging (via CI pipelines)
Test Coverage and Quality
Don’t just focus on test coverage percentages. Consider:
- Are you testing edge cases?
- Do your tests verify behavior, not implementation?
- Are your tests maintainable and readable?
Enhancing Team Collaboration
Even solo developers need to collaborate with their future selves. Effective collaboration practices keep workflows smooth and minimize confusion.
Clear Communication Channels
Establish when to use different communication methods:
- Chat: Quick questions and informal discussions
- Issue trackers: Feature requests, bug reports, and task tracking
- Documentation: Long-lasting knowledge that needs to be referenced
- Meetings: Complex discussions that require real-time interaction
Documentation as Part of the Workflow
Documentation should be created and updated as part of the development process, not as an afterthought:
- Use README files to explain project setup and basic usage
- Maintain API documentation (tools like Swagger or OpenAPI can help)
- Document design decisions and architectural choices
- Keep a changelog to track significant changes
Code Reviews
Effective code reviews improve code quality and share knowledge:
- Focus on readability, maintainability, and correctness
- Use automated tools to handle style and formatting issues
- Be constructive and specific in feedback
- Consider pair programming for complex features
Knowledge Sharing
Prevent knowledge silos through deliberate sharing:
- Regular tech talks or lunch-and-learns
- Rotating responsibilities
- Pair programming sessions
- Shared bookmarks and learning resources
Continuous Learning and Improvement
The most efficient workflows evolve over time through deliberate improvement.
Retrospectives
Regular retrospectives help identify areas for improvement:
- What went well in our recent work?
- What could be improved?
- What actions can we take to improve?
Even individual developers can benefit from personal retrospectives.
Learning Time
Allocate dedicated time for learning:
- Studying new technologies or techniques
- Taking online courses
- Reading technical books
- Practicing algorithm problems
Staying Updated
The tech landscape evolves rapidly. Stay current through:
- Following relevant blogs and newsletters
- Participating in communities
- Attending conferences or watching recordings
- Experimenting with new tools in side projects
Essential Tools for Modern Development Workflows
The right tools can dramatically improve workflow efficiency. Here are categories of tools every developer should consider:
IDE and Editor Extensions
- ESLint/TSLint: Catch errors and enforce coding standards
- Prettier: Automatic code formatting
- GitLens: Enhanced Git capabilities within the editor
- Code Snippets: Quick templates for common patterns
Terminal Tools
- Oh My Zsh: Enhanced terminal experience
- fzf: Fuzzy finder for quick file navigation
- tldr: Simplified, example-based man pages
- httpie or curl: API testing from the command line
Code Quality Tools
- SonarQube/SonarLint: Static code analysis
- Jest/Mocha/Pytest: Testing frameworks
- Codecov/Istanbul: Test coverage reporting
Collaboration Tools
- Jira/Trello/Asana: Task and project management
- Slack/Microsoft Teams: Team communication
- Notion/Confluence: Documentation and knowledge management
Development Environment Tools
- Docker: Containerization for consistent environments
- nvm/pyenv: Language version management
- Postman/Insomnia: API development and testing
Measuring and Tracking Workflow Improvements
To improve your workflow, you need to measure it. Here are metrics and approaches to consider:
Development Metrics
- Lead Time: Time from task creation to deployment
- Cycle Time: Time from starting work to completion
- Build Time: Duration of build and test processes
- Defect Rate: Number of bugs found after implementation
Personal Productivity Metrics
- Focus Time: Uninterrupted coding sessions
- Context Switches: Number of times you change tasks
- Learning Time: Hours dedicated to skill improvement
Tracking Tools
- Time tracking apps (Toggl, RescueTime)
- Project management metrics (Jira, GitHub insights)
- CI/CD pipeline metrics
- Personal journals or notes
Improvement Framework
Use a structured approach to improvement:
- Measure current state
- Identify bottlenecks or pain points
- Implement targeted improvements
- Measure results
- Repeat
Conclusion: Building Your Optimal Workflow
Improving your development workflow is not a one-time effort but an ongoing process. The most efficient workflows are those that evolve with your needs and incorporate new tools and practices as they become relevant.
Remember that the goal is not to adopt every best practice or use every tool mentioned in this guide. Instead, focus on identifying your specific pain points and addressing them systematically.
Start with small changes that offer the biggest impact:
- Automate one repetitive task
- Improve your environment setup
- Implement a testing strategy
- Enhance your version control practices
As you make these improvements, you’ll likely find that your coding becomes more enjoyable and your productivity increases. You’ll spend less time on mechanical tasks and more time solving interesting problems.
For those preparing for technical interviews or working on algorithm challenges, an efficient workflow is particularly valuable. It allows you to focus on understanding the problem and developing a solution rather than fighting with your tools or environment.
The journey to an optimal development workflow is continuous, but each step you take improves your effectiveness as a developer. Start today by identifying one aspect of your workflow that could be improved, and take action to address it.
Your future self will thank you for the investment in a more efficient, less frustrating development experience.
Next Steps
- Audit your current workflow to identify inefficiencies
- Choose one area from this guide to focus on first
- Implement changes incrementally
- Measure the impact of your changes
- Share your improvements with colleagues
By continuously refining your development workflow, you’ll not only become more productive but also enhance the quality of your code and reduce the mental overhead of your work. This leaves more cognitive resources available for solving complex problems and learning new skills, creating a virtuous cycle of improvement in your programming journey.