Why Your Development Tools Aren’t Improving Code Quality
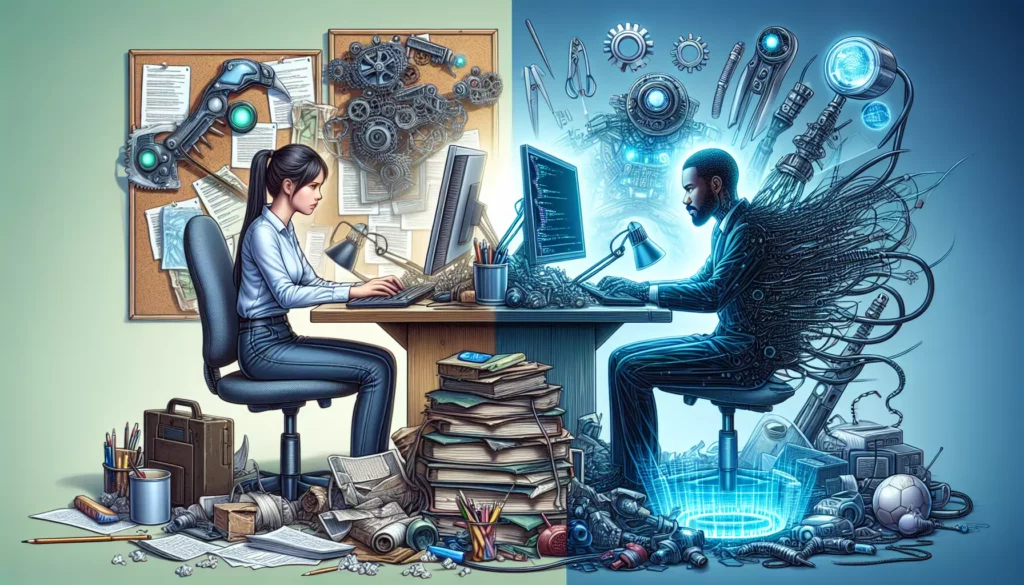
In today’s software development landscape, we’re inundated with tools promising to elevate our code quality. Static analyzers, linters, formatters, IDEs with AI assistants, and a plethora of testing frameworks all claim to be the silver bullet for writing better code. Yet many engineering teams still struggle with maintaining high quality standards in their codebases despite investing heavily in these tools.
The disconnect isn’t in the tools themselves but in how we approach code quality. At AlgoCademy, we’ve observed thousands of developers at various skill levels and found that true code quality improvements come from a deeper understanding of fundamental principles rather than just adding more tools to your workflow.
The False Promise of Tool-Driven Quality
Development tools can mask deeper issues in your approach to writing code. Let’s explore why relying solely on tools might not be delivering the quality improvements you expect.
Tools Address Symptoms, Not Root Causes
Most code quality tools excel at identifying specific patterns or issues in code. Linters flag style inconsistencies, static analyzers find potential bugs, and test coverage tools measure how much of your code is tested. However, these tools cannot address the fundamental understanding gaps that lead to poor design choices.
Consider this Python example that passes most linting checks but contains deeper issues:
def process_data(data):
result = []
for i in range(len(data)):
if data[i] is not None and data[i] > 0:
result.append(data[i] * 2)
else:
result.append(0)
return result
While this code might pass basic linting, it fails to use Pythonic patterns, creates unnecessary temporary lists, and uses an index-based loop instead of direct iteration. No linter will suggest the more elegant solution:
def process_data(data):
return [(x * 2 if x and x > 0 else 0) for x in data]
The root issue here isn’t about syntax or style, but about understanding Python’s idioms and design patterns.
The Illusion of Comprehensive Coverage
Many development teams put great faith in their test coverage metrics, aiming for that coveted 100% coverage. However, high test coverage doesn’t necessarily translate to high-quality code or fewer bugs.
Consider this JavaScript function with 100% test coverage:
function calculateDiscount(price, discountPercent) {
return price * (1 - discountPercent / 100);
}
You might test this with a few cases:
test('applies 20% discount correctly', () => {
expect(calculateDiscount(100, 20)).toBe(80);
});
test('applies 0% discount correctly', () => {
expect(calculateDiscount(100, 0)).toBe(100);
});
These tests give you 100% coverage, but they miss critical edge cases:
- What happens with negative prices?
- What about negative discount percentages?
- How does it handle discounts greater than 100%?
- What about non-numeric inputs?
The coverage metric gives a false sense of security while leaving significant vulnerabilities unaddressed.
Configuration Complexity
Many teams spend countless hours configuring tools rather than writing code. ESLint configurations can span hundreds of lines. CI/CD pipelines become increasingly complex. The cognitive overhead of maintaining these configurations can outweigh their benefits.
Consider this excerpt from a real-world ESLint configuration:
{
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended",
"prettier"
],
"plugins": [
"react",
"react-hooks",
"@typescript-eslint",
"prettier",
"import"
],
"rules": {
"no-console": ["error", { "allow": ["warn", "error"] }],
"no-unused-vars": "off",
"@typescript-eslint/no-unused-vars": ["error"],
"react/prop-types": "off",
"react-hooks/rules-of-hooks": "error",
"react-hooks/exhaustive-deps": "warn",
"prettier/prettier": ["error", {
"singleQuote": true,
"trailingComma": "all",
"bracketSpacing": true,
"jsxBracketSameLine": false,
"tabWidth": 2,
"semi": true
}],
// ... dozens more rules
}
}
The complexity of such configurations can become a burden, especially for new team members who need to understand why certain rules are in place.
The Knowledge Gap Problem
The core issue isn’t insufficient tooling but insufficient understanding. Let’s explore the knowledge gaps that tools can’t bridge.
Algorithmic Thinking
No amount of linting or testing can compensate for poor algorithmic choices. Consider this inefficient solution to finding duplicates in an array:
function findDuplicates(array) {
const duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
}
}
}
return duplicates;
}
This O(n²) solution with additional includes checks will perform poorly for large inputs. A developer with stronger algorithmic thinking would immediately recognize the more efficient approach:
function findDuplicates(array) {
const seen = new Set();
const duplicates = new Set();
for (const item of array) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return [...duplicates];
}
This O(n) solution demonstrates the value of algorithmic knowledge that no tool can provide automatically.
Design Pattern Literacy
Understanding when and how to apply design patterns separates proficient developers from novices. Consider this Java code that violates the Single Responsibility Principle:
public class UserManager {
private Database db;
public UserManager() {
this.db = new Database("jdbc:mysql://localhost:3306/users");
}
public User createUser(String name, String email) {
// Validate email
if (!email.matches("^[A-Za-z0-9+_.-]+@(.+)$")) {
throw new IllegalArgumentException("Invalid email format");
}
// Check if user exists
if (this.userExists(email)) {
throw new IllegalArgumentException("User already exists");
}
// Create user
User user = new User(name, email);
// Generate password and send email
String password = this.generateRandomPassword();
user.setPassword(this.hashPassword(password));
this.sendWelcomeEmail(user, password);
// Save to database
String query = "INSERT INTO users (name, email, password) VALUES (?, ?, ?)";
this.db.execute(query, user.getName(), user.getEmail(), user.getPassword());
return user;
}
private boolean userExists(String email) {
// Database query to check if user exists
return false;
}
private String generateRandomPassword() {
// Generate random password
return "randompassword";
}
private String hashPassword(String password) {
// Hash password
return "hashedpassword";
}
private void sendWelcomeEmail(User user, String password) {
// Send welcome email with password
}
}
This class violates multiple design principles by handling database connections, email validation, password generation, password hashing, and email sending. A more experienced developer would recognize the need to separate these concerns:
public class UserService {
private final UserRepository userRepository;
private final EmailValidator emailValidator;
private final PasswordService passwordService;
private final EmailService emailService;
public UserService(
UserRepository userRepository,
EmailValidator emailValidator,
PasswordService passwordService,
EmailService emailService) {
this.userRepository = userRepository;
this.emailValidator = emailValidator;
this.passwordService = passwordService;
this.emailService = emailService;
}
public User createUser(String name, String email) {
if (!emailValidator.isValid(email)) {
throw new IllegalArgumentException("Invalid email format");
}
if (userRepository.existsByEmail(email)) {
throw new IllegalArgumentException("User already exists");
}
User user = new User(name, email);
String rawPassword = passwordService.generateRandomPassword();
user.setPassword(passwordService.hash(rawPassword));
userRepository.save(user);
emailService.sendWelcomeEmail(user, rawPassword);
return user;
}
}
No static analyzer can transform the first approach into the second. This requires understanding design principles like Single Responsibility, Dependency Injection, and separation of concerns.
Testing Philosophy
Many developers struggle with writing meaningful tests that verify behavior rather than implementation. Consider these React component tests:
// Implementation-focused test
test('calls setCount when button is clicked', () => {
const setCount = jest.fn();
const { getByText } = render(<Counter count={0} setCount={setCount} />);
fireEvent.click(getByText('Increment'));
expect(setCount).toHaveBeenCalledWith(1);
});
// Behavior-focused test
test('increments the counter when increment button is clicked', () => {
const { getByText, getByTestId } = render(<App />);
expect(getByTestId('count-display')).toHaveTextContent('0');
fireEvent.click(getByText('Increment'));
expect(getByTestId('count-display')).toHaveTextContent('1');
});
The second test focuses on the actual behavior from the user's perspective, making it more resilient to implementation changes. Understanding this distinction comes from experience and testing philosophy, not from tools.
The Cultural Dimension
Beyond technical knowledge, organizational culture plays a crucial role in code quality that tools cannot address.
The Pressure of Deadlines
When teams face tight deadlines, quality often suffers. Developers might skip writing tests, take shortcuts, or ignore warnings from static analyzers. No tool can enforce quality standards when deadlines loom and management prioritizes shipping over correctness.
This common scenario leads to a cycle of technical debt:
- Deadline pressure leads to shortcuts
- Shortcuts create technical debt
- Technical debt slows future development
- Slower development creates more deadline pressure
Breaking this cycle requires cultural changes, not more tools.
The Code Review Ritual
Many teams treat code reviews as a checkbox rather than a learning opportunity. Reviewers might focus on superficial issues like formatting (which tools can handle) while missing architectural problems or design flaws.
Consider these two code review comments:
// Superficial feedback
"You should add a space after the if statement."
"Please use camelCase for variable names."
// Substantive feedback
"This method has too many responsibilities. Consider extracting the validation logic into a separate validator class."
"Using a recursive approach here could lead to stack overflow with large inputs. Consider an iterative solution instead."
The latter comments address fundamental issues that tools cannot easily detect and require human expertise to identify.
Knowledge Sharing Practices
In teams where knowledge is siloed, code quality suffers across the codebase. Senior developers might write high-quality code in their areas while other parts of the codebase deteriorate. Tools cannot bridge this gap without deliberate knowledge sharing practices:
- Pair programming sessions
- Architecture decision records
- Technical brown bags
- Mentorship programs
These practices distribute knowledge throughout the team in ways that tools cannot.
The Path Forward: Beyond Tools
If tools alone aren't the answer, what is? Let's explore strategies that actually improve code quality by addressing the root causes.
Invest in Developer Education
The most effective way to improve code quality is to improve developer knowledge. This means investing in:
- Algorithmic thinking training: Help developers understand time and space complexity and recognize common patterns.
- Design principles education: Teach SOLID principles, design patterns, and when to apply them.
- Language mastery: Encourage deep understanding of language features rather than superficial syntax knowledge.
At AlgoCademy, we've found that developers who master these fundamentals write higher-quality code regardless of the tools they use.
Embrace Deliberate Practice
Improving code quality requires deliberate practice, not just writing more code. This means:
- Code reviews with specific learning goals: "In this review, let's focus on error handling patterns."
- Refactoring exercises: Regular sessions where the team refactors existing code to apply new patterns or techniques.
- Coding challenges: Structured problems that emphasize specific skills like optimization or clean design.
These practices develop the judgment and intuition that tools cannot provide.
Cultivate a Quality-Focused Culture
Cultural changes that support quality include:
- Realistic scheduling: Build time for testing and refactoring into project timelines.
- Celebrating quality wins: Recognize improvements in code quality alongside feature deliveries.
- Continuous learning expectations: Make learning and improvement part of everyone's job description.
When quality becomes a shared value rather than an afterthought, tools become enablers rather than enforcers.
Use Tools Strategically
Tools still have their place, but they should be used strategically:
- Automate the mundane: Use formatters and linters to handle style consistency so humans can focus on substance.
- Measure what matters: Focus on meaningful metrics like defect rates rather than just test coverage.
- Customize judiciously: Adapt tools to your team's needs rather than adopting every available rule.
The goal is to let tools handle what they do best while preserving human judgment for what matters most.
Case Study: Transforming Code Quality at Scale
Let's look at how one organization transformed their approach to code quality by focusing beyond tools.
The Starting Point
A midsize fintech company was struggling with code quality despite having invested in:
- A comprehensive CI/CD pipeline
- Static analysis tools integrated into their workflow
- Strict code coverage requirements (85%+)
- Mandatory code reviews
Despite these measures, they faced recurring issues:
- Production bugs in critical flows
- Increasing technical debt
- Slow feature delivery
- Developer frustration
The Intervention
Rather than adding more tools, they implemented a knowledge-focused approach:
- Weekly learning sessions: Each team dedicated 2 hours per week to learning, rotating topics between algorithms, design patterns, and language features.
- Architectural decision records: Teams documented key design decisions with rationales, creating a knowledge base for future reference.
- Refactoring Fridays: Every other Friday was dedicated to paying down technical debt through focused refactoring.
- Mentorship pairs: Senior developers were paired with junior developers for regular knowledge transfer.
The Results
After six months, the company saw:
- 40% reduction in production defects
- Improved velocity in feature delivery
- Higher developer satisfaction and retention
- More consistent code quality across teams
Notably, they achieved these improvements without adding any new tools to their workflow. In fact, they simplified some of their tool configurations to focus on the most impactful rules.
Practical Steps to Improve Your Code Quality Today
Based on our experience at AlgoCademy and the case study above, here are practical steps you can take to improve code quality beyond tools:
For Individual Developers
- Study one design pattern each week: Pick a pattern, understand its use cases, and try to implement it in a small project.
- Practice algorithmic thinking: Solve one algorithm problem daily, focusing on efficiency and clarity.
- Read high-quality code: Study well-regarded open-source projects in your language to absorb good patterns.
- Refactor your old code: Revisit code you wrote months ago and improve it with your current knowledge.
- Write tests before fixing bugs: When you encounter a bug, write a failing test first to ensure you understand the issue.
For Team Leaders
- Implement learning rotations: Have team members take turns teaching concepts to the rest of the team.
- Start an architecture review board: Create a forum where significant design decisions are discussed and documented.
- Conduct targeted code reviews: Focus each review session on specific aspects like error handling or performance.
- Measure meaningful metrics: Track defect rates, time spent on maintenance, and developer satisfaction rather than just code coverage.
- Budget time for improvement: Explicitly allocate time in sprints for refactoring and learning.
For Organizations
- Invest in training: Provide access to courses, books, and conferences focused on fundamental skills.
- Recognize quality contributions: Create recognition programs for improvements in code quality and knowledge sharing.
- Support communities of practice: Foster cross-team groups focused on specific areas like testing or performance.
- Align incentives: Ensure that promotion criteria include code quality and mentorship, not just feature delivery.
- Create space for experimentation: Allow teams to try new approaches and learn from failures without penalty.
Conclusion: The Human Element in Code Quality
Tools are valuable allies in the quest for code quality, but they cannot replace the human elements that truly drive excellence: knowledge, judgment, communication, and culture. The best code emerges from environments where developers deeply understand fundamental principles, deliberately practice their craft, and work within cultures that value quality.
At AlgoCademy, we've observed that the most successful developers aren't those with the most elaborate tool chains but those who invest consistently in deepening their understanding of core concepts. They use tools as amplifiers of their knowledge rather than substitutes for it.
As you evaluate your approach to code quality, consider whether you're overinvesting in tools while underinvesting in the human factors that truly determine outcomes. The path to better code often leads not through more sophisticated tooling but through more sophisticated thinking.
Remember that great code is ultimately a product of great minds. When you invest in developing those minds, quality follows naturally, with or without the latest tools.
Further Resources
- Books:
- "Clean Code" by Robert C. Martin
- "Refactoring" by Martin Fowler
- "Design Patterns" by Gamma, Helm, Johnson, and Vlissides
- Online Learning:
- AlgoCademy's Algorithm Mastery course
- MIT OpenCourseWare's Design Patterns course
- Stanford's free Computer Science courses
- Communities:
- Stack Exchange Code Review
- Language-specific Discord communities
- Local meetup groups focused on software craftsmanship
The journey to better code quality is ongoing, but with a focus on the fundamentals rather than just tools, you'll build a foundation that serves you throughout your career.