Why Your Development Scripts Aren’t Automating Enough
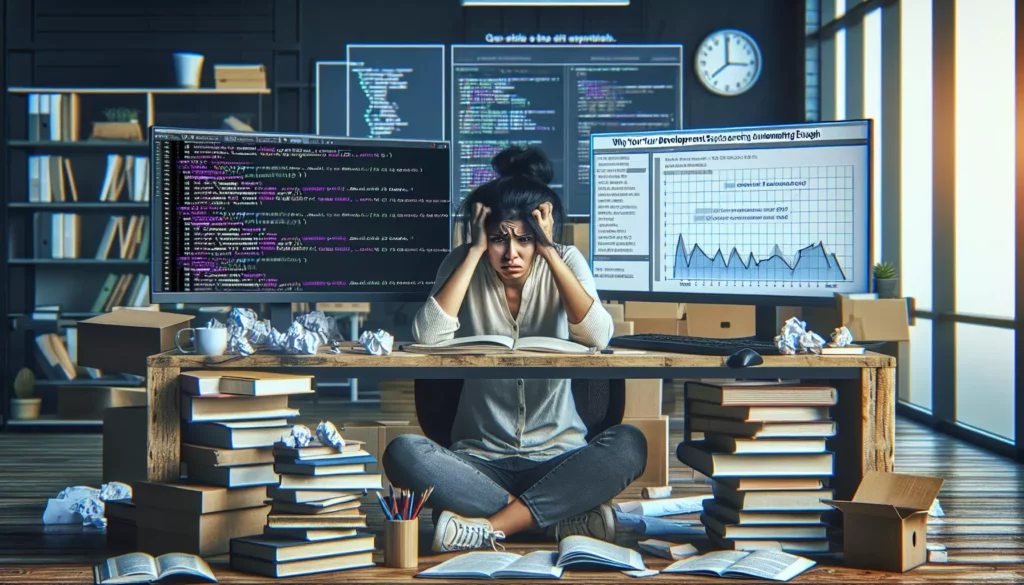
In the fast-paced world of software development, automation is not just a convenience—it’s a necessity. Development scripts serve as the backbone of modern software engineering workflows, handling everything from building and testing to deployment and monitoring. Yet, despite their critical role, many development teams find themselves manually performing tasks that could—and should—be automated.
If you’re a software engineer or part of a development team, you’ve likely experienced the frustration of repetitive tasks consuming valuable time that could be spent on more creative and impactful work. The question is: why aren’t your development scripts doing more?
In this comprehensive guide, we’ll explore the common pitfalls of development scripts, identify opportunities for enhanced automation, and provide practical strategies to transform your development workflow into a more efficient, error-resistant machine.
The Current State of Development Automation
Before diving into the problems, let’s establish a baseline understanding of what development scripts typically automate today:
- Building code and compiling assets
- Running test suites
- Deploying code to various environments
- Database migrations and schema updates
- Environment setup and configuration
- Dependency management
While these represent significant progress from the manual processes of the past, they often represent just the tip of the automation iceberg. Many teams stop at these basic automations, missing opportunities to streamline their workflow further.
Signs Your Scripts Aren’t Automating Enough
How do you know if your development scripts are underperforming? Look for these telltale signs:
1. Developers Perform Repetitive Tasks Manually
If your team members frequently perform the same sequence of commands or actions, that’s a clear indication that automation is lacking. Every repetitive task is an opportunity for a script.
2. Onboarding New Team Members Takes Days
When bringing new developers onto your project requires extensive documentation reading and manual setup processes, your automation is insufficient. Ideally, a new team member should be able to run a single command to set up their development environment.
3. Frequent Human Errors in Routine Processes
If your team regularly encounters issues due to missed steps in a process or inconsistent execution of tasks, automation could eliminate these problems entirely.
4. Long Feedback Cycles
When developers have to wait significant time between making changes and seeing the results, it often indicates insufficient automation in the testing and feedback processes.
5. Deployment Anxiety
If deployments cause stress and require numerous manual checks and balances, your deployment automation needs improvement.
Common Limitations in Development Scripts
Understanding why development scripts often fall short is the first step toward improving them. Here are the most common limitations:
1. Script Fragmentation
Many development environments suffer from script fragmentation—having dozens of small scripts that each handle a specific task but don’t integrate well with each other. This creates a disjointed workflow where developers must manually chain scripts together.
For example, a team might have separate scripts for:
- Setting up the development environment
- Building the application
- Running tests
- Deploying to staging
- Deploying to production
Without integration, developers must manually execute each script in sequence, waiting for one to complete before starting the next. This introduces opportunities for error and wastes time.
2. Insufficient Error Handling
Many scripts are written with the “happy path” in mind—they work perfectly when everything goes as expected but fail spectacularly when encountering unexpected conditions. Robust error handling is often an afterthought, leading to scripts that:
- Fail silently without indicating what went wrong
- Leave systems in inconsistent states after failure
- Provide cryptic error messages that don’t help resolve the issue
- Lack retry mechanisms for transient failures
3. Platform Dependency
Scripts that work on one developer’s machine but fail on another’s are a common source of frustration. This usually stems from implicit dependencies on:
- Specific operating systems
- Locally installed tools and their versions
- Environment variables that aren’t explicitly set
- Filesystem paths and assumptions
4. Poor Documentation and Discoverability
Even when useful scripts exist, team members may not know about them or understand how to use them effectively. This leads to the paradoxical situation where automation exists but isn’t utilized.
5. Limited Scope
Many development scripts focus solely on the build-test-deploy pipeline, ignoring numerous other aspects of the development process that could benefit from automation, such as:
- Code quality checks
- Documentation generation
- Performance monitoring and alerting
- Data generation for testing
- Environment cleanup and maintenance
Untapped Automation Opportunities
Now that we’ve identified common limitations, let’s explore areas where additional automation could significantly improve your development workflow:
1. Development Environment Setup and Maintenance
The ideal development environment setup should be a one-command process that handles everything from code checkout to dependency installation. Consider automating:
- Installation of required tools and dependencies
- Configuration of development databases with test data
- Setting up local services (cache, search, etc.)
- Applying proper configuration for local development
- Regular updates of dependencies and tools
Example of a comprehensive setup script in Python:
#!/usr/bin/env python3
import os
import subprocess
import sys
def run_command(command, error_message):
result = subprocess.run(command, shell=True)
if result.returncode != 0:
print(f"Error: {error_message}")
sys.exit(1)
def main():
print("Setting up development environment...")
# Clone repository if not already done
if not os.path.exists("./src"):
run_command(
"git clone https://github.com/your-org/your-project.git src",
"Failed to clone repository"
)
# Install dependencies
run_command(
"cd src && npm install",
"Failed to install Node.js dependencies"
)
# Set up database
run_command(
"docker-compose up -d database",
"Failed to start database container"
)
# Run migrations
run_command(
"cd src && npm run migrate",
"Failed to run database migrations"
)
# Seed with test data
run_command(
"cd src && npm run seed",
"Failed to seed database with test data"
)
print("Development environment ready! Start the application with:")
print("cd src && npm start")
if __name__ == "__main__":
main()
2. Intelligent Testing Workflows
Basic test automation runs all tests every time, but more sophisticated approaches can save significant time:
- Test impact analysis to run only tests affected by recent changes
- Parallelization of tests across multiple cores or machines
- Prioritization of tests based on historical failure rates
- Automatic retry of flaky tests
- Visual regression testing for UI components
3. Code Quality Automation
Beyond simple linting, automate comprehensive code quality checks:
- Static analysis to identify potential bugs and security vulnerabilities
- Complexity analysis to highlight code that needs refactoring
- Dependency vulnerability scanning
- Code style enforcement
- Automated code reviews for common issues
A pre-commit hook script that runs various code quality checks:
#!/bin/bash
echo "Running pre-commit checks..."
# Run linter
echo "Running ESLint..."
npx eslint --fix .
if [ $? -ne 0 ]; then
echo "ESLint found issues that couldn't be automatically fixed."
exit 1
fi
# Run type checking
echo "Running TypeScript compiler..."
npx tsc --noEmit
if [ $? -ne 0 ]; then
echo "TypeScript found type errors."
exit 1
fi
# Run unit tests related to changed files
echo "Running tests for changed files..."
CHANGED_FILES=$(git diff --cached --name-only --diff-filter=ACM | grep -E '\.ts$|\.tsx$')
if [ -n "$CHANGED_FILES" ]; then
npx jest --findRelatedTests $CHANGED_FILES
if [ $? -ne 0 ]; then
echo "Tests failed for changed files."
exit 1
fi
fi
# Check for dependency vulnerabilities
echo "Checking for vulnerable dependencies..."
npm audit --production
if [ $? -ne 0 ]; then
echo "Security vulnerabilities found in dependencies."
echo "Run 'npm audit fix' to attempt automatic fixes."
exit 1
fi
echo "All pre-commit checks passed!"
exit 0
4. Documentation Generation
Documentation that’s separate from code quickly becomes outdated. Automate documentation to keep it in sync:
- API documentation generated from code comments or annotations
- README updates based on project structure and dependencies
- Release notes compiled from commit messages
- Architecture diagrams updated based on code changes
- Usage examples verified through tests
5. Monitoring and Feedback Systems
Automation shouldn’t stop at deployment—extend it to monitoring and feedback:
- Automatic performance regression detection
- User experience monitoring and alerting
- A/B test setup and analysis
- Automatic rollback triggered by error rate spikes
- Usage analytics collection and reporting
6. Cross-functional Workflow Integration
Development doesn’t happen in isolation. Automate the integration with other parts of the organization:
- Ticket status updates based on code changes
- Stakeholder notifications for feature completions
- Release announcement generation
- Customer support briefings about new features
- Marketing material updates based on new functionality
Best Practices for More Effective Development Scripts
To overcome the limitations discussed earlier and tap into these opportunities, follow these best practices when creating development scripts:
1. Design for Composability
Create scripts that do one thing well and can be easily combined with others. This Unix philosophy enables complex workflows through simple building blocks.
For example, instead of a monolithic deployment script, create separate scripts for:
- Building the application
- Running tests
- Creating deployment artifacts
- Uploading artifacts to a repository
- Deploying artifacts to an environment
- Verifying deployment success
Then create a master script that composes these components but still allows individual execution when needed.
2. Implement Robust Error Handling
Scripts should fail loudly and clearly, providing actionable information about what went wrong and how to fix it.
Key principles for error handling in scripts:
- Set error flags like
set -e
in Bash to fail on any error - Include context in error messages
- Implement rollback procedures for failed operations
- Log detailed information about the environment when errors occur
- Consider retry logic for operations that might fail due to transient issues
Example of improved error handling in a Bash script:
#!/bin/bash
# Exit immediately if a command exits with a non-zero status
set -e
# Pipe failures are also treated as errors
set -o pipefail
# Function to handle errors
handle_error() {
local line=$1
local command=$2
local code=$3
echo "Error on line $line: Command '$command' exited with status $code"
# Perform cleanup if necessary
if [ -d "./temp_build" ]; then
echo "Cleaning up temporary build directory..."
rm -rf ./temp_build
fi
# Notify the team if this is a critical script
if [ "$NOTIFY_ON_FAILURE" = "true" ]; then
curl -X POST -H "Content-Type: application/json" \
-d "{\"text\":\"Build script failed: $command exited with status $code\"}" \
$SLACK_WEBHOOK_URL
fi
exit $code
}
# Set up error trap
trap 'handle_error $LINENO "$BASH_COMMAND" $?' ERR
# Script logic begins
echo "Starting build process..."
# Create temporary directory
mkdir -p ./temp_build
# Install dependencies
echo "Installing dependencies..."
npm install --no-audit --no-fund || { echo "Dependency installation failed"; exit 1; }
# Run tests
echo "Running tests..."
npm test || { echo "Tests failed"; exit 1; }
# Build the application
echo "Building application..."
npm run build --production || { echo "Build failed"; exit 1; }
# Deploy if all previous steps succeeded
echo "Deploying application..."
./scripts/deploy.sh || { echo "Deployment failed"; exit 1; }
echo "Build and deploy completed successfully!"
3. Ensure Platform Independence
Scripts should work consistently across different environments by:
- Using containerization (Docker) to encapsulate dependencies
- Explicitly checking for and installing required tools
- Using relative paths and environment variables instead of hardcoded paths
- Testing scripts on different platforms (Windows, macOS, Linux)
- Documenting any platform-specific requirements or limitations
4. Improve Discoverability and Documentation
Make scripts easy to find and use:
- Implement a standardized command structure (e.g.,
./scripts/run.sh [command]
) - Include self-documentation capabilities (
--help
flags) - Create a central registry of available scripts
- Add descriptive comments explaining what each script does and how to use it
- Use tools like
make
or npm scripts to provide a consistent interface
Example of a self-documenting Makefile:
# Makefile for development workflow
.PHONY: help setup develop test build deploy clean
help: ## Show this help message
@echo 'Usage: make [target]'
@echo ''
@echo 'Targets:'
@grep -E '^[a-zA-Z_-]+:.*?## .*$$' $(MAKEFILE_LIST) | sort | awk 'BEGIN {FS = ":.*?## "}; {printf "\033[36m%-30s\033[0m %s\n", $$1, $$2}'
setup: ## Set up development environment
@echo "Setting up development environment..."
./scripts/setup.sh
develop: ## Start development server
@echo "Starting development server..."
npm run dev
test: ## Run test suite
@echo "Running tests..."
npm test
build: ## Build for production
@echo "Building for production..."
npm run build
deploy: build ## Deploy to production
@echo "Deploying to production..."
./scripts/deploy.sh production
clean: ## Clean up build artifacts
@echo "Cleaning up..."
rm -rf node_modules build dist .cache
# Default target
.DEFAULT_GOAL := help
5. Implement Comprehensive Logging
Good logging is essential for troubleshooting and understanding script execution:
- Log the start and completion of each major step
- Include timestamps in log messages
- Use different log levels (info, warning, error) appropriately
- Store logs in a centralized location
- Implement log rotation for scripts that generate large logs
6. Use Configuration Over Hardcoding
Scripts should be configurable without code changes:
- Use configuration files for environment-specific settings
- Support command-line arguments for runtime configuration
- Implement reasonable defaults that can be overridden
- Use environment variables for sensitive information
- Validate configuration before proceeding with execution
Advanced Automation Techniques
For teams ready to take their automation to the next level, consider these advanced techniques:
1. Meta-Automation: Automating the Creation of Automation
Create tools that generate scripts and configurations based on project analysis. For example:
- Scaffolding tools that create boilerplate code and associated test scripts
- Configuration generators that analyze your project and create optimal build configurations
- Script templates that enforce best practices and standardization
2. Event-Driven Automation
Instead of manually triggering scripts, implement event listeners that automatically respond to changes:
- File watchers that trigger builds when source files change
- Repository webhooks that initiate processes on commit or pull request
- Monitoring systems that trigger remediation scripts when anomalies are detected
3. Machine Learning for Optimization
Apply machine learning to optimize your automation:
- Predictive test selection based on code changes and historical test results
- Resource allocation optimization for build and test processes
- Anomaly detection in build and deployment metrics
- Failure prediction and preemptive remediation
4. Declarative Automation
Shift from imperative scripts (specifying how to do something) to declarative configurations (specifying what you want):
- Infrastructure as Code (IaC) for environment provisioning
- Pipeline as Code for CI/CD processes
- Policy as Code for governance and compliance
Example of declarative CI/CD with GitHub Actions:
name: CI/CD Pipeline
on:
push:
branches: [ main, develop ]
pull_request:
branches: [ main, develop ]
jobs:
quality:
name: Code Quality
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '16'
cache: 'npm'
- name: Install dependencies
run: npm ci
- name: Lint code
run: npm run lint
- name: Check formatting
run: npm run format:check
- name: Check types
run: npm run type-check
- name: Security audit
run: npm audit --production
test:
name: Test
needs: quality
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '16'
cache: 'npm'
- name: Install dependencies
run: npm ci
- name: Run unit tests
run: npm test -- --coverage
- name: Upload coverage
uses: codecov/codecov-action@v3
build:
name: Build
needs: test
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '16'
cache: 'npm'
- name: Install dependencies
run: npm ci
- name: Build application
run: npm run build
- name: Upload build artifacts
uses: actions/upload-artifact@v3
with:
name: build
path: build/
deploy:
name: Deploy
if: github.event_name == 'push' && github.ref == 'refs/heads/main'
needs: build
runs-on: ubuntu-latest
environment: production
steps:
- uses: actions/checkout@v3
- name: Download build artifacts
uses: actions/download-artifact@v3
with:
name: build
path: build/
- name: Deploy to production
run: |
echo "Deploying to production..."
# Deployment commands here
Implementing a Culture of Automation
Technical solutions alone aren’t enough—creating a culture that values and prioritizes automation is essential:
1. Allocate Time for Automation
Explicitly budget time for creating and improving automation:
- Include automation tasks in sprint planning
- Set aside “automation Fridays” or similar dedicated time
- Recognize and reward automation contributions
2. Establish Automation Standards
Create guidelines and standards for automation in your organization:
- Script naming conventions
- Documentation requirements
- Testing expectations for automation itself
- Error handling and logging standards
- Security considerations
3. Measure Automation ROI
Track and communicate the benefits of automation:
- Time saved through automated processes
- Reduction in errors and incidents
- Faster feedback cycles
- Improved developer satisfaction
4. Create Automation Champions
Designate team members to advocate for and support automation efforts:
- Provide training and resources
- Review and improve existing automation
- Help other team members create effective scripts
- Stay current with automation best practices and tools
Case Study: Transforming Development Workflow Through Enhanced Automation
Let’s examine a real-world example of how enhanced automation transformed a development team’s workflow:
Before: Manual Processes and Basic Scripts
A mid-sized software company with 50 developers was experiencing:
- 2-3 day onboarding process for new developers
- 45-minute average build and test cycle
- Weekly deployments that often failed and required manual intervention
- Frequent merge conflicts and integration issues
- Inconsistent code quality across the codebase
Their automation was limited to basic build scripts and a simple CI pipeline that ran all tests on every commit.
The Automation Initiative
The team embarked on a three-month initiative to enhance their automation:
- Month 1: Created a comprehensive development environment setup script that reduced onboarding to 2 hours
- Month 2: Implemented intelligent test selection and parallelization, reducing test time by 70%
- Month 3: Developed a fully automated deployment pipeline with canary deployments and automatic rollback
After: Transformed Development Experience
Six months after implementing enhanced automation:
- Onboarding time decreased from 2-3 days to 2 hours
- Build and test cycle reduced from 45 minutes to 12 minutes
- Deployment frequency increased from weekly to daily
- Deployment failures decreased by 80%
- Code quality improved significantly due to automated checks
- Developer satisfaction scores increased by 35%
The ROI was clear: The three months spent on automation improvements saved an estimated 20 developer-months within the first year.
Tools to Enhance Your Automation
Several tools can help you implement the automation strategies discussed in this article:
Build and Task Automation
- Make: The classic build automation tool
- Gradle: Flexible build automation system
- npm/yarn scripts: Task runners for JavaScript projects
- Gulp/Grunt: JavaScript task runners
- Rake: Ruby build program similar to Make
Containerization and Environment Management
- Docker: Container platform for consistent environments
- Docker Compose: Multi-container application orchestration
- Vagrant: Virtual machine management for development environments
- nvm/rbenv/pyenv: Language version managers
CI/CD Platforms
- Jenkins: Automation server with extensive plugin ecosystem
- GitHub Actions: CI/CD integrated with GitHub
- GitLab CI: CI/CD integrated with GitLab
- CircleCI: Cloud-based CI/CD service
- Travis CI: Distributed CI service
Testing and Quality Assurance
- Jest: JavaScript testing framework with test selection capabilities
- Cypress: End-to-end testing framework
- SonarQube: Continuous code quality platform
- ESLint/Prettier: Code quality and formatting tools
- Storybook: UI component development and testing
Infrastructure as Code
- Terraform: Infrastructure provisioning across cloud providers
- AWS CloudFormation: Infrastructure as code for AWS
- Pulumi: Infrastructure as code using programming languages
- Ansible: Configuration management and deployment
Conclusion: The Path to Automation Excellence
Development scripts that don’t automate enough are costing your team valuable time, introducing unnecessary risks, and limiting your productivity. By identifying the gaps in your current automation, implementing the best practices outlined in this article, and fostering a culture that values automation, you can transform your development workflow.
Remember that automation is not a one-time effort but an ongoing process of improvement. Start with the highest-impact areas, measure the results, and continuously expand your automation coverage. Each manual task eliminated is time returned to your team for more creative and valuable work.
The most successful development teams differentiate themselves not by working harder but by working smarter—and comprehensive automation is a cornerstone of working smarter in software development.
Begin your automation journey today by identifying one repetitive task your team performs manually and creating a script to automate it. From there, gradually expand your automation coverage until your development workflow becomes a model of efficiency and reliability.
Your future self and team members will thank you for the time and frustration saved through thoughtful, comprehensive automation.