Why Your Development Environment Looks Professional But Your Code Doesn’t
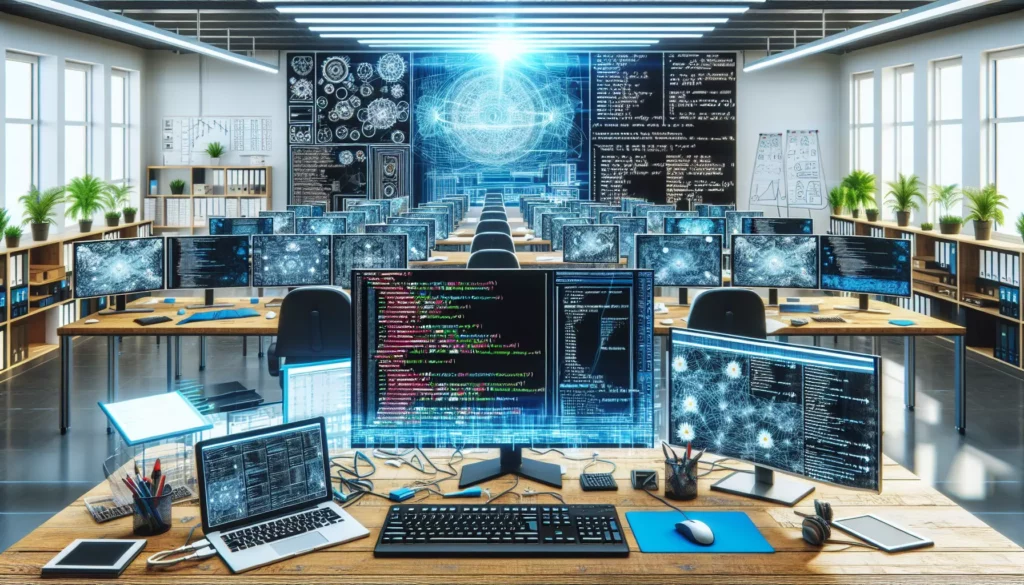
In the world of software development, appearances can be deceiving. Many developers spend hours perfecting their development environment with color-coordinated themes, carefully chosen fonts, and an array of powerful extensions. Yet, when it comes to the actual code they write, the quality often falls short of the professional look they’ve cultivated around it.
This disconnect between a polished development environment and subpar code quality is more common than you might think. It’s like having a state-of-the-art kitchen but still making mediocre meals. The tools alone don’t make you a chef, just as a fancy IDE doesn’t automatically make you write better code.
In this comprehensive guide, we’ll explore why this phenomenon occurs, how to identify if you’ve fallen into this trap, and most importantly, how to ensure your code lives up to the professional standards suggested by your development setup.
The Allure of the Perfect Development Environment
Let’s start by understanding why developers invest so much time in customizing their development environments.
The Visual Appeal Factor
There’s something deeply satisfying about a well-configured IDE with a sleek dark theme, colorful syntax highlighting, and custom font. It feels professional, and it’s visually appealing to work in such an environment for hours on end.
Social media and developer communities have amplified this trend. Platforms like GitHub, Twitter, and Reddit are filled with screenshots of beautiful development setups that garner likes and comments. This visual sharing culture has turned the development environment into a form of self-expression and professional identity.
The Productivity Promise
Customizing your development environment isn’t just about aesthetics. Many developers genuinely believe that the right setup will significantly boost their productivity. And there’s truth to this belief. Features like:
- Intelligent code completion
- Integrated debugging tools
- Git integration
- Keyboard shortcuts
- Multiple terminal instances
These can indeed make you more efficient. However, these tools only enhance existing skills; they don’t replace them.
Tool Acquisition as Skill Substitution
There’s a psychological comfort in acquiring new tools instead of building new skills. Installing a linting extension takes minutes, while learning proper code structure takes months or years of practice. This can lead to what I call “tool accumulation syndrome” where developers continuously add new extensions and configurations without correspondingly improving their coding abilities.
Signs Your Code Doesn’t Match Your Environment
How do you know if you’ve fallen into the trap of having a professional-looking environment but substandard code? Here are some telltale signs:
Inconsistent Naming Conventions
One of the most basic indicators is inconsistent naming throughout your codebase. Despite having tools that can automatically format your code, you might still see a mix of:
- camelCase, snake_case, and PascalCase used interchangeably
- Abbreviated variable names in some places and verbose ones in others
- Inconsistent prefixes or suffixes for similar types of variables
For example, look at this JavaScript function:
function get_user_data(userId) {
const UserName = fetchUserName(userId);
const userAddress = fetchAddress(userId);
const u_age = calculateAge(userId);
return {
name: UserName,
addr: userAddress,
age: u_age
};
}
Despite potentially being written in a beautiful VS Code setup with perfect syntax highlighting, this function exhibits poor naming consistency that makes it harder to read and maintain.
Lack of Documentation
Another sign is sparse or nonexistent documentation. Professional code should be well-documented, not just with comments explaining complex logic, but also with:
- Function and method documentation that explains purpose, parameters, and return values
- Module-level documentation explaining responsibilities
- Architecture documentation showing how components interact
If your only documentation is a hastily written README.md file, that’s a red flag.
Poor Error Handling
Novice code often handles the “happy path” but neglects proper error handling. Consider this Python example:
def process_data(file_path):
data = open(file_path).read()
processed = data.split(',')
result = [float(x) * 2 for x in processed]
return result
This function will crash if:
- The file doesn’t exist
- The file can’t be read due to permissions
- The data isn’t comma-separated
- Any element can’t be converted to a float
A more robust version would include proper error handling:
def process_data(file_path):
try:
with open(file_path, 'r') as file:
data = file.read()
if not data:
return []
processed = data.split(',')
result = []
for item in processed:
try:
result.append(float(item) * 2)
except ValueError:
logger.warning(f"Could not convert '{item}' to float, skipping")
return result
except FileNotFoundError:
logger.error(f"File not found: {file_path}")
return []
except PermissionError:
logger.error(f"Permission denied for file: {file_path}")
return []
except Exception as e:
logger.error(f"Unexpected error processing file: {str(e)}")
return []
Lack of Testing
A beautiful IDE filled with code that has no tests is like a shiny car with no safety features. Professional code includes:
- Unit tests for individual functions and methods
- Integration tests for component interactions
- End-to-end tests for critical user journeys
- Test coverage reporting
If you’re not writing tests, it doesn’t matter how nice your terminal font looks.
Overengineering Simple Problems
Ironically, developers with fancy setups sometimes overengineer simple solutions. They might use complex design patterns, unnecessary abstractions, or the latest framework features for problems that could be solved more simply.
For instance, creating a microservice architecture for a small application that could be a monolith, or implementing a complex state management solution for a simple form.
The Disconnect: Why It Happens
Understanding why this disconnect occurs can help address the root causes:
Instant Gratification vs. Long-Term Learning
Customizing your development environment provides immediate visual feedback and satisfaction. You can install a theme and see the results instantly. Learning to write clean, maintainable code takes much longer and doesn’t provide the same immediate dopamine hit.
Focusing on Tools Instead of Fundamentals
Many developers, especially those who are self-taught or come from bootcamps, may focus on learning specific tools and technologies rather than fundamental programming principles. They know how to use React, but not when to use the Adapter pattern or how to properly structure a codebase.
The Dunning-Kruger Effect
The Dunning-Kruger effect suggests that beginners often overestimate their abilities. A developer might believe their code is excellent because they don’t yet have the knowledge to recognize its flaws. Their beautiful IDE gives them a false sense of professionalism that their code doesn’t yet merit.
Prioritizing Speed Over Quality
In many work environments, especially startups or companies with tight deadlines, there’s pressure to deliver quickly. This can lead developers to prioritize getting something working over doing it right, resulting in technical debt that accumulates over time.
Bridging the Gap: Aligning Code Quality with Your Environment
Now that we understand the problem, let’s look at practical steps to ensure your code lives up to the professional standards suggested by your development setup.
Invest in Learning Fundamentals
Before diving into another IDE extension, consider investing that time in strengthening your programming fundamentals:
- Data Structures and Algorithms: Understanding these fundamentals helps you write more efficient code
- Design Patterns: Learn common patterns to solve recurring problems elegantly
- Clean Code Principles: Study books like Robert C. Martin’s “Clean Code” to understand what makes code maintainable
- System Design: Learn how to architect solutions that scale and remain maintainable
Platforms like AlgoCademy can be invaluable here, providing structured learning paths that focus on building these fundamental skills rather than just teaching you how to use the latest framework.
Establish and Follow Coding Standards
Coding standards provide a framework for consistent, readable code. They cover aspects like:
- Naming conventions for variables, functions, classes, etc.
- Code formatting (indentation, line length, etc.)
- Comment and documentation requirements
- File organization
- Error handling practices
Many languages and frameworks have established style guides you can adopt:
- JavaScript: Airbnb JavaScript Style Guide or Google JavaScript Style Guide
- Python: PEP 8
- Java: Google Java Style Guide
- Ruby: The Ruby Style Guide
The good news is that once you’ve chosen a style guide, you can configure many of those fancy IDE extensions to help enforce it!
Leverage Tools for Improvement, Not Just Appearance
Your development environment should do more than just look pretty. Use tools that actively improve your code quality:
- Linters: Tools like ESLint, Pylint, or RuboCop can catch potential bugs and style issues
- Static Analysis Tools: SonarQube, CodeClimate, or similar tools can identify code smells and security vulnerabilities
- Code Formatters: Prettier, Black, or Google’s Java Format can automatically format your code to meet style guidelines
- Test Runners: Configure your IDE to run tests automatically when you save files
For example, in VS Code, you might set up:
{
"editor.formatOnSave": true,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true
},
"eslint.validate": ["javascript", "typescript"],
"editor.defaultFormatter": "esbenp.prettier-vscode"
}
This configuration will automatically format your JavaScript and TypeScript files using Prettier and fix ESLint issues whenever you save a file.
Practice Code Reviews
Code reviews are one of the most effective ways to improve code quality. They provide:
- External validation of your code’s quality
- Opportunities to learn from more experienced developers
- A chance to explain and defend your design decisions
- Exposure to different approaches and perspectives
If you’re working solo, consider:
- Joining open source projects where your code will be reviewed
- Finding a coding buddy or mentor for reciprocal reviews
- Using platforms like CodeReview Stack Exchange
- Reviewing your own code after a few days away from it
Build a Testing Discipline
Testing is a crucial aspect of professional code development. Start building a testing discipline by:
- Learning test-driven development (TDD) practices
- Setting coverage goals for your codebase
- Writing tests for bugs before fixing them
- Including test requirements in your definition of “done” for any feature
A simple example of a unit test in JavaScript using Jest:
// Function to test
function sum(a, b) {
return a + b;
}
// Test suite
describe('sum function', () => {
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
test('adds negative numbers correctly', () => {
expect(sum(-1, -2)).toBe(-3);
});
test('adds zero correctly', () => {
expect(sum(0, 5)).toBe(5);
});
});
Refactor Regularly
Refactoring is the process of restructuring existing code without changing its external behavior. Regular refactoring helps keep code clean and prevents technical debt from accumulating.
Some refactoring practices to adopt:
- Schedule regular “refactoring days” where you focus solely on improving existing code
- Follow the “boy scout rule”: Always leave the code better than you found it
- Use the “rule of three”: When you see the same pattern three times, it’s time to refactor it into a reusable component
- Break down large functions or methods into smaller, more focused ones
Learn From Real-World Codebases
One of the best ways to improve your code quality is to study high-quality codebases. Look at popular open-source projects known for good code quality:
- React for JavaScript
- Django for Python
- Ruby on Rails for Ruby
- Spring for Java
Pay attention to how these projects:
- Structure their code
- Name variables and functions
- Document code
- Handle errors
- Write tests
Common Code Quality Issues and How to Fix Them
Let’s look at some common code quality issues and how to address them.
Function Bloat
Functions that do too much are hard to understand, test, and maintain.
Before:
function processUserData(userId) {
// Fetch user data
const userData = fetchUserFromDatabase(userId);
// Validate user data
if (!userData.name || !userData.email) {
throw new Error('Invalid user data');
}
// Format data for display
const formattedName = userData.name.toUpperCase();
const formattedEmail = userData.email.toLowerCase();
// Calculate user statistics
const postCount = countUserPosts(userId);
const commentCount = countUserComments(userId);
const totalEngagement = postCount + commentCount;
// Generate report
const report = `
User: ${formattedName}
Email: ${formattedEmail}
Posts: ${postCount}
Comments: ${commentCount}
Total Engagement: ${totalEngagement}
`;
// Save report to database
saveReportToDatabase(userId, report);
// Send email notification
sendEmailNotification(userData.email, 'Your user report is ready', report);
return report;
}
After refactoring:
function fetchAndValidateUser(userId) {
const userData = fetchUserFromDatabase(userId);
if (!userData.name || !userData.email) {
throw new Error('Invalid user data');
}
return userData;
}
function formatUserData(userData) {
return {
name: userData.name.toUpperCase(),
email: userData.email.toLowerCase()
};
}
function calculateUserStatistics(userId) {
const postCount = countUserPosts(userId);
const commentCount = countUserComments(userId);
return {
posts: postCount,
comments: commentCount,
totalEngagement: postCount + commentCount
};
}
function generateUserReport(formattedData, statistics) {
return `
User: ${formattedData.name}
Email: ${formattedData.email}
Posts: ${statistics.posts}
Comments: ${statistics.comments}
Total Engagement: ${statistics.totalEngagement}
`;
}
function processUserData(userId) {
const userData = fetchAndValidateUser(userId);
const formattedData = formatUserData(userData);
const statistics = calculateUserStatistics(userId);
const report = generateUserReport(formattedData, statistics);
saveReportToDatabase(userId, report);
sendEmailNotification(userData.email, 'Your user report is ready', report);
return report;
}
The refactored version is more modular, easier to test, and each function has a single responsibility.
Inconsistent Error Handling
Inconsistent error handling can lead to unpredictable application behavior.
Before:
function getUserData(userId) {
try {
return database.fetchUser(userId);
} catch (error) {
console.log('Error fetching user:', error);
}
}
function getUserPosts(userId) {
try {
return database.fetchPosts(userId);
} catch (error) {
return [];
}
}
function getUserComments(userId) {
// No error handling at all
return database.fetchComments(userId);
}
After standardizing error handling:
// Centralized error handler
function handleDatabaseError(operation, error) {
logger.error(`Database error during ${operation}:`, error);
if (error.code === 'CONNECTION_ERROR') {
throw new DatabaseConnectionError(error.message);
} else if (error.code === 'NOT_FOUND') {
return null;
} else {
throw new DatabaseOperationError(operation, error.message);
}
}
function getUserData(userId) {
try {
return database.fetchUser(userId);
} catch (error) {
return handleDatabaseError('user fetch', error);
}
}
function getUserPosts(userId) {
try {
return database.fetchPosts(userId);
} catch (error) {
return handleDatabaseError('posts fetch', error);
}
}
function getUserComments(userId) {
try {
return database.fetchComments(userId);
} catch (error) {
return handleDatabaseError('comments fetch', error);
}
}
Poor Variable Naming
Cryptic variable names make code harder to understand and maintain.
Before:
function calc(a, b, c, t) {
const res = a * b;
let tmp = 0;
if (t) {
tmp = res * c;
} else {
tmp = res / c;
}
return tmp;
}
After improving variable names:
function calculateAdjustedProduct(baseValue, multiplier, adjustmentFactor, shouldMultiply) {
const baseProduct = baseValue * multiplier;
let adjustedResult;
if (shouldMultiply) {
adjustedResult = baseProduct * adjustmentFactor;
} else {
adjustedResult = baseProduct / adjustmentFactor;
}
return adjustedResult;
}
The Evolution of a Professional Developer
Becoming a professional developer is a journey that involves more than just setting up a sleek development environment. It’s about evolving your skills, practices, and mindset.
From Tool Collector to Craftsperson
Early in your career, it’s common to focus on collecting tools and technologies. You might learn multiple frameworks, languages, and development environments. This is a necessary phase, but it’s just the beginning.
As you mature as a developer, you start to shift from being a tool collector to being a craftsperson. You become more discerning about which tools truly enhance your work, and you develop deeper expertise with the ones that matter most.
From Feature Completer to Problem Solver
Novice developers often measure success by completing features or making things work. Professional developers measure success by solving problems effectively, creating maintainable solutions, and anticipating future needs.
This shift in perspective changes how you approach coding:
- Instead of asking “How can I make this work?”, you ask “What’s the best way to solve this problem?”
- Instead of celebrating when a feature works, you celebrate when it works, is well-tested, and is maintainable
- Instead of moving quickly to the next feature, you take time to refactor and improve what you’ve built
From Solo Coder to Team Contributor
Professional development rarely happens in isolation. As you grow, you’ll start to see your code as part of a larger ecosystem that other developers need to understand and work with.
This perspective shift leads to practices like:
- Writing code with readability for others in mind
- Documenting not just what your code does, but why certain decisions were made
- Participating actively in code reviews, both giving and receiving feedback
- Mentoring less experienced developers
Balancing Aesthetics and Substance
It’s important to note that having a beautiful, well-configured development environment isn’t bad. In fact, it can be beneficial for your productivity and enjoyment. The key is to ensure that your code quality matches the professional appearance of your tools.
When to Invest in Your Environment
Investing time in your development environment makes sense when:
- It genuinely improves your productivity (e.g., keyboard shortcuts that save time)
- It helps enforce good practices (e.g., linters that catch common mistakes)
- It makes you more comfortable during long coding sessions
- You’ve already established good coding practices and are looking to optimize
When to Focus on Code Quality Instead
You should prioritize improving your code quality over your environment when:
- You find yourself struggling to understand code you wrote months ago
- Other developers have difficulty working with your code
- You spend more time fixing bugs than adding features
- Your projects become increasingly difficult to maintain as they grow
Conclusion: Substance Over Style
A professional-looking development environment can be satisfying and even beneficial, but it’s the quality of your code that truly defines you as a developer. As you progress in your career, strive to ensure that the substance of your work matches its style.
Remember that becoming a professional developer is a journey, not a destination. Even the most experienced developers continue to learn and improve their craft. The key is to remain humble, curious, and committed to growth.
Start by identifying areas where your code quality might not match your environment’s polish, then systematically address them using the strategies outlined in this article. Over time, you’ll develop coding habits and skills that truly reflect the professional image your development environment projects.
The most beautiful code isn’t the one with the prettiest syntax highlighting or the most exotic font. It’s the code that solves problems effectively, can be easily understood and maintained by others, and stands the test of time. That’s the true mark of a professional developer.
So by all means, customize your terminal and perfect your IDE setup, but never forget that what matters most is the quality of the code you write within it. After all, your users and fellow developers will experience your code, not your development environment.