Why Your Deployment Process Is Causing Production Issues
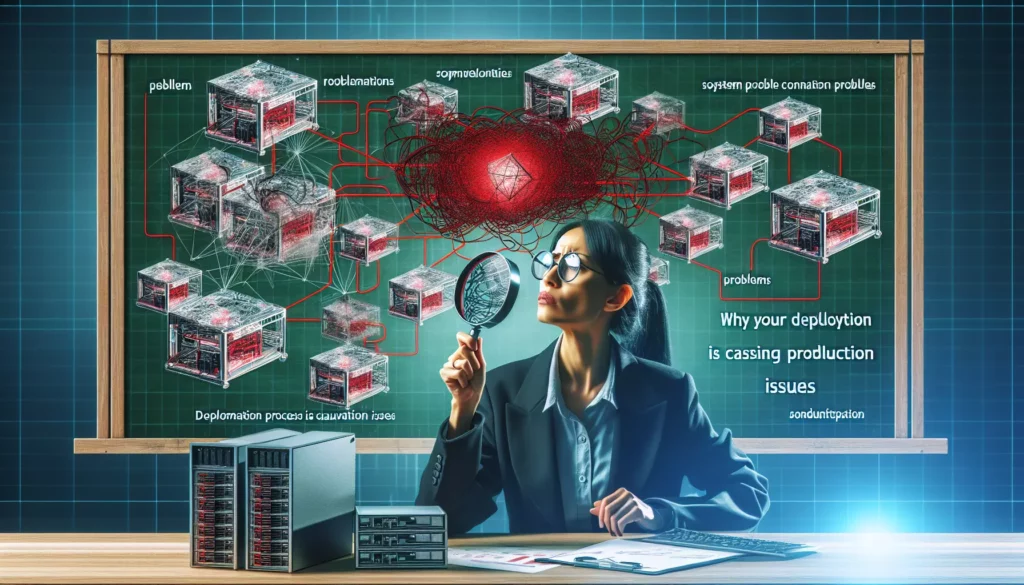
In the fast-paced world of software development, getting code from development to production quickly is essential. However, many teams find themselves caught in a frustrating cycle: they deploy new features or fixes, only to discover new problems in production. If your team is experiencing frequent production issues after deployments, your deployment process itself might be the culprit.
At AlgoCademy, we’ve worked with hundreds of engineering teams to improve their development practices. One common pattern we’ve observed is that teams often underestimate the importance of a robust deployment process. In this comprehensive guide, we’ll explore why your current deployment approach might be causing production issues and provide actionable strategies to fix these problems.
Table of Contents
- Understanding Deployment-Related Production Issues
- Common Deployment Process Pitfalls
- Inadequate Monitoring and Detection Systems
- Testing Gaps and Environment Disparities
- Modern Deployment Strategies to Reduce Risk
- Building Robust CI/CD Pipelines
- Establishing Effective Rollback Procedures
- Post-Deployment Verification and Monitoring
- Team Culture and Deployment Practices
- Conclusion: Building a Better Deployment Process
Understanding Deployment-Related Production Issues
Before diving into specific problems, it’s important to understand what constitutes a deployment-related production issue. These are problems that emerge in your production environment following a deployment and can include:
- Functionality that worked in testing but fails in production
- Performance degradation or system slowdowns
- Increased error rates or unexpected exceptions
- Service outages or downtime
- Data inconsistencies or corruption
- Security vulnerabilities exposed by new code
Production issues are particularly costly because they directly impact users, potentially damaging trust in your product. According to studies, the average cost of downtime for enterprises can range from $100,000 to over $1 million per hour, depending on the industry and size of the organization.
Common Deployment Process Pitfalls
Let’s examine the most common deployment process problems that lead to production issues:
1. Manual Deployment Steps
Human error is one of the leading causes of deployment failures. When deployments involve numerous manual steps, mistakes become inevitable. Common manual deployment errors include:
- Forgetting to update configuration files
- Missing steps in a deployment checklist
- Deploying the wrong version or branch
- Inconsistent execution of deployment steps
Solution: Automate your deployment process as much as possible. Create scripts for repetitive tasks and implement continuous deployment pipelines that can execute the same steps consistently every time.
2. “Big Bang” Deployments
Deploying large amounts of code at once substantially increases risk. When multiple features or fixes are bundled together in a single deployment, it becomes difficult to:
- Isolate the cause of any issues that arise
- Perform thorough testing of all changes
- Roll back specific problematic features without losing other valuable updates
Solution: Embrace smaller, more frequent deployments. This approach, often called “continuous deployment” or “progressive delivery,” reduces risk by limiting the scope of each deployment and making it easier to identify the source of problems.
3. Inadequate Environment Parity
One of the most common phrases in software development is “but it worked on my machine!” This problem often stems from differences between development, testing, and production environments. These differences can include:
- Different operating systems or versions
- Varying dependency versions
- Distinct configuration settings
- Different hardware specifications or cloud resources
- Disparate data sets
Solution: Strive for environment parity using containerization technologies like Docker, which encapsulate your application and its dependencies. Infrastructure as Code (IaC) tools like Terraform or AWS CloudFormation can also help ensure consistency across environments.
// Example Docker configuration ensuring environment parity
// Dockerfile
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
4. Insufficient Deployment Documentation
Without clear documentation, team members may not understand the deployment process fully, leading to inconsistent execution and knowledge silos. This becomes especially problematic when:
- New team members join and need to perform deployments
- The primary deployment “expert” is unavailable
- Emergency deployments need to be performed under pressure
Solution: Create comprehensive, up-to-date deployment documentation that includes step-by-step procedures, architecture diagrams, configuration details, and troubleshooting guides. Regularly review and update this documentation.
Inadequate Monitoring and Detection Systems
Even with the best deployment processes, issues can still occur. The difference between minor hiccups and major incidents often comes down to how quickly you can detect and respond to problems.
1. Lack of Real-Time Monitoring
Without proper monitoring, issues can persist for hours or even days before being noticed. This delay dramatically increases the impact of deployment problems.
Solution: Implement comprehensive monitoring that covers:
- Application Performance Monitoring (APM): Tools like New Relic, Datadog, or Dynatrace that track application performance metrics
- Infrastructure Monitoring: Tracking CPU, memory, disk usage, and network traffic
- Log Aggregation: Centralized logging with tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Graylog
- Synthetic Monitoring: Automated tests that simulate user interactions to detect issues before users do
2. Missing Alerting Thresholds
Collecting monitoring data is only useful if you have mechanisms to alert you when metrics indicate problems.
Solution: Define clear alerting thresholds based on your application’s normal behavior. Implement alerting for:
- Error rate spikes
- Latency increases
- Unusual traffic patterns
- Resource utilization (CPU, memory, disk) beyond normal ranges
- Failed health checks
// Example Prometheus alerting rule for API error rate
groups:
- name: api.rules
rules:
- alert: HighErrorRate
expr: sum(rate(http_requests_total{status=~"5.."}[5m])) / sum(rate(http_requests_total[5m])) > 0.05
for: 1m
labels:
severity: critical
annotations:
summary: "High API Error Rate"
description: "Error rate is above 5% for more than 1 minute."
3. Ineffective Health Checks
Many teams implement overly simplistic health checks that fail to detect real issues. A service might respond to a basic ping but still be failing to process transactions correctly.
Solution: Implement deep health checks that verify:
- Connectivity to dependent services and databases
- Ability to process typical transactions
- Proper configuration loading
- Resource availability
// Example of a more comprehensive health check endpoint in Node.js
app.get('/health', async (req, res) => {
try {
// Check database connection
await db.query('SELECT 1');
// Check cache connection
await cache.ping();
// Check external API connectivity
await axios.get('https://api.example.com/health');
// Check file system access
await fs.promises.access('./config');
res.status(200).json({ status: 'healthy' });
} catch (error) {
console.error('Health check failed:', error);
res.status(500).json({
status: 'unhealthy',
error: error.message
});
}
});
Testing Gaps and Environment Disparities
Inadequate testing is a major contributor to deployment-related production issues. Let’s explore common testing gaps and how to address them:
1. Insufficient Test Coverage
Many teams focus heavily on unit tests but neglect other crucial testing types, leaving gaps where issues can hide until production.
Solution: Implement a comprehensive testing strategy that includes:
- Unit Tests: Testing individual components in isolation
- Integration Tests: Testing how components work together
- End-to-End Tests: Testing complete user flows
- Performance Tests: Verifying system performance under load
- Security Tests: Identifying potential vulnerabilities
- Chaos Testing: Deliberately introducing failures to test resilience
2. Test Data Limitations
Testing with small or artificially clean datasets often fails to uncover issues that emerge with real-world data volumes and edge cases.
Solution: Enhance your test data strategy by:
- Using anonymized copies of production data for testing
- Creating test data generators that produce realistic data volumes and variations
- Maintaining a library of edge cases based on production incidents
- Testing with deliberately malformed or unexpected inputs
3. Neglecting Non-Functional Requirements
Teams often focus on verifying that features work correctly but neglect to test non-functional aspects like performance, security, and accessibility.
Solution: Incorporate specialized testing for non-functional requirements:
- Load Testing: Tools like JMeter, Gatling, or Locust to simulate high traffic
- Security Scanning: Static Application Security Testing (SAST) and Dynamic Application Security Testing (DAST) tools
- Accessibility Testing: Tools like Axe or Lighthouse to verify compliance with accessibility standards
- Resilience Testing: Tools like Chaos Monkey to test system behavior during failures
4. Inconsistent Testing Practices
When testing practices vary between team members or over time, quality becomes inconsistent and issues slip through.
Solution: Standardize testing practices by:
- Creating testing guidelines and standards
- Implementing automated test suites that run as part of your CI/CD pipeline
- Conducting regular test reviews alongside code reviews
- Tracking test coverage metrics
// Example Jest configuration enforcing test coverage thresholds
// jest.config.js
module.exports = {
collectCoverage: true,
coverageThreshold: {
global: {
branches: 80,
functions: 80,
lines: 80,
statements: 80
}
},
// Other configuration options...
};
Modern Deployment Strategies to Reduce Risk
The way you deploy code can significantly impact the risk of production issues. Modern deployment strategies focus on minimizing risk through controlled, incremental changes.
1. Blue-Green Deployments
Blue-green deployment involves maintaining two identical production environments (blue and green). At any time, only one environment is live and serving production traffic.
How it works:
- Deploy the new version to the inactive environment (e.g., green)
- Run tests on the green environment to verify functionality
- Switch traffic from blue to green by updating routing or load balancer configuration
- Keep the old blue environment available for quick rollback if needed
Benefits:
- Zero downtime deployments
- Instant rollback capability
- Opportunity to test in a production-identical environment before exposing users
2. Canary Deployments
Canary deployments involve gradually routing a small percentage of traffic to the new version and monitoring for issues before expanding to all users.
How it works:
- Deploy the new version alongside the existing version
- Route a small percentage (e.g., 5%) of traffic to the new version
- Monitor for errors, performance issues, or other problems
- Gradually increase traffic to the new version if no issues are detected
- If problems arise, route all traffic back to the old version
Benefits:
- Limits the impact of issues to a small subset of users
- Provides early warning of problems before full deployment
- Allows for performance comparison between versions
// Example of canary deployment configuration in Kubernetes with Istio
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: my-service
spec:
hosts:
- my-service
http:
- route:
- destination:
host: my-service
subset: v1
weight: 90
- destination:
host: my-service
subset: v2
weight: 10
3. Feature Flags
Feature flags (or feature toggles) allow you to deploy code to production but control its activation through configuration rather than deployment.
How it works:
- Deploy code with new features wrapped in conditional logic (the feature flag)
- Keep the feature disabled in production initially
- Enable the feature for internal users or a small percentage of users
- Gradually roll out the feature to more users
- If issues arise, disable the feature without requiring a deployment
Benefits:
- Separates deployment from feature release
- Enables quick feature disabling without code changes
- Allows for A/B testing and gradual rollouts
- Supports targeted releases to specific user segments
// Example of feature flag implementation
function renderCheckoutButton(user) {
if (featureFlags.isEnabled('new-checkout-flow', user)) {
return <NewCheckoutButton />;
} else {
return <LegacyCheckoutButton />;
}
}
Building Robust CI/CD Pipelines
Continuous Integration and Continuous Deployment (CI/CD) pipelines automate the process of building, testing, and deploying code. A well-designed CI/CD pipeline can dramatically reduce deployment-related production issues.
1. Pipeline Stages and Gates
Effective CI/CD pipelines include multiple stages with quality gates that prevent problematic code from advancing to production.
Key pipeline stages:
- Build: Compile code and create deployable artifacts
- Unit Tests: Run fast, focused tests on individual components
- Static Analysis: Check code quality, security vulnerabilities, and adherence to standards
- Integration Tests: Verify components work together correctly
- Deployment to Staging: Deploy to a production-like environment
- End-to-End Tests: Run comprehensive tests on the staging environment
- Performance Tests: Verify system performance under load
- Security Tests: Scan for vulnerabilities
- Approval Gate: Require manual approval for production deployment
- Production Deployment: Deploy to production using safe deployment strategies
- Post-Deployment Verification: Verify the deployment was successful
// Example GitHub Actions workflow with multiple stages
name: CI/CD Pipeline
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Build
run: npm ci && npm run build
test:
needs: build
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Unit Tests
run: npm test
- name: Static Analysis
run: npm run lint
deploy-staging:
needs: test
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Deploy to Staging
run: ./deploy.sh staging
- name: Integration Tests
run: npm run test:integration
- name: E2E Tests
run: npm run test:e2e
deploy-production:
needs: deploy-staging
runs-on: ubuntu-latest
environment: production
steps:
- uses: actions/checkout@v2
- name: Deploy to Production
run: ./deploy.sh production
- name: Verify Deployment
run: ./verify-deployment.sh
2. Pipeline Reliability
A CI/CD pipeline is only effective if it’s reliable. Flaky tests or inconsistent builds can lead teams to ignore or bypass pipeline failures, defeating their purpose.
Improving pipeline reliability:
- Address flaky tests immediately rather than allowing them to be ignored
- Use deterministic build processes (e.g., lock dependency versions)
- Implement retry mechanisms for intermittent external service failures
- Monitor pipeline performance and optimize slow steps
- Use dedicated infrastructure for critical pipeline stages
3. Artifact Management
Properly managing build artifacts ensures that what you test is exactly what you deploy.
Best practices:
- Build artifacts once and promote the same artifact through environments
- Version artifacts consistently
- Store artifacts in a secure, versioned repository
- Implement checksums to verify artifact integrity
- Retain artifacts for rollback purposes
Establishing Effective Rollback Procedures
No matter how thorough your testing and deployment processes are, issues will occasionally make it to production. Having a well-defined rollback procedure is essential for minimizing the impact of these issues.
1. Automated Rollback Capabilities
The ability to quickly revert to a previous working state is crucial for minimizing downtime.
Implementation strategies:
- Maintain deployment history with the ability to deploy previous versions
- Use immutable infrastructure where possible to simplify rollbacks
- Implement one-click or automated rollback triggers
- Practice rollbacks regularly to ensure they work when needed
// Example rollback script
#!/bin/bash
# rollback.sh
# Get the previous deployment version
PREVIOUS_VERSION=$(cat .deployment_history | tail -n 2 | head -n 1)
echo "Rolling back to version: $PREVIOUS_VERSION"
# Deploy the previous version
./deploy.sh $PREVIOUS_VERSION
# Verify the rollback
./verify-deployment.sh
if [ $? -eq 0 ]; then
echo "Rollback successful"
else
echo "Rollback failed, manual intervention required"
./alert-team.sh "Rollback failed"
exit 1
fi
2. Database Rollback Strategies
Database changes are often the most challenging aspect of rollbacks. Schema changes can be particularly problematic if they’re not backward compatible.
Approaches to database rollbacks:
- Versioned Migrations: Use tools like Flyway or Liquibase to manage database schema changes
- Backward Compatibility: Design schema changes to be compatible with both old and new code
- Blue-Green for Databases: Maintain parallel database schemas during transitions
- Database Snapshots: Take snapshots before deployments for potential restoration
3. Defining Rollback Criteria
Clear criteria for when to initiate a rollback help teams make quick decisions during incidents.
Sample rollback criteria:
- Error rate exceeds 1% for more than 5 minutes
- Response time increases by more than 100% compared to baseline
- Critical user flows (e.g., checkout, login) are broken
- Security vulnerability is detected
- Data integrity issues are observed
Post-Deployment Verification and Monitoring
The moments immediately following a deployment are critical for catching issues before they impact many users. A robust post-deployment verification process can significantly reduce the impact of production issues.
1. Smoke Testing
Smoke tests verify that the most critical functionality works after deployment. These should be automated and run immediately after each deployment.
Key characteristics of effective smoke tests:
- Focus on critical user journeys
- Run quickly (typically under 5 minutes)
- Test end-to-end functionality including integrations
- Verify both positive scenarios and key error handling
// Example smoke test script using Cypress
describe('Post-Deployment Smoke Tests', () => {
it('Users can log in', () => {
cy.visit('/login');
cy.get('#username').type('testuser');
cy.get('#password').type('password123');
cy.get('#login-button').click();
cy.url().should('include', '/dashboard');
cy.get('.user-greeting').should('contain', 'Welcome, Test User');
});
it('Users can search for products', () => {
cy.visit('/');
cy.get('#search-input').type('laptop');
cy.get('#search-button').click();
cy.get('.search-results').should('be.visible');
cy.get('.product-card').should('have.length.at.least', 1);
});
it('Users can add items to cart', () => {
cy.visit('/products/1');
cy.get('#add-to-cart-button').click();
cy.get('.cart-count').should('contain', '1');
});
});
2. Graduated Exposure
Even with thorough testing, it’s wise to limit the initial exposure of new deployments to minimize potential impact.
Graduated exposure strategies:
- Internal Users First: Deploy to employees or internal users before external customers
- Percentage-Based Rollout: Start with a small percentage of users and gradually increase
- Geographic Rollout: Deploy to one region at a time
- Off-Peak Timing: Deploy during periods of lower usage
3. Enhanced Monitoring During Deployment Windows
During and immediately after deployments, monitoring should be heightened to quickly catch any issues.
Post-deployment monitoring practices:
- Reduce alert thresholds temporarily to catch subtle issues
- Implement deployment markers in monitoring dashboards
- Set up temporary dashboards specific to the features being deployed
- Assign team members to actively monitor systems during deployment windows
- Compare key metrics before and after deployment
// Example Datadog monitor with tighter thresholds after deployment
{
"name": "Post-Deployment API Error Rate",
"type": "query alert",
"query": "sum(last_5m):sum:api.errors{*} / sum:api.requests{*} * 100 > 0.5",
"message": "Error rate exceeded 0.5% after deployment. @devops-team",
"tags": ["service:api", "stage:post-deployment"],
"options": {
"thresholds": {
"critical": 0.5, // Normal threshold might be 2%
"warning": 0.2
},
"notify_no_data": true,
"notify_audit": false,
"timeout_h": 0,
"include_tags": true,
"no_data_timeframe": 10,
"evaluation_delay": 900
}
}
Team Culture and Deployment Practices
Beyond technical solutions, team culture plays a crucial role in preventing deployment-related production issues.
1. Ownership and Accountability
Teams that take ownership of their deployments tend to build more reliable systems.
Building a culture of ownership:
- “You build it, you run it” philosophy where development teams are responsible for operating their services
- Rotate on-call responsibilities among all team members
- Include operational readiness in definition of done
- Celebrate both successful feature launches and operational improvements
2. Learning from Failures
When production issues do occur, they present valuable learning opportunities.
Effective post-incident practices:
- Conduct blameless postmortems focused on systemic issues rather than individual mistakes
- Document incidents and their resolutions in a knowledge base
- Convert lessons learned into concrete process improvements
- Share learnings across teams
3. Continuous Improvement
The best deployment processes evolve over time based on experience and changing requirements.
Approaches to continuous improvement:
- Regularly review deployment metrics (frequency, success rate, lead time, recovery time)
- Conduct periodic retrospectives focused specifically on deployment processes
- Stay current with industry best practices and tools
- Implement small, incremental improvements rather than massive process overhauls
Conclusion: Building a Better Deployment Process
Deployment-related production issues are not inevitable. With the right processes, tools, and culture, you can dramatically reduce their frequency and impact. Let’s recap the key strategies for improving your deployment process:
- Automate extensively to reduce human error and increase consistency
- Deploy smaller changes more frequently to reduce risk and simplify troubleshooting
- Implement comprehensive testing across multiple dimensions (functionality, performance, security)
- Use modern deployment strategies like blue-green, canary, and feature flags to control risk
- Build robust CI/CD pipelines with quality gates at each stage
- Establish effective rollback procedures for when issues do occur
- Verify deployments immediately with automated smoke tests and enhanced monitoring
- Foster a culture of ownership and learning that treats failures as opportunities for improvement
Remember that improving your deployment process is itself an iterative journey. Start by identifying your biggest pain points and addressing them one by one. Over time, these incremental improvements will compound to create a deployment process that supports rapid innovation while maintaining high reliability.
At AlgoCademy, we’ve seen teams transform their deployment processes from sources of stress and uncertainty to competitive advantages that enable them to ship features faster and more reliably than their competitors. By applying the principles outlined in this guide, your team can achieve the same results.
Are you experiencing deployment-related production issues? Which aspects of your deployment process do you think need the most improvement? Start the conversation with your team today, and begin your journey toward more reliable deployments.