Why Your Defensive Programming Is Creating Bugs
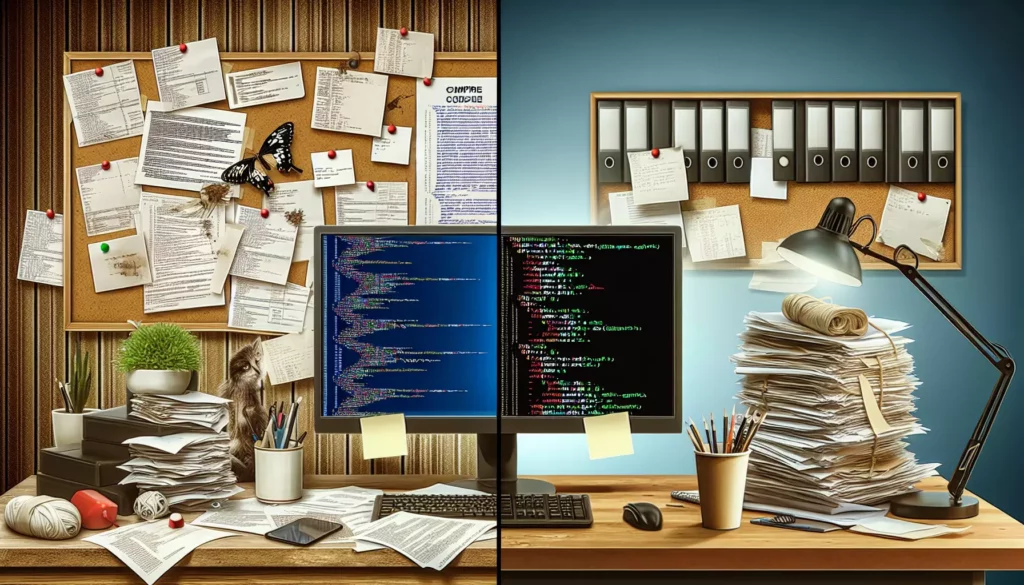
Defensive programming is often touted as a best practice that helps create robust, error-resistant code. We’re taught to validate inputs, check for edge cases, and handle exceptions gracefully. But what if I told you that your well-intentioned defensive programming could actually be introducing bugs rather than preventing them?
In this article, we’ll explore how overzealous defensive programming can lead to unexpected consequences, making your code harder to debug, maintain, and reason about. We’ll look at real examples of when defensive programming goes wrong and provide guidelines for when and how to apply defensive techniques effectively.
What Is Defensive Programming?
Before we dive into the pitfalls, let’s clarify what defensive programming actually means. Defensive programming is a set of practices designed to anticipate and handle potential errors or unexpected inputs. The goal is to create software that continues to function correctly even when faced with unexpected conditions.
Common defensive programming techniques include:
- Input validation
- Error handling and exception management
- Assertions and preconditions
- Null checks
- Boundary condition handling
- Defensive copying of mutable objects
When applied judiciously, these techniques can prevent crashes, security vulnerabilities, and data corruption. But like any programming pattern, defensive programming can be misused or overused.
The Hidden Costs of Excessive Defensive Programming
1. Silent Failures Mask Real Problems
One of the most insidious problems with overly defensive code is that it can silently swallow errors rather than exposing them. Consider this example:
public void processUserData(UserData userData) {
try {
// Process the user data
saveToDatabase(userData);
notifyUser(userData.getEmail());
updateStats(userData.getUsername());
} catch (Exception e) {
// Just log and continue
logger.warn("Something went wrong processing user data", e);
}
}
At first glance, this might seem reasonable. We’re “defensively” catching any exceptions to prevent a system crash. But this approach has serious flaws:
- If
saveToDatabase()
fails, the code will still try to notify the user and update stats, potentially leaving the system in an inconsistent state. - The generic log message provides little context about what actually failed.
- The caller has no way to know that something went wrong.
This kind of blanket error handling masks real problems and can make debugging a nightmare. It’s often better to let errors propagate or to handle specific exceptions with specific recovery strategies.
2. Unnecessary Null Checks Complicate Code
Null checks are perhaps the most common form of defensive programming, but they can quickly get out of hand:
public String formatUserInfo(User user) {
if (user != null) {
String name = user.getName();
if (name != null) {
String firstName = name.split(" ")[0];
if (firstName != null) {
return "Welcome, " + firstName;
}
}
}
return "Welcome, Guest";
}
This code is difficult to read and maintain due to the nested conditionals. In many cases, these checks are unnecessary and indicate deeper design issues. For instance, if a User
object should always have a name, then the proper solution is to ensure that invariant at the object creation level, not to defensively check for null at every use site.
3. Excessive Error Handling Obscures Business Logic
When error handling dominates your code, the actual business logic becomes harder to discern:
public double calculateDiscount(Order order) {
if (order == null) {
logger.error("Null order received");
return 0.0;
}
List<OrderItem> items = order.getItems();
if (items == null || items.isEmpty()) {
logger.warn("Order has no items");
return 0.0;
}
Customer customer = order.getCustomer();
if (customer == null) {
logger.error("Order has no associated customer");
return 0.0;
}
MembershipLevel level = customer.getMembershipLevel();
if (level == null) {
logger.warn("Customer has no membership level");
return 0.0;
}
// Finally, the actual business logic
return level.getDiscountPercentage() * 0.01;
}
In this example, the actual calculation is a single line at the end, while the defensive checks dominate the method. This makes the code harder to understand and maintain.
4. Defensive Programming Can Hide Contract Violations
Consider a method that’s supposed to process a list of valid user IDs:
public void processUserIds(List<String> userIds) {
for (String userId : userIds) {
if (userId != null && !userId.isEmpty()) {
try {
User user = userService.findById(userId);
if (user != null) {
processUser(user);
}
} catch (Exception e) {
logger.warn("Failed to process user with ID: " + userId, e);
}
}
}
}
This method silently skips null or empty user IDs and continues if a user can’t be found or processed. But what if the presence of invalid IDs indicates a bug in the calling code? By defensively handling these cases, we’re allowing contract violations to go unnoticed, potentially masking serious issues.
Real World Example: The Mars Climate Orbiter Disaster
The Mars Climate Orbiter mission failure in 1999 provides a striking example of how defensive programming can contribute to catastrophic failures. The spacecraft was lost because one team used English units (pound-seconds) while another used metric units (newton-seconds) for a crucial calculation.
The software could have included defensive checks to detect the unit mismatch by validating that values fell within expected ranges. However, even if such checks existed, they might have been implemented as silent corrections rather than errors:
// Hypothetical defensive code that could have been used
public void processThrust(double thrustValue, String unit) {
double thrustInNewtons;
if (unit.equals("pound-seconds")) {
// Convert to newton-seconds
thrustInNewtons = thrustValue * 4.44822;
} else if (unit.equals("newton-seconds")) {
thrustInNewtons = thrustValue;
} else {
// Defensive fallback - assume newtons
logger.warn("Unknown thrust unit: {}. Assuming newton-seconds.", unit);
thrustInNewtons = thrustValue;
}
applyThrust(thrustInNewtons);
}
This kind of defensive code would have silently accepted incorrect units, logging a warning but proceeding with potentially disastrous calculations. A better approach would have been to fail fast with a clear error when encountering unexpected units, forcing the issue to be addressed before launch.
When Defensive Programming Creates Security Vulnerabilities
Ironically, defensive programming intended to improve security can sometimes create vulnerabilities. Consider this example of input sanitization:
public String sanitizeUserInput(String input) {
if (input == null) {
return "";
}
// Remove potentially dangerous characters
String sanitized = input.replaceAll("<script>", "")
.replaceAll("</script>", "")
.replaceAll("javascript:", "");
return sanitized;
}
This approach attempts to defensively remove dangerous patterns from user input, but it’s actually creating a false sense of security. Attackers can easily bypass this sanitization with variations like <ScRiPt>
or <scr<script>ipt>
. A more robust approach would be to use a proper HTML sanitization library or to escape output when rendering.
The Performance Impact of Defensive Code
Defensive programming can also impact performance, particularly in performance-critical sections of code:
public double calculateAverage(List<Double> values) {
if (values == null) {
return 0.0;
}
if (values.isEmpty()) {
return 0.0;
}
double sum = 0.0;
int count = 0;
for (Double value : values) {
if (value != null) {
sum += value;
count++;
}
}
if (count == 0) {
return 0.0;
}
return sum / count;
}
In a high-performance environment where this method might be called millions of times, these defensive checks add overhead. If the contract of the method specifies that the input list should never contain null values, then these checks are unnecessarily slowing down the code.
A Better Approach: Strategic Defensive Programming
Rather than applying defensive programming everywhere, a more effective approach is to be strategic about where and how you implement defensive measures. Here are some guidelines:
1. Define Clear Contracts and Fail Fast at Boundaries
Apply thorough validation at your system’s boundaries (API endpoints, user inputs, file parsing), but rely on contracts and preconditions for internal methods:
// At the boundary
public Response processUserRequest(UserRequest request) {
// Validate the request thoroughly
if (request == null) {
return Response.badRequest("Request cannot be null");
}
if (request.getUserId() == null || request.getUserId().isEmpty()) {
return Response.badRequest("User ID is required");
}
// Once validated, internal methods can assume valid data
User user = userService.findUserById(request.getUserId());
return processUserInternal(user, request);
}
// Internal method with preconditions
private Response processUserInternal(User user, UserRequest request) {
// Can assume user and request are non-null due to contract
// Focus on business logic instead of defensive checks
// ...
}
2. Use Assertions for Impossible Conditions
Assertions are perfect for documenting and checking conditions that should be impossible if your code is correct:
public void processOrder(Order order, PaymentStatus status) {
// This should never happen if our code is correct
assert order != null : "Order should never be null at this point";
assert status != null : "Payment status should never be null";
if (status == PaymentStatus.PAID) {
fulfillOrder(order);
} else {
sendPaymentReminder(order);
}
}
Assertions document your assumptions and help catch bugs during development and testing, but don’t add runtime overhead in production (when assertions are disabled).
3. Let Exceptions Propagate When Appropriate
Not every exception needs to be caught and handled locally:
// Before: Overly defensive
public void updateUserProfile(User user) {
try {
userRepository.save(user);
} catch (Exception e) {
logger.error("Failed to update user profile", e);
}
}
// After: Let exceptions propagate
public void updateUserProfile(User user) throws RepositoryException {
userRepository.save(user);
// Let the caller decide how to handle exceptions
}
By letting exceptions propagate, you give callers the flexibility to handle errors in a way that makes sense for their context.
4. Use Null Object Pattern Instead of Null Checks
Instead of checking for null throughout your code, consider using the Null Object pattern:
// Instead of null checks everywhere
public class UserService {
public User findUserById(String id) {
User user = repository.findById(id);
return user != null ? user : User.GUEST;
}
}
// Null object implementation
public class User {
public static final User GUEST = new User("guest", "Guest User", Collections.emptyList());
// Regular user fields and methods
}
// Now client code doesn't need null checks
public void greetUser(String userId) {
User user = userService.findUserById(userId);
System.out.println("Hello, " + user.getName());
}
5. Leverage Type Systems and Language Features
Modern languages often provide features to reduce the need for defensive programming:
// Java Optional
public Optional<User> findUserById(String id) {
return Optional.ofNullable(repository.findById(id));
}
// Client code handles the Optional explicitly
userService.findUserById(userId).ifPresent(user -> {
processUser(user);
});
// Or with pattern matching in newer Java versions
if (userService.findUserById(userId) instanceof Optional<User> optUser && optUser.isPresent()) {
User user = optUser.get();
processUser(user);
}
Case Study: Refactoring Overly Defensive Code
Let’s look at a complete example of refactoring overly defensive code to a more balanced approach:
Original (Overly Defensive) Code
public class OrderProcessor {
private Logger logger = LoggerFactory.getLogger(OrderProcessor.class);
public boolean processOrder(Order order) {
if (order == null) {
logger.warn("Null order received");
return false;
}
Customer customer = order.getCustomer();
if (customer == null) {
logger.warn("Order has no customer: {}", order.getId());
return false;
}
List<OrderItem> items = order.getItems();
if (items == null || items.isEmpty()) {
logger.warn("Order has no items: {}", order.getId());
return false;
}
double total = 0.0;
for (OrderItem item : items) {
if (item != null) {
Product product = item.getProduct();
if (product != null) {
double price = product.getPrice();
int quantity = item.getQuantity();
if (quantity > 0) {
total += price * quantity;
}
}
}
}
try {
PaymentResult result = paymentService.processPayment(customer, total);
if (result != null && result.isSuccessful()) {
try {
orderRepository.save(order);
try {
notificationService.sendOrderConfirmation(order);
return true;
} catch (Exception e) {
logger.error("Failed to send confirmation for order: {}", order.getId(), e);
return true; // Order was still processed successfully
}
} catch (Exception e) {
logger.error("Failed to save order: {}", order.getId(), e);
return false;
}
} else {
logger.warn("Payment failed for order: {}", order.getId());
return false;
}
} catch (Exception e) {
logger.error("Error processing payment for order: {}", order.getId(), e);
return false;
}
}
}
Refactored Code
public class OrderProcessor {
private static final Logger logger = LoggerFactory.getLogger(OrderProcessor.class);
/**
* Process an order by calculating the total, charging the customer,
* saving the order, and sending confirmation.
*
* @param order A valid order with customer and items
* @return true if order was successfully processed
* @throws ValidationException if the order fails validation
* @throws PaymentException if payment processing fails
* @throws PersistenceException if saving the order fails
*/
public boolean processOrder(Order order) throws ValidationException,
PaymentException,
PersistenceException {
validateOrder(order);
double total = calculateOrderTotal(order);
PaymentResult result = paymentService.processPayment(order.getCustomer(), total);
if (!result.isSuccessful()) {
logger.info("Payment declined for order: {}", order.getId());
return false;
}
orderRepository.save(order);
try {
notificationService.sendOrderConfirmation(order);
} catch (NotificationException e) {
// Non-critical failure - log but don't fail the order processing
logger.warn("Failed to send confirmation for order: {}", order.getId(), e);
}
return true;
}
private void validateOrder(Order order) throws ValidationException {
if (order == null) {
throw new ValidationException("Order cannot be null");
}
if (order.getCustomer() == null) {
throw new ValidationException("Order must have a customer");
}
if (order.getItems() == null || order.getItems().isEmpty()) {
throw new ValidationException("Order must have at least one item");
}
}
private double calculateOrderTotal(Order order) {
return order.getItems().stream()
.filter(Objects::nonNull)
.mapToDouble(item -> {
Product product = item.getProduct();
return product.getPrice() * Math.max(0, item.getQuantity());
})
.sum();
}
}
Key improvements in the refactored code:
- Clear method contract with documented exceptions
- Validation separated into a dedicated method
- Business logic is more visible and not buried in defensive code
- Appropriate exception handling – letting critical exceptions propagate while catching non-critical ones
- Simplified calculation using streams
- No deeply nested conditionals
Balancing Security and Robustness with Clean Code
Defensive programming doesn’t have to mean cluttered, hard-to-maintain code. The key is to strike a balance between defensiveness and clarity:
Do:
- Validate inputs at system boundaries
- Use assertions to document and check invariants
- Fail fast when contracts are violated
- Handle specific exceptions with specific recovery strategies
- Use language features like Optional to handle nullable values cleanly
Don’t:
- Catch and swallow exceptions without proper handling
- Add null checks to every method parameter
- Return null when you could throw an exception or return an Optional
- Hide bugs with overly permissive error recovery
- Implement defensive checks that duplicate validation done elsewhere
Tools and Practices That Reduce the Need for Defensive Programming
Several modern practices and tools can help reduce the need for defensive programming:
1. Static Analysis Tools
Tools like Spotbugs, SonarQube, or language-specific linters can catch many potential issues at compile time:
- Null dereference warnings
- Resource leak detection
- Unchecked exceptions
- Potential concurrency issues
2. Property-Based Testing
Property-based testing tools like jqwik (Java), QuickCheck (Haskell), or Hypothesis (Python) can automatically generate test cases to find edge cases you might not have considered:
@Property
void absoluteValueIsAlwaysPositive(@ForAll int value) {
int result = Math.abs(value);
Assertions.assertTrue(result >= 0);
}
3. Design by Contract
Languages and libraries that support Design by Contract (like Eiffel or Java with Contracts) allow you to formally specify preconditions, postconditions, and invariants:
/**
* @pre amount > 0
* @pre balance >= amount
* @post balance == old(balance) - amount
*/
public void withdraw(double amount) {
balance -= amount;
}
4. Immutable Data Structures
Using immutable objects eliminates entire categories of bugs and reduces the need for defensive copying:
// With mutable objects, defensive copying is needed
public List<String> getTags() {
return new ArrayList<>(this.tags); // Defensive copy
}
// With immutable collections, no defensive copying needed
public ImmutableList<String> getTags() {
return this.tags; // Safe to return directly
}
5. Type Systems That Prevent Null References
Languages like Kotlin, Swift, or Rust have type systems that help avoid null reference issues:
// Kotlin example
fun processUser(user: User) {
// No null check needed - compiler ensures user is non-null
println(user.name)
}
fun findUser(id: String): User? {
// Return type explicitly indicates nullable
return repository.findById(id)
}
// Caller must handle null case
val user = findUser(id)
if (user != null) {
processUser(user)
}
// Or using safe call operator
findUser(id)?.let { processUser(it) }
Conclusion: Finding the Right Balance
Defensive programming is a valuable technique when applied judiciously. The key is to find the right balance between defending against truly exceptional conditions and maintaining clean, readable code.
Instead of reflexively adding defensive code everywhere, ask yourself:
- Is this a system boundary where input validation is critical?
- Does this defensive check document an important invariant?
- Am I hiding a bug that should be fixed instead?
- Could I use language features or design patterns to eliminate the need for this check?
Remember that the goal of defensive programming is to create more robust software, not to complicate your codebase or mask underlying issues. By being strategic about where and how you apply defensive techniques, you can write code that’s both robust and maintainable.
The next time you’re about to add another null check or try-catch block, pause and consider whether you’re preventing a bug or potentially creating one. Your future self (and your teammates) will thank you for striking the right balance.