Why Your Debugging Tools Aren’t Helping Solve Real Issues
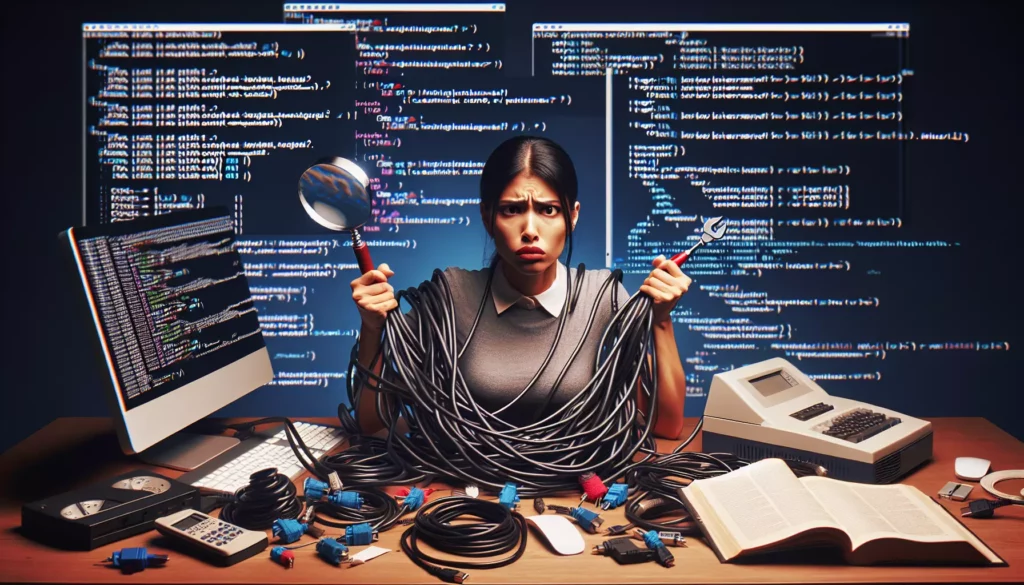
As developers, we’ve all been there: staring at error messages, stepping through code line by line, and still struggling to find that elusive bug. Despite having access to powerful debugging tools, we often find ourselves stuck in debugging loops that seem to go nowhere. But why do our sophisticated debugging tools sometimes fail to help us solve real issues?
In this comprehensive guide, we’ll explore the limitations of traditional debugging approaches, why they fall short, and how to adopt more effective debugging strategies that address the root causes of problems rather than just their symptoms.
Table of Contents
- Traditional Debugging Tools and Their Limitations
- The Debugging Mindset Problem
- Why Bugs Are Often Systemic Issues
- Effective Debugging Strategies for Real World Problems
- Case Studies: When Debugging Tools Failed
- A Better Approach to Debugging
- Conclusion
Traditional Debugging Tools and Their Limitations
Modern IDEs come packed with impressive debugging features: breakpoints, watch expressions, call stack analysis, and more. These tools are incredibly useful for certain types of bugs, but they have significant limitations when dealing with complex, real world issues.
The Limitations of Breakpoints and Step Debugging
Step debugging is perhaps the most commonly used debugging technique. It allows developers to execute code line by line, examining the state at each step. While powerful, step debugging has several limitations:
- Time consumption: Stepping through large codebases is extremely time consuming, especially when you’re not sure where the problem might be.
- Observer effect: The act of debugging can sometimes alter the behavior of the program, especially in timing sensitive or multi threaded applications.
- Context blindness: When focused on individual lines of code, you often miss the bigger picture of how components interact.
- Non deterministic issues: Race conditions, timing related bugs, and issues that only occur in production environments often can’t be reproduced in step debugging.
Consider this scenario: your web application works perfectly in development but experiences intermittent failures in production. Setting breakpoints won’t help because the issue only manifests under specific load conditions that can’t be replicated in a debugging environment.
The Problem with Log Based Debugging
Logging is another staple in the debugging toolkit. Developers often pepper their code with log statements to track execution flow and variable states. However, log based debugging comes with its own set of challenges:
- Log overload: Too many log statements create noise that makes it difficult to identify relevant information.
- Missing context: Logs often lack the full context needed to understand complex interactions between components.
- After the fact analysis: Logs can tell you what happened, but often not why it happened.
- Performance impact: Excessive logging can significantly impact application performance, potentially masking or altering the actual issue.
Why Static Analysis Tools Fall Short
Static code analyzers and linters are excellent for catching certain classes of bugs before they even make it to runtime. Tools like ESLint for JavaScript, mypy for Python, or SonarQube for multiple languages can identify potential issues through code analysis. However, they have clear limitations:
- False positives: These tools often flag code that isn’t actually problematic.
- False negatives: They miss many bugs that depend on runtime behavior or complex interactions.
- Logic errors: Static analyzers can’t catch logical errors where the code is syntactically correct but doesn’t do what was intended.
- Domain specific issues: They lack understanding of your application’s specific domain requirements.
For example, a static analyzer might help you catch a null reference exception, but it won’t identify that your e commerce checkout flow has a logical flaw that only appears when specific products are combined in a cart.
The Debugging Mindset Problem
Beyond the technical limitations of debugging tools, there’s a more fundamental issue at play: the debugging mindset most developers adopt.
Symptom Focused vs. Root Cause Analysis
Many developers fall into the trap of focusing on symptoms rather than root causes. When an error occurs, the natural instinct is to fix the immediate issue rather than understanding why it happened in the first place.
For example, if a web application is throwing a null reference exception, the quick fix might be to add a null check. But the real question should be: why is a null value reaching this point in the code? Is it due to:
- A missing validation earlier in the process?
- An incorrect assumption about the data flow?
- A misunderstanding of the API contract?
- A race condition in asynchronous code?
Addressing the symptom might temporarily resolve the issue, but without tackling the root cause, similar bugs will likely reappear in different forms.
The Confirmation Bias in Debugging
Confirmation bias is a cognitive trap that affects debugging in profound ways. When developers have a hypothesis about what’s causing a bug, they tend to look for evidence that confirms their theory while ignoring contradictory information.
This leads to debugging sessions where we convince ourselves that we’ve found the issue, only to discover later that we were looking in the wrong place entirely. Our debugging tools amplify this problem by giving us the ability to focus narrowly on the areas we already suspect, potentially missing the actual cause.
Consider this common scenario:
function processUserData(userData) {
// Developer suspects the bug is here
const formattedData = formatData(userData);
// But the actual issue is in this function
const validatedData = validateData(formattedData);
return processValidatedData(validatedData);
}
If you’re convinced the bug is in the formatData
function, you might set breakpoints there, examine its inputs and outputs in detail, and miss entirely that validateData
is silently corrupting the data.
The Tool Familiarity Trap
Another mindset issue is our tendency to use the debugging tools we’re most familiar with, regardless of whether they’re appropriate for the problem at hand. As the saying goes, “When you have a hammer, everything looks like a nail.”
If you’re most comfortable with the browser’s DevTools debugger, you might spend hours stepping through frontend code when the real issue is in the API response data. Or if you rely heavily on logging, you might add more and more log statements instead of using a more appropriate tool like a network analyzer or performance profiler.
Why Bugs Are Often Systemic Issues
Many of the most challenging bugs in software development aren’t isolated issues in a single function or module; they’re systemic problems that emerge from the interaction of multiple components.
The Emergence of Complex Bugs
Complex systems exhibit emergent behavior that can’t be predicted by analyzing individual components in isolation. This is particularly true in modern software architectures with:
- Microservices communicating over networks
- Asynchronous and event driven programming
- Distributed databases and caching layers
- Third party integrations and APIs
- Concurrent and parallel execution
In these environments, bugs often emerge from the interaction patterns between components rather than from flaws in any single component. Traditional debugging tools, which focus on the execution of code within a single process or thread, are ill equipped to identify these systemic issues.
State Management Complexity
State management is one of the most common sources of bugs in modern applications. As systems grow more complex, tracking how state changes propagate through the application becomes increasingly difficult.
Consider a React application with Redux for state management, communicating with a backend API that manages its own state, all while maintaining local state in the browser. A bug might manifest in the UI but originate from a subtle interaction between these different state management systems.
Traditional debuggers can show you the state at a specific point in time, but they struggle to help you understand how that state evolved and which interactions contributed to an incorrect state.
Timing and Concurrency Issues
Some of the most insidious bugs involve timing and concurrency. These issues are particularly challenging because:
- They often can’t be reliably reproduced in a debugging environment
- The act of debugging (which slows execution) can mask the problem
- They depend on specific sequences of events that may vary between runs
- They might only appear under certain load conditions
For example, consider this simplified JavaScript code that might contain a race condition:
async function fetchUserData() {
const userData = await fetchFromAPI('/user');
updateUserInterface(userData);
}
async function fetchPreferences() {
const preferences = await fetchFromAPI('/preferences');
applyPreferences(preferences);
}
// Called when page loads
function initializePage() {
fetchUserData();
fetchPreferences();
}
If applyPreferences
depends on the UI being already updated with user data, this code might work 99% of the time when fetchUserData
completes first. But occasionally, when fetchPreferences
completes first, subtle bugs appear. Traditional debugging tools might not help identify this issue, especially if it rarely occurs during development.
Effective Debugging Strategies for Real World Problems
Now that we’ve explored why traditional debugging tools often fall short, let’s examine more effective strategies for tackling complex, real world bugs.
System Level Observability
Rather than focusing solely on code execution, effective debugging often requires system level observability. This means implementing tools and practices that give you visibility into the behavior of your entire system:
- Distributed tracing: Tools like Jaeger, Zipkin, or AWS X Ray can track requests as they flow through multiple services, helping you identify where things go wrong in a distributed system.
- Metrics collection: Monitoring key metrics can help identify patterns and anomalies that point to underlying issues. Tools like Prometheus, Grafana, or Datadog excel at this.
- Structured logging: Moving beyond simple log messages to structured logging with context enables more powerful analysis. Consider using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk.
- Health checks and synthetic transactions: Regularly testing critical paths through your application can help identify issues before users encounter them.
By implementing these observability practices, you create a foundation for understanding system behavior that goes far beyond what traditional debuggers can offer.
Hypothesis Driven Debugging
Rather than randomly trying different approaches when debugging, adopt a scientific, hypothesis driven method:
- Observe: Gather as much information as possible about the bug, including when it occurs, what the symptoms are, and any patterns you can identify.
- Hypothesize: Formulate a specific, testable hypothesis about what might be causing the issue.
- Test: Design a targeted experiment to test your hypothesis. This might involve adding specific instrumentation, modifying code, or creating a simplified reproduction case.
- Analyze: Evaluate the results of your test. Did it confirm or refute your hypothesis?
- Refine: Based on what you learned, refine your hypothesis or formulate a new one, then repeat the process.
This approach helps avoid the common pitfalls of random debugging and confirmation bias, making your debugging process more methodical and effective.
Simplification and Isolation
When facing complex bugs, one of the most powerful strategies is to simplify and isolate the problem:
- Create minimal reproduction cases: Strip away everything that’s not essential to reproducing the bug. This often reveals the core issue more clearly.
- Use feature flags: Implement feature flags that allow you to turn specific functionality on or off, helping isolate which components contribute to an issue.
- Mock dependencies: Replace complex dependencies with simplified mock implementations to eliminate variables.
- Binary search debugging: Systematically disable half of your system at a time to narrow down where the problem lies.
For example, if you’re experiencing performance issues in a complex web application, you might create a simplified version with just the core functionality, then gradually add components back until the performance problem reappears.
Leveraging Production Data Safely
Some bugs only appear in production environments due to scale, real user behavior, or environmental factors. Effectively debugging these issues requires strategies for safely learning from production:
- Shadowing production traffic: Copy real production requests to a test environment to reproduce issues without affecting users.
- Canary deployments: Roll out changes to a small percentage of users to identify issues before full deployment.
- Feature flags in production: Use feature flags to enable experimental code or debugging instrumentation for specific subsets of users or requests.
- Production debugging tools: Implement tools that allow safe inspection of production systems, such as debugging proxies or enhanced logging that can be temporarily enabled.
These approaches help bridge the gap between development and production environments, making it possible to identify and fix issues that traditional debugging tools can’t catch.
Case Studies: When Debugging Tools Failed
Let’s examine some real world scenarios where traditional debugging approaches failed to solve complex problems, and explore the alternative approaches that eventually led to solutions.
Case Study 1: The Mysterious Memory Leak
A team was developing a Node.js application that would run fine for several days before gradually slowing down and eventually crashing with out of memory errors. Traditional debugging approaches failed:
- Memory snapshots in development couldn’t reproduce the issue
- Code reviews didn’t reveal any obvious memory leaks
- Adding more logging just increased memory usage, making the problem worse
The Solution: The team implemented a production monitoring solution that periodically captured and analyzed memory usage patterns. This revealed that a third party library was caching API responses without limits. The fix was simple once identified: configure a maximum cache size. But this issue would never have been found with traditional debugging tools because it only manifested after days of specific usage patterns.
Case Study 2: The Intermittent Payment Failure
An e commerce platform was experiencing occasional payment processing failures. The issue was particularly troubling because:
- It affected only about 0.5% of transactions
- It couldn’t be reproduced in testing environments
- Logs showed successful API calls to the payment processor
- Debugging with breakpoints was impossible as it never occurred during development
The Solution: The breakthrough came when the team implemented distributed tracing across their microservices architecture. The traces revealed that in certain rare cases, a race condition between the inventory service and the payment service was allowing customers to purchase out of stock items. When the inventory check eventually failed, the payment would be processed but the order would fail, leaving customers charged without receiving confirmation. This systemic issue couldn’t be identified by debugging any single service in isolation.
Case Study 3: The Database Performance Mystery
A web application was experiencing sporadic performance issues, with database queries occasionally taking 10-20 times longer than normal. The team tried:
- Analyzing query execution plans, which looked optimal
- Adding indexes based on query analysis
- Optimizing SQL queries
- Profiling the database server
None of these approaches identified the root cause.
The Solution: The team eventually correlated the performance issues with their deployment schedule and discovered that an automated database statistics update was running concurrently with peak traffic times after each deployment. This systemic issue involving deployment processes, database maintenance, and traffic patterns couldn’t be identified through code level debugging.
Lessons from the Case Studies
These case studies highlight several important lessons:
- Complex bugs often involve interactions between multiple systems
- Some issues only manifest under specific conditions that can’t be easily reproduced
- Looking beyond the code to processes, configurations, and system interactions is often necessary
- Correlation of data from multiple sources can reveal patterns that aren’t visible when looking at individual components
A Better Approach to Debugging
Based on the limitations we’ve explored and the lessons from real world case studies, here’s a more effective approach to debugging that goes beyond traditional tools.
Design for Debuggability
The most effective debugging often starts before bugs even occur, by designing systems that are easier to debug:
- Build with observability in mind: Instrument critical paths in your code with appropriate telemetry from the beginning.
- Design clear component boundaries: Well defined interfaces between components make it easier to isolate and test individual parts of the system.
- Implement circuit breakers and bulkheads: Design your system to fail in predictable, contained ways rather than with cascading failures.
- Create debugging hooks: Build in mechanisms that allow for enhanced debugging information to be collected when needed.
For example, consider implementing a debug mode that can be enabled for specific requests:
function processOrder(order, options = {}) {
const debugMode = options.debug || false;
if (debugMode) {
enableEnhancedLogging();
captureFullState();
}
// Normal processing logic
validateOrder(order);
processPayment(order);
if (debugMode) {
return {
result: order,
debugInfo: collectDebugInformation()
};
}
return order;
}
Holistic Debugging Approaches
Rather than relying solely on code level debugging tools, adopt a more holistic approach:
- System mapping: Create visual maps of your system’s components and their interactions to help identify potential failure points.
- Cross functional debugging: Involve team members with different expertise (frontend, backend, operations, etc.) in debugging sessions for complex issues.
- User journey analysis: Trace issues through the entire user journey rather than focusing only on where errors are reported.
- Environmental analysis: Consider how infrastructure, configuration, and external dependencies might contribute to issues.
This approach recognizes that bugs often exist in the spaces between traditional areas of focus.
Building a Debugging Toolkit Beyond Traditional Tools
Expand your debugging toolkit beyond traditional debuggers and loggers:
- Network analysis tools: Tools like Wireshark, Charles Proxy, or browser network panels can reveal issues in API calls and data transfer.
- Time travel debugging: Tools like rr for C/C++, Replay.io for JavaScript, or Thonny for Python allow you to record program execution and then move forward and backward through it.
- Chaos engineering tools: Controlled fault injection with tools like Chaos Monkey can reveal how your system behaves under unexpected conditions.
- Performance profilers: Tools like Flame Graphs, Chrome DevTools Performance tab, or language specific profilers help identify performance bottlenecks.
- State visualization tools: Tools that can visualize application state over time, such as Redux DevTools for React applications.
By combining these tools with traditional debugging approaches, you create a more comprehensive toolkit for addressing real world issues.
Collaborative Debugging Practices
Finally, recognize that debugging complex issues is often a team sport:
- Pair debugging: Work with another developer to debug issues, combining different perspectives and expertise.
- Bug bounties and hackathons: For particularly challenging issues, consider involving a wider group through internal bug bounties or dedicated debugging sessions.
- Post mortem analysis: After resolving significant issues, conduct thorough post mortems to understand root causes and improve systems.
- Knowledge sharing: Create a culture of sharing debugging techniques and lessons learned to improve the team’s collective debugging capabilities.
These collaborative practices help overcome individual blind spots and biases that can prevent effective debugging.
Conclusion
Traditional debugging tools remain valuable, but they’re simply not enough for tackling the complex, systemic issues that emerge in modern software systems. By recognizing the limitations of these tools and adopting more holistic, system oriented debugging approaches, you can more effectively solve the real issues that affect your applications.
The key takeaways from this exploration are:
- Look beyond the code to understand system interactions and environmental factors
- Design for debuggability from the beginning
- Adopt a scientific, hypothesis driven approach to debugging
- Expand your toolkit beyond traditional debuggers and loggers
- Leverage observability and telemetry to understand system behavior
- Collaborate across specialties to debug complex issues
By shifting your debugging mindset and practices in these ways, you’ll be better equipped to solve the kinds of complex, real world issues that traditional debugging tools often can’t help with. Remember, effective debugging isn’t just about finding and fixing bugs; it’s about understanding your system more deeply and using that understanding to build more robust software.
The next time you find yourself stuck in a debugging loop, step back and consider whether you’re using the right approach for the problem at hand. The most powerful debugging tool remains the developer’s mind, especially when equipped with a diverse toolkit and systematic methodology.