Why Your Database Queries Are Slow Despite Proper Indexing
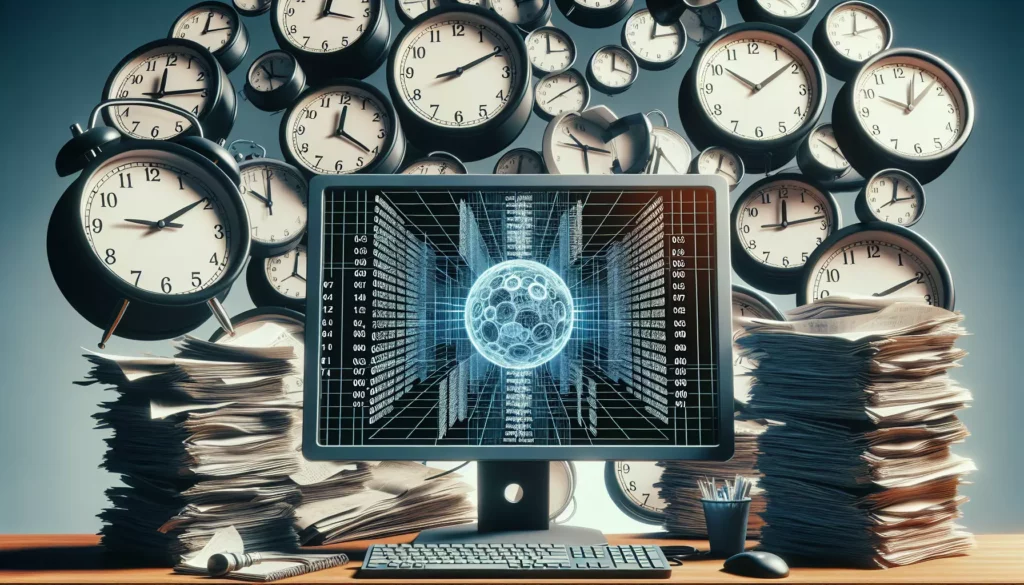
You’ve done everything right. You’ve created indexes on all the right columns. You’ve even analyzed your query execution plans. Yet somehow, your database queries are still crawling along at a frustrating pace. What gives?
Database performance optimization is often more art than science. Even with seemingly proper indexing in place, there are numerous subtle factors that can cause queries to underperform. In this comprehensive guide, we’ll explore the hidden reasons why your queries might still be slow despite your best indexing efforts, and provide actionable solutions to address these issues.
Table of Contents
- Understanding Indexes: Beyond the Basics
- Query Structure Problems
- Data Distribution and Statistics Issues
- Hardware and Configuration Limitations
- Workload and Concurrency Factors
- Advanced Optimization Techniques
- Case Study: Transforming a Slow Query
- Conclusion
Understanding Indexes: Beyond the Basics
Before diving into the problems, let’s ensure we have a solid understanding of how indexes really work. Creating an index doesn’t automatically guarantee it will be used effectively.
Index Selection and the Query Optimizer
Database query optimizers make decisions about which indexes to use based on complex algorithms and statistics. Sometimes, what seems like the obvious index choice to a human might not be what the optimizer selects.
For instance, if you have an index on a column with low cardinality (few unique values), the optimizer might determine a full table scan is actually more efficient than using the index. This is because retrieving a large percentage of rows through an index can be less efficient than simply scanning the entire table.
To check which indexes your database is actually using:
- In MySQL: Use
EXPLAIN
before your query - In PostgreSQL: Use
EXPLAIN ANALYZE
- In SQL Server: Use
SET STATISTICS IO ON; SET STATISTICS TIME ON;
before your query - In Oracle: Use
EXPLAIN PLAN FOR
followed bySELECT * FROM TABLE(DBMS_XPLAN.DISPLAY)
Composite Indexes and Column Order
A common misconception is that having individual indexes on columns A and B is equivalent to having a composite index on (A, B). This is not true! The order of columns in composite indexes matters significantly.
Consider a composite index on (last_name, first_name). This index will be efficient for queries that:
- Filter on last_name only
- Filter on both last_name and first_name
But it will not be efficient for queries that only filter on first_name. This is because indexes work from left to right.
For example, in PostgreSQL, this query would use the index effectively:
SELECT * FROM users WHERE last_name = 'Smith' AND first_name = 'John';
But this query likely wouldn’t use the index efficiently:
SELECT * FROM users WHERE first_name = 'John';
Index Types and Their Applications
Different database systems offer various specialized index types, each suited for specific query patterns:
- B-tree indexes: General-purpose indexes good for equality and range queries
- Hash indexes: Excellent for equality comparisons but useless for ranges
- GIN/GiST indexes (PostgreSQL): Optimized for full-text search and complex data types
- Spatial indexes: Designed for geographic data
- Bitmap indexes: Efficient for low-cardinality columns in data warehousing
Using the wrong index type for your query pattern can lead to suboptimal performance. For instance, a hash index won’t help with a range query, even if it’s on the right column.
Query Structure Problems
Sometimes the issue isn’t with your indexes but with how your queries are structured. Let’s explore common query-related issues that can nullify the benefits of your indexes.
Function-Based Conditions
One of the most common reasons indexes aren’t used is when functions are applied to indexed columns in WHERE clauses. When you apply a function to a column, the database typically can’t use an index on that column.
For example, this query likely won’t use an index on the created_at column:
SELECT * FROM orders WHERE EXTRACT(YEAR FROM created_at) = 2023;
Instead, consider rewriting it as:
SELECT * FROM orders WHERE created_at >= '2023-01-01' AND created_at < '2024-01-01';
For cases where you frequently query based on functions, consider creating a function-based index (supported in PostgreSQL, Oracle, and SQL Server):
CREATE INDEX idx_orders_year ON orders (EXTRACT(YEAR FROM created_at));
Implicit Type Conversions
When your query involves comparing columns of different data types, the database performs implicit type conversions. These conversions often prevent index usage.
For instance, if user_id is an INTEGER column with an index:
-- This might not use the index
SELECT * FROM users WHERE user_id = '42';
The string '42' is implicitly converted to an integer, potentially bypassing the index. Always use the correct data type in your queries:
-- This will use the index
SELECT * FROM users WHERE user_id = 42;
LIKE Queries with Leading Wildcards
While LIKE queries with patterns that don't start with a wildcard (%) can use indexes, those with leading wildcards typically cannot:
-- Can use an index
SELECT * FROM products WHERE product_name LIKE 'Apple%';
-- Typically cannot use an index effectively
SELECT * FROM products WHERE product_name LIKE '%Phone%';
For full-text search capabilities, consider using specialized features:
- PostgreSQL: Use the
pg_trgm
extension with GIN indexes - MySQL: Use FULLTEXT indexes
- SQL Server: Use Full-Text Search
- Oracle: Use Oracle Text
OR Conditions vs. UNION
Queries with OR conditions sometimes can't use indexes efficiently, especially when different columns are involved:
SELECT * FROM customers
WHERE last_name = 'Smith' OR email = 'john@example.com';
Consider rewriting with UNION:
SELECT * FROM customers WHERE last_name = 'Smith'
UNION
SELECT * FROM customers WHERE email = 'john@example.com';
This allows each part to use its respective index, although be cautious as UNION removes duplicates by default (use UNION ALL if that's not desired).
Suboptimal JOIN Order
The order in which tables are joined can significantly impact performance. While query optimizers generally do a good job, they sometimes make suboptimal choices, especially with complex queries involving many tables.
In some database systems, you can provide hints to influence join order. For example, in SQL Server:
SELECT o.order_id, c.customer_name
FROM orders o
INNER JOIN /*+ LEADING(customers) */ customers c
ON o.customer_id = c.customer_id;
Data Distribution and Statistics Issues
Sometimes the problem isn't with your queries or indexes but with how the database understands your data.
Outdated Statistics
Database query optimizers rely on statistics about your data to make decisions. If these statistics are outdated, the optimizer might make poor choices about index usage.
For example, if a table initially had 100 rows when statistics were gathered, but now has 1 million rows, the optimizer might still think a full table scan is efficient.
Ensure statistics are up to date:
- PostgreSQL:
ANALYZE tablename;
- MySQL:
ANALYZE TABLE tablename;
- SQL Server:
UPDATE STATISTICS tablename;
- Oracle:
EXEC DBMS_STATS.GATHER_TABLE_STATS('schema', 'tablename');
Skewed Data Distribution
When data is unevenly distributed, queries that work well for certain values might perform poorly for others. For example, if 90% of your users are from the United States, a query filtering for U.S. users might not use an index on the country column effectively.
Consider using filtered or partial indexes (supported in PostgreSQL and SQL Server) for these cases:
-- PostgreSQL partial index for non-US customers
CREATE INDEX idx_customers_country_non_us
ON customers(country)
WHERE country != 'United States';
Parameter Sniffing Issues
In systems that use prepared statements or stored procedures, a phenomenon called "parameter sniffing" can cause performance issues. The database might optimize a query for the first set of parameters it sees, which might be suboptimal for subsequent parameter values.
In SQL Server, you can address this with query hints:
-- Force the optimizer to create a new plan
SELECT * FROM orders WHERE customer_id = @customer_id OPTION(RECOMPILE);
In PostgreSQL, you might need to use EXECUTE
with dynamic SQL in functions to avoid this issue.
Hardware and Configuration Limitations
Sometimes the issue is simply that your database server lacks the resources to perform efficiently, or its configuration is suboptimal.
Insufficient Memory
If your database server doesn't have enough memory to hold frequently accessed data and indexes, it will resort to disk I/O, which is orders of magnitude slower.
Key memory-related settings to check:
- PostgreSQL:
shared_buffers
,work_mem
,maintenance_work_mem
- MySQL:
innodb_buffer_pool_size
- SQL Server: Max Server Memory setting
- Oracle: SGA and PGA sizes
Monitoring tools can help you determine if your server is memory-constrained. Look for high rates of disk I/O during query execution.
Disk I/O Bottlenecks
Even with adequate memory, slow disks can bottleneck your database performance, especially for write-heavy workloads or queries that can't be satisfied from memory.
Consider:
- Upgrading to SSDs if still using HDDs
- Using RAID configurations for better I/O performance
- In cloud environments, selecting storage options with higher IOPS
Suboptimal Configuration
Database systems have numerous configuration parameters that can significantly impact performance. Default configurations are often conservative and not optimized for specific workloads.
Key areas to review:
- Connection pooling: Establishing new connections is expensive; use connection pooling in your application
- Query cache settings: In MySQL, the query cache can help or hurt performance depending on your workload
- Autovacuum settings: In PostgreSQL, suboptimal autovacuum settings can lead to bloat and performance degradation
- Transaction log settings: Write performance can be impacted by transaction log configuration
Workload and Concurrency Factors
Sometimes queries that perform well in isolation become slow under real-world conditions due to workload characteristics.
Lock Contention
When multiple transactions try to access the same data simultaneously, they may block each other, causing seemingly simple queries to take much longer than expected.
To identify lock contention:
- PostgreSQL: Query the
pg_locks
table - MySQL: Use
SHOW PROCESSLIST
and look for queries in "Locked" state - SQL Server: Use
sys.dm_tran_locks
DMV - Oracle: Query
V$LOCK
To reduce lock contention:
- Keep transactions short and focused
- Consider using optimistic concurrency control instead of locks where appropriate
- Review isolation levels; sometimes a lower isolation level provides adequate protection with better concurrency
Connection Overload
Too many concurrent connections can overwhelm your database server, causing all queries to slow down. Each connection consumes memory and CPU resources.
Monitor your active connections and set appropriate limits. Use connection pooling in your application to reuse connections rather than creating new ones for each operation.
Background Processes Impact
Database systems perform various background tasks that can impact query performance:
- PostgreSQL: Autovacuum processes clean up dead tuples
- MySQL: InnoDB background threads for various tasks
- SQL Server: Checkpoint process, statistics auto-update
- Oracle: DBWR (Database Writer) and other background processes
If a slow query coincides with these background activities, it might be affected. Consider scheduling intensive background tasks during off-peak hours.
Advanced Optimization Techniques
When standard indexing isn't enough, consider these more advanced approaches.
Index-Only Scans
If your query only needs data that's contained within the index itself, the database can perform an index-only scan, avoiding the need to access the table data at all. This can be substantially faster.
For example, if you have an index on (customer_id, order_date) and run:
SELECT customer_id, order_date
FROM orders
WHERE customer_id = 42;
The database might be able to satisfy this query using only the index, without touching the table data.
To leverage this:
- Include all needed columns in your index (using INCLUDE in SQL Server or PostgreSQL)
- In PostgreSQL, ensure VACUUM runs regularly to update the visibility map
Materialized Views
For complex queries that are run frequently but don't need up-to-the-second data, materialized views can dramatically improve performance by precomputing and storing results.
In PostgreSQL:
CREATE MATERIALIZED VIEW mv_monthly_sales AS
SELECT
date_trunc('month', order_date) as month,
product_id,
SUM(quantity) as units_sold,
SUM(price * quantity) as revenue
FROM orders
GROUP BY 1, 2;
You'll need to refresh the materialized view periodically:
REFRESH MATERIALIZED VIEW mv_monthly_sales;
Table Partitioning
For very large tables, partitioning divides data into smaller, more manageable pieces based on a key like date or region. This can significantly improve query performance by allowing the database to scan only relevant partitions.
In PostgreSQL 10+:
CREATE TABLE orders (
order_id SERIAL,
customer_id INTEGER,
order_date DATE,
amount DECIMAL(10,2)
) PARTITION BY RANGE (order_date);
CREATE TABLE orders_2022 PARTITION OF orders
FOR VALUES FROM ('2022-01-01') TO ('2023-01-01');
CREATE TABLE orders_2023 PARTITION OF orders
FOR VALUES FROM ('2023-01-01') TO ('2024-01-01');
Now, a query filtering on order_date will only scan the relevant partition:
SELECT * FROM orders WHERE order_date BETWEEN '2023-01-01' AND '2023-01-31';
Query Rewriting and Denormalization
Sometimes the most effective approach is to fundamentally change how data is structured or accessed:
- Denormalization: Storing redundant data to avoid joins
- Precalculating results: Storing computed values rather than calculating them on the fly
- Caching: Using application-level caching for frequently accessed, relatively static data
These approaches trade some data integrity or storage efficiency for performance gains.
Case Study: Transforming a Slow Query
Let's walk through optimizing a real-world slow query despite seemingly proper indexing.
The Initial Situation
Consider this query from an e-commerce application:
SELECT
o.order_id,
o.order_date,
c.customer_name,
SUM(oi.quantity * oi.unit_price) as total_amount
FROM
orders o
JOIN customers c ON o.customer_id = c.customer_id
JOIN order_items oi ON o.order_id = oi.order_id
WHERE
o.order_date BETWEEN '2023-01-01' AND '2023-12-31'
AND c.country = 'Canada'
GROUP BY
o.order_id, o.order_date, c.customer_name
ORDER BY
o.order_date DESC
LIMIT 100;
The database has these indexes:
- orders: Primary key on order_id, index on customer_id, index on order_date
- customers: Primary key on customer_id, index on country
- order_items: Primary key on (order_id, item_id), index on product_id
Despite these indexes, the query takes over 10 seconds to execute.
Diagnosing the Issues
Running EXPLAIN ANALYZE reveals several issues:
- The query is joining tables in a suboptimal order, starting with orders and then filtering by country later
- The GROUP BY operation is using a memory-intensive hash aggregate
- The sort operation for ORDER BY is performed after aggregation on a large result set
Step 1: Optimize Join Order
First, let's help the query optimizer by starting with the most restrictive filter:
SELECT
o.order_id,
o.order_date,
c.customer_name,
SUM(oi.quantity * oi.unit_price) as total_amount
FROM
customers c
JOIN orders o ON c.customer_id = o.customer_id
JOIN order_items oi ON o.order_id = oi.order_id
WHERE
c.country = 'Canada'
AND o.order_date BETWEEN '2023-01-01' AND '2023-12-31'
GROUP BY
o.order_id, o.order_date, c.customer_name
ORDER BY
o.order_date DESC
LIMIT 100;
This simple reordering reduces the working set early in the execution plan.
Step 2: Add a Covering Index
Let's create a composite index that covers all the fields needed from the orders table:
CREATE INDEX idx_orders_customer_date ON orders(customer_id, order_date DESC);
This index supports both the join condition and the date filtering, plus it's already sorted in descending date order.
Step 3: Consider a Materialized View
For even better performance, we could create a materialized view that precomputes the order totals:
CREATE MATERIALIZED VIEW mv_order_totals AS
SELECT
o.order_id,
o.order_date,
o.customer_id,
SUM(oi.quantity * oi.unit_price) as total_amount
FROM
orders o
JOIN order_items oi ON o.order_id = oi.order_id
GROUP BY
o.order_id, o.order_date, o.customer_id;
Then our query becomes:
SELECT
ot.order_id,
ot.order_date,
c.customer_name,
ot.total_amount
FROM
customers c
JOIN mv_order_totals ot ON c.customer_id = ot.customer_id
WHERE
c.country = 'Canada'
AND ot.order_date BETWEEN '2023-01-01' AND '2023-12-31'
ORDER BY
ot.order_date DESC
LIMIT 100;
The Results
After these optimizations:
- The original query took over 10 seconds
- After join reordering and the new index: 500ms
- With the materialized view approach: 50ms
That's a 200x improvement! And we didn't need to change any hardware or major database configuration.
Conclusion
When database queries remain slow despite proper indexing, the solution often lies in understanding the subtle interplay between your specific data, query patterns, and the database system's optimizer behavior. We've explored numerous factors that can cause unexpected performance issues:
- Index selection and usage challenges
- Query structure problems that prevent index usage
- Data distribution and statistics issues
- Hardware and configuration limitations
- Workload and concurrency factors
We've also covered advanced techniques to address these issues, from composite indexes and index-only scans to materialized views and table partitioning.
Remember that database optimization is an iterative process. Monitor your queries in production, analyze their execution plans, and be prepared to adjust your strategy as your data and access patterns evolve. Sometimes, the most effective solution involves rethinking your database schema or application design rather than simply tweaking indexes.
By applying the principles and techniques discussed in this guide, you'll be well-equipped to diagnose and resolve even the most perplexing database performance issues, ensuring your applications remain responsive and efficient as they grow.
As a final note, always test your optimizations in an environment that closely resembles production before deploying changes. What works well in development might behave differently under real-world conditions and data volumes.