Why Your Continuous Integration Pipeline Keeps Failing (And How to Fix It)
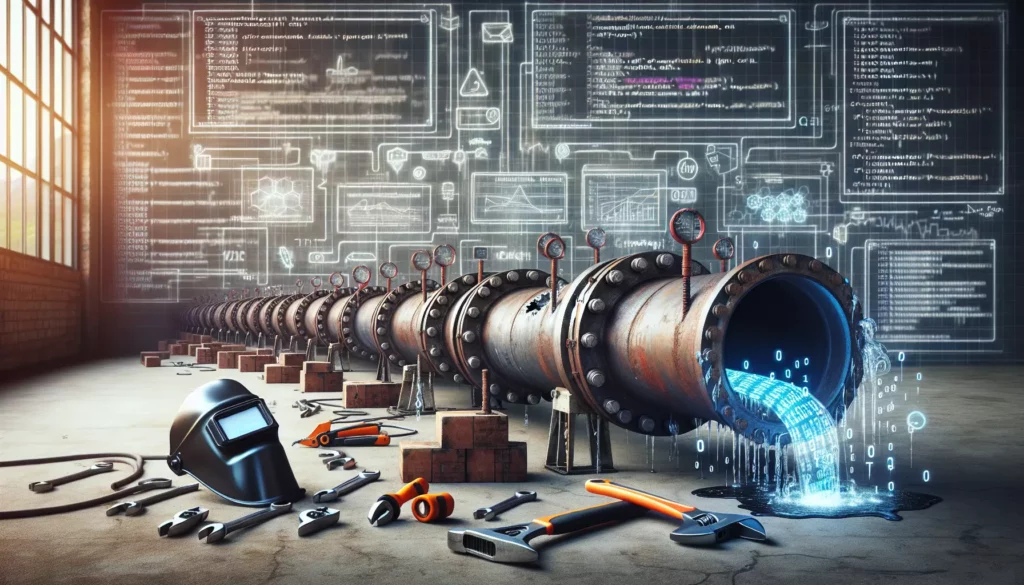
In the fast paced world of software development, continuous integration (CI) pipelines have become essential for teams aiming to deliver high quality code consistently. However, many developers find themselves repeatedly facing the frustration of failed builds, mysterious test errors, and pipelines that seem to work locally but break in CI environments. If you’re nodding your head in agreement, you’re not alone.
This comprehensive guide will dive deep into the most common reasons why CI pipelines fail and provide practical solutions to help you build more robust, reliable automation. By understanding these failure points, you’ll be able to create more stable pipelines, reduce debugging time, and ultimately ship better code faster.
Table of Contents
- Understanding CI Pipeline Failures
- Environment and Configuration Issues
- Test Flakiness and Instability
- Dependency Management Problems
- Resource Constraints and Performance Issues
- Integration Gaps Between Tools
- Code Quality and Static Analysis Failures
- Security Scanning Failures
- Effective Debugging Strategies
- Best Practices for Robust CI Pipelines
- Conclusion
1. Understanding CI Pipeline Failures
Before diving into specific issues, it’s important to understand what a CI pipeline failure actually means. A failing pipeline is essentially a signal that something in your development process needs attention. Rather than viewing failures as obstacles, they should be seen as valuable feedback mechanisms that protect your codebase from potential issues.
CI failures typically fall into a few major categories:
- Build failures: Code doesn’t compile or package properly
- Test failures: Automated tests don’t pass
- Environment issues: Discrepancies between development and CI environments
- Dependency problems: Missing or incompatible dependencies
- Resource constraints: Timeouts or memory limitations
- Configuration errors: Incorrect pipeline configuration
Now, let’s explore each of these areas in detail and learn how to address them effectively.
2. Environment and Configuration Issues
One of the most common sources of CI pipeline failures stems from discrepancies between development environments and CI environments. The infamous “it works on my machine” problem is real, and it can cause significant frustration.
Common Environment Issues
- Different operating systems: Developing on macOS but running CI on Linux
- Inconsistent tool versions: Using different versions of compilers, interpreters, or build tools
- Missing environment variables: Configuration that exists locally but not in CI
- File path differences: Using absolute paths or platform specific path separators
- Timezone and locale differences: Tests that depend on specific date/time formatting
Solutions for Environment Issues
Use Containerization
Docker containers provide a consistent environment across development and CI systems. By defining your environment in a Dockerfile, you ensure everyone uses identical setups.
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["npm", "test"]
Implement Configuration as Code
Store all configuration in version controlled files rather than relying on manual setup. Tools like Terraform, Ansible, or even simple shell scripts can help ensure consistency.
Define Environment Variables Properly
Document all required environment variables and provide sensible defaults when possible. Most CI systems offer secure ways to store sensitive values:
# Example .env.example file to document required variables
DATABASE_URL=postgresql://localhost:5432/myapp
API_KEY=your_api_key_here
DEBUG=false
Use Path Relativity
Always use relative paths in your code and configuration. For cross platform compatibility, use path manipulation libraries rather than hardcoded separators:
// JavaScript example
const path = require('path');
const configPath = path.join(__dirname, 'config', 'settings.json');
Implement Environment Parity
Tools like GitHub Codespaces, GitPod, or even simple Vagrant configurations can help ensure developers work in environments that match production and CI closely.
3. Test Flakiness and Instability
Flaky tests are those that sometimes pass and sometimes fail without any actual code changes. They are one of the most frustrating causes of pipeline failures because they’re often difficult to reproduce and debug.
Common Causes of Test Flakiness
- Race conditions: Tests that depend on specific timing
- Resource contention: Tests competing for shared resources
- External dependencies: Reliance on third party services
- Order dependency: Tests that only pass in a specific execution order
- Insufficient waiting: Not properly waiting for asynchronous operations
- Improper cleanup: Tests that don’t clean up after themselves
Solutions for Test Flakiness
Implement Proper Isolation
Ensure each test runs in isolation without depending on the state from other tests. Use setup and teardown methods to create clean environments for each test.
// JavaScript test example with proper setup/teardown
describe('User service', () => {
let testDatabase;
beforeEach(async () => {
// Create fresh database for each test
testDatabase = await createTestDatabase();
});
afterEach(async () => {
// Clean up after test
await testDatabase.cleanup();
});
test('should create user', async () => {
// Test with clean database
});
});
Mock External Dependencies
Replace calls to external APIs or services with mocks or stubs to eliminate network related flakiness:
// Python example using unittest.mock
@patch('app.services.payment_gateway.charge')
def test_payment_processing(self, mock_charge):
mock_charge.return_value = {'success': True, 'id': '12345'}
result = process_payment(100, 'usd', 'card_token')
self.assertTrue(result.is_successful)
mock_charge.assert_called_once()
Implement Retry Logic for Flaky Tests
For tests that are inherently difficult to stabilize, consider implementing retry logic. While this doesn’t solve the root cause, it can improve pipeline reliability:
// Jest example with retry plugin
jest.retryTimes(3)
test('occasionally flaky integration test', () => {
// Test implementation
});
Use Asynchronous Testing Properly
Make sure you’re correctly handling async operations in tests, using appropriate waiting mechanisms:
// JavaScript async test example
test('async operation completes', async () => {
const result = await asyncOperation();
expect(result).toBe('expected value');
});
Implement Quarantine for Known Flaky Tests
Separate known flaky tests into a different test suite that doesn’t block the main pipeline. This allows you to fix them incrementally without disrupting the team.
4. Dependency Management Problems
Dependency issues are another major source of CI failures. These occur when your application depends on external libraries or services that aren’t correctly configured in the pipeline.
Common Dependency Problems
- Missing dependencies: Required packages not installed in CI
- Version conflicts: Incompatible versions of libraries
- Transitive dependency issues: Conflicts in dependencies of dependencies
- Network failures: Inability to download dependencies during build
- Private package access: Lack of authentication for private repositories
Solutions for Dependency Problems
Use Lock Files
Lock files specify exact versions of all dependencies, including transitive ones. Most package managers support them:
- npm/yarn: package-lock.json or yarn.lock
- Python: requirements.txt with pinned versions or Pipfile.lock
- Ruby: Gemfile.lock
- Go: go.sum
Implement Dependency Caching
Most CI systems support caching dependencies to speed up builds and reduce network related failures:
# GitHub Actions example with caching
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
with:
node-version: '14'
- name: Cache dependencies
uses: actions/cache@v2
with:
path: ~/.npm
key: ${{ runner.os }}-node-${{ hashFiles('**/package-lock.json') }}
- run: npm ci
- run: npm test
Use Private Repository Authentication
For private dependencies, configure proper authentication in your CI environment:
# .npmrc example for private registry
@mycompany:registry=https://npm.mycompany.com/
//npm.mycompany.com/:_authToken=${NPM_TOKEN}
Implement Dependency Scanning
Regularly scan dependencies for security vulnerabilities and incompatibilities. Tools like Dependabot, Snyk, or OWASP Dependency Check can automate this process.
Consider Vendoring Dependencies
For critical dependencies or environments with limited network access, consider vendoring (including dependencies directly in your repository).
5. Resource Constraints and Performance Issues
CI environments often have different resource constraints than development machines. This can lead to timeouts, memory issues, and other performance related failures.
Common Resource Constraint Issues
- Build timeouts: CI jobs exceeding allocated time limits
- Memory exhaustion: Processes requiring more memory than available
- CPU limitations: Slower processing affecting time sensitive tests
- Disk space issues: Insufficient storage for build artifacts
- Network bandwidth: Slow downloads or uploads
Solutions for Resource Constraints
Optimize Test Execution
Run tests in parallel when possible and implement test sharding to distribute the workload:
# CircleCI example of test parallelism
version: 2.1
jobs:
test:
parallelism: 4
steps:
- checkout
- run:
name: Run tests in parallel
command: |
TESTFILES=$(find test -name "*_test.js" | circleci tests split --split-by=timings)
npm test $TESTFILES
Implement Build Caching
Cache build artifacts between runs to reduce build times:
# Gradle example with caching
apply plugin: 'java'
// Enable Gradle's build cache
org.gradle.caching=true
Monitor Resource Usage
Add monitoring to your CI jobs to identify resource bottlenecks:
# Bash script to monitor memory during test execution
#!/bin/bash
(
while true; do
ps -o pid,rss,command -p $$ | grep -v grep
sleep 1
done
) &
MONITOR_PID=$!
# Run your tests
npm test
# Kill the monitoring process
kill $MONITOR_PID
Use Appropriate CI Machine Sizes
Configure your CI provider to use machines with sufficient resources for your workload. This might cost more but can significantly improve reliability and developer productivity.
Implement Timeouts Strategically
Add explicit timeouts to tests and CI steps to prevent indefinite hanging:
# GitHub Actions timeout example
jobs:
build:
runs-on: ubuntu-latest
timeout-minutes: 30
steps:
- uses: actions/checkout@v2
- name: Build with timeout
timeout-minutes: 10
run: ./build.sh
6. Integration Gaps Between Tools
Modern CI pipelines often involve multiple tools and services working together. Gaps in this integration can lead to failures that are difficult to diagnose.
Common Integration Issues
- Authentication failures: Inability to access required services
- API changes: Updates to external APIs breaking integration
- Webhook failures: Communication breakdowns between systems
- Plugin compatibility: Outdated or incompatible CI plugins
- Data format mismatches: Different systems expecting different formats
Solutions for Integration Issues
Implement Integration Testing for CI
Create specific tests that verify your CI pipeline’s integration points work correctly:
#!/bin/bash
# Simple script to test if authentication to a service works
response=$(curl -s -o /dev/null -w "%{http_code}" -H "Authorization: Bearer $API_TOKEN" https://api.example.com/status)
if [ "$response" -ne 200 ]; then
echo "Authentication test failed with status $response"
exit 1
fi
echo "Authentication test passed"
Use API Versioning
When integrating with external APIs, always specify versions to prevent breaking changes:
# Example using a versioned API
curl -H "Accept: application/vnd.github.v3+json" https://api.github.com/repos/octocat/hello-world
Implement Circuit Breakers
Use circuit breaker patterns to gracefully handle integration failures:
# Python example with circuit breaker pattern
from pybreaker import CircuitBreaker
breaker = CircuitBreaker(fail_max=3, reset_timeout=30)
@breaker
def call_external_service():
return requests.get("https://api.example.com/data")
Use Integration Simulation
For testing, simulate external integrations with tools like WireMock or Prism:
# Docker compose example with mock service
version: '3'
services:
app:
build: .
depends_on:
- mock-api
environment:
- API_URL=http://mock-api:8080
mock-api:
image: stoplight/prism:4
command: mock -h 0.0.0.0 /api/openapi.yaml
volumes:
- ./api:/api
7. Code Quality and Static Analysis Failures
Many CI pipelines include code quality checks and static analysis tools that can cause failures when they detect issues.
Common Code Quality Issues
- Linting errors: Code style or formatting issues
- Code complexity: Functions or methods that are too complex
- Duplicate code: Repeated code patterns
- Code coverage: Insufficient test coverage
- Code smells: Problematic patterns identified by static analysis
Solutions for Code Quality Issues
Integrate Linting in Development
Run linters locally before committing to catch issues early:
# Example pre-commit hook for linting
#!/bin/sh
npx eslint . --ext .js,.jsx,.ts,.tsx
if [ $? -ne 0 ]; then
echo "Linting failed, fix errors before committing"
exit 1
fi
Automate Code Formatting
Use tools that automatically format code to prevent style related failures:
# Package.json example with format script
{
"scripts": {
"format": "prettier --write \"**/*.{js,jsx,ts,tsx,json,md}\"",
"precommit": "npm run format && npm run lint"
}
}
Set Appropriate Thresholds
Configure quality tools with appropriate thresholds that balance quality with practicality:
# Example SonarQube quality gate configuration
sonar.qualitygate.name=Standard
sonar.qualitygate.conditions=\
metric=coverage,op=LT,error=80;\
metric=code_smells,op=GT,error=100;\
metric=bugs,op=GT,error=0;\
metric=vulnerabilities,op=GT,error=0
Gradually Improve Code Quality
For existing projects, gradually improve quality rather than enforcing perfection immediately:
# ESLint configuration with overrides for legacy code
{
"rules": {
"complexity": ["error", 10]
},
"overrides": [
{
"files": ["src/legacy/**/*.js"],
"rules": {
"complexity": ["warn", 20]
}
}
]
}
8. Security Scanning Failures
Security scans in CI pipelines can fail due to detected vulnerabilities or misconfigurations.
Common Security Scanning Issues
- Dependency vulnerabilities: Known security issues in libraries
- Secrets detection: Accidentally committed credentials or tokens
- Container vulnerabilities: Security issues in container images
- SAST findings: Static Application Security Testing issues
- License compliance: Unauthorized or incompatible licenses
Solutions for Security Scanning Issues
Implement Pre commit Hooks for Secrets
Prevent secrets from being committed using tools like git-secrets:
# Setup git-secrets hooks
git secrets --install
git secrets --register-aws
git secrets --add 'private_key'
git secrets --add 'api_key'
Regularly Update Dependencies
Set up automated dependency updates with security fixes:
# GitHub Dependabot configuration
# .github/dependabot.yml
version: 2
updates:
- package-ecosystem: "npm"
directory: "/"
schedule:
interval: "weekly"
labels:
- "dependencies"
ignore:
- dependency-name: "express"
versions: ["4.x.x"]
Use Security Scanning with Baseline
For existing projects, establish a baseline and focus on preventing new issues:
# OWASP ZAP baseline scan example
zap-baseline.py -t https://example.com -c config.conf -B baseline.json
Implement Security as Code
Define security policies as code to ensure consistency:
# Example Terraform security policy
resource "aws_s3_bucket" "data" {
bucket = "my-data-bucket"
acl = "private"
server_side_encryption_configuration {
rule {
apply_server_side_encryption_by_default {
sse_algorithm = "AES256"
}
}
}
}
9. Effective Debugging Strategies
When your CI pipeline fails despite your best efforts, effective debugging strategies are essential.
Key Debugging Approaches
Enhance Logging
Add detailed logging to help identify issues:
# Bash example with enhanced logging
set -x # Print commands before execution
echo "Starting build process..."
npm ci
echo "Dependencies installed, starting tests..."
npm test
Reproduce Locally
Create a local environment that mimics CI as closely as possible:
# Docker example to reproduce CI environment
docker run --rm -it -v $(pwd):/app -w /app ubuntu:20.04 bash
# Inside container
apt-get update && apt-get install -y nodejs npm
npm ci
npm test
Use Interactive Debug Sessions
Many CI providers allow interactive debugging sessions:
# GitHub Actions example with tmate for debugging
- name: Setup tmate session
uses: mxschmitt/action-tmate@v3
if: ${{ failure() }}
Implement Failure Snapshots
Capture the state of the environment when failures occur:
# Jenkins example with artifacts
post {
failure {
sh 'tar -czf debug-info.tar.gz logs/ screenshots/ reports/'
archiveArtifacts artifacts: 'debug-info.tar.gz', fingerprint: true
}
}
Use Bisection for Regression Issues
For issues that appeared after certain changes, use bisection to identify the problematic commit:
# Git bisect example
git bisect start
git bisect bad # Current commit is broken
git bisect good v1.0.0 # This version worked
# Git will checkout commits to test
# After testing each commit, mark it:
git bisect good # If this commit works
# or
git bisect bad # If this commit has the issue
# Eventually git will identify the first bad commit
10. Best Practices for Robust CI Pipelines
To build CI pipelines that rarely fail for the wrong reasons, consider these best practices:
Design Principles for Reliable CI
Keep Pipelines Fast
Fast feedback is crucial for developer productivity:
- Split pipelines into stages with the fastest checks first
- Implement test parallelization
- Use incremental builds when possible
- Consider separating slow tests into nightly builds
Make Pipelines Deterministic
Eliminate randomness and ensure consistent results:
- Use fixed seeds for any random processes
- Pin all dependency versions
- Control environment variables explicitly
- Set specific timezones and locales
Build in Observability
Make it easy to understand what’s happening in your pipeline:
- Implement detailed logging
- Add timing information for steps
- Generate visual reports for test results
- Maintain historical metrics on pipeline performance
Implement Progressive Delivery
Reduce risk by implementing progressive validation:
- Start with quick smoke tests
- Follow with more thorough unit and integration tests
- Run full end to end tests only when earlier stages pass
- Consider canary deployments for production changes
Practice Infrastructure as Code
Define your CI infrastructure using code:
- Store pipeline configurations in version control
- Use templates for common patterns
- Implement self service for teams to configure their own pipelines
- Test pipeline changes in isolation before merging
Conclusion
A failing CI pipeline isn’t just an annoyance—it’s valuable feedback that something in your development process needs attention. By addressing the common issues outlined in this guide, you can transform your CI pipeline from a source of frustration into a reliable ally that helps you deliver better software.
Remember that building reliable CI pipelines is an iterative process. Start by addressing the most frequent causes of failure, implement monitoring to identify recurring issues, and continuously refine your approach based on what you learn.
The time invested in improving your CI process will pay dividends through increased developer productivity, higher code quality, and more reliable software delivery. Your future self and your team will thank you for the effort.
By tackling these common CI pipeline issues systematically, you’ll spend less time debugging mysterious failures and more time doing what you do best—building great software.