Why Your Containerization Strategy Is Causing Deployment Issues
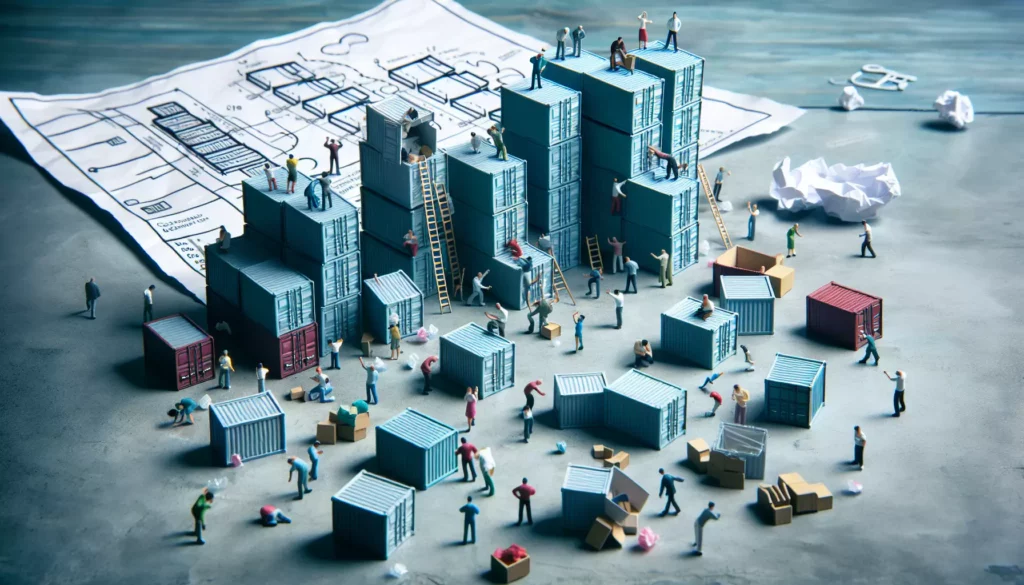
In the fast-paced world of software development, containerization has become a cornerstone technology for deploying applications efficiently. However, many organizations find themselves facing unexpected challenges and deployment issues despite adopting containers. This comprehensive guide explores common containerization strategy pitfalls that may be causing your deployment headaches and provides actionable solutions to overcome them.
Understanding Containerization and Its Promise
Containerization, popularized by Docker and enhanced by orchestration platforms like Kubernetes, promised to solve the age-old problem of “it works on my machine” by packaging applications and their dependencies into isolated, portable units. The theoretical benefits are compelling:
- Consistent environments across development, testing, and production
- Faster deployment cycles
- Improved resource utilization
- Simplified scaling
- Enhanced application isolation
Yet, the reality for many teams is quite different. Let’s examine why your containerization strategy might be causing more problems than it solves.
Common Containerization Strategy Issues
1. Misunderstanding Container Purpose and Scope
One of the most fundamental issues occurs when teams misunderstand what containers are meant to do. Containers are not lightweight virtual machines; they’re application runtime environments.
Problem: Many developers treat containers like VMs, packing multiple services, databases, and processes into a single container. This anti-pattern negates many containerization benefits.
Solution: Follow the “one process per container” principle. Design your containers to run a single application or service, making them more maintainable, scalable, and aligned with microservices architecture principles.
// Bad practice
FROM ubuntu:20.04
RUN apt-get update && apt-get install -y nginx mysql-server redis
COPY . /app
CMD ["bash", "start-everything.sh"]
// Better practice
// In separate Dockerfiles for each service
FROM nginx:alpine
COPY ./static-files /usr/share/nginx/html
EXPOSE 80
CMD ["nginx", "-g", "daemon off;"]
2. Inefficient Image Building Practices
Container images are the foundation of your deployment strategy, but inefficient building practices can lead to bloated, insecure, and slow-to-deploy containers.
Problem: Large image sizes due to unnecessary files, improper layer caching, and using full OS images when minimal versions would suffice.
Solution: Implement these best practices:
- Use multi-stage builds to separate build environments from runtime environments
- Leverage proper layer caching by ordering Dockerfile instructions strategically
- Choose slim or alpine base images when possible
- Implement proper .dockerignore files
// Before: Single-stage build with unnecessary dependencies
FROM node:14
WORKDIR /app
COPY . .
RUN npm install
CMD ["npm", "start"]
// After: Multi-stage build with minimal final image
FROM node:14 AS builder
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
FROM node:14-alpine
WORKDIR /app
COPY --from=builder /app/dist ./dist
COPY --from=builder /app/node_modules ./node_modules
COPY package*.json ./
EXPOSE 3000
CMD ["npm", "start"]
3. Security Vulnerabilities in Container Images
Containers can introduce significant security risks when not properly configured and maintained.
Problem: Running containers as root, not scanning for vulnerabilities, and using outdated base images with known security issues.
Solution: Implement a robust container security strategy:
- Run containers with non-root users
- Regularly scan images for vulnerabilities using tools like Trivy, Clair, or Snyk
- Implement immutable infrastructure practices
- Keep base images updated
// Adding a non-root user to your Dockerfile
FROM python:3.9-slim
RUN apt-get update && apt-get install -y --no-install-recommends \
package1 package2 && \
apt-get clean && \
rm -rf /var/lib/apt/lists/*
# Create non-root user
RUN groupadd -r appuser && useradd -r -g appuser appuser
WORKDIR /app
COPY --chown=appuser:appuser . .
# Switch to non-root user
USER appuser
CMD ["python", "app.py"]
4. Inadequate Resource Management
Containers need proper resource constraints to coexist efficiently on host systems.
Problem: Not setting resource limits leads to containers competing for resources, causing unpredictable performance and potential outages.
Solution: Set appropriate CPU, memory, and I/O limits for your containers based on their actual needs:
// Kubernetes example with resource limits
apiVersion: v1
kind: Pod
metadata:
name: frontend
spec:
containers:
- name: app
image: myapp:1.0
resources:
requests:
memory: "64Mi"
cpu: "250m"
limits:
memory: "128Mi"
cpu: "500m"
For Docker Compose:
version: '3'
services:
webapp:
image: myapp:latest
deploy:
resources:
limits:
cpus: '0.5'
memory: 128M
reservations:
cpus: '0.25'
memory: 64M
5. Configuration Management Challenges
Managing configuration across different environments remains a significant challenge in containerized deployments.
Problem: Hardcoded configurations, secrets in images, and environment-specific settings causing deployment failures.
Solution: Implement a robust configuration management strategy:
- Use environment variables for runtime configuration
- Leverage config maps and secrets in Kubernetes
- Implement service discovery mechanisms
- Consider GitOps approaches for configuration management
// Using environment variables in Dockerfile
FROM node:14-alpine
WORKDIR /app
COPY . .
RUN npm install
# Default values that can be overridden at runtime
ENV NODE_ENV=production
ENV SERVER_PORT=3000
ENV DB_HOST=localhost
EXPOSE $SERVER_PORT
CMD ["npm", "start"]
6. Networking Complexity
Container networking introduces layers of complexity that can lead to connectivity issues and security vulnerabilities.
Problem: Misconfigured networks, port conflicts, and service discovery issues causing intermittent connection failures.
Solution: Develop a clear networking strategy:
- Understand container networking modes (bridge, host, overlay)
- Implement proper service discovery
- Use network policies to control traffic flow
- Consider a service mesh for complex microservices architectures
// Kubernetes network policy example
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: api-allow
spec:
podSelector:
matchLabels:
app: api
ingress:
- from:
- podSelector:
matchLabels:
app: frontend
ports:
- protocol: TCP
port: 8080
7. Orchestration Complexity
While container orchestration platforms like Kubernetes solve many deployment challenges, they introduce significant complexity.
Problem: Over-engineering container orchestration, choosing complex solutions for simple problems, and lack of expertise leading to misconfigurations.
Solution: Right-size your orchestration strategy:
- Evaluate if you actually need Kubernetes or if simpler solutions like Docker Compose or AWS ECS might suffice
- Start with managed Kubernetes services rather than building from scratch
- Invest in training and tooling to reduce complexity
- Consider platform engineering approaches to abstract complexity from developers
8. Persistent Storage Challenges
Containers are ephemeral by design, which creates challenges for applications that require persistent data.
Problem: Data loss during container restarts, performance issues with mounted volumes, and stateful applications struggling in containerized environments.
Solution: Implement proper storage strategies:
- Use volume mounts for persistent data
- Leverage cloud-native storage solutions
- Consider StatefulSets in Kubernetes for stateful applications
- Implement proper backup and recovery procedures
// Kubernetes StatefulSet example for a database
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: postgres
spec:
serviceName: "postgres"
replicas: 1
selector:
matchLabels:
app: postgres
template:
metadata:
labels:
app: postgres
spec:
containers:
- name: postgres
image: postgres:13
ports:
- containerPort: 5432
volumeMounts:
- name: postgres-data
mountPath: /var/lib/postgresql/data
volumeClaimTemplates:
- metadata:
name: postgres-data
spec:
accessModes: ["ReadWriteOnce"]
resources:
requests:
storage: 10Gi
9. Monitoring and Observability Gaps
Traditional monitoring approaches often fall short in containerized environments due to their dynamic and ephemeral nature.
Problem: Lack of visibility into container health, performance, and issues, making troubleshooting difficult.
Solution: Implement container-aware monitoring and observability:
- Use tools designed for container monitoring (Prometheus, Grafana, Datadog)
- Implement distributed tracing (Jaeger, Zipkin)
- Centralize log management
- Create meaningful health checks and readiness probes
// Kubernetes liveness and readiness probes
apiVersion: v1
kind: Pod
metadata:
name: web-app
spec:
containers:
- name: web-app
image: myapp:1.0
ports:
- containerPort: 8080
livenessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 15
periodSeconds: 10
readinessProbe:
httpGet:
path: /ready
port: 8080
initialDelaySeconds: 5
periodSeconds: 5
10. CI/CD Pipeline Integration Issues
Containerization requires rethinking your CI/CD pipelines to fully realize the benefits of container-based deployments.
Problem: Inefficient build processes, manual interventions, and lack of automated testing leading to deployment failures.
Solution: Modernize your CI/CD pipeline for containers:
- Automate image building and testing
- Implement container registry scanning
- Use infrastructure as code for deployment definitions
- Implement progressive delivery patterns (blue/green, canary)
// GitHub Actions workflow example for containerized app
name: Build and Deploy
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Build and test
run: |
docker build -t myapp:${{ github.sha }} .
docker run myapp:${{ github.sha }} npm test
- name: Scan for vulnerabilities
uses: aquasecurity/trivy-action@master
with:
image-ref: 'myapp:${{ github.sha }}'
format: 'table'
exit-code: '1'
severity: 'CRITICAL,HIGH'
- name: Push to registry
uses: docker/build-push-action@v2
with:
push: true
tags: myregistry/myapp:${{ github.sha }},myregistry/myapp:latest
Developing a Robust Containerization Strategy
Now that we’ve identified common issues, let’s explore how to develop a more effective containerization strategy.
Start with Clear Objectives
Before diving into containerization, clearly define what you hope to achieve:
- Are you primarily focused on development environment consistency?
- Is deployment speed your main concern?
- Are you implementing microservices architecture?
- Do you need to improve resource utilization?
Different objectives may lead to different containerization approaches.
Right-Size Your Container Strategy
Not every application needs Kubernetes. Consider these options based on complexity:
- Simple applications: Docker Compose or single-node solutions
- Medium complexity: Managed container services (AWS ECS, Azure Container Instances)
- Complex microservices: Kubernetes (preferably managed like EKS, AKS, or GKE)
Invest in Developer Experience
Containers should make developers’ lives easier, not harder:
- Provide standardized development environments with Docker Compose
- Create reusable container templates and base images
- Implement local Kubernetes development with tools like Minikube, k3d, or Docker Desktop
- Automate common tasks with scripts and tools
// Example docker-compose.yml for local development
version: '3'
services:
app:
build: .
volumes:
- .:/app
- /app/node_modules
ports:
- "3000:3000"
environment:
- NODE_ENV=development
- DB_HOST=db
depends_on:
- db
db:
image: postgres:13
environment:
- POSTGRES_PASSWORD=devpassword
- POSTGRES_USER=devuser
- POSTGRES_DB=devdb
volumes:
- postgres-data:/var/lib/postgresql/data
ports:
- "5432:5432"
volumes:
postgres-data:
Implement Container Governance
As your container usage grows, governance becomes crucial:
- Establish image building standards and best practices
- Implement automated security scanning
- Create policies for resource allocation
- Define lifecycle management procedures for containers and images
Build Container Expertise Incrementally
Container technologies have a steep learning curve. Plan for gradual adoption:
- Start with containerizing non-critical applications
- Build expertise through training and hands-on experience
- Document learnings and best practices
- Create internal champions and communities of practice
Case Study: Refactoring a Problematic Containerization Strategy
Let’s examine a hypothetical case study of a company that encountered deployment issues after adopting containers and how they resolved them.
The Initial Approach
A mid-sized software company decided to containerize their monolithic Java application to improve deployment efficiency. Their initial approach included:
- A single large container containing the entire application
- Running the container as root
- No resource limits defined
- Configuration hardcoded in the image
- Direct deployment to production after local testing
The Problems
After containerization, they experienced:
- Longer deployment times due to large image sizes
- Production outages caused by resource contention
- Security vulnerabilities identified during an audit
- Configuration drift between environments
- Difficulty troubleshooting issues in production
The Solution
The company implemented the following changes:
- Breaking down the monolith: They separated the application into smaller, purpose-specific containers.
- Optimizing images: They implemented multi-stage builds and Alpine-based images to reduce size.
- Security improvements: They configured non-root users, implemented vulnerability scanning, and removed sensitive data from images.
- Resource management: They added appropriate CPU and memory limits based on load testing.
- Configuration management: They externalized configuration using environment variables and config maps.
- CI/CD pipeline: They automated the build, test, and deployment process with proper staging environments.
- Monitoring: They implemented Prometheus for metrics, ELK stack for logs, and proper health checks.
The Results
After these changes, the company experienced:
- 50% reduction in deployment time
- 90% fewer production incidents
- Improved resource utilization
- Better visibility into application performance
- More confidence in the deployment process
Advanced Containerization Strategies
Once you’ve resolved the basic issues, consider these advanced strategies to further improve your containerization approach:
Service Mesh Implementation
For complex microservices architectures, a service mesh like Istio or Linkerd can help manage service-to-service communication, providing:
- Traffic management
- Security with mutual TLS
- Observability
- Policy enforcement
GitOps for Container Deployments
GitOps brings the Git workflow to Kubernetes deployments:
- Infrastructure and deployment configurations are stored in Git
- Changes trigger automatic deployments
- System state is continuously reconciled with the desired state
- Tools like ArgoCD or Flux can implement this pattern
Zero-Trust Security Model
Implement a zero-trust approach to container security:
- Default deny network policies
- Mutual TLS for all service communication
- Runtime security monitoring
- Least privilege access controls
// Kubernetes default deny network policy
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: default-deny-all
spec:
podSelector: {}
policyTypes:
- Ingress
- Egress
Progressive Delivery Patterns
Implement advanced deployment strategies:
- Blue/Green deployments: Run two identical environments and switch traffic
- Canary releases: Gradually route traffic to new versions
- Feature flags: Control feature availability independent of deployment
Containerization Best Practices Checklist
Use this checklist to evaluate and improve your containerization strategy:
Container Design
- One service/process per container
- Proper base image selection (minimal, secure)
- Efficient layer caching in Dockerfiles
- Non-root user configuration
- Proper health checks implemented
Security
- Regular vulnerability scanning
- No secrets in images
- Proper network policies
- Image signing and verification
- Limited container capabilities
Configuration Management
- Externalized configuration
- Environment-specific settings managed properly
- Secrets management solution
- Service discovery mechanism
Resource Management
- CPU and memory limits defined
- Resource requests specified
- Proper storage configuration
- Horizontal scaling configured
Observability
- Centralized logging
- Container-aware monitoring
- Distributed tracing
- Alerting configured
CI/CD Integration
- Automated image building
- Automated testing in containers
- Security scanning in pipeline
- Deployment automation
Tools to Improve Your Containerization Strategy
Consider these tools to address specific containerization challenges:
Container Building and Optimization
- BuildKit: Advanced Docker image building
- Buildpacks: Automated container image building without Dockerfiles
- Dive: Analyze Docker image layers
- Docker Slim: Automatically optimize and secure Docker containers
Security
- Trivy: Container vulnerability scanner
- Clair: Static analysis of vulnerabilities in containers
- Anchore: Deep container inspection and policy enforcement
- Falco: Runtime security monitoring
Orchestration and Management
- Helm: Kubernetes package manager
- Kustomize: Kubernetes configuration customization
- Lens: Kubernetes IDE for simplified management
- Rancher: Multi-cluster Kubernetes management
Observability
- Prometheus: Metrics collection and alerting
- Grafana: Metrics visualization
- Jaeger: Distributed tracing
- Loki: Log aggregation system
CI/CD
- ArgoCD: GitOps continuous delivery tool
- Tekton: Kubernetes-native CI/CD
- Skaffold: Local Kubernetes development
- Kaniko: Building container images in Kubernetes
Conclusion: Building a Sustainable Containerization Strategy
Containerization offers tremendous benefits for application deployment and management, but only when implemented strategically. The issues discussed in this article represent common pitfalls that organizations encounter when adopting containers.
To build a sustainable containerization strategy:
- Start small: Begin with simple applications and gradually increase complexity
- Focus on fundamentals: Ensure proper container design, security, and configuration management
- Invest in education: Build container expertise throughout your team
- Iterate and improve: Regularly review and refine your approach based on lessons learned
- Balance complexity: Only add complexity when it provides clear benefits
By addressing the issues outlined in this article and implementing the recommended solutions, you can transform your containerization strategy from a source of deployment problems into a competitive advantage that delivers on the promise of faster, more reliable software delivery.
Remember that containerization is not the goal itself but rather a means to achieve better software delivery outcomes. Keep your focus on those outcomes, and let that guide your containerization journey.