Why Your Computer Science Degree Isn’t Enough to Pass Tech Interviews
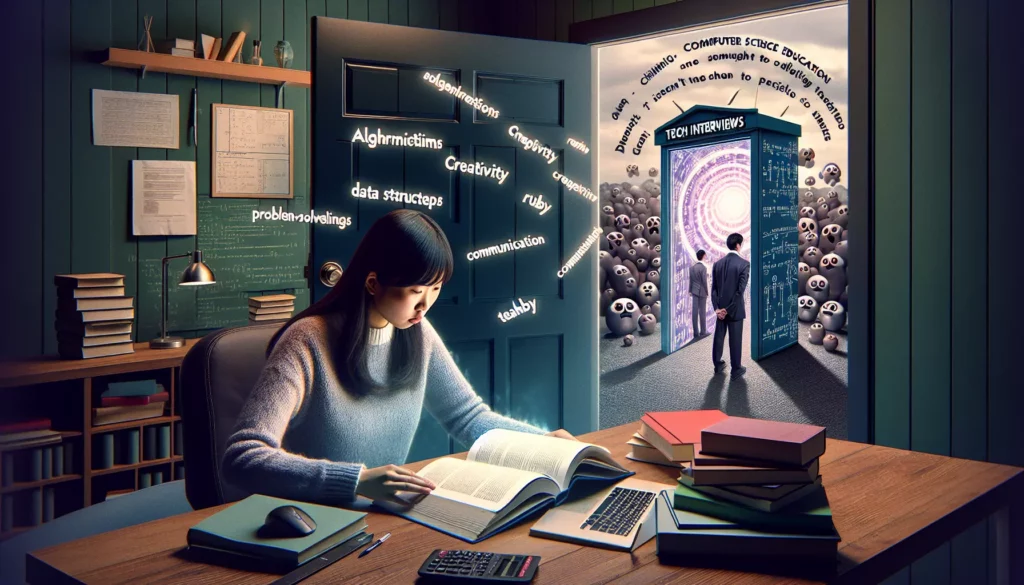
You’ve spent four years (or more) earning your Computer Science degree. You’ve aced algorithms, mastered data structures, and can recite the time complexity of quicksort in your sleep. Now it’s time to land that dream job at a top tech company. You send out applications, and finally, the email arrives: “We’d like to invite you for a technical interview.”
Confidence surges through you. After all, you have a CS degree from a reputable university. You know your stuff, right?
Then reality hits. The technical interview questions bear little resemblance to your coursework. The pressure is intense. Your mind goes blank when asked to solve a seemingly simple problem on a whiteboard or a shared coding environment. You struggle, fumble, and leave the interview doubting everything you thought you knew.
If this scenario sounds familiar, you’re not alone. Many CS graduates discover that their degree, while valuable, hasn’t adequately prepared them for the unique challenges of technical interviews. Let’s explore why this gap exists and what you can do to bridge it.
The Disconnect Between Academia and Industry Interviews
Computer Science education is designed to provide a broad theoretical foundation. It teaches you how computers work, the principles of programming languages, and the mathematical concepts that underpin computing. These are all valuable, but technical interviews at top companies often require a different set of skills.
Theoretical vs. Practical Focus
In university, you might spend weeks learning about compiler design or the mathematical proofs behind certain algorithms. While fascinating and important, these topics rarely come up directly in coding interviews. Instead, interviews focus on practical problem-solving using data structures and algorithms.
Dr. Sarah Thompson, who transitioned from academia to becoming a technical interviewer at a major tech company, explains: “In university, we teach students to understand the ‘why’ behind computing concepts. In interviews, companies want to see if candidates can apply these concepts to solve novel problems under pressure. It’s the difference between knowing how quicksort works theoretically versus being able to implement a sorting solution for a specific problem on the spot.”
Different Evaluation Metrics
In school, you’re evaluated on assignments completed over days or weeks, and exams that test your knowledge recall. In technical interviews, you’re judged on:
- How quickly you can understand a new problem
- Your thought process as you work through solutions
- Your ability to write working code in real-time
- How you handle hints and feedback
- Your communication skills while solving problems
These are skills that many CS programs simply don’t emphasize or evaluate.
What Tech Interviews Actually Test
To understand the gap better, let’s examine what technical interviews at companies like Google, Amazon, and Facebook actually assess.
Problem-Solving Under Pressure
Technical interviews deliberately create a high-pressure environment. You’re solving problems while someone watches and evaluates you. This pressure-cooker situation tests not just your technical knowledge, but your ability to perform under stress.
Mark Johnson, a senior engineer who conducts interviews at a FAANG company, notes: “We’re not just looking for candidates who know algorithms. We want people who can stay calm, think clearly, and communicate effectively even when they’re struggling. That’s what the job actually requires.”
Pattern Recognition
Many interview questions require you to recognize patterns and apply the appropriate algorithmic approach. This pattern recognition is a skill developed through practice with many different problems, not just understanding the theory.
For example, when presented with a problem about finding the shortest path in a network, you need to quickly recognize that this is a graph problem that might require Dijkstra’s algorithm or breadth-first search. This pattern matching happens almost instantaneously for experienced engineers but can be challenging for new graduates who haven’t developed this mental catalog.
Code Fluency
Universities often allow students to write code at their own pace, with access to documentation, Stack Overflow, and other resources. Technical interviews require you to write clean, working code quickly without these aids.
This fluency comes from writing code regularly and solving problems from scratch, not from understanding theoretical concepts or completing guided assignments.
Communication Skills
Perhaps surprisingly to many CS graduates, technical interviews place enormous emphasis on how you communicate your thought process. Can you clearly articulate your approach? Do you ask clarifying questions? Can you explain trade-offs between different solutions?
Emily Chen, a technical recruiter with over a decade of experience, explains: “I’ve seen brilliant programmers fail interviews because they couldn’t explain their thinking. Communication isn’t an afterthought in these roles; it’s central to working effectively on engineering teams.”
Common Interview Topics That CS Programs Underemphasize
While CS programs cover most of the theoretical knowledge needed for interviews, certain topics often receive insufficient practical attention:
Dynamic Programming
Dynamic programming is a technique for solving complex problems by breaking them down into simpler subproblems. While many CS curricula cover the concept, students rarely get enough practice implementing dynamic programming solutions to develop the pattern recognition needed in interviews.
Consider this classic dynamic programming problem that frequently appears in interviews:
Given a set of items with weights and values, determine the maximum value you can carry in a knapsack of capacity W.
Input:
values[] = {60, 100, 120}
weights[] = {10, 20, 30}
W = 50
Output: 220
While you might understand the principle of the 0/1 knapsack problem from your algorithms class, implementing it efficiently under pressure is another matter entirely.
Graph Algorithms
Graph problems appear frequently in interviews, but many CS graduates haven’t had enough practice implementing depth-first search, breadth-first search, or more complex algorithms like Dijkstra’s or A* from scratch.
Here’s a typical graph problem you might face:
Given a 2D grid of 1s (land) and 0s (water), count the number of islands.
An island is surrounded by water and is formed by connecting adjacent lands horizontally or vertically.
Example:
Input:
[
[1,1,0,0,0],
[1,1,0,0,0],
[0,0,1,0,0],
[0,0,0,1,1]
]
Output: 3
Recognizing this as a connected components problem and implementing a clean DFS or BFS solution requires practice that goes beyond most course assignments.
System Design
For more senior roles, system design questions evaluate your ability to architect scalable systems. Few undergraduate programs adequately prepare students for questions like “Design Twitter” or “Build a distributed cache.”
These questions require understanding distributed systems, database scaling, caching strategies, and microservice architecture at a practical level, not just theoretically.
Optimization Techniques
While big-O analysis is covered in most algorithms courses, the practical skill of optimizing code is often underdeveloped. Interviewers want to see if you can take a working solution and make it more efficient in terms of time or space complexity.
Why Even Top Students Struggle
Even students who graduate with high GPAs from prestigious CS programs often find themselves unprepared for technical interviews. Here’s why:
Different Success Metrics
Academic success is measured by understanding concepts and completing assignments correctly, often with generous timeframes. Interview success requires rapid problem-solving, coding fluency, and performance under pressure.
Dr. Michael Roberts, a computer science professor who also consults for tech companies, observes: “Our highest-achieving students often excel at deeply understanding concepts and writing thorough, well-documented code. But interviews reward speed and adaptability more than thoroughness. It’s a different skill set.”
Limited Exposure to Interview-Style Problems
Most coursework problems are designed to teach specific concepts, not to test problem-solving ability in the way interviews do. Interview questions are intentionally tricky and often combine multiple concepts in unexpected ways.
Lack of Practice Under Pressure
Few students regularly practice solving problems while someone watches and evaluates them. This performance aspect of interviews can be particularly challenging for those who haven’t experienced it before.
Real-World Example: The Palindrome Substrings Problem
Consider this interview question:
Given a string, find the number of palindromic substrings in it.
Example:
Input: "abc"
Output: 3
Explanation: Three palindromic strings: "a", "b", "c"
Input: "aaa"
Output: 6
Explanation: Six palindromic strings: "a", "a", "a", "aa", "aa", "aaa"
A student might recognize this as a dynamic programming problem but struggle to implement the solution cleanly under pressure:
def countSubstrings(s):
n = len(s)
dp = [[False for _ in range(n)] for _ in range(n)]
count = 0
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
count += 1
# Check for substrings of length 2
for i in range(n-1):
if s[i] == s[i+1]:
dp[i][i+1] = True
count += 1
# Check for substrings of length 3 or more
for length in range(3, n+1):
for i in range(n-length+1):
j = i + length - 1
if s[i] == s[j] and dp[i+1][j-1]:
dp[i][j] = True
count += 1
return count
The pressure of the interview setting makes it difficult to reason through this solution, especially if you haven’t practiced similar problems before.
How to Bridge the Gap
If your CS degree isn’t enough to ace technical interviews, what can you do to prepare? Here are effective strategies to bridge the gap:
Develop a Structured Practice Routine
Consistent practice is the single most important factor in interview preparation. Develop a routine that includes:
- Daily problem-solving: Aim to solve at least one or two new problems each day
- Topic-based practice: Focus on specific areas (arrays, strings, trees, graphs, etc.) for a week at a time
- Mixed review sessions: Regularly practice problems from different topics to build pattern recognition
Jason Zhang, who received offers from three FAANG companies after initially struggling with interviews, shares: “The turning point for me was committing to daily practice. I solved 200 problems in the three months before my interviews. The patterns started to become second nature.”
Use Interview-Focused Resources
Several resources are specifically designed to prepare you for technical interviews:
- Platforms like AlgoCademy: Provides guided practice with interview-style questions and AI-powered assistance
- LeetCode, HackerRank, and similar sites: Offer large collections of interview problems with test cases
- Interview preparation books: “Cracking the Coding Interview” and “Elements of Programming Interviews” are classics for a reason
- Algorithm visualization tools: Help build intuition for how algorithms work
Practice Under Interview Conditions
To build comfort with the pressure of real interviews:
- Use a timer when solving problems
- Practice writing code on a whiteboard or in a simple text editor without auto-completion
- Explain your thought process out loud as you solve problems
- Find a practice partner for mock interviews
Sarah Kim, now a software engineer at Google, recalls: “Mock interviews were crucial for me. Having someone watch me code was uncomfortable at first, but it helped me get used to the pressure. By my fifth or sixth mock interview, I was much more comfortable thinking out loud.”
Focus on Understanding, Not Just Memorization
While it’s tempting to memorize solutions to common problems, understanding the underlying patterns is more valuable:
- After solving a problem, study alternative solutions and understand their trade-offs
- Group similar problems to recognize patterns
- Implement the same algorithm multiple times from scratch to build fluency
Alex Rivera, who coaches software engineers for interviews, emphasizes: “Don’t just check off problems as ‘solved.’ Ask yourself: Could I solve this again from scratch tomorrow? Do I understand why this approach works better than alternatives? That deeper understanding is what translates to interview success.”
Build Your Problem-Solving Framework
Develop a systematic approach to tackling interview problems:
- Clarify the problem: Ask questions to ensure you understand requirements and constraints
- Work through examples: Use simple cases to understand the problem better
- Identify a brute force solution: Start with the simplest approach that works
- Optimize: Consider how to improve time and space complexity
- Test your solution: Walk through your code with examples and edge cases
Having this framework helps you approach any problem methodically, even when nervous or under pressure.
The Skills Gap by Interview Topic
Let’s examine specific areas where CS graduates often need additional preparation:
Data Structures and Algorithms
What most CS programs cover: Theoretical understanding of data structures and algorithms, big-O analysis, basic implementation exercises.
What interviews require: Ability to quickly identify which data structure or algorithm fits a problem, implement complex algorithms from scratch, optimize solutions for edge cases.
How to bridge the gap: Practice implementing data structures from scratch. Solve problems that require combining multiple data structures. Focus on pattern recognition across similar problems.
Example: Using the Right Data Structure
Consider this interview question:
Design a data structure that supports the following operations in O(1) time:
- insert(val): Inserts an item val to the collection.
- remove(val): Removes an item val from the collection if present.
- getRandom(): Returns a random element from the current collection of elements.
The optimal solution uses a combination of a hash map and an array:
class RandomizedSet:
def __init__(self):
self.dict = {} # val -> index
self.list = [] # list of values
def insert(self, val):
if val in self.dict:
return False
self.dict[val] = len(self.list)
self.list.append(val)
return True
def remove(self, val):
if val not in self.dict:
return False
# Move the last element to the place of the element to delete
last_element = self.list[-1]
idx = self.dict[val]
self.list[idx] = last_element
self.dict[last_element] = idx
# Remove the last element
self.list.pop()
del self.dict[val]
return True
def getRandom(self):
import random
return random.choice(self.list)
This solution requires not just knowing data structures, but understanding their properties deeply enough to combine them creatively.
System Design
What most CS programs cover: Basic database concepts, networking fundamentals, some distributed systems theory.
What interviews require: Ability to design scalable, reliable systems that address real-world constraints; knowledge of modern architectural patterns; understanding of trade-offs between different approaches.
How to bridge the gap: Study system design resources specifically. Build distributed systems projects. Read engineering blogs from major tech companies about their architecture.
Example: Designing a URL Shortener
A common system design question asks candidates to design a URL shortening service like bit.ly. This requires considering:
- How to generate unique short URLs
- Database design for storing URL mappings
- Caching strategies for popular URLs
- Handling high read/write traffic
- Analytics and monitoring
Few CS programs prepare students to think through all these aspects comprehensively.
Coding Implementation
What most CS programs cover: Programming fundamentals, syntax, basic debugging.
What interviews require: Writing clean, error-free code quickly without IDE support; handling edge cases; optimizing for readability.
How to bridge the gap: Practice coding without IDE features. Implement solutions on a whiteboard or simple text editor. Have others review your code for style and clarity.
Example: String Manipulation Gotchas
Consider this seemingly simple problem:
Write a function to check if a string is a palindrome, considering only alphanumeric characters and ignoring case.
Example:
"A man, a plan, a canal: Panama" should return true.
A clean implementation requires careful handling of edge cases:
def isPalindrome(s):
# Convert to lowercase and filter out non-alphanumeric characters
filtered_chars = [c.lower() for c in s if c.isalnum()]
# Check if the string is equal to its reverse
return filtered_chars == filtered_chars[::-1]
Writing this without syntax errors or logical bugs under pressure is harder than it looks, especially without IDE support.
The Role of Soft Skills in Technical Interviews
Technical knowledge alone isn’t enough. Soft skills play a crucial role in interview success:
Communication
Interviewers evaluate how clearly you can explain your thought process. Practice verbalizing your approach as you solve problems. Explain why you’re choosing certain data structures or algorithms.
Maria Gonzalez, an engineering manager who conducts interviews, explains: “I’ve seen candidates with perfect technical solutions fail because they couldn’t explain their reasoning. Clear communication is as important as the solution itself.”
Handling Feedback
Interviewers often provide hints or suggest alternative approaches. How you respond to this feedback is critical. Show that you can incorporate suggestions without becoming defensive.
Asking Clarifying Questions
Don’t rush to code. Take time to fully understand the problem by asking thoughtful questions about requirements, constraints, and edge cases. This demonstrates thoroughness and attention to detail.
Managing Stress
Interviews are inherently stressful. Develop techniques to stay calm under pressure, such as deep breathing or positive self-talk. Remember that some nervousness is normal and even experienced engineers feel it.
Creating Your Interview Preparation Plan
Based on the gaps we’ve identified, here’s a comprehensive preparation plan:
For Recent or Soon-to-Be Graduates
3-6 months before interviews:
- Review core data structures and algorithms
- Start solving 1-2 problems daily on platforms like AlgoCademy or LeetCode
- Join or form a study group for accountability
- Read “Cracking the Coding Interview” or similar resources
1-3 months before interviews:
- Increase practice to 2-3 problems daily
- Begin mock interviews with peers or using platforms that offer them
- Focus on topic areas where you’re weakest
- Practice explaining your solutions out loud
Final month:
- Continue daily practice but focus on reviewing and reinforcing patterns
- Conduct several full-length mock interviews
- Review common behavioral questions
- Prepare questions to ask your interviewers
For Those Already in the Workforce
If you’re a working professional preparing for interviews:
- Allocate consistent time for practice (e.g., 1-2 hours daily)
- Leverage your professional experience in system design discussions
- Focus on modern frameworks and technologies relevant to your target companies
- Network with employees at target companies to understand their interview process
Sample Weekly Schedule
Here’s what an effective weekly preparation schedule might look like:
- Monday: Array and string problems (1-2 hours)
- Tuesday: Linked list and tree problems (1-2 hours)
- Wednesday: Graph algorithms (1-2 hours)
- Thursday: Dynamic programming (1-2 hours)
- Friday: System design or object-oriented design (1-2 hours)
- Saturday: Mock interview with a peer (1-2 hours)
- Sunday: Review and reflect on the week’s progress (1 hour)
Learning from Failure: The Iteration Process
Even with preparation, you may not succeed in your first interviews. This is normal and part of the learning process.
Turning Rejection into Growth
After each interview, regardless of outcome:
- Write down the questions you were asked
- Note areas where you struggled
- Solve the problems you couldn’t solve during the interview
- Research better approaches to the problems you did solve
David Park, who interviewed at seven companies before landing his dream job, shares: “Each rejection taught me something valuable. I kept a journal of questions and my mistakes. By my final interviews, I had addressed most of my weaknesses and felt much more confident.”
Recognizing Patterns in Feedback
Look for patterns in the feedback you receive:
- Are you consistently struggling with a particular type of problem?
- Do interviewers mention the same areas for improvement?
- Are you running out of time on certain questions?
Use these patterns to focus your preparation more effectively.
Beyond Technical Preparation: The Full Interview Picture
While this article focuses on the technical aspects of interviews, remember that companies evaluate candidates holistically:
Company Research
Understanding a company’s products, culture, and values can help you tailor your responses and ask insightful questions. This demonstrates genuine interest and helps you determine if the company is a good fit for you.
Behavioral Questions
Prepare stories about your projects, challenges you’ve overcome, and times you’ve demonstrated leadership or teamwork. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
Asking Thoughtful Questions
The questions you ask interviewers reveal your priorities and how you think. Prepare questions about the team’s challenges, engineering culture, and growth opportunities.
Conclusion: Bridging the Gap
Your Computer Science degree provides a valuable foundation, but technical interviews require additional skills and preparation. The gap between academic learning and interview success is real, but it can be bridged with dedicated practice and the right approach.
Remember that technical interviews are a skill in themselves—one that improves with practice, feedback, and persistence. By understanding what interviews actually test and creating a structured preparation plan, you can transform from a knowledgeable CS graduate to a confident interview performer.
The journey from classroom to career may include some interview disappointments, but each experience brings you closer to success. Be patient with yourself, celebrate your progress, and keep pushing forward. Your CS degree got you started—now it’s time to build the specific skills that will get you hired.
As you prepare, remember that the goal isn’t just to pass interviews but to become the kind of problem-solver who thrives in real engineering environments. The skills you develop during interview preparation—quick thinking, clear communication, and elegant problem-solving—will serve you throughout your career.
Your CS degree isn’t enough on its own, but combined with targeted practice and perseverance, it creates the foundation for a successful tech career. The gap exists, but you have everything you need to bridge it.