Why Your Coding Test Solutions Aren’t Meeting Requirements
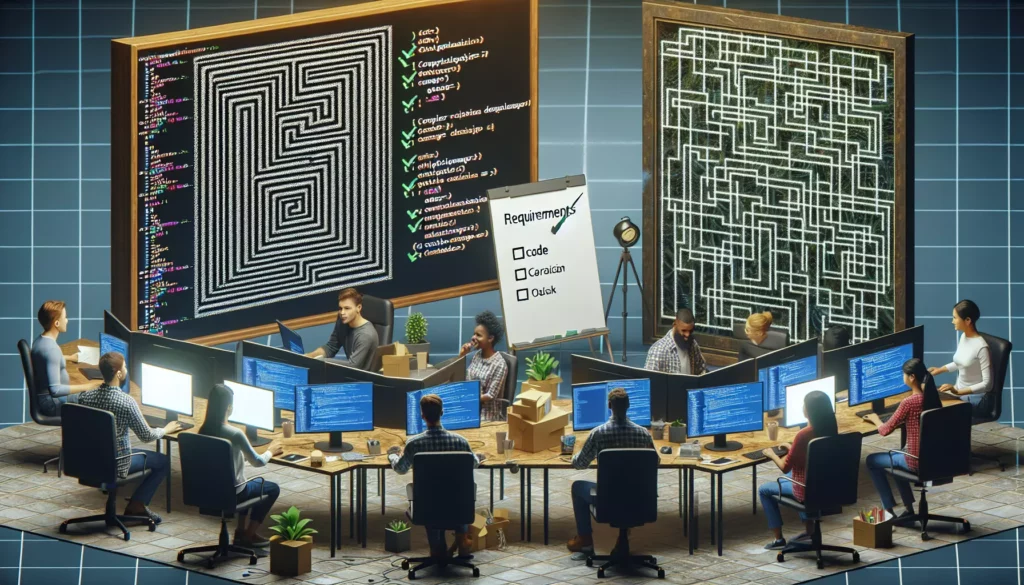
Coding tests are a fundamental part of the technical interview process, especially for roles at major tech companies. Yet many developers find that despite their technical knowledge, their solutions frequently fail to meet the requirements set by interviewers or automated testing systems. This disconnect can be frustrating and career-limiting, but it’s often the result of specific patterns and oversights that can be addressed with the right approach.
In this comprehensive guide, we’ll explore the common reasons why your coding test solutions might not be meeting requirements, and provide actionable strategies to help you bridge this gap. Whether you’re preparing for interviews at FAANG companies or working to improve your algorithmic problem-solving skills, understanding these principles can significantly improve your success rate.
Table of Contents
- Understanding the Requirements: The Foundation of Success
- Common Mistakes That Lead to Requirement Failures
- Failing to Handle Edge Cases
- Efficiency Concerns: When Correct Isn’t Good Enough
- Code Organization and Readability
- The Test-Driven Approach to Meeting Requirements
- Communication: The Overlooked Skill in Coding Tests
- Preparation Strategies That Ensure Requirement Compliance
- Learning From Failures: Turning Rejections Into Growth
- Conclusion: The Path Forward
Understanding the Requirements: The Foundation of Success
The first and most critical step in solving any coding problem is thoroughly understanding what’s being asked. This might seem obvious, but misinterpreting requirements is the single most common reason for solution failure.
The Anatomy of a Coding Problem
A well-defined coding problem typically includes:
- Problem statement: The overall task or challenge
- Input specifications: What data your function or program will receive
- Output specifications: What your function or program should return
- Constraints: Limitations on input size, time, memory, etc.
- Examples: Sample inputs and expected outputs
Missing or misunderstanding any of these components can lead to a solution that doesn’t meet requirements.
Active Reading Techniques
To ensure you fully grasp the requirements:
- Read the problem statement multiple times. First for a general understanding, then for details.
- Highlight key information. Identify the input/output formats, constraints, and special cases.
- Restate the problem in your own words. This helps verify your understanding.
- Work through the examples manually. Trace the expected logic step by step.
- Create additional examples. Generate your own test cases to confirm your understanding.
Clarifying Ambiguities
In real interviews, don’t hesitate to ask clarifying questions. In fact, interviewers often intentionally leave ambiguities to test your communication skills and attention to detail.
Questions you might ask include:
- “Can I assume all inputs will be valid?”
- “How should I handle edge cases like empty inputs or extremely large values?”
- “Is there a specific time or space complexity requirement?”
- “Should I prioritize readability or performance in my solution?”
Even when practicing alone, consider what clarifications might be needed and how they would affect your approach.
Common Mistakes That Lead to Requirement Failures
Even with a solid understanding of the requirements, several common mistakes can cause your solutions to fall short.
Rushing to Code
Many developers immediately start coding after reading a problem. This impulse often leads to solutions that miss key requirements or need significant refactoring later.
Instead, take time to:
- Plan your approach. Sketch the algorithm or solution strategy before writing any code.
- Consider alternative approaches. The first solution that comes to mind might not be optimal.
- Identify potential challenges. Think about what aspects of the problem might be tricky to implement.
Misinterpreting Input/Output Formats
A technically correct algorithm can still fail if it doesn’t handle the expected input format or produce the required output format.
Common format mistakes include:
- Not handling input as the correct data type (e.g., treating a string as an integer)
- Returning results in the wrong order or structure
- Ignoring formatting requirements (e.g., rounding, precision, or specific string formats)
- Mishandling delimiters or separators in input/output
Always double-check your function signatures and return values against the requirements.
Overlooking Constraints
Problem constraints aren’t just suggestions—they’re crucial parts of the requirements that often dictate which approaches will work.
Pay special attention to:
- Input size limits. These often hint at the expected time complexity.
- Time constraints. Some platforms specify execution time limits.
- Memory constraints. Your solution might need to be memory-efficient.
- Language-specific constraints. Some problems may restrict certain libraries or functions.
A solution that works for small inputs but times out on larger ones fails to meet the requirements just as much as one that produces incorrect results.
Failing to Handle Edge Cases
Edge cases are the scenarios that occur at the extremes of possible inputs. They’re often overlooked during solution development but frequently cause failures in testing.
Common Edge Cases to Consider
- Empty inputs: Empty arrays, strings, or null/undefined values
- Boundary values: Minimum or maximum possible inputs
- Single-element inputs: When your algorithm expects to process multiple elements
- Duplicate values: When uniqueness might be assumed but not guaranteed
- Negative numbers: Especially in problems that might seem to only involve positive values
- Zero: Often a special case, particularly in division or as a boundary value
- Extremely large values: Which might cause overflow or precision issues
Systematic Edge Case Testing
Develop a systematic approach to identifying and testing edge cases:
- List potential edge cases before starting to code.
- Create specific test cases for each edge scenario.
- Test edge cases individually to isolate their effects.
- Incorporate edge case handling into your main solution.
- Verify edge case behavior after completing your implementation.
Example: Edge Case Analysis
Consider a function that finds the average of an array of numbers:
function calculateAverage(numbers) {
let sum = 0;
for (let num of numbers) {
sum += num;
}
return sum / numbers.length;
}
This function fails on several edge cases:
- Empty array: Causes division by zero
- Array with extremely large numbers: Might cause overflow
- Array with decimal values: Might cause precision issues
A more robust implementation would be:
function calculateAverage(numbers) {
// Handle empty array
if (!numbers || numbers.length === 0) {
return 0; // Or throw an error, depending on requirements
}
let sum = 0;
for (let num of numbers) {
sum += num;
}
return sum / numbers.length;
}
Efficiency Concerns: When Correct Isn’t Good Enough
In many coding tests, especially for competitive positions, producing the correct output is necessary but not sufficient. Your solution must also be efficient in terms of time and space complexity.
Understanding Time and Space Complexity
Time complexity measures how the execution time of your algorithm grows with the input size. Space complexity measures how much memory your algorithm requires as the input size increases.
Common complexity classes (from most to least efficient):
- O(1): Constant time/space (independent of input size)
- O(log n): Logarithmic (common in binary search, balanced trees)
- O(n): Linear (processing each input element once)
- O(n log n): Linearithmic (common in efficient sorting algorithms)
- O(n²): Quadratic (nested loops over the input)
- O(2^n): Exponential (often seen in naive recursive solutions)
Optimizing Your Solutions
To improve efficiency:
- Analyze your algorithm’s complexity before implementing it.
- Look for redundant operations that can be eliminated.
- Consider using appropriate data structures (hash maps for O(1) lookups, etc.).
- Apply algorithm design patterns like dynamic programming, greedy algorithms, or divide and conquer.
- Trade space for time when appropriate (caching, precomputation).
Recognizing Efficiency Requirements
How to determine the expected efficiency of your solution:
- Check the constraints. Large input sizes (e.g., n ≤ 10^5) usually require at least O(n log n) solutions.
- Look for time limits. Some platforms specify execution time constraints.
- Consider the context. Interview questions for senior roles or competitive programming often have implicit efficiency expectations.
Example: Efficiency Optimization
Consider finding pairs in an array that sum to a target value:
Inefficient approach (O(n²)):
function findPairs(nums, target) {
const result = [];
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
result.push([nums[i], nums[j]]);
}
}
}
return result;
}
Efficient approach (O(n)):
function findPairs(nums, target) {
const result = [];
const seen = new Set();
for (const num of nums) {
const complement = target - num;
if (seen.has(complement)) {
result.push([complement, num]);
}
seen.add(num);
}
return result;
}
Code Organization and Readability
While functional correctness and efficiency are primary concerns, the organization and readability of your code also matter—especially in interview settings where humans evaluate your solution.
Clean Code Principles
- Meaningful variable and function names. Names should clearly indicate purpose.
- Consistent formatting. Maintain uniform indentation, spacing, and naming conventions.
- Appropriate comments. Explain complex logic or non-obvious decisions, but avoid over-commenting.
- Single responsibility principle. Each function should do one thing well.
- DRY (Don’t Repeat Yourself). Extract repeated logic into helper functions.
Structured Problem-Solving
Organize your solution in a logical flow:
- Input validation. Check for invalid inputs and handle edge cases.
- Preprocessing. Prepare data structures or initial values.
- Core algorithm. Implement the main logic of your solution.
- Result formatting. Ensure the output matches the required format.
Modularization
Break complex solutions into smaller, manageable components:
// Instead of one large function
function solveProblem(input) {
// Input validation
if (!isValidInput(input)) {
return null;
}
// Preprocessing
const processedData = preprocessInput(input);
// Core algorithm
const result = computeResult(processedData);
// Result formatting
return formatOutput(result);
}
// Helper functions
function isValidInput(input) { /* ... */ }
function preprocessInput(input) { /* ... */ }
function computeResult(data) { /* ... */ }
function formatOutput(result) { /* ... */ }
Balancing Brevity and Clarity
While concise code is generally good, extreme brevity can reduce readability. Find the right balance:
- Use language features (like array methods) to write compact code when they improve clarity.
- Avoid complex one-liners that sacrifice understandability.
- Consider the context—some interviews may value different aspects of code style.
The Test-Driven Approach to Meeting Requirements
A test-driven approach can significantly improve your ability to meet requirements by forcing you to think about expected behaviors before writing solution code.
The Test-Driven Development Cycle
- Write a test that defines the expected behavior for a specific aspect of the problem.
- Run the test to confirm it fails (since you haven’t implemented the solution yet).
- Write the minimal code needed to pass the test.
- Run the test to confirm it passes.
- Refactor your code while ensuring the test continues to pass.
- Repeat with additional tests for other aspects of the problem.
Creating Comprehensive Test Cases
Develop test cases that cover:
- Basic functionality. Tests for the core requirements with typical inputs.
- Edge cases. Tests for boundary conditions and special inputs.
- Error handling. Tests for invalid inputs and expected error responses.
- Performance scenarios. Tests with large inputs to verify efficiency.
Implementing Tests in Coding Interviews
Even in environments without formal testing frameworks, you can apply test-driven principles:
function solve(arr) {
// Your solution implementation
}
// Test cases
function runTests() {
console.log("Test 1: Basic case");
const result1 = solve([1, 2, 3]);
console.assert(result1 === 6, `Expected 6, got ${result1}`);
console.log("Test 2: Empty array");
const result2 = solve([]);
console.assert(result2 === 0, `Expected 0, got ${result2}`);
console.log("Test 3: Negative numbers");
const result3 = solve([-1, -2, 3]);
console.assert(result3 === 0, `Expected 0, got ${result3}`);
console.log("All tests completed");
}
runTests();
Benefits of Test-Driven Development
- Forces you to understand requirements before coding
- Provides immediate feedback on your implementation
- Helps identify edge cases early
- Creates a safety net for refactoring
- Builds confidence in your solution
Communication: The Overlooked Skill in Coding Tests
In live interview settings, communication is as important as your technical solution. Even in automated assessments, your code communicates your thinking to evaluators who review it later.
Thinking Aloud During Live Interviews
When solving problems in real-time with an interviewer:
- Narrate your understanding of the problem before coding.
- Share your approach and the reasoning behind it.
- Vocalize trade-offs you’re considering between different solutions.
- Explain key decisions as you implement your solution.
- Discuss the complexity and potential optimizations of your approach.
Documenting Your Solution
For take-home assessments or self-documentation:
/**
* Finds the maximum subarray sum using Kadane's algorithm.
*
* Time Complexity: O(n) where n is the length of the input array
* Space Complexity: O(1) as we only use a constant amount of extra space
*
* @param {number[]} nums - The input array of integers
* @return {number} The maximum subarray sum
*/
function maxSubArray(nums) {
// Handle edge case
if (nums.length === 0) return 0;
let currentMax = nums[0];
let globalMax = nums[0];
// Iterate from the second element
for (let i = 1; i < nums.length; i++) {
// Either take the current element alone or add it to the previous subarray
currentMax = Math.max(nums[i], currentMax + nums[i]);
// Update the global maximum if we found a better subarray
globalMax = Math.max(globalMax, currentMax);
}
return globalMax;
}
Asking the Right Questions
Strategic questions demonstrate your thoroughness and problem-solving approach:
- Requirement clarification: “Should I handle invalid inputs? What should the function return in that case?”
- Assumption validation: “I’m assuming the input array is unsorted. Is that correct?”
- Approach confirmation: “I’m thinking of using a hash map to optimize lookups. Does that sound reasonable?”
- Feedback seeking: “I’ve outlined my approach. Do you see any issues or have any suggestions before I start coding?”
Handling Mistakes and Feedback
How you respond to errors or interviewer feedback is crucial:
- Acknowledge mistakes without excessive self-criticism
- Demonstrate your ability to incorporate feedback quickly
- Show resilience when facing challenges
- Use debugging as an opportunity to showcase your problem-solving process
Preparation Strategies That Ensure Requirement Compliance
Consistent preparation with a focus on requirement compliance can dramatically improve your performance in coding tests.
Deliberate Practice Techniques
- Solve problems under timed conditions. This simulates the pressure of real tests.
- Implement full solutions. Don’t just sketch algorithms; write complete, runnable code.
- Test your solutions rigorously. Create comprehensive test cases for each problem.
- Review and refactor. After solving a problem, look for ways to improve your solution.
- Study solution patterns. Familiarize yourself with common algorithmic approaches.
Creating a Systematic Approach
Develop a checklist to ensure you consistently meet requirements:
- Read the problem statement completely (twice)
- Identify input/output formats and constraints
- List potential edge cases
- Determine an initial approach and analyze its complexity
- Consider alternative approaches if needed
- Implement a solution with clear organization
- Test with provided examples
- Test with edge cases
- Optimize if necessary
- Review for correctness and style
Building a Knowledge Base
Create a personal repository of problem-solving patterns:
- Algorithm templates. Standard implementations of common algorithms (BFS, DFS, binary search, etc.)
- Data structure implementations. Custom implementations of trees, graphs, heaps, etc.
- Problem-solving strategies. Approaches for specific types of problems (two-pointer, sliding window, etc.)
- Personal error patterns. Document mistakes you frequently make to avoid repeating them
Mock Interviews and Peer Review
External feedback is invaluable:
- Practice with peers to simulate interview conditions
- Request code reviews from more experienced developers
- Use platforms that provide automated feedback on your solutions
- Review recorded mock interviews to identify improvement areas
Learning From Failures: Turning Rejections Into Growth
Everyone faces rejection in technical interviews. What separates successful candidates is how they learn from these experiences.
Analyzing Failed Solutions
When your solution doesn’t meet requirements:
- Identify the specific failure point. Was it a misunderstood requirement, an overlooked edge case, or an efficiency issue?
- Understand why your approach failed. What assumption or oversight led to the issue?
- Study the correct solution. What approach would have worked, and why?
- Recreate the solution independently. Don’t just read the correct solution; implement it yourself.
Creating a Failure Log
Document your learning systematically:
// Problem: Two Sum
// Date: 2023-05-15
// My Approach: Nested loops (O(n²))
// Why It Failed: Time limit exceeded for large inputs
// Correct Approach: Hash map to store seen values (O(n))
// Key Learning: When looking for pairs/complements, hash maps can often reduce time complexity
// Similar Problems: Three Sum, Four Sum
Developing Pattern Recognition
Over time, you’ll recognize patterns in both problems and your own mistakes:
- Categorize problems by their underlying patterns
- Identify your recurring weaknesses (e.g., off-by-one errors, mishandling edge cases)
- Create targeted practice to address specific weaknesses
- Build a mental library of problem transformations (recognizing when a new problem is similar to one you’ve seen before)
Maintaining Resilience
The psychological aspect of handling rejection is crucial:
- View failures as learning opportunities rather than judgments of your ability
- Recognize that even experienced developers face rejections
- Celebrate small improvements and incremental progress
- Build a support network of peers who understand the process
Conclusion: The Path Forward
Meeting requirements in coding tests is a skill that improves with deliberate practice and systematic approach. By understanding common failure points and implementing the strategies outlined in this guide, you can significantly increase your success rate in technical interviews and coding assessments.
Remember these key principles:
- Understand before coding. Take the time to fully grasp the requirements.
- Plan your approach. Consider multiple solutions and their trade-offs.
- Test comprehensively. Account for edge cases and efficiency requirements.
- Communicate clearly. Express your thinking process through both code and conversation.
- Learn from failure. Use rejections as opportunities to identify and address weaknesses.
The journey to mastering coding interviews is marathon, not a sprint. Each problem you solve, whether successfully or not, contributes to your growth as a developer. By focusing on requirement compliance alongside technical skills, you’ll build the foundation for success in even the most competitive technical interviews.
Remember that the goal isn’t just to pass interviews but to become a better problem solver—a skill that will serve you throughout your career in software development. Keep practicing, stay resilient, and trust in the process of continuous improvement.