Why Your Coding Standards Aren’t Improving Team Output
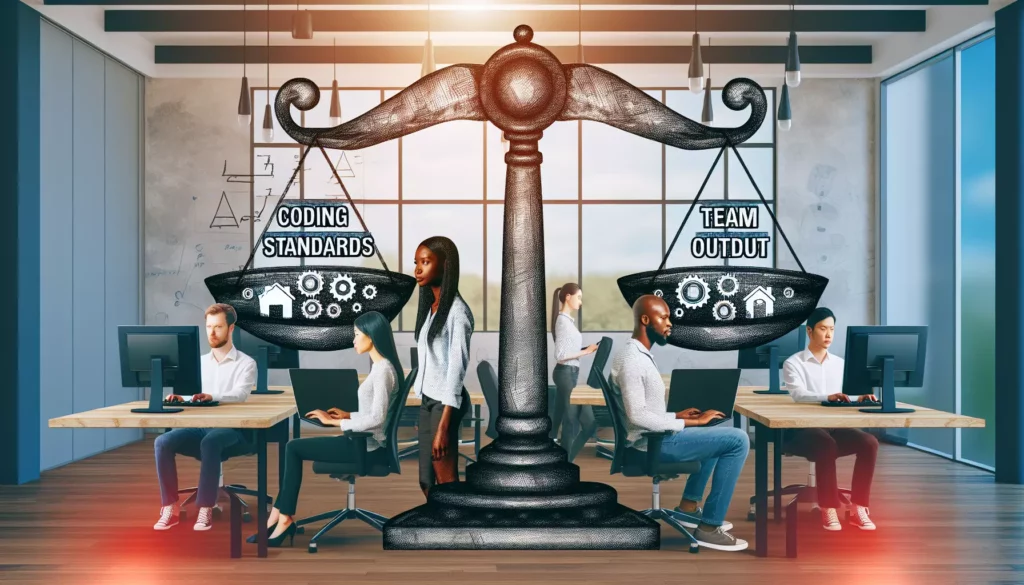
In the fast paced world of software development, teams often implement coding standards with the expectation that they’ll immediately boost productivity, enhance code quality, and streamline collaboration. Yet many organizations find themselves disappointed when these standards fail to deliver the promised results.
If your team has established coding standards but isn’t seeing the expected improvements in output, you’re not alone. This disconnect between expectation and reality is common but fixable.
In this comprehensive guide, we’ll explore why coding standards sometimes fall short, what might be happening within your team dynamics, and practical strategies to transform your standards from mere documentation into powerful drivers of team performance.
Understanding the Gap Between Standards and Results
Before diving into solutions, let’s examine why coding standards often fail to improve team output:
The Implementation Illusion
Many teams believe that simply documenting standards and making them available is sufficient. They create extensive style guides, formatting rules, and best practices documents, then wonder why code quality doesn’t magically improve.
The reality is that having standards and living by standards are entirely different matters. Documentation without implementation creates what we might call an “implementation illusion” – the false belief that because standards exist on paper, they exist in practice.
The Compliance vs. Commitment Problem
When standards are imposed without buy in, developers may technically comply while lacking genuine commitment. This creates a situation where code passes automated checks but doesn’t embrace the spirit of the standards.
Consider a team where indentation rules are strictly enforced by automated tools. Developers might ensure their code passes these checks while ignoring more important principles like clear naming conventions or proper error handling that aren’t as easily verified automatically.
The Missing “Why” Factor
Standards that explain what to do without explaining why often lead to inconsistent application. When developers don’t understand the reasoning behind a standard, they’re more likely to view it as arbitrary and less likely to apply it thoughtfully.
For example, a standard requiring comprehensive error handling might be inconsistently applied if developers don’t understand how it improves system reliability and user experience.
Signs Your Coding Standards Aren’t Working
How can you tell if your standards aren’t delivering? Look for these warning signs:
Inconsistent Code Quality
If code quality varies significantly between team members or projects despite having standards in place, your standards aren’t effectively guiding development practices.
Frequent Disagreements During Code Reviews
When code reviews regularly devolve into debates about how code should be structured or formatted, it suggests your standards aren’t clear enough or aren’t accepted by the team.
Standards Bypass
If your team frequently creates exceptions or bypasses standards to meet deadlines, it indicates the standards aren’t practical for your actual working conditions.
Declining Velocity Despite Standards
If team velocity decreases after implementing standards, it might indicate that the standards are too rigid, too complex, or not aligned with how your team actually works.
The Hidden Reasons Your Standards Aren’t Improving Output
Let’s explore the deeper, often overlooked reasons why coding standards fail to enhance team performance:
1. Standards Created in Isolation
When standards are created by architects or tech leads without input from the developers who will use them daily, they often miss practical considerations that affect implementation.
Standards developed collaboratively tend to be more practical and receive greater buy in from the team. Without this collaborative approach, standards can feel imposed rather than supportive.
2. Standards That Ignore Team Context
Every development team has unique constraints, strengths, and challenges. Standards that work well for a team of senior developers might be inappropriate for a team with varied experience levels.
For example, requiring advanced design patterns in a team where many developers are still learning fundamental concepts can create frustration and actually decrease code quality as developers struggle to implement patterns they don’t fully understand.
3. Overly Rigid or Comprehensive Standards
Standards that attempt to govern every aspect of coding can become overwhelming and impractical. When developers need to reference a 100 page document to write a simple function, the cognitive overhead reduces productivity rather than enhancing it.
Successful standards focus on the most important aspects of code quality for your specific context and leave room for developer discretion in less critical areas.
4. Lack of Continuous Evolution
Static standards quickly become outdated as technologies, frameworks, and best practices evolve. Standards that aren’t regularly reviewed and updated can actually hinder progress by enforcing outdated practices.
For instance, standards written for an older version of a programming language might not leverage new features that could significantly improve code quality and developer productivity.
5. Missing Enforcement Mechanisms
Standards without appropriate enforcement mechanisms rely entirely on developer discipline and memory. In the pressure of deadlines and complex problem solving, even the most diligent developers can forget to apply standards consistently.
Effective standards implementation usually requires a combination of automated enforcement (for aspects that can be automated) and cultural reinforcement (for aspects that cannot).
Transforming Standards into Productivity Boosters
Now that we understand why standards often fail to improve output, let’s explore practical strategies to transform your coding standards from paper documents into powerful productivity tools:
1. Develop Standards Collaboratively
Include representatives from all experience levels in your standards development process. This ensures standards are practical for everyone and creates natural advocates within the team.
Consider a workshop approach where the team collectively identifies the most important aspects of code quality for your specific context and agrees on standards that address these priorities.
Example collaborative approach:
- Conduct a workshop with the entire development team
- Identify pain points in the current codebase
- Collectively determine which standards would address these issues
- Draft standards together, with developers contributing examples
- Review and refine the standards as a team
2. Start Small and Expand Gradually
Rather than implementing comprehensive standards all at once, begin with a small set of high impact standards and gradually expand as these become second nature to the team.
For example, you might start with naming conventions and basic formatting rules, then add more sophisticated standards around error handling, testing requirements, or design patterns as the team adapts.
3. Explain the “Why” Behind Each Standard
For each standard, clearly explain:
- Why it matters
- What problems it solves
- How it benefits the team and product
This context helps developers understand when a standard is crucial to follow strictly and when there might be room for flexibility.
For example, instead of simply stating “Use dependency injection for all services,” explain how this practice improves testability, facilitates future changes, and prevents tightly coupled code that becomes difficult to maintain.
4. Automate What Can Be Automated
Use linters, code formatters, and static analysis tools to automatically enforce standards that can be codified. This reduces cognitive load on developers and ensures consistent application of basic standards.
Tools like ESLint for JavaScript, Checkstyle for Java, or Rubocop for Ruby can enforce many common standards without requiring manual verification.
Example setup for JavaScript projects:
// .eslintrc.js
module.exports = {
"extends": ["airbnb"],
"rules": {
"no-console": "error",
"max-len": ["error", { "code": 100 }],
"prefer-const": "error",
"no-unused-vars": "error"
}
};
5. Integrate Standards into Your Development Workflow
Standards should be integrated into everyday development processes rather than existing as separate considerations. This might include:
- Including standards verification in your CI/CD pipeline
- Adding standards compliance to your definition of “done”
- Creating pull request templates that prompt developers to verify standards compliance
- Incorporating standards review into your code review process
Example PR template that encourages standards compliance:
# Pull Request
## Description
[Describe your changes here]
## Standards Checklist
- [ ] Code follows naming conventions
- [ ] Functions have single responsibility
- [ ] Error handling follows team standards
- [ ] Unit tests cover new functionality
- [ ] Documentation updated
## Related Issues
[Link to related issues here]
6. Create Living Documentation with Examples
Abstract standards are difficult to apply consistently. Enhance your standards documentation with concrete examples of both compliant and non compliant code to illustrate each standard clearly.
Organize your documentation in a searchable, easily navigable format so developers can quickly find guidance when needed.
Example of documentation with clear examples:
// ❌ Avoid: Ambiguous function name
function process(data) {
// implementation
}
// ✅ Better: Clear, specific function name
function validateUserInput(data) {
// implementation
}
7. Foster a Culture of Quality
Standards work best in a culture that values code quality. Foster this culture by:
- Celebrating improvements in code quality
- Allocating time for refactoring and standards implementation
- Leading by example, with senior team members demonstrating commitment to standards
- Encouraging constructive feedback during code reviews
Remember that culture is shaped by what you celebrate and what you tolerate. If you praise speed at the expense of quality, standards will be seen as obstacles rather than assets.
8. Regularly Review and Evolve Standards
Schedule regular reviews of your standards to ensure they remain relevant and effective. During these reviews:
- Evaluate which standards are working well and which are causing friction
- Consider new technologies or practices that might warrant new standards
- Remove or modify standards that no longer serve your team’s needs
- Incorporate feedback from team members about their experience with the standards
This evolutionary approach ensures your standards remain a living, useful tool rather than becoming outdated constraints.
Case Study: Transforming Standards at a Growing Startup
Let’s examine how one company successfully transformed their approach to coding standards:
TechNova, a growing startup with 25 developers, had implemented comprehensive coding standards early in their development. However, as the team grew and pressure to deliver increased, they noticed their standards weren’t improving output – in fact, developers seemed increasingly frustrated by them.
The Problem
Upon investigation, they discovered several issues:
- The standards had been created by the original CTO without input from the growing team
- The documentation was extensive but lacked practical examples
- Many standards couldn’t be automatically verified, creating inconsistent application
- New developers found the standards overwhelming and unclear
The Solution
TechNova took the following steps to transform their standards:
- Collaborative Revision: They formed a standards committee with representatives from all levels of experience to revise the standards.
- Prioritization: They identified the 20% of standards that addressed 80% of their quality issues and focused on these first.
- Automation: They implemented linters and code formatters to automatically enforce formatting standards.
- Documentation Overhaul: They rewrote the standards documentation with clear examples, rationales, and a searchable format.
- Integration: They integrated standards verification into their CI/CD pipeline and pull request process.
- Training: They conducted workshops to help developers understand and apply the standards effectively.
The Results
Six months after implementing these changes, TechNova saw significant improvements:
- 50% reduction in bugs related to code structure and organization
- 40% faster onboarding for new developers
- 30% reduction in time spent on code reviews
- Improved developer satisfaction and reduced friction around standards
The key insight: Standards became a tool that empowered developers rather than a burden that constrained them.
Common Pitfalls to Avoid
As you work to improve your coding standards implementation, watch out for these common pitfalls:
1. The Perfectionism Trap
Seeking perfect standards that cover every scenario can lead to analysis paralysis or overly complex guidelines. Focus on practical standards that address your most significant issues rather than theoretical perfection.
2. Confusing Preferences with Principles
Not all coding practices are equally important. Distinguish between fundamental principles that significantly impact code quality (like error handling strategies) and preferences that are more subjective (like whether to use tabs or spaces for indentation).
Apply rigid standards to principles while allowing more flexibility for preferences.
3. Neglecting the Human Factor
Standards implementation is as much about people as it is about technology. If you focus solely on the technical aspects without considering team dynamics, learning curves, and individual working styles, your standards are unlikely to succeed.
4. Failing to Lead by Example
If senior team members or project leaders don’t follow the standards, other developers will quickly conclude that the standards aren’t actually important. Leadership commitment to standards is essential for team wide adoption.
5. Overreliance on Tools
While automation is valuable, not all aspects of code quality can be verified by tools. Overreliance on automated checks can create a false sense of security while missing important qualitative aspects of good code.
Measuring the Impact of Improved Standards
How do you know if your improved approach to standards is actually working? Consider tracking these metrics:
1. Defect Density
Track the number of bugs per thousand lines of code before and after improving your standards implementation. Effective standards should reduce defect density over time.
2. Code Review Efficiency
Measure the time spent on code reviews and the nature of review comments. As standards become more effective, reviews should focus less on basic issues and more on architectural and functional concerns.
3. Onboarding Time
Track how quickly new team members become productive. Clear, well implemented standards should reduce the time needed for new developers to understand and contribute to the codebase.
4. Developer Satisfaction
Regularly survey your team about their experience with the standards. Are they helpful? Burdensome? Clear? Confusing? This qualitative feedback is invaluable for continuous improvement.
5. Technical Debt Accumulation
Monitor the rate at which technical debt accumulates in your codebase. Effective standards should slow the accumulation of technical debt and may even help reduce existing debt.
Tailoring Standards to Your Team’s Maturity
Different teams require different approaches to standards based on their maturity level:
Beginner Teams
Teams with many junior developers or those new to a technology stack benefit from:
- More prescriptive standards with detailed explanations
- Extensive examples of correct implementations
- Regular training sessions on standards application
- Automated enforcement where possible
- Frequent, supportive code reviews
Intermediate Teams
Teams with a mix of experience levels work well with:
- A balance of prescriptive rules and guiding principles
- Peer mentoring to share standards knowledge
- Regular standards discussions during retrospectives
- Rotation of standards “champions” to build ownership
Advanced Teams
Highly experienced teams typically benefit from:
- Higher level principles rather than detailed prescriptions
- Focus on architectural standards over syntactic details
- Team ownership of standards evolution
- Regular reflection on standards effectiveness
Conclusion: From Standards to Standard of Excellence
Coding standards alone don’t improve team output – it’s how they’re created, implemented, and evolved that makes the difference. When standards are collaborative, practical, automated where possible, and integrated into daily workflows, they transform from bureaucratic hurdles into powerful tools for quality and productivity.
The most successful teams don’t just have standards; they have a living standards practice that grows with the team and adapts to changing needs. They use standards not as rigid rules but as enabling constraints that channel creativity and expertise toward consistently excellent outcomes.
If your coding standards aren’t improving team output, don’t abandon them – transform them. With the strategies outlined in this article, you can evolve your standards from documentation that’s referenced occasionally into a foundation for a genuine culture of quality.
Remember that the ultimate goal isn’t compliance with standards; it’s creating a team environment where excellent code is the natural outcome of your development process. When standards support this goal rather than standing in its way, you’ll see the improvements in team output you’ve been looking for.
FAQ: Coding Standards Transformation
How long does it typically take to see results from improved coding standards?
Most teams begin to see measurable improvements within 2-3 months of implementing a more effective standards approach. However, the full benefits, particularly in areas like reduced technical debt and improved system stability, may take 6-12 months to fully materialize.
Should we create our own standards from scratch or adopt industry standards?
A hybrid approach often works best. Start with established industry standards for your technology stack, then adapt them to your specific context and needs. This gives you the benefit of best practices while ensuring the standards are practical for your team.
How do we handle disagreements about standards within the team?
Create a clear process for standards evolution that includes a way to propose changes, discuss them, and make decisions. Some teams use a voting system for more subjective standards, while others designate a standards committee to make final decisions after gathering input.
What’s the right balance between automated and manual standards enforcement?
Automate everything that can be objectively verified: formatting, basic syntax rules, naming patterns, etc. Reserve manual review for higher level concerns like architecture, design patterns, and code organization that require human judgment.
How do we maintain standards while meeting tight deadlines?
Integrate standards into your definition of “done” rather than treating them as optional extras. When standards are part of the development process rather than additional work, they’re less likely to be sacrificed under pressure. Additionally, automated enforcement helps maintain basic standards even during crunch periods.